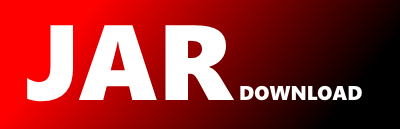
tech.aroma.thrift.authentication.service.CreateTokenRequest Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aroma-thrift Show documentation
Show all versions of aroma-thrift Show documentation
Part of the Aroma Project.
This project contains the Service and Model Definitions.
From this the Server and Client interfaces are generated for the Aroma Service.
/**
* Autogenerated by Thrift Compiler (0.9.3)
*
* DO NOT EDIT UNLESS YOU ARE SURE THAT YOU KNOW WHAT YOU ARE DOING
* @generated
*/
package tech.aroma.thrift.authentication.service;
import org.apache.thrift.scheme.IScheme;
import org.apache.thrift.scheme.SchemeFactory;
import org.apache.thrift.scheme.StandardScheme;
import org.apache.thrift.scheme.TupleScheme;
import org.apache.thrift.protocol.TTupleProtocol;
import org.apache.thrift.protocol.TProtocolException;
import org.apache.thrift.EncodingUtils;
import org.apache.thrift.TException;
import org.apache.thrift.async.AsyncMethodCallback;
import org.apache.thrift.server.AbstractNonblockingServer.*;
import java.util.List;
import java.util.ArrayList;
import java.util.Map;
import java.util.HashMap;
import java.util.EnumMap;
import java.util.Set;
import java.util.HashSet;
import java.util.EnumSet;
import java.util.Collections;
import java.util.BitSet;
import java.nio.ByteBuffer;
import java.util.Arrays;
import javax.annotation.Generated;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
@SuppressWarnings({"cast", "rawtypes", "serial", "unchecked"})
@Generated(value = "Autogenerated by Thrift Compiler (0.9.3)", date = "2016-05-10")
public class CreateTokenRequest implements org.apache.thrift.TBase, java.io.Serializable, Cloneable, Comparable {
private static final org.apache.thrift.protocol.TStruct STRUCT_DESC = new org.apache.thrift.protocol.TStruct("CreateTokenRequest");
private static final org.apache.thrift.protocol.TField OWNER_ID_FIELD_DESC = new org.apache.thrift.protocol.TField("ownerId", org.apache.thrift.protocol.TType.STRING, (short)1);
private static final org.apache.thrift.protocol.TField LIFETIME_FIELD_DESC = new org.apache.thrift.protocol.TField("lifetime", org.apache.thrift.protocol.TType.STRUCT, (short)2);
private static final org.apache.thrift.protocol.TField DESIRED_TOKEN_TYPE_FIELD_DESC = new org.apache.thrift.protocol.TField("desiredTokenType", org.apache.thrift.protocol.TType.I32, (short)3);
private static final org.apache.thrift.protocol.TField OWNER_NAME_FIELD_DESC = new org.apache.thrift.protocol.TField("ownerName", org.apache.thrift.protocol.TType.STRING, (short)4);
private static final org.apache.thrift.protocol.TField ORGANIZATION_ID_FIELD_DESC = new org.apache.thrift.protocol.TField("organizationId", org.apache.thrift.protocol.TType.STRING, (short)5);
private static final org.apache.thrift.protocol.TField ORGANIZATION_NAME_FIELD_DESC = new org.apache.thrift.protocol.TField("organizationName", org.apache.thrift.protocol.TType.STRING, (short)6);
private static final Map, SchemeFactory> schemes = new HashMap, SchemeFactory>();
static {
schemes.put(StandardScheme.class, new CreateTokenRequestStandardSchemeFactory());
schemes.put(TupleScheme.class, new CreateTokenRequestTupleSchemeFactory());
}
/**
* The ID of the entity that will own the token.
* For Application tokens, this is the Application ID.
* For User Tokens, this is the userId.
*/
public String ownerId; // required
/**
* The desired length of time to keep the Token alive and valid.
* If not present, will be set to DEFAULT_TOKEN_LIFETIME.
*/
public tech.aroma.thrift.LengthOfTime lifetime; // optional
/**
* This is required, and determines the kind of Token created.
*/
public tech.aroma.thrift.authentication.TokenType desiredTokenType; // required
/**
* Optional stores the name of the entity owning the token, for instance App name or user's email.
*/
public String ownerName; // optional
public String organizationId; // optional
public String organizationName; // optional
/** The set of fields this struct contains, along with convenience methods for finding and manipulating them. */
public enum _Fields implements org.apache.thrift.TFieldIdEnum {
/**
* The ID of the entity that will own the token.
* For Application tokens, this is the Application ID.
* For User Tokens, this is the userId.
*/
OWNER_ID((short)1, "ownerId"),
/**
* The desired length of time to keep the Token alive and valid.
* If not present, will be set to DEFAULT_TOKEN_LIFETIME.
*/
LIFETIME((short)2, "lifetime"),
/**
* This is required, and determines the kind of Token created.
*/
DESIRED_TOKEN_TYPE((short)3, "desiredTokenType"),
/**
* Optional stores the name of the entity owning the token, for instance App name or user's email.
*/
OWNER_NAME((short)4, "ownerName"),
ORGANIZATION_ID((short)5, "organizationId"),
ORGANIZATION_NAME((short)6, "organizationName");
private static final Map byName = new HashMap();
static {
for (_Fields field : EnumSet.allOf(_Fields.class)) {
byName.put(field.getFieldName(), field);
}
}
/**
* Find the _Fields constant that matches fieldId, or null if its not found.
*/
public static _Fields findByThriftId(int fieldId) {
switch(fieldId) {
case 1: // OWNER_ID
return OWNER_ID;
case 2: // LIFETIME
return LIFETIME;
case 3: // DESIRED_TOKEN_TYPE
return DESIRED_TOKEN_TYPE;
case 4: // OWNER_NAME
return OWNER_NAME;
case 5: // ORGANIZATION_ID
return ORGANIZATION_ID;
case 6: // ORGANIZATION_NAME
return ORGANIZATION_NAME;
default:
return null;
}
}
/**
* Find the _Fields constant that matches fieldId, throwing an exception
* if it is not found.
*/
public static _Fields findByThriftIdOrThrow(int fieldId) {
_Fields fields = findByThriftId(fieldId);
if (fields == null) throw new IllegalArgumentException("Field " + fieldId + " doesn't exist!");
return fields;
}
/**
* Find the _Fields constant that matches name, or null if its not found.
*/
public static _Fields findByName(String name) {
return byName.get(name);
}
private final short _thriftId;
private final String _fieldName;
_Fields(short thriftId, String fieldName) {
_thriftId = thriftId;
_fieldName = fieldName;
}
public short getThriftFieldId() {
return _thriftId;
}
public String getFieldName() {
return _fieldName;
}
}
// isset id assignments
private static final _Fields optionals[] = {_Fields.LIFETIME,_Fields.OWNER_NAME,_Fields.ORGANIZATION_ID,_Fields.ORGANIZATION_NAME};
public static final Map<_Fields, org.apache.thrift.meta_data.FieldMetaData> metaDataMap;
static {
Map<_Fields, org.apache.thrift.meta_data.FieldMetaData> tmpMap = new EnumMap<_Fields, org.apache.thrift.meta_data.FieldMetaData>(_Fields.class);
tmpMap.put(_Fields.OWNER_ID, new org.apache.thrift.meta_data.FieldMetaData("ownerId", org.apache.thrift.TFieldRequirementType.DEFAULT,
new org.apache.thrift.meta_data.FieldValueMetaData(org.apache.thrift.protocol.TType.STRING , "uuid")));
tmpMap.put(_Fields.LIFETIME, new org.apache.thrift.meta_data.FieldMetaData("lifetime", org.apache.thrift.TFieldRequirementType.OPTIONAL,
new org.apache.thrift.meta_data.FieldValueMetaData(org.apache.thrift.protocol.TType.STRUCT , "LengthOfTime")));
tmpMap.put(_Fields.DESIRED_TOKEN_TYPE, new org.apache.thrift.meta_data.FieldMetaData("desiredTokenType", org.apache.thrift.TFieldRequirementType.DEFAULT,
new org.apache.thrift.meta_data.FieldValueMetaData(org.apache.thrift.protocol.TType.ENUM , "TokenType")));
tmpMap.put(_Fields.OWNER_NAME, new org.apache.thrift.meta_data.FieldMetaData("ownerName", org.apache.thrift.TFieldRequirementType.OPTIONAL,
new org.apache.thrift.meta_data.FieldValueMetaData(org.apache.thrift.protocol.TType.STRING)));
tmpMap.put(_Fields.ORGANIZATION_ID, new org.apache.thrift.meta_data.FieldMetaData("organizationId", org.apache.thrift.TFieldRequirementType.OPTIONAL,
new org.apache.thrift.meta_data.FieldValueMetaData(org.apache.thrift.protocol.TType.STRING , "uuid")));
tmpMap.put(_Fields.ORGANIZATION_NAME, new org.apache.thrift.meta_data.FieldMetaData("organizationName", org.apache.thrift.TFieldRequirementType.OPTIONAL,
new org.apache.thrift.meta_data.FieldValueMetaData(org.apache.thrift.protocol.TType.STRING)));
metaDataMap = Collections.unmodifiableMap(tmpMap);
org.apache.thrift.meta_data.FieldMetaData.addStructMetaDataMap(CreateTokenRequest.class, metaDataMap);
}
public CreateTokenRequest() {
}
public CreateTokenRequest(
String ownerId,
tech.aroma.thrift.authentication.TokenType desiredTokenType)
{
this();
this.ownerId = ownerId;
this.desiredTokenType = desiredTokenType;
}
/**
* Performs a deep copy on other.
*/
public CreateTokenRequest(CreateTokenRequest other) {
if (other.isSetOwnerId()) {
this.ownerId = other.ownerId;
}
if (other.isSetLifetime()) {
this.lifetime = other.lifetime;
}
if (other.isSetDesiredTokenType()) {
this.desiredTokenType = other.desiredTokenType;
}
if (other.isSetOwnerName()) {
this.ownerName = other.ownerName;
}
if (other.isSetOrganizationId()) {
this.organizationId = other.organizationId;
}
if (other.isSetOrganizationName()) {
this.organizationName = other.organizationName;
}
}
public CreateTokenRequest deepCopy() {
return new CreateTokenRequest(this);
}
@Override
public void clear() {
this.ownerId = null;
this.lifetime = null;
this.desiredTokenType = null;
this.ownerName = null;
this.organizationId = null;
this.organizationName = null;
}
/**
* The ID of the entity that will own the token.
* For Application tokens, this is the Application ID.
* For User Tokens, this is the userId.
*/
public String getOwnerId() {
return this.ownerId;
}
/**
* The ID of the entity that will own the token.
* For Application tokens, this is the Application ID.
* For User Tokens, this is the userId.
*/
public CreateTokenRequest setOwnerId(String ownerId) {
this.ownerId = ownerId;
return this;
}
public void unsetOwnerId() {
this.ownerId = null;
}
/** Returns true if field ownerId is set (has been assigned a value) and false otherwise */
public boolean isSetOwnerId() {
return this.ownerId != null;
}
public void setOwnerIdIsSet(boolean value) {
if (!value) {
this.ownerId = null;
}
}
/**
* The desired length of time to keep the Token alive and valid.
* If not present, will be set to DEFAULT_TOKEN_LIFETIME.
*/
public tech.aroma.thrift.LengthOfTime getLifetime() {
return this.lifetime;
}
/**
* The desired length of time to keep the Token alive and valid.
* If not present, will be set to DEFAULT_TOKEN_LIFETIME.
*/
public CreateTokenRequest setLifetime(tech.aroma.thrift.LengthOfTime lifetime) {
this.lifetime = lifetime;
return this;
}
public void unsetLifetime() {
this.lifetime = null;
}
/** Returns true if field lifetime is set (has been assigned a value) and false otherwise */
public boolean isSetLifetime() {
return this.lifetime != null;
}
public void setLifetimeIsSet(boolean value) {
if (!value) {
this.lifetime = null;
}
}
/**
* This is required, and determines the kind of Token created.
*/
public tech.aroma.thrift.authentication.TokenType getDesiredTokenType() {
return this.desiredTokenType;
}
/**
* This is required, and determines the kind of Token created.
*/
public CreateTokenRequest setDesiredTokenType(tech.aroma.thrift.authentication.TokenType desiredTokenType) {
this.desiredTokenType = desiredTokenType;
return this;
}
public void unsetDesiredTokenType() {
this.desiredTokenType = null;
}
/** Returns true if field desiredTokenType is set (has been assigned a value) and false otherwise */
public boolean isSetDesiredTokenType() {
return this.desiredTokenType != null;
}
public void setDesiredTokenTypeIsSet(boolean value) {
if (!value) {
this.desiredTokenType = null;
}
}
/**
* Optional stores the name of the entity owning the token, for instance App name or user's email.
*/
public String getOwnerName() {
return this.ownerName;
}
/**
* Optional stores the name of the entity owning the token, for instance App name or user's email.
*/
public CreateTokenRequest setOwnerName(String ownerName) {
this.ownerName = ownerName;
return this;
}
public void unsetOwnerName() {
this.ownerName = null;
}
/** Returns true if field ownerName is set (has been assigned a value) and false otherwise */
public boolean isSetOwnerName() {
return this.ownerName != null;
}
public void setOwnerNameIsSet(boolean value) {
if (!value) {
this.ownerName = null;
}
}
public String getOrganizationId() {
return this.organizationId;
}
public CreateTokenRequest setOrganizationId(String organizationId) {
this.organizationId = organizationId;
return this;
}
public void unsetOrganizationId() {
this.organizationId = null;
}
/** Returns true if field organizationId is set (has been assigned a value) and false otherwise */
public boolean isSetOrganizationId() {
return this.organizationId != null;
}
public void setOrganizationIdIsSet(boolean value) {
if (!value) {
this.organizationId = null;
}
}
public String getOrganizationName() {
return this.organizationName;
}
public CreateTokenRequest setOrganizationName(String organizationName) {
this.organizationName = organizationName;
return this;
}
public void unsetOrganizationName() {
this.organizationName = null;
}
/** Returns true if field organizationName is set (has been assigned a value) and false otherwise */
public boolean isSetOrganizationName() {
return this.organizationName != null;
}
public void setOrganizationNameIsSet(boolean value) {
if (!value) {
this.organizationName = null;
}
}
public void setFieldValue(_Fields field, Object value) {
switch (field) {
case OWNER_ID:
if (value == null) {
unsetOwnerId();
} else {
setOwnerId((String)value);
}
break;
case LIFETIME:
if (value == null) {
unsetLifetime();
} else {
setLifetime((tech.aroma.thrift.LengthOfTime)value);
}
break;
case DESIRED_TOKEN_TYPE:
if (value == null) {
unsetDesiredTokenType();
} else {
setDesiredTokenType((tech.aroma.thrift.authentication.TokenType)value);
}
break;
case OWNER_NAME:
if (value == null) {
unsetOwnerName();
} else {
setOwnerName((String)value);
}
break;
case ORGANIZATION_ID:
if (value == null) {
unsetOrganizationId();
} else {
setOrganizationId((String)value);
}
break;
case ORGANIZATION_NAME:
if (value == null) {
unsetOrganizationName();
} else {
setOrganizationName((String)value);
}
break;
}
}
public Object getFieldValue(_Fields field) {
switch (field) {
case OWNER_ID:
return getOwnerId();
case LIFETIME:
return getLifetime();
case DESIRED_TOKEN_TYPE:
return getDesiredTokenType();
case OWNER_NAME:
return getOwnerName();
case ORGANIZATION_ID:
return getOrganizationId();
case ORGANIZATION_NAME:
return getOrganizationName();
}
throw new IllegalStateException();
}
/** Returns true if field corresponding to fieldID is set (has been assigned a value) and false otherwise */
public boolean isSet(_Fields field) {
if (field == null) {
throw new IllegalArgumentException();
}
switch (field) {
case OWNER_ID:
return isSetOwnerId();
case LIFETIME:
return isSetLifetime();
case DESIRED_TOKEN_TYPE:
return isSetDesiredTokenType();
case OWNER_NAME:
return isSetOwnerName();
case ORGANIZATION_ID:
return isSetOrganizationId();
case ORGANIZATION_NAME:
return isSetOrganizationName();
}
throw new IllegalStateException();
}
@Override
public boolean equals(Object that) {
if (that == null)
return false;
if (that instanceof CreateTokenRequest)
return this.equals((CreateTokenRequest)that);
return false;
}
public boolean equals(CreateTokenRequest that) {
if (that == null)
return false;
boolean this_present_ownerId = true && this.isSetOwnerId();
boolean that_present_ownerId = true && that.isSetOwnerId();
if (this_present_ownerId || that_present_ownerId) {
if (!(this_present_ownerId && that_present_ownerId))
return false;
if (!this.ownerId.equals(that.ownerId))
return false;
}
boolean this_present_lifetime = true && this.isSetLifetime();
boolean that_present_lifetime = true && that.isSetLifetime();
if (this_present_lifetime || that_present_lifetime) {
if (!(this_present_lifetime && that_present_lifetime))
return false;
if (!this.lifetime.equals(that.lifetime))
return false;
}
boolean this_present_desiredTokenType = true && this.isSetDesiredTokenType();
boolean that_present_desiredTokenType = true && that.isSetDesiredTokenType();
if (this_present_desiredTokenType || that_present_desiredTokenType) {
if (!(this_present_desiredTokenType && that_present_desiredTokenType))
return false;
if (!this.desiredTokenType.equals(that.desiredTokenType))
return false;
}
boolean this_present_ownerName = true && this.isSetOwnerName();
boolean that_present_ownerName = true && that.isSetOwnerName();
if (this_present_ownerName || that_present_ownerName) {
if (!(this_present_ownerName && that_present_ownerName))
return false;
if (!this.ownerName.equals(that.ownerName))
return false;
}
boolean this_present_organizationId = true && this.isSetOrganizationId();
boolean that_present_organizationId = true && that.isSetOrganizationId();
if (this_present_organizationId || that_present_organizationId) {
if (!(this_present_organizationId && that_present_organizationId))
return false;
if (!this.organizationId.equals(that.organizationId))
return false;
}
boolean this_present_organizationName = true && this.isSetOrganizationName();
boolean that_present_organizationName = true && that.isSetOrganizationName();
if (this_present_organizationName || that_present_organizationName) {
if (!(this_present_organizationName && that_present_organizationName))
return false;
if (!this.organizationName.equals(that.organizationName))
return false;
}
return true;
}
@Override
public int hashCode() {
List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy