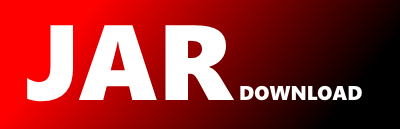
tech.aroma.thrift.reactions.AromaMatcher Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aroma-thrift Show documentation
Show all versions of aroma-thrift Show documentation
Part of the Aroma Project.
This project contains the Service and Model Definitions.
From this the Server and Client interfaces are generated for the Aroma Service.
/**
* Autogenerated by Thrift Compiler (0.9.3)
*
* DO NOT EDIT UNLESS YOU ARE SURE THAT YOU KNOW WHAT YOU ARE DOING
* @generated
*/
package tech.aroma.thrift.reactions;
import org.apache.thrift.scheme.IScheme;
import org.apache.thrift.scheme.SchemeFactory;
import org.apache.thrift.scheme.StandardScheme;
import org.apache.thrift.scheme.TupleScheme;
import org.apache.thrift.protocol.TTupleProtocol;
import org.apache.thrift.protocol.TProtocolException;
import org.apache.thrift.EncodingUtils;
import org.apache.thrift.TException;
import org.apache.thrift.async.AsyncMethodCallback;
import org.apache.thrift.server.AbstractNonblockingServer.*;
import java.util.List;
import java.util.ArrayList;
import java.util.Map;
import java.util.HashMap;
import java.util.EnumMap;
import java.util.Set;
import java.util.HashSet;
import java.util.EnumSet;
import java.util.Collections;
import java.util.BitSet;
import java.nio.ByteBuffer;
import java.util.Arrays;
import javax.annotation.Generated;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
@SuppressWarnings({"cast", "rawtypes", "serial", "unchecked"})
public class AromaMatcher extends org.apache.thrift.TUnion {
private static final org.apache.thrift.protocol.TStruct STRUCT_DESC = new org.apache.thrift.protocol.TStruct("AromaMatcher");
private static final org.apache.thrift.protocol.TField ALL_FIELD_DESC = new org.apache.thrift.protocol.TField("all", org.apache.thrift.protocol.TType.STRUCT, (short)1);
private static final org.apache.thrift.protocol.TField TITLE_IS_FIELD_DESC = new org.apache.thrift.protocol.TField("titleIs", org.apache.thrift.protocol.TType.STRUCT, (short)2);
private static final org.apache.thrift.protocol.TField TITLE_IS_NOT_FIELD_DESC = new org.apache.thrift.protocol.TField("titleIsNot", org.apache.thrift.protocol.TType.STRUCT, (short)3);
private static final org.apache.thrift.protocol.TField TITLE_CONTAINS_FIELD_DESC = new org.apache.thrift.protocol.TField("titleContains", org.apache.thrift.protocol.TType.STRUCT, (short)4);
private static final org.apache.thrift.protocol.TField TITLE_DOES_NOT_CONTAIN_FIELD_DESC = new org.apache.thrift.protocol.TField("titleDoesNotContain", org.apache.thrift.protocol.TType.STRUCT, (short)5);
private static final org.apache.thrift.protocol.TField BODY_IS_FIELD_DESC = new org.apache.thrift.protocol.TField("bodyIs", org.apache.thrift.protocol.TType.STRUCT, (short)6);
private static final org.apache.thrift.protocol.TField BODY_CONTAINS_FIELD_DESC = new org.apache.thrift.protocol.TField("bodyContains", org.apache.thrift.protocol.TType.STRUCT, (short)7);
private static final org.apache.thrift.protocol.TField BODY_DOES_NOT_CONTAIN_FIELD_DESC = new org.apache.thrift.protocol.TField("bodyDoesNotContain", org.apache.thrift.protocol.TType.STRUCT, (short)8);
private static final org.apache.thrift.protocol.TField URGENCY_EQUALS_FIELD_DESC = new org.apache.thrift.protocol.TField("urgencyEquals", org.apache.thrift.protocol.TType.STRUCT, (short)9);
private static final org.apache.thrift.protocol.TField HOSTNAME_IS_FIELD_DESC = new org.apache.thrift.protocol.TField("hostnameIs", org.apache.thrift.protocol.TType.STRUCT, (short)10);
private static final org.apache.thrift.protocol.TField HOSTNAME_CONTAINS_FIELD_DESC = new org.apache.thrift.protocol.TField("hostnameContains", org.apache.thrift.protocol.TType.STRUCT, (short)11);
private static final org.apache.thrift.protocol.TField HOSTNAME_DOES_NOT_CONTAIN_FIELD_DESC = new org.apache.thrift.protocol.TField("hostnameDoesNotContain", org.apache.thrift.protocol.TType.STRUCT, (short)12);
private static final org.apache.thrift.protocol.TField APPLICATION_IS_FIELD_DESC = new org.apache.thrift.protocol.TField("applicationIs", org.apache.thrift.protocol.TType.STRUCT, (short)13);
private static final org.apache.thrift.protocol.TField APPLICATION_IS_NOT_FIELD_DESC = new org.apache.thrift.protocol.TField("applicationIsNot", org.apache.thrift.protocol.TType.STRUCT, (short)14);
/** The set of fields this struct contains, along with convenience methods for finding and manipulating them. */
public enum _Fields implements org.apache.thrift.TFieldIdEnum {
ALL((short)1, "all"),
TITLE_IS((short)2, "titleIs"),
TITLE_IS_NOT((short)3, "titleIsNot"),
TITLE_CONTAINS((short)4, "titleContains"),
TITLE_DOES_NOT_CONTAIN((short)5, "titleDoesNotContain"),
BODY_IS((short)6, "bodyIs"),
BODY_CONTAINS((short)7, "bodyContains"),
BODY_DOES_NOT_CONTAIN((short)8, "bodyDoesNotContain"),
URGENCY_EQUALS((short)9, "urgencyEquals"),
HOSTNAME_IS((short)10, "hostnameIs"),
HOSTNAME_CONTAINS((short)11, "hostnameContains"),
HOSTNAME_DOES_NOT_CONTAIN((short)12, "hostnameDoesNotContain"),
APPLICATION_IS((short)13, "applicationIs"),
APPLICATION_IS_NOT((short)14, "applicationIsNot");
private static final Map byName = new HashMap();
static {
for (_Fields field : EnumSet.allOf(_Fields.class)) {
byName.put(field.getFieldName(), field);
}
}
/**
* Find the _Fields constant that matches fieldId, or null if its not found.
*/
public static _Fields findByThriftId(int fieldId) {
switch(fieldId) {
case 1: // ALL
return ALL;
case 2: // TITLE_IS
return TITLE_IS;
case 3: // TITLE_IS_NOT
return TITLE_IS_NOT;
case 4: // TITLE_CONTAINS
return TITLE_CONTAINS;
case 5: // TITLE_DOES_NOT_CONTAIN
return TITLE_DOES_NOT_CONTAIN;
case 6: // BODY_IS
return BODY_IS;
case 7: // BODY_CONTAINS
return BODY_CONTAINS;
case 8: // BODY_DOES_NOT_CONTAIN
return BODY_DOES_NOT_CONTAIN;
case 9: // URGENCY_EQUALS
return URGENCY_EQUALS;
case 10: // HOSTNAME_IS
return HOSTNAME_IS;
case 11: // HOSTNAME_CONTAINS
return HOSTNAME_CONTAINS;
case 12: // HOSTNAME_DOES_NOT_CONTAIN
return HOSTNAME_DOES_NOT_CONTAIN;
case 13: // APPLICATION_IS
return APPLICATION_IS;
case 14: // APPLICATION_IS_NOT
return APPLICATION_IS_NOT;
default:
return null;
}
}
/**
* Find the _Fields constant that matches fieldId, throwing an exception
* if it is not found.
*/
public static _Fields findByThriftIdOrThrow(int fieldId) {
_Fields fields = findByThriftId(fieldId);
if (fields == null) throw new IllegalArgumentException("Field " + fieldId + " doesn't exist!");
return fields;
}
/**
* Find the _Fields constant that matches name, or null if its not found.
*/
public static _Fields findByName(String name) {
return byName.get(name);
}
private final short _thriftId;
private final String _fieldName;
_Fields(short thriftId, String fieldName) {
_thriftId = thriftId;
_fieldName = fieldName;
}
public short getThriftFieldId() {
return _thriftId;
}
public String getFieldName() {
return _fieldName;
}
}
public static final Map<_Fields, org.apache.thrift.meta_data.FieldMetaData> metaDataMap;
static {
Map<_Fields, org.apache.thrift.meta_data.FieldMetaData> tmpMap = new EnumMap<_Fields, org.apache.thrift.meta_data.FieldMetaData>(_Fields.class);
tmpMap.put(_Fields.ALL, new org.apache.thrift.meta_data.FieldMetaData("all", org.apache.thrift.TFieldRequirementType.DEFAULT,
new org.apache.thrift.meta_data.StructMetaData(org.apache.thrift.protocol.TType.STRUCT, MatcherAll.class)));
tmpMap.put(_Fields.TITLE_IS, new org.apache.thrift.meta_data.FieldMetaData("titleIs", org.apache.thrift.TFieldRequirementType.DEFAULT,
new org.apache.thrift.meta_data.StructMetaData(org.apache.thrift.protocol.TType.STRUCT, MatcherTitleIs.class)));
tmpMap.put(_Fields.TITLE_IS_NOT, new org.apache.thrift.meta_data.FieldMetaData("titleIsNot", org.apache.thrift.TFieldRequirementType.DEFAULT,
new org.apache.thrift.meta_data.StructMetaData(org.apache.thrift.protocol.TType.STRUCT, MatcherTitleIsNot.class)));
tmpMap.put(_Fields.TITLE_CONTAINS, new org.apache.thrift.meta_data.FieldMetaData("titleContains", org.apache.thrift.TFieldRequirementType.DEFAULT,
new org.apache.thrift.meta_data.StructMetaData(org.apache.thrift.protocol.TType.STRUCT, MatcherTitleContains.class)));
tmpMap.put(_Fields.TITLE_DOES_NOT_CONTAIN, new org.apache.thrift.meta_data.FieldMetaData("titleDoesNotContain", org.apache.thrift.TFieldRequirementType.DEFAULT,
new org.apache.thrift.meta_data.StructMetaData(org.apache.thrift.protocol.TType.STRUCT, MatcherTitleDoesNotContain.class)));
tmpMap.put(_Fields.BODY_IS, new org.apache.thrift.meta_data.FieldMetaData("bodyIs", org.apache.thrift.TFieldRequirementType.DEFAULT,
new org.apache.thrift.meta_data.StructMetaData(org.apache.thrift.protocol.TType.STRUCT, MatcherBodyIs.class)));
tmpMap.put(_Fields.BODY_CONTAINS, new org.apache.thrift.meta_data.FieldMetaData("bodyContains", org.apache.thrift.TFieldRequirementType.DEFAULT,
new org.apache.thrift.meta_data.StructMetaData(org.apache.thrift.protocol.TType.STRUCT, MatcherBodyContains.class)));
tmpMap.put(_Fields.BODY_DOES_NOT_CONTAIN, new org.apache.thrift.meta_data.FieldMetaData("bodyDoesNotContain", org.apache.thrift.TFieldRequirementType.DEFAULT,
new org.apache.thrift.meta_data.StructMetaData(org.apache.thrift.protocol.TType.STRUCT, MatcherBodyDoesNotContain.class)));
tmpMap.put(_Fields.URGENCY_EQUALS, new org.apache.thrift.meta_data.FieldMetaData("urgencyEquals", org.apache.thrift.TFieldRequirementType.DEFAULT,
new org.apache.thrift.meta_data.StructMetaData(org.apache.thrift.protocol.TType.STRUCT, MatcherUrgencyIs.class)));
tmpMap.put(_Fields.HOSTNAME_IS, new org.apache.thrift.meta_data.FieldMetaData("hostnameIs", org.apache.thrift.TFieldRequirementType.DEFAULT,
new org.apache.thrift.meta_data.StructMetaData(org.apache.thrift.protocol.TType.STRUCT, MatcherHostnameIs.class)));
tmpMap.put(_Fields.HOSTNAME_CONTAINS, new org.apache.thrift.meta_data.FieldMetaData("hostnameContains", org.apache.thrift.TFieldRequirementType.DEFAULT,
new org.apache.thrift.meta_data.StructMetaData(org.apache.thrift.protocol.TType.STRUCT, MatcherHostnameContains.class)));
tmpMap.put(_Fields.HOSTNAME_DOES_NOT_CONTAIN, new org.apache.thrift.meta_data.FieldMetaData("hostnameDoesNotContain", org.apache.thrift.TFieldRequirementType.DEFAULT,
new org.apache.thrift.meta_data.StructMetaData(org.apache.thrift.protocol.TType.STRUCT, MatcherHostnameDoesNotContain.class)));
tmpMap.put(_Fields.APPLICATION_IS, new org.apache.thrift.meta_data.FieldMetaData("applicationIs", org.apache.thrift.TFieldRequirementType.DEFAULT,
new org.apache.thrift.meta_data.StructMetaData(org.apache.thrift.protocol.TType.STRUCT, MatcherApplicationIs.class)));
tmpMap.put(_Fields.APPLICATION_IS_NOT, new org.apache.thrift.meta_data.FieldMetaData("applicationIsNot", org.apache.thrift.TFieldRequirementType.DEFAULT,
new org.apache.thrift.meta_data.StructMetaData(org.apache.thrift.protocol.TType.STRUCT, MatcherApplicationIsNot.class)));
metaDataMap = Collections.unmodifiableMap(tmpMap);
org.apache.thrift.meta_data.FieldMetaData.addStructMetaDataMap(AromaMatcher.class, metaDataMap);
}
public AromaMatcher() {
super();
}
public AromaMatcher(_Fields setField, Object value) {
super(setField, value);
}
public AromaMatcher(AromaMatcher other) {
super(other);
}
public AromaMatcher deepCopy() {
return new AromaMatcher(this);
}
public static AromaMatcher all(MatcherAll value) {
AromaMatcher x = new AromaMatcher();
x.setAll(value);
return x;
}
public static AromaMatcher titleIs(MatcherTitleIs value) {
AromaMatcher x = new AromaMatcher();
x.setTitleIs(value);
return x;
}
public static AromaMatcher titleIsNot(MatcherTitleIsNot value) {
AromaMatcher x = new AromaMatcher();
x.setTitleIsNot(value);
return x;
}
public static AromaMatcher titleContains(MatcherTitleContains value) {
AromaMatcher x = new AromaMatcher();
x.setTitleContains(value);
return x;
}
public static AromaMatcher titleDoesNotContain(MatcherTitleDoesNotContain value) {
AromaMatcher x = new AromaMatcher();
x.setTitleDoesNotContain(value);
return x;
}
public static AromaMatcher bodyIs(MatcherBodyIs value) {
AromaMatcher x = new AromaMatcher();
x.setBodyIs(value);
return x;
}
public static AromaMatcher bodyContains(MatcherBodyContains value) {
AromaMatcher x = new AromaMatcher();
x.setBodyContains(value);
return x;
}
public static AromaMatcher bodyDoesNotContain(MatcherBodyDoesNotContain value) {
AromaMatcher x = new AromaMatcher();
x.setBodyDoesNotContain(value);
return x;
}
public static AromaMatcher urgencyEquals(MatcherUrgencyIs value) {
AromaMatcher x = new AromaMatcher();
x.setUrgencyEquals(value);
return x;
}
public static AromaMatcher hostnameIs(MatcherHostnameIs value) {
AromaMatcher x = new AromaMatcher();
x.setHostnameIs(value);
return x;
}
public static AromaMatcher hostnameContains(MatcherHostnameContains value) {
AromaMatcher x = new AromaMatcher();
x.setHostnameContains(value);
return x;
}
public static AromaMatcher hostnameDoesNotContain(MatcherHostnameDoesNotContain value) {
AromaMatcher x = new AromaMatcher();
x.setHostnameDoesNotContain(value);
return x;
}
public static AromaMatcher applicationIs(MatcherApplicationIs value) {
AromaMatcher x = new AromaMatcher();
x.setApplicationIs(value);
return x;
}
public static AromaMatcher applicationIsNot(MatcherApplicationIsNot value) {
AromaMatcher x = new AromaMatcher();
x.setApplicationIsNot(value);
return x;
}
@Override
protected void checkType(_Fields setField, Object value) throws ClassCastException {
switch (setField) {
case ALL:
if (value instanceof MatcherAll) {
break;
}
throw new ClassCastException("Was expecting value of type MatcherAll for field 'all', but got " + value.getClass().getSimpleName());
case TITLE_IS:
if (value instanceof MatcherTitleIs) {
break;
}
throw new ClassCastException("Was expecting value of type MatcherTitleIs for field 'titleIs', but got " + value.getClass().getSimpleName());
case TITLE_IS_NOT:
if (value instanceof MatcherTitleIsNot) {
break;
}
throw new ClassCastException("Was expecting value of type MatcherTitleIsNot for field 'titleIsNot', but got " + value.getClass().getSimpleName());
case TITLE_CONTAINS:
if (value instanceof MatcherTitleContains) {
break;
}
throw new ClassCastException("Was expecting value of type MatcherTitleContains for field 'titleContains', but got " + value.getClass().getSimpleName());
case TITLE_DOES_NOT_CONTAIN:
if (value instanceof MatcherTitleDoesNotContain) {
break;
}
throw new ClassCastException("Was expecting value of type MatcherTitleDoesNotContain for field 'titleDoesNotContain', but got " + value.getClass().getSimpleName());
case BODY_IS:
if (value instanceof MatcherBodyIs) {
break;
}
throw new ClassCastException("Was expecting value of type MatcherBodyIs for field 'bodyIs', but got " + value.getClass().getSimpleName());
case BODY_CONTAINS:
if (value instanceof MatcherBodyContains) {
break;
}
throw new ClassCastException("Was expecting value of type MatcherBodyContains for field 'bodyContains', but got " + value.getClass().getSimpleName());
case BODY_DOES_NOT_CONTAIN:
if (value instanceof MatcherBodyDoesNotContain) {
break;
}
throw new ClassCastException("Was expecting value of type MatcherBodyDoesNotContain for field 'bodyDoesNotContain', but got " + value.getClass().getSimpleName());
case URGENCY_EQUALS:
if (value instanceof MatcherUrgencyIs) {
break;
}
throw new ClassCastException("Was expecting value of type MatcherUrgencyIs for field 'urgencyEquals', but got " + value.getClass().getSimpleName());
case HOSTNAME_IS:
if (value instanceof MatcherHostnameIs) {
break;
}
throw new ClassCastException("Was expecting value of type MatcherHostnameIs for field 'hostnameIs', but got " + value.getClass().getSimpleName());
case HOSTNAME_CONTAINS:
if (value instanceof MatcherHostnameContains) {
break;
}
throw new ClassCastException("Was expecting value of type MatcherHostnameContains for field 'hostnameContains', but got " + value.getClass().getSimpleName());
case HOSTNAME_DOES_NOT_CONTAIN:
if (value instanceof MatcherHostnameDoesNotContain) {
break;
}
throw new ClassCastException("Was expecting value of type MatcherHostnameDoesNotContain for field 'hostnameDoesNotContain', but got " + value.getClass().getSimpleName());
case APPLICATION_IS:
if (value instanceof MatcherApplicationIs) {
break;
}
throw new ClassCastException("Was expecting value of type MatcherApplicationIs for field 'applicationIs', but got " + value.getClass().getSimpleName());
case APPLICATION_IS_NOT:
if (value instanceof MatcherApplicationIsNot) {
break;
}
throw new ClassCastException("Was expecting value of type MatcherApplicationIsNot for field 'applicationIsNot', but got " + value.getClass().getSimpleName());
default:
throw new IllegalArgumentException("Unknown field id " + setField);
}
}
@Override
protected Object standardSchemeReadValue(org.apache.thrift.protocol.TProtocol iprot, org.apache.thrift.protocol.TField field) throws org.apache.thrift.TException {
_Fields setField = _Fields.findByThriftId(field.id);
if (setField != null) {
switch (setField) {
case ALL:
if (field.type == ALL_FIELD_DESC.type) {
MatcherAll all;
all = new MatcherAll();
all.read(iprot);
return all;
} else {
org.apache.thrift.protocol.TProtocolUtil.skip(iprot, field.type);
return null;
}
case TITLE_IS:
if (field.type == TITLE_IS_FIELD_DESC.type) {
MatcherTitleIs titleIs;
titleIs = new MatcherTitleIs();
titleIs.read(iprot);
return titleIs;
} else {
org.apache.thrift.protocol.TProtocolUtil.skip(iprot, field.type);
return null;
}
case TITLE_IS_NOT:
if (field.type == TITLE_IS_NOT_FIELD_DESC.type) {
MatcherTitleIsNot titleIsNot;
titleIsNot = new MatcherTitleIsNot();
titleIsNot.read(iprot);
return titleIsNot;
} else {
org.apache.thrift.protocol.TProtocolUtil.skip(iprot, field.type);
return null;
}
case TITLE_CONTAINS:
if (field.type == TITLE_CONTAINS_FIELD_DESC.type) {
MatcherTitleContains titleContains;
titleContains = new MatcherTitleContains();
titleContains.read(iprot);
return titleContains;
} else {
org.apache.thrift.protocol.TProtocolUtil.skip(iprot, field.type);
return null;
}
case TITLE_DOES_NOT_CONTAIN:
if (field.type == TITLE_DOES_NOT_CONTAIN_FIELD_DESC.type) {
MatcherTitleDoesNotContain titleDoesNotContain;
titleDoesNotContain = new MatcherTitleDoesNotContain();
titleDoesNotContain.read(iprot);
return titleDoesNotContain;
} else {
org.apache.thrift.protocol.TProtocolUtil.skip(iprot, field.type);
return null;
}
case BODY_IS:
if (field.type == BODY_IS_FIELD_DESC.type) {
MatcherBodyIs bodyIs;
bodyIs = new MatcherBodyIs();
bodyIs.read(iprot);
return bodyIs;
} else {
org.apache.thrift.protocol.TProtocolUtil.skip(iprot, field.type);
return null;
}
case BODY_CONTAINS:
if (field.type == BODY_CONTAINS_FIELD_DESC.type) {
MatcherBodyContains bodyContains;
bodyContains = new MatcherBodyContains();
bodyContains.read(iprot);
return bodyContains;
} else {
org.apache.thrift.protocol.TProtocolUtil.skip(iprot, field.type);
return null;
}
case BODY_DOES_NOT_CONTAIN:
if (field.type == BODY_DOES_NOT_CONTAIN_FIELD_DESC.type) {
MatcherBodyDoesNotContain bodyDoesNotContain;
bodyDoesNotContain = new MatcherBodyDoesNotContain();
bodyDoesNotContain.read(iprot);
return bodyDoesNotContain;
} else {
org.apache.thrift.protocol.TProtocolUtil.skip(iprot, field.type);
return null;
}
case URGENCY_EQUALS:
if (field.type == URGENCY_EQUALS_FIELD_DESC.type) {
MatcherUrgencyIs urgencyEquals;
urgencyEquals = new MatcherUrgencyIs();
urgencyEquals.read(iprot);
return urgencyEquals;
} else {
org.apache.thrift.protocol.TProtocolUtil.skip(iprot, field.type);
return null;
}
case HOSTNAME_IS:
if (field.type == HOSTNAME_IS_FIELD_DESC.type) {
MatcherHostnameIs hostnameIs;
hostnameIs = new MatcherHostnameIs();
hostnameIs.read(iprot);
return hostnameIs;
} else {
org.apache.thrift.protocol.TProtocolUtil.skip(iprot, field.type);
return null;
}
case HOSTNAME_CONTAINS:
if (field.type == HOSTNAME_CONTAINS_FIELD_DESC.type) {
MatcherHostnameContains hostnameContains;
hostnameContains = new MatcherHostnameContains();
hostnameContains.read(iprot);
return hostnameContains;
} else {
org.apache.thrift.protocol.TProtocolUtil.skip(iprot, field.type);
return null;
}
case HOSTNAME_DOES_NOT_CONTAIN:
if (field.type == HOSTNAME_DOES_NOT_CONTAIN_FIELD_DESC.type) {
MatcherHostnameDoesNotContain hostnameDoesNotContain;
hostnameDoesNotContain = new MatcherHostnameDoesNotContain();
hostnameDoesNotContain.read(iprot);
return hostnameDoesNotContain;
} else {
org.apache.thrift.protocol.TProtocolUtil.skip(iprot, field.type);
return null;
}
case APPLICATION_IS:
if (field.type == APPLICATION_IS_FIELD_DESC.type) {
MatcherApplicationIs applicationIs;
applicationIs = new MatcherApplicationIs();
applicationIs.read(iprot);
return applicationIs;
} else {
org.apache.thrift.protocol.TProtocolUtil.skip(iprot, field.type);
return null;
}
case APPLICATION_IS_NOT:
if (field.type == APPLICATION_IS_NOT_FIELD_DESC.type) {
MatcherApplicationIsNot applicationIsNot;
applicationIsNot = new MatcherApplicationIsNot();
applicationIsNot.read(iprot);
return applicationIsNot;
} else {
org.apache.thrift.protocol.TProtocolUtil.skip(iprot, field.type);
return null;
}
default:
throw new IllegalStateException("setField wasn't null, but didn't match any of the case statements!");
}
} else {
org.apache.thrift.protocol.TProtocolUtil.skip(iprot, field.type);
return null;
}
}
@Override
protected void standardSchemeWriteValue(org.apache.thrift.protocol.TProtocol oprot) throws org.apache.thrift.TException {
switch (setField_) {
case ALL:
MatcherAll all = (MatcherAll)value_;
all.write(oprot);
return;
case TITLE_IS:
MatcherTitleIs titleIs = (MatcherTitleIs)value_;
titleIs.write(oprot);
return;
case TITLE_IS_NOT:
MatcherTitleIsNot titleIsNot = (MatcherTitleIsNot)value_;
titleIsNot.write(oprot);
return;
case TITLE_CONTAINS:
MatcherTitleContains titleContains = (MatcherTitleContains)value_;
titleContains.write(oprot);
return;
case TITLE_DOES_NOT_CONTAIN:
MatcherTitleDoesNotContain titleDoesNotContain = (MatcherTitleDoesNotContain)value_;
titleDoesNotContain.write(oprot);
return;
case BODY_IS:
MatcherBodyIs bodyIs = (MatcherBodyIs)value_;
bodyIs.write(oprot);
return;
case BODY_CONTAINS:
MatcherBodyContains bodyContains = (MatcherBodyContains)value_;
bodyContains.write(oprot);
return;
case BODY_DOES_NOT_CONTAIN:
MatcherBodyDoesNotContain bodyDoesNotContain = (MatcherBodyDoesNotContain)value_;
bodyDoesNotContain.write(oprot);
return;
case URGENCY_EQUALS:
MatcherUrgencyIs urgencyEquals = (MatcherUrgencyIs)value_;
urgencyEquals.write(oprot);
return;
case HOSTNAME_IS:
MatcherHostnameIs hostnameIs = (MatcherHostnameIs)value_;
hostnameIs.write(oprot);
return;
case HOSTNAME_CONTAINS:
MatcherHostnameContains hostnameContains = (MatcherHostnameContains)value_;
hostnameContains.write(oprot);
return;
case HOSTNAME_DOES_NOT_CONTAIN:
MatcherHostnameDoesNotContain hostnameDoesNotContain = (MatcherHostnameDoesNotContain)value_;
hostnameDoesNotContain.write(oprot);
return;
case APPLICATION_IS:
MatcherApplicationIs applicationIs = (MatcherApplicationIs)value_;
applicationIs.write(oprot);
return;
case APPLICATION_IS_NOT:
MatcherApplicationIsNot applicationIsNot = (MatcherApplicationIsNot)value_;
applicationIsNot.write(oprot);
return;
default:
throw new IllegalStateException("Cannot write union with unknown field " + setField_);
}
}
@Override
protected Object tupleSchemeReadValue(org.apache.thrift.protocol.TProtocol iprot, short fieldID) throws org.apache.thrift.TException {
_Fields setField = _Fields.findByThriftId(fieldID);
if (setField != null) {
switch (setField) {
case ALL:
MatcherAll all;
all = new MatcherAll();
all.read(iprot);
return all;
case TITLE_IS:
MatcherTitleIs titleIs;
titleIs = new MatcherTitleIs();
titleIs.read(iprot);
return titleIs;
case TITLE_IS_NOT:
MatcherTitleIsNot titleIsNot;
titleIsNot = new MatcherTitleIsNot();
titleIsNot.read(iprot);
return titleIsNot;
case TITLE_CONTAINS:
MatcherTitleContains titleContains;
titleContains = new MatcherTitleContains();
titleContains.read(iprot);
return titleContains;
case TITLE_DOES_NOT_CONTAIN:
MatcherTitleDoesNotContain titleDoesNotContain;
titleDoesNotContain = new MatcherTitleDoesNotContain();
titleDoesNotContain.read(iprot);
return titleDoesNotContain;
case BODY_IS:
MatcherBodyIs bodyIs;
bodyIs = new MatcherBodyIs();
bodyIs.read(iprot);
return bodyIs;
case BODY_CONTAINS:
MatcherBodyContains bodyContains;
bodyContains = new MatcherBodyContains();
bodyContains.read(iprot);
return bodyContains;
case BODY_DOES_NOT_CONTAIN:
MatcherBodyDoesNotContain bodyDoesNotContain;
bodyDoesNotContain = new MatcherBodyDoesNotContain();
bodyDoesNotContain.read(iprot);
return bodyDoesNotContain;
case URGENCY_EQUALS:
MatcherUrgencyIs urgencyEquals;
urgencyEquals = new MatcherUrgencyIs();
urgencyEquals.read(iprot);
return urgencyEquals;
case HOSTNAME_IS:
MatcherHostnameIs hostnameIs;
hostnameIs = new MatcherHostnameIs();
hostnameIs.read(iprot);
return hostnameIs;
case HOSTNAME_CONTAINS:
MatcherHostnameContains hostnameContains;
hostnameContains = new MatcherHostnameContains();
hostnameContains.read(iprot);
return hostnameContains;
case HOSTNAME_DOES_NOT_CONTAIN:
MatcherHostnameDoesNotContain hostnameDoesNotContain;
hostnameDoesNotContain = new MatcherHostnameDoesNotContain();
hostnameDoesNotContain.read(iprot);
return hostnameDoesNotContain;
case APPLICATION_IS:
MatcherApplicationIs applicationIs;
applicationIs = new MatcherApplicationIs();
applicationIs.read(iprot);
return applicationIs;
case APPLICATION_IS_NOT:
MatcherApplicationIsNot applicationIsNot;
applicationIsNot = new MatcherApplicationIsNot();
applicationIsNot.read(iprot);
return applicationIsNot;
default:
throw new IllegalStateException("setField wasn't null, but didn't match any of the case statements!");
}
} else {
throw new TProtocolException("Couldn't find a field with field id " + fieldID);
}
}
@Override
protected void tupleSchemeWriteValue(org.apache.thrift.protocol.TProtocol oprot) throws org.apache.thrift.TException {
switch (setField_) {
case ALL:
MatcherAll all = (MatcherAll)value_;
all.write(oprot);
return;
case TITLE_IS:
MatcherTitleIs titleIs = (MatcherTitleIs)value_;
titleIs.write(oprot);
return;
case TITLE_IS_NOT:
MatcherTitleIsNot titleIsNot = (MatcherTitleIsNot)value_;
titleIsNot.write(oprot);
return;
case TITLE_CONTAINS:
MatcherTitleContains titleContains = (MatcherTitleContains)value_;
titleContains.write(oprot);
return;
case TITLE_DOES_NOT_CONTAIN:
MatcherTitleDoesNotContain titleDoesNotContain = (MatcherTitleDoesNotContain)value_;
titleDoesNotContain.write(oprot);
return;
case BODY_IS:
MatcherBodyIs bodyIs = (MatcherBodyIs)value_;
bodyIs.write(oprot);
return;
case BODY_CONTAINS:
MatcherBodyContains bodyContains = (MatcherBodyContains)value_;
bodyContains.write(oprot);
return;
case BODY_DOES_NOT_CONTAIN:
MatcherBodyDoesNotContain bodyDoesNotContain = (MatcherBodyDoesNotContain)value_;
bodyDoesNotContain.write(oprot);
return;
case URGENCY_EQUALS:
MatcherUrgencyIs urgencyEquals = (MatcherUrgencyIs)value_;
urgencyEquals.write(oprot);
return;
case HOSTNAME_IS:
MatcherHostnameIs hostnameIs = (MatcherHostnameIs)value_;
hostnameIs.write(oprot);
return;
case HOSTNAME_CONTAINS:
MatcherHostnameContains hostnameContains = (MatcherHostnameContains)value_;
hostnameContains.write(oprot);
return;
case HOSTNAME_DOES_NOT_CONTAIN:
MatcherHostnameDoesNotContain hostnameDoesNotContain = (MatcherHostnameDoesNotContain)value_;
hostnameDoesNotContain.write(oprot);
return;
case APPLICATION_IS:
MatcherApplicationIs applicationIs = (MatcherApplicationIs)value_;
applicationIs.write(oprot);
return;
case APPLICATION_IS_NOT:
MatcherApplicationIsNot applicationIsNot = (MatcherApplicationIsNot)value_;
applicationIsNot.write(oprot);
return;
default:
throw new IllegalStateException("Cannot write union with unknown field " + setField_);
}
}
@Override
protected org.apache.thrift.protocol.TField getFieldDesc(_Fields setField) {
switch (setField) {
case ALL:
return ALL_FIELD_DESC;
case TITLE_IS:
return TITLE_IS_FIELD_DESC;
case TITLE_IS_NOT:
return TITLE_IS_NOT_FIELD_DESC;
case TITLE_CONTAINS:
return TITLE_CONTAINS_FIELD_DESC;
case TITLE_DOES_NOT_CONTAIN:
return TITLE_DOES_NOT_CONTAIN_FIELD_DESC;
case BODY_IS:
return BODY_IS_FIELD_DESC;
case BODY_CONTAINS:
return BODY_CONTAINS_FIELD_DESC;
case BODY_DOES_NOT_CONTAIN:
return BODY_DOES_NOT_CONTAIN_FIELD_DESC;
case URGENCY_EQUALS:
return URGENCY_EQUALS_FIELD_DESC;
case HOSTNAME_IS:
return HOSTNAME_IS_FIELD_DESC;
case HOSTNAME_CONTAINS:
return HOSTNAME_CONTAINS_FIELD_DESC;
case HOSTNAME_DOES_NOT_CONTAIN:
return HOSTNAME_DOES_NOT_CONTAIN_FIELD_DESC;
case APPLICATION_IS:
return APPLICATION_IS_FIELD_DESC;
case APPLICATION_IS_NOT:
return APPLICATION_IS_NOT_FIELD_DESC;
default:
throw new IllegalArgumentException("Unknown field id " + setField);
}
}
@Override
protected org.apache.thrift.protocol.TStruct getStructDesc() {
return STRUCT_DESC;
}
@Override
protected _Fields enumForId(short id) {
return _Fields.findByThriftIdOrThrow(id);
}
public _Fields fieldForId(int fieldId) {
return _Fields.findByThriftId(fieldId);
}
public MatcherAll getAll() {
if (getSetField() == _Fields.ALL) {
return (MatcherAll)getFieldValue();
} else {
throw new RuntimeException("Cannot get field 'all' because union is currently set to " + getFieldDesc(getSetField()).name);
}
}
public void setAll(MatcherAll value) {
if (value == null) throw new NullPointerException();
setField_ = _Fields.ALL;
value_ = value;
}
public MatcherTitleIs getTitleIs() {
if (getSetField() == _Fields.TITLE_IS) {
return (MatcherTitleIs)getFieldValue();
} else {
throw new RuntimeException("Cannot get field 'titleIs' because union is currently set to " + getFieldDesc(getSetField()).name);
}
}
public void setTitleIs(MatcherTitleIs value) {
if (value == null) throw new NullPointerException();
setField_ = _Fields.TITLE_IS;
value_ = value;
}
public MatcherTitleIsNot getTitleIsNot() {
if (getSetField() == _Fields.TITLE_IS_NOT) {
return (MatcherTitleIsNot)getFieldValue();
} else {
throw new RuntimeException("Cannot get field 'titleIsNot' because union is currently set to " + getFieldDesc(getSetField()).name);
}
}
public void setTitleIsNot(MatcherTitleIsNot value) {
if (value == null) throw new NullPointerException();
setField_ = _Fields.TITLE_IS_NOT;
value_ = value;
}
public MatcherTitleContains getTitleContains() {
if (getSetField() == _Fields.TITLE_CONTAINS) {
return (MatcherTitleContains)getFieldValue();
} else {
throw new RuntimeException("Cannot get field 'titleContains' because union is currently set to " + getFieldDesc(getSetField()).name);
}
}
public void setTitleContains(MatcherTitleContains value) {
if (value == null) throw new NullPointerException();
setField_ = _Fields.TITLE_CONTAINS;
value_ = value;
}
public MatcherTitleDoesNotContain getTitleDoesNotContain() {
if (getSetField() == _Fields.TITLE_DOES_NOT_CONTAIN) {
return (MatcherTitleDoesNotContain)getFieldValue();
} else {
throw new RuntimeException("Cannot get field 'titleDoesNotContain' because union is currently set to " + getFieldDesc(getSetField()).name);
}
}
public void setTitleDoesNotContain(MatcherTitleDoesNotContain value) {
if (value == null) throw new NullPointerException();
setField_ = _Fields.TITLE_DOES_NOT_CONTAIN;
value_ = value;
}
public MatcherBodyIs getBodyIs() {
if (getSetField() == _Fields.BODY_IS) {
return (MatcherBodyIs)getFieldValue();
} else {
throw new RuntimeException("Cannot get field 'bodyIs' because union is currently set to " + getFieldDesc(getSetField()).name);
}
}
public void setBodyIs(MatcherBodyIs value) {
if (value == null) throw new NullPointerException();
setField_ = _Fields.BODY_IS;
value_ = value;
}
public MatcherBodyContains getBodyContains() {
if (getSetField() == _Fields.BODY_CONTAINS) {
return (MatcherBodyContains)getFieldValue();
} else {
throw new RuntimeException("Cannot get field 'bodyContains' because union is currently set to " + getFieldDesc(getSetField()).name);
}
}
public void setBodyContains(MatcherBodyContains value) {
if (value == null) throw new NullPointerException();
setField_ = _Fields.BODY_CONTAINS;
value_ = value;
}
public MatcherBodyDoesNotContain getBodyDoesNotContain() {
if (getSetField() == _Fields.BODY_DOES_NOT_CONTAIN) {
return (MatcherBodyDoesNotContain)getFieldValue();
} else {
throw new RuntimeException("Cannot get field 'bodyDoesNotContain' because union is currently set to " + getFieldDesc(getSetField()).name);
}
}
public void setBodyDoesNotContain(MatcherBodyDoesNotContain value) {
if (value == null) throw new NullPointerException();
setField_ = _Fields.BODY_DOES_NOT_CONTAIN;
value_ = value;
}
public MatcherUrgencyIs getUrgencyEquals() {
if (getSetField() == _Fields.URGENCY_EQUALS) {
return (MatcherUrgencyIs)getFieldValue();
} else {
throw new RuntimeException("Cannot get field 'urgencyEquals' because union is currently set to " + getFieldDesc(getSetField()).name);
}
}
public void setUrgencyEquals(MatcherUrgencyIs value) {
if (value == null) throw new NullPointerException();
setField_ = _Fields.URGENCY_EQUALS;
value_ = value;
}
public MatcherHostnameIs getHostnameIs() {
if (getSetField() == _Fields.HOSTNAME_IS) {
return (MatcherHostnameIs)getFieldValue();
} else {
throw new RuntimeException("Cannot get field 'hostnameIs' because union is currently set to " + getFieldDesc(getSetField()).name);
}
}
public void setHostnameIs(MatcherHostnameIs value) {
if (value == null) throw new NullPointerException();
setField_ = _Fields.HOSTNAME_IS;
value_ = value;
}
public MatcherHostnameContains getHostnameContains() {
if (getSetField() == _Fields.HOSTNAME_CONTAINS) {
return (MatcherHostnameContains)getFieldValue();
} else {
throw new RuntimeException("Cannot get field 'hostnameContains' because union is currently set to " + getFieldDesc(getSetField()).name);
}
}
public void setHostnameContains(MatcherHostnameContains value) {
if (value == null) throw new NullPointerException();
setField_ = _Fields.HOSTNAME_CONTAINS;
value_ = value;
}
public MatcherHostnameDoesNotContain getHostnameDoesNotContain() {
if (getSetField() == _Fields.HOSTNAME_DOES_NOT_CONTAIN) {
return (MatcherHostnameDoesNotContain)getFieldValue();
} else {
throw new RuntimeException("Cannot get field 'hostnameDoesNotContain' because union is currently set to " + getFieldDesc(getSetField()).name);
}
}
public void setHostnameDoesNotContain(MatcherHostnameDoesNotContain value) {
if (value == null) throw new NullPointerException();
setField_ = _Fields.HOSTNAME_DOES_NOT_CONTAIN;
value_ = value;
}
public MatcherApplicationIs getApplicationIs() {
if (getSetField() == _Fields.APPLICATION_IS) {
return (MatcherApplicationIs)getFieldValue();
} else {
throw new RuntimeException("Cannot get field 'applicationIs' because union is currently set to " + getFieldDesc(getSetField()).name);
}
}
public void setApplicationIs(MatcherApplicationIs value) {
if (value == null) throw new NullPointerException();
setField_ = _Fields.APPLICATION_IS;
value_ = value;
}
public MatcherApplicationIsNot getApplicationIsNot() {
if (getSetField() == _Fields.APPLICATION_IS_NOT) {
return (MatcherApplicationIsNot)getFieldValue();
} else {
throw new RuntimeException("Cannot get field 'applicationIsNot' because union is currently set to " + getFieldDesc(getSetField()).name);
}
}
public void setApplicationIsNot(MatcherApplicationIsNot value) {
if (value == null) throw new NullPointerException();
setField_ = _Fields.APPLICATION_IS_NOT;
value_ = value;
}
public boolean isSetAll() {
return setField_ == _Fields.ALL;
}
public boolean isSetTitleIs() {
return setField_ == _Fields.TITLE_IS;
}
public boolean isSetTitleIsNot() {
return setField_ == _Fields.TITLE_IS_NOT;
}
public boolean isSetTitleContains() {
return setField_ == _Fields.TITLE_CONTAINS;
}
public boolean isSetTitleDoesNotContain() {
return setField_ == _Fields.TITLE_DOES_NOT_CONTAIN;
}
public boolean isSetBodyIs() {
return setField_ == _Fields.BODY_IS;
}
public boolean isSetBodyContains() {
return setField_ == _Fields.BODY_CONTAINS;
}
public boolean isSetBodyDoesNotContain() {
return setField_ == _Fields.BODY_DOES_NOT_CONTAIN;
}
public boolean isSetUrgencyEquals() {
return setField_ == _Fields.URGENCY_EQUALS;
}
public boolean isSetHostnameIs() {
return setField_ == _Fields.HOSTNAME_IS;
}
public boolean isSetHostnameContains() {
return setField_ == _Fields.HOSTNAME_CONTAINS;
}
public boolean isSetHostnameDoesNotContain() {
return setField_ == _Fields.HOSTNAME_DOES_NOT_CONTAIN;
}
public boolean isSetApplicationIs() {
return setField_ == _Fields.APPLICATION_IS;
}
public boolean isSetApplicationIsNot() {
return setField_ == _Fields.APPLICATION_IS_NOT;
}
public boolean equals(Object other) {
if (other instanceof AromaMatcher) {
return equals((AromaMatcher)other);
} else {
return false;
}
}
public boolean equals(AromaMatcher other) {
return other != null && getSetField() == other.getSetField() && getFieldValue().equals(other.getFieldValue());
}
@Override
public int compareTo(AromaMatcher other) {
int lastComparison = org.apache.thrift.TBaseHelper.compareTo(getSetField(), other.getSetField());
if (lastComparison == 0) {
return org.apache.thrift.TBaseHelper.compareTo(getFieldValue(), other.getFieldValue());
}
return lastComparison;
}
@Override
public int hashCode() {
List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy