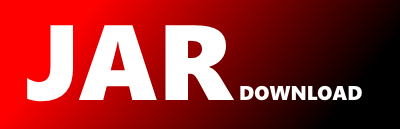
tech.aroma.thrift.reactions.Reaction Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aroma-thrift Show documentation
Show all versions of aroma-thrift Show documentation
Part of the Aroma Project.
This project contains the Service and Model Definitions.
From this the Server and Client interfaces are generated for the Aroma Service.
/**
* Autogenerated by Thrift Compiler (0.9.3)
*
* DO NOT EDIT UNLESS YOU ARE SURE THAT YOU KNOW WHAT YOU ARE DOING
* @generated
*/
package tech.aroma.thrift.reactions;
import org.apache.thrift.scheme.IScheme;
import org.apache.thrift.scheme.SchemeFactory;
import org.apache.thrift.scheme.StandardScheme;
import org.apache.thrift.scheme.TupleScheme;
import org.apache.thrift.protocol.TTupleProtocol;
import org.apache.thrift.protocol.TProtocolException;
import org.apache.thrift.EncodingUtils;
import org.apache.thrift.TException;
import org.apache.thrift.async.AsyncMethodCallback;
import org.apache.thrift.server.AbstractNonblockingServer.*;
import java.util.List;
import java.util.ArrayList;
import java.util.Map;
import java.util.HashMap;
import java.util.EnumMap;
import java.util.Set;
import java.util.HashSet;
import java.util.EnumSet;
import java.util.Collections;
import java.util.BitSet;
import java.nio.ByteBuffer;
import java.util.Arrays;
import javax.annotation.Generated;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
@SuppressWarnings({"cast", "rawtypes", "serial", "unchecked"})
@Generated(value = "Autogenerated by Thrift Compiler (0.9.3)", date = "2016-05-10")
public class Reaction implements org.apache.thrift.TBase, java.io.Serializable, Cloneable, Comparable {
private static final org.apache.thrift.protocol.TStruct STRUCT_DESC = new org.apache.thrift.protocol.TStruct("Reaction");
private static final org.apache.thrift.protocol.TField MATCHERS_FIELD_DESC = new org.apache.thrift.protocol.TField("matchers", org.apache.thrift.protocol.TType.LIST, (short)1);
private static final org.apache.thrift.protocol.TField ACTIONS_FIELD_DESC = new org.apache.thrift.protocol.TField("actions", org.apache.thrift.protocol.TType.LIST, (short)2);
private static final org.apache.thrift.protocol.TField NAME_FIELD_DESC = new org.apache.thrift.protocol.TField("name", org.apache.thrift.protocol.TType.STRING, (short)3);
private static final Map, SchemeFactory> schemes = new HashMap, SchemeFactory>();
static {
schemes.put(StandardScheme.class, new ReactionStandardSchemeFactory());
schemes.put(TupleScheme.class, new ReactionTupleSchemeFactory());
}
public List matchers; // required
public List actions; // required
public String name; // required
/** The set of fields this struct contains, along with convenience methods for finding and manipulating them. */
public enum _Fields implements org.apache.thrift.TFieldIdEnum {
MATCHERS((short)1, "matchers"),
ACTIONS((short)2, "actions"),
NAME((short)3, "name");
private static final Map byName = new HashMap();
static {
for (_Fields field : EnumSet.allOf(_Fields.class)) {
byName.put(field.getFieldName(), field);
}
}
/**
* Find the _Fields constant that matches fieldId, or null if its not found.
*/
public static _Fields findByThriftId(int fieldId) {
switch(fieldId) {
case 1: // MATCHERS
return MATCHERS;
case 2: // ACTIONS
return ACTIONS;
case 3: // NAME
return NAME;
default:
return null;
}
}
/**
* Find the _Fields constant that matches fieldId, throwing an exception
* if it is not found.
*/
public static _Fields findByThriftIdOrThrow(int fieldId) {
_Fields fields = findByThriftId(fieldId);
if (fields == null) throw new IllegalArgumentException("Field " + fieldId + " doesn't exist!");
return fields;
}
/**
* Find the _Fields constant that matches name, or null if its not found.
*/
public static _Fields findByName(String name) {
return byName.get(name);
}
private final short _thriftId;
private final String _fieldName;
_Fields(short thriftId, String fieldName) {
_thriftId = thriftId;
_fieldName = fieldName;
}
public short getThriftFieldId() {
return _thriftId;
}
public String getFieldName() {
return _fieldName;
}
}
// isset id assignments
public static final Map<_Fields, org.apache.thrift.meta_data.FieldMetaData> metaDataMap;
static {
Map<_Fields, org.apache.thrift.meta_data.FieldMetaData> tmpMap = new EnumMap<_Fields, org.apache.thrift.meta_data.FieldMetaData>(_Fields.class);
tmpMap.put(_Fields.MATCHERS, new org.apache.thrift.meta_data.FieldMetaData("matchers", org.apache.thrift.TFieldRequirementType.DEFAULT,
new org.apache.thrift.meta_data.ListMetaData(org.apache.thrift.protocol.TType.LIST,
new org.apache.thrift.meta_data.StructMetaData(org.apache.thrift.protocol.TType.STRUCT, AromaMatcher.class))));
tmpMap.put(_Fields.ACTIONS, new org.apache.thrift.meta_data.FieldMetaData("actions", org.apache.thrift.TFieldRequirementType.DEFAULT,
new org.apache.thrift.meta_data.ListMetaData(org.apache.thrift.protocol.TType.LIST,
new org.apache.thrift.meta_data.StructMetaData(org.apache.thrift.protocol.TType.STRUCT, AromaAction.class))));
tmpMap.put(_Fields.NAME, new org.apache.thrift.meta_data.FieldMetaData("name", org.apache.thrift.TFieldRequirementType.DEFAULT,
new org.apache.thrift.meta_data.FieldValueMetaData(org.apache.thrift.protocol.TType.STRING)));
metaDataMap = Collections.unmodifiableMap(tmpMap);
org.apache.thrift.meta_data.FieldMetaData.addStructMetaDataMap(Reaction.class, metaDataMap);
}
public Reaction() {
this.matchers = new ArrayList();
this.actions = new ArrayList();
}
public Reaction(
List matchers,
List actions,
String name)
{
this();
this.matchers = matchers;
this.actions = actions;
this.name = name;
}
/**
* Performs a deep copy on other.
*/
public Reaction(Reaction other) {
if (other.isSetMatchers()) {
List __this__matchers = new ArrayList(other.matchers.size());
for (AromaMatcher other_element : other.matchers) {
__this__matchers.add(new AromaMatcher(other_element));
}
this.matchers = __this__matchers;
}
if (other.isSetActions()) {
List __this__actions = new ArrayList(other.actions.size());
for (AromaAction other_element : other.actions) {
__this__actions.add(new AromaAction(other_element));
}
this.actions = __this__actions;
}
if (other.isSetName()) {
this.name = other.name;
}
}
public Reaction deepCopy() {
return new Reaction(this);
}
@Override
public void clear() {
this.matchers = new ArrayList();
this.actions = new ArrayList();
this.name = null;
}
public int getMatchersSize() {
return (this.matchers == null) ? 0 : this.matchers.size();
}
public java.util.Iterator getMatchersIterator() {
return (this.matchers == null) ? null : this.matchers.iterator();
}
public void addToMatchers(AromaMatcher elem) {
if (this.matchers == null) {
this.matchers = new ArrayList();
}
this.matchers.add(elem);
}
public List getMatchers() {
return this.matchers;
}
public Reaction setMatchers(List matchers) {
this.matchers = matchers;
return this;
}
public void unsetMatchers() {
this.matchers = null;
}
/** Returns true if field matchers is set (has been assigned a value) and false otherwise */
public boolean isSetMatchers() {
return this.matchers != null;
}
public void setMatchersIsSet(boolean value) {
if (!value) {
this.matchers = null;
}
}
public int getActionsSize() {
return (this.actions == null) ? 0 : this.actions.size();
}
public java.util.Iterator getActionsIterator() {
return (this.actions == null) ? null : this.actions.iterator();
}
public void addToActions(AromaAction elem) {
if (this.actions == null) {
this.actions = new ArrayList();
}
this.actions.add(elem);
}
public List getActions() {
return this.actions;
}
public Reaction setActions(List actions) {
this.actions = actions;
return this;
}
public void unsetActions() {
this.actions = null;
}
/** Returns true if field actions is set (has been assigned a value) and false otherwise */
public boolean isSetActions() {
return this.actions != null;
}
public void setActionsIsSet(boolean value) {
if (!value) {
this.actions = null;
}
}
public String getName() {
return this.name;
}
public Reaction setName(String name) {
this.name = name;
return this;
}
public void unsetName() {
this.name = null;
}
/** Returns true if field name is set (has been assigned a value) and false otherwise */
public boolean isSetName() {
return this.name != null;
}
public void setNameIsSet(boolean value) {
if (!value) {
this.name = null;
}
}
public void setFieldValue(_Fields field, Object value) {
switch (field) {
case MATCHERS:
if (value == null) {
unsetMatchers();
} else {
setMatchers((List)value);
}
break;
case ACTIONS:
if (value == null) {
unsetActions();
} else {
setActions((List)value);
}
break;
case NAME:
if (value == null) {
unsetName();
} else {
setName((String)value);
}
break;
}
}
public Object getFieldValue(_Fields field) {
switch (field) {
case MATCHERS:
return getMatchers();
case ACTIONS:
return getActions();
case NAME:
return getName();
}
throw new IllegalStateException();
}
/** Returns true if field corresponding to fieldID is set (has been assigned a value) and false otherwise */
public boolean isSet(_Fields field) {
if (field == null) {
throw new IllegalArgumentException();
}
switch (field) {
case MATCHERS:
return isSetMatchers();
case ACTIONS:
return isSetActions();
case NAME:
return isSetName();
}
throw new IllegalStateException();
}
@Override
public boolean equals(Object that) {
if (that == null)
return false;
if (that instanceof Reaction)
return this.equals((Reaction)that);
return false;
}
public boolean equals(Reaction that) {
if (that == null)
return false;
boolean this_present_matchers = true && this.isSetMatchers();
boolean that_present_matchers = true && that.isSetMatchers();
if (this_present_matchers || that_present_matchers) {
if (!(this_present_matchers && that_present_matchers))
return false;
if (!this.matchers.equals(that.matchers))
return false;
}
boolean this_present_actions = true && this.isSetActions();
boolean that_present_actions = true && that.isSetActions();
if (this_present_actions || that_present_actions) {
if (!(this_present_actions && that_present_actions))
return false;
if (!this.actions.equals(that.actions))
return false;
}
boolean this_present_name = true && this.isSetName();
boolean that_present_name = true && that.isSetName();
if (this_present_name || that_present_name) {
if (!(this_present_name && that_present_name))
return false;
if (!this.name.equals(that.name))
return false;
}
return true;
}
@Override
public int hashCode() {
List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy