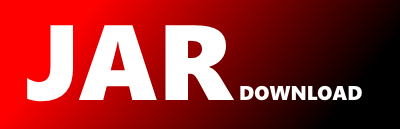
tech.aroma.thrift.Message Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aroma-thrift Show documentation
Show all versions of aroma-thrift Show documentation
Part of the Aroma Project.
This project contains the Service and Model Definitions.
From this the Server and Client interfaces are generated for the Aroma Service.
/**
* Autogenerated by Thrift Compiler (0.9.3)
*
* DO NOT EDIT UNLESS YOU ARE SURE THAT YOU KNOW WHAT YOU ARE DOING
* @generated
*/
package tech.aroma.thrift;
import org.apache.thrift.scheme.IScheme;
import org.apache.thrift.scheme.SchemeFactory;
import org.apache.thrift.scheme.StandardScheme;
import org.apache.thrift.scheme.TupleScheme;
import org.apache.thrift.protocol.TTupleProtocol;
import org.apache.thrift.protocol.TProtocolException;
import org.apache.thrift.EncodingUtils;
import org.apache.thrift.TException;
import org.apache.thrift.async.AsyncMethodCallback;
import org.apache.thrift.server.AbstractNonblockingServer.*;
import java.util.List;
import java.util.ArrayList;
import java.util.Map;
import java.util.HashMap;
import java.util.EnumMap;
import java.util.Set;
import java.util.HashSet;
import java.util.EnumSet;
import java.util.Collections;
import java.util.BitSet;
import java.nio.ByteBuffer;
import java.util.Arrays;
import javax.annotation.Generated;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
@SuppressWarnings({"cast", "rawtypes", "serial", "unchecked"})
@Generated(value = "Autogenerated by Thrift Compiler (0.9.3)", date = "2016-05-10")
public class Message implements org.apache.thrift.TBase, java.io.Serializable, Cloneable, Comparable {
private static final org.apache.thrift.protocol.TStruct STRUCT_DESC = new org.apache.thrift.protocol.TStruct("Message");
private static final org.apache.thrift.protocol.TField MESSAGE_ID_FIELD_DESC = new org.apache.thrift.protocol.TField("messageId", org.apache.thrift.protocol.TType.STRING, (short)1);
private static final org.apache.thrift.protocol.TField BODY_FIELD_DESC = new org.apache.thrift.protocol.TField("body", org.apache.thrift.protocol.TType.STRING, (short)2);
private static final org.apache.thrift.protocol.TField URGENCY_FIELD_DESC = new org.apache.thrift.protocol.TField("urgency", org.apache.thrift.protocol.TType.I32, (short)3);
private static final org.apache.thrift.protocol.TField TIME_OF_CREATION_FIELD_DESC = new org.apache.thrift.protocol.TField("timeOfCreation", org.apache.thrift.protocol.TType.I64, (short)4);
private static final org.apache.thrift.protocol.TField TIME_MESSAGE_RECEIVED_FIELD_DESC = new org.apache.thrift.protocol.TField("timeMessageReceived", org.apache.thrift.protocol.TType.I64, (short)5);
private static final org.apache.thrift.protocol.TField APPLICATION_NAME_FIELD_DESC = new org.apache.thrift.protocol.TField("applicationName", org.apache.thrift.protocol.TType.STRING, (short)6);
private static final org.apache.thrift.protocol.TField HOSTNAME_FIELD_DESC = new org.apache.thrift.protocol.TField("hostname", org.apache.thrift.protocol.TType.STRING, (short)7);
private static final org.apache.thrift.protocol.TField MAC_ADDRESS_FIELD_DESC = new org.apache.thrift.protocol.TField("macAddress", org.apache.thrift.protocol.TType.STRING, (short)8);
private static final org.apache.thrift.protocol.TField IS_TRUNCATED_FIELD_DESC = new org.apache.thrift.protocol.TField("isTruncated", org.apache.thrift.protocol.TType.BOOL, (short)9);
private static final org.apache.thrift.protocol.TField TITLE_FIELD_DESC = new org.apache.thrift.protocol.TField("title", org.apache.thrift.protocol.TType.STRING, (short)10);
private static final org.apache.thrift.protocol.TField APPLICATION_ID_FIELD_DESC = new org.apache.thrift.protocol.TField("applicationId", org.apache.thrift.protocol.TType.STRING, (short)11);
private static final org.apache.thrift.protocol.TField DEVICE_NAME_FIELD_DESC = new org.apache.thrift.protocol.TField("deviceName", org.apache.thrift.protocol.TType.STRING, (short)12);
private static final Map, SchemeFactory> schemes = new HashMap, SchemeFactory>();
static {
schemes.put(StandardScheme.class, new MessageStandardSchemeFactory());
schemes.put(TupleScheme.class, new MessageTupleSchemeFactory());
}
/**
* Each message has a unique ID
*/
public String messageId; // required
/**
* The body represents the Message's Payload, i.e. the actual message.
* If the Message Body is too long, it may be truncated. The complete
* body can then be loaded in a separate request.
*/
public String body; // optional
/**
*
* @see Urgency
*/
public Urgency urgency; // required
/**
* The time the message was created on the Application side
*/
public long timeOfCreation; // required
/**
* The time the message was received on the Server side
*/
public long timeMessageReceived; // required
/**
* Name of the Application that sent the message.
*/
public String applicationName; // required
/**
* The Network Hostname of the Device sending the message, ideally the FQDM.
*/
public String hostname; // optional
public String macAddress; // optional
public boolean isTruncated; // optional
public String title; // required
/**
* The ID of the Application the Message was sent from.
*/
public String applicationId; // required
public String deviceName; // optional
/** The set of fields this struct contains, along with convenience methods for finding and manipulating them. */
public enum _Fields implements org.apache.thrift.TFieldIdEnum {
/**
* Each message has a unique ID
*/
MESSAGE_ID((short)1, "messageId"),
/**
* The body represents the Message's Payload, i.e. the actual message.
* If the Message Body is too long, it may be truncated. The complete
* body can then be loaded in a separate request.
*/
BODY((short)2, "body"),
/**
*
* @see Urgency
*/
URGENCY((short)3, "urgency"),
/**
* The time the message was created on the Application side
*/
TIME_OF_CREATION((short)4, "timeOfCreation"),
/**
* The time the message was received on the Server side
*/
TIME_MESSAGE_RECEIVED((short)5, "timeMessageReceived"),
/**
* Name of the Application that sent the message.
*/
APPLICATION_NAME((short)6, "applicationName"),
/**
* The Network Hostname of the Device sending the message, ideally the FQDM.
*/
HOSTNAME((short)7, "hostname"),
MAC_ADDRESS((short)8, "macAddress"),
IS_TRUNCATED((short)9, "isTruncated"),
TITLE((short)10, "title"),
/**
* The ID of the Application the Message was sent from.
*/
APPLICATION_ID((short)11, "applicationId"),
DEVICE_NAME((short)12, "deviceName");
private static final Map byName = new HashMap();
static {
for (_Fields field : EnumSet.allOf(_Fields.class)) {
byName.put(field.getFieldName(), field);
}
}
/**
* Find the _Fields constant that matches fieldId, or null if its not found.
*/
public static _Fields findByThriftId(int fieldId) {
switch(fieldId) {
case 1: // MESSAGE_ID
return MESSAGE_ID;
case 2: // BODY
return BODY;
case 3: // URGENCY
return URGENCY;
case 4: // TIME_OF_CREATION
return TIME_OF_CREATION;
case 5: // TIME_MESSAGE_RECEIVED
return TIME_MESSAGE_RECEIVED;
case 6: // APPLICATION_NAME
return APPLICATION_NAME;
case 7: // HOSTNAME
return HOSTNAME;
case 8: // MAC_ADDRESS
return MAC_ADDRESS;
case 9: // IS_TRUNCATED
return IS_TRUNCATED;
case 10: // TITLE
return TITLE;
case 11: // APPLICATION_ID
return APPLICATION_ID;
case 12: // DEVICE_NAME
return DEVICE_NAME;
default:
return null;
}
}
/**
* Find the _Fields constant that matches fieldId, throwing an exception
* if it is not found.
*/
public static _Fields findByThriftIdOrThrow(int fieldId) {
_Fields fields = findByThriftId(fieldId);
if (fields == null) throw new IllegalArgumentException("Field " + fieldId + " doesn't exist!");
return fields;
}
/**
* Find the _Fields constant that matches name, or null if its not found.
*/
public static _Fields findByName(String name) {
return byName.get(name);
}
private final short _thriftId;
private final String _fieldName;
_Fields(short thriftId, String fieldName) {
_thriftId = thriftId;
_fieldName = fieldName;
}
public short getThriftFieldId() {
return _thriftId;
}
public String getFieldName() {
return _fieldName;
}
}
// isset id assignments
private static final int __TIMEOFCREATION_ISSET_ID = 0;
private static final int __TIMEMESSAGERECEIVED_ISSET_ID = 1;
private static final int __ISTRUNCATED_ISSET_ID = 2;
private byte __isset_bitfield = 0;
private static final _Fields optionals[] = {_Fields.BODY,_Fields.HOSTNAME,_Fields.MAC_ADDRESS,_Fields.IS_TRUNCATED,_Fields.DEVICE_NAME};
public static final Map<_Fields, org.apache.thrift.meta_data.FieldMetaData> metaDataMap;
static {
Map<_Fields, org.apache.thrift.meta_data.FieldMetaData> tmpMap = new EnumMap<_Fields, org.apache.thrift.meta_data.FieldMetaData>(_Fields.class);
tmpMap.put(_Fields.MESSAGE_ID, new org.apache.thrift.meta_data.FieldMetaData("messageId", org.apache.thrift.TFieldRequirementType.DEFAULT,
new org.apache.thrift.meta_data.FieldValueMetaData(org.apache.thrift.protocol.TType.STRING , "uuid")));
tmpMap.put(_Fields.BODY, new org.apache.thrift.meta_data.FieldMetaData("body", org.apache.thrift.TFieldRequirementType.OPTIONAL,
new org.apache.thrift.meta_data.FieldValueMetaData(org.apache.thrift.protocol.TType.STRING)));
tmpMap.put(_Fields.URGENCY, new org.apache.thrift.meta_data.FieldMetaData("urgency", org.apache.thrift.TFieldRequirementType.DEFAULT,
new org.apache.thrift.meta_data.EnumMetaData(org.apache.thrift.protocol.TType.ENUM, Urgency.class)));
tmpMap.put(_Fields.TIME_OF_CREATION, new org.apache.thrift.meta_data.FieldMetaData("timeOfCreation", org.apache.thrift.TFieldRequirementType.DEFAULT,
new org.apache.thrift.meta_data.FieldValueMetaData(org.apache.thrift.protocol.TType.I64 , "timestamp")));
tmpMap.put(_Fields.TIME_MESSAGE_RECEIVED, new org.apache.thrift.meta_data.FieldMetaData("timeMessageReceived", org.apache.thrift.TFieldRequirementType.DEFAULT,
new org.apache.thrift.meta_data.FieldValueMetaData(org.apache.thrift.protocol.TType.I64 , "timestamp")));
tmpMap.put(_Fields.APPLICATION_NAME, new org.apache.thrift.meta_data.FieldMetaData("applicationName", org.apache.thrift.TFieldRequirementType.DEFAULT,
new org.apache.thrift.meta_data.FieldValueMetaData(org.apache.thrift.protocol.TType.STRING)));
tmpMap.put(_Fields.HOSTNAME, new org.apache.thrift.meta_data.FieldMetaData("hostname", org.apache.thrift.TFieldRequirementType.OPTIONAL,
new org.apache.thrift.meta_data.FieldValueMetaData(org.apache.thrift.protocol.TType.STRING)));
tmpMap.put(_Fields.MAC_ADDRESS, new org.apache.thrift.meta_data.FieldMetaData("macAddress", org.apache.thrift.TFieldRequirementType.OPTIONAL,
new org.apache.thrift.meta_data.FieldValueMetaData(org.apache.thrift.protocol.TType.STRING)));
tmpMap.put(_Fields.IS_TRUNCATED, new org.apache.thrift.meta_data.FieldMetaData("isTruncated", org.apache.thrift.TFieldRequirementType.OPTIONAL,
new org.apache.thrift.meta_data.FieldValueMetaData(org.apache.thrift.protocol.TType.BOOL)));
tmpMap.put(_Fields.TITLE, new org.apache.thrift.meta_data.FieldMetaData("title", org.apache.thrift.TFieldRequirementType.DEFAULT,
new org.apache.thrift.meta_data.FieldValueMetaData(org.apache.thrift.protocol.TType.STRING)));
tmpMap.put(_Fields.APPLICATION_ID, new org.apache.thrift.meta_data.FieldMetaData("applicationId", org.apache.thrift.TFieldRequirementType.DEFAULT,
new org.apache.thrift.meta_data.FieldValueMetaData(org.apache.thrift.protocol.TType.STRING , "uuid")));
tmpMap.put(_Fields.DEVICE_NAME, new org.apache.thrift.meta_data.FieldMetaData("deviceName", org.apache.thrift.TFieldRequirementType.OPTIONAL,
new org.apache.thrift.meta_data.FieldValueMetaData(org.apache.thrift.protocol.TType.STRING)));
metaDataMap = Collections.unmodifiableMap(tmpMap);
org.apache.thrift.meta_data.FieldMetaData.addStructMetaDataMap(Message.class, metaDataMap);
}
public Message() {
this.urgency = tech.aroma.thrift.Urgency.LOW;
this.isTruncated = false;
}
public Message(
String messageId,
Urgency urgency,
long timeOfCreation,
long timeMessageReceived,
String applicationName,
String title,
String applicationId)
{
this();
this.messageId = messageId;
this.urgency = urgency;
this.timeOfCreation = timeOfCreation;
setTimeOfCreationIsSet(true);
this.timeMessageReceived = timeMessageReceived;
setTimeMessageReceivedIsSet(true);
this.applicationName = applicationName;
this.title = title;
this.applicationId = applicationId;
}
/**
* Performs a deep copy on other.
*/
public Message(Message other) {
__isset_bitfield = other.__isset_bitfield;
if (other.isSetMessageId()) {
this.messageId = other.messageId;
}
if (other.isSetBody()) {
this.body = other.body;
}
if (other.isSetUrgency()) {
this.urgency = other.urgency;
}
this.timeOfCreation = other.timeOfCreation;
this.timeMessageReceived = other.timeMessageReceived;
if (other.isSetApplicationName()) {
this.applicationName = other.applicationName;
}
if (other.isSetHostname()) {
this.hostname = other.hostname;
}
if (other.isSetMacAddress()) {
this.macAddress = other.macAddress;
}
this.isTruncated = other.isTruncated;
if (other.isSetTitle()) {
this.title = other.title;
}
if (other.isSetApplicationId()) {
this.applicationId = other.applicationId;
}
if (other.isSetDeviceName()) {
this.deviceName = other.deviceName;
}
}
public Message deepCopy() {
return new Message(this);
}
@Override
public void clear() {
this.messageId = null;
this.body = null;
this.urgency = tech.aroma.thrift.Urgency.LOW;
setTimeOfCreationIsSet(false);
this.timeOfCreation = 0;
setTimeMessageReceivedIsSet(false);
this.timeMessageReceived = 0;
this.applicationName = null;
this.hostname = null;
this.macAddress = null;
this.isTruncated = false;
this.title = null;
this.applicationId = null;
this.deviceName = null;
}
/**
* Each message has a unique ID
*/
public String getMessageId() {
return this.messageId;
}
/**
* Each message has a unique ID
*/
public Message setMessageId(String messageId) {
this.messageId = messageId;
return this;
}
public void unsetMessageId() {
this.messageId = null;
}
/** Returns true if field messageId is set (has been assigned a value) and false otherwise */
public boolean isSetMessageId() {
return this.messageId != null;
}
public void setMessageIdIsSet(boolean value) {
if (!value) {
this.messageId = null;
}
}
/**
* The body represents the Message's Payload, i.e. the actual message.
* If the Message Body is too long, it may be truncated. The complete
* body can then be loaded in a separate request.
*/
public String getBody() {
return this.body;
}
/**
* The body represents the Message's Payload, i.e. the actual message.
* If the Message Body is too long, it may be truncated. The complete
* body can then be loaded in a separate request.
*/
public Message setBody(String body) {
this.body = body;
return this;
}
public void unsetBody() {
this.body = null;
}
/** Returns true if field body is set (has been assigned a value) and false otherwise */
public boolean isSetBody() {
return this.body != null;
}
public void setBodyIsSet(boolean value) {
if (!value) {
this.body = null;
}
}
/**
*
* @see Urgency
*/
public Urgency getUrgency() {
return this.urgency;
}
/**
*
* @see Urgency
*/
public Message setUrgency(Urgency urgency) {
this.urgency = urgency;
return this;
}
public void unsetUrgency() {
this.urgency = null;
}
/** Returns true if field urgency is set (has been assigned a value) and false otherwise */
public boolean isSetUrgency() {
return this.urgency != null;
}
public void setUrgencyIsSet(boolean value) {
if (!value) {
this.urgency = null;
}
}
/**
* The time the message was created on the Application side
*/
public long getTimeOfCreation() {
return this.timeOfCreation;
}
/**
* The time the message was created on the Application side
*/
public Message setTimeOfCreation(long timeOfCreation) {
this.timeOfCreation = timeOfCreation;
setTimeOfCreationIsSet(true);
return this;
}
public void unsetTimeOfCreation() {
__isset_bitfield = EncodingUtils.clearBit(__isset_bitfield, __TIMEOFCREATION_ISSET_ID);
}
/** Returns true if field timeOfCreation is set (has been assigned a value) and false otherwise */
public boolean isSetTimeOfCreation() {
return EncodingUtils.testBit(__isset_bitfield, __TIMEOFCREATION_ISSET_ID);
}
public void setTimeOfCreationIsSet(boolean value) {
__isset_bitfield = EncodingUtils.setBit(__isset_bitfield, __TIMEOFCREATION_ISSET_ID, value);
}
/**
* The time the message was received on the Server side
*/
public long getTimeMessageReceived() {
return this.timeMessageReceived;
}
/**
* The time the message was received on the Server side
*/
public Message setTimeMessageReceived(long timeMessageReceived) {
this.timeMessageReceived = timeMessageReceived;
setTimeMessageReceivedIsSet(true);
return this;
}
public void unsetTimeMessageReceived() {
__isset_bitfield = EncodingUtils.clearBit(__isset_bitfield, __TIMEMESSAGERECEIVED_ISSET_ID);
}
/** Returns true if field timeMessageReceived is set (has been assigned a value) and false otherwise */
public boolean isSetTimeMessageReceived() {
return EncodingUtils.testBit(__isset_bitfield, __TIMEMESSAGERECEIVED_ISSET_ID);
}
public void setTimeMessageReceivedIsSet(boolean value) {
__isset_bitfield = EncodingUtils.setBit(__isset_bitfield, __TIMEMESSAGERECEIVED_ISSET_ID, value);
}
/**
* Name of the Application that sent the message.
*/
public String getApplicationName() {
return this.applicationName;
}
/**
* Name of the Application that sent the message.
*/
public Message setApplicationName(String applicationName) {
this.applicationName = applicationName;
return this;
}
public void unsetApplicationName() {
this.applicationName = null;
}
/** Returns true if field applicationName is set (has been assigned a value) and false otherwise */
public boolean isSetApplicationName() {
return this.applicationName != null;
}
public void setApplicationNameIsSet(boolean value) {
if (!value) {
this.applicationName = null;
}
}
/**
* The Network Hostname of the Device sending the message, ideally the FQDM.
*/
public String getHostname() {
return this.hostname;
}
/**
* The Network Hostname of the Device sending the message, ideally the FQDM.
*/
public Message setHostname(String hostname) {
this.hostname = hostname;
return this;
}
public void unsetHostname() {
this.hostname = null;
}
/** Returns true if field hostname is set (has been assigned a value) and false otherwise */
public boolean isSetHostname() {
return this.hostname != null;
}
public void setHostnameIsSet(boolean value) {
if (!value) {
this.hostname = null;
}
}
public String getMacAddress() {
return this.macAddress;
}
public Message setMacAddress(String macAddress) {
this.macAddress = macAddress;
return this;
}
public void unsetMacAddress() {
this.macAddress = null;
}
/** Returns true if field macAddress is set (has been assigned a value) and false otherwise */
public boolean isSetMacAddress() {
return this.macAddress != null;
}
public void setMacAddressIsSet(boolean value) {
if (!value) {
this.macAddress = null;
}
}
public boolean isIsTruncated() {
return this.isTruncated;
}
public Message setIsTruncated(boolean isTruncated) {
this.isTruncated = isTruncated;
setIsTruncatedIsSet(true);
return this;
}
public void unsetIsTruncated() {
__isset_bitfield = EncodingUtils.clearBit(__isset_bitfield, __ISTRUNCATED_ISSET_ID);
}
/** Returns true if field isTruncated is set (has been assigned a value) and false otherwise */
public boolean isSetIsTruncated() {
return EncodingUtils.testBit(__isset_bitfield, __ISTRUNCATED_ISSET_ID);
}
public void setIsTruncatedIsSet(boolean value) {
__isset_bitfield = EncodingUtils.setBit(__isset_bitfield, __ISTRUNCATED_ISSET_ID, value);
}
public String getTitle() {
return this.title;
}
public Message setTitle(String title) {
this.title = title;
return this;
}
public void unsetTitle() {
this.title = null;
}
/** Returns true if field title is set (has been assigned a value) and false otherwise */
public boolean isSetTitle() {
return this.title != null;
}
public void setTitleIsSet(boolean value) {
if (!value) {
this.title = null;
}
}
/**
* The ID of the Application the Message was sent from.
*/
public String getApplicationId() {
return this.applicationId;
}
/**
* The ID of the Application the Message was sent from.
*/
public Message setApplicationId(String applicationId) {
this.applicationId = applicationId;
return this;
}
public void unsetApplicationId() {
this.applicationId = null;
}
/** Returns true if field applicationId is set (has been assigned a value) and false otherwise */
public boolean isSetApplicationId() {
return this.applicationId != null;
}
public void setApplicationIdIsSet(boolean value) {
if (!value) {
this.applicationId = null;
}
}
public String getDeviceName() {
return this.deviceName;
}
public Message setDeviceName(String deviceName) {
this.deviceName = deviceName;
return this;
}
public void unsetDeviceName() {
this.deviceName = null;
}
/** Returns true if field deviceName is set (has been assigned a value) and false otherwise */
public boolean isSetDeviceName() {
return this.deviceName != null;
}
public void setDeviceNameIsSet(boolean value) {
if (!value) {
this.deviceName = null;
}
}
public void setFieldValue(_Fields field, Object value) {
switch (field) {
case MESSAGE_ID:
if (value == null) {
unsetMessageId();
} else {
setMessageId((String)value);
}
break;
case BODY:
if (value == null) {
unsetBody();
} else {
setBody((String)value);
}
break;
case URGENCY:
if (value == null) {
unsetUrgency();
} else {
setUrgency((Urgency)value);
}
break;
case TIME_OF_CREATION:
if (value == null) {
unsetTimeOfCreation();
} else {
setTimeOfCreation((Long)value);
}
break;
case TIME_MESSAGE_RECEIVED:
if (value == null) {
unsetTimeMessageReceived();
} else {
setTimeMessageReceived((Long)value);
}
break;
case APPLICATION_NAME:
if (value == null) {
unsetApplicationName();
} else {
setApplicationName((String)value);
}
break;
case HOSTNAME:
if (value == null) {
unsetHostname();
} else {
setHostname((String)value);
}
break;
case MAC_ADDRESS:
if (value == null) {
unsetMacAddress();
} else {
setMacAddress((String)value);
}
break;
case IS_TRUNCATED:
if (value == null) {
unsetIsTruncated();
} else {
setIsTruncated((Boolean)value);
}
break;
case TITLE:
if (value == null) {
unsetTitle();
} else {
setTitle((String)value);
}
break;
case APPLICATION_ID:
if (value == null) {
unsetApplicationId();
} else {
setApplicationId((String)value);
}
break;
case DEVICE_NAME:
if (value == null) {
unsetDeviceName();
} else {
setDeviceName((String)value);
}
break;
}
}
public Object getFieldValue(_Fields field) {
switch (field) {
case MESSAGE_ID:
return getMessageId();
case BODY:
return getBody();
case URGENCY:
return getUrgency();
case TIME_OF_CREATION:
return getTimeOfCreation();
case TIME_MESSAGE_RECEIVED:
return getTimeMessageReceived();
case APPLICATION_NAME:
return getApplicationName();
case HOSTNAME:
return getHostname();
case MAC_ADDRESS:
return getMacAddress();
case IS_TRUNCATED:
return isIsTruncated();
case TITLE:
return getTitle();
case APPLICATION_ID:
return getApplicationId();
case DEVICE_NAME:
return getDeviceName();
}
throw new IllegalStateException();
}
/** Returns true if field corresponding to fieldID is set (has been assigned a value) and false otherwise */
public boolean isSet(_Fields field) {
if (field == null) {
throw new IllegalArgumentException();
}
switch (field) {
case MESSAGE_ID:
return isSetMessageId();
case BODY:
return isSetBody();
case URGENCY:
return isSetUrgency();
case TIME_OF_CREATION:
return isSetTimeOfCreation();
case TIME_MESSAGE_RECEIVED:
return isSetTimeMessageReceived();
case APPLICATION_NAME:
return isSetApplicationName();
case HOSTNAME:
return isSetHostname();
case MAC_ADDRESS:
return isSetMacAddress();
case IS_TRUNCATED:
return isSetIsTruncated();
case TITLE:
return isSetTitle();
case APPLICATION_ID:
return isSetApplicationId();
case DEVICE_NAME:
return isSetDeviceName();
}
throw new IllegalStateException();
}
@Override
public boolean equals(Object that) {
if (that == null)
return false;
if (that instanceof Message)
return this.equals((Message)that);
return false;
}
public boolean equals(Message that) {
if (that == null)
return false;
boolean this_present_messageId = true && this.isSetMessageId();
boolean that_present_messageId = true && that.isSetMessageId();
if (this_present_messageId || that_present_messageId) {
if (!(this_present_messageId && that_present_messageId))
return false;
if (!this.messageId.equals(that.messageId))
return false;
}
boolean this_present_body = true && this.isSetBody();
boolean that_present_body = true && that.isSetBody();
if (this_present_body || that_present_body) {
if (!(this_present_body && that_present_body))
return false;
if (!this.body.equals(that.body))
return false;
}
boolean this_present_urgency = true && this.isSetUrgency();
boolean that_present_urgency = true && that.isSetUrgency();
if (this_present_urgency || that_present_urgency) {
if (!(this_present_urgency && that_present_urgency))
return false;
if (!this.urgency.equals(that.urgency))
return false;
}
boolean this_present_timeOfCreation = true;
boolean that_present_timeOfCreation = true;
if (this_present_timeOfCreation || that_present_timeOfCreation) {
if (!(this_present_timeOfCreation && that_present_timeOfCreation))
return false;
if (this.timeOfCreation != that.timeOfCreation)
return false;
}
boolean this_present_timeMessageReceived = true;
boolean that_present_timeMessageReceived = true;
if (this_present_timeMessageReceived || that_present_timeMessageReceived) {
if (!(this_present_timeMessageReceived && that_present_timeMessageReceived))
return false;
if (this.timeMessageReceived != that.timeMessageReceived)
return false;
}
boolean this_present_applicationName = true && this.isSetApplicationName();
boolean that_present_applicationName = true && that.isSetApplicationName();
if (this_present_applicationName || that_present_applicationName) {
if (!(this_present_applicationName && that_present_applicationName))
return false;
if (!this.applicationName.equals(that.applicationName))
return false;
}
boolean this_present_hostname = true && this.isSetHostname();
boolean that_present_hostname = true && that.isSetHostname();
if (this_present_hostname || that_present_hostname) {
if (!(this_present_hostname && that_present_hostname))
return false;
if (!this.hostname.equals(that.hostname))
return false;
}
boolean this_present_macAddress = true && this.isSetMacAddress();
boolean that_present_macAddress = true && that.isSetMacAddress();
if (this_present_macAddress || that_present_macAddress) {
if (!(this_present_macAddress && that_present_macAddress))
return false;
if (!this.macAddress.equals(that.macAddress))
return false;
}
boolean this_present_isTruncated = true && this.isSetIsTruncated();
boolean that_present_isTruncated = true && that.isSetIsTruncated();
if (this_present_isTruncated || that_present_isTruncated) {
if (!(this_present_isTruncated && that_present_isTruncated))
return false;
if (this.isTruncated != that.isTruncated)
return false;
}
boolean this_present_title = true && this.isSetTitle();
boolean that_present_title = true && that.isSetTitle();
if (this_present_title || that_present_title) {
if (!(this_present_title && that_present_title))
return false;
if (!this.title.equals(that.title))
return false;
}
boolean this_present_applicationId = true && this.isSetApplicationId();
boolean that_present_applicationId = true && that.isSetApplicationId();
if (this_present_applicationId || that_present_applicationId) {
if (!(this_present_applicationId && that_present_applicationId))
return false;
if (!this.applicationId.equals(that.applicationId))
return false;
}
boolean this_present_deviceName = true && this.isSetDeviceName();
boolean that_present_deviceName = true && that.isSetDeviceName();
if (this_present_deviceName || that_present_deviceName) {
if (!(this_present_deviceName && that_present_deviceName))
return false;
if (!this.deviceName.equals(that.deviceName))
return false;
}
return true;
}
@Override
public int hashCode() {
List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy