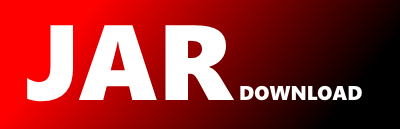
tech.aroma.thrift.events.Event Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aroma-thrift Show documentation
Show all versions of aroma-thrift Show documentation
Part of the Aroma Project.
This project contains the Service and Model Definitions.
From this the Server and Client interfaces are generated for the Aroma Service.
/**
* Autogenerated by Thrift Compiler (0.9.3)
*
* DO NOT EDIT UNLESS YOU ARE SURE THAT YOU KNOW WHAT YOU ARE DOING
* @generated
*/
package tech.aroma.thrift.events;
import org.apache.thrift.scheme.IScheme;
import org.apache.thrift.scheme.SchemeFactory;
import org.apache.thrift.scheme.StandardScheme;
import org.apache.thrift.scheme.TupleScheme;
import org.apache.thrift.protocol.TTupleProtocol;
import org.apache.thrift.protocol.TProtocolException;
import org.apache.thrift.EncodingUtils;
import org.apache.thrift.TException;
import org.apache.thrift.async.AsyncMethodCallback;
import org.apache.thrift.server.AbstractNonblockingServer.*;
import java.util.List;
import java.util.ArrayList;
import java.util.Map;
import java.util.HashMap;
import java.util.EnumMap;
import java.util.Set;
import java.util.HashSet;
import java.util.EnumSet;
import java.util.Collections;
import java.util.BitSet;
import java.nio.ByteBuffer;
import java.util.Arrays;
import javax.annotation.Generated;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
@SuppressWarnings({"cast", "rawtypes", "serial", "unchecked"})
/**
* A Notification defines an Event, and the time that it happened.
*/
@Generated(value = "Autogenerated by Thrift Compiler (0.9.3)", date = "2016-05-10")
public class Event implements org.apache.thrift.TBase, java.io.Serializable, Cloneable, Comparable {
private static final org.apache.thrift.protocol.TStruct STRUCT_DESC = new org.apache.thrift.protocol.TStruct("Event");
private static final org.apache.thrift.protocol.TField EVENT_ID_FIELD_DESC = new org.apache.thrift.protocol.TField("eventId", org.apache.thrift.protocol.TType.STRING, (short)1);
private static final org.apache.thrift.protocol.TField USER_ID_OF_ACTOR_FIELD_DESC = new org.apache.thrift.protocol.TField("userIdOfActor", org.apache.thrift.protocol.TType.STRING, (short)2);
private static final org.apache.thrift.protocol.TField ACTOR_FIELD_DESC = new org.apache.thrift.protocol.TField("actor", org.apache.thrift.protocol.TType.STRUCT, (short)3);
private static final org.apache.thrift.protocol.TField APPLICATION_ID_FIELD_DESC = new org.apache.thrift.protocol.TField("applicationId", org.apache.thrift.protocol.TType.STRING, (short)4);
private static final org.apache.thrift.protocol.TField APPLICATION_FIELD_DESC = new org.apache.thrift.protocol.TField("application", org.apache.thrift.protocol.TType.STRUCT, (short)5);
private static final org.apache.thrift.protocol.TField EVENT_TYPE_FIELD_DESC = new org.apache.thrift.protocol.TField("eventType", org.apache.thrift.protocol.TType.STRUCT, (short)6);
private static final org.apache.thrift.protocol.TField TIMESTAMP_FIELD_DESC = new org.apache.thrift.protocol.TField("timestamp", org.apache.thrift.protocol.TType.I64, (short)7);
private static final Map, SchemeFactory> schemes = new HashMap, SchemeFactory>();
static {
schemes.put(StandardScheme.class, new EventStandardSchemeFactory());
schemes.put(TupleScheme.class, new EventTupleSchemeFactory());
}
public String eventId; // required
public String userIdOfActor; // required
public tech.aroma.thrift.User actor; // optional
public String applicationId; // required
public tech.aroma.thrift.Application application; // optional
public EventType eventType; // required
public long timestamp; // required
/** The set of fields this struct contains, along with convenience methods for finding and manipulating them. */
public enum _Fields implements org.apache.thrift.TFieldIdEnum {
EVENT_ID((short)1, "eventId"),
USER_ID_OF_ACTOR((short)2, "userIdOfActor"),
ACTOR((short)3, "actor"),
APPLICATION_ID((short)4, "applicationId"),
APPLICATION((short)5, "application"),
EVENT_TYPE((short)6, "eventType"),
TIMESTAMP((short)7, "timestamp");
private static final Map byName = new HashMap();
static {
for (_Fields field : EnumSet.allOf(_Fields.class)) {
byName.put(field.getFieldName(), field);
}
}
/**
* Find the _Fields constant that matches fieldId, or null if its not found.
*/
public static _Fields findByThriftId(int fieldId) {
switch(fieldId) {
case 1: // EVENT_ID
return EVENT_ID;
case 2: // USER_ID_OF_ACTOR
return USER_ID_OF_ACTOR;
case 3: // ACTOR
return ACTOR;
case 4: // APPLICATION_ID
return APPLICATION_ID;
case 5: // APPLICATION
return APPLICATION;
case 6: // EVENT_TYPE
return EVENT_TYPE;
case 7: // TIMESTAMP
return TIMESTAMP;
default:
return null;
}
}
/**
* Find the _Fields constant that matches fieldId, throwing an exception
* if it is not found.
*/
public static _Fields findByThriftIdOrThrow(int fieldId) {
_Fields fields = findByThriftId(fieldId);
if (fields == null) throw new IllegalArgumentException("Field " + fieldId + " doesn't exist!");
return fields;
}
/**
* Find the _Fields constant that matches name, or null if its not found.
*/
public static _Fields findByName(String name) {
return byName.get(name);
}
private final short _thriftId;
private final String _fieldName;
_Fields(short thriftId, String fieldName) {
_thriftId = thriftId;
_fieldName = fieldName;
}
public short getThriftFieldId() {
return _thriftId;
}
public String getFieldName() {
return _fieldName;
}
}
// isset id assignments
private static final int __TIMESTAMP_ISSET_ID = 0;
private byte __isset_bitfield = 0;
private static final _Fields optionals[] = {_Fields.ACTOR,_Fields.APPLICATION};
public static final Map<_Fields, org.apache.thrift.meta_data.FieldMetaData> metaDataMap;
static {
Map<_Fields, org.apache.thrift.meta_data.FieldMetaData> tmpMap = new EnumMap<_Fields, org.apache.thrift.meta_data.FieldMetaData>(_Fields.class);
tmpMap.put(_Fields.EVENT_ID, new org.apache.thrift.meta_data.FieldMetaData("eventId", org.apache.thrift.TFieldRequirementType.DEFAULT,
new org.apache.thrift.meta_data.FieldValueMetaData(org.apache.thrift.protocol.TType.STRING , "uuid")));
tmpMap.put(_Fields.USER_ID_OF_ACTOR, new org.apache.thrift.meta_data.FieldMetaData("userIdOfActor", org.apache.thrift.TFieldRequirementType.DEFAULT,
new org.apache.thrift.meta_data.FieldValueMetaData(org.apache.thrift.protocol.TType.STRING , "uuid")));
tmpMap.put(_Fields.ACTOR, new org.apache.thrift.meta_data.FieldMetaData("actor", org.apache.thrift.TFieldRequirementType.OPTIONAL,
new org.apache.thrift.meta_data.FieldValueMetaData(org.apache.thrift.protocol.TType.STRUCT , "User")));
tmpMap.put(_Fields.APPLICATION_ID, new org.apache.thrift.meta_data.FieldMetaData("applicationId", org.apache.thrift.TFieldRequirementType.DEFAULT,
new org.apache.thrift.meta_data.FieldValueMetaData(org.apache.thrift.protocol.TType.STRING , "uuid")));
tmpMap.put(_Fields.APPLICATION, new org.apache.thrift.meta_data.FieldMetaData("application", org.apache.thrift.TFieldRequirementType.OPTIONAL,
new org.apache.thrift.meta_data.FieldValueMetaData(org.apache.thrift.protocol.TType.STRUCT , "Application")));
tmpMap.put(_Fields.EVENT_TYPE, new org.apache.thrift.meta_data.FieldMetaData("eventType", org.apache.thrift.TFieldRequirementType.DEFAULT,
new org.apache.thrift.meta_data.StructMetaData(org.apache.thrift.protocol.TType.STRUCT, EventType.class)));
tmpMap.put(_Fields.TIMESTAMP, new org.apache.thrift.meta_data.FieldMetaData("timestamp", org.apache.thrift.TFieldRequirementType.DEFAULT,
new org.apache.thrift.meta_data.FieldValueMetaData(org.apache.thrift.protocol.TType.I64 , "timestamp")));
metaDataMap = Collections.unmodifiableMap(tmpMap);
org.apache.thrift.meta_data.FieldMetaData.addStructMetaDataMap(Event.class, metaDataMap);
}
public Event() {
}
public Event(
String eventId,
String userIdOfActor,
String applicationId,
EventType eventType,
long timestamp)
{
this();
this.eventId = eventId;
this.userIdOfActor = userIdOfActor;
this.applicationId = applicationId;
this.eventType = eventType;
this.timestamp = timestamp;
setTimestampIsSet(true);
}
/**
* Performs a deep copy on other.
*/
public Event(Event other) {
__isset_bitfield = other.__isset_bitfield;
if (other.isSetEventId()) {
this.eventId = other.eventId;
}
if (other.isSetUserIdOfActor()) {
this.userIdOfActor = other.userIdOfActor;
}
if (other.isSetActor()) {
this.actor = other.actor;
}
if (other.isSetApplicationId()) {
this.applicationId = other.applicationId;
}
if (other.isSetApplication()) {
this.application = other.application;
}
if (other.isSetEventType()) {
this.eventType = new EventType(other.eventType);
}
this.timestamp = other.timestamp;
}
public Event deepCopy() {
return new Event(this);
}
@Override
public void clear() {
this.eventId = null;
this.userIdOfActor = null;
this.actor = null;
this.applicationId = null;
this.application = null;
this.eventType = null;
setTimestampIsSet(false);
this.timestamp = 0;
}
public String getEventId() {
return this.eventId;
}
public Event setEventId(String eventId) {
this.eventId = eventId;
return this;
}
public void unsetEventId() {
this.eventId = null;
}
/** Returns true if field eventId is set (has been assigned a value) and false otherwise */
public boolean isSetEventId() {
return this.eventId != null;
}
public void setEventIdIsSet(boolean value) {
if (!value) {
this.eventId = null;
}
}
public String getUserIdOfActor() {
return this.userIdOfActor;
}
public Event setUserIdOfActor(String userIdOfActor) {
this.userIdOfActor = userIdOfActor;
return this;
}
public void unsetUserIdOfActor() {
this.userIdOfActor = null;
}
/** Returns true if field userIdOfActor is set (has been assigned a value) and false otherwise */
public boolean isSetUserIdOfActor() {
return this.userIdOfActor != null;
}
public void setUserIdOfActorIsSet(boolean value) {
if (!value) {
this.userIdOfActor = null;
}
}
public tech.aroma.thrift.User getActor() {
return this.actor;
}
public Event setActor(tech.aroma.thrift.User actor) {
this.actor = actor;
return this;
}
public void unsetActor() {
this.actor = null;
}
/** Returns true if field actor is set (has been assigned a value) and false otherwise */
public boolean isSetActor() {
return this.actor != null;
}
public void setActorIsSet(boolean value) {
if (!value) {
this.actor = null;
}
}
public String getApplicationId() {
return this.applicationId;
}
public Event setApplicationId(String applicationId) {
this.applicationId = applicationId;
return this;
}
public void unsetApplicationId() {
this.applicationId = null;
}
/** Returns true if field applicationId is set (has been assigned a value) and false otherwise */
public boolean isSetApplicationId() {
return this.applicationId != null;
}
public void setApplicationIdIsSet(boolean value) {
if (!value) {
this.applicationId = null;
}
}
public tech.aroma.thrift.Application getApplication() {
return this.application;
}
public Event setApplication(tech.aroma.thrift.Application application) {
this.application = application;
return this;
}
public void unsetApplication() {
this.application = null;
}
/** Returns true if field application is set (has been assigned a value) and false otherwise */
public boolean isSetApplication() {
return this.application != null;
}
public void setApplicationIsSet(boolean value) {
if (!value) {
this.application = null;
}
}
public EventType getEventType() {
return this.eventType;
}
public Event setEventType(EventType eventType) {
this.eventType = eventType;
return this;
}
public void unsetEventType() {
this.eventType = null;
}
/** Returns true if field eventType is set (has been assigned a value) and false otherwise */
public boolean isSetEventType() {
return this.eventType != null;
}
public void setEventTypeIsSet(boolean value) {
if (!value) {
this.eventType = null;
}
}
public long getTimestamp() {
return this.timestamp;
}
public Event setTimestamp(long timestamp) {
this.timestamp = timestamp;
setTimestampIsSet(true);
return this;
}
public void unsetTimestamp() {
__isset_bitfield = EncodingUtils.clearBit(__isset_bitfield, __TIMESTAMP_ISSET_ID);
}
/** Returns true if field timestamp is set (has been assigned a value) and false otherwise */
public boolean isSetTimestamp() {
return EncodingUtils.testBit(__isset_bitfield, __TIMESTAMP_ISSET_ID);
}
public void setTimestampIsSet(boolean value) {
__isset_bitfield = EncodingUtils.setBit(__isset_bitfield, __TIMESTAMP_ISSET_ID, value);
}
public void setFieldValue(_Fields field, Object value) {
switch (field) {
case EVENT_ID:
if (value == null) {
unsetEventId();
} else {
setEventId((String)value);
}
break;
case USER_ID_OF_ACTOR:
if (value == null) {
unsetUserIdOfActor();
} else {
setUserIdOfActor((String)value);
}
break;
case ACTOR:
if (value == null) {
unsetActor();
} else {
setActor((tech.aroma.thrift.User)value);
}
break;
case APPLICATION_ID:
if (value == null) {
unsetApplicationId();
} else {
setApplicationId((String)value);
}
break;
case APPLICATION:
if (value == null) {
unsetApplication();
} else {
setApplication((tech.aroma.thrift.Application)value);
}
break;
case EVENT_TYPE:
if (value == null) {
unsetEventType();
} else {
setEventType((EventType)value);
}
break;
case TIMESTAMP:
if (value == null) {
unsetTimestamp();
} else {
setTimestamp((Long)value);
}
break;
}
}
public Object getFieldValue(_Fields field) {
switch (field) {
case EVENT_ID:
return getEventId();
case USER_ID_OF_ACTOR:
return getUserIdOfActor();
case ACTOR:
return getActor();
case APPLICATION_ID:
return getApplicationId();
case APPLICATION:
return getApplication();
case EVENT_TYPE:
return getEventType();
case TIMESTAMP:
return getTimestamp();
}
throw new IllegalStateException();
}
/** Returns true if field corresponding to fieldID is set (has been assigned a value) and false otherwise */
public boolean isSet(_Fields field) {
if (field == null) {
throw new IllegalArgumentException();
}
switch (field) {
case EVENT_ID:
return isSetEventId();
case USER_ID_OF_ACTOR:
return isSetUserIdOfActor();
case ACTOR:
return isSetActor();
case APPLICATION_ID:
return isSetApplicationId();
case APPLICATION:
return isSetApplication();
case EVENT_TYPE:
return isSetEventType();
case TIMESTAMP:
return isSetTimestamp();
}
throw new IllegalStateException();
}
@Override
public boolean equals(Object that) {
if (that == null)
return false;
if (that instanceof Event)
return this.equals((Event)that);
return false;
}
public boolean equals(Event that) {
if (that == null)
return false;
boolean this_present_eventId = true && this.isSetEventId();
boolean that_present_eventId = true && that.isSetEventId();
if (this_present_eventId || that_present_eventId) {
if (!(this_present_eventId && that_present_eventId))
return false;
if (!this.eventId.equals(that.eventId))
return false;
}
boolean this_present_userIdOfActor = true && this.isSetUserIdOfActor();
boolean that_present_userIdOfActor = true && that.isSetUserIdOfActor();
if (this_present_userIdOfActor || that_present_userIdOfActor) {
if (!(this_present_userIdOfActor && that_present_userIdOfActor))
return false;
if (!this.userIdOfActor.equals(that.userIdOfActor))
return false;
}
boolean this_present_actor = true && this.isSetActor();
boolean that_present_actor = true && that.isSetActor();
if (this_present_actor || that_present_actor) {
if (!(this_present_actor && that_present_actor))
return false;
if (!this.actor.equals(that.actor))
return false;
}
boolean this_present_applicationId = true && this.isSetApplicationId();
boolean that_present_applicationId = true && that.isSetApplicationId();
if (this_present_applicationId || that_present_applicationId) {
if (!(this_present_applicationId && that_present_applicationId))
return false;
if (!this.applicationId.equals(that.applicationId))
return false;
}
boolean this_present_application = true && this.isSetApplication();
boolean that_present_application = true && that.isSetApplication();
if (this_present_application || that_present_application) {
if (!(this_present_application && that_present_application))
return false;
if (!this.application.equals(that.application))
return false;
}
boolean this_present_eventType = true && this.isSetEventType();
boolean that_present_eventType = true && that.isSetEventType();
if (this_present_eventType || that_present_eventType) {
if (!(this_present_eventType && that_present_eventType))
return false;
if (!this.eventType.equals(that.eventType))
return false;
}
boolean this_present_timestamp = true;
boolean that_present_timestamp = true;
if (this_present_timestamp || that_present_timestamp) {
if (!(this_present_timestamp && that_present_timestamp))
return false;
if (this.timestamp != that.timestamp)
return false;
}
return true;
}
@Override
public int hashCode() {
List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy