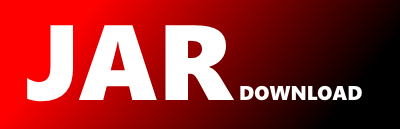
tech.aroma.thrift.User Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aroma-thrift Show documentation
Show all versions of aroma-thrift Show documentation
Part of the Aroma Project.
This project contains the Service and Model Definitions.
From this the Server and Client interfaces are generated for the Aroma Service.
/**
* Autogenerated by Thrift Compiler (0.9.3)
*
* DO NOT EDIT UNLESS YOU ARE SURE THAT YOU KNOW WHAT YOU ARE DOING
* @generated
*/
package tech.aroma.thrift;
import org.apache.thrift.scheme.IScheme;
import org.apache.thrift.scheme.SchemeFactory;
import org.apache.thrift.scheme.StandardScheme;
import org.apache.thrift.scheme.TupleScheme;
import org.apache.thrift.protocol.TTupleProtocol;
import org.apache.thrift.protocol.TProtocolException;
import org.apache.thrift.EncodingUtils;
import org.apache.thrift.TException;
import org.apache.thrift.async.AsyncMethodCallback;
import org.apache.thrift.server.AbstractNonblockingServer.*;
import java.util.List;
import java.util.ArrayList;
import java.util.Map;
import java.util.HashMap;
import java.util.EnumMap;
import java.util.Set;
import java.util.HashSet;
import java.util.EnumSet;
import java.util.Collections;
import java.util.BitSet;
import java.nio.ByteBuffer;
import java.util.Arrays;
import javax.annotation.Generated;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
@SuppressWarnings({"cast", "rawtypes", "serial", "unchecked"})
/**
* A User represents a Human who is a uses the Service.
*/
@Generated(value = "Autogenerated by Thrift Compiler (0.9.3)", date = "2016-05-10")
public class User implements org.apache.thrift.TBase, java.io.Serializable, Cloneable, Comparable {
private static final org.apache.thrift.protocol.TStruct STRUCT_DESC = new org.apache.thrift.protocol.TStruct("User");
private static final org.apache.thrift.protocol.TField EMAIL_FIELD_DESC = new org.apache.thrift.protocol.TField("email", org.apache.thrift.protocol.TType.STRING, (short)1);
private static final org.apache.thrift.protocol.TField USER_ID_FIELD_DESC = new org.apache.thrift.protocol.TField("userId", org.apache.thrift.protocol.TType.STRING, (short)2);
private static final org.apache.thrift.protocol.TField NAME_FIELD_DESC = new org.apache.thrift.protocol.TField("name", org.apache.thrift.protocol.TType.STRING, (short)3);
private static final org.apache.thrift.protocol.TField ROLES_FIELD_DESC = new org.apache.thrift.protocol.TField("roles", org.apache.thrift.protocol.TType.SET, (short)4);
private static final org.apache.thrift.protocol.TField PROFILE_IMAGE_FIELD_DESC = new org.apache.thrift.protocol.TField("profileImage", org.apache.thrift.protocol.TType.STRUCT, (short)5);
private static final org.apache.thrift.protocol.TField PROFILE_IMAGE_LINK_FIELD_DESC = new org.apache.thrift.protocol.TField("profileImageLink", org.apache.thrift.protocol.TType.STRING, (short)6);
private static final org.apache.thrift.protocol.TField GITHUB_PROFILE_FIELD_DESC = new org.apache.thrift.protocol.TField("githubProfile", org.apache.thrift.protocol.TType.STRING, (short)7);
private static final org.apache.thrift.protocol.TField FIRST_NAME_FIELD_DESC = new org.apache.thrift.protocol.TField("firstName", org.apache.thrift.protocol.TType.STRING, (short)8);
private static final org.apache.thrift.protocol.TField MIDDLE_NAME_FIELD_DESC = new org.apache.thrift.protocol.TField("middleName", org.apache.thrift.protocol.TType.STRING, (short)9);
private static final org.apache.thrift.protocol.TField LAST_NAME_FIELD_DESC = new org.apache.thrift.protocol.TField("lastName", org.apache.thrift.protocol.TType.STRING, (short)10);
private static final org.apache.thrift.protocol.TField BIRTHDATE_FIELD_DESC = new org.apache.thrift.protocol.TField("birthdate", org.apache.thrift.protocol.TType.I64, (short)11);
private static final org.apache.thrift.protocol.TField TIME_USER_JOINED_FIELD_DESC = new org.apache.thrift.protocol.TField("timeUserJoined", org.apache.thrift.protocol.TType.I64, (short)12);
private static final Map, SchemeFactory> schemes = new HashMap, SchemeFactory>();
static {
schemes.put(StandardScheme.class, new UserStandardSchemeFactory());
schemes.put(TupleScheme.class, new UserTupleSchemeFactory());
}
/**
* A person's email address is also considered their identifying trait.
*/
public String email; // required
public String userId; // required
public String name; // optional
/**
* A Person can be more than one thing at once. By default, we assume a developer.
*/
public Set roles; // required
public Image profileImage; // optional
public String profileImageLink; // optional
public String githubProfile; // optional
public String firstName; // optional
public String middleName; // optional
public String lastName; // optional
public long birthdate; // optional
public long timeUserJoined; // optional
/** The set of fields this struct contains, along with convenience methods for finding and manipulating them. */
public enum _Fields implements org.apache.thrift.TFieldIdEnum {
/**
* A person's email address is also considered their identifying trait.
*/
EMAIL((short)1, "email"),
USER_ID((short)2, "userId"),
NAME((short)3, "name"),
/**
* A Person can be more than one thing at once. By default, we assume a developer.
*/
ROLES((short)4, "roles"),
PROFILE_IMAGE((short)5, "profileImage"),
PROFILE_IMAGE_LINK((short)6, "profileImageLink"),
GITHUB_PROFILE((short)7, "githubProfile"),
FIRST_NAME((short)8, "firstName"),
MIDDLE_NAME((short)9, "middleName"),
LAST_NAME((short)10, "lastName"),
BIRTHDATE((short)11, "birthdate"),
TIME_USER_JOINED((short)12, "timeUserJoined");
private static final Map byName = new HashMap();
static {
for (_Fields field : EnumSet.allOf(_Fields.class)) {
byName.put(field.getFieldName(), field);
}
}
/**
* Find the _Fields constant that matches fieldId, or null if its not found.
*/
public static _Fields findByThriftId(int fieldId) {
switch(fieldId) {
case 1: // EMAIL
return EMAIL;
case 2: // USER_ID
return USER_ID;
case 3: // NAME
return NAME;
case 4: // ROLES
return ROLES;
case 5: // PROFILE_IMAGE
return PROFILE_IMAGE;
case 6: // PROFILE_IMAGE_LINK
return PROFILE_IMAGE_LINK;
case 7: // GITHUB_PROFILE
return GITHUB_PROFILE;
case 8: // FIRST_NAME
return FIRST_NAME;
case 9: // MIDDLE_NAME
return MIDDLE_NAME;
case 10: // LAST_NAME
return LAST_NAME;
case 11: // BIRTHDATE
return BIRTHDATE;
case 12: // TIME_USER_JOINED
return TIME_USER_JOINED;
default:
return null;
}
}
/**
* Find the _Fields constant that matches fieldId, throwing an exception
* if it is not found.
*/
public static _Fields findByThriftIdOrThrow(int fieldId) {
_Fields fields = findByThriftId(fieldId);
if (fields == null) throw new IllegalArgumentException("Field " + fieldId + " doesn't exist!");
return fields;
}
/**
* Find the _Fields constant that matches name, or null if its not found.
*/
public static _Fields findByName(String name) {
return byName.get(name);
}
private final short _thriftId;
private final String _fieldName;
_Fields(short thriftId, String fieldName) {
_thriftId = thriftId;
_fieldName = fieldName;
}
public short getThriftFieldId() {
return _thriftId;
}
public String getFieldName() {
return _fieldName;
}
}
// isset id assignments
private static final int __BIRTHDATE_ISSET_ID = 0;
private static final int __TIMEUSERJOINED_ISSET_ID = 1;
private byte __isset_bitfield = 0;
private static final _Fields optionals[] = {_Fields.NAME,_Fields.PROFILE_IMAGE,_Fields.PROFILE_IMAGE_LINK,_Fields.GITHUB_PROFILE,_Fields.FIRST_NAME,_Fields.MIDDLE_NAME,_Fields.LAST_NAME,_Fields.BIRTHDATE,_Fields.TIME_USER_JOINED};
public static final Map<_Fields, org.apache.thrift.meta_data.FieldMetaData> metaDataMap;
static {
Map<_Fields, org.apache.thrift.meta_data.FieldMetaData> tmpMap = new EnumMap<_Fields, org.apache.thrift.meta_data.FieldMetaData>(_Fields.class);
tmpMap.put(_Fields.EMAIL, new org.apache.thrift.meta_data.FieldMetaData("email", org.apache.thrift.TFieldRequirementType.DEFAULT,
new org.apache.thrift.meta_data.FieldValueMetaData(org.apache.thrift.protocol.TType.STRING)));
tmpMap.put(_Fields.USER_ID, new org.apache.thrift.meta_data.FieldMetaData("userId", org.apache.thrift.TFieldRequirementType.DEFAULT,
new org.apache.thrift.meta_data.FieldValueMetaData(org.apache.thrift.protocol.TType.STRING , "uuid")));
tmpMap.put(_Fields.NAME, new org.apache.thrift.meta_data.FieldMetaData("name", org.apache.thrift.TFieldRequirementType.OPTIONAL,
new org.apache.thrift.meta_data.FieldValueMetaData(org.apache.thrift.protocol.TType.STRING)));
tmpMap.put(_Fields.ROLES, new org.apache.thrift.meta_data.FieldMetaData("roles", org.apache.thrift.TFieldRequirementType.DEFAULT,
new org.apache.thrift.meta_data.SetMetaData(org.apache.thrift.protocol.TType.SET,
new org.apache.thrift.meta_data.EnumMetaData(org.apache.thrift.protocol.TType.ENUM, Role.class))));
tmpMap.put(_Fields.PROFILE_IMAGE, new org.apache.thrift.meta_data.FieldMetaData("profileImage", org.apache.thrift.TFieldRequirementType.OPTIONAL,
new org.apache.thrift.meta_data.StructMetaData(org.apache.thrift.protocol.TType.STRUCT, Image.class)));
tmpMap.put(_Fields.PROFILE_IMAGE_LINK, new org.apache.thrift.meta_data.FieldMetaData("profileImageLink", org.apache.thrift.TFieldRequirementType.OPTIONAL,
new org.apache.thrift.meta_data.FieldValueMetaData(org.apache.thrift.protocol.TType.STRING , "uuid")));
tmpMap.put(_Fields.GITHUB_PROFILE, new org.apache.thrift.meta_data.FieldMetaData("githubProfile", org.apache.thrift.TFieldRequirementType.OPTIONAL,
new org.apache.thrift.meta_data.FieldValueMetaData(org.apache.thrift.protocol.TType.STRING)));
tmpMap.put(_Fields.FIRST_NAME, new org.apache.thrift.meta_data.FieldMetaData("firstName", org.apache.thrift.TFieldRequirementType.OPTIONAL,
new org.apache.thrift.meta_data.FieldValueMetaData(org.apache.thrift.protocol.TType.STRING)));
tmpMap.put(_Fields.MIDDLE_NAME, new org.apache.thrift.meta_data.FieldMetaData("middleName", org.apache.thrift.TFieldRequirementType.OPTIONAL,
new org.apache.thrift.meta_data.FieldValueMetaData(org.apache.thrift.protocol.TType.STRING)));
tmpMap.put(_Fields.LAST_NAME, new org.apache.thrift.meta_data.FieldMetaData("lastName", org.apache.thrift.TFieldRequirementType.OPTIONAL,
new org.apache.thrift.meta_data.FieldValueMetaData(org.apache.thrift.protocol.TType.STRING)));
tmpMap.put(_Fields.BIRTHDATE, new org.apache.thrift.meta_data.FieldMetaData("birthdate", org.apache.thrift.TFieldRequirementType.OPTIONAL,
new org.apache.thrift.meta_data.FieldValueMetaData(org.apache.thrift.protocol.TType.I64 , "timestamp")));
tmpMap.put(_Fields.TIME_USER_JOINED, new org.apache.thrift.meta_data.FieldMetaData("timeUserJoined", org.apache.thrift.TFieldRequirementType.OPTIONAL,
new org.apache.thrift.meta_data.FieldValueMetaData(org.apache.thrift.protocol.TType.I64 , "timestamp")));
metaDataMap = Collections.unmodifiableMap(tmpMap);
org.apache.thrift.meta_data.FieldMetaData.addStructMetaDataMap(User.class, metaDataMap);
}
public User() {
this.roles = new HashSet();
this.roles.add(tech.aroma.thrift.Role.DEVELOPER);
}
public User(
String email,
String userId,
Set roles)
{
this();
this.email = email;
this.userId = userId;
this.roles = roles;
}
/**
* Performs a deep copy on other.
*/
public User(User other) {
__isset_bitfield = other.__isset_bitfield;
if (other.isSetEmail()) {
this.email = other.email;
}
if (other.isSetUserId()) {
this.userId = other.userId;
}
if (other.isSetName()) {
this.name = other.name;
}
if (other.isSetRoles()) {
Set __this__roles = new HashSet(other.roles.size());
for (Role other_element : other.roles) {
__this__roles.add(other_element);
}
this.roles = __this__roles;
}
if (other.isSetProfileImage()) {
this.profileImage = new Image(other.profileImage);
}
if (other.isSetProfileImageLink()) {
this.profileImageLink = other.profileImageLink;
}
if (other.isSetGithubProfile()) {
this.githubProfile = other.githubProfile;
}
if (other.isSetFirstName()) {
this.firstName = other.firstName;
}
if (other.isSetMiddleName()) {
this.middleName = other.middleName;
}
if (other.isSetLastName()) {
this.lastName = other.lastName;
}
this.birthdate = other.birthdate;
this.timeUserJoined = other.timeUserJoined;
}
public User deepCopy() {
return new User(this);
}
@Override
public void clear() {
this.email = null;
this.userId = null;
this.name = null;
this.roles = new HashSet();
this.roles.add(tech.aroma.thrift.Role.DEVELOPER);
this.profileImage = null;
this.profileImageLink = null;
this.githubProfile = null;
this.firstName = null;
this.middleName = null;
this.lastName = null;
setBirthdateIsSet(false);
this.birthdate = 0;
setTimeUserJoinedIsSet(false);
this.timeUserJoined = 0;
}
/**
* A person's email address is also considered their identifying trait.
*/
public String getEmail() {
return this.email;
}
/**
* A person's email address is also considered their identifying trait.
*/
public User setEmail(String email) {
this.email = email;
return this;
}
public void unsetEmail() {
this.email = null;
}
/** Returns true if field email is set (has been assigned a value) and false otherwise */
public boolean isSetEmail() {
return this.email != null;
}
public void setEmailIsSet(boolean value) {
if (!value) {
this.email = null;
}
}
public String getUserId() {
return this.userId;
}
public User setUserId(String userId) {
this.userId = userId;
return this;
}
public void unsetUserId() {
this.userId = null;
}
/** Returns true if field userId is set (has been assigned a value) and false otherwise */
public boolean isSetUserId() {
return this.userId != null;
}
public void setUserIdIsSet(boolean value) {
if (!value) {
this.userId = null;
}
}
public String getName() {
return this.name;
}
public User setName(String name) {
this.name = name;
return this;
}
public void unsetName() {
this.name = null;
}
/** Returns true if field name is set (has been assigned a value) and false otherwise */
public boolean isSetName() {
return this.name != null;
}
public void setNameIsSet(boolean value) {
if (!value) {
this.name = null;
}
}
public int getRolesSize() {
return (this.roles == null) ? 0 : this.roles.size();
}
public java.util.Iterator getRolesIterator() {
return (this.roles == null) ? null : this.roles.iterator();
}
public void addToRoles(Role elem) {
if (this.roles == null) {
this.roles = new HashSet();
}
this.roles.add(elem);
}
/**
* A Person can be more than one thing at once. By default, we assume a developer.
*/
public Set getRoles() {
return this.roles;
}
/**
* A Person can be more than one thing at once. By default, we assume a developer.
*/
public User setRoles(Set roles) {
this.roles = roles;
return this;
}
public void unsetRoles() {
this.roles = null;
}
/** Returns true if field roles is set (has been assigned a value) and false otherwise */
public boolean isSetRoles() {
return this.roles != null;
}
public void setRolesIsSet(boolean value) {
if (!value) {
this.roles = null;
}
}
public Image getProfileImage() {
return this.profileImage;
}
public User setProfileImage(Image profileImage) {
this.profileImage = profileImage;
return this;
}
public void unsetProfileImage() {
this.profileImage = null;
}
/** Returns true if field profileImage is set (has been assigned a value) and false otherwise */
public boolean isSetProfileImage() {
return this.profileImage != null;
}
public void setProfileImageIsSet(boolean value) {
if (!value) {
this.profileImage = null;
}
}
public String getProfileImageLink() {
return this.profileImageLink;
}
public User setProfileImageLink(String profileImageLink) {
this.profileImageLink = profileImageLink;
return this;
}
public void unsetProfileImageLink() {
this.profileImageLink = null;
}
/** Returns true if field profileImageLink is set (has been assigned a value) and false otherwise */
public boolean isSetProfileImageLink() {
return this.profileImageLink != null;
}
public void setProfileImageLinkIsSet(boolean value) {
if (!value) {
this.profileImageLink = null;
}
}
public String getGithubProfile() {
return this.githubProfile;
}
public User setGithubProfile(String githubProfile) {
this.githubProfile = githubProfile;
return this;
}
public void unsetGithubProfile() {
this.githubProfile = null;
}
/** Returns true if field githubProfile is set (has been assigned a value) and false otherwise */
public boolean isSetGithubProfile() {
return this.githubProfile != null;
}
public void setGithubProfileIsSet(boolean value) {
if (!value) {
this.githubProfile = null;
}
}
public String getFirstName() {
return this.firstName;
}
public User setFirstName(String firstName) {
this.firstName = firstName;
return this;
}
public void unsetFirstName() {
this.firstName = null;
}
/** Returns true if field firstName is set (has been assigned a value) and false otherwise */
public boolean isSetFirstName() {
return this.firstName != null;
}
public void setFirstNameIsSet(boolean value) {
if (!value) {
this.firstName = null;
}
}
public String getMiddleName() {
return this.middleName;
}
public User setMiddleName(String middleName) {
this.middleName = middleName;
return this;
}
public void unsetMiddleName() {
this.middleName = null;
}
/** Returns true if field middleName is set (has been assigned a value) and false otherwise */
public boolean isSetMiddleName() {
return this.middleName != null;
}
public void setMiddleNameIsSet(boolean value) {
if (!value) {
this.middleName = null;
}
}
public String getLastName() {
return this.lastName;
}
public User setLastName(String lastName) {
this.lastName = lastName;
return this;
}
public void unsetLastName() {
this.lastName = null;
}
/** Returns true if field lastName is set (has been assigned a value) and false otherwise */
public boolean isSetLastName() {
return this.lastName != null;
}
public void setLastNameIsSet(boolean value) {
if (!value) {
this.lastName = null;
}
}
public long getBirthdate() {
return this.birthdate;
}
public User setBirthdate(long birthdate) {
this.birthdate = birthdate;
setBirthdateIsSet(true);
return this;
}
public void unsetBirthdate() {
__isset_bitfield = EncodingUtils.clearBit(__isset_bitfield, __BIRTHDATE_ISSET_ID);
}
/** Returns true if field birthdate is set (has been assigned a value) and false otherwise */
public boolean isSetBirthdate() {
return EncodingUtils.testBit(__isset_bitfield, __BIRTHDATE_ISSET_ID);
}
public void setBirthdateIsSet(boolean value) {
__isset_bitfield = EncodingUtils.setBit(__isset_bitfield, __BIRTHDATE_ISSET_ID, value);
}
public long getTimeUserJoined() {
return this.timeUserJoined;
}
public User setTimeUserJoined(long timeUserJoined) {
this.timeUserJoined = timeUserJoined;
setTimeUserJoinedIsSet(true);
return this;
}
public void unsetTimeUserJoined() {
__isset_bitfield = EncodingUtils.clearBit(__isset_bitfield, __TIMEUSERJOINED_ISSET_ID);
}
/** Returns true if field timeUserJoined is set (has been assigned a value) and false otherwise */
public boolean isSetTimeUserJoined() {
return EncodingUtils.testBit(__isset_bitfield, __TIMEUSERJOINED_ISSET_ID);
}
public void setTimeUserJoinedIsSet(boolean value) {
__isset_bitfield = EncodingUtils.setBit(__isset_bitfield, __TIMEUSERJOINED_ISSET_ID, value);
}
public void setFieldValue(_Fields field, Object value) {
switch (field) {
case EMAIL:
if (value == null) {
unsetEmail();
} else {
setEmail((String)value);
}
break;
case USER_ID:
if (value == null) {
unsetUserId();
} else {
setUserId((String)value);
}
break;
case NAME:
if (value == null) {
unsetName();
} else {
setName((String)value);
}
break;
case ROLES:
if (value == null) {
unsetRoles();
} else {
setRoles((Set)value);
}
break;
case PROFILE_IMAGE:
if (value == null) {
unsetProfileImage();
} else {
setProfileImage((Image)value);
}
break;
case PROFILE_IMAGE_LINK:
if (value == null) {
unsetProfileImageLink();
} else {
setProfileImageLink((String)value);
}
break;
case GITHUB_PROFILE:
if (value == null) {
unsetGithubProfile();
} else {
setGithubProfile((String)value);
}
break;
case FIRST_NAME:
if (value == null) {
unsetFirstName();
} else {
setFirstName((String)value);
}
break;
case MIDDLE_NAME:
if (value == null) {
unsetMiddleName();
} else {
setMiddleName((String)value);
}
break;
case LAST_NAME:
if (value == null) {
unsetLastName();
} else {
setLastName((String)value);
}
break;
case BIRTHDATE:
if (value == null) {
unsetBirthdate();
} else {
setBirthdate((Long)value);
}
break;
case TIME_USER_JOINED:
if (value == null) {
unsetTimeUserJoined();
} else {
setTimeUserJoined((Long)value);
}
break;
}
}
public Object getFieldValue(_Fields field) {
switch (field) {
case EMAIL:
return getEmail();
case USER_ID:
return getUserId();
case NAME:
return getName();
case ROLES:
return getRoles();
case PROFILE_IMAGE:
return getProfileImage();
case PROFILE_IMAGE_LINK:
return getProfileImageLink();
case GITHUB_PROFILE:
return getGithubProfile();
case FIRST_NAME:
return getFirstName();
case MIDDLE_NAME:
return getMiddleName();
case LAST_NAME:
return getLastName();
case BIRTHDATE:
return getBirthdate();
case TIME_USER_JOINED:
return getTimeUserJoined();
}
throw new IllegalStateException();
}
/** Returns true if field corresponding to fieldID is set (has been assigned a value) and false otherwise */
public boolean isSet(_Fields field) {
if (field == null) {
throw new IllegalArgumentException();
}
switch (field) {
case EMAIL:
return isSetEmail();
case USER_ID:
return isSetUserId();
case NAME:
return isSetName();
case ROLES:
return isSetRoles();
case PROFILE_IMAGE:
return isSetProfileImage();
case PROFILE_IMAGE_LINK:
return isSetProfileImageLink();
case GITHUB_PROFILE:
return isSetGithubProfile();
case FIRST_NAME:
return isSetFirstName();
case MIDDLE_NAME:
return isSetMiddleName();
case LAST_NAME:
return isSetLastName();
case BIRTHDATE:
return isSetBirthdate();
case TIME_USER_JOINED:
return isSetTimeUserJoined();
}
throw new IllegalStateException();
}
@Override
public boolean equals(Object that) {
if (that == null)
return false;
if (that instanceof User)
return this.equals((User)that);
return false;
}
public boolean equals(User that) {
if (that == null)
return false;
boolean this_present_email = true && this.isSetEmail();
boolean that_present_email = true && that.isSetEmail();
if (this_present_email || that_present_email) {
if (!(this_present_email && that_present_email))
return false;
if (!this.email.equals(that.email))
return false;
}
boolean this_present_userId = true && this.isSetUserId();
boolean that_present_userId = true && that.isSetUserId();
if (this_present_userId || that_present_userId) {
if (!(this_present_userId && that_present_userId))
return false;
if (!this.userId.equals(that.userId))
return false;
}
boolean this_present_name = true && this.isSetName();
boolean that_present_name = true && that.isSetName();
if (this_present_name || that_present_name) {
if (!(this_present_name && that_present_name))
return false;
if (!this.name.equals(that.name))
return false;
}
boolean this_present_roles = true && this.isSetRoles();
boolean that_present_roles = true && that.isSetRoles();
if (this_present_roles || that_present_roles) {
if (!(this_present_roles && that_present_roles))
return false;
if (!this.roles.equals(that.roles))
return false;
}
boolean this_present_profileImage = true && this.isSetProfileImage();
boolean that_present_profileImage = true && that.isSetProfileImage();
if (this_present_profileImage || that_present_profileImage) {
if (!(this_present_profileImage && that_present_profileImage))
return false;
if (!this.profileImage.equals(that.profileImage))
return false;
}
boolean this_present_profileImageLink = true && this.isSetProfileImageLink();
boolean that_present_profileImageLink = true && that.isSetProfileImageLink();
if (this_present_profileImageLink || that_present_profileImageLink) {
if (!(this_present_profileImageLink && that_present_profileImageLink))
return false;
if (!this.profileImageLink.equals(that.profileImageLink))
return false;
}
boolean this_present_githubProfile = true && this.isSetGithubProfile();
boolean that_present_githubProfile = true && that.isSetGithubProfile();
if (this_present_githubProfile || that_present_githubProfile) {
if (!(this_present_githubProfile && that_present_githubProfile))
return false;
if (!this.githubProfile.equals(that.githubProfile))
return false;
}
boolean this_present_firstName = true && this.isSetFirstName();
boolean that_present_firstName = true && that.isSetFirstName();
if (this_present_firstName || that_present_firstName) {
if (!(this_present_firstName && that_present_firstName))
return false;
if (!this.firstName.equals(that.firstName))
return false;
}
boolean this_present_middleName = true && this.isSetMiddleName();
boolean that_present_middleName = true && that.isSetMiddleName();
if (this_present_middleName || that_present_middleName) {
if (!(this_present_middleName && that_present_middleName))
return false;
if (!this.middleName.equals(that.middleName))
return false;
}
boolean this_present_lastName = true && this.isSetLastName();
boolean that_present_lastName = true && that.isSetLastName();
if (this_present_lastName || that_present_lastName) {
if (!(this_present_lastName && that_present_lastName))
return false;
if (!this.lastName.equals(that.lastName))
return false;
}
boolean this_present_birthdate = true && this.isSetBirthdate();
boolean that_present_birthdate = true && that.isSetBirthdate();
if (this_present_birthdate || that_present_birthdate) {
if (!(this_present_birthdate && that_present_birthdate))
return false;
if (this.birthdate != that.birthdate)
return false;
}
boolean this_present_timeUserJoined = true && this.isSetTimeUserJoined();
boolean that_present_timeUserJoined = true && that.isSetTimeUserJoined();
if (this_present_timeUserJoined || that_present_timeUserJoined) {
if (!(this_present_timeUserJoined && that_present_timeUserJoined))
return false;
if (this.timeUserJoined != that.timeUserJoined)
return false;
}
return true;
}
@Override
public int hashCode() {
List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy