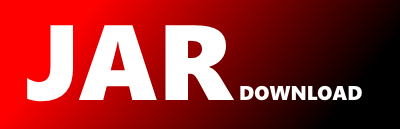
tech.aroma.thrift.email.EmailMessage Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aroma-thrift Show documentation
Show all versions of aroma-thrift Show documentation
Part of the Aroma Project.
This project contains the Service and Model Definitions.
From this the Server and Client interfaces are generated for the Aroma Service.
/**
* Autogenerated by Thrift Compiler (0.9.3)
*
* DO NOT EDIT UNLESS YOU ARE SURE THAT YOU KNOW WHAT YOU ARE DOING
* @generated
*/
package tech.aroma.thrift.email;
import org.apache.thrift.scheme.IScheme;
import org.apache.thrift.scheme.SchemeFactory;
import org.apache.thrift.scheme.StandardScheme;
import org.apache.thrift.scheme.TupleScheme;
import org.apache.thrift.protocol.TTupleProtocol;
import org.apache.thrift.protocol.TProtocolException;
import org.apache.thrift.EncodingUtils;
import org.apache.thrift.TException;
import org.apache.thrift.async.AsyncMethodCallback;
import org.apache.thrift.server.AbstractNonblockingServer.*;
import java.util.List;
import java.util.ArrayList;
import java.util.Map;
import java.util.HashMap;
import java.util.EnumMap;
import java.util.Set;
import java.util.HashSet;
import java.util.EnumSet;
import java.util.Collections;
import java.util.BitSet;
import java.nio.ByteBuffer;
import java.util.Arrays;
import javax.annotation.Generated;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
@SuppressWarnings({"cast", "rawtypes", "serial", "unchecked"})
public class EmailMessage extends org.apache.thrift.TUnion {
private static final org.apache.thrift.protocol.TStruct STRUCT_DESC = new org.apache.thrift.protocol.TStruct("EmailMessage");
private static final org.apache.thrift.protocol.TField NEW_APP_FIELD_DESC = new org.apache.thrift.protocol.TField("newApp", org.apache.thrift.protocol.TType.STRUCT, (short)1);
private static final org.apache.thrift.protocol.TField NEW_USER_FIELD_DESC = new org.apache.thrift.protocol.TField("newUser", org.apache.thrift.protocol.TType.STRUCT, (short)2);
/** The set of fields this struct contains, along with convenience methods for finding and manipulating them. */
public enum _Fields implements org.apache.thrift.TFieldIdEnum {
NEW_APP((short)1, "newApp"),
NEW_USER((short)2, "newUser");
private static final Map byName = new HashMap();
static {
for (_Fields field : EnumSet.allOf(_Fields.class)) {
byName.put(field.getFieldName(), field);
}
}
/**
* Find the _Fields constant that matches fieldId, or null if its not found.
*/
public static _Fields findByThriftId(int fieldId) {
switch(fieldId) {
case 1: // NEW_APP
return NEW_APP;
case 2: // NEW_USER
return NEW_USER;
default:
return null;
}
}
/**
* Find the _Fields constant that matches fieldId, throwing an exception
* if it is not found.
*/
public static _Fields findByThriftIdOrThrow(int fieldId) {
_Fields fields = findByThriftId(fieldId);
if (fields == null) throw new IllegalArgumentException("Field " + fieldId + " doesn't exist!");
return fields;
}
/**
* Find the _Fields constant that matches name, or null if its not found.
*/
public static _Fields findByName(String name) {
return byName.get(name);
}
private final short _thriftId;
private final String _fieldName;
_Fields(short thriftId, String fieldName) {
_thriftId = thriftId;
_fieldName = fieldName;
}
public short getThriftFieldId() {
return _thriftId;
}
public String getFieldName() {
return _fieldName;
}
}
public static final Map<_Fields, org.apache.thrift.meta_data.FieldMetaData> metaDataMap;
static {
Map<_Fields, org.apache.thrift.meta_data.FieldMetaData> tmpMap = new EnumMap<_Fields, org.apache.thrift.meta_data.FieldMetaData>(_Fields.class);
tmpMap.put(_Fields.NEW_APP, new org.apache.thrift.meta_data.FieldMetaData("newApp", org.apache.thrift.TFieldRequirementType.DEFAULT,
new org.apache.thrift.meta_data.StructMetaData(org.apache.thrift.protocol.TType.STRUCT, EmailNewApplication.class)));
tmpMap.put(_Fields.NEW_USER, new org.apache.thrift.meta_data.FieldMetaData("newUser", org.apache.thrift.TFieldRequirementType.DEFAULT,
new org.apache.thrift.meta_data.StructMetaData(org.apache.thrift.protocol.TType.STRUCT, EmailNewUserRegistration.class)));
metaDataMap = Collections.unmodifiableMap(tmpMap);
org.apache.thrift.meta_data.FieldMetaData.addStructMetaDataMap(EmailMessage.class, metaDataMap);
}
public EmailMessage() {
super();
}
public EmailMessage(_Fields setField, Object value) {
super(setField, value);
}
public EmailMessage(EmailMessage other) {
super(other);
}
public EmailMessage deepCopy() {
return new EmailMessage(this);
}
public static EmailMessage newApp(EmailNewApplication value) {
EmailMessage x = new EmailMessage();
x.setNewApp(value);
return x;
}
public static EmailMessage newUser(EmailNewUserRegistration value) {
EmailMessage x = new EmailMessage();
x.setNewUser(value);
return x;
}
@Override
protected void checkType(_Fields setField, Object value) throws ClassCastException {
switch (setField) {
case NEW_APP:
if (value instanceof EmailNewApplication) {
break;
}
throw new ClassCastException("Was expecting value of type EmailNewApplication for field 'newApp', but got " + value.getClass().getSimpleName());
case NEW_USER:
if (value instanceof EmailNewUserRegistration) {
break;
}
throw new ClassCastException("Was expecting value of type EmailNewUserRegistration for field 'newUser', but got " + value.getClass().getSimpleName());
default:
throw new IllegalArgumentException("Unknown field id " + setField);
}
}
@Override
protected Object standardSchemeReadValue(org.apache.thrift.protocol.TProtocol iprot, org.apache.thrift.protocol.TField field) throws org.apache.thrift.TException {
_Fields setField = _Fields.findByThriftId(field.id);
if (setField != null) {
switch (setField) {
case NEW_APP:
if (field.type == NEW_APP_FIELD_DESC.type) {
EmailNewApplication newApp;
newApp = new EmailNewApplication();
newApp.read(iprot);
return newApp;
} else {
org.apache.thrift.protocol.TProtocolUtil.skip(iprot, field.type);
return null;
}
case NEW_USER:
if (field.type == NEW_USER_FIELD_DESC.type) {
EmailNewUserRegistration newUser;
newUser = new EmailNewUserRegistration();
newUser.read(iprot);
return newUser;
} else {
org.apache.thrift.protocol.TProtocolUtil.skip(iprot, field.type);
return null;
}
default:
throw new IllegalStateException("setField wasn't null, but didn't match any of the case statements!");
}
} else {
org.apache.thrift.protocol.TProtocolUtil.skip(iprot, field.type);
return null;
}
}
@Override
protected void standardSchemeWriteValue(org.apache.thrift.protocol.TProtocol oprot) throws org.apache.thrift.TException {
switch (setField_) {
case NEW_APP:
EmailNewApplication newApp = (EmailNewApplication)value_;
newApp.write(oprot);
return;
case NEW_USER:
EmailNewUserRegistration newUser = (EmailNewUserRegistration)value_;
newUser.write(oprot);
return;
default:
throw new IllegalStateException("Cannot write union with unknown field " + setField_);
}
}
@Override
protected Object tupleSchemeReadValue(org.apache.thrift.protocol.TProtocol iprot, short fieldID) throws org.apache.thrift.TException {
_Fields setField = _Fields.findByThriftId(fieldID);
if (setField != null) {
switch (setField) {
case NEW_APP:
EmailNewApplication newApp;
newApp = new EmailNewApplication();
newApp.read(iprot);
return newApp;
case NEW_USER:
EmailNewUserRegistration newUser;
newUser = new EmailNewUserRegistration();
newUser.read(iprot);
return newUser;
default:
throw new IllegalStateException("setField wasn't null, but didn't match any of the case statements!");
}
} else {
throw new TProtocolException("Couldn't find a field with field id " + fieldID);
}
}
@Override
protected void tupleSchemeWriteValue(org.apache.thrift.protocol.TProtocol oprot) throws org.apache.thrift.TException {
switch (setField_) {
case NEW_APP:
EmailNewApplication newApp = (EmailNewApplication)value_;
newApp.write(oprot);
return;
case NEW_USER:
EmailNewUserRegistration newUser = (EmailNewUserRegistration)value_;
newUser.write(oprot);
return;
default:
throw new IllegalStateException("Cannot write union with unknown field " + setField_);
}
}
@Override
protected org.apache.thrift.protocol.TField getFieldDesc(_Fields setField) {
switch (setField) {
case NEW_APP:
return NEW_APP_FIELD_DESC;
case NEW_USER:
return NEW_USER_FIELD_DESC;
default:
throw new IllegalArgumentException("Unknown field id " + setField);
}
}
@Override
protected org.apache.thrift.protocol.TStruct getStructDesc() {
return STRUCT_DESC;
}
@Override
protected _Fields enumForId(short id) {
return _Fields.findByThriftIdOrThrow(id);
}
public _Fields fieldForId(int fieldId) {
return _Fields.findByThriftId(fieldId);
}
public EmailNewApplication getNewApp() {
if (getSetField() == _Fields.NEW_APP) {
return (EmailNewApplication)getFieldValue();
} else {
throw new RuntimeException("Cannot get field 'newApp' because union is currently set to " + getFieldDesc(getSetField()).name);
}
}
public void setNewApp(EmailNewApplication value) {
if (value == null) throw new NullPointerException();
setField_ = _Fields.NEW_APP;
value_ = value;
}
public EmailNewUserRegistration getNewUser() {
if (getSetField() == _Fields.NEW_USER) {
return (EmailNewUserRegistration)getFieldValue();
} else {
throw new RuntimeException("Cannot get field 'newUser' because union is currently set to " + getFieldDesc(getSetField()).name);
}
}
public void setNewUser(EmailNewUserRegistration value) {
if (value == null) throw new NullPointerException();
setField_ = _Fields.NEW_USER;
value_ = value;
}
public boolean isSetNewApp() {
return setField_ == _Fields.NEW_APP;
}
public boolean isSetNewUser() {
return setField_ == _Fields.NEW_USER;
}
public boolean equals(Object other) {
if (other instanceof EmailMessage) {
return equals((EmailMessage)other);
} else {
return false;
}
}
public boolean equals(EmailMessage other) {
return other != null && getSetField() == other.getSetField() && getFieldValue().equals(other.getFieldValue());
}
@Override
public int compareTo(EmailMessage other) {
int lastComparison = org.apache.thrift.TBaseHelper.compareTo(getSetField(), other.getSetField());
if (lastComparison == 0) {
return org.apache.thrift.TBaseHelper.compareTo(getFieldValue(), other.getFieldValue());
}
return lastComparison;
}
@Override
public int hashCode() {
List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy