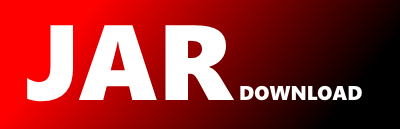
tech.aroma.thrift.authentication.AromaAccount Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aroma-thrift Show documentation
Show all versions of aroma-thrift Show documentation
Part of the Aroma Project.
This project contains the Service and Model Definitions.
From this the Server and Client interfaces are generated for the Aroma Service.
/**
* Autogenerated by Thrift Compiler (0.9.3)
*
* DO NOT EDIT UNLESS YOU ARE SURE THAT YOU KNOW WHAT YOU ARE DOING
* @generated
*/
package tech.aroma.thrift.authentication;
import org.apache.thrift.scheme.IScheme;
import org.apache.thrift.scheme.SchemeFactory;
import org.apache.thrift.scheme.StandardScheme;
import org.apache.thrift.scheme.TupleScheme;
import org.apache.thrift.protocol.TTupleProtocol;
import org.apache.thrift.protocol.TProtocolException;
import org.apache.thrift.EncodingUtils;
import org.apache.thrift.TException;
import org.apache.thrift.async.AsyncMethodCallback;
import org.apache.thrift.server.AbstractNonblockingServer.*;
import java.util.List;
import java.util.ArrayList;
import java.util.Map;
import java.util.HashMap;
import java.util.EnumMap;
import java.util.Set;
import java.util.HashSet;
import java.util.EnumSet;
import java.util.Collections;
import java.util.BitSet;
import java.nio.ByteBuffer;
import java.util.Arrays;
import javax.annotation.Generated;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
@SuppressWarnings({"cast", "rawtypes", "serial", "unchecked"})
/**
* An Account registered with our System.
*/
@Generated(value = "Autogenerated by Thrift Compiler (0.9.3)", date = "2016-05-10")
public class AromaAccount implements org.apache.thrift.TBase, java.io.Serializable, Cloneable, Comparable {
private static final org.apache.thrift.protocol.TStruct STRUCT_DESC = new org.apache.thrift.protocol.TStruct("AromaAccount");
private static final org.apache.thrift.protocol.TField EMAIL_FIELD_DESC = new org.apache.thrift.protocol.TField("email", org.apache.thrift.protocol.TType.STRING, (short)1);
private static final org.apache.thrift.protocol.TField PASSWORD_FIELD_DESC = new org.apache.thrift.protocol.TField("password", org.apache.thrift.protocol.TType.STRUCT, (short)2);
private static final org.apache.thrift.protocol.TField NAME_FIELD_DESC = new org.apache.thrift.protocol.TField("name", org.apache.thrift.protocol.TType.STRING, (short)3);
private static final org.apache.thrift.protocol.TField PROFILE_IMAGE_FIELD_DESC = new org.apache.thrift.protocol.TField("profileImage", org.apache.thrift.protocol.TType.STRUCT, (short)4);
private static final org.apache.thrift.protocol.TField ROLE_FIELD_DESC = new org.apache.thrift.protocol.TField("role", org.apache.thrift.protocol.TType.I32, (short)5);
private static final Map, SchemeFactory> schemes = new HashMap, SchemeFactory>();
static {
schemes.put(StandardScheme.class, new AromaAccountStandardSchemeFactory());
schemes.put(TupleScheme.class, new AromaAccountTupleSchemeFactory());
}
public String email; // required
public Password password; // required
public String name; // required
public tech.aroma.thrift.Image profileImage; // required
/**
*
* @see tech.aroma.thrift.Role
*/
public tech.aroma.thrift.Role role; // optional
/** The set of fields this struct contains, along with convenience methods for finding and manipulating them. */
public enum _Fields implements org.apache.thrift.TFieldIdEnum {
EMAIL((short)1, "email"),
PASSWORD((short)2, "password"),
NAME((short)3, "name"),
PROFILE_IMAGE((short)4, "profileImage"),
/**
*
* @see tech.aroma.thrift.Role
*/
ROLE((short)5, "role");
private static final Map byName = new HashMap();
static {
for (_Fields field : EnumSet.allOf(_Fields.class)) {
byName.put(field.getFieldName(), field);
}
}
/**
* Find the _Fields constant that matches fieldId, or null if its not found.
*/
public static _Fields findByThriftId(int fieldId) {
switch(fieldId) {
case 1: // EMAIL
return EMAIL;
case 2: // PASSWORD
return PASSWORD;
case 3: // NAME
return NAME;
case 4: // PROFILE_IMAGE
return PROFILE_IMAGE;
case 5: // ROLE
return ROLE;
default:
return null;
}
}
/**
* Find the _Fields constant that matches fieldId, throwing an exception
* if it is not found.
*/
public static _Fields findByThriftIdOrThrow(int fieldId) {
_Fields fields = findByThriftId(fieldId);
if (fields == null) throw new IllegalArgumentException("Field " + fieldId + " doesn't exist!");
return fields;
}
/**
* Find the _Fields constant that matches name, or null if its not found.
*/
public static _Fields findByName(String name) {
return byName.get(name);
}
private final short _thriftId;
private final String _fieldName;
_Fields(short thriftId, String fieldName) {
_thriftId = thriftId;
_fieldName = fieldName;
}
public short getThriftFieldId() {
return _thriftId;
}
public String getFieldName() {
return _fieldName;
}
}
// isset id assignments
private static final _Fields optionals[] = {_Fields.ROLE};
public static final Map<_Fields, org.apache.thrift.meta_data.FieldMetaData> metaDataMap;
static {
Map<_Fields, org.apache.thrift.meta_data.FieldMetaData> tmpMap = new EnumMap<_Fields, org.apache.thrift.meta_data.FieldMetaData>(_Fields.class);
tmpMap.put(_Fields.EMAIL, new org.apache.thrift.meta_data.FieldMetaData("email", org.apache.thrift.TFieldRequirementType.DEFAULT,
new org.apache.thrift.meta_data.FieldValueMetaData(org.apache.thrift.protocol.TType.STRING)));
tmpMap.put(_Fields.PASSWORD, new org.apache.thrift.meta_data.FieldMetaData("password", org.apache.thrift.TFieldRequirementType.DEFAULT,
new org.apache.thrift.meta_data.StructMetaData(org.apache.thrift.protocol.TType.STRUCT, Password.class)));
tmpMap.put(_Fields.NAME, new org.apache.thrift.meta_data.FieldMetaData("name", org.apache.thrift.TFieldRequirementType.DEFAULT,
new org.apache.thrift.meta_data.FieldValueMetaData(org.apache.thrift.protocol.TType.STRING)));
tmpMap.put(_Fields.PROFILE_IMAGE, new org.apache.thrift.meta_data.FieldMetaData("profileImage", org.apache.thrift.TFieldRequirementType.DEFAULT,
new org.apache.thrift.meta_data.StructMetaData(org.apache.thrift.protocol.TType.STRUCT, tech.aroma.thrift.Image.class)));
tmpMap.put(_Fields.ROLE, new org.apache.thrift.meta_data.FieldMetaData("role", org.apache.thrift.TFieldRequirementType.OPTIONAL,
new org.apache.thrift.meta_data.EnumMetaData(org.apache.thrift.protocol.TType.ENUM, tech.aroma.thrift.Role.class)));
metaDataMap = Collections.unmodifiableMap(tmpMap);
org.apache.thrift.meta_data.FieldMetaData.addStructMetaDataMap(AromaAccount.class, metaDataMap);
}
public AromaAccount() {
}
public AromaAccount(
String email,
Password password,
String name,
tech.aroma.thrift.Image profileImage)
{
this();
this.email = email;
this.password = password;
this.name = name;
this.profileImage = profileImage;
}
/**
* Performs a deep copy on other.
*/
public AromaAccount(AromaAccount other) {
if (other.isSetEmail()) {
this.email = other.email;
}
if (other.isSetPassword()) {
this.password = new Password(other.password);
}
if (other.isSetName()) {
this.name = other.name;
}
if (other.isSetProfileImage()) {
this.profileImage = new tech.aroma.thrift.Image(other.profileImage);
}
if (other.isSetRole()) {
this.role = other.role;
}
}
public AromaAccount deepCopy() {
return new AromaAccount(this);
}
@Override
public void clear() {
this.email = null;
this.password = null;
this.name = null;
this.profileImage = null;
this.role = null;
}
public String getEmail() {
return this.email;
}
public AromaAccount setEmail(String email) {
this.email = email;
return this;
}
public void unsetEmail() {
this.email = null;
}
/** Returns true if field email is set (has been assigned a value) and false otherwise */
public boolean isSetEmail() {
return this.email != null;
}
public void setEmailIsSet(boolean value) {
if (!value) {
this.email = null;
}
}
public Password getPassword() {
return this.password;
}
public AromaAccount setPassword(Password password) {
this.password = password;
return this;
}
public void unsetPassword() {
this.password = null;
}
/** Returns true if field password is set (has been assigned a value) and false otherwise */
public boolean isSetPassword() {
return this.password != null;
}
public void setPasswordIsSet(boolean value) {
if (!value) {
this.password = null;
}
}
public String getName() {
return this.name;
}
public AromaAccount setName(String name) {
this.name = name;
return this;
}
public void unsetName() {
this.name = null;
}
/** Returns true if field name is set (has been assigned a value) and false otherwise */
public boolean isSetName() {
return this.name != null;
}
public void setNameIsSet(boolean value) {
if (!value) {
this.name = null;
}
}
public tech.aroma.thrift.Image getProfileImage() {
return this.profileImage;
}
public AromaAccount setProfileImage(tech.aroma.thrift.Image profileImage) {
this.profileImage = profileImage;
return this;
}
public void unsetProfileImage() {
this.profileImage = null;
}
/** Returns true if field profileImage is set (has been assigned a value) and false otherwise */
public boolean isSetProfileImage() {
return this.profileImage != null;
}
public void setProfileImageIsSet(boolean value) {
if (!value) {
this.profileImage = null;
}
}
/**
*
* @see tech.aroma.thrift.Role
*/
public tech.aroma.thrift.Role getRole() {
return this.role;
}
/**
*
* @see tech.aroma.thrift.Role
*/
public AromaAccount setRole(tech.aroma.thrift.Role role) {
this.role = role;
return this;
}
public void unsetRole() {
this.role = null;
}
/** Returns true if field role is set (has been assigned a value) and false otherwise */
public boolean isSetRole() {
return this.role != null;
}
public void setRoleIsSet(boolean value) {
if (!value) {
this.role = null;
}
}
public void setFieldValue(_Fields field, Object value) {
switch (field) {
case EMAIL:
if (value == null) {
unsetEmail();
} else {
setEmail((String)value);
}
break;
case PASSWORD:
if (value == null) {
unsetPassword();
} else {
setPassword((Password)value);
}
break;
case NAME:
if (value == null) {
unsetName();
} else {
setName((String)value);
}
break;
case PROFILE_IMAGE:
if (value == null) {
unsetProfileImage();
} else {
setProfileImage((tech.aroma.thrift.Image)value);
}
break;
case ROLE:
if (value == null) {
unsetRole();
} else {
setRole((tech.aroma.thrift.Role)value);
}
break;
}
}
public Object getFieldValue(_Fields field) {
switch (field) {
case EMAIL:
return getEmail();
case PASSWORD:
return getPassword();
case NAME:
return getName();
case PROFILE_IMAGE:
return getProfileImage();
case ROLE:
return getRole();
}
throw new IllegalStateException();
}
/** Returns true if field corresponding to fieldID is set (has been assigned a value) and false otherwise */
public boolean isSet(_Fields field) {
if (field == null) {
throw new IllegalArgumentException();
}
switch (field) {
case EMAIL:
return isSetEmail();
case PASSWORD:
return isSetPassword();
case NAME:
return isSetName();
case PROFILE_IMAGE:
return isSetProfileImage();
case ROLE:
return isSetRole();
}
throw new IllegalStateException();
}
@Override
public boolean equals(Object that) {
if (that == null)
return false;
if (that instanceof AromaAccount)
return this.equals((AromaAccount)that);
return false;
}
public boolean equals(AromaAccount that) {
if (that == null)
return false;
boolean this_present_email = true && this.isSetEmail();
boolean that_present_email = true && that.isSetEmail();
if (this_present_email || that_present_email) {
if (!(this_present_email && that_present_email))
return false;
if (!this.email.equals(that.email))
return false;
}
boolean this_present_password = true && this.isSetPassword();
boolean that_present_password = true && that.isSetPassword();
if (this_present_password || that_present_password) {
if (!(this_present_password && that_present_password))
return false;
if (!this.password.equals(that.password))
return false;
}
boolean this_present_name = true && this.isSetName();
boolean that_present_name = true && that.isSetName();
if (this_present_name || that_present_name) {
if (!(this_present_name && that_present_name))
return false;
if (!this.name.equals(that.name))
return false;
}
boolean this_present_profileImage = true && this.isSetProfileImage();
boolean that_present_profileImage = true && that.isSetProfileImage();
if (this_present_profileImage || that_present_profileImage) {
if (!(this_present_profileImage && that_present_profileImage))
return false;
if (!this.profileImage.equals(that.profileImage))
return false;
}
boolean this_present_role = true && this.isSetRole();
boolean that_present_role = true && that.isSetRole();
if (this_present_role || that_present_role) {
if (!(this_present_role && that_present_role))
return false;
if (!this.role.equals(that.role))
return false;
}
return true;
}
@Override
public int hashCode() {
List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy