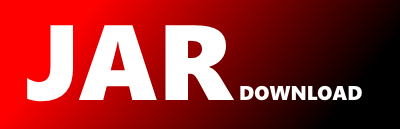
tech.aroma.data.memory.MemoryFollowerRepository Maven / Gradle / Ivy
/*
* Copyright 2017 RedRoma, Inc.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package tech.aroma.data.memory;
import java.util.List;
import java.util.Map;
import java.util.stream.Collectors;
import com.google.common.base.Objects;
import org.apache.thrift.TException;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import sir.wellington.alchemy.collections.lists.Lists;
import sir.wellington.alchemy.collections.maps.Maps;
import tech.aroma.data.FollowerRepository;
import tech.aroma.thrift.Application;
import tech.aroma.thrift.User;
import tech.aroma.thrift.exceptions.InvalidArgumentException;
import static tech.aroma.data.assertions.RequestAssertions.validApplication;
import static tech.aroma.data.assertions.RequestAssertions.validUser;
import static tech.sirwellington.alchemy.arguments.Arguments.*;
import static tech.sirwellington.alchemy.arguments.assertions.StringAssertions.*;
/**
*
* @author SirWellington
*/
final class MemoryFollowerRepository implements FollowerRepository
{
private final static Logger LOG = LoggerFactory.getLogger(MemoryFollowerRepository.class);
private final Map> userFollowings = Maps.createSynchronized();
private final Map> applicationFollowers = Maps.createSynchronized();
@Override
public void saveFollowing(User user, Application application) throws TException
{
checkThat(user)
.throwing(InvalidArgumentException.class)
.is(validUser());
checkThat(application)
.throwing(InvalidArgumentException.class)
.is(validApplication());
String userId = user.userId;
String appId = application.applicationId;
List applications = userFollowings.getOrDefault(userId, Lists.create());
applications.add(application);
userFollowings.put(userId, applications);
List followers = applicationFollowers.getOrDefault(appId, Lists.create());
followers.add(user);
applicationFollowers.put(appId, followers);
}
@Override
public void deleteFollowing(String userId, String applicationId) throws TException
{
checkThat(userId, applicationId)
.throwing(InvalidArgumentException.class)
.are(nonEmptyString());
List apps = userFollowings.getOrDefault(userId, Lists.emptyList());
apps = apps.stream()
.filter(app -> !Objects.equal(applicationId, app.applicationId))
.collect(Collectors.toList());
userFollowings.put(userId, apps);
List followers = applicationFollowers.getOrDefault(applicationId, Lists.emptyList());
followers = followers.stream()
.filter(user -> !Objects.equal(userId, user.userId))
.collect(Collectors.toList());
applicationFollowers.put(applicationId, followers);
}
@Override
public boolean followingExists(String userId, String applicationId) throws TException
{
checkThat(userId, applicationId)
.throwing(InvalidArgumentException.class)
.usingMessage("missing arguments")
.are(nonEmptyString());
return userFollowsApp(userId, applicationId) &&
appHasFollower(applicationId, userId);
}
@Override
public List getApplicationsFollowedBy(String userId) throws TException
{
checkThat(userId)
.throwing(InvalidArgumentException.class)
.usingMessage("missing userId")
.is(nonEmptyString());
return userFollowings.getOrDefault(userId, Lists.emptyList());
}
@Override
public List getApplicationFollowers(String applicationId) throws TException
{
return applicationFollowers.getOrDefault(applicationId, Lists.emptyList());
}
private boolean userFollowsApp(String userId, String applicationId)
{
List apps = userFollowings.get(userId);
if(Lists.isEmpty(apps))
{
return false;
}
return apps.stream()
.anyMatch(app -> Objects.equal(applicationId, app.applicationId));
}
private boolean appHasFollower(String applicationId, String userId)
{
List followers = applicationFollowers.get(applicationId);
if(Lists.isEmpty(followers))
{
return false;
}
return followers.stream()
.anyMatch(user -> Objects.equal(userId, user.userId));
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy