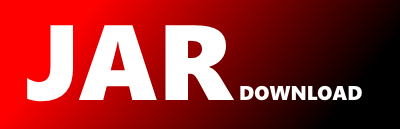
su.nlq.prometheus.jmx.MBeansCollector Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of jmx-prometheus-collector Show documentation
Show all versions of jmx-prometheus-collector Show documentation
Pipe for JMX MBeans to Prometheus
package su.nlq.prometheus.jmx;
import org.jetbrains.annotations.NotNull;
import org.jetbrains.annotations.Nullable;
import su.nlq.prometheus.jmx.logging.Logger;
import javax.management.*;
import java.io.IOException;
import java.util.*;
import java.util.function.Predicate;
import java.util.stream.Collectors;
public final class MBeansCollector {
private final @NotNull Collection names;
private final @NotNull Predicate predicate;
public MBeansCollector(@NotNull List names, @NotNull List exclude) {
this.names = getObjectNames(names);
this.predicate = new BlacklistQuery(getObjectNames(exclude));
}
public @NotNull Collection collect(@NotNull MBeanServerConnection connection) {
return names.isEmpty()
? query(connection, null)
: names.stream().flatMap(name -> query(connection, name).stream()).collect(Collectors.toSet());
}
private static @NotNull Collection getObjectNames(@NotNull Iterable names) {
final Collection result = new ArrayList<>();
names.forEach(name -> {
try {
result.add(new ObjectName(name));
} catch (MalformedObjectNameException e) {
Logger.instance.debug("Failed to construct " + name, e);
}
});
return result;
}
private @NotNull Collection query(@NotNull MBeanServerConnection connection, @Nullable ObjectName name) {
try {
return connection.queryMBeans(name, null).stream().filter(predicate).collect(Collectors.toSet());
} catch (IOException e) {
Logger.instance.debug("Failed to query beans of '" + name + '\'', e);
return Collections.emptySet();
}
}
private static final class BlacklistQuery implements Predicate {
private final @NotNull Collection blacklist;
public BlacklistQuery(@NotNull Collection blacklist) {
this.blacklist = blacklist;
}
@Override
public boolean test(@NotNull ObjectInstance object) {
return !blacklist.contains(object.getObjectName());
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy