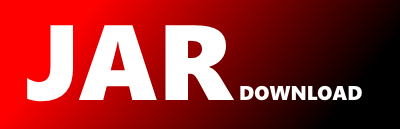
tech.aroma.thrift.endpoint.ApplicationEndpoint Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aroma-thrift Show documentation
Show all versions of aroma-thrift Show documentation
Part of the Aroma Project.
This project contains the Service and Model Definitions.
From this the Server and Client interfaces are generated for the Aroma Service.
/**
* Autogenerated by Thrift Compiler (0.9.3)
*
* DO NOT EDIT UNLESS YOU ARE SURE THAT YOU KNOW WHAT YOU ARE DOING
* @generated
*/
package tech.aroma.thrift.endpoint;
import org.apache.thrift.scheme.IScheme;
import org.apache.thrift.scheme.SchemeFactory;
import org.apache.thrift.scheme.StandardScheme;
import org.apache.thrift.scheme.TupleScheme;
import org.apache.thrift.protocol.TTupleProtocol;
import org.apache.thrift.protocol.TProtocolException;
import org.apache.thrift.EncodingUtils;
import org.apache.thrift.TException;
import org.apache.thrift.async.AsyncMethodCallback;
import org.apache.thrift.server.AbstractNonblockingServer.*;
import java.util.List;
import java.util.ArrayList;
import java.util.Map;
import java.util.HashMap;
import java.util.EnumMap;
import java.util.Set;
import java.util.HashSet;
import java.util.EnumSet;
import java.util.Collections;
import java.util.BitSet;
import java.nio.ByteBuffer;
import java.util.Arrays;
import javax.annotation.Generated;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
@SuppressWarnings({"cast", "rawtypes", "serial", "unchecked"})
@Generated(value = "Autogenerated by Thrift Compiler (0.9.3)", date = "2016-05-10")
public class ApplicationEndpoint {
/**
* This service is implemented by Applications wishing to be "poked"
* for health checks.
*/
public interface Iface {
public HealthPokeResponse healthPoke(HealthPokeRequest request) throws tech.aroma.thrift.exceptions.OperationFailedException, org.apache.thrift.TException;
}
public interface AsyncIface {
public void healthPoke(HealthPokeRequest request, org.apache.thrift.async.AsyncMethodCallback resultHandler) throws org.apache.thrift.TException;
}
public static class Client extends org.apache.thrift.TServiceClient implements Iface {
public static class Factory implements org.apache.thrift.TServiceClientFactory {
public Factory() {}
public Client getClient(org.apache.thrift.protocol.TProtocol prot) {
return new Client(prot);
}
public Client getClient(org.apache.thrift.protocol.TProtocol iprot, org.apache.thrift.protocol.TProtocol oprot) {
return new Client(iprot, oprot);
}
}
public Client(org.apache.thrift.protocol.TProtocol prot)
{
super(prot, prot);
}
public Client(org.apache.thrift.protocol.TProtocol iprot, org.apache.thrift.protocol.TProtocol oprot) {
super(iprot, oprot);
}
public HealthPokeResponse healthPoke(HealthPokeRequest request) throws tech.aroma.thrift.exceptions.OperationFailedException, org.apache.thrift.TException
{
send_healthPoke(request);
return recv_healthPoke();
}
public void send_healthPoke(HealthPokeRequest request) throws org.apache.thrift.TException
{
healthPoke_args args = new healthPoke_args();
args.setRequest(request);
sendBase("healthPoke", args);
}
public HealthPokeResponse recv_healthPoke() throws tech.aroma.thrift.exceptions.OperationFailedException, org.apache.thrift.TException
{
healthPoke_result result = new healthPoke_result();
receiveBase(result, "healthPoke");
if (result.isSetSuccess()) {
return result.success;
}
if (result.ex1 != null) {
throw result.ex1;
}
throw new org.apache.thrift.TApplicationException(org.apache.thrift.TApplicationException.MISSING_RESULT, "healthPoke failed: unknown result");
}
}
public static class AsyncClient extends org.apache.thrift.async.TAsyncClient implements AsyncIface {
public static class Factory implements org.apache.thrift.async.TAsyncClientFactory {
private org.apache.thrift.async.TAsyncClientManager clientManager;
private org.apache.thrift.protocol.TProtocolFactory protocolFactory;
public Factory(org.apache.thrift.async.TAsyncClientManager clientManager, org.apache.thrift.protocol.TProtocolFactory protocolFactory) {
this.clientManager = clientManager;
this.protocolFactory = protocolFactory;
}
public AsyncClient getAsyncClient(org.apache.thrift.transport.TNonblockingTransport transport) {
return new AsyncClient(protocolFactory, clientManager, transport);
}
}
public AsyncClient(org.apache.thrift.protocol.TProtocolFactory protocolFactory, org.apache.thrift.async.TAsyncClientManager clientManager, org.apache.thrift.transport.TNonblockingTransport transport) {
super(protocolFactory, clientManager, transport);
}
public void healthPoke(HealthPokeRequest request, org.apache.thrift.async.AsyncMethodCallback resultHandler) throws org.apache.thrift.TException {
checkReady();
healthPoke_call method_call = new healthPoke_call(request, resultHandler, this, ___protocolFactory, ___transport);
this.___currentMethod = method_call;
___manager.call(method_call);
}
public static class healthPoke_call extends org.apache.thrift.async.TAsyncMethodCall {
private HealthPokeRequest request;
public healthPoke_call(HealthPokeRequest request, org.apache.thrift.async.AsyncMethodCallback resultHandler, org.apache.thrift.async.TAsyncClient client, org.apache.thrift.protocol.TProtocolFactory protocolFactory, org.apache.thrift.transport.TNonblockingTransport transport) throws org.apache.thrift.TException {
super(client, protocolFactory, transport, resultHandler, false);
this.request = request;
}
public void write_args(org.apache.thrift.protocol.TProtocol prot) throws org.apache.thrift.TException {
prot.writeMessageBegin(new org.apache.thrift.protocol.TMessage("healthPoke", org.apache.thrift.protocol.TMessageType.CALL, 0));
healthPoke_args args = new healthPoke_args();
args.setRequest(request);
args.write(prot);
prot.writeMessageEnd();
}
public HealthPokeResponse getResult() throws tech.aroma.thrift.exceptions.OperationFailedException, org.apache.thrift.TException {
if (getState() != org.apache.thrift.async.TAsyncMethodCall.State.RESPONSE_READ) {
throw new IllegalStateException("Method call not finished!");
}
org.apache.thrift.transport.TMemoryInputTransport memoryTransport = new org.apache.thrift.transport.TMemoryInputTransport(getFrameBuffer().array());
org.apache.thrift.protocol.TProtocol prot = client.getProtocolFactory().getProtocol(memoryTransport);
return (new Client(prot)).recv_healthPoke();
}
}
}
public static class Processor extends org.apache.thrift.TBaseProcessor implements org.apache.thrift.TProcessor {
private static final Logger LOGGER = LoggerFactory.getLogger(Processor.class.getName());
public Processor(I iface) {
super(iface, getProcessMap(new HashMap>()));
}
protected Processor(I iface, Map> processMap) {
super(iface, getProcessMap(processMap));
}
private static Map> getProcessMap(Map> processMap) {
processMap.put("healthPoke", new healthPoke());
return processMap;
}
public static class healthPoke extends org.apache.thrift.ProcessFunction {
public healthPoke() {
super("healthPoke");
}
public healthPoke_args getEmptyArgsInstance() {
return new healthPoke_args();
}
protected boolean isOneway() {
return false;
}
public healthPoke_result getResult(I iface, healthPoke_args args) throws org.apache.thrift.TException {
healthPoke_result result = new healthPoke_result();
try {
result.success = iface.healthPoke(args.request);
} catch (tech.aroma.thrift.exceptions.OperationFailedException ex1) {
result.ex1 = ex1;
}
return result;
}
}
}
public static class AsyncProcessor extends org.apache.thrift.TBaseAsyncProcessor {
private static final Logger LOGGER = LoggerFactory.getLogger(AsyncProcessor.class.getName());
public AsyncProcessor(I iface) {
super(iface, getProcessMap(new HashMap>()));
}
protected AsyncProcessor(I iface, Map> processMap) {
super(iface, getProcessMap(processMap));
}
private static Map> getProcessMap(Map> processMap) {
processMap.put("healthPoke", new healthPoke());
return processMap;
}
public static class healthPoke extends org.apache.thrift.AsyncProcessFunction {
public healthPoke() {
super("healthPoke");
}
public healthPoke_args getEmptyArgsInstance() {
return new healthPoke_args();
}
public AsyncMethodCallback getResultHandler(final AsyncFrameBuffer fb, final int seqid) {
final org.apache.thrift.AsyncProcessFunction fcall = this;
return new AsyncMethodCallback() {
public void onComplete(HealthPokeResponse o) {
healthPoke_result result = new healthPoke_result();
result.success = o;
try {
fcall.sendResponse(fb,result, org.apache.thrift.protocol.TMessageType.REPLY,seqid);
return;
} catch (Exception e) {
LOGGER.error("Exception writing to internal frame buffer", e);
}
fb.close();
}
public void onError(Exception e) {
byte msgType = org.apache.thrift.protocol.TMessageType.REPLY;
org.apache.thrift.TBase msg;
healthPoke_result result = new healthPoke_result();
if (e instanceof tech.aroma.thrift.exceptions.OperationFailedException) {
result.ex1 = (tech.aroma.thrift.exceptions.OperationFailedException) e;
result.setEx1IsSet(true);
msg = result;
}
else
{
msgType = org.apache.thrift.protocol.TMessageType.EXCEPTION;
msg = (org.apache.thrift.TBase)new org.apache.thrift.TApplicationException(org.apache.thrift.TApplicationException.INTERNAL_ERROR, e.getMessage());
}
try {
fcall.sendResponse(fb,msg,msgType,seqid);
return;
} catch (Exception ex) {
LOGGER.error("Exception writing to internal frame buffer", ex);
}
fb.close();
}
};
}
protected boolean isOneway() {
return false;
}
public void start(I iface, healthPoke_args args, org.apache.thrift.async.AsyncMethodCallback resultHandler) throws TException {
iface.healthPoke(args.request,resultHandler);
}
}
}
public static class healthPoke_args implements org.apache.thrift.TBase, java.io.Serializable, Cloneable, Comparable {
private static final org.apache.thrift.protocol.TStruct STRUCT_DESC = new org.apache.thrift.protocol.TStruct("healthPoke_args");
private static final org.apache.thrift.protocol.TField REQUEST_FIELD_DESC = new org.apache.thrift.protocol.TField("request", org.apache.thrift.protocol.TType.STRUCT, (short)1);
private static final Map, SchemeFactory> schemes = new HashMap, SchemeFactory>();
static {
schemes.put(StandardScheme.class, new healthPoke_argsStandardSchemeFactory());
schemes.put(TupleScheme.class, new healthPoke_argsTupleSchemeFactory());
}
public HealthPokeRequest request; // required
/** The set of fields this struct contains, along with convenience methods for finding and manipulating them. */
public enum _Fields implements org.apache.thrift.TFieldIdEnum {
REQUEST((short)1, "request");
private static final Map byName = new HashMap();
static {
for (_Fields field : EnumSet.allOf(_Fields.class)) {
byName.put(field.getFieldName(), field);
}
}
/**
* Find the _Fields constant that matches fieldId, or null if its not found.
*/
public static _Fields findByThriftId(int fieldId) {
switch(fieldId) {
case 1: // REQUEST
return REQUEST;
default:
return null;
}
}
/**
* Find the _Fields constant that matches fieldId, throwing an exception
* if it is not found.
*/
public static _Fields findByThriftIdOrThrow(int fieldId) {
_Fields fields = findByThriftId(fieldId);
if (fields == null) throw new IllegalArgumentException("Field " + fieldId + " doesn't exist!");
return fields;
}
/**
* Find the _Fields constant that matches name, or null if its not found.
*/
public static _Fields findByName(String name) {
return byName.get(name);
}
private final short _thriftId;
private final String _fieldName;
_Fields(short thriftId, String fieldName) {
_thriftId = thriftId;
_fieldName = fieldName;
}
public short getThriftFieldId() {
return _thriftId;
}
public String getFieldName() {
return _fieldName;
}
}
// isset id assignments
public static final Map<_Fields, org.apache.thrift.meta_data.FieldMetaData> metaDataMap;
static {
Map<_Fields, org.apache.thrift.meta_data.FieldMetaData> tmpMap = new EnumMap<_Fields, org.apache.thrift.meta_data.FieldMetaData>(_Fields.class);
tmpMap.put(_Fields.REQUEST, new org.apache.thrift.meta_data.FieldMetaData("request", org.apache.thrift.TFieldRequirementType.DEFAULT,
new org.apache.thrift.meta_data.StructMetaData(org.apache.thrift.protocol.TType.STRUCT, HealthPokeRequest.class)));
metaDataMap = Collections.unmodifiableMap(tmpMap);
org.apache.thrift.meta_data.FieldMetaData.addStructMetaDataMap(healthPoke_args.class, metaDataMap);
}
public healthPoke_args() {
}
public healthPoke_args(
HealthPokeRequest request)
{
this();
this.request = request;
}
/**
* Performs a deep copy on other.
*/
public healthPoke_args(healthPoke_args other) {
if (other.isSetRequest()) {
this.request = new HealthPokeRequest(other.request);
}
}
public healthPoke_args deepCopy() {
return new healthPoke_args(this);
}
@Override
public void clear() {
this.request = null;
}
public HealthPokeRequest getRequest() {
return this.request;
}
public healthPoke_args setRequest(HealthPokeRequest request) {
this.request = request;
return this;
}
public void unsetRequest() {
this.request = null;
}
/** Returns true if field request is set (has been assigned a value) and false otherwise */
public boolean isSetRequest() {
return this.request != null;
}
public void setRequestIsSet(boolean value) {
if (!value) {
this.request = null;
}
}
public void setFieldValue(_Fields field, Object value) {
switch (field) {
case REQUEST:
if (value == null) {
unsetRequest();
} else {
setRequest((HealthPokeRequest)value);
}
break;
}
}
public Object getFieldValue(_Fields field) {
switch (field) {
case REQUEST:
return getRequest();
}
throw new IllegalStateException();
}
/** Returns true if field corresponding to fieldID is set (has been assigned a value) and false otherwise */
public boolean isSet(_Fields field) {
if (field == null) {
throw new IllegalArgumentException();
}
switch (field) {
case REQUEST:
return isSetRequest();
}
throw new IllegalStateException();
}
@Override
public boolean equals(Object that) {
if (that == null)
return false;
if (that instanceof healthPoke_args)
return this.equals((healthPoke_args)that);
return false;
}
public boolean equals(healthPoke_args that) {
if (that == null)
return false;
boolean this_present_request = true && this.isSetRequest();
boolean that_present_request = true && that.isSetRequest();
if (this_present_request || that_present_request) {
if (!(this_present_request && that_present_request))
return false;
if (!this.request.equals(that.request))
return false;
}
return true;
}
@Override
public int hashCode() {
List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy