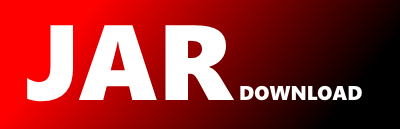
tech.aroma.thrift.authentication.GithubToken Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aroma-thrift Show documentation
Show all versions of aroma-thrift Show documentation
Part of the Aroma Project.
This project contains the Service and Model Definitions.
From this the Server and Client interfaces are generated for the Aroma Service.
/**
* Autogenerated by Thrift Compiler (0.9.3)
*
* DO NOT EDIT UNLESS YOU ARE SURE THAT YOU KNOW WHAT YOU ARE DOING
* @generated
*/
package tech.aroma.thrift.authentication;
import org.apache.thrift.scheme.IScheme;
import org.apache.thrift.scheme.SchemeFactory;
import org.apache.thrift.scheme.StandardScheme;
import org.apache.thrift.scheme.TupleScheme;
import org.apache.thrift.protocol.TTupleProtocol;
import org.apache.thrift.protocol.TProtocolException;
import org.apache.thrift.EncodingUtils;
import org.apache.thrift.TException;
import org.apache.thrift.async.AsyncMethodCallback;
import org.apache.thrift.server.AbstractNonblockingServer.*;
import java.util.List;
import java.util.ArrayList;
import java.util.Map;
import java.util.HashMap;
import java.util.EnumMap;
import java.util.Set;
import java.util.HashSet;
import java.util.EnumSet;
import java.util.Collections;
import java.util.BitSet;
import java.nio.ByteBuffer;
import java.util.Arrays;
import javax.annotation.Generated;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
@SuppressWarnings({"cast", "rawtypes", "serial", "unchecked"})
@Generated(value = "Autogenerated by Thrift Compiler (0.9.3)", date = "2016-05-10")
public class GithubToken implements org.apache.thrift.TBase, java.io.Serializable, Cloneable, Comparable {
private static final org.apache.thrift.protocol.TStruct STRUCT_DESC = new org.apache.thrift.protocol.TStruct("GithubToken");
private static final org.apache.thrift.protocol.TField USERNAME_FIELD_DESC = new org.apache.thrift.protocol.TField("username", org.apache.thrift.protocol.TType.STRING, (short)1);
private static final org.apache.thrift.protocol.TField EMAIL_FIELD_DESC = new org.apache.thrift.protocol.TField("email", org.apache.thrift.protocol.TType.STRING, (short)2);
private static final org.apache.thrift.protocol.TField OAUTH_TOKEN_FIELD_DESC = new org.apache.thrift.protocol.TField("oauthToken", org.apache.thrift.protocol.TType.STRING, (short)3);
private static final Map, SchemeFactory> schemes = new HashMap, SchemeFactory>();
static {
schemes.put(StandardScheme.class, new GithubTokenStandardSchemeFactory());
schemes.put(TupleScheme.class, new GithubTokenTupleSchemeFactory());
}
public String username; // required
public String email; // required
public String oauthToken; // required
/** The set of fields this struct contains, along with convenience methods for finding and manipulating them. */
public enum _Fields implements org.apache.thrift.TFieldIdEnum {
USERNAME((short)1, "username"),
EMAIL((short)2, "email"),
OAUTH_TOKEN((short)3, "oauthToken");
private static final Map byName = new HashMap();
static {
for (_Fields field : EnumSet.allOf(_Fields.class)) {
byName.put(field.getFieldName(), field);
}
}
/**
* Find the _Fields constant that matches fieldId, or null if its not found.
*/
public static _Fields findByThriftId(int fieldId) {
switch(fieldId) {
case 1: // USERNAME
return USERNAME;
case 2: // EMAIL
return EMAIL;
case 3: // OAUTH_TOKEN
return OAUTH_TOKEN;
default:
return null;
}
}
/**
* Find the _Fields constant that matches fieldId, throwing an exception
* if it is not found.
*/
public static _Fields findByThriftIdOrThrow(int fieldId) {
_Fields fields = findByThriftId(fieldId);
if (fields == null) throw new IllegalArgumentException("Field " + fieldId + " doesn't exist!");
return fields;
}
/**
* Find the _Fields constant that matches name, or null if its not found.
*/
public static _Fields findByName(String name) {
return byName.get(name);
}
private final short _thriftId;
private final String _fieldName;
_Fields(short thriftId, String fieldName) {
_thriftId = thriftId;
_fieldName = fieldName;
}
public short getThriftFieldId() {
return _thriftId;
}
public String getFieldName() {
return _fieldName;
}
}
// isset id assignments
public static final Map<_Fields, org.apache.thrift.meta_data.FieldMetaData> metaDataMap;
static {
Map<_Fields, org.apache.thrift.meta_data.FieldMetaData> tmpMap = new EnumMap<_Fields, org.apache.thrift.meta_data.FieldMetaData>(_Fields.class);
tmpMap.put(_Fields.USERNAME, new org.apache.thrift.meta_data.FieldMetaData("username", org.apache.thrift.TFieldRequirementType.DEFAULT,
new org.apache.thrift.meta_data.FieldValueMetaData(org.apache.thrift.protocol.TType.STRING)));
tmpMap.put(_Fields.EMAIL, new org.apache.thrift.meta_data.FieldMetaData("email", org.apache.thrift.TFieldRequirementType.DEFAULT,
new org.apache.thrift.meta_data.FieldValueMetaData(org.apache.thrift.protocol.TType.STRING)));
tmpMap.put(_Fields.OAUTH_TOKEN, new org.apache.thrift.meta_data.FieldMetaData("oauthToken", org.apache.thrift.TFieldRequirementType.REQUIRED,
new org.apache.thrift.meta_data.FieldValueMetaData(org.apache.thrift.protocol.TType.STRING)));
metaDataMap = Collections.unmodifiableMap(tmpMap);
org.apache.thrift.meta_data.FieldMetaData.addStructMetaDataMap(GithubToken.class, metaDataMap);
}
public GithubToken() {
}
public GithubToken(
String username,
String email,
String oauthToken)
{
this();
this.username = username;
this.email = email;
this.oauthToken = oauthToken;
}
/**
* Performs a deep copy on other.
*/
public GithubToken(GithubToken other) {
if (other.isSetUsername()) {
this.username = other.username;
}
if (other.isSetEmail()) {
this.email = other.email;
}
if (other.isSetOauthToken()) {
this.oauthToken = other.oauthToken;
}
}
public GithubToken deepCopy() {
return new GithubToken(this);
}
@Override
public void clear() {
this.username = null;
this.email = null;
this.oauthToken = null;
}
public String getUsername() {
return this.username;
}
public GithubToken setUsername(String username) {
this.username = username;
return this;
}
public void unsetUsername() {
this.username = null;
}
/** Returns true if field username is set (has been assigned a value) and false otherwise */
public boolean isSetUsername() {
return this.username != null;
}
public void setUsernameIsSet(boolean value) {
if (!value) {
this.username = null;
}
}
public String getEmail() {
return this.email;
}
public GithubToken setEmail(String email) {
this.email = email;
return this;
}
public void unsetEmail() {
this.email = null;
}
/** Returns true if field email is set (has been assigned a value) and false otherwise */
public boolean isSetEmail() {
return this.email != null;
}
public void setEmailIsSet(boolean value) {
if (!value) {
this.email = null;
}
}
public String getOauthToken() {
return this.oauthToken;
}
public GithubToken setOauthToken(String oauthToken) {
this.oauthToken = oauthToken;
return this;
}
public void unsetOauthToken() {
this.oauthToken = null;
}
/** Returns true if field oauthToken is set (has been assigned a value) and false otherwise */
public boolean isSetOauthToken() {
return this.oauthToken != null;
}
public void setOauthTokenIsSet(boolean value) {
if (!value) {
this.oauthToken = null;
}
}
public void setFieldValue(_Fields field, Object value) {
switch (field) {
case USERNAME:
if (value == null) {
unsetUsername();
} else {
setUsername((String)value);
}
break;
case EMAIL:
if (value == null) {
unsetEmail();
} else {
setEmail((String)value);
}
break;
case OAUTH_TOKEN:
if (value == null) {
unsetOauthToken();
} else {
setOauthToken((String)value);
}
break;
}
}
public Object getFieldValue(_Fields field) {
switch (field) {
case USERNAME:
return getUsername();
case EMAIL:
return getEmail();
case OAUTH_TOKEN:
return getOauthToken();
}
throw new IllegalStateException();
}
/** Returns true if field corresponding to fieldID is set (has been assigned a value) and false otherwise */
public boolean isSet(_Fields field) {
if (field == null) {
throw new IllegalArgumentException();
}
switch (field) {
case USERNAME:
return isSetUsername();
case EMAIL:
return isSetEmail();
case OAUTH_TOKEN:
return isSetOauthToken();
}
throw new IllegalStateException();
}
@Override
public boolean equals(Object that) {
if (that == null)
return false;
if (that instanceof GithubToken)
return this.equals((GithubToken)that);
return false;
}
public boolean equals(GithubToken that) {
if (that == null)
return false;
boolean this_present_username = true && this.isSetUsername();
boolean that_present_username = true && that.isSetUsername();
if (this_present_username || that_present_username) {
if (!(this_present_username && that_present_username))
return false;
if (!this.username.equals(that.username))
return false;
}
boolean this_present_email = true && this.isSetEmail();
boolean that_present_email = true && that.isSetEmail();
if (this_present_email || that_present_email) {
if (!(this_present_email && that_present_email))
return false;
if (!this.email.equals(that.email))
return false;
}
boolean this_present_oauthToken = true && this.isSetOauthToken();
boolean that_present_oauthToken = true && that.isSetOauthToken();
if (this_present_oauthToken || that_present_oauthToken) {
if (!(this_present_oauthToken && that_present_oauthToken))
return false;
if (!this.oauthToken.equals(that.oauthToken))
return false;
}
return true;
}
@Override
public int hashCode() {
List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy