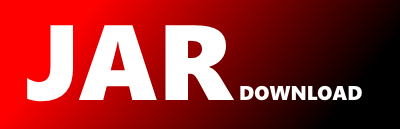
tech.aroma.thrift.Organization Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aroma-thrift Show documentation
Show all versions of aroma-thrift Show documentation
Part of the Aroma Project.
This project contains the Service and Model Definitions.
From this the Server and Client interfaces are generated for the Aroma Service.
/**
* Autogenerated by Thrift Compiler (0.9.3)
*
* DO NOT EDIT UNLESS YOU ARE SURE THAT YOU KNOW WHAT YOU ARE DOING
* @generated
*/
package tech.aroma.thrift;
import org.apache.thrift.scheme.IScheme;
import org.apache.thrift.scheme.SchemeFactory;
import org.apache.thrift.scheme.StandardScheme;
import org.apache.thrift.scheme.TupleScheme;
import org.apache.thrift.protocol.TTupleProtocol;
import org.apache.thrift.protocol.TProtocolException;
import org.apache.thrift.EncodingUtils;
import org.apache.thrift.TException;
import org.apache.thrift.async.AsyncMethodCallback;
import org.apache.thrift.server.AbstractNonblockingServer.*;
import java.util.List;
import java.util.ArrayList;
import java.util.Map;
import java.util.HashMap;
import java.util.EnumMap;
import java.util.Set;
import java.util.HashSet;
import java.util.EnumSet;
import java.util.Collections;
import java.util.BitSet;
import java.nio.ByteBuffer;
import java.util.Arrays;
import javax.annotation.Generated;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
@SuppressWarnings({"cast", "rawtypes", "serial", "unchecked"})
/**
* An Organization can represent a Company or group of People.
*/
@Generated(value = "Autogenerated by Thrift Compiler (0.9.3)", date = "2016-05-10")
public class Organization implements org.apache.thrift.TBase, java.io.Serializable, Cloneable, Comparable {
private static final org.apache.thrift.protocol.TStruct STRUCT_DESC = new org.apache.thrift.protocol.TStruct("Organization");
private static final org.apache.thrift.protocol.TField ORGANIZATION_ID_FIELD_DESC = new org.apache.thrift.protocol.TField("organizationId", org.apache.thrift.protocol.TType.STRING, (short)1);
private static final org.apache.thrift.protocol.TField ORGANIZATION_NAME_FIELD_DESC = new org.apache.thrift.protocol.TField("organizationName", org.apache.thrift.protocol.TType.STRING, (short)2);
private static final org.apache.thrift.protocol.TField WEBSITE_FIELD_DESC = new org.apache.thrift.protocol.TField("website", org.apache.thrift.protocol.TType.STRING, (short)3);
private static final org.apache.thrift.protocol.TField LOGO_FIELD_DESC = new org.apache.thrift.protocol.TField("logo", org.apache.thrift.protocol.TType.STRUCT, (short)4);
private static final org.apache.thrift.protocol.TField TECH_STACK_FIELD_DESC = new org.apache.thrift.protocol.TField("techStack", org.apache.thrift.protocol.TType.STRING, (short)5);
private static final org.apache.thrift.protocol.TField OWNERS_FIELD_DESC = new org.apache.thrift.protocol.TField("owners", org.apache.thrift.protocol.TType.LIST, (short)6);
private static final org.apache.thrift.protocol.TField ORGANIZATION_EMAIL_FIELD_DESC = new org.apache.thrift.protocol.TField("organizationEmail", org.apache.thrift.protocol.TType.STRING, (short)7);
private static final org.apache.thrift.protocol.TField STOCK_MARKET_SYMBOL_FIELD_DESC = new org.apache.thrift.protocol.TField("stockMarketSymbol", org.apache.thrift.protocol.TType.STRING, (short)8);
private static final org.apache.thrift.protocol.TField LOGO_LINK_FIELD_DESC = new org.apache.thrift.protocol.TField("logoLink", org.apache.thrift.protocol.TType.STRING, (short)9);
private static final org.apache.thrift.protocol.TField INDUSTRY_FIELD_DESC = new org.apache.thrift.protocol.TField("industry", org.apache.thrift.protocol.TType.I32, (short)10);
private static final org.apache.thrift.protocol.TField TIER_FIELD_DESC = new org.apache.thrift.protocol.TField("tier", org.apache.thrift.protocol.TType.I32, (short)11);
private static final org.apache.thrift.protocol.TField ORGANIZATION_DESCRIPTION_FIELD_DESC = new org.apache.thrift.protocol.TField("organizationDescription", org.apache.thrift.protocol.TType.STRING, (short)12);
private static final org.apache.thrift.protocol.TField GITHUB_PROFILE_FIELD_DESC = new org.apache.thrift.protocol.TField("githubProfile", org.apache.thrift.protocol.TType.STRING, (short)13);
private static final Map, SchemeFactory> schemes = new HashMap, SchemeFactory>();
static {
schemes.put(StandardScheme.class, new OrganizationStandardSchemeFactory());
schemes.put(TupleScheme.class, new OrganizationTupleSchemeFactory());
}
public String organizationId; // required
public String organizationName; // required
public String website; // optional
public Image logo; // optional
public String techStack; // optional
public List owners; // optional
public String organizationEmail; // optional
public String stockMarketSymbol; // optional
public String logoLink; // optional
/**
*
* @see Industry
*/
public Industry industry; // optional
/**
*
* @see Tier
*/
public Tier tier; // optional
public String organizationDescription; // optional
/**
* A Link to the Org's GitHub Page, if available.
*/
public String githubProfile; // optional
/** The set of fields this struct contains, along with convenience methods for finding and manipulating them. */
public enum _Fields implements org.apache.thrift.TFieldIdEnum {
ORGANIZATION_ID((short)1, "organizationId"),
ORGANIZATION_NAME((short)2, "organizationName"),
WEBSITE((short)3, "website"),
LOGO((short)4, "logo"),
TECH_STACK((short)5, "techStack"),
OWNERS((short)6, "owners"),
ORGANIZATION_EMAIL((short)7, "organizationEmail"),
STOCK_MARKET_SYMBOL((short)8, "stockMarketSymbol"),
LOGO_LINK((short)9, "logoLink"),
/**
*
* @see Industry
*/
INDUSTRY((short)10, "industry"),
/**
*
* @see Tier
*/
TIER((short)11, "tier"),
ORGANIZATION_DESCRIPTION((short)12, "organizationDescription"),
/**
* A Link to the Org's GitHub Page, if available.
*/
GITHUB_PROFILE((short)13, "githubProfile");
private static final Map byName = new HashMap();
static {
for (_Fields field : EnumSet.allOf(_Fields.class)) {
byName.put(field.getFieldName(), field);
}
}
/**
* Find the _Fields constant that matches fieldId, or null if its not found.
*/
public static _Fields findByThriftId(int fieldId) {
switch(fieldId) {
case 1: // ORGANIZATION_ID
return ORGANIZATION_ID;
case 2: // ORGANIZATION_NAME
return ORGANIZATION_NAME;
case 3: // WEBSITE
return WEBSITE;
case 4: // LOGO
return LOGO;
case 5: // TECH_STACK
return TECH_STACK;
case 6: // OWNERS
return OWNERS;
case 7: // ORGANIZATION_EMAIL
return ORGANIZATION_EMAIL;
case 8: // STOCK_MARKET_SYMBOL
return STOCK_MARKET_SYMBOL;
case 9: // LOGO_LINK
return LOGO_LINK;
case 10: // INDUSTRY
return INDUSTRY;
case 11: // TIER
return TIER;
case 12: // ORGANIZATION_DESCRIPTION
return ORGANIZATION_DESCRIPTION;
case 13: // GITHUB_PROFILE
return GITHUB_PROFILE;
default:
return null;
}
}
/**
* Find the _Fields constant that matches fieldId, throwing an exception
* if it is not found.
*/
public static _Fields findByThriftIdOrThrow(int fieldId) {
_Fields fields = findByThriftId(fieldId);
if (fields == null) throw new IllegalArgumentException("Field " + fieldId + " doesn't exist!");
return fields;
}
/**
* Find the _Fields constant that matches name, or null if its not found.
*/
public static _Fields findByName(String name) {
return byName.get(name);
}
private final short _thriftId;
private final String _fieldName;
_Fields(short thriftId, String fieldName) {
_thriftId = thriftId;
_fieldName = fieldName;
}
public short getThriftFieldId() {
return _thriftId;
}
public String getFieldName() {
return _fieldName;
}
}
// isset id assignments
private static final _Fields optionals[] = {_Fields.WEBSITE,_Fields.LOGO,_Fields.TECH_STACK,_Fields.OWNERS,_Fields.ORGANIZATION_EMAIL,_Fields.STOCK_MARKET_SYMBOL,_Fields.LOGO_LINK,_Fields.INDUSTRY,_Fields.TIER,_Fields.ORGANIZATION_DESCRIPTION,_Fields.GITHUB_PROFILE};
public static final Map<_Fields, org.apache.thrift.meta_data.FieldMetaData> metaDataMap;
static {
Map<_Fields, org.apache.thrift.meta_data.FieldMetaData> tmpMap = new EnumMap<_Fields, org.apache.thrift.meta_data.FieldMetaData>(_Fields.class);
tmpMap.put(_Fields.ORGANIZATION_ID, new org.apache.thrift.meta_data.FieldMetaData("organizationId", org.apache.thrift.TFieldRequirementType.DEFAULT,
new org.apache.thrift.meta_data.FieldValueMetaData(org.apache.thrift.protocol.TType.STRING , "uuid")));
tmpMap.put(_Fields.ORGANIZATION_NAME, new org.apache.thrift.meta_data.FieldMetaData("organizationName", org.apache.thrift.TFieldRequirementType.DEFAULT,
new org.apache.thrift.meta_data.FieldValueMetaData(org.apache.thrift.protocol.TType.STRING)));
tmpMap.put(_Fields.WEBSITE, new org.apache.thrift.meta_data.FieldMetaData("website", org.apache.thrift.TFieldRequirementType.OPTIONAL,
new org.apache.thrift.meta_data.FieldValueMetaData(org.apache.thrift.protocol.TType.STRING)));
tmpMap.put(_Fields.LOGO, new org.apache.thrift.meta_data.FieldMetaData("logo", org.apache.thrift.TFieldRequirementType.OPTIONAL,
new org.apache.thrift.meta_data.StructMetaData(org.apache.thrift.protocol.TType.STRUCT, Image.class)));
tmpMap.put(_Fields.TECH_STACK, new org.apache.thrift.meta_data.FieldMetaData("techStack", org.apache.thrift.TFieldRequirementType.OPTIONAL,
new org.apache.thrift.meta_data.FieldValueMetaData(org.apache.thrift.protocol.TType.STRING)));
tmpMap.put(_Fields.OWNERS, new org.apache.thrift.meta_data.FieldMetaData("owners", org.apache.thrift.TFieldRequirementType.OPTIONAL,
new org.apache.thrift.meta_data.ListMetaData(org.apache.thrift.protocol.TType.LIST,
new org.apache.thrift.meta_data.FieldValueMetaData(org.apache.thrift.protocol.TType.STRING))));
tmpMap.put(_Fields.ORGANIZATION_EMAIL, new org.apache.thrift.meta_data.FieldMetaData("organizationEmail", org.apache.thrift.TFieldRequirementType.OPTIONAL,
new org.apache.thrift.meta_data.FieldValueMetaData(org.apache.thrift.protocol.TType.STRING)));
tmpMap.put(_Fields.STOCK_MARKET_SYMBOL, new org.apache.thrift.meta_data.FieldMetaData("stockMarketSymbol", org.apache.thrift.TFieldRequirementType.OPTIONAL,
new org.apache.thrift.meta_data.FieldValueMetaData(org.apache.thrift.protocol.TType.STRING)));
tmpMap.put(_Fields.LOGO_LINK, new org.apache.thrift.meta_data.FieldMetaData("logoLink", org.apache.thrift.TFieldRequirementType.OPTIONAL,
new org.apache.thrift.meta_data.FieldValueMetaData(org.apache.thrift.protocol.TType.STRING)));
tmpMap.put(_Fields.INDUSTRY, new org.apache.thrift.meta_data.FieldMetaData("industry", org.apache.thrift.TFieldRequirementType.OPTIONAL,
new org.apache.thrift.meta_data.EnumMetaData(org.apache.thrift.protocol.TType.ENUM, Industry.class)));
tmpMap.put(_Fields.TIER, new org.apache.thrift.meta_data.FieldMetaData("tier", org.apache.thrift.TFieldRequirementType.OPTIONAL,
new org.apache.thrift.meta_data.EnumMetaData(org.apache.thrift.protocol.TType.ENUM, Tier.class)));
tmpMap.put(_Fields.ORGANIZATION_DESCRIPTION, new org.apache.thrift.meta_data.FieldMetaData("organizationDescription", org.apache.thrift.TFieldRequirementType.OPTIONAL,
new org.apache.thrift.meta_data.FieldValueMetaData(org.apache.thrift.protocol.TType.STRING)));
tmpMap.put(_Fields.GITHUB_PROFILE, new org.apache.thrift.meta_data.FieldMetaData("githubProfile", org.apache.thrift.TFieldRequirementType.OPTIONAL,
new org.apache.thrift.meta_data.FieldValueMetaData(org.apache.thrift.protocol.TType.STRING)));
metaDataMap = Collections.unmodifiableMap(tmpMap);
org.apache.thrift.meta_data.FieldMetaData.addStructMetaDataMap(Organization.class, metaDataMap);
}
public Organization() {
this.tier = tech.aroma.thrift.Tier.FREE;
}
public Organization(
String organizationId,
String organizationName)
{
this();
this.organizationId = organizationId;
this.organizationName = organizationName;
}
/**
* Performs a deep copy on other.
*/
public Organization(Organization other) {
if (other.isSetOrganizationId()) {
this.organizationId = other.organizationId;
}
if (other.isSetOrganizationName()) {
this.organizationName = other.organizationName;
}
if (other.isSetWebsite()) {
this.website = other.website;
}
if (other.isSetLogo()) {
this.logo = new Image(other.logo);
}
if (other.isSetTechStack()) {
this.techStack = other.techStack;
}
if (other.isSetOwners()) {
List __this__owners = new ArrayList(other.owners);
this.owners = __this__owners;
}
if (other.isSetOrganizationEmail()) {
this.organizationEmail = other.organizationEmail;
}
if (other.isSetStockMarketSymbol()) {
this.stockMarketSymbol = other.stockMarketSymbol;
}
if (other.isSetLogoLink()) {
this.logoLink = other.logoLink;
}
if (other.isSetIndustry()) {
this.industry = other.industry;
}
if (other.isSetTier()) {
this.tier = other.tier;
}
if (other.isSetOrganizationDescription()) {
this.organizationDescription = other.organizationDescription;
}
if (other.isSetGithubProfile()) {
this.githubProfile = other.githubProfile;
}
}
public Organization deepCopy() {
return new Organization(this);
}
@Override
public void clear() {
this.organizationId = null;
this.organizationName = null;
this.website = null;
this.logo = null;
this.techStack = null;
this.owners = null;
this.organizationEmail = null;
this.stockMarketSymbol = null;
this.logoLink = null;
this.industry = null;
this.tier = tech.aroma.thrift.Tier.FREE;
this.organizationDescription = null;
this.githubProfile = null;
}
public String getOrganizationId() {
return this.organizationId;
}
public Organization setOrganizationId(String organizationId) {
this.organizationId = organizationId;
return this;
}
public void unsetOrganizationId() {
this.organizationId = null;
}
/** Returns true if field organizationId is set (has been assigned a value) and false otherwise */
public boolean isSetOrganizationId() {
return this.organizationId != null;
}
public void setOrganizationIdIsSet(boolean value) {
if (!value) {
this.organizationId = null;
}
}
public String getOrganizationName() {
return this.organizationName;
}
public Organization setOrganizationName(String organizationName) {
this.organizationName = organizationName;
return this;
}
public void unsetOrganizationName() {
this.organizationName = null;
}
/** Returns true if field organizationName is set (has been assigned a value) and false otherwise */
public boolean isSetOrganizationName() {
return this.organizationName != null;
}
public void setOrganizationNameIsSet(boolean value) {
if (!value) {
this.organizationName = null;
}
}
public String getWebsite() {
return this.website;
}
public Organization setWebsite(String website) {
this.website = website;
return this;
}
public void unsetWebsite() {
this.website = null;
}
/** Returns true if field website is set (has been assigned a value) and false otherwise */
public boolean isSetWebsite() {
return this.website != null;
}
public void setWebsiteIsSet(boolean value) {
if (!value) {
this.website = null;
}
}
public Image getLogo() {
return this.logo;
}
public Organization setLogo(Image logo) {
this.logo = logo;
return this;
}
public void unsetLogo() {
this.logo = null;
}
/** Returns true if field logo is set (has been assigned a value) and false otherwise */
public boolean isSetLogo() {
return this.logo != null;
}
public void setLogoIsSet(boolean value) {
if (!value) {
this.logo = null;
}
}
public String getTechStack() {
return this.techStack;
}
public Organization setTechStack(String techStack) {
this.techStack = techStack;
return this;
}
public void unsetTechStack() {
this.techStack = null;
}
/** Returns true if field techStack is set (has been assigned a value) and false otherwise */
public boolean isSetTechStack() {
return this.techStack != null;
}
public void setTechStackIsSet(boolean value) {
if (!value) {
this.techStack = null;
}
}
public int getOwnersSize() {
return (this.owners == null) ? 0 : this.owners.size();
}
public java.util.Iterator getOwnersIterator() {
return (this.owners == null) ? null : this.owners.iterator();
}
public void addToOwners(String elem) {
if (this.owners == null) {
this.owners = new ArrayList();
}
this.owners.add(elem);
}
public List getOwners() {
return this.owners;
}
public Organization setOwners(List owners) {
this.owners = owners;
return this;
}
public void unsetOwners() {
this.owners = null;
}
/** Returns true if field owners is set (has been assigned a value) and false otherwise */
public boolean isSetOwners() {
return this.owners != null;
}
public void setOwnersIsSet(boolean value) {
if (!value) {
this.owners = null;
}
}
public String getOrganizationEmail() {
return this.organizationEmail;
}
public Organization setOrganizationEmail(String organizationEmail) {
this.organizationEmail = organizationEmail;
return this;
}
public void unsetOrganizationEmail() {
this.organizationEmail = null;
}
/** Returns true if field organizationEmail is set (has been assigned a value) and false otherwise */
public boolean isSetOrganizationEmail() {
return this.organizationEmail != null;
}
public void setOrganizationEmailIsSet(boolean value) {
if (!value) {
this.organizationEmail = null;
}
}
public String getStockMarketSymbol() {
return this.stockMarketSymbol;
}
public Organization setStockMarketSymbol(String stockMarketSymbol) {
this.stockMarketSymbol = stockMarketSymbol;
return this;
}
public void unsetStockMarketSymbol() {
this.stockMarketSymbol = null;
}
/** Returns true if field stockMarketSymbol is set (has been assigned a value) and false otherwise */
public boolean isSetStockMarketSymbol() {
return this.stockMarketSymbol != null;
}
public void setStockMarketSymbolIsSet(boolean value) {
if (!value) {
this.stockMarketSymbol = null;
}
}
public String getLogoLink() {
return this.logoLink;
}
public Organization setLogoLink(String logoLink) {
this.logoLink = logoLink;
return this;
}
public void unsetLogoLink() {
this.logoLink = null;
}
/** Returns true if field logoLink is set (has been assigned a value) and false otherwise */
public boolean isSetLogoLink() {
return this.logoLink != null;
}
public void setLogoLinkIsSet(boolean value) {
if (!value) {
this.logoLink = null;
}
}
/**
*
* @see Industry
*/
public Industry getIndustry() {
return this.industry;
}
/**
*
* @see Industry
*/
public Organization setIndustry(Industry industry) {
this.industry = industry;
return this;
}
public void unsetIndustry() {
this.industry = null;
}
/** Returns true if field industry is set (has been assigned a value) and false otherwise */
public boolean isSetIndustry() {
return this.industry != null;
}
public void setIndustryIsSet(boolean value) {
if (!value) {
this.industry = null;
}
}
/**
*
* @see Tier
*/
public Tier getTier() {
return this.tier;
}
/**
*
* @see Tier
*/
public Organization setTier(Tier tier) {
this.tier = tier;
return this;
}
public void unsetTier() {
this.tier = null;
}
/** Returns true if field tier is set (has been assigned a value) and false otherwise */
public boolean isSetTier() {
return this.tier != null;
}
public void setTierIsSet(boolean value) {
if (!value) {
this.tier = null;
}
}
public String getOrganizationDescription() {
return this.organizationDescription;
}
public Organization setOrganizationDescription(String organizationDescription) {
this.organizationDescription = organizationDescription;
return this;
}
public void unsetOrganizationDescription() {
this.organizationDescription = null;
}
/** Returns true if field organizationDescription is set (has been assigned a value) and false otherwise */
public boolean isSetOrganizationDescription() {
return this.organizationDescription != null;
}
public void setOrganizationDescriptionIsSet(boolean value) {
if (!value) {
this.organizationDescription = null;
}
}
/**
* A Link to the Org's GitHub Page, if available.
*/
public String getGithubProfile() {
return this.githubProfile;
}
/**
* A Link to the Org's GitHub Page, if available.
*/
public Organization setGithubProfile(String githubProfile) {
this.githubProfile = githubProfile;
return this;
}
public void unsetGithubProfile() {
this.githubProfile = null;
}
/** Returns true if field githubProfile is set (has been assigned a value) and false otherwise */
public boolean isSetGithubProfile() {
return this.githubProfile != null;
}
public void setGithubProfileIsSet(boolean value) {
if (!value) {
this.githubProfile = null;
}
}
public void setFieldValue(_Fields field, Object value) {
switch (field) {
case ORGANIZATION_ID:
if (value == null) {
unsetOrganizationId();
} else {
setOrganizationId((String)value);
}
break;
case ORGANIZATION_NAME:
if (value == null) {
unsetOrganizationName();
} else {
setOrganizationName((String)value);
}
break;
case WEBSITE:
if (value == null) {
unsetWebsite();
} else {
setWebsite((String)value);
}
break;
case LOGO:
if (value == null) {
unsetLogo();
} else {
setLogo((Image)value);
}
break;
case TECH_STACK:
if (value == null) {
unsetTechStack();
} else {
setTechStack((String)value);
}
break;
case OWNERS:
if (value == null) {
unsetOwners();
} else {
setOwners((List)value);
}
break;
case ORGANIZATION_EMAIL:
if (value == null) {
unsetOrganizationEmail();
} else {
setOrganizationEmail((String)value);
}
break;
case STOCK_MARKET_SYMBOL:
if (value == null) {
unsetStockMarketSymbol();
} else {
setStockMarketSymbol((String)value);
}
break;
case LOGO_LINK:
if (value == null) {
unsetLogoLink();
} else {
setLogoLink((String)value);
}
break;
case INDUSTRY:
if (value == null) {
unsetIndustry();
} else {
setIndustry((Industry)value);
}
break;
case TIER:
if (value == null) {
unsetTier();
} else {
setTier((Tier)value);
}
break;
case ORGANIZATION_DESCRIPTION:
if (value == null) {
unsetOrganizationDescription();
} else {
setOrganizationDescription((String)value);
}
break;
case GITHUB_PROFILE:
if (value == null) {
unsetGithubProfile();
} else {
setGithubProfile((String)value);
}
break;
}
}
public Object getFieldValue(_Fields field) {
switch (field) {
case ORGANIZATION_ID:
return getOrganizationId();
case ORGANIZATION_NAME:
return getOrganizationName();
case WEBSITE:
return getWebsite();
case LOGO:
return getLogo();
case TECH_STACK:
return getTechStack();
case OWNERS:
return getOwners();
case ORGANIZATION_EMAIL:
return getOrganizationEmail();
case STOCK_MARKET_SYMBOL:
return getStockMarketSymbol();
case LOGO_LINK:
return getLogoLink();
case INDUSTRY:
return getIndustry();
case TIER:
return getTier();
case ORGANIZATION_DESCRIPTION:
return getOrganizationDescription();
case GITHUB_PROFILE:
return getGithubProfile();
}
throw new IllegalStateException();
}
/** Returns true if field corresponding to fieldID is set (has been assigned a value) and false otherwise */
public boolean isSet(_Fields field) {
if (field == null) {
throw new IllegalArgumentException();
}
switch (field) {
case ORGANIZATION_ID:
return isSetOrganizationId();
case ORGANIZATION_NAME:
return isSetOrganizationName();
case WEBSITE:
return isSetWebsite();
case LOGO:
return isSetLogo();
case TECH_STACK:
return isSetTechStack();
case OWNERS:
return isSetOwners();
case ORGANIZATION_EMAIL:
return isSetOrganizationEmail();
case STOCK_MARKET_SYMBOL:
return isSetStockMarketSymbol();
case LOGO_LINK:
return isSetLogoLink();
case INDUSTRY:
return isSetIndustry();
case TIER:
return isSetTier();
case ORGANIZATION_DESCRIPTION:
return isSetOrganizationDescription();
case GITHUB_PROFILE:
return isSetGithubProfile();
}
throw new IllegalStateException();
}
@Override
public boolean equals(Object that) {
if (that == null)
return false;
if (that instanceof Organization)
return this.equals((Organization)that);
return false;
}
public boolean equals(Organization that) {
if (that == null)
return false;
boolean this_present_organizationId = true && this.isSetOrganizationId();
boolean that_present_organizationId = true && that.isSetOrganizationId();
if (this_present_organizationId || that_present_organizationId) {
if (!(this_present_organizationId && that_present_organizationId))
return false;
if (!this.organizationId.equals(that.organizationId))
return false;
}
boolean this_present_organizationName = true && this.isSetOrganizationName();
boolean that_present_organizationName = true && that.isSetOrganizationName();
if (this_present_organizationName || that_present_organizationName) {
if (!(this_present_organizationName && that_present_organizationName))
return false;
if (!this.organizationName.equals(that.organizationName))
return false;
}
boolean this_present_website = true && this.isSetWebsite();
boolean that_present_website = true && that.isSetWebsite();
if (this_present_website || that_present_website) {
if (!(this_present_website && that_present_website))
return false;
if (!this.website.equals(that.website))
return false;
}
boolean this_present_logo = true && this.isSetLogo();
boolean that_present_logo = true && that.isSetLogo();
if (this_present_logo || that_present_logo) {
if (!(this_present_logo && that_present_logo))
return false;
if (!this.logo.equals(that.logo))
return false;
}
boolean this_present_techStack = true && this.isSetTechStack();
boolean that_present_techStack = true && that.isSetTechStack();
if (this_present_techStack || that_present_techStack) {
if (!(this_present_techStack && that_present_techStack))
return false;
if (!this.techStack.equals(that.techStack))
return false;
}
boolean this_present_owners = true && this.isSetOwners();
boolean that_present_owners = true && that.isSetOwners();
if (this_present_owners || that_present_owners) {
if (!(this_present_owners && that_present_owners))
return false;
if (!this.owners.equals(that.owners))
return false;
}
boolean this_present_organizationEmail = true && this.isSetOrganizationEmail();
boolean that_present_organizationEmail = true && that.isSetOrganizationEmail();
if (this_present_organizationEmail || that_present_organizationEmail) {
if (!(this_present_organizationEmail && that_present_organizationEmail))
return false;
if (!this.organizationEmail.equals(that.organizationEmail))
return false;
}
boolean this_present_stockMarketSymbol = true && this.isSetStockMarketSymbol();
boolean that_present_stockMarketSymbol = true && that.isSetStockMarketSymbol();
if (this_present_stockMarketSymbol || that_present_stockMarketSymbol) {
if (!(this_present_stockMarketSymbol && that_present_stockMarketSymbol))
return false;
if (!this.stockMarketSymbol.equals(that.stockMarketSymbol))
return false;
}
boolean this_present_logoLink = true && this.isSetLogoLink();
boolean that_present_logoLink = true && that.isSetLogoLink();
if (this_present_logoLink || that_present_logoLink) {
if (!(this_present_logoLink && that_present_logoLink))
return false;
if (!this.logoLink.equals(that.logoLink))
return false;
}
boolean this_present_industry = true && this.isSetIndustry();
boolean that_present_industry = true && that.isSetIndustry();
if (this_present_industry || that_present_industry) {
if (!(this_present_industry && that_present_industry))
return false;
if (!this.industry.equals(that.industry))
return false;
}
boolean this_present_tier = true && this.isSetTier();
boolean that_present_tier = true && that.isSetTier();
if (this_present_tier || that_present_tier) {
if (!(this_present_tier && that_present_tier))
return false;
if (!this.tier.equals(that.tier))
return false;
}
boolean this_present_organizationDescription = true && this.isSetOrganizationDescription();
boolean that_present_organizationDescription = true && that.isSetOrganizationDescription();
if (this_present_organizationDescription || that_present_organizationDescription) {
if (!(this_present_organizationDescription && that_present_organizationDescription))
return false;
if (!this.organizationDescription.equals(that.organizationDescription))
return false;
}
boolean this_present_githubProfile = true && this.isSetGithubProfile();
boolean that_present_githubProfile = true && that.isSetGithubProfile();
if (this_present_githubProfile || that_present_githubProfile) {
if (!(this_present_githubProfile && that_present_githubProfile))
return false;
if (!this.githubProfile.equals(that.githubProfile))
return false;
}
return true;
}
@Override
public int hashCode() {
List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy