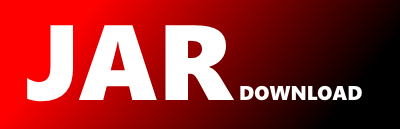
tech.aroma.thrift.LengthOfTime Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aroma-thrift Show documentation
Show all versions of aroma-thrift Show documentation
Part of the Aroma Project.
This project contains the Service and Model Definitions.
From this the Server and Client interfaces are generated for the Aroma Service.
/**
* Autogenerated by Thrift Compiler (0.9.3)
*
* DO NOT EDIT UNLESS YOU ARE SURE THAT YOU KNOW WHAT YOU ARE DOING
* @generated
*/
package tech.aroma.thrift;
import org.apache.thrift.scheme.IScheme;
import org.apache.thrift.scheme.SchemeFactory;
import org.apache.thrift.scheme.StandardScheme;
import org.apache.thrift.scheme.TupleScheme;
import org.apache.thrift.protocol.TTupleProtocol;
import org.apache.thrift.protocol.TProtocolException;
import org.apache.thrift.EncodingUtils;
import org.apache.thrift.TException;
import org.apache.thrift.async.AsyncMethodCallback;
import org.apache.thrift.server.AbstractNonblockingServer.*;
import java.util.List;
import java.util.ArrayList;
import java.util.Map;
import java.util.HashMap;
import java.util.EnumMap;
import java.util.Set;
import java.util.HashSet;
import java.util.EnumSet;
import java.util.Collections;
import java.util.BitSet;
import java.nio.ByteBuffer;
import java.util.Arrays;
import javax.annotation.Generated;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
@SuppressWarnings({"cast", "rawtypes", "serial", "unchecked"})
/**
* Defines a Period of Time, or a Length of Time.
*/
@Generated(value = "Autogenerated by Thrift Compiler (0.9.3)", date = "2016-05-10")
public class LengthOfTime implements org.apache.thrift.TBase, java.io.Serializable, Cloneable, Comparable {
private static final org.apache.thrift.protocol.TStruct STRUCT_DESC = new org.apache.thrift.protocol.TStruct("LengthOfTime");
private static final org.apache.thrift.protocol.TField UNIT_FIELD_DESC = new org.apache.thrift.protocol.TField("unit", org.apache.thrift.protocol.TType.I32, (short)1);
private static final org.apache.thrift.protocol.TField VALUE_FIELD_DESC = new org.apache.thrift.protocol.TField("value", org.apache.thrift.protocol.TType.I64, (short)2);
private static final Map, SchemeFactory> schemes = new HashMap, SchemeFactory>();
static {
schemes.put(StandardScheme.class, new LengthOfTimeStandardSchemeFactory());
schemes.put(TupleScheme.class, new LengthOfTimeTupleSchemeFactory());
}
/**
*
* @see TimeUnit
*/
public TimeUnit unit; // required
/**
* The Value must be non-negative
*/
public long value; // required
/** The set of fields this struct contains, along with convenience methods for finding and manipulating them. */
public enum _Fields implements org.apache.thrift.TFieldIdEnum {
/**
*
* @see TimeUnit
*/
UNIT((short)1, "unit"),
/**
* The Value must be non-negative
*/
VALUE((short)2, "value");
private static final Map byName = new HashMap();
static {
for (_Fields field : EnumSet.allOf(_Fields.class)) {
byName.put(field.getFieldName(), field);
}
}
/**
* Find the _Fields constant that matches fieldId, or null if its not found.
*/
public static _Fields findByThriftId(int fieldId) {
switch(fieldId) {
case 1: // UNIT
return UNIT;
case 2: // VALUE
return VALUE;
default:
return null;
}
}
/**
* Find the _Fields constant that matches fieldId, throwing an exception
* if it is not found.
*/
public static _Fields findByThriftIdOrThrow(int fieldId) {
_Fields fields = findByThriftId(fieldId);
if (fields == null) throw new IllegalArgumentException("Field " + fieldId + " doesn't exist!");
return fields;
}
/**
* Find the _Fields constant that matches name, or null if its not found.
*/
public static _Fields findByName(String name) {
return byName.get(name);
}
private final short _thriftId;
private final String _fieldName;
_Fields(short thriftId, String fieldName) {
_thriftId = thriftId;
_fieldName = fieldName;
}
public short getThriftFieldId() {
return _thriftId;
}
public String getFieldName() {
return _fieldName;
}
}
// isset id assignments
private static final int __VALUE_ISSET_ID = 0;
private byte __isset_bitfield = 0;
public static final Map<_Fields, org.apache.thrift.meta_data.FieldMetaData> metaDataMap;
static {
Map<_Fields, org.apache.thrift.meta_data.FieldMetaData> tmpMap = new EnumMap<_Fields, org.apache.thrift.meta_data.FieldMetaData>(_Fields.class);
tmpMap.put(_Fields.UNIT, new org.apache.thrift.meta_data.FieldMetaData("unit", org.apache.thrift.TFieldRequirementType.REQUIRED,
new org.apache.thrift.meta_data.EnumMetaData(org.apache.thrift.protocol.TType.ENUM, TimeUnit.class)));
tmpMap.put(_Fields.VALUE, new org.apache.thrift.meta_data.FieldMetaData("value", org.apache.thrift.TFieldRequirementType.REQUIRED,
new org.apache.thrift.meta_data.FieldValueMetaData(org.apache.thrift.protocol.TType.I64 , "long")));
metaDataMap = Collections.unmodifiableMap(tmpMap);
org.apache.thrift.meta_data.FieldMetaData.addStructMetaDataMap(LengthOfTime.class, metaDataMap);
}
public LengthOfTime() {
}
public LengthOfTime(
TimeUnit unit,
long value)
{
this();
this.unit = unit;
this.value = value;
setValueIsSet(true);
}
/**
* Performs a deep copy on other.
*/
public LengthOfTime(LengthOfTime other) {
__isset_bitfield = other.__isset_bitfield;
if (other.isSetUnit()) {
this.unit = other.unit;
}
this.value = other.value;
}
public LengthOfTime deepCopy() {
return new LengthOfTime(this);
}
@Override
public void clear() {
this.unit = null;
setValueIsSet(false);
this.value = 0;
}
/**
*
* @see TimeUnit
*/
public TimeUnit getUnit() {
return this.unit;
}
/**
*
* @see TimeUnit
*/
public LengthOfTime setUnit(TimeUnit unit) {
this.unit = unit;
return this;
}
public void unsetUnit() {
this.unit = null;
}
/** Returns true if field unit is set (has been assigned a value) and false otherwise */
public boolean isSetUnit() {
return this.unit != null;
}
public void setUnitIsSet(boolean value) {
if (!value) {
this.unit = null;
}
}
/**
* The Value must be non-negative
*/
public long getValue() {
return this.value;
}
/**
* The Value must be non-negative
*/
public LengthOfTime setValue(long value) {
this.value = value;
setValueIsSet(true);
return this;
}
public void unsetValue() {
__isset_bitfield = EncodingUtils.clearBit(__isset_bitfield, __VALUE_ISSET_ID);
}
/** Returns true if field value is set (has been assigned a value) and false otherwise */
public boolean isSetValue() {
return EncodingUtils.testBit(__isset_bitfield, __VALUE_ISSET_ID);
}
public void setValueIsSet(boolean value) {
__isset_bitfield = EncodingUtils.setBit(__isset_bitfield, __VALUE_ISSET_ID, value);
}
public void setFieldValue(_Fields field, Object value) {
switch (field) {
case UNIT:
if (value == null) {
unsetUnit();
} else {
setUnit((TimeUnit)value);
}
break;
case VALUE:
if (value == null) {
unsetValue();
} else {
setValue((Long)value);
}
break;
}
}
public Object getFieldValue(_Fields field) {
switch (field) {
case UNIT:
return getUnit();
case VALUE:
return getValue();
}
throw new IllegalStateException();
}
/** Returns true if field corresponding to fieldID is set (has been assigned a value) and false otherwise */
public boolean isSet(_Fields field) {
if (field == null) {
throw new IllegalArgumentException();
}
switch (field) {
case UNIT:
return isSetUnit();
case VALUE:
return isSetValue();
}
throw new IllegalStateException();
}
@Override
public boolean equals(Object that) {
if (that == null)
return false;
if (that instanceof LengthOfTime)
return this.equals((LengthOfTime)that);
return false;
}
public boolean equals(LengthOfTime that) {
if (that == null)
return false;
boolean this_present_unit = true && this.isSetUnit();
boolean that_present_unit = true && that.isSetUnit();
if (this_present_unit || that_present_unit) {
if (!(this_present_unit && that_present_unit))
return false;
if (!this.unit.equals(that.unit))
return false;
}
boolean this_present_value = true;
boolean that_present_value = true;
if (this_present_value || that_present_value) {
if (!(this_present_value && that_present_value))
return false;
if (this.value != that.value)
return false;
}
return true;
}
@Override
public int hashCode() {
List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy