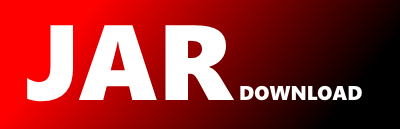
tech.aroma.thrift.authentication.UserToken Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aroma-thrift Show documentation
Show all versions of aroma-thrift Show documentation
Part of the Aroma Project.
This project contains the Service and Model Definitions.
From this the Server and Client interfaces are generated for the Aroma Service.
/**
* Autogenerated by Thrift Compiler (0.9.3)
*
* DO NOT EDIT UNLESS YOU ARE SURE THAT YOU KNOW WHAT YOU ARE DOING
* @generated
*/
package tech.aroma.thrift.authentication;
import org.apache.thrift.scheme.IScheme;
import org.apache.thrift.scheme.SchemeFactory;
import org.apache.thrift.scheme.StandardScheme;
import org.apache.thrift.scheme.TupleScheme;
import org.apache.thrift.protocol.TTupleProtocol;
import org.apache.thrift.protocol.TProtocolException;
import org.apache.thrift.EncodingUtils;
import org.apache.thrift.TException;
import org.apache.thrift.async.AsyncMethodCallback;
import org.apache.thrift.server.AbstractNonblockingServer.*;
import java.util.List;
import java.util.ArrayList;
import java.util.Map;
import java.util.HashMap;
import java.util.EnumMap;
import java.util.Set;
import java.util.HashSet;
import java.util.EnumSet;
import java.util.Collections;
import java.util.BitSet;
import java.nio.ByteBuffer;
import java.util.Arrays;
import javax.annotation.Generated;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
@SuppressWarnings({"cast", "rawtypes", "serial", "unchecked"})
@Generated(value = "Autogenerated by Thrift Compiler (0.9.3)", date = "2016-05-10")
public class UserToken implements org.apache.thrift.TBase, java.io.Serializable, Cloneable, Comparable {
private static final org.apache.thrift.protocol.TStruct STRUCT_DESC = new org.apache.thrift.protocol.TStruct("UserToken");
private static final org.apache.thrift.protocol.TField TOKEN_ID_FIELD_DESC = new org.apache.thrift.protocol.TField("tokenId", org.apache.thrift.protocol.TType.STRING, (short)1);
private static final org.apache.thrift.protocol.TField TIME_OF_EXPIRATION_FIELD_DESC = new org.apache.thrift.protocol.TField("timeOfExpiration", org.apache.thrift.protocol.TType.I64, (short)2);
private static final org.apache.thrift.protocol.TField ORGANIZATION_FIELD_DESC = new org.apache.thrift.protocol.TField("organization", org.apache.thrift.protocol.TType.STRING, (short)3);
private static final org.apache.thrift.protocol.TField IS_OAUTH_TOKEN_FIELD_DESC = new org.apache.thrift.protocol.TField("isOauthToken", org.apache.thrift.protocol.TType.BOOL, (short)4);
private static final org.apache.thrift.protocol.TField OAUTH_PROVIDER_FIELD_DESC = new org.apache.thrift.protocol.TField("oauthProvider", org.apache.thrift.protocol.TType.STRING, (short)5);
private static final org.apache.thrift.protocol.TField USER_ID_FIELD_DESC = new org.apache.thrift.protocol.TField("userId", org.apache.thrift.protocol.TType.STRING, (short)6);
private static final Map, SchemeFactory> schemes = new HashMap, SchemeFactory>();
static {
schemes.put(StandardScheme.class, new UserTokenStandardSchemeFactory());
schemes.put(TupleScheme.class, new UserTokenTupleSchemeFactory());
}
public String tokenId; // required
public long timeOfExpiration; // required
public String organization; // optional
public boolean isOauthToken; // optional
public String oauthProvider; // optional
public String userId; // required
/** The set of fields this struct contains, along with convenience methods for finding and manipulating them. */
public enum _Fields implements org.apache.thrift.TFieldIdEnum {
TOKEN_ID((short)1, "tokenId"),
TIME_OF_EXPIRATION((short)2, "timeOfExpiration"),
ORGANIZATION((short)3, "organization"),
IS_OAUTH_TOKEN((short)4, "isOauthToken"),
OAUTH_PROVIDER((short)5, "oauthProvider"),
USER_ID((short)6, "userId");
private static final Map byName = new HashMap();
static {
for (_Fields field : EnumSet.allOf(_Fields.class)) {
byName.put(field.getFieldName(), field);
}
}
/**
* Find the _Fields constant that matches fieldId, or null if its not found.
*/
public static _Fields findByThriftId(int fieldId) {
switch(fieldId) {
case 1: // TOKEN_ID
return TOKEN_ID;
case 2: // TIME_OF_EXPIRATION
return TIME_OF_EXPIRATION;
case 3: // ORGANIZATION
return ORGANIZATION;
case 4: // IS_OAUTH_TOKEN
return IS_OAUTH_TOKEN;
case 5: // OAUTH_PROVIDER
return OAUTH_PROVIDER;
case 6: // USER_ID
return USER_ID;
default:
return null;
}
}
/**
* Find the _Fields constant that matches fieldId, throwing an exception
* if it is not found.
*/
public static _Fields findByThriftIdOrThrow(int fieldId) {
_Fields fields = findByThriftId(fieldId);
if (fields == null) throw new IllegalArgumentException("Field " + fieldId + " doesn't exist!");
return fields;
}
/**
* Find the _Fields constant that matches name, or null if its not found.
*/
public static _Fields findByName(String name) {
return byName.get(name);
}
private final short _thriftId;
private final String _fieldName;
_Fields(short thriftId, String fieldName) {
_thriftId = thriftId;
_fieldName = fieldName;
}
public short getThriftFieldId() {
return _thriftId;
}
public String getFieldName() {
return _fieldName;
}
}
// isset id assignments
private static final int __TIMEOFEXPIRATION_ISSET_ID = 0;
private static final int __ISOAUTHTOKEN_ISSET_ID = 1;
private byte __isset_bitfield = 0;
private static final _Fields optionals[] = {_Fields.ORGANIZATION,_Fields.IS_OAUTH_TOKEN,_Fields.OAUTH_PROVIDER};
public static final Map<_Fields, org.apache.thrift.meta_data.FieldMetaData> metaDataMap;
static {
Map<_Fields, org.apache.thrift.meta_data.FieldMetaData> tmpMap = new EnumMap<_Fields, org.apache.thrift.meta_data.FieldMetaData>(_Fields.class);
tmpMap.put(_Fields.TOKEN_ID, new org.apache.thrift.meta_data.FieldMetaData("tokenId", org.apache.thrift.TFieldRequirementType.DEFAULT,
new org.apache.thrift.meta_data.FieldValueMetaData(org.apache.thrift.protocol.TType.STRING)));
tmpMap.put(_Fields.TIME_OF_EXPIRATION, new org.apache.thrift.meta_data.FieldMetaData("timeOfExpiration", org.apache.thrift.TFieldRequirementType.DEFAULT,
new org.apache.thrift.meta_data.FieldValueMetaData(org.apache.thrift.protocol.TType.I64 , "timestamp")));
tmpMap.put(_Fields.ORGANIZATION, new org.apache.thrift.meta_data.FieldMetaData("organization", org.apache.thrift.TFieldRequirementType.OPTIONAL,
new org.apache.thrift.meta_data.FieldValueMetaData(org.apache.thrift.protocol.TType.STRING)));
tmpMap.put(_Fields.IS_OAUTH_TOKEN, new org.apache.thrift.meta_data.FieldMetaData("isOauthToken", org.apache.thrift.TFieldRequirementType.OPTIONAL,
new org.apache.thrift.meta_data.FieldValueMetaData(org.apache.thrift.protocol.TType.BOOL)));
tmpMap.put(_Fields.OAUTH_PROVIDER, new org.apache.thrift.meta_data.FieldMetaData("oauthProvider", org.apache.thrift.TFieldRequirementType.OPTIONAL,
new org.apache.thrift.meta_data.FieldValueMetaData(org.apache.thrift.protocol.TType.STRING)));
tmpMap.put(_Fields.USER_ID, new org.apache.thrift.meta_data.FieldMetaData("userId", org.apache.thrift.TFieldRequirementType.DEFAULT,
new org.apache.thrift.meta_data.FieldValueMetaData(org.apache.thrift.protocol.TType.STRING , "uuid")));
metaDataMap = Collections.unmodifiableMap(tmpMap);
org.apache.thrift.meta_data.FieldMetaData.addStructMetaDataMap(UserToken.class, metaDataMap);
}
public UserToken() {
this.isOauthToken = false;
}
public UserToken(
String tokenId,
long timeOfExpiration,
String userId)
{
this();
this.tokenId = tokenId;
this.timeOfExpiration = timeOfExpiration;
setTimeOfExpirationIsSet(true);
this.userId = userId;
}
/**
* Performs a deep copy on other.
*/
public UserToken(UserToken other) {
__isset_bitfield = other.__isset_bitfield;
if (other.isSetTokenId()) {
this.tokenId = other.tokenId;
}
this.timeOfExpiration = other.timeOfExpiration;
if (other.isSetOrganization()) {
this.organization = other.organization;
}
this.isOauthToken = other.isOauthToken;
if (other.isSetOauthProvider()) {
this.oauthProvider = other.oauthProvider;
}
if (other.isSetUserId()) {
this.userId = other.userId;
}
}
public UserToken deepCopy() {
return new UserToken(this);
}
@Override
public void clear() {
this.tokenId = null;
setTimeOfExpirationIsSet(false);
this.timeOfExpiration = 0;
this.organization = null;
this.isOauthToken = false;
this.oauthProvider = null;
this.userId = null;
}
public String getTokenId() {
return this.tokenId;
}
public UserToken setTokenId(String tokenId) {
this.tokenId = tokenId;
return this;
}
public void unsetTokenId() {
this.tokenId = null;
}
/** Returns true if field tokenId is set (has been assigned a value) and false otherwise */
public boolean isSetTokenId() {
return this.tokenId != null;
}
public void setTokenIdIsSet(boolean value) {
if (!value) {
this.tokenId = null;
}
}
public long getTimeOfExpiration() {
return this.timeOfExpiration;
}
public UserToken setTimeOfExpiration(long timeOfExpiration) {
this.timeOfExpiration = timeOfExpiration;
setTimeOfExpirationIsSet(true);
return this;
}
public void unsetTimeOfExpiration() {
__isset_bitfield = EncodingUtils.clearBit(__isset_bitfield, __TIMEOFEXPIRATION_ISSET_ID);
}
/** Returns true if field timeOfExpiration is set (has been assigned a value) and false otherwise */
public boolean isSetTimeOfExpiration() {
return EncodingUtils.testBit(__isset_bitfield, __TIMEOFEXPIRATION_ISSET_ID);
}
public void setTimeOfExpirationIsSet(boolean value) {
__isset_bitfield = EncodingUtils.setBit(__isset_bitfield, __TIMEOFEXPIRATION_ISSET_ID, value);
}
public String getOrganization() {
return this.organization;
}
public UserToken setOrganization(String organization) {
this.organization = organization;
return this;
}
public void unsetOrganization() {
this.organization = null;
}
/** Returns true if field organization is set (has been assigned a value) and false otherwise */
public boolean isSetOrganization() {
return this.organization != null;
}
public void setOrganizationIsSet(boolean value) {
if (!value) {
this.organization = null;
}
}
public boolean isIsOauthToken() {
return this.isOauthToken;
}
public UserToken setIsOauthToken(boolean isOauthToken) {
this.isOauthToken = isOauthToken;
setIsOauthTokenIsSet(true);
return this;
}
public void unsetIsOauthToken() {
__isset_bitfield = EncodingUtils.clearBit(__isset_bitfield, __ISOAUTHTOKEN_ISSET_ID);
}
/** Returns true if field isOauthToken is set (has been assigned a value) and false otherwise */
public boolean isSetIsOauthToken() {
return EncodingUtils.testBit(__isset_bitfield, __ISOAUTHTOKEN_ISSET_ID);
}
public void setIsOauthTokenIsSet(boolean value) {
__isset_bitfield = EncodingUtils.setBit(__isset_bitfield, __ISOAUTHTOKEN_ISSET_ID, value);
}
public String getOauthProvider() {
return this.oauthProvider;
}
public UserToken setOauthProvider(String oauthProvider) {
this.oauthProvider = oauthProvider;
return this;
}
public void unsetOauthProvider() {
this.oauthProvider = null;
}
/** Returns true if field oauthProvider is set (has been assigned a value) and false otherwise */
public boolean isSetOauthProvider() {
return this.oauthProvider != null;
}
public void setOauthProviderIsSet(boolean value) {
if (!value) {
this.oauthProvider = null;
}
}
public String getUserId() {
return this.userId;
}
public UserToken setUserId(String userId) {
this.userId = userId;
return this;
}
public void unsetUserId() {
this.userId = null;
}
/** Returns true if field userId is set (has been assigned a value) and false otherwise */
public boolean isSetUserId() {
return this.userId != null;
}
public void setUserIdIsSet(boolean value) {
if (!value) {
this.userId = null;
}
}
public void setFieldValue(_Fields field, Object value) {
switch (field) {
case TOKEN_ID:
if (value == null) {
unsetTokenId();
} else {
setTokenId((String)value);
}
break;
case TIME_OF_EXPIRATION:
if (value == null) {
unsetTimeOfExpiration();
} else {
setTimeOfExpiration((Long)value);
}
break;
case ORGANIZATION:
if (value == null) {
unsetOrganization();
} else {
setOrganization((String)value);
}
break;
case IS_OAUTH_TOKEN:
if (value == null) {
unsetIsOauthToken();
} else {
setIsOauthToken((Boolean)value);
}
break;
case OAUTH_PROVIDER:
if (value == null) {
unsetOauthProvider();
} else {
setOauthProvider((String)value);
}
break;
case USER_ID:
if (value == null) {
unsetUserId();
} else {
setUserId((String)value);
}
break;
}
}
public Object getFieldValue(_Fields field) {
switch (field) {
case TOKEN_ID:
return getTokenId();
case TIME_OF_EXPIRATION:
return getTimeOfExpiration();
case ORGANIZATION:
return getOrganization();
case IS_OAUTH_TOKEN:
return isIsOauthToken();
case OAUTH_PROVIDER:
return getOauthProvider();
case USER_ID:
return getUserId();
}
throw new IllegalStateException();
}
/** Returns true if field corresponding to fieldID is set (has been assigned a value) and false otherwise */
public boolean isSet(_Fields field) {
if (field == null) {
throw new IllegalArgumentException();
}
switch (field) {
case TOKEN_ID:
return isSetTokenId();
case TIME_OF_EXPIRATION:
return isSetTimeOfExpiration();
case ORGANIZATION:
return isSetOrganization();
case IS_OAUTH_TOKEN:
return isSetIsOauthToken();
case OAUTH_PROVIDER:
return isSetOauthProvider();
case USER_ID:
return isSetUserId();
}
throw new IllegalStateException();
}
@Override
public boolean equals(Object that) {
if (that == null)
return false;
if (that instanceof UserToken)
return this.equals((UserToken)that);
return false;
}
public boolean equals(UserToken that) {
if (that == null)
return false;
boolean this_present_tokenId = true && this.isSetTokenId();
boolean that_present_tokenId = true && that.isSetTokenId();
if (this_present_tokenId || that_present_tokenId) {
if (!(this_present_tokenId && that_present_tokenId))
return false;
if (!this.tokenId.equals(that.tokenId))
return false;
}
boolean this_present_timeOfExpiration = true;
boolean that_present_timeOfExpiration = true;
if (this_present_timeOfExpiration || that_present_timeOfExpiration) {
if (!(this_present_timeOfExpiration && that_present_timeOfExpiration))
return false;
if (this.timeOfExpiration != that.timeOfExpiration)
return false;
}
boolean this_present_organization = true && this.isSetOrganization();
boolean that_present_organization = true && that.isSetOrganization();
if (this_present_organization || that_present_organization) {
if (!(this_present_organization && that_present_organization))
return false;
if (!this.organization.equals(that.organization))
return false;
}
boolean this_present_isOauthToken = true && this.isSetIsOauthToken();
boolean that_present_isOauthToken = true && that.isSetIsOauthToken();
if (this_present_isOauthToken || that_present_isOauthToken) {
if (!(this_present_isOauthToken && that_present_isOauthToken))
return false;
if (this.isOauthToken != that.isOauthToken)
return false;
}
boolean this_present_oauthProvider = true && this.isSetOauthProvider();
boolean that_present_oauthProvider = true && that.isSetOauthProvider();
if (this_present_oauthProvider || that_present_oauthProvider) {
if (!(this_present_oauthProvider && that_present_oauthProvider))
return false;
if (!this.oauthProvider.equals(that.oauthProvider))
return false;
}
boolean this_present_userId = true && this.isSetUserId();
boolean that_present_userId = true && that.isSetUserId();
if (this_present_userId || that_present_userId) {
if (!(this_present_userId && that_present_userId))
return false;
if (!this.userId.equals(that.userId))
return false;
}
return true;
}
@Override
public int hashCode() {
List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy