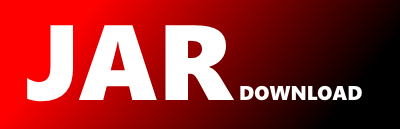
tech.aroma.service.operations.GetFullMessageOperation Maven / Gradle / Ivy
/*
* Copyright 2016 RedRoma.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package tech.aroma.service.operations;
import javax.inject.Inject;
import org.apache.thrift.TException;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import tech.aroma.data.MessageRepository;
import tech.aroma.thrift.Message;
import tech.aroma.thrift.exceptions.InvalidArgumentException;
import tech.aroma.thrift.service.GetFullMessageRequest;
import tech.aroma.thrift.service.GetFullMessageResponse;
import tech.sirwellington.alchemy.arguments.AlchemyAssertion;
import tech.sirwellington.alchemy.thrift.operations.ThriftOperation;
import static tech.aroma.data.assertions.RequestAssertions.validApplicationId;
import static tech.aroma.data.assertions.RequestAssertions.validMessageId;
import static tech.sirwellington.alchemy.arguments.Arguments.checkThat;
import static tech.sirwellington.alchemy.arguments.assertions.Assertions.notNull;
/**
*
* @author SirWellington
*/
final class GetFullMessageOperation implements ThriftOperation
{
private final static Logger LOG = LoggerFactory.getLogger(GetFullMessageOperation.class);
private final MessageRepository messageRepo;
@Inject
GetFullMessageOperation(MessageRepository messageRepo)
{
checkThat(messageRepo).is(notNull());
this.messageRepo = messageRepo;
}
@Override
public GetFullMessageResponse process(GetFullMessageRequest request) throws TException
{
checkThat(request)
.throwing(ex -> new InvalidArgumentException(ex.getMessage()))
.is(good());
String appId = request.applicationId;
String messageId = request.messageId;
Message message = messageRepo.getMessage(appId, messageId);
return new GetFullMessageResponse(message);
}
private AlchemyAssertion good()
{
return request ->
{
checkThat(request)
.usingMessage("missing request")
.is(notNull());
checkThat(request.messageId)
.is(validMessageId());
checkThat(request.applicationId)
.is(validApplicationId());
};
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy