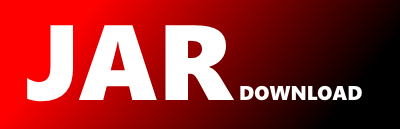
tech.aroma.thrift.service.AromaService Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aroma-thrift Show documentation
Show all versions of aroma-thrift Show documentation
Part of the Aroma Project.
This project contains the Service and Model Definitions.
From this the Server and Client interfaces are generated for the Aroma Service.
/**
* Autogenerated by Thrift Compiler (0.9.3)
*
* DO NOT EDIT UNLESS YOU ARE SURE THAT YOU KNOW WHAT YOU ARE DOING
* @generated
*/
package tech.aroma.thrift.service;
import org.apache.thrift.scheme.IScheme;
import org.apache.thrift.scheme.SchemeFactory;
import org.apache.thrift.scheme.StandardScheme;
import org.apache.thrift.scheme.TupleScheme;
import org.apache.thrift.protocol.TTupleProtocol;
import org.apache.thrift.protocol.TProtocolException;
import org.apache.thrift.EncodingUtils;
import org.apache.thrift.TException;
import org.apache.thrift.async.AsyncMethodCallback;
import org.apache.thrift.server.AbstractNonblockingServer.*;
import java.util.List;
import java.util.ArrayList;
import java.util.Map;
import java.util.HashMap;
import java.util.EnumMap;
import java.util.Set;
import java.util.HashSet;
import java.util.EnumSet;
import java.util.Collections;
import java.util.BitSet;
import java.nio.ByteBuffer;
import java.util.Arrays;
import javax.annotation.Generated;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
@SuppressWarnings({"cast", "rawtypes", "serial", "unchecked"})
@Generated(value = "Autogenerated by Thrift Compiler (0.9.3)", date = "2016-05-10")
public class AromaService {
/**
* The Aroma Service is designed to be used by People, and not Applications.
* They provide query interfaces and Authentication/Authorization over Data.
*/
public interface Iface {
public double getApiVersion() throws org.apache.thrift.TException;
public DeleteApplicationResponse deleteApplication(DeleteApplicationRequest request) throws tech.aroma.thrift.exceptions.OperationFailedException, tech.aroma.thrift.exceptions.InvalidArgumentException, tech.aroma.thrift.exceptions.InvalidTokenException, tech.aroma.thrift.exceptions.ApplicationDoesNotExistException, tech.aroma.thrift.exceptions.UnauthorizedException, org.apache.thrift.TException;
public DeleteMessageResponse deleteMessage(DeleteMessageRequest request) throws tech.aroma.thrift.exceptions.OperationFailedException, tech.aroma.thrift.exceptions.InvalidArgumentException, tech.aroma.thrift.exceptions.InvalidTokenException, tech.aroma.thrift.exceptions.MessageDoesNotExistException, tech.aroma.thrift.exceptions.UnauthorizedException, org.apache.thrift.TException;
public DismissMessageResponse dismissMessage(DismissMessageRequest request) throws tech.aroma.thrift.exceptions.OperationFailedException, tech.aroma.thrift.exceptions.InvalidArgumentException, tech.aroma.thrift.exceptions.InvalidTokenException, tech.aroma.thrift.exceptions.MessageDoesNotExistException, tech.aroma.thrift.exceptions.UnauthorizedException, org.apache.thrift.TException;
/**
* Provision a New Application to keep tabs on.
*
* #user
*
* @param request
*/
public ProvisionApplicationResponse provisionApplication(ProvisionApplicationRequest request) throws tech.aroma.thrift.exceptions.OperationFailedException, tech.aroma.thrift.exceptions.InvalidArgumentException, tech.aroma.thrift.exceptions.InvalidTokenException, tech.aroma.thrift.exceptions.ApplicationDoesNotExistException, tech.aroma.thrift.exceptions.UnauthorizedException, org.apache.thrift.TException;
/**
* Regenerate an Application Token in case the existing one is lost, forgotten, or compromised.
* Keep in mind that this will invalidate any prior existing Application Tokens.
* Only an "owner" can perform this operation.
*
* #owner
*
* @param request
*/
public RegenerateApplicationTokenResponse regenerateToken(RegenerateApplicationTokenRequest request) throws tech.aroma.thrift.exceptions.OperationFailedException, tech.aroma.thrift.exceptions.InvalidArgumentException, tech.aroma.thrift.exceptions.InvalidTokenException, tech.aroma.thrift.exceptions.ApplicationDoesNotExistException, tech.aroma.thrift.exceptions.UnauthorizedException, org.apache.thrift.TException;
/**
* Register an existing Application for Health Pokes. The Aroma Service
* will then periodically poke the Application for health status.
*
* #owner
*
* @param request
*/
public RegisterHealthCheckResponse registerHealthCheck(RegisterHealthCheckRequest request) throws tech.aroma.thrift.exceptions.OperationFailedException, tech.aroma.thrift.exceptions.InvalidArgumentException, tech.aroma.thrift.exceptions.InvalidTokenException, tech.aroma.thrift.exceptions.ApplicationDoesNotExistException, tech.aroma.thrift.exceptions.UnauthorizedException, org.apache.thrift.TException;
/**
* Removes a previously saved channel.
*
* @param request
*/
public RemoveSavedChannelResponse removeSavedChannel(RemoveSavedChannelRequest request) throws tech.aroma.thrift.exceptions.OperationFailedException, tech.aroma.thrift.exceptions.InvalidArgumentException, tech.aroma.thrift.exceptions.InvalidTokenException, tech.aroma.thrift.exceptions.UnauthorizedException, tech.aroma.thrift.exceptions.ChannelDoesNotExistException, org.apache.thrift.TException;
/**
* Renew an Application Token that is close to being expired.
* Only an "owner" can perform this operation.
*
* #owner
*
* @param request
*/
public RenewApplicationTokenResponse renewApplicationToken(RenewApplicationTokenRequest request) throws tech.aroma.thrift.exceptions.OperationFailedException, tech.aroma.thrift.exceptions.InvalidArgumentException, tech.aroma.thrift.exceptions.InvalidTokenException, tech.aroma.thrift.exceptions.ApplicationDoesNotExistException, tech.aroma.thrift.exceptions.UnauthorizedException, org.apache.thrift.TException;
/**
* Saves a user's channel for future reference.
*
* @param request
*/
public SaveChannelResponse saveChannel(SaveChannelRequest request) throws tech.aroma.thrift.exceptions.OperationFailedException, tech.aroma.thrift.exceptions.InvalidArgumentException, tech.aroma.thrift.exceptions.InvalidTokenException, tech.aroma.thrift.exceptions.UnauthorizedException, org.apache.thrift.TException;
/**
* Sign in to the App and get a User Token in return.
*
* #user
*
* @param request
*/
public SignInResponse signIn(SignInRequest request) throws tech.aroma.thrift.exceptions.OperationFailedException, tech.aroma.thrift.exceptions.InvalidArgumentException, tech.aroma.thrift.exceptions.InvalidCredentialsException, tech.aroma.thrift.exceptions.UserDoesNotExistException, org.apache.thrift.TException;
/**
* Sign Up for an Aroma Account.
*
* @param request
*/
public SignUpResponse signUp(SignUpRequest request) throws tech.aroma.thrift.exceptions.OperationFailedException, tech.aroma.thrift.exceptions.InvalidArgumentException, tech.aroma.thrift.exceptions.InvalidCredentialsException, tech.aroma.thrift.exceptions.AccountAlreadyExistsException, org.apache.thrift.TException;
/**
* Snoozes a Channel momentarily, so that it won't be notified of new alerts and messages.
*
* @param request
*/
public SnoozeChannelResponse snoozeChannel(SnoozeChannelRequest request) throws tech.aroma.thrift.exceptions.OperationFailedException, tech.aroma.thrift.exceptions.InvalidArgumentException, tech.aroma.thrift.exceptions.InvalidTokenException, tech.aroma.thrift.exceptions.UnauthorizedException, tech.aroma.thrift.exceptions.ChannelDoesNotExistException, org.apache.thrift.TException;
/**
* Subscribe to an existing application to get notifications.
*
* #user
*
* @param request
*/
public FollowApplicationResponse followApplication(FollowApplicationRequest request) throws tech.aroma.thrift.exceptions.OperationFailedException, tech.aroma.thrift.exceptions.InvalidArgumentException, tech.aroma.thrift.exceptions.InvalidTokenException, tech.aroma.thrift.exceptions.ApplicationDoesNotExistException, tech.aroma.thrift.exceptions.UnauthorizedException, org.apache.thrift.TException;
public UnfollowApplicationResponse unfollowApplication(UnfollowApplicationRequest request) throws tech.aroma.thrift.exceptions.OperationFailedException, tech.aroma.thrift.exceptions.InvalidArgumentException, tech.aroma.thrift.exceptions.InvalidTokenException, tech.aroma.thrift.exceptions.ApplicationDoesNotExistException, tech.aroma.thrift.exceptions.UnauthorizedException, org.apache.thrift.TException;
/**
* #owner
*
* @param request
*/
public UpdateApplicationResponse updateApplication(UpdateApplicationRequest request) throws tech.aroma.thrift.exceptions.OperationFailedException, tech.aroma.thrift.exceptions.InvalidArgumentException, tech.aroma.thrift.exceptions.InvalidTokenException, tech.aroma.thrift.exceptions.ApplicationDoesNotExistException, tech.aroma.thrift.exceptions.UnauthorizedException, org.apache.thrift.TException;
public UpdateReactionsResponse updateReactions(UpdateReactionsRequest request) throws tech.aroma.thrift.exceptions.OperationFailedException, tech.aroma.thrift.exceptions.InvalidArgumentException, tech.aroma.thrift.exceptions.InvalidTokenException, tech.aroma.thrift.exceptions.ApplicationDoesNotExistException, tech.aroma.thrift.exceptions.UnauthorizedException, org.apache.thrift.TException;
/**
* Get all of the User-Related activities that have happened recently.
*
* #user
*
* @param request
*/
public GetActivityResponse getActivity(GetActivityRequest request) throws tech.aroma.thrift.exceptions.OperationFailedException, tech.aroma.thrift.exceptions.InvalidArgumentException, tech.aroma.thrift.exceptions.InvalidTokenException, org.apache.thrift.TException;
/**
* Get details about an Application from it's unique ID
*
* #user
*
* @param request
*/
public GetApplicationInfoResponse getApplicationInfo(GetApplicationInfoRequest request) throws tech.aroma.thrift.exceptions.OperationFailedException, tech.aroma.thrift.exceptions.InvalidArgumentException, tech.aroma.thrift.exceptions.InvalidTokenException, tech.aroma.thrift.exceptions.ApplicationDoesNotExistException, tech.aroma.thrift.exceptions.UnauthorizedException, org.apache.thrift.TException;
public GetBuzzResponse getBuzz(GetBuzzRequest request) throws tech.aroma.thrift.exceptions.OperationFailedException, tech.aroma.thrift.exceptions.InvalidArgumentException, tech.aroma.thrift.exceptions.InvalidTokenException, tech.aroma.thrift.exceptions.ApplicationDoesNotExistException, tech.aroma.thrift.exceptions.UnauthorizedException, org.apache.thrift.TException;
public GetDashboardResponse getDashboard(GetDashboardRequest request) throws tech.aroma.thrift.exceptions.OperationFailedException, tech.aroma.thrift.exceptions.InvalidArgumentException, tech.aroma.thrift.exceptions.InvalidTokenException, org.apache.thrift.TException;
/**
* Get an Application's Messages.
*
* @param request
*/
public GetApplicationMessagesResponse getApplicationMessages(GetApplicationMessagesRequest request) throws tech.aroma.thrift.exceptions.OperationFailedException, tech.aroma.thrift.exceptions.InvalidArgumentException, tech.aroma.thrift.exceptions.InvalidTokenException, tech.aroma.thrift.exceptions.UnauthorizedException, tech.aroma.thrift.exceptions.ApplicationDoesNotExistException, org.apache.thrift.TException;
/**
* Get Messages in a User's Inbox
*
* @param request
*/
public GetInboxResponse getInbox(GetInboxRequest request) throws tech.aroma.thrift.exceptions.OperationFailedException, tech.aroma.thrift.exceptions.InvalidArgumentException, tech.aroma.thrift.exceptions.InvalidTokenException, org.apache.thrift.TException;
/**
* In case the Message body has been truncated, use this operation
* to load the full message.
*
* @param request
*/
public GetFullMessageResponse getFullMessage(GetFullMessageRequest request) throws tech.aroma.thrift.exceptions.OperationFailedException, tech.aroma.thrift.exceptions.InvalidArgumentException, tech.aroma.thrift.exceptions.InvalidTokenException, org.apache.thrift.TException;
/**
* Request to get Media stored by the Aroma Service.
*
* @param request
*/
public GetMediaResponse getMedia(GetMediaRequest request) throws tech.aroma.thrift.exceptions.OperationFailedException, tech.aroma.thrift.exceptions.InvalidArgumentException, tech.aroma.thrift.exceptions.InvalidTokenException, tech.aroma.thrift.exceptions.DoesNotExistException, tech.aroma.thrift.exceptions.UnauthorizedException, org.apache.thrift.TException;
public GetApplicationsOwnedByResponse getApplicationsOwnedBy(GetApplicationsOwnedByRequest request) throws tech.aroma.thrift.exceptions.OperationFailedException, tech.aroma.thrift.exceptions.InvalidArgumentException, tech.aroma.thrift.exceptions.InvalidTokenException, org.apache.thrift.TException;
public GetApplicationsFollowedByResponse getApplicationsFollowedBy(GetApplicationsFollowedByRequest request) throws tech.aroma.thrift.exceptions.OperationFailedException, tech.aroma.thrift.exceptions.InvalidArgumentException, tech.aroma.thrift.exceptions.InvalidTokenException, org.apache.thrift.TException;
public GetMySavedChannelsResponse getMySavedChannels(GetMySavedChannelsRequest request) throws tech.aroma.thrift.exceptions.OperationFailedException, tech.aroma.thrift.exceptions.InvalidArgumentException, tech.aroma.thrift.exceptions.InvalidTokenException, org.apache.thrift.TException;
public GetReactionsResponse getReactions(GetReactionsRequest request) throws tech.aroma.thrift.exceptions.OperationFailedException, tech.aroma.thrift.exceptions.InvalidArgumentException, tech.aroma.thrift.exceptions.InvalidTokenException, tech.aroma.thrift.exceptions.ApplicationDoesNotExistException, tech.aroma.thrift.exceptions.UnauthorizedException, org.apache.thrift.TException;
public GetUserInfoResponse getUserInfo(GetUserInfoRequest request) throws tech.aroma.thrift.exceptions.OperationFailedException, tech.aroma.thrift.exceptions.InvalidArgumentException, tech.aroma.thrift.exceptions.InvalidTokenException, tech.aroma.thrift.exceptions.UnauthorizedException, tech.aroma.thrift.exceptions.UserDoesNotExistException, org.apache.thrift.TException;
/**
* Perform a Search on all the applications registered to Aroma by searching for its title.
*
* #user
*
* @param request
*/
public SearchForApplicationsResponse searchForApplications(SearchForApplicationsRequest request) throws tech.aroma.thrift.exceptions.OperationFailedException, tech.aroma.thrift.exceptions.InvalidArgumentException, tech.aroma.thrift.exceptions.InvalidTokenException, tech.aroma.thrift.exceptions.UnauthorizedException, org.apache.thrift.TException;
}
public interface AsyncIface {
public void getApiVersion(org.apache.thrift.async.AsyncMethodCallback resultHandler) throws org.apache.thrift.TException;
public void deleteApplication(DeleteApplicationRequest request, org.apache.thrift.async.AsyncMethodCallback resultHandler) throws org.apache.thrift.TException;
public void deleteMessage(DeleteMessageRequest request, org.apache.thrift.async.AsyncMethodCallback resultHandler) throws org.apache.thrift.TException;
public void dismissMessage(DismissMessageRequest request, org.apache.thrift.async.AsyncMethodCallback resultHandler) throws org.apache.thrift.TException;
public void provisionApplication(ProvisionApplicationRequest request, org.apache.thrift.async.AsyncMethodCallback resultHandler) throws org.apache.thrift.TException;
public void regenerateToken(RegenerateApplicationTokenRequest request, org.apache.thrift.async.AsyncMethodCallback resultHandler) throws org.apache.thrift.TException;
public void registerHealthCheck(RegisterHealthCheckRequest request, org.apache.thrift.async.AsyncMethodCallback resultHandler) throws org.apache.thrift.TException;
public void removeSavedChannel(RemoveSavedChannelRequest request, org.apache.thrift.async.AsyncMethodCallback resultHandler) throws org.apache.thrift.TException;
public void renewApplicationToken(RenewApplicationTokenRequest request, org.apache.thrift.async.AsyncMethodCallback resultHandler) throws org.apache.thrift.TException;
public void saveChannel(SaveChannelRequest request, org.apache.thrift.async.AsyncMethodCallback resultHandler) throws org.apache.thrift.TException;
public void signIn(SignInRequest request, org.apache.thrift.async.AsyncMethodCallback resultHandler) throws org.apache.thrift.TException;
public void signUp(SignUpRequest request, org.apache.thrift.async.AsyncMethodCallback resultHandler) throws org.apache.thrift.TException;
public void snoozeChannel(SnoozeChannelRequest request, org.apache.thrift.async.AsyncMethodCallback resultHandler) throws org.apache.thrift.TException;
public void followApplication(FollowApplicationRequest request, org.apache.thrift.async.AsyncMethodCallback resultHandler) throws org.apache.thrift.TException;
public void unfollowApplication(UnfollowApplicationRequest request, org.apache.thrift.async.AsyncMethodCallback resultHandler) throws org.apache.thrift.TException;
public void updateApplication(UpdateApplicationRequest request, org.apache.thrift.async.AsyncMethodCallback resultHandler) throws org.apache.thrift.TException;
public void updateReactions(UpdateReactionsRequest request, org.apache.thrift.async.AsyncMethodCallback resultHandler) throws org.apache.thrift.TException;
public void getActivity(GetActivityRequest request, org.apache.thrift.async.AsyncMethodCallback resultHandler) throws org.apache.thrift.TException;
public void getApplicationInfo(GetApplicationInfoRequest request, org.apache.thrift.async.AsyncMethodCallback resultHandler) throws org.apache.thrift.TException;
public void getBuzz(GetBuzzRequest request, org.apache.thrift.async.AsyncMethodCallback resultHandler) throws org.apache.thrift.TException;
public void getDashboard(GetDashboardRequest request, org.apache.thrift.async.AsyncMethodCallback resultHandler) throws org.apache.thrift.TException;
public void getApplicationMessages(GetApplicationMessagesRequest request, org.apache.thrift.async.AsyncMethodCallback resultHandler) throws org.apache.thrift.TException;
public void getInbox(GetInboxRequest request, org.apache.thrift.async.AsyncMethodCallback resultHandler) throws org.apache.thrift.TException;
public void getFullMessage(GetFullMessageRequest request, org.apache.thrift.async.AsyncMethodCallback resultHandler) throws org.apache.thrift.TException;
public void getMedia(GetMediaRequest request, org.apache.thrift.async.AsyncMethodCallback resultHandler) throws org.apache.thrift.TException;
public void getApplicationsOwnedBy(GetApplicationsOwnedByRequest request, org.apache.thrift.async.AsyncMethodCallback resultHandler) throws org.apache.thrift.TException;
public void getApplicationsFollowedBy(GetApplicationsFollowedByRequest request, org.apache.thrift.async.AsyncMethodCallback resultHandler) throws org.apache.thrift.TException;
public void getMySavedChannels(GetMySavedChannelsRequest request, org.apache.thrift.async.AsyncMethodCallback resultHandler) throws org.apache.thrift.TException;
public void getReactions(GetReactionsRequest request, org.apache.thrift.async.AsyncMethodCallback resultHandler) throws org.apache.thrift.TException;
public void getUserInfo(GetUserInfoRequest request, org.apache.thrift.async.AsyncMethodCallback resultHandler) throws org.apache.thrift.TException;
public void searchForApplications(SearchForApplicationsRequest request, org.apache.thrift.async.AsyncMethodCallback resultHandler) throws org.apache.thrift.TException;
}
public static class Client extends org.apache.thrift.TServiceClient implements Iface {
public static class Factory implements org.apache.thrift.TServiceClientFactory {
public Factory() {}
public Client getClient(org.apache.thrift.protocol.TProtocol prot) {
return new Client(prot);
}
public Client getClient(org.apache.thrift.protocol.TProtocol iprot, org.apache.thrift.protocol.TProtocol oprot) {
return new Client(iprot, oprot);
}
}
public Client(org.apache.thrift.protocol.TProtocol prot)
{
super(prot, prot);
}
public Client(org.apache.thrift.protocol.TProtocol iprot, org.apache.thrift.protocol.TProtocol oprot) {
super(iprot, oprot);
}
public double getApiVersion() throws org.apache.thrift.TException
{
send_getApiVersion();
return recv_getApiVersion();
}
public void send_getApiVersion() throws org.apache.thrift.TException
{
getApiVersion_args args = new getApiVersion_args();
sendBase("getApiVersion", args);
}
public double recv_getApiVersion() throws org.apache.thrift.TException
{
getApiVersion_result result = new getApiVersion_result();
receiveBase(result, "getApiVersion");
if (result.isSetSuccess()) {
return result.success;
}
throw new org.apache.thrift.TApplicationException(org.apache.thrift.TApplicationException.MISSING_RESULT, "getApiVersion failed: unknown result");
}
public DeleteApplicationResponse deleteApplication(DeleteApplicationRequest request) throws tech.aroma.thrift.exceptions.OperationFailedException, tech.aroma.thrift.exceptions.InvalidArgumentException, tech.aroma.thrift.exceptions.InvalidTokenException, tech.aroma.thrift.exceptions.ApplicationDoesNotExistException, tech.aroma.thrift.exceptions.UnauthorizedException, org.apache.thrift.TException
{
send_deleteApplication(request);
return recv_deleteApplication();
}
public void send_deleteApplication(DeleteApplicationRequest request) throws org.apache.thrift.TException
{
deleteApplication_args args = new deleteApplication_args();
args.setRequest(request);
sendBase("deleteApplication", args);
}
public DeleteApplicationResponse recv_deleteApplication() throws tech.aroma.thrift.exceptions.OperationFailedException, tech.aroma.thrift.exceptions.InvalidArgumentException, tech.aroma.thrift.exceptions.InvalidTokenException, tech.aroma.thrift.exceptions.ApplicationDoesNotExistException, tech.aroma.thrift.exceptions.UnauthorizedException, org.apache.thrift.TException
{
deleteApplication_result result = new deleteApplication_result();
receiveBase(result, "deleteApplication");
if (result.isSetSuccess()) {
return result.success;
}
if (result.ex1 != null) {
throw result.ex1;
}
if (result.ex2 != null) {
throw result.ex2;
}
if (result.ex3 != null) {
throw result.ex3;
}
if (result.ex4 != null) {
throw result.ex4;
}
if (result.ex5 != null) {
throw result.ex5;
}
throw new org.apache.thrift.TApplicationException(org.apache.thrift.TApplicationException.MISSING_RESULT, "deleteApplication failed: unknown result");
}
public DeleteMessageResponse deleteMessage(DeleteMessageRequest request) throws tech.aroma.thrift.exceptions.OperationFailedException, tech.aroma.thrift.exceptions.InvalidArgumentException, tech.aroma.thrift.exceptions.InvalidTokenException, tech.aroma.thrift.exceptions.MessageDoesNotExistException, tech.aroma.thrift.exceptions.UnauthorizedException, org.apache.thrift.TException
{
send_deleteMessage(request);
return recv_deleteMessage();
}
public void send_deleteMessage(DeleteMessageRequest request) throws org.apache.thrift.TException
{
deleteMessage_args args = new deleteMessage_args();
args.setRequest(request);
sendBase("deleteMessage", args);
}
public DeleteMessageResponse recv_deleteMessage() throws tech.aroma.thrift.exceptions.OperationFailedException, tech.aroma.thrift.exceptions.InvalidArgumentException, tech.aroma.thrift.exceptions.InvalidTokenException, tech.aroma.thrift.exceptions.MessageDoesNotExistException, tech.aroma.thrift.exceptions.UnauthorizedException, org.apache.thrift.TException
{
deleteMessage_result result = new deleteMessage_result();
receiveBase(result, "deleteMessage");
if (result.isSetSuccess()) {
return result.success;
}
if (result.ex1 != null) {
throw result.ex1;
}
if (result.ex2 != null) {
throw result.ex2;
}
if (result.ex3 != null) {
throw result.ex3;
}
if (result.ex4 != null) {
throw result.ex4;
}
if (result.ex5 != null) {
throw result.ex5;
}
throw new org.apache.thrift.TApplicationException(org.apache.thrift.TApplicationException.MISSING_RESULT, "deleteMessage failed: unknown result");
}
public DismissMessageResponse dismissMessage(DismissMessageRequest request) throws tech.aroma.thrift.exceptions.OperationFailedException, tech.aroma.thrift.exceptions.InvalidArgumentException, tech.aroma.thrift.exceptions.InvalidTokenException, tech.aroma.thrift.exceptions.MessageDoesNotExistException, tech.aroma.thrift.exceptions.UnauthorizedException, org.apache.thrift.TException
{
send_dismissMessage(request);
return recv_dismissMessage();
}
public void send_dismissMessage(DismissMessageRequest request) throws org.apache.thrift.TException
{
dismissMessage_args args = new dismissMessage_args();
args.setRequest(request);
sendBase("dismissMessage", args);
}
public DismissMessageResponse recv_dismissMessage() throws tech.aroma.thrift.exceptions.OperationFailedException, tech.aroma.thrift.exceptions.InvalidArgumentException, tech.aroma.thrift.exceptions.InvalidTokenException, tech.aroma.thrift.exceptions.MessageDoesNotExistException, tech.aroma.thrift.exceptions.UnauthorizedException, org.apache.thrift.TException
{
dismissMessage_result result = new dismissMessage_result();
receiveBase(result, "dismissMessage");
if (result.isSetSuccess()) {
return result.success;
}
if (result.ex1 != null) {
throw result.ex1;
}
if (result.ex2 != null) {
throw result.ex2;
}
if (result.ex3 != null) {
throw result.ex3;
}
if (result.ex4 != null) {
throw result.ex4;
}
if (result.ex5 != null) {
throw result.ex5;
}
throw new org.apache.thrift.TApplicationException(org.apache.thrift.TApplicationException.MISSING_RESULT, "dismissMessage failed: unknown result");
}
public ProvisionApplicationResponse provisionApplication(ProvisionApplicationRequest request) throws tech.aroma.thrift.exceptions.OperationFailedException, tech.aroma.thrift.exceptions.InvalidArgumentException, tech.aroma.thrift.exceptions.InvalidTokenException, tech.aroma.thrift.exceptions.ApplicationDoesNotExistException, tech.aroma.thrift.exceptions.UnauthorizedException, org.apache.thrift.TException
{
send_provisionApplication(request);
return recv_provisionApplication();
}
public void send_provisionApplication(ProvisionApplicationRequest request) throws org.apache.thrift.TException
{
provisionApplication_args args = new provisionApplication_args();
args.setRequest(request);
sendBase("provisionApplication", args);
}
public ProvisionApplicationResponse recv_provisionApplication() throws tech.aroma.thrift.exceptions.OperationFailedException, tech.aroma.thrift.exceptions.InvalidArgumentException, tech.aroma.thrift.exceptions.InvalidTokenException, tech.aroma.thrift.exceptions.ApplicationDoesNotExistException, tech.aroma.thrift.exceptions.UnauthorizedException, org.apache.thrift.TException
{
provisionApplication_result result = new provisionApplication_result();
receiveBase(result, "provisionApplication");
if (result.isSetSuccess()) {
return result.success;
}
if (result.ex1 != null) {
throw result.ex1;
}
if (result.ex2 != null) {
throw result.ex2;
}
if (result.ex3 != null) {
throw result.ex3;
}
if (result.ex4 != null) {
throw result.ex4;
}
if (result.ex5 != null) {
throw result.ex5;
}
throw new org.apache.thrift.TApplicationException(org.apache.thrift.TApplicationException.MISSING_RESULT, "provisionApplication failed: unknown result");
}
public RegenerateApplicationTokenResponse regenerateToken(RegenerateApplicationTokenRequest request) throws tech.aroma.thrift.exceptions.OperationFailedException, tech.aroma.thrift.exceptions.InvalidArgumentException, tech.aroma.thrift.exceptions.InvalidTokenException, tech.aroma.thrift.exceptions.ApplicationDoesNotExistException, tech.aroma.thrift.exceptions.UnauthorizedException, org.apache.thrift.TException
{
send_regenerateToken(request);
return recv_regenerateToken();
}
public void send_regenerateToken(RegenerateApplicationTokenRequest request) throws org.apache.thrift.TException
{
regenerateToken_args args = new regenerateToken_args();
args.setRequest(request);
sendBase("regenerateToken", args);
}
public RegenerateApplicationTokenResponse recv_regenerateToken() throws tech.aroma.thrift.exceptions.OperationFailedException, tech.aroma.thrift.exceptions.InvalidArgumentException, tech.aroma.thrift.exceptions.InvalidTokenException, tech.aroma.thrift.exceptions.ApplicationDoesNotExistException, tech.aroma.thrift.exceptions.UnauthorizedException, org.apache.thrift.TException
{
regenerateToken_result result = new regenerateToken_result();
receiveBase(result, "regenerateToken");
if (result.isSetSuccess()) {
return result.success;
}
if (result.ex1 != null) {
throw result.ex1;
}
if (result.ex2 != null) {
throw result.ex2;
}
if (result.ex3 != null) {
throw result.ex3;
}
if (result.ex4 != null) {
throw result.ex4;
}
if (result.ex5 != null) {
throw result.ex5;
}
throw new org.apache.thrift.TApplicationException(org.apache.thrift.TApplicationException.MISSING_RESULT, "regenerateToken failed: unknown result");
}
public RegisterHealthCheckResponse registerHealthCheck(RegisterHealthCheckRequest request) throws tech.aroma.thrift.exceptions.OperationFailedException, tech.aroma.thrift.exceptions.InvalidArgumentException, tech.aroma.thrift.exceptions.InvalidTokenException, tech.aroma.thrift.exceptions.ApplicationDoesNotExistException, tech.aroma.thrift.exceptions.UnauthorizedException, org.apache.thrift.TException
{
send_registerHealthCheck(request);
return recv_registerHealthCheck();
}
public void send_registerHealthCheck(RegisterHealthCheckRequest request) throws org.apache.thrift.TException
{
registerHealthCheck_args args = new registerHealthCheck_args();
args.setRequest(request);
sendBase("registerHealthCheck", args);
}
public RegisterHealthCheckResponse recv_registerHealthCheck() throws tech.aroma.thrift.exceptions.OperationFailedException, tech.aroma.thrift.exceptions.InvalidArgumentException, tech.aroma.thrift.exceptions.InvalidTokenException, tech.aroma.thrift.exceptions.ApplicationDoesNotExistException, tech.aroma.thrift.exceptions.UnauthorizedException, org.apache.thrift.TException
{
registerHealthCheck_result result = new registerHealthCheck_result();
receiveBase(result, "registerHealthCheck");
if (result.isSetSuccess()) {
return result.success;
}
if (result.ex1 != null) {
throw result.ex1;
}
if (result.ex2 != null) {
throw result.ex2;
}
if (result.ex3 != null) {
throw result.ex3;
}
if (result.ex4 != null) {
throw result.ex4;
}
if (result.ex5 != null) {
throw result.ex5;
}
throw new org.apache.thrift.TApplicationException(org.apache.thrift.TApplicationException.MISSING_RESULT, "registerHealthCheck failed: unknown result");
}
public RemoveSavedChannelResponse removeSavedChannel(RemoveSavedChannelRequest request) throws tech.aroma.thrift.exceptions.OperationFailedException, tech.aroma.thrift.exceptions.InvalidArgumentException, tech.aroma.thrift.exceptions.InvalidTokenException, tech.aroma.thrift.exceptions.UnauthorizedException, tech.aroma.thrift.exceptions.ChannelDoesNotExistException, org.apache.thrift.TException
{
send_removeSavedChannel(request);
return recv_removeSavedChannel();
}
public void send_removeSavedChannel(RemoveSavedChannelRequest request) throws org.apache.thrift.TException
{
removeSavedChannel_args args = new removeSavedChannel_args();
args.setRequest(request);
sendBase("removeSavedChannel", args);
}
public RemoveSavedChannelResponse recv_removeSavedChannel() throws tech.aroma.thrift.exceptions.OperationFailedException, tech.aroma.thrift.exceptions.InvalidArgumentException, tech.aroma.thrift.exceptions.InvalidTokenException, tech.aroma.thrift.exceptions.UnauthorizedException, tech.aroma.thrift.exceptions.ChannelDoesNotExistException, org.apache.thrift.TException
{
removeSavedChannel_result result = new removeSavedChannel_result();
receiveBase(result, "removeSavedChannel");
if (result.isSetSuccess()) {
return result.success;
}
if (result.ex1 != null) {
throw result.ex1;
}
if (result.ex2 != null) {
throw result.ex2;
}
if (result.ex3 != null) {
throw result.ex3;
}
if (result.ex4 != null) {
throw result.ex4;
}
if (result.ex5 != null) {
throw result.ex5;
}
throw new org.apache.thrift.TApplicationException(org.apache.thrift.TApplicationException.MISSING_RESULT, "removeSavedChannel failed: unknown result");
}
public RenewApplicationTokenResponse renewApplicationToken(RenewApplicationTokenRequest request) throws tech.aroma.thrift.exceptions.OperationFailedException, tech.aroma.thrift.exceptions.InvalidArgumentException, tech.aroma.thrift.exceptions.InvalidTokenException, tech.aroma.thrift.exceptions.ApplicationDoesNotExistException, tech.aroma.thrift.exceptions.UnauthorizedException, org.apache.thrift.TException
{
send_renewApplicationToken(request);
return recv_renewApplicationToken();
}
public void send_renewApplicationToken(RenewApplicationTokenRequest request) throws org.apache.thrift.TException
{
renewApplicationToken_args args = new renewApplicationToken_args();
args.setRequest(request);
sendBase("renewApplicationToken", args);
}
public RenewApplicationTokenResponse recv_renewApplicationToken() throws tech.aroma.thrift.exceptions.OperationFailedException, tech.aroma.thrift.exceptions.InvalidArgumentException, tech.aroma.thrift.exceptions.InvalidTokenException, tech.aroma.thrift.exceptions.ApplicationDoesNotExistException, tech.aroma.thrift.exceptions.UnauthorizedException, org.apache.thrift.TException
{
renewApplicationToken_result result = new renewApplicationToken_result();
receiveBase(result, "renewApplicationToken");
if (result.isSetSuccess()) {
return result.success;
}
if (result.ex1 != null) {
throw result.ex1;
}
if (result.ex2 != null) {
throw result.ex2;
}
if (result.ex3 != null) {
throw result.ex3;
}
if (result.ex4 != null) {
throw result.ex4;
}
if (result.ex5 != null) {
throw result.ex5;
}
throw new org.apache.thrift.TApplicationException(org.apache.thrift.TApplicationException.MISSING_RESULT, "renewApplicationToken failed: unknown result");
}
public SaveChannelResponse saveChannel(SaveChannelRequest request) throws tech.aroma.thrift.exceptions.OperationFailedException, tech.aroma.thrift.exceptions.InvalidArgumentException, tech.aroma.thrift.exceptions.InvalidTokenException, tech.aroma.thrift.exceptions.UnauthorizedException, org.apache.thrift.TException
{
send_saveChannel(request);
return recv_saveChannel();
}
public void send_saveChannel(SaveChannelRequest request) throws org.apache.thrift.TException
{
saveChannel_args args = new saveChannel_args();
args.setRequest(request);
sendBase("saveChannel", args);
}
public SaveChannelResponse recv_saveChannel() throws tech.aroma.thrift.exceptions.OperationFailedException, tech.aroma.thrift.exceptions.InvalidArgumentException, tech.aroma.thrift.exceptions.InvalidTokenException, tech.aroma.thrift.exceptions.UnauthorizedException, org.apache.thrift.TException
{
saveChannel_result result = new saveChannel_result();
receiveBase(result, "saveChannel");
if (result.isSetSuccess()) {
return result.success;
}
if (result.ex1 != null) {
throw result.ex1;
}
if (result.ex2 != null) {
throw result.ex2;
}
if (result.ex3 != null) {
throw result.ex3;
}
if (result.ex4 != null) {
throw result.ex4;
}
throw new org.apache.thrift.TApplicationException(org.apache.thrift.TApplicationException.MISSING_RESULT, "saveChannel failed: unknown result");
}
public SignInResponse signIn(SignInRequest request) throws tech.aroma.thrift.exceptions.OperationFailedException, tech.aroma.thrift.exceptions.InvalidArgumentException, tech.aroma.thrift.exceptions.InvalidCredentialsException, tech.aroma.thrift.exceptions.UserDoesNotExistException, org.apache.thrift.TException
{
send_signIn(request);
return recv_signIn();
}
public void send_signIn(SignInRequest request) throws org.apache.thrift.TException
{
signIn_args args = new signIn_args();
args.setRequest(request);
sendBase("signIn", args);
}
public SignInResponse recv_signIn() throws tech.aroma.thrift.exceptions.OperationFailedException, tech.aroma.thrift.exceptions.InvalidArgumentException, tech.aroma.thrift.exceptions.InvalidCredentialsException, tech.aroma.thrift.exceptions.UserDoesNotExistException, org.apache.thrift.TException
{
signIn_result result = new signIn_result();
receiveBase(result, "signIn");
if (result.isSetSuccess()) {
return result.success;
}
if (result.ex1 != null) {
throw result.ex1;
}
if (result.ex2 != null) {
throw result.ex2;
}
if (result.ex3 != null) {
throw result.ex3;
}
if (result.ex4 != null) {
throw result.ex4;
}
throw new org.apache.thrift.TApplicationException(org.apache.thrift.TApplicationException.MISSING_RESULT, "signIn failed: unknown result");
}
public SignUpResponse signUp(SignUpRequest request) throws tech.aroma.thrift.exceptions.OperationFailedException, tech.aroma.thrift.exceptions.InvalidArgumentException, tech.aroma.thrift.exceptions.InvalidCredentialsException, tech.aroma.thrift.exceptions.AccountAlreadyExistsException, org.apache.thrift.TException
{
send_signUp(request);
return recv_signUp();
}
public void send_signUp(SignUpRequest request) throws org.apache.thrift.TException
{
signUp_args args = new signUp_args();
args.setRequest(request);
sendBase("signUp", args);
}
public SignUpResponse recv_signUp() throws tech.aroma.thrift.exceptions.OperationFailedException, tech.aroma.thrift.exceptions.InvalidArgumentException, tech.aroma.thrift.exceptions.InvalidCredentialsException, tech.aroma.thrift.exceptions.AccountAlreadyExistsException, org.apache.thrift.TException
{
signUp_result result = new signUp_result();
receiveBase(result, "signUp");
if (result.isSetSuccess()) {
return result.success;
}
if (result.ex1 != null) {
throw result.ex1;
}
if (result.ex2 != null) {
throw result.ex2;
}
if (result.ex3 != null) {
throw result.ex3;
}
if (result.ex4 != null) {
throw result.ex4;
}
throw new org.apache.thrift.TApplicationException(org.apache.thrift.TApplicationException.MISSING_RESULT, "signUp failed: unknown result");
}
public SnoozeChannelResponse snoozeChannel(SnoozeChannelRequest request) throws tech.aroma.thrift.exceptions.OperationFailedException, tech.aroma.thrift.exceptions.InvalidArgumentException, tech.aroma.thrift.exceptions.InvalidTokenException, tech.aroma.thrift.exceptions.UnauthorizedException, tech.aroma.thrift.exceptions.ChannelDoesNotExistException, org.apache.thrift.TException
{
send_snoozeChannel(request);
return recv_snoozeChannel();
}
public void send_snoozeChannel(SnoozeChannelRequest request) throws org.apache.thrift.TException
{
snoozeChannel_args args = new snoozeChannel_args();
args.setRequest(request);
sendBase("snoozeChannel", args);
}
public SnoozeChannelResponse recv_snoozeChannel() throws tech.aroma.thrift.exceptions.OperationFailedException, tech.aroma.thrift.exceptions.InvalidArgumentException, tech.aroma.thrift.exceptions.InvalidTokenException, tech.aroma.thrift.exceptions.UnauthorizedException, tech.aroma.thrift.exceptions.ChannelDoesNotExistException, org.apache.thrift.TException
{
snoozeChannel_result result = new snoozeChannel_result();
receiveBase(result, "snoozeChannel");
if (result.isSetSuccess()) {
return result.success;
}
if (result.ex1 != null) {
throw result.ex1;
}
if (result.ex2 != null) {
throw result.ex2;
}
if (result.ex3 != null) {
throw result.ex3;
}
if (result.ex4 != null) {
throw result.ex4;
}
if (result.ex5 != null) {
throw result.ex5;
}
throw new org.apache.thrift.TApplicationException(org.apache.thrift.TApplicationException.MISSING_RESULT, "snoozeChannel failed: unknown result");
}
public FollowApplicationResponse followApplication(FollowApplicationRequest request) throws tech.aroma.thrift.exceptions.OperationFailedException, tech.aroma.thrift.exceptions.InvalidArgumentException, tech.aroma.thrift.exceptions.InvalidTokenException, tech.aroma.thrift.exceptions.ApplicationDoesNotExistException, tech.aroma.thrift.exceptions.UnauthorizedException, org.apache.thrift.TException
{
send_followApplication(request);
return recv_followApplication();
}
public void send_followApplication(FollowApplicationRequest request) throws org.apache.thrift.TException
{
followApplication_args args = new followApplication_args();
args.setRequest(request);
sendBase("followApplication", args);
}
public FollowApplicationResponse recv_followApplication() throws tech.aroma.thrift.exceptions.OperationFailedException, tech.aroma.thrift.exceptions.InvalidArgumentException, tech.aroma.thrift.exceptions.InvalidTokenException, tech.aroma.thrift.exceptions.ApplicationDoesNotExistException, tech.aroma.thrift.exceptions.UnauthorizedException, org.apache.thrift.TException
{
followApplication_result result = new followApplication_result();
receiveBase(result, "followApplication");
if (result.isSetSuccess()) {
return result.success;
}
if (result.ex1 != null) {
throw result.ex1;
}
if (result.ex2 != null) {
throw result.ex2;
}
if (result.ex3 != null) {
throw result.ex3;
}
if (result.ex4 != null) {
throw result.ex4;
}
if (result.ex5 != null) {
throw result.ex5;
}
throw new org.apache.thrift.TApplicationException(org.apache.thrift.TApplicationException.MISSING_RESULT, "followApplication failed: unknown result");
}
public UnfollowApplicationResponse unfollowApplication(UnfollowApplicationRequest request) throws tech.aroma.thrift.exceptions.OperationFailedException, tech.aroma.thrift.exceptions.InvalidArgumentException, tech.aroma.thrift.exceptions.InvalidTokenException, tech.aroma.thrift.exceptions.ApplicationDoesNotExistException, tech.aroma.thrift.exceptions.UnauthorizedException, org.apache.thrift.TException
{
send_unfollowApplication(request);
return recv_unfollowApplication();
}
public void send_unfollowApplication(UnfollowApplicationRequest request) throws org.apache.thrift.TException
{
unfollowApplication_args args = new unfollowApplication_args();
args.setRequest(request);
sendBase("unfollowApplication", args);
}
public UnfollowApplicationResponse recv_unfollowApplication() throws tech.aroma.thrift.exceptions.OperationFailedException, tech.aroma.thrift.exceptions.InvalidArgumentException, tech.aroma.thrift.exceptions.InvalidTokenException, tech.aroma.thrift.exceptions.ApplicationDoesNotExistException, tech.aroma.thrift.exceptions.UnauthorizedException, org.apache.thrift.TException
{
unfollowApplication_result result = new unfollowApplication_result();
receiveBase(result, "unfollowApplication");
if (result.isSetSuccess()) {
return result.success;
}
if (result.ex1 != null) {
throw result.ex1;
}
if (result.ex2 != null) {
throw result.ex2;
}
if (result.ex3 != null) {
throw result.ex3;
}
if (result.ex4 != null) {
throw result.ex4;
}
if (result.ex5 != null) {
throw result.ex5;
}
throw new org.apache.thrift.TApplicationException(org.apache.thrift.TApplicationException.MISSING_RESULT, "unfollowApplication failed: unknown result");
}
public UpdateApplicationResponse updateApplication(UpdateApplicationRequest request) throws tech.aroma.thrift.exceptions.OperationFailedException, tech.aroma.thrift.exceptions.InvalidArgumentException, tech.aroma.thrift.exceptions.InvalidTokenException, tech.aroma.thrift.exceptions.ApplicationDoesNotExistException, tech.aroma.thrift.exceptions.UnauthorizedException, org.apache.thrift.TException
{
send_updateApplication(request);
return recv_updateApplication();
}
public void send_updateApplication(UpdateApplicationRequest request) throws org.apache.thrift.TException
{
updateApplication_args args = new updateApplication_args();
args.setRequest(request);
sendBase("updateApplication", args);
}
public UpdateApplicationResponse recv_updateApplication() throws tech.aroma.thrift.exceptions.OperationFailedException, tech.aroma.thrift.exceptions.InvalidArgumentException, tech.aroma.thrift.exceptions.InvalidTokenException, tech.aroma.thrift.exceptions.ApplicationDoesNotExistException, tech.aroma.thrift.exceptions.UnauthorizedException, org.apache.thrift.TException
{
updateApplication_result result = new updateApplication_result();
receiveBase(result, "updateApplication");
if (result.isSetSuccess()) {
return result.success;
}
if (result.ex1 != null) {
throw result.ex1;
}
if (result.ex2 != null) {
throw result.ex2;
}
if (result.ex3 != null) {
throw result.ex3;
}
if (result.ex4 != null) {
throw result.ex4;
}
if (result.ex5 != null) {
throw result.ex5;
}
throw new org.apache.thrift.TApplicationException(org.apache.thrift.TApplicationException.MISSING_RESULT, "updateApplication failed: unknown result");
}
public UpdateReactionsResponse updateReactions(UpdateReactionsRequest request) throws tech.aroma.thrift.exceptions.OperationFailedException, tech.aroma.thrift.exceptions.InvalidArgumentException, tech.aroma.thrift.exceptions.InvalidTokenException, tech.aroma.thrift.exceptions.ApplicationDoesNotExistException, tech.aroma.thrift.exceptions.UnauthorizedException, org.apache.thrift.TException
{
send_updateReactions(request);
return recv_updateReactions();
}
public void send_updateReactions(UpdateReactionsRequest request) throws org.apache.thrift.TException
{
updateReactions_args args = new updateReactions_args();
args.setRequest(request);
sendBase("updateReactions", args);
}
public UpdateReactionsResponse recv_updateReactions() throws tech.aroma.thrift.exceptions.OperationFailedException, tech.aroma.thrift.exceptions.InvalidArgumentException, tech.aroma.thrift.exceptions.InvalidTokenException, tech.aroma.thrift.exceptions.ApplicationDoesNotExistException, tech.aroma.thrift.exceptions.UnauthorizedException, org.apache.thrift.TException
{
updateReactions_result result = new updateReactions_result();
receiveBase(result, "updateReactions");
if (result.isSetSuccess()) {
return result.success;
}
if (result.ex1 != null) {
throw result.ex1;
}
if (result.ex2 != null) {
throw result.ex2;
}
if (result.ex3 != null) {
throw result.ex3;
}
if (result.ex4 != null) {
throw result.ex4;
}
if (result.ex5 != null) {
throw result.ex5;
}
throw new org.apache.thrift.TApplicationException(org.apache.thrift.TApplicationException.MISSING_RESULT, "updateReactions failed: unknown result");
}
public GetActivityResponse getActivity(GetActivityRequest request) throws tech.aroma.thrift.exceptions.OperationFailedException, tech.aroma.thrift.exceptions.InvalidArgumentException, tech.aroma.thrift.exceptions.InvalidTokenException, org.apache.thrift.TException
{
send_getActivity(request);
return recv_getActivity();
}
public void send_getActivity(GetActivityRequest request) throws org.apache.thrift.TException
{
getActivity_args args = new getActivity_args();
args.setRequest(request);
sendBase("getActivity", args);
}
public GetActivityResponse recv_getActivity() throws tech.aroma.thrift.exceptions.OperationFailedException, tech.aroma.thrift.exceptions.InvalidArgumentException, tech.aroma.thrift.exceptions.InvalidTokenException, org.apache.thrift.TException
{
getActivity_result result = new getActivity_result();
receiveBase(result, "getActivity");
if (result.isSetSuccess()) {
return result.success;
}
if (result.ex1 != null) {
throw result.ex1;
}
if (result.ex2 != null) {
throw result.ex2;
}
if (result.ex3 != null) {
throw result.ex3;
}
throw new org.apache.thrift.TApplicationException(org.apache.thrift.TApplicationException.MISSING_RESULT, "getActivity failed: unknown result");
}
public GetApplicationInfoResponse getApplicationInfo(GetApplicationInfoRequest request) throws tech.aroma.thrift.exceptions.OperationFailedException, tech.aroma.thrift.exceptions.InvalidArgumentException, tech.aroma.thrift.exceptions.InvalidTokenException, tech.aroma.thrift.exceptions.ApplicationDoesNotExistException, tech.aroma.thrift.exceptions.UnauthorizedException, org.apache.thrift.TException
{
send_getApplicationInfo(request);
return recv_getApplicationInfo();
}
public void send_getApplicationInfo(GetApplicationInfoRequest request) throws org.apache.thrift.TException
{
getApplicationInfo_args args = new getApplicationInfo_args();
args.setRequest(request);
sendBase("getApplicationInfo", args);
}
public GetApplicationInfoResponse recv_getApplicationInfo() throws tech.aroma.thrift.exceptions.OperationFailedException, tech.aroma.thrift.exceptions.InvalidArgumentException, tech.aroma.thrift.exceptions.InvalidTokenException, tech.aroma.thrift.exceptions.ApplicationDoesNotExistException, tech.aroma.thrift.exceptions.UnauthorizedException, org.apache.thrift.TException
{
getApplicationInfo_result result = new getApplicationInfo_result();
receiveBase(result, "getApplicationInfo");
if (result.isSetSuccess()) {
return result.success;
}
if (result.ex1 != null) {
throw result.ex1;
}
if (result.ex2 != null) {
throw result.ex2;
}
if (result.ex3 != null) {
throw result.ex3;
}
if (result.ex4 != null) {
throw result.ex4;
}
if (result.ex5 != null) {
throw result.ex5;
}
throw new org.apache.thrift.TApplicationException(org.apache.thrift.TApplicationException.MISSING_RESULT, "getApplicationInfo failed: unknown result");
}
public GetBuzzResponse getBuzz(GetBuzzRequest request) throws tech.aroma.thrift.exceptions.OperationFailedException, tech.aroma.thrift.exceptions.InvalidArgumentException, tech.aroma.thrift.exceptions.InvalidTokenException, tech.aroma.thrift.exceptions.ApplicationDoesNotExistException, tech.aroma.thrift.exceptions.UnauthorizedException, org.apache.thrift.TException
{
send_getBuzz(request);
return recv_getBuzz();
}
public void send_getBuzz(GetBuzzRequest request) throws org.apache.thrift.TException
{
getBuzz_args args = new getBuzz_args();
args.setRequest(request);
sendBase("getBuzz", args);
}
public GetBuzzResponse recv_getBuzz() throws tech.aroma.thrift.exceptions.OperationFailedException, tech.aroma.thrift.exceptions.InvalidArgumentException, tech.aroma.thrift.exceptions.InvalidTokenException, tech.aroma.thrift.exceptions.ApplicationDoesNotExistException, tech.aroma.thrift.exceptions.UnauthorizedException, org.apache.thrift.TException
{
getBuzz_result result = new getBuzz_result();
receiveBase(result, "getBuzz");
if (result.isSetSuccess()) {
return result.success;
}
if (result.ex1 != null) {
throw result.ex1;
}
if (result.ex2 != null) {
throw result.ex2;
}
if (result.ex3 != null) {
throw result.ex3;
}
if (result.ex4 != null) {
throw result.ex4;
}
if (result.ex5 != null) {
throw result.ex5;
}
throw new org.apache.thrift.TApplicationException(org.apache.thrift.TApplicationException.MISSING_RESULT, "getBuzz failed: unknown result");
}
public GetDashboardResponse getDashboard(GetDashboardRequest request) throws tech.aroma.thrift.exceptions.OperationFailedException, tech.aroma.thrift.exceptions.InvalidArgumentException, tech.aroma.thrift.exceptions.InvalidTokenException, org.apache.thrift.TException
{
send_getDashboard(request);
return recv_getDashboard();
}
public void send_getDashboard(GetDashboardRequest request) throws org.apache.thrift.TException
{
getDashboard_args args = new getDashboard_args();
args.setRequest(request);
sendBase("getDashboard", args);
}
public GetDashboardResponse recv_getDashboard() throws tech.aroma.thrift.exceptions.OperationFailedException, tech.aroma.thrift.exceptions.InvalidArgumentException, tech.aroma.thrift.exceptions.InvalidTokenException, org.apache.thrift.TException
{
getDashboard_result result = new getDashboard_result();
receiveBase(result, "getDashboard");
if (result.isSetSuccess()) {
return result.success;
}
if (result.ex1 != null) {
throw result.ex1;
}
if (result.ex2 != null) {
throw result.ex2;
}
if (result.ex3 != null) {
throw result.ex3;
}
throw new org.apache.thrift.TApplicationException(org.apache.thrift.TApplicationException.MISSING_RESULT, "getDashboard failed: unknown result");
}
public GetApplicationMessagesResponse getApplicationMessages(GetApplicationMessagesRequest request) throws tech.aroma.thrift.exceptions.OperationFailedException, tech.aroma.thrift.exceptions.InvalidArgumentException, tech.aroma.thrift.exceptions.InvalidTokenException, tech.aroma.thrift.exceptions.UnauthorizedException, tech.aroma.thrift.exceptions.ApplicationDoesNotExistException, org.apache.thrift.TException
{
send_getApplicationMessages(request);
return recv_getApplicationMessages();
}
public void send_getApplicationMessages(GetApplicationMessagesRequest request) throws org.apache.thrift.TException
{
getApplicationMessages_args args = new getApplicationMessages_args();
args.setRequest(request);
sendBase("getApplicationMessages", args);
}
public GetApplicationMessagesResponse recv_getApplicationMessages() throws tech.aroma.thrift.exceptions.OperationFailedException, tech.aroma.thrift.exceptions.InvalidArgumentException, tech.aroma.thrift.exceptions.InvalidTokenException, tech.aroma.thrift.exceptions.UnauthorizedException, tech.aroma.thrift.exceptions.ApplicationDoesNotExistException, org.apache.thrift.TException
{
getApplicationMessages_result result = new getApplicationMessages_result();
receiveBase(result, "getApplicationMessages");
if (result.isSetSuccess()) {
return result.success;
}
if (result.ex1 != null) {
throw result.ex1;
}
if (result.ex2 != null) {
throw result.ex2;
}
if (result.ex3 != null) {
throw result.ex3;
}
if (result.ex4 != null) {
throw result.ex4;
}
if (result.ex5 != null) {
throw result.ex5;
}
throw new org.apache.thrift.TApplicationException(org.apache.thrift.TApplicationException.MISSING_RESULT, "getApplicationMessages failed: unknown result");
}
public GetInboxResponse getInbox(GetInboxRequest request) throws tech.aroma.thrift.exceptions.OperationFailedException, tech.aroma.thrift.exceptions.InvalidArgumentException, tech.aroma.thrift.exceptions.InvalidTokenException, org.apache.thrift.TException
{
send_getInbox(request);
return recv_getInbox();
}
public void send_getInbox(GetInboxRequest request) throws org.apache.thrift.TException
{
getInbox_args args = new getInbox_args();
args.setRequest(request);
sendBase("getInbox", args);
}
public GetInboxResponse recv_getInbox() throws tech.aroma.thrift.exceptions.OperationFailedException, tech.aroma.thrift.exceptions.InvalidArgumentException, tech.aroma.thrift.exceptions.InvalidTokenException, org.apache.thrift.TException
{
getInbox_result result = new getInbox_result();
receiveBase(result, "getInbox");
if (result.isSetSuccess()) {
return result.success;
}
if (result.ex1 != null) {
throw result.ex1;
}
if (result.ex2 != null) {
throw result.ex2;
}
if (result.ex3 != null) {
throw result.ex3;
}
throw new org.apache.thrift.TApplicationException(org.apache.thrift.TApplicationException.MISSING_RESULT, "getInbox failed: unknown result");
}
public GetFullMessageResponse getFullMessage(GetFullMessageRequest request) throws tech.aroma.thrift.exceptions.OperationFailedException, tech.aroma.thrift.exceptions.InvalidArgumentException, tech.aroma.thrift.exceptions.InvalidTokenException, org.apache.thrift.TException
{
send_getFullMessage(request);
return recv_getFullMessage();
}
public void send_getFullMessage(GetFullMessageRequest request) throws org.apache.thrift.TException
{
getFullMessage_args args = new getFullMessage_args();
args.setRequest(request);
sendBase("getFullMessage", args);
}
public GetFullMessageResponse recv_getFullMessage() throws tech.aroma.thrift.exceptions.OperationFailedException, tech.aroma.thrift.exceptions.InvalidArgumentException, tech.aroma.thrift.exceptions.InvalidTokenException, org.apache.thrift.TException
{
getFullMessage_result result = new getFullMessage_result();
receiveBase(result, "getFullMessage");
if (result.isSetSuccess()) {
return result.success;
}
if (result.ex1 != null) {
throw result.ex1;
}
if (result.ex2 != null) {
throw result.ex2;
}
if (result.ex3 != null) {
throw result.ex3;
}
throw new org.apache.thrift.TApplicationException(org.apache.thrift.TApplicationException.MISSING_RESULT, "getFullMessage failed: unknown result");
}
public GetMediaResponse getMedia(GetMediaRequest request) throws tech.aroma.thrift.exceptions.OperationFailedException, tech.aroma.thrift.exceptions.InvalidArgumentException, tech.aroma.thrift.exceptions.InvalidTokenException, tech.aroma.thrift.exceptions.DoesNotExistException, tech.aroma.thrift.exceptions.UnauthorizedException, org.apache.thrift.TException
{
send_getMedia(request);
return recv_getMedia();
}
public void send_getMedia(GetMediaRequest request) throws org.apache.thrift.TException
{
getMedia_args args = new getMedia_args();
args.setRequest(request);
sendBase("getMedia", args);
}
public GetMediaResponse recv_getMedia() throws tech.aroma.thrift.exceptions.OperationFailedException, tech.aroma.thrift.exceptions.InvalidArgumentException, tech.aroma.thrift.exceptions.InvalidTokenException, tech.aroma.thrift.exceptions.DoesNotExistException, tech.aroma.thrift.exceptions.UnauthorizedException, org.apache.thrift.TException
{
getMedia_result result = new getMedia_result();
receiveBase(result, "getMedia");
if (result.isSetSuccess()) {
return result.success;
}
if (result.ex1 != null) {
throw result.ex1;
}
if (result.ex2 != null) {
throw result.ex2;
}
if (result.ex3 != null) {
throw result.ex3;
}
if (result.ex4 != null) {
throw result.ex4;
}
if (result.ex5 != null) {
throw result.ex5;
}
throw new org.apache.thrift.TApplicationException(org.apache.thrift.TApplicationException.MISSING_RESULT, "getMedia failed: unknown result");
}
public GetApplicationsOwnedByResponse getApplicationsOwnedBy(GetApplicationsOwnedByRequest request) throws tech.aroma.thrift.exceptions.OperationFailedException, tech.aroma.thrift.exceptions.InvalidArgumentException, tech.aroma.thrift.exceptions.InvalidTokenException, org.apache.thrift.TException
{
send_getApplicationsOwnedBy(request);
return recv_getApplicationsOwnedBy();
}
public void send_getApplicationsOwnedBy(GetApplicationsOwnedByRequest request) throws org.apache.thrift.TException
{
getApplicationsOwnedBy_args args = new getApplicationsOwnedBy_args();
args.setRequest(request);
sendBase("getApplicationsOwnedBy", args);
}
public GetApplicationsOwnedByResponse recv_getApplicationsOwnedBy() throws tech.aroma.thrift.exceptions.OperationFailedException, tech.aroma.thrift.exceptions.InvalidArgumentException, tech.aroma.thrift.exceptions.InvalidTokenException, org.apache.thrift.TException
{
getApplicationsOwnedBy_result result = new getApplicationsOwnedBy_result();
receiveBase(result, "getApplicationsOwnedBy");
if (result.isSetSuccess()) {
return result.success;
}
if (result.ex1 != null) {
throw result.ex1;
}
if (result.ex2 != null) {
throw result.ex2;
}
if (result.ex3 != null) {
throw result.ex3;
}
throw new org.apache.thrift.TApplicationException(org.apache.thrift.TApplicationException.MISSING_RESULT, "getApplicationsOwnedBy failed: unknown result");
}
public GetApplicationsFollowedByResponse getApplicationsFollowedBy(GetApplicationsFollowedByRequest request) throws tech.aroma.thrift.exceptions.OperationFailedException, tech.aroma.thrift.exceptions.InvalidArgumentException, tech.aroma.thrift.exceptions.InvalidTokenException, org.apache.thrift.TException
{
send_getApplicationsFollowedBy(request);
return recv_getApplicationsFollowedBy();
}
public void send_getApplicationsFollowedBy(GetApplicationsFollowedByRequest request) throws org.apache.thrift.TException
{
getApplicationsFollowedBy_args args = new getApplicationsFollowedBy_args();
args.setRequest(request);
sendBase("getApplicationsFollowedBy", args);
}
public GetApplicationsFollowedByResponse recv_getApplicationsFollowedBy() throws tech.aroma.thrift.exceptions.OperationFailedException, tech.aroma.thrift.exceptions.InvalidArgumentException, tech.aroma.thrift.exceptions.InvalidTokenException, org.apache.thrift.TException
{
getApplicationsFollowedBy_result result = new getApplicationsFollowedBy_result();
receiveBase(result, "getApplicationsFollowedBy");
if (result.isSetSuccess()) {
return result.success;
}
if (result.ex1 != null) {
throw result.ex1;
}
if (result.ex2 != null) {
throw result.ex2;
}
if (result.ex3 != null) {
throw result.ex3;
}
throw new org.apache.thrift.TApplicationException(org.apache.thrift.TApplicationException.MISSING_RESULT, "getApplicationsFollowedBy failed: unknown result");
}
public GetMySavedChannelsResponse getMySavedChannels(GetMySavedChannelsRequest request) throws tech.aroma.thrift.exceptions.OperationFailedException, tech.aroma.thrift.exceptions.InvalidArgumentException, tech.aroma.thrift.exceptions.InvalidTokenException, org.apache.thrift.TException
{
send_getMySavedChannels(request);
return recv_getMySavedChannels();
}
public void send_getMySavedChannels(GetMySavedChannelsRequest request) throws org.apache.thrift.TException
{
getMySavedChannels_args args = new getMySavedChannels_args();
args.setRequest(request);
sendBase("getMySavedChannels", args);
}
public GetMySavedChannelsResponse recv_getMySavedChannels() throws tech.aroma.thrift.exceptions.OperationFailedException, tech.aroma.thrift.exceptions.InvalidArgumentException, tech.aroma.thrift.exceptions.InvalidTokenException, org.apache.thrift.TException
{
getMySavedChannels_result result = new getMySavedChannels_result();
receiveBase(result, "getMySavedChannels");
if (result.isSetSuccess()) {
return result.success;
}
if (result.ex1 != null) {
throw result.ex1;
}
if (result.ex2 != null) {
throw result.ex2;
}
if (result.ex3 != null) {
throw result.ex3;
}
throw new org.apache.thrift.TApplicationException(org.apache.thrift.TApplicationException.MISSING_RESULT, "getMySavedChannels failed: unknown result");
}
public GetReactionsResponse getReactions(GetReactionsRequest request) throws tech.aroma.thrift.exceptions.OperationFailedException, tech.aroma.thrift.exceptions.InvalidArgumentException, tech.aroma.thrift.exceptions.InvalidTokenException, tech.aroma.thrift.exceptions.ApplicationDoesNotExistException, tech.aroma.thrift.exceptions.UnauthorizedException, org.apache.thrift.TException
{
send_getReactions(request);
return recv_getReactions();
}
public void send_getReactions(GetReactionsRequest request) throws org.apache.thrift.TException
{
getReactions_args args = new getReactions_args();
args.setRequest(request);
sendBase("getReactions", args);
}
public GetReactionsResponse recv_getReactions() throws tech.aroma.thrift.exceptions.OperationFailedException, tech.aroma.thrift.exceptions.InvalidArgumentException, tech.aroma.thrift.exceptions.InvalidTokenException, tech.aroma.thrift.exceptions.ApplicationDoesNotExistException, tech.aroma.thrift.exceptions.UnauthorizedException, org.apache.thrift.TException
{
getReactions_result result = new getReactions_result();
receiveBase(result, "getReactions");
if (result.isSetSuccess()) {
return result.success;
}
if (result.ex1 != null) {
throw result.ex1;
}
if (result.ex2 != null) {
throw result.ex2;
}
if (result.ex3 != null) {
throw result.ex3;
}
if (result.ex4 != null) {
throw result.ex4;
}
if (result.ex5 != null) {
throw result.ex5;
}
throw new org.apache.thrift.TApplicationException(org.apache.thrift.TApplicationException.MISSING_RESULT, "getReactions failed: unknown result");
}
public GetUserInfoResponse getUserInfo(GetUserInfoRequest request) throws tech.aroma.thrift.exceptions.OperationFailedException, tech.aroma.thrift.exceptions.InvalidArgumentException, tech.aroma.thrift.exceptions.InvalidTokenException, tech.aroma.thrift.exceptions.UnauthorizedException, tech.aroma.thrift.exceptions.UserDoesNotExistException, org.apache.thrift.TException
{
send_getUserInfo(request);
return recv_getUserInfo();
}
public void send_getUserInfo(GetUserInfoRequest request) throws org.apache.thrift.TException
{
getUserInfo_args args = new getUserInfo_args();
args.setRequest(request);
sendBase("getUserInfo", args);
}
public GetUserInfoResponse recv_getUserInfo() throws tech.aroma.thrift.exceptions.OperationFailedException, tech.aroma.thrift.exceptions.InvalidArgumentException, tech.aroma.thrift.exceptions.InvalidTokenException, tech.aroma.thrift.exceptions.UnauthorizedException, tech.aroma.thrift.exceptions.UserDoesNotExistException, org.apache.thrift.TException
{
getUserInfo_result result = new getUserInfo_result();
receiveBase(result, "getUserInfo");
if (result.isSetSuccess()) {
return result.success;
}
if (result.ex1 != null) {
throw result.ex1;
}
if (result.ex2 != null) {
throw result.ex2;
}
if (result.ex3 != null) {
throw result.ex3;
}
if (result.ex4 != null) {
throw result.ex4;
}
if (result.ex5 != null) {
throw result.ex5;
}
throw new org.apache.thrift.TApplicationException(org.apache.thrift.TApplicationException.MISSING_RESULT, "getUserInfo failed: unknown result");
}
public SearchForApplicationsResponse searchForApplications(SearchForApplicationsRequest request) throws tech.aroma.thrift.exceptions.OperationFailedException, tech.aroma.thrift.exceptions.InvalidArgumentException, tech.aroma.thrift.exceptions.InvalidTokenException, tech.aroma.thrift.exceptions.UnauthorizedException, org.apache.thrift.TException
{
send_searchForApplications(request);
return recv_searchForApplications();
}
public void send_searchForApplications(SearchForApplicationsRequest request) throws org.apache.thrift.TException
{
searchForApplications_args args = new searchForApplications_args();
args.setRequest(request);
sendBase("searchForApplications", args);
}
public SearchForApplicationsResponse recv_searchForApplications() throws tech.aroma.thrift.exceptions.OperationFailedException, tech.aroma.thrift.exceptions.InvalidArgumentException, tech.aroma.thrift.exceptions.InvalidTokenException, tech.aroma.thrift.exceptions.UnauthorizedException, org.apache.thrift.TException
{
searchForApplications_result result = new searchForApplications_result();
receiveBase(result, "searchForApplications");
if (result.isSetSuccess()) {
return result.success;
}
if (result.ex1 != null) {
throw result.ex1;
}
if (result.ex2 != null) {
throw result.ex2;
}
if (result.ex3 != null) {
throw result.ex3;
}
if (result.ex4 != null) {
throw result.ex4;
}
throw new org.apache.thrift.TApplicationException(org.apache.thrift.TApplicationException.MISSING_RESULT, "searchForApplications failed: unknown result");
}
}
public static class AsyncClient extends org.apache.thrift.async.TAsyncClient implements AsyncIface {
public static class Factory implements org.apache.thrift.async.TAsyncClientFactory {
private org.apache.thrift.async.TAsyncClientManager clientManager;
private org.apache.thrift.protocol.TProtocolFactory protocolFactory;
public Factory(org.apache.thrift.async.TAsyncClientManager clientManager, org.apache.thrift.protocol.TProtocolFactory protocolFactory) {
this.clientManager = clientManager;
this.protocolFactory = protocolFactory;
}
public AsyncClient getAsyncClient(org.apache.thrift.transport.TNonblockingTransport transport) {
return new AsyncClient(protocolFactory, clientManager, transport);
}
}
public AsyncClient(org.apache.thrift.protocol.TProtocolFactory protocolFactory, org.apache.thrift.async.TAsyncClientManager clientManager, org.apache.thrift.transport.TNonblockingTransport transport) {
super(protocolFactory, clientManager, transport);
}
public void getApiVersion(org.apache.thrift.async.AsyncMethodCallback resultHandler) throws org.apache.thrift.TException {
checkReady();
getApiVersion_call method_call = new getApiVersion_call(resultHandler, this, ___protocolFactory, ___transport);
this.___currentMethod = method_call;
___manager.call(method_call);
}
public static class getApiVersion_call extends org.apache.thrift.async.TAsyncMethodCall {
public getApiVersion_call(org.apache.thrift.async.AsyncMethodCallback resultHandler, org.apache.thrift.async.TAsyncClient client, org.apache.thrift.protocol.TProtocolFactory protocolFactory, org.apache.thrift.transport.TNonblockingTransport transport) throws org.apache.thrift.TException {
super(client, protocolFactory, transport, resultHandler, false);
}
public void write_args(org.apache.thrift.protocol.TProtocol prot) throws org.apache.thrift.TException {
prot.writeMessageBegin(new org.apache.thrift.protocol.TMessage("getApiVersion", org.apache.thrift.protocol.TMessageType.CALL, 0));
getApiVersion_args args = new getApiVersion_args();
args.write(prot);
prot.writeMessageEnd();
}
public double getResult() throws org.apache.thrift.TException {
if (getState() != org.apache.thrift.async.TAsyncMethodCall.State.RESPONSE_READ) {
throw new IllegalStateException("Method call not finished!");
}
org.apache.thrift.transport.TMemoryInputTransport memoryTransport = new org.apache.thrift.transport.TMemoryInputTransport(getFrameBuffer().array());
org.apache.thrift.protocol.TProtocol prot = client.getProtocolFactory().getProtocol(memoryTransport);
return (new Client(prot)).recv_getApiVersion();
}
}
public void deleteApplication(DeleteApplicationRequest request, org.apache.thrift.async.AsyncMethodCallback resultHandler) throws org.apache.thrift.TException {
checkReady();
deleteApplication_call method_call = new deleteApplication_call(request, resultHandler, this, ___protocolFactory, ___transport);
this.___currentMethod = method_call;
___manager.call(method_call);
}
public static class deleteApplication_call extends org.apache.thrift.async.TAsyncMethodCall {
private DeleteApplicationRequest request;
public deleteApplication_call(DeleteApplicationRequest request, org.apache.thrift.async.AsyncMethodCallback resultHandler, org.apache.thrift.async.TAsyncClient client, org.apache.thrift.protocol.TProtocolFactory protocolFactory, org.apache.thrift.transport.TNonblockingTransport transport) throws org.apache.thrift.TException {
super(client, protocolFactory, transport, resultHandler, false);
this.request = request;
}
public void write_args(org.apache.thrift.protocol.TProtocol prot) throws org.apache.thrift.TException {
prot.writeMessageBegin(new org.apache.thrift.protocol.TMessage("deleteApplication", org.apache.thrift.protocol.TMessageType.CALL, 0));
deleteApplication_args args = new deleteApplication_args();
args.setRequest(request);
args.write(prot);
prot.writeMessageEnd();
}
public DeleteApplicationResponse getResult() throws tech.aroma.thrift.exceptions.OperationFailedException, tech.aroma.thrift.exceptions.InvalidArgumentException, tech.aroma.thrift.exceptions.InvalidTokenException, tech.aroma.thrift.exceptions.ApplicationDoesNotExistException, tech.aroma.thrift.exceptions.UnauthorizedException, org.apache.thrift.TException {
if (getState() != org.apache.thrift.async.TAsyncMethodCall.State.RESPONSE_READ) {
throw new IllegalStateException("Method call not finished!");
}
org.apache.thrift.transport.TMemoryInputTransport memoryTransport = new org.apache.thrift.transport.TMemoryInputTransport(getFrameBuffer().array());
org.apache.thrift.protocol.TProtocol prot = client.getProtocolFactory().getProtocol(memoryTransport);
return (new Client(prot)).recv_deleteApplication();
}
}
public void deleteMessage(DeleteMessageRequest request, org.apache.thrift.async.AsyncMethodCallback resultHandler) throws org.apache.thrift.TException {
checkReady();
deleteMessage_call method_call = new deleteMessage_call(request, resultHandler, this, ___protocolFactory, ___transport);
this.___currentMethod = method_call;
___manager.call(method_call);
}
public static class deleteMessage_call extends org.apache.thrift.async.TAsyncMethodCall {
private DeleteMessageRequest request;
public deleteMessage_call(DeleteMessageRequest request, org.apache.thrift.async.AsyncMethodCallback resultHandler, org.apache.thrift.async.TAsyncClient client, org.apache.thrift.protocol.TProtocolFactory protocolFactory, org.apache.thrift.transport.TNonblockingTransport transport) throws org.apache.thrift.TException {
super(client, protocolFactory, transport, resultHandler, false);
this.request = request;
}
public void write_args(org.apache.thrift.protocol.TProtocol prot) throws org.apache.thrift.TException {
prot.writeMessageBegin(new org.apache.thrift.protocol.TMessage("deleteMessage", org.apache.thrift.protocol.TMessageType.CALL, 0));
deleteMessage_args args = new deleteMessage_args();
args.setRequest(request);
args.write(prot);
prot.writeMessageEnd();
}
public DeleteMessageResponse getResult() throws tech.aroma.thrift.exceptions.OperationFailedException, tech.aroma.thrift.exceptions.InvalidArgumentException, tech.aroma.thrift.exceptions.InvalidTokenException, tech.aroma.thrift.exceptions.MessageDoesNotExistException, tech.aroma.thrift.exceptions.UnauthorizedException, org.apache.thrift.TException {
if (getState() != org.apache.thrift.async.TAsyncMethodCall.State.RESPONSE_READ) {
throw new IllegalStateException("Method call not finished!");
}
org.apache.thrift.transport.TMemoryInputTransport memoryTransport = new org.apache.thrift.transport.TMemoryInputTransport(getFrameBuffer().array());
org.apache.thrift.protocol.TProtocol prot = client.getProtocolFactory().getProtocol(memoryTransport);
return (new Client(prot)).recv_deleteMessage();
}
}
public void dismissMessage(DismissMessageRequest request, org.apache.thrift.async.AsyncMethodCallback resultHandler) throws org.apache.thrift.TException {
checkReady();
dismissMessage_call method_call = new dismissMessage_call(request, resultHandler, this, ___protocolFactory, ___transport);
this.___currentMethod = method_call;
___manager.call(method_call);
}
public static class dismissMessage_call extends org.apache.thrift.async.TAsyncMethodCall {
private DismissMessageRequest request;
public dismissMessage_call(DismissMessageRequest request, org.apache.thrift.async.AsyncMethodCallback resultHandler, org.apache.thrift.async.TAsyncClient client, org.apache.thrift.protocol.TProtocolFactory protocolFactory, org.apache.thrift.transport.TNonblockingTransport transport) throws org.apache.thrift.TException {
super(client, protocolFactory, transport, resultHandler, false);
this.request = request;
}
public void write_args(org.apache.thrift.protocol.TProtocol prot) throws org.apache.thrift.TException {
prot.writeMessageBegin(new org.apache.thrift.protocol.TMessage("dismissMessage", org.apache.thrift.protocol.TMessageType.CALL, 0));
dismissMessage_args args = new dismissMessage_args();
args.setRequest(request);
args.write(prot);
prot.writeMessageEnd();
}
public DismissMessageResponse getResult() throws tech.aroma.thrift.exceptions.OperationFailedException, tech.aroma.thrift.exceptions.InvalidArgumentException, tech.aroma.thrift.exceptions.InvalidTokenException, tech.aroma.thrift.exceptions.MessageDoesNotExistException, tech.aroma.thrift.exceptions.UnauthorizedException, org.apache.thrift.TException {
if (getState() != org.apache.thrift.async.TAsyncMethodCall.State.RESPONSE_READ) {
throw new IllegalStateException("Method call not finished!");
}
org.apache.thrift.transport.TMemoryInputTransport memoryTransport = new org.apache.thrift.transport.TMemoryInputTransport(getFrameBuffer().array());
org.apache.thrift.protocol.TProtocol prot = client.getProtocolFactory().getProtocol(memoryTransport);
return (new Client(prot)).recv_dismissMessage();
}
}
public void provisionApplication(ProvisionApplicationRequest request, org.apache.thrift.async.AsyncMethodCallback resultHandler) throws org.apache.thrift.TException {
checkReady();
provisionApplication_call method_call = new provisionApplication_call(request, resultHandler, this, ___protocolFactory, ___transport);
this.___currentMethod = method_call;
___manager.call(method_call);
}
public static class provisionApplication_call extends org.apache.thrift.async.TAsyncMethodCall {
private ProvisionApplicationRequest request;
public provisionApplication_call(ProvisionApplicationRequest request, org.apache.thrift.async.AsyncMethodCallback resultHandler, org.apache.thrift.async.TAsyncClient client, org.apache.thrift.protocol.TProtocolFactory protocolFactory, org.apache.thrift.transport.TNonblockingTransport transport) throws org.apache.thrift.TException {
super(client, protocolFactory, transport, resultHandler, false);
this.request = request;
}
public void write_args(org.apache.thrift.protocol.TProtocol prot) throws org.apache.thrift.TException {
prot.writeMessageBegin(new org.apache.thrift.protocol.TMessage("provisionApplication", org.apache.thrift.protocol.TMessageType.CALL, 0));
provisionApplication_args args = new provisionApplication_args();
args.setRequest(request);
args.write(prot);
prot.writeMessageEnd();
}
public ProvisionApplicationResponse getResult() throws tech.aroma.thrift.exceptions.OperationFailedException, tech.aroma.thrift.exceptions.InvalidArgumentException, tech.aroma.thrift.exceptions.InvalidTokenException, tech.aroma.thrift.exceptions.ApplicationDoesNotExistException, tech.aroma.thrift.exceptions.UnauthorizedException, org.apache.thrift.TException {
if (getState() != org.apache.thrift.async.TAsyncMethodCall.State.RESPONSE_READ) {
throw new IllegalStateException("Method call not finished!");
}
org.apache.thrift.transport.TMemoryInputTransport memoryTransport = new org.apache.thrift.transport.TMemoryInputTransport(getFrameBuffer().array());
org.apache.thrift.protocol.TProtocol prot = client.getProtocolFactory().getProtocol(memoryTransport);
return (new Client(prot)).recv_provisionApplication();
}
}
public void regenerateToken(RegenerateApplicationTokenRequest request, org.apache.thrift.async.AsyncMethodCallback resultHandler) throws org.apache.thrift.TException {
checkReady();
regenerateToken_call method_call = new regenerateToken_call(request, resultHandler, this, ___protocolFactory, ___transport);
this.___currentMethod = method_call;
___manager.call(method_call);
}
public static class regenerateToken_call extends org.apache.thrift.async.TAsyncMethodCall {
private RegenerateApplicationTokenRequest request;
public regenerateToken_call(RegenerateApplicationTokenRequest request, org.apache.thrift.async.AsyncMethodCallback resultHandler, org.apache.thrift.async.TAsyncClient client, org.apache.thrift.protocol.TProtocolFactory protocolFactory, org.apache.thrift.transport.TNonblockingTransport transport) throws org.apache.thrift.TException {
super(client, protocolFactory, transport, resultHandler, false);
this.request = request;
}
public void write_args(org.apache.thrift.protocol.TProtocol prot) throws org.apache.thrift.TException {
prot.writeMessageBegin(new org.apache.thrift.protocol.TMessage("regenerateToken", org.apache.thrift.protocol.TMessageType.CALL, 0));
regenerateToken_args args = new regenerateToken_args();
args.setRequest(request);
args.write(prot);
prot.writeMessageEnd();
}
public RegenerateApplicationTokenResponse getResult() throws tech.aroma.thrift.exceptions.OperationFailedException, tech.aroma.thrift.exceptions.InvalidArgumentException, tech.aroma.thrift.exceptions.InvalidTokenException, tech.aroma.thrift.exceptions.ApplicationDoesNotExistException, tech.aroma.thrift.exceptions.UnauthorizedException, org.apache.thrift.TException {
if (getState() != org.apache.thrift.async.TAsyncMethodCall.State.RESPONSE_READ) {
throw new IllegalStateException("Method call not finished!");
}
org.apache.thrift.transport.TMemoryInputTransport memoryTransport = new org.apache.thrift.transport.TMemoryInputTransport(getFrameBuffer().array());
org.apache.thrift.protocol.TProtocol prot = client.getProtocolFactory().getProtocol(memoryTransport);
return (new Client(prot)).recv_regenerateToken();
}
}
public void registerHealthCheck(RegisterHealthCheckRequest request, org.apache.thrift.async.AsyncMethodCallback resultHandler) throws org.apache.thrift.TException {
checkReady();
registerHealthCheck_call method_call = new registerHealthCheck_call(request, resultHandler, this, ___protocolFactory, ___transport);
this.___currentMethod = method_call;
___manager.call(method_call);
}
public static class registerHealthCheck_call extends org.apache.thrift.async.TAsyncMethodCall {
private RegisterHealthCheckRequest request;
public registerHealthCheck_call(RegisterHealthCheckRequest request, org.apache.thrift.async.AsyncMethodCallback resultHandler, org.apache.thrift.async.TAsyncClient client, org.apache.thrift.protocol.TProtocolFactory protocolFactory, org.apache.thrift.transport.TNonblockingTransport transport) throws org.apache.thrift.TException {
super(client, protocolFactory, transport, resultHandler, false);
this.request = request;
}
public void write_args(org.apache.thrift.protocol.TProtocol prot) throws org.apache.thrift.TException {
prot.writeMessageBegin(new org.apache.thrift.protocol.TMessage("registerHealthCheck", org.apache.thrift.protocol.TMessageType.CALL, 0));
registerHealthCheck_args args = new registerHealthCheck_args();
args.setRequest(request);
args.write(prot);
prot.writeMessageEnd();
}
public RegisterHealthCheckResponse getResult() throws tech.aroma.thrift.exceptions.OperationFailedException, tech.aroma.thrift.exceptions.InvalidArgumentException, tech.aroma.thrift.exceptions.InvalidTokenException, tech.aroma.thrift.exceptions.ApplicationDoesNotExistException, tech.aroma.thrift.exceptions.UnauthorizedException, org.apache.thrift.TException {
if (getState() != org.apache.thrift.async.TAsyncMethodCall.State.RESPONSE_READ) {
throw new IllegalStateException("Method call not finished!");
}
org.apache.thrift.transport.TMemoryInputTransport memoryTransport = new org.apache.thrift.transport.TMemoryInputTransport(getFrameBuffer().array());
org.apache.thrift.protocol.TProtocol prot = client.getProtocolFactory().getProtocol(memoryTransport);
return (new Client(prot)).recv_registerHealthCheck();
}
}
public void removeSavedChannel(RemoveSavedChannelRequest request, org.apache.thrift.async.AsyncMethodCallback resultHandler) throws org.apache.thrift.TException {
checkReady();
removeSavedChannel_call method_call = new removeSavedChannel_call(request, resultHandler, this, ___protocolFactory, ___transport);
this.___currentMethod = method_call;
___manager.call(method_call);
}
public static class removeSavedChannel_call extends org.apache.thrift.async.TAsyncMethodCall {
private RemoveSavedChannelRequest request;
public removeSavedChannel_call(RemoveSavedChannelRequest request, org.apache.thrift.async.AsyncMethodCallback resultHandler, org.apache.thrift.async.TAsyncClient client, org.apache.thrift.protocol.TProtocolFactory protocolFactory, org.apache.thrift.transport.TNonblockingTransport transport) throws org.apache.thrift.TException {
super(client, protocolFactory, transport, resultHandler, false);
this.request = request;
}
public void write_args(org.apache.thrift.protocol.TProtocol prot) throws org.apache.thrift.TException {
prot.writeMessageBegin(new org.apache.thrift.protocol.TMessage("removeSavedChannel", org.apache.thrift.protocol.TMessageType.CALL, 0));
removeSavedChannel_args args = new removeSavedChannel_args();
args.setRequest(request);
args.write(prot);
prot.writeMessageEnd();
}
public RemoveSavedChannelResponse getResult() throws tech.aroma.thrift.exceptions.OperationFailedException, tech.aroma.thrift.exceptions.InvalidArgumentException, tech.aroma.thrift.exceptions.InvalidTokenException, tech.aroma.thrift.exceptions.UnauthorizedException, tech.aroma.thrift.exceptions.ChannelDoesNotExistException, org.apache.thrift.TException {
if (getState() != org.apache.thrift.async.TAsyncMethodCall.State.RESPONSE_READ) {
throw new IllegalStateException("Method call not finished!");
}
org.apache.thrift.transport.TMemoryInputTransport memoryTransport = new org.apache.thrift.transport.TMemoryInputTransport(getFrameBuffer().array());
org.apache.thrift.protocol.TProtocol prot = client.getProtocolFactory().getProtocol(memoryTransport);
return (new Client(prot)).recv_removeSavedChannel();
}
}
public void renewApplicationToken(RenewApplicationTokenRequest request, org.apache.thrift.async.AsyncMethodCallback resultHandler) throws org.apache.thrift.TException {
checkReady();
renewApplicationToken_call method_call = new renewApplicationToken_call(request, resultHandler, this, ___protocolFactory, ___transport);
this.___currentMethod = method_call;
___manager.call(method_call);
}
public static class renewApplicationToken_call extends org.apache.thrift.async.TAsyncMethodCall {
private RenewApplicationTokenRequest request;
public renewApplicationToken_call(RenewApplicationTokenRequest request, org.apache.thrift.async.AsyncMethodCallback resultHandler, org.apache.thrift.async.TAsyncClient client, org.apache.thrift.protocol.TProtocolFactory protocolFactory, org.apache.thrift.transport.TNonblockingTransport transport) throws org.apache.thrift.TException {
super(client, protocolFactory, transport, resultHandler, false);
this.request = request;
}
public void write_args(org.apache.thrift.protocol.TProtocol prot) throws org.apache.thrift.TException {
prot.writeMessageBegin(new org.apache.thrift.protocol.TMessage("renewApplicationToken", org.apache.thrift.protocol.TMessageType.CALL, 0));
renewApplicationToken_args args = new renewApplicationToken_args();
args.setRequest(request);
args.write(prot);
prot.writeMessageEnd();
}
public RenewApplicationTokenResponse getResult() throws tech.aroma.thrift.exceptions.OperationFailedException, tech.aroma.thrift.exceptions.InvalidArgumentException, tech.aroma.thrift.exceptions.InvalidTokenException, tech.aroma.thrift.exceptions.ApplicationDoesNotExistException, tech.aroma.thrift.exceptions.UnauthorizedException, org.apache.thrift.TException {
if (getState() != org.apache.thrift.async.TAsyncMethodCall.State.RESPONSE_READ) {
throw new IllegalStateException("Method call not finished!");
}
org.apache.thrift.transport.TMemoryInputTransport memoryTransport = new org.apache.thrift.transport.TMemoryInputTransport(getFrameBuffer().array());
org.apache.thrift.protocol.TProtocol prot = client.getProtocolFactory().getProtocol(memoryTransport);
return (new Client(prot)).recv_renewApplicationToken();
}
}
public void saveChannel(SaveChannelRequest request, org.apache.thrift.async.AsyncMethodCallback resultHandler) throws org.apache.thrift.TException {
checkReady();
saveChannel_call method_call = new saveChannel_call(request, resultHandler, this, ___protocolFactory, ___transport);
this.___currentMethod = method_call;
___manager.call(method_call);
}
public static class saveChannel_call extends org.apache.thrift.async.TAsyncMethodCall {
private SaveChannelRequest request;
public saveChannel_call(SaveChannelRequest request, org.apache.thrift.async.AsyncMethodCallback resultHandler, org.apache.thrift.async.TAsyncClient client, org.apache.thrift.protocol.TProtocolFactory protocolFactory, org.apache.thrift.transport.TNonblockingTransport transport) throws org.apache.thrift.TException {
super(client, protocolFactory, transport, resultHandler, false);
this.request = request;
}
public void write_args(org.apache.thrift.protocol.TProtocol prot) throws org.apache.thrift.TException {
prot.writeMessageBegin(new org.apache.thrift.protocol.TMessage("saveChannel", org.apache.thrift.protocol.TMessageType.CALL, 0));
saveChannel_args args = new saveChannel_args();
args.setRequest(request);
args.write(prot);
prot.writeMessageEnd();
}
public SaveChannelResponse getResult() throws tech.aroma.thrift.exceptions.OperationFailedException, tech.aroma.thrift.exceptions.InvalidArgumentException, tech.aroma.thrift.exceptions.InvalidTokenException, tech.aroma.thrift.exceptions.UnauthorizedException, org.apache.thrift.TException {
if (getState() != org.apache.thrift.async.TAsyncMethodCall.State.RESPONSE_READ) {
throw new IllegalStateException("Method call not finished!");
}
org.apache.thrift.transport.TMemoryInputTransport memoryTransport = new org.apache.thrift.transport.TMemoryInputTransport(getFrameBuffer().array());
org.apache.thrift.protocol.TProtocol prot = client.getProtocolFactory().getProtocol(memoryTransport);
return (new Client(prot)).recv_saveChannel();
}
}
public void signIn(SignInRequest request, org.apache.thrift.async.AsyncMethodCallback resultHandler) throws org.apache.thrift.TException {
checkReady();
signIn_call method_call = new signIn_call(request, resultHandler, this, ___protocolFactory, ___transport);
this.___currentMethod = method_call;
___manager.call(method_call);
}
public static class signIn_call extends org.apache.thrift.async.TAsyncMethodCall {
private SignInRequest request;
public signIn_call(SignInRequest request, org.apache.thrift.async.AsyncMethodCallback resultHandler, org.apache.thrift.async.TAsyncClient client, org.apache.thrift.protocol.TProtocolFactory protocolFactory, org.apache.thrift.transport.TNonblockingTransport transport) throws org.apache.thrift.TException {
super(client, protocolFactory, transport, resultHandler, false);
this.request = request;
}
public void write_args(org.apache.thrift.protocol.TProtocol prot) throws org.apache.thrift.TException {
prot.writeMessageBegin(new org.apache.thrift.protocol.TMessage("signIn", org.apache.thrift.protocol.TMessageType.CALL, 0));
signIn_args args = new signIn_args();
args.setRequest(request);
args.write(prot);
prot.writeMessageEnd();
}
public SignInResponse getResult() throws tech.aroma.thrift.exceptions.OperationFailedException, tech.aroma.thrift.exceptions.InvalidArgumentException, tech.aroma.thrift.exceptions.InvalidCredentialsException, tech.aroma.thrift.exceptions.UserDoesNotExistException, org.apache.thrift.TException {
if (getState() != org.apache.thrift.async.TAsyncMethodCall.State.RESPONSE_READ) {
throw new IllegalStateException("Method call not finished!");
}
org.apache.thrift.transport.TMemoryInputTransport memoryTransport = new org.apache.thrift.transport.TMemoryInputTransport(getFrameBuffer().array());
org.apache.thrift.protocol.TProtocol prot = client.getProtocolFactory().getProtocol(memoryTransport);
return (new Client(prot)).recv_signIn();
}
}
public void signUp(SignUpRequest request, org.apache.thrift.async.AsyncMethodCallback resultHandler) throws org.apache.thrift.TException {
checkReady();
signUp_call method_call = new signUp_call(request, resultHandler, this, ___protocolFactory, ___transport);
this.___currentMethod = method_call;
___manager.call(method_call);
}
public static class signUp_call extends org.apache.thrift.async.TAsyncMethodCall {
private SignUpRequest request;
public signUp_call(SignUpRequest request, org.apache.thrift.async.AsyncMethodCallback resultHandler, org.apache.thrift.async.TAsyncClient client, org.apache.thrift.protocol.TProtocolFactory protocolFactory, org.apache.thrift.transport.TNonblockingTransport transport) throws org.apache.thrift.TException {
super(client, protocolFactory, transport, resultHandler, false);
this.request = request;
}
public void write_args(org.apache.thrift.protocol.TProtocol prot) throws org.apache.thrift.TException {
prot.writeMessageBegin(new org.apache.thrift.protocol.TMessage("signUp", org.apache.thrift.protocol.TMessageType.CALL, 0));
signUp_args args = new signUp_args();
args.setRequest(request);
args.write(prot);
prot.writeMessageEnd();
}
public SignUpResponse getResult() throws tech.aroma.thrift.exceptions.OperationFailedException, tech.aroma.thrift.exceptions.InvalidArgumentException, tech.aroma.thrift.exceptions.InvalidCredentialsException, tech.aroma.thrift.exceptions.AccountAlreadyExistsException, org.apache.thrift.TException {
if (getState() != org.apache.thrift.async.TAsyncMethodCall.State.RESPONSE_READ) {
throw new IllegalStateException("Method call not finished!");
}
org.apache.thrift.transport.TMemoryInputTransport memoryTransport = new org.apache.thrift.transport.TMemoryInputTransport(getFrameBuffer().array());
org.apache.thrift.protocol.TProtocol prot = client.getProtocolFactory().getProtocol(memoryTransport);
return (new Client(prot)).recv_signUp();
}
}
public void snoozeChannel(SnoozeChannelRequest request, org.apache.thrift.async.AsyncMethodCallback resultHandler) throws org.apache.thrift.TException {
checkReady();
snoozeChannel_call method_call = new snoozeChannel_call(request, resultHandler, this, ___protocolFactory, ___transport);
this.___currentMethod = method_call;
___manager.call(method_call);
}
public static class snoozeChannel_call extends org.apache.thrift.async.TAsyncMethodCall {
private SnoozeChannelRequest request;
public snoozeChannel_call(SnoozeChannelRequest request, org.apache.thrift.async.AsyncMethodCallback resultHandler, org.apache.thrift.async.TAsyncClient client, org.apache.thrift.protocol.TProtocolFactory protocolFactory, org.apache.thrift.transport.TNonblockingTransport transport) throws org.apache.thrift.TException {
super(client, protocolFactory, transport, resultHandler, false);
this.request = request;
}
public void write_args(org.apache.thrift.protocol.TProtocol prot) throws org.apache.thrift.TException {
prot.writeMessageBegin(new org.apache.thrift.protocol.TMessage("snoozeChannel", org.apache.thrift.protocol.TMessageType.CALL, 0));
snoozeChannel_args args = new snoozeChannel_args();
args.setRequest(request);
args.write(prot);
prot.writeMessageEnd();
}
public SnoozeChannelResponse getResult() throws tech.aroma.thrift.exceptions.OperationFailedException, tech.aroma.thrift.exceptions.InvalidArgumentException, tech.aroma.thrift.exceptions.InvalidTokenException, tech.aroma.thrift.exceptions.UnauthorizedException, tech.aroma.thrift.exceptions.ChannelDoesNotExistException, org.apache.thrift.TException {
if (getState() != org.apache.thrift.async.TAsyncMethodCall.State.RESPONSE_READ) {
throw new IllegalStateException("Method call not finished!");
}
org.apache.thrift.transport.TMemoryInputTransport memoryTransport = new org.apache.thrift.transport.TMemoryInputTransport(getFrameBuffer().array());
org.apache.thrift.protocol.TProtocol prot = client.getProtocolFactory().getProtocol(memoryTransport);
return (new Client(prot)).recv_snoozeChannel();
}
}
public void followApplication(FollowApplicationRequest request, org.apache.thrift.async.AsyncMethodCallback resultHandler) throws org.apache.thrift.TException {
checkReady();
followApplication_call method_call = new followApplication_call(request, resultHandler, this, ___protocolFactory, ___transport);
this.___currentMethod = method_call;
___manager.call(method_call);
}
public static class followApplication_call extends org.apache.thrift.async.TAsyncMethodCall {
private FollowApplicationRequest request;
public followApplication_call(FollowApplicationRequest request, org.apache.thrift.async.AsyncMethodCallback resultHandler, org.apache.thrift.async.TAsyncClient client, org.apache.thrift.protocol.TProtocolFactory protocolFactory, org.apache.thrift.transport.TNonblockingTransport transport) throws org.apache.thrift.TException {
super(client, protocolFactory, transport, resultHandler, false);
this.request = request;
}
public void write_args(org.apache.thrift.protocol.TProtocol prot) throws org.apache.thrift.TException {
prot.writeMessageBegin(new org.apache.thrift.protocol.TMessage("followApplication", org.apache.thrift.protocol.TMessageType.CALL, 0));
followApplication_args args = new followApplication_args();
args.setRequest(request);
args.write(prot);
prot.writeMessageEnd();
}
public FollowApplicationResponse getResult() throws tech.aroma.thrift.exceptions.OperationFailedException, tech.aroma.thrift.exceptions.InvalidArgumentException, tech.aroma.thrift.exceptions.InvalidTokenException, tech.aroma.thrift.exceptions.ApplicationDoesNotExistException, tech.aroma.thrift.exceptions.UnauthorizedException, org.apache.thrift.TException {
if (getState() != org.apache.thrift.async.TAsyncMethodCall.State.RESPONSE_READ) {
throw new IllegalStateException("Method call not finished!");
}
org.apache.thrift.transport.TMemoryInputTransport memoryTransport = new org.apache.thrift.transport.TMemoryInputTransport(getFrameBuffer().array());
org.apache.thrift.protocol.TProtocol prot = client.getProtocolFactory().getProtocol(memoryTransport);
return (new Client(prot)).recv_followApplication();
}
}
public void unfollowApplication(UnfollowApplicationRequest request, org.apache.thrift.async.AsyncMethodCallback resultHandler) throws org.apache.thrift.TException {
checkReady();
unfollowApplication_call method_call = new unfollowApplication_call(request, resultHandler, this, ___protocolFactory, ___transport);
this.___currentMethod = method_call;
___manager.call(method_call);
}
public static class unfollowApplication_call extends org.apache.thrift.async.TAsyncMethodCall {
private UnfollowApplicationRequest request;
public unfollowApplication_call(UnfollowApplicationRequest request, org.apache.thrift.async.AsyncMethodCallback resultHandler, org.apache.thrift.async.TAsyncClient client, org.apache.thrift.protocol.TProtocolFactory protocolFactory, org.apache.thrift.transport.TNonblockingTransport transport) throws org.apache.thrift.TException {
super(client, protocolFactory, transport, resultHandler, false);
this.request = request;
}
public void write_args(org.apache.thrift.protocol.TProtocol prot) throws org.apache.thrift.TException {
prot.writeMessageBegin(new org.apache.thrift.protocol.TMessage("unfollowApplication", org.apache.thrift.protocol.TMessageType.CALL, 0));
unfollowApplication_args args = new unfollowApplication_args();
args.setRequest(request);
args.write(prot);
prot.writeMessageEnd();
}
public UnfollowApplicationResponse getResult() throws tech.aroma.thrift.exceptions.OperationFailedException, tech.aroma.thrift.exceptions.InvalidArgumentException, tech.aroma.thrift.exceptions.InvalidTokenException, tech.aroma.thrift.exceptions.ApplicationDoesNotExistException, tech.aroma.thrift.exceptions.UnauthorizedException, org.apache.thrift.TException {
if (getState() != org.apache.thrift.async.TAsyncMethodCall.State.RESPONSE_READ) {
throw new IllegalStateException("Method call not finished!");
}
org.apache.thrift.transport.TMemoryInputTransport memoryTransport = new org.apache.thrift.transport.TMemoryInputTransport(getFrameBuffer().array());
org.apache.thrift.protocol.TProtocol prot = client.getProtocolFactory().getProtocol(memoryTransport);
return (new Client(prot)).recv_unfollowApplication();
}
}
public void updateApplication(UpdateApplicationRequest request, org.apache.thrift.async.AsyncMethodCallback resultHandler) throws org.apache.thrift.TException {
checkReady();
updateApplication_call method_call = new updateApplication_call(request, resultHandler, this, ___protocolFactory, ___transport);
this.___currentMethod = method_call;
___manager.call(method_call);
}
public static class updateApplication_call extends org.apache.thrift.async.TAsyncMethodCall {
private UpdateApplicationRequest request;
public updateApplication_call(UpdateApplicationRequest request, org.apache.thrift.async.AsyncMethodCallback resultHandler, org.apache.thrift.async.TAsyncClient client, org.apache.thrift.protocol.TProtocolFactory protocolFactory, org.apache.thrift.transport.TNonblockingTransport transport) throws org.apache.thrift.TException {
super(client, protocolFactory, transport, resultHandler, false);
this.request = request;
}
public void write_args(org.apache.thrift.protocol.TProtocol prot) throws org.apache.thrift.TException {
prot.writeMessageBegin(new org.apache.thrift.protocol.TMessage("updateApplication", org.apache.thrift.protocol.TMessageType.CALL, 0));
updateApplication_args args = new updateApplication_args();
args.setRequest(request);
args.write(prot);
prot.writeMessageEnd();
}
public UpdateApplicationResponse getResult() throws tech.aroma.thrift.exceptions.OperationFailedException, tech.aroma.thrift.exceptions.InvalidArgumentException, tech.aroma.thrift.exceptions.InvalidTokenException, tech.aroma.thrift.exceptions.ApplicationDoesNotExistException, tech.aroma.thrift.exceptions.UnauthorizedException, org.apache.thrift.TException {
if (getState() != org.apache.thrift.async.TAsyncMethodCall.State.RESPONSE_READ) {
throw new IllegalStateException("Method call not finished!");
}
org.apache.thrift.transport.TMemoryInputTransport memoryTransport = new org.apache.thrift.transport.TMemoryInputTransport(getFrameBuffer().array());
org.apache.thrift.protocol.TProtocol prot = client.getProtocolFactory().getProtocol(memoryTransport);
return (new Client(prot)).recv_updateApplication();
}
}
public void updateReactions(UpdateReactionsRequest request, org.apache.thrift.async.AsyncMethodCallback resultHandler) throws org.apache.thrift.TException {
checkReady();
updateReactions_call method_call = new updateReactions_call(request, resultHandler, this, ___protocolFactory, ___transport);
this.___currentMethod = method_call;
___manager.call(method_call);
}
public static class updateReactions_call extends org.apache.thrift.async.TAsyncMethodCall {
private UpdateReactionsRequest request;
public updateReactions_call(UpdateReactionsRequest request, org.apache.thrift.async.AsyncMethodCallback resultHandler, org.apache.thrift.async.TAsyncClient client, org.apache.thrift.protocol.TProtocolFactory protocolFactory, org.apache.thrift.transport.TNonblockingTransport transport) throws org.apache.thrift.TException {
super(client, protocolFactory, transport, resultHandler, false);
this.request = request;
}
public void write_args(org.apache.thrift.protocol.TProtocol prot) throws org.apache.thrift.TException {
prot.writeMessageBegin(new org.apache.thrift.protocol.TMessage("updateReactions", org.apache.thrift.protocol.TMessageType.CALL, 0));
updateReactions_args args = new updateReactions_args();
args.setRequest(request);
args.write(prot);
prot.writeMessageEnd();
}
public UpdateReactionsResponse getResult() throws tech.aroma.thrift.exceptions.OperationFailedException, tech.aroma.thrift.exceptions.InvalidArgumentException, tech.aroma.thrift.exceptions.InvalidTokenException, tech.aroma.thrift.exceptions.ApplicationDoesNotExistException, tech.aroma.thrift.exceptions.UnauthorizedException, org.apache.thrift.TException {
if (getState() != org.apache.thrift.async.TAsyncMethodCall.State.RESPONSE_READ) {
throw new IllegalStateException("Method call not finished!");
}
org.apache.thrift.transport.TMemoryInputTransport memoryTransport = new org.apache.thrift.transport.TMemoryInputTransport(getFrameBuffer().array());
org.apache.thrift.protocol.TProtocol prot = client.getProtocolFactory().getProtocol(memoryTransport);
return (new Client(prot)).recv_updateReactions();
}
}
public void getActivity(GetActivityRequest request, org.apache.thrift.async.AsyncMethodCallback resultHandler) throws org.apache.thrift.TException {
checkReady();
getActivity_call method_call = new getActivity_call(request, resultHandler, this, ___protocolFactory, ___transport);
this.___currentMethod = method_call;
___manager.call(method_call);
}
public static class getActivity_call extends org.apache.thrift.async.TAsyncMethodCall {
private GetActivityRequest request;
public getActivity_call(GetActivityRequest request, org.apache.thrift.async.AsyncMethodCallback resultHandler, org.apache.thrift.async.TAsyncClient client, org.apache.thrift.protocol.TProtocolFactory protocolFactory, org.apache.thrift.transport.TNonblockingTransport transport) throws org.apache.thrift.TException {
super(client, protocolFactory, transport, resultHandler, false);
this.request = request;
}
public void write_args(org.apache.thrift.protocol.TProtocol prot) throws org.apache.thrift.TException {
prot.writeMessageBegin(new org.apache.thrift.protocol.TMessage("getActivity", org.apache.thrift.protocol.TMessageType.CALL, 0));
getActivity_args args = new getActivity_args();
args.setRequest(request);
args.write(prot);
prot.writeMessageEnd();
}
public GetActivityResponse getResult() throws tech.aroma.thrift.exceptions.OperationFailedException, tech.aroma.thrift.exceptions.InvalidArgumentException, tech.aroma.thrift.exceptions.InvalidTokenException, org.apache.thrift.TException {
if (getState() != org.apache.thrift.async.TAsyncMethodCall.State.RESPONSE_READ) {
throw new IllegalStateException("Method call not finished!");
}
org.apache.thrift.transport.TMemoryInputTransport memoryTransport = new org.apache.thrift.transport.TMemoryInputTransport(getFrameBuffer().array());
org.apache.thrift.protocol.TProtocol prot = client.getProtocolFactory().getProtocol(memoryTransport);
return (new Client(prot)).recv_getActivity();
}
}
public void getApplicationInfo(GetApplicationInfoRequest request, org.apache.thrift.async.AsyncMethodCallback resultHandler) throws org.apache.thrift.TException {
checkReady();
getApplicationInfo_call method_call = new getApplicationInfo_call(request, resultHandler, this, ___protocolFactory, ___transport);
this.___currentMethod = method_call;
___manager.call(method_call);
}
public static class getApplicationInfo_call extends org.apache.thrift.async.TAsyncMethodCall {
private GetApplicationInfoRequest request;
public getApplicationInfo_call(GetApplicationInfoRequest request, org.apache.thrift.async.AsyncMethodCallback resultHandler, org.apache.thrift.async.TAsyncClient client, org.apache.thrift.protocol.TProtocolFactory protocolFactory, org.apache.thrift.transport.TNonblockingTransport transport) throws org.apache.thrift.TException {
super(client, protocolFactory, transport, resultHandler, false);
this.request = request;
}
public void write_args(org.apache.thrift.protocol.TProtocol prot) throws org.apache.thrift.TException {
prot.writeMessageBegin(new org.apache.thrift.protocol.TMessage("getApplicationInfo", org.apache.thrift.protocol.TMessageType.CALL, 0));
getApplicationInfo_args args = new getApplicationInfo_args();
args.setRequest(request);
args.write(prot);
prot.writeMessageEnd();
}
public GetApplicationInfoResponse getResult() throws tech.aroma.thrift.exceptions.OperationFailedException, tech.aroma.thrift.exceptions.InvalidArgumentException, tech.aroma.thrift.exceptions.InvalidTokenException, tech.aroma.thrift.exceptions.ApplicationDoesNotExistException, tech.aroma.thrift.exceptions.UnauthorizedException, org.apache.thrift.TException {
if (getState() != org.apache.thrift.async.TAsyncMethodCall.State.RESPONSE_READ) {
throw new IllegalStateException("Method call not finished!");
}
org.apache.thrift.transport.TMemoryInputTransport memoryTransport = new org.apache.thrift.transport.TMemoryInputTransport(getFrameBuffer().array());
org.apache.thrift.protocol.TProtocol prot = client.getProtocolFactory().getProtocol(memoryTransport);
return (new Client(prot)).recv_getApplicationInfo();
}
}
public void getBuzz(GetBuzzRequest request, org.apache.thrift.async.AsyncMethodCallback resultHandler) throws org.apache.thrift.TException {
checkReady();
getBuzz_call method_call = new getBuzz_call(request, resultHandler, this, ___protocolFactory, ___transport);
this.___currentMethod = method_call;
___manager.call(method_call);
}
public static class getBuzz_call extends org.apache.thrift.async.TAsyncMethodCall {
private GetBuzzRequest request;
public getBuzz_call(GetBuzzRequest request, org.apache.thrift.async.AsyncMethodCallback resultHandler, org.apache.thrift.async.TAsyncClient client, org.apache.thrift.protocol.TProtocolFactory protocolFactory, org.apache.thrift.transport.TNonblockingTransport transport) throws org.apache.thrift.TException {
super(client, protocolFactory, transport, resultHandler, false);
this.request = request;
}
public void write_args(org.apache.thrift.protocol.TProtocol prot) throws org.apache.thrift.TException {
prot.writeMessageBegin(new org.apache.thrift.protocol.TMessage("getBuzz", org.apache.thrift.protocol.TMessageType.CALL, 0));
getBuzz_args args = new getBuzz_args();
args.setRequest(request);
args.write(prot);
prot.writeMessageEnd();
}
public GetBuzzResponse getResult() throws tech.aroma.thrift.exceptions.OperationFailedException, tech.aroma.thrift.exceptions.InvalidArgumentException, tech.aroma.thrift.exceptions.InvalidTokenException, tech.aroma.thrift.exceptions.ApplicationDoesNotExistException, tech.aroma.thrift.exceptions.UnauthorizedException, org.apache.thrift.TException {
if (getState() != org.apache.thrift.async.TAsyncMethodCall.State.RESPONSE_READ) {
throw new IllegalStateException("Method call not finished!");
}
org.apache.thrift.transport.TMemoryInputTransport memoryTransport = new org.apache.thrift.transport.TMemoryInputTransport(getFrameBuffer().array());
org.apache.thrift.protocol.TProtocol prot = client.getProtocolFactory().getProtocol(memoryTransport);
return (new Client(prot)).recv_getBuzz();
}
}
public void getDashboard(GetDashboardRequest request, org.apache.thrift.async.AsyncMethodCallback resultHandler) throws org.apache.thrift.TException {
checkReady();
getDashboard_call method_call = new getDashboard_call(request, resultHandler, this, ___protocolFactory, ___transport);
this.___currentMethod = method_call;
___manager.call(method_call);
}
public static class getDashboard_call extends org.apache.thrift.async.TAsyncMethodCall {
private GetDashboardRequest request;
public getDashboard_call(GetDashboardRequest request, org.apache.thrift.async.AsyncMethodCallback resultHandler, org.apache.thrift.async.TAsyncClient client, org.apache.thrift.protocol.TProtocolFactory protocolFactory, org.apache.thrift.transport.TNonblockingTransport transport) throws org.apache.thrift.TException {
super(client, protocolFactory, transport, resultHandler, false);
this.request = request;
}
public void write_args(org.apache.thrift.protocol.TProtocol prot) throws org.apache.thrift.TException {
prot.writeMessageBegin(new org.apache.thrift.protocol.TMessage("getDashboard", org.apache.thrift.protocol.TMessageType.CALL, 0));
getDashboard_args args = new getDashboard_args();
args.setRequest(request);
args.write(prot);
prot.writeMessageEnd();
}
public GetDashboardResponse getResult() throws tech.aroma.thrift.exceptions.OperationFailedException, tech.aroma.thrift.exceptions.InvalidArgumentException, tech.aroma.thrift.exceptions.InvalidTokenException, org.apache.thrift.TException {
if (getState() != org.apache.thrift.async.TAsyncMethodCall.State.RESPONSE_READ) {
throw new IllegalStateException("Method call not finished!");
}
org.apache.thrift.transport.TMemoryInputTransport memoryTransport = new org.apache.thrift.transport.TMemoryInputTransport(getFrameBuffer().array());
org.apache.thrift.protocol.TProtocol prot = client.getProtocolFactory().getProtocol(memoryTransport);
return (new Client(prot)).recv_getDashboard();
}
}
public void getApplicationMessages(GetApplicationMessagesRequest request, org.apache.thrift.async.AsyncMethodCallback resultHandler) throws org.apache.thrift.TException {
checkReady();
getApplicationMessages_call method_call = new getApplicationMessages_call(request, resultHandler, this, ___protocolFactory, ___transport);
this.___currentMethod = method_call;
___manager.call(method_call);
}
public static class getApplicationMessages_call extends org.apache.thrift.async.TAsyncMethodCall {
private GetApplicationMessagesRequest request;
public getApplicationMessages_call(GetApplicationMessagesRequest request, org.apache.thrift.async.AsyncMethodCallback resultHandler, org.apache.thrift.async.TAsyncClient client, org.apache.thrift.protocol.TProtocolFactory protocolFactory, org.apache.thrift.transport.TNonblockingTransport transport) throws org.apache.thrift.TException {
super(client, protocolFactory, transport, resultHandler, false);
this.request = request;
}
public void write_args(org.apache.thrift.protocol.TProtocol prot) throws org.apache.thrift.TException {
prot.writeMessageBegin(new org.apache.thrift.protocol.TMessage("getApplicationMessages", org.apache.thrift.protocol.TMessageType.CALL, 0));
getApplicationMessages_args args = new getApplicationMessages_args();
args.setRequest(request);
args.write(prot);
prot.writeMessageEnd();
}
public GetApplicationMessagesResponse getResult() throws tech.aroma.thrift.exceptions.OperationFailedException, tech.aroma.thrift.exceptions.InvalidArgumentException, tech.aroma.thrift.exceptions.InvalidTokenException, tech.aroma.thrift.exceptions.UnauthorizedException, tech.aroma.thrift.exceptions.ApplicationDoesNotExistException, org.apache.thrift.TException {
if (getState() != org.apache.thrift.async.TAsyncMethodCall.State.RESPONSE_READ) {
throw new IllegalStateException("Method call not finished!");
}
org.apache.thrift.transport.TMemoryInputTransport memoryTransport = new org.apache.thrift.transport.TMemoryInputTransport(getFrameBuffer().array());
org.apache.thrift.protocol.TProtocol prot = client.getProtocolFactory().getProtocol(memoryTransport);
return (new Client(prot)).recv_getApplicationMessages();
}
}
public void getInbox(GetInboxRequest request, org.apache.thrift.async.AsyncMethodCallback resultHandler) throws org.apache.thrift.TException {
checkReady();
getInbox_call method_call = new getInbox_call(request, resultHandler, this, ___protocolFactory, ___transport);
this.___currentMethod = method_call;
___manager.call(method_call);
}
public static class getInbox_call extends org.apache.thrift.async.TAsyncMethodCall {
private GetInboxRequest request;
public getInbox_call(GetInboxRequest request, org.apache.thrift.async.AsyncMethodCallback resultHandler, org.apache.thrift.async.TAsyncClient client, org.apache.thrift.protocol.TProtocolFactory protocolFactory, org.apache.thrift.transport.TNonblockingTransport transport) throws org.apache.thrift.TException {
super(client, protocolFactory, transport, resultHandler, false);
this.request = request;
}
public void write_args(org.apache.thrift.protocol.TProtocol prot) throws org.apache.thrift.TException {
prot.writeMessageBegin(new org.apache.thrift.protocol.TMessage("getInbox", org.apache.thrift.protocol.TMessageType.CALL, 0));
getInbox_args args = new getInbox_args();
args.setRequest(request);
args.write(prot);
prot.writeMessageEnd();
}
public GetInboxResponse getResult() throws tech.aroma.thrift.exceptions.OperationFailedException, tech.aroma.thrift.exceptions.InvalidArgumentException, tech.aroma.thrift.exceptions.InvalidTokenException, org.apache.thrift.TException {
if (getState() != org.apache.thrift.async.TAsyncMethodCall.State.RESPONSE_READ) {
throw new IllegalStateException("Method call not finished!");
}
org.apache.thrift.transport.TMemoryInputTransport memoryTransport = new org.apache.thrift.transport.TMemoryInputTransport(getFrameBuffer().array());
org.apache.thrift.protocol.TProtocol prot = client.getProtocolFactory().getProtocol(memoryTransport);
return (new Client(prot)).recv_getInbox();
}
}
public void getFullMessage(GetFullMessageRequest request, org.apache.thrift.async.AsyncMethodCallback resultHandler) throws org.apache.thrift.TException {
checkReady();
getFullMessage_call method_call = new getFullMessage_call(request, resultHandler, this, ___protocolFactory, ___transport);
this.___currentMethod = method_call;
___manager.call(method_call);
}
public static class getFullMessage_call extends org.apache.thrift.async.TAsyncMethodCall {
private GetFullMessageRequest request;
public getFullMessage_call(GetFullMessageRequest request, org.apache.thrift.async.AsyncMethodCallback resultHandler, org.apache.thrift.async.TAsyncClient client, org.apache.thrift.protocol.TProtocolFactory protocolFactory, org.apache.thrift.transport.TNonblockingTransport transport) throws org.apache.thrift.TException {
super(client, protocolFactory, transport, resultHandler, false);
this.request = request;
}
public void write_args(org.apache.thrift.protocol.TProtocol prot) throws org.apache.thrift.TException {
prot.writeMessageBegin(new org.apache.thrift.protocol.TMessage("getFullMessage", org.apache.thrift.protocol.TMessageType.CALL, 0));
getFullMessage_args args = new getFullMessage_args();
args.setRequest(request);
args.write(prot);
prot.writeMessageEnd();
}
public GetFullMessageResponse getResult() throws tech.aroma.thrift.exceptions.OperationFailedException, tech.aroma.thrift.exceptions.InvalidArgumentException, tech.aroma.thrift.exceptions.InvalidTokenException, org.apache.thrift.TException {
if (getState() != org.apache.thrift.async.TAsyncMethodCall.State.RESPONSE_READ) {
throw new IllegalStateException("Method call not finished!");
}
org.apache.thrift.transport.TMemoryInputTransport memoryTransport = new org.apache.thrift.transport.TMemoryInputTransport(getFrameBuffer().array());
org.apache.thrift.protocol.TProtocol prot = client.getProtocolFactory().getProtocol(memoryTransport);
return (new Client(prot)).recv_getFullMessage();
}
}
public void getMedia(GetMediaRequest request, org.apache.thrift.async.AsyncMethodCallback resultHandler) throws org.apache.thrift.TException {
checkReady();
getMedia_call method_call = new getMedia_call(request, resultHandler, this, ___protocolFactory, ___transport);
this.___currentMethod = method_call;
___manager.call(method_call);
}
public static class getMedia_call extends org.apache.thrift.async.TAsyncMethodCall {
private GetMediaRequest request;
public getMedia_call(GetMediaRequest request, org.apache.thrift.async.AsyncMethodCallback resultHandler, org.apache.thrift.async.TAsyncClient client, org.apache.thrift.protocol.TProtocolFactory protocolFactory, org.apache.thrift.transport.TNonblockingTransport transport) throws org.apache.thrift.TException {
super(client, protocolFactory, transport, resultHandler, false);
this.request = request;
}
public void write_args(org.apache.thrift.protocol.TProtocol prot) throws org.apache.thrift.TException {
prot.writeMessageBegin(new org.apache.thrift.protocol.TMessage("getMedia", org.apache.thrift.protocol.TMessageType.CALL, 0));
getMedia_args args = new getMedia_args();
args.setRequest(request);
args.write(prot);
prot.writeMessageEnd();
}
public GetMediaResponse getResult() throws tech.aroma.thrift.exceptions.OperationFailedException, tech.aroma.thrift.exceptions.InvalidArgumentException, tech.aroma.thrift.exceptions.InvalidTokenException, tech.aroma.thrift.exceptions.DoesNotExistException, tech.aroma.thrift.exceptions.UnauthorizedException, org.apache.thrift.TException {
if (getState() != org.apache.thrift.async.TAsyncMethodCall.State.RESPONSE_READ) {
throw new IllegalStateException("Method call not finished!");
}
org.apache.thrift.transport.TMemoryInputTransport memoryTransport = new org.apache.thrift.transport.TMemoryInputTransport(getFrameBuffer().array());
org.apache.thrift.protocol.TProtocol prot = client.getProtocolFactory().getProtocol(memoryTransport);
return (new Client(prot)).recv_getMedia();
}
}
public void getApplicationsOwnedBy(GetApplicationsOwnedByRequest request, org.apache.thrift.async.AsyncMethodCallback resultHandler) throws org.apache.thrift.TException {
checkReady();
getApplicationsOwnedBy_call method_call = new getApplicationsOwnedBy_call(request, resultHandler, this, ___protocolFactory, ___transport);
this.___currentMethod = method_call;
___manager.call(method_call);
}
public static class getApplicationsOwnedBy_call extends org.apache.thrift.async.TAsyncMethodCall {
private GetApplicationsOwnedByRequest request;
public getApplicationsOwnedBy_call(GetApplicationsOwnedByRequest request, org.apache.thrift.async.AsyncMethodCallback resultHandler, org.apache.thrift.async.TAsyncClient client, org.apache.thrift.protocol.TProtocolFactory protocolFactory, org.apache.thrift.transport.TNonblockingTransport transport) throws org.apache.thrift.TException {
super(client, protocolFactory, transport, resultHandler, false);
this.request = request;
}
public void write_args(org.apache.thrift.protocol.TProtocol prot) throws org.apache.thrift.TException {
prot.writeMessageBegin(new org.apache.thrift.protocol.TMessage("getApplicationsOwnedBy", org.apache.thrift.protocol.TMessageType.CALL, 0));
getApplicationsOwnedBy_args args = new getApplicationsOwnedBy_args();
args.setRequest(request);
args.write(prot);
prot.writeMessageEnd();
}
public GetApplicationsOwnedByResponse getResult() throws tech.aroma.thrift.exceptions.OperationFailedException, tech.aroma.thrift.exceptions.InvalidArgumentException, tech.aroma.thrift.exceptions.InvalidTokenException, org.apache.thrift.TException {
if (getState() != org.apache.thrift.async.TAsyncMethodCall.State.RESPONSE_READ) {
throw new IllegalStateException("Method call not finished!");
}
org.apache.thrift.transport.TMemoryInputTransport memoryTransport = new org.apache.thrift.transport.TMemoryInputTransport(getFrameBuffer().array());
org.apache.thrift.protocol.TProtocol prot = client.getProtocolFactory().getProtocol(memoryTransport);
return (new Client(prot)).recv_getApplicationsOwnedBy();
}
}
public void getApplicationsFollowedBy(GetApplicationsFollowedByRequest request, org.apache.thrift.async.AsyncMethodCallback resultHandler) throws org.apache.thrift.TException {
checkReady();
getApplicationsFollowedBy_call method_call = new getApplicationsFollowedBy_call(request, resultHandler, this, ___protocolFactory, ___transport);
this.___currentMethod = method_call;
___manager.call(method_call);
}
public static class getApplicationsFollowedBy_call extends org.apache.thrift.async.TAsyncMethodCall {
private GetApplicationsFollowedByRequest request;
public getApplicationsFollowedBy_call(GetApplicationsFollowedByRequest request, org.apache.thrift.async.AsyncMethodCallback resultHandler, org.apache.thrift.async.TAsyncClient client, org.apache.thrift.protocol.TProtocolFactory protocolFactory, org.apache.thrift.transport.TNonblockingTransport transport) throws org.apache.thrift.TException {
super(client, protocolFactory, transport, resultHandler, false);
this.request = request;
}
public void write_args(org.apache.thrift.protocol.TProtocol prot) throws org.apache.thrift.TException {
prot.writeMessageBegin(new org.apache.thrift.protocol.TMessage("getApplicationsFollowedBy", org.apache.thrift.protocol.TMessageType.CALL, 0));
getApplicationsFollowedBy_args args = new getApplicationsFollowedBy_args();
args.setRequest(request);
args.write(prot);
prot.writeMessageEnd();
}
public GetApplicationsFollowedByResponse getResult() throws tech.aroma.thrift.exceptions.OperationFailedException, tech.aroma.thrift.exceptions.InvalidArgumentException, tech.aroma.thrift.exceptions.InvalidTokenException, org.apache.thrift.TException {
if (getState() != org.apache.thrift.async.TAsyncMethodCall.State.RESPONSE_READ) {
throw new IllegalStateException("Method call not finished!");
}
org.apache.thrift.transport.TMemoryInputTransport memoryTransport = new org.apache.thrift.transport.TMemoryInputTransport(getFrameBuffer().array());
org.apache.thrift.protocol.TProtocol prot = client.getProtocolFactory().getProtocol(memoryTransport);
return (new Client(prot)).recv_getApplicationsFollowedBy();
}
}
public void getMySavedChannels(GetMySavedChannelsRequest request, org.apache.thrift.async.AsyncMethodCallback resultHandler) throws org.apache.thrift.TException {
checkReady();
getMySavedChannels_call method_call = new getMySavedChannels_call(request, resultHandler, this, ___protocolFactory, ___transport);
this.___currentMethod = method_call;
___manager.call(method_call);
}
public static class getMySavedChannels_call extends org.apache.thrift.async.TAsyncMethodCall {
private GetMySavedChannelsRequest request;
public getMySavedChannels_call(GetMySavedChannelsRequest request, org.apache.thrift.async.AsyncMethodCallback resultHandler, org.apache.thrift.async.TAsyncClient client, org.apache.thrift.protocol.TProtocolFactory protocolFactory, org.apache.thrift.transport.TNonblockingTransport transport) throws org.apache.thrift.TException {
super(client, protocolFactory, transport, resultHandler, false);
this.request = request;
}
public void write_args(org.apache.thrift.protocol.TProtocol prot) throws org.apache.thrift.TException {
prot.writeMessageBegin(new org.apache.thrift.protocol.TMessage("getMySavedChannels", org.apache.thrift.protocol.TMessageType.CALL, 0));
getMySavedChannels_args args = new getMySavedChannels_args();
args.setRequest(request);
args.write(prot);
prot.writeMessageEnd();
}
public GetMySavedChannelsResponse getResult() throws tech.aroma.thrift.exceptions.OperationFailedException, tech.aroma.thrift.exceptions.InvalidArgumentException, tech.aroma.thrift.exceptions.InvalidTokenException, org.apache.thrift.TException {
if (getState() != org.apache.thrift.async.TAsyncMethodCall.State.RESPONSE_READ) {
throw new IllegalStateException("Method call not finished!");
}
org.apache.thrift.transport.TMemoryInputTransport memoryTransport = new org.apache.thrift.transport.TMemoryInputTransport(getFrameBuffer().array());
org.apache.thrift.protocol.TProtocol prot = client.getProtocolFactory().getProtocol(memoryTransport);
return (new Client(prot)).recv_getMySavedChannels();
}
}
public void getReactions(GetReactionsRequest request, org.apache.thrift.async.AsyncMethodCallback resultHandler) throws org.apache.thrift.TException {
checkReady();
getReactions_call method_call = new getReactions_call(request, resultHandler, this, ___protocolFactory, ___transport);
this.___currentMethod = method_call;
___manager.call(method_call);
}
public static class getReactions_call extends org.apache.thrift.async.TAsyncMethodCall {
private GetReactionsRequest request;
public getReactions_call(GetReactionsRequest request, org.apache.thrift.async.AsyncMethodCallback resultHandler, org.apache.thrift.async.TAsyncClient client, org.apache.thrift.protocol.TProtocolFactory protocolFactory, org.apache.thrift.transport.TNonblockingTransport transport) throws org.apache.thrift.TException {
super(client, protocolFactory, transport, resultHandler, false);
this.request = request;
}
public void write_args(org.apache.thrift.protocol.TProtocol prot) throws org.apache.thrift.TException {
prot.writeMessageBegin(new org.apache.thrift.protocol.TMessage("getReactions", org.apache.thrift.protocol.TMessageType.CALL, 0));
getReactions_args args = new getReactions_args();
args.setRequest(request);
args.write(prot);
prot.writeMessageEnd();
}
public GetReactionsResponse getResult() throws tech.aroma.thrift.exceptions.OperationFailedException, tech.aroma.thrift.exceptions.InvalidArgumentException, tech.aroma.thrift.exceptions.InvalidTokenException, tech.aroma.thrift.exceptions.ApplicationDoesNotExistException, tech.aroma.thrift.exceptions.UnauthorizedException, org.apache.thrift.TException {
if (getState() != org.apache.thrift.async.TAsyncMethodCall.State.RESPONSE_READ) {
throw new IllegalStateException("Method call not finished!");
}
org.apache.thrift.transport.TMemoryInputTransport memoryTransport = new org.apache.thrift.transport.TMemoryInputTransport(getFrameBuffer().array());
org.apache.thrift.protocol.TProtocol prot = client.getProtocolFactory().getProtocol(memoryTransport);
return (new Client(prot)).recv_getReactions();
}
}
public void getUserInfo(GetUserInfoRequest request, org.apache.thrift.async.AsyncMethodCallback resultHandler) throws org.apache.thrift.TException {
checkReady();
getUserInfo_call method_call = new getUserInfo_call(request, resultHandler, this, ___protocolFactory, ___transport);
this.___currentMethod = method_call;
___manager.call(method_call);
}
public static class getUserInfo_call extends org.apache.thrift.async.TAsyncMethodCall {
private GetUserInfoRequest request;
public getUserInfo_call(GetUserInfoRequest request, org.apache.thrift.async.AsyncMethodCallback resultHandler, org.apache.thrift.async.TAsyncClient client, org.apache.thrift.protocol.TProtocolFactory protocolFactory, org.apache.thrift.transport.TNonblockingTransport transport) throws org.apache.thrift.TException {
super(client, protocolFactory, transport, resultHandler, false);
this.request = request;
}
public void write_args(org.apache.thrift.protocol.TProtocol prot) throws org.apache.thrift.TException {
prot.writeMessageBegin(new org.apache.thrift.protocol.TMessage("getUserInfo", org.apache.thrift.protocol.TMessageType.CALL, 0));
getUserInfo_args args = new getUserInfo_args();
args.setRequest(request);
args.write(prot);
prot.writeMessageEnd();
}
public GetUserInfoResponse getResult() throws tech.aroma.thrift.exceptions.OperationFailedException, tech.aroma.thrift.exceptions.InvalidArgumentException, tech.aroma.thrift.exceptions.InvalidTokenException, tech.aroma.thrift.exceptions.UnauthorizedException, tech.aroma.thrift.exceptions.UserDoesNotExistException, org.apache.thrift.TException {
if (getState() != org.apache.thrift.async.TAsyncMethodCall.State.RESPONSE_READ) {
throw new IllegalStateException("Method call not finished!");
}
org.apache.thrift.transport.TMemoryInputTransport memoryTransport = new org.apache.thrift.transport.TMemoryInputTransport(getFrameBuffer().array());
org.apache.thrift.protocol.TProtocol prot = client.getProtocolFactory().getProtocol(memoryTransport);
return (new Client(prot)).recv_getUserInfo();
}
}
public void searchForApplications(SearchForApplicationsRequest request, org.apache.thrift.async.AsyncMethodCallback resultHandler) throws org.apache.thrift.TException {
checkReady();
searchForApplications_call method_call = new searchForApplications_call(request, resultHandler, this, ___protocolFactory, ___transport);
this.___currentMethod = method_call;
___manager.call(method_call);
}
public static class searchForApplications_call extends org.apache.thrift.async.TAsyncMethodCall {
private SearchForApplicationsRequest request;
public searchForApplications_call(SearchForApplicationsRequest request, org.apache.thrift.async.AsyncMethodCallback resultHandler, org.apache.thrift.async.TAsyncClient client, org.apache.thrift.protocol.TProtocolFactory protocolFactory, org.apache.thrift.transport.TNonblockingTransport transport) throws org.apache.thrift.TException {
super(client, protocolFactory, transport, resultHandler, false);
this.request = request;
}
public void write_args(org.apache.thrift.protocol.TProtocol prot) throws org.apache.thrift.TException {
prot.writeMessageBegin(new org.apache.thrift.protocol.TMessage("searchForApplications", org.apache.thrift.protocol.TMessageType.CALL, 0));
searchForApplications_args args = new searchForApplications_args();
args.setRequest(request);
args.write(prot);
prot.writeMessageEnd();
}
public SearchForApplicationsResponse getResult() throws tech.aroma.thrift.exceptions.OperationFailedException, tech.aroma.thrift.exceptions.InvalidArgumentException, tech.aroma.thrift.exceptions.InvalidTokenException, tech.aroma.thrift.exceptions.UnauthorizedException, org.apache.thrift.TException {
if (getState() != org.apache.thrift.async.TAsyncMethodCall.State.RESPONSE_READ) {
throw new IllegalStateException("Method call not finished!");
}
org.apache.thrift.transport.TMemoryInputTransport memoryTransport = new org.apache.thrift.transport.TMemoryInputTransport(getFrameBuffer().array());
org.apache.thrift.protocol.TProtocol prot = client.getProtocolFactory().getProtocol(memoryTransport);
return (new Client(prot)).recv_searchForApplications();
}
}
}
public static class Processor extends org.apache.thrift.TBaseProcessor implements org.apache.thrift.TProcessor {
private static final Logger LOGGER = LoggerFactory.getLogger(Processor.class.getName());
public Processor(I iface) {
super(iface, getProcessMap(new HashMap>()));
}
protected Processor(I iface, Map> processMap) {
super(iface, getProcessMap(processMap));
}
private static Map> getProcessMap(Map> processMap) {
processMap.put("getApiVersion", new getApiVersion());
processMap.put("deleteApplication", new deleteApplication());
processMap.put("deleteMessage", new deleteMessage());
processMap.put("dismissMessage", new dismissMessage());
processMap.put("provisionApplication", new provisionApplication());
processMap.put("regenerateToken", new regenerateToken());
processMap.put("registerHealthCheck", new registerHealthCheck());
processMap.put("removeSavedChannel", new removeSavedChannel());
processMap.put("renewApplicationToken", new renewApplicationToken());
processMap.put("saveChannel", new saveChannel());
processMap.put("signIn", new signIn());
processMap.put("signUp", new signUp());
processMap.put("snoozeChannel", new snoozeChannel());
processMap.put("followApplication", new followApplication());
processMap.put("unfollowApplication", new unfollowApplication());
processMap.put("updateApplication", new updateApplication());
processMap.put("updateReactions", new updateReactions());
processMap.put("getActivity", new getActivity());
processMap.put("getApplicationInfo", new getApplicationInfo());
processMap.put("getBuzz", new getBuzz());
processMap.put("getDashboard", new getDashboard());
processMap.put("getApplicationMessages", new getApplicationMessages());
processMap.put("getInbox", new getInbox());
processMap.put("getFullMessage", new getFullMessage());
processMap.put("getMedia", new getMedia());
processMap.put("getApplicationsOwnedBy", new getApplicationsOwnedBy());
processMap.put("getApplicationsFollowedBy", new getApplicationsFollowedBy());
processMap.put("getMySavedChannels", new getMySavedChannels());
processMap.put("getReactions", new getReactions());
processMap.put("getUserInfo", new getUserInfo());
processMap.put("searchForApplications", new searchForApplications());
return processMap;
}
public static class getApiVersion extends org.apache.thrift.ProcessFunction {
public getApiVersion() {
super("getApiVersion");
}
public getApiVersion_args getEmptyArgsInstance() {
return new getApiVersion_args();
}
protected boolean isOneway() {
return false;
}
public getApiVersion_result getResult(I iface, getApiVersion_args args) throws org.apache.thrift.TException {
getApiVersion_result result = new getApiVersion_result();
result.success = iface.getApiVersion();
result.setSuccessIsSet(true);
return result;
}
}
public static class deleteApplication extends org.apache.thrift.ProcessFunction {
public deleteApplication() {
super("deleteApplication");
}
public deleteApplication_args getEmptyArgsInstance() {
return new deleteApplication_args();
}
protected boolean isOneway() {
return false;
}
public deleteApplication_result getResult(I iface, deleteApplication_args args) throws org.apache.thrift.TException {
deleteApplication_result result = new deleteApplication_result();
try {
result.success = iface.deleteApplication(args.request);
} catch (tech.aroma.thrift.exceptions.OperationFailedException ex1) {
result.ex1 = ex1;
} catch (tech.aroma.thrift.exceptions.InvalidArgumentException ex2) {
result.ex2 = ex2;
} catch (tech.aroma.thrift.exceptions.InvalidTokenException ex3) {
result.ex3 = ex3;
} catch (tech.aroma.thrift.exceptions.ApplicationDoesNotExistException ex4) {
result.ex4 = ex4;
} catch (tech.aroma.thrift.exceptions.UnauthorizedException ex5) {
result.ex5 = ex5;
}
return result;
}
}
public static class deleteMessage extends org.apache.thrift.ProcessFunction {
public deleteMessage() {
super("deleteMessage");
}
public deleteMessage_args getEmptyArgsInstance() {
return new deleteMessage_args();
}
protected boolean isOneway() {
return false;
}
public deleteMessage_result getResult(I iface, deleteMessage_args args) throws org.apache.thrift.TException {
deleteMessage_result result = new deleteMessage_result();
try {
result.success = iface.deleteMessage(args.request);
} catch (tech.aroma.thrift.exceptions.OperationFailedException ex1) {
result.ex1 = ex1;
} catch (tech.aroma.thrift.exceptions.InvalidArgumentException ex2) {
result.ex2 = ex2;
} catch (tech.aroma.thrift.exceptions.InvalidTokenException ex3) {
result.ex3 = ex3;
} catch (tech.aroma.thrift.exceptions.MessageDoesNotExistException ex4) {
result.ex4 = ex4;
} catch (tech.aroma.thrift.exceptions.UnauthorizedException ex5) {
result.ex5 = ex5;
}
return result;
}
}
public static class dismissMessage extends org.apache.thrift.ProcessFunction {
public dismissMessage() {
super("dismissMessage");
}
public dismissMessage_args getEmptyArgsInstance() {
return new dismissMessage_args();
}
protected boolean isOneway() {
return false;
}
public dismissMessage_result getResult(I iface, dismissMessage_args args) throws org.apache.thrift.TException {
dismissMessage_result result = new dismissMessage_result();
try {
result.success = iface.dismissMessage(args.request);
} catch (tech.aroma.thrift.exceptions.OperationFailedException ex1) {
result.ex1 = ex1;
} catch (tech.aroma.thrift.exceptions.InvalidArgumentException ex2) {
result.ex2 = ex2;
} catch (tech.aroma.thrift.exceptions.InvalidTokenException ex3) {
result.ex3 = ex3;
} catch (tech.aroma.thrift.exceptions.MessageDoesNotExistException ex4) {
result.ex4 = ex4;
} catch (tech.aroma.thrift.exceptions.UnauthorizedException ex5) {
result.ex5 = ex5;
}
return result;
}
}
public static class provisionApplication extends org.apache.thrift.ProcessFunction {
public provisionApplication() {
super("provisionApplication");
}
public provisionApplication_args getEmptyArgsInstance() {
return new provisionApplication_args();
}
protected boolean isOneway() {
return false;
}
public provisionApplication_result getResult(I iface, provisionApplication_args args) throws org.apache.thrift.TException {
provisionApplication_result result = new provisionApplication_result();
try {
result.success = iface.provisionApplication(args.request);
} catch (tech.aroma.thrift.exceptions.OperationFailedException ex1) {
result.ex1 = ex1;
} catch (tech.aroma.thrift.exceptions.InvalidArgumentException ex2) {
result.ex2 = ex2;
} catch (tech.aroma.thrift.exceptions.InvalidTokenException ex3) {
result.ex3 = ex3;
} catch (tech.aroma.thrift.exceptions.ApplicationDoesNotExistException ex4) {
result.ex4 = ex4;
} catch (tech.aroma.thrift.exceptions.UnauthorizedException ex5) {
result.ex5 = ex5;
}
return result;
}
}
public static class regenerateToken extends org.apache.thrift.ProcessFunction {
public regenerateToken() {
super("regenerateToken");
}
public regenerateToken_args getEmptyArgsInstance() {
return new regenerateToken_args();
}
protected boolean isOneway() {
return false;
}
public regenerateToken_result getResult(I iface, regenerateToken_args args) throws org.apache.thrift.TException {
regenerateToken_result result = new regenerateToken_result();
try {
result.success = iface.regenerateToken(args.request);
} catch (tech.aroma.thrift.exceptions.OperationFailedException ex1) {
result.ex1 = ex1;
} catch (tech.aroma.thrift.exceptions.InvalidArgumentException ex2) {
result.ex2 = ex2;
} catch (tech.aroma.thrift.exceptions.InvalidTokenException ex3) {
result.ex3 = ex3;
} catch (tech.aroma.thrift.exceptions.ApplicationDoesNotExistException ex4) {
result.ex4 = ex4;
} catch (tech.aroma.thrift.exceptions.UnauthorizedException ex5) {
result.ex5 = ex5;
}
return result;
}
}
public static class registerHealthCheck extends org.apache.thrift.ProcessFunction {
public registerHealthCheck() {
super("registerHealthCheck");
}
public registerHealthCheck_args getEmptyArgsInstance() {
return new registerHealthCheck_args();
}
protected boolean isOneway() {
return false;
}
public registerHealthCheck_result getResult(I iface, registerHealthCheck_args args) throws org.apache.thrift.TException {
registerHealthCheck_result result = new registerHealthCheck_result();
try {
result.success = iface.registerHealthCheck(args.request);
} catch (tech.aroma.thrift.exceptions.OperationFailedException ex1) {
result.ex1 = ex1;
} catch (tech.aroma.thrift.exceptions.InvalidArgumentException ex2) {
result.ex2 = ex2;
} catch (tech.aroma.thrift.exceptions.InvalidTokenException ex3) {
result.ex3 = ex3;
} catch (tech.aroma.thrift.exceptions.ApplicationDoesNotExistException ex4) {
result.ex4 = ex4;
} catch (tech.aroma.thrift.exceptions.UnauthorizedException ex5) {
result.ex5 = ex5;
}
return result;
}
}
public static class removeSavedChannel extends org.apache.thrift.ProcessFunction {
public removeSavedChannel() {
super("removeSavedChannel");
}
public removeSavedChannel_args getEmptyArgsInstance() {
return new removeSavedChannel_args();
}
protected boolean isOneway() {
return false;
}
public removeSavedChannel_result getResult(I iface, removeSavedChannel_args args) throws org.apache.thrift.TException {
removeSavedChannel_result result = new removeSavedChannel_result();
try {
result.success = iface.removeSavedChannel(args.request);
} catch (tech.aroma.thrift.exceptions.OperationFailedException ex1) {
result.ex1 = ex1;
} catch (tech.aroma.thrift.exceptions.InvalidArgumentException ex2) {
result.ex2 = ex2;
} catch (tech.aroma.thrift.exceptions.InvalidTokenException ex3) {
result.ex3 = ex3;
} catch (tech.aroma.thrift.exceptions.UnauthorizedException ex4) {
result.ex4 = ex4;
} catch (tech.aroma.thrift.exceptions.ChannelDoesNotExistException ex5) {
result.ex5 = ex5;
}
return result;
}
}
public static class renewApplicationToken extends org.apache.thrift.ProcessFunction {
public renewApplicationToken() {
super("renewApplicationToken");
}
public renewApplicationToken_args getEmptyArgsInstance() {
return new renewApplicationToken_args();
}
protected boolean isOneway() {
return false;
}
public renewApplicationToken_result getResult(I iface, renewApplicationToken_args args) throws org.apache.thrift.TException {
renewApplicationToken_result result = new renewApplicationToken_result();
try {
result.success = iface.renewApplicationToken(args.request);
} catch (tech.aroma.thrift.exceptions.OperationFailedException ex1) {
result.ex1 = ex1;
} catch (tech.aroma.thrift.exceptions.InvalidArgumentException ex2) {
result.ex2 = ex2;
} catch (tech.aroma.thrift.exceptions.InvalidTokenException ex3) {
result.ex3 = ex3;
} catch (tech.aroma.thrift.exceptions.ApplicationDoesNotExistException ex4) {
result.ex4 = ex4;
} catch (tech.aroma.thrift.exceptions.UnauthorizedException ex5) {
result.ex5 = ex5;
}
return result;
}
}
public static class saveChannel extends org.apache.thrift.ProcessFunction {
public saveChannel() {
super("saveChannel");
}
public saveChannel_args getEmptyArgsInstance() {
return new saveChannel_args();
}
protected boolean isOneway() {
return false;
}
public saveChannel_result getResult(I iface, saveChannel_args args) throws org.apache.thrift.TException {
saveChannel_result result = new saveChannel_result();
try {
result.success = iface.saveChannel(args.request);
} catch (tech.aroma.thrift.exceptions.OperationFailedException ex1) {
result.ex1 = ex1;
} catch (tech.aroma.thrift.exceptions.InvalidArgumentException ex2) {
result.ex2 = ex2;
} catch (tech.aroma.thrift.exceptions.InvalidTokenException ex3) {
result.ex3 = ex3;
} catch (tech.aroma.thrift.exceptions.UnauthorizedException ex4) {
result.ex4 = ex4;
}
return result;
}
}
public static class signIn extends org.apache.thrift.ProcessFunction {
public signIn() {
super("signIn");
}
public signIn_args getEmptyArgsInstance() {
return new signIn_args();
}
protected boolean isOneway() {
return false;
}
public signIn_result getResult(I iface, signIn_args args) throws org.apache.thrift.TException {
signIn_result result = new signIn_result();
try {
result.success = iface.signIn(args.request);
} catch (tech.aroma.thrift.exceptions.OperationFailedException ex1) {
result.ex1 = ex1;
} catch (tech.aroma.thrift.exceptions.InvalidArgumentException ex2) {
result.ex2 = ex2;
} catch (tech.aroma.thrift.exceptions.InvalidCredentialsException ex3) {
result.ex3 = ex3;
} catch (tech.aroma.thrift.exceptions.UserDoesNotExistException ex4) {
result.ex4 = ex4;
}
return result;
}
}
public static class signUp extends org.apache.thrift.ProcessFunction {
public signUp() {
super("signUp");
}
public signUp_args getEmptyArgsInstance() {
return new signUp_args();
}
protected boolean isOneway() {
return false;
}
public signUp_result getResult(I iface, signUp_args args) throws org.apache.thrift.TException {
signUp_result result = new signUp_result();
try {
result.success = iface.signUp(args.request);
} catch (tech.aroma.thrift.exceptions.OperationFailedException ex1) {
result.ex1 = ex1;
} catch (tech.aroma.thrift.exceptions.InvalidArgumentException ex2) {
result.ex2 = ex2;
} catch (tech.aroma.thrift.exceptions.InvalidCredentialsException ex3) {
result.ex3 = ex3;
} catch (tech.aroma.thrift.exceptions.AccountAlreadyExistsException ex4) {
result.ex4 = ex4;
}
return result;
}
}
public static class snoozeChannel extends org.apache.thrift.ProcessFunction {
public snoozeChannel() {
super("snoozeChannel");
}
public snoozeChannel_args getEmptyArgsInstance() {
return new snoozeChannel_args();
}
protected boolean isOneway() {
return false;
}
public snoozeChannel_result getResult(I iface, snoozeChannel_args args) throws org.apache.thrift.TException {
snoozeChannel_result result = new snoozeChannel_result();
try {
result.success = iface.snoozeChannel(args.request);
} catch (tech.aroma.thrift.exceptions.OperationFailedException ex1) {
result.ex1 = ex1;
} catch (tech.aroma.thrift.exceptions.InvalidArgumentException ex2) {
result.ex2 = ex2;
} catch (tech.aroma.thrift.exceptions.InvalidTokenException ex3) {
result.ex3 = ex3;
} catch (tech.aroma.thrift.exceptions.UnauthorizedException ex4) {
result.ex4 = ex4;
} catch (tech.aroma.thrift.exceptions.ChannelDoesNotExistException ex5) {
result.ex5 = ex5;
}
return result;
}
}
public static class followApplication extends org.apache.thrift.ProcessFunction {
public followApplication() {
super("followApplication");
}
public followApplication_args getEmptyArgsInstance() {
return new followApplication_args();
}
protected boolean isOneway() {
return false;
}
public followApplication_result getResult(I iface, followApplication_args args) throws org.apache.thrift.TException {
followApplication_result result = new followApplication_result();
try {
result.success = iface.followApplication(args.request);
} catch (tech.aroma.thrift.exceptions.OperationFailedException ex1) {
result.ex1 = ex1;
} catch (tech.aroma.thrift.exceptions.InvalidArgumentException ex2) {
result.ex2 = ex2;
} catch (tech.aroma.thrift.exceptions.InvalidTokenException ex3) {
result.ex3 = ex3;
} catch (tech.aroma.thrift.exceptions.ApplicationDoesNotExistException ex4) {
result.ex4 = ex4;
} catch (tech.aroma.thrift.exceptions.UnauthorizedException ex5) {
result.ex5 = ex5;
}
return result;
}
}
public static class unfollowApplication extends org.apache.thrift.ProcessFunction {
public unfollowApplication() {
super("unfollowApplication");
}
public unfollowApplication_args getEmptyArgsInstance() {
return new unfollowApplication_args();
}
protected boolean isOneway() {
return false;
}
public unfollowApplication_result getResult(I iface, unfollowApplication_args args) throws org.apache.thrift.TException {
unfollowApplication_result result = new unfollowApplication_result();
try {
result.success = iface.unfollowApplication(args.request);
} catch (tech.aroma.thrift.exceptions.OperationFailedException ex1) {
result.ex1 = ex1;
} catch (tech.aroma.thrift.exceptions.InvalidArgumentException ex2) {
result.ex2 = ex2;
} catch (tech.aroma.thrift.exceptions.InvalidTokenException ex3) {
result.ex3 = ex3;
} catch (tech.aroma.thrift.exceptions.ApplicationDoesNotExistException ex4) {
result.ex4 = ex4;
} catch (tech.aroma.thrift.exceptions.UnauthorizedException ex5) {
result.ex5 = ex5;
}
return result;
}
}
public static class updateApplication extends org.apache.thrift.ProcessFunction {
public updateApplication() {
super("updateApplication");
}
public updateApplication_args getEmptyArgsInstance() {
return new updateApplication_args();
}
protected boolean isOneway() {
return false;
}
public updateApplication_result getResult(I iface, updateApplication_args args) throws org.apache.thrift.TException {
updateApplication_result result = new updateApplication_result();
try {
result.success = iface.updateApplication(args.request);
} catch (tech.aroma.thrift.exceptions.OperationFailedException ex1) {
result.ex1 = ex1;
} catch (tech.aroma.thrift.exceptions.InvalidArgumentException ex2) {
result.ex2 = ex2;
} catch (tech.aroma.thrift.exceptions.InvalidTokenException ex3) {
result.ex3 = ex3;
} catch (tech.aroma.thrift.exceptions.ApplicationDoesNotExistException ex4) {
result.ex4 = ex4;
} catch (tech.aroma.thrift.exceptions.UnauthorizedException ex5) {
result.ex5 = ex5;
}
return result;
}
}
public static class updateReactions extends org.apache.thrift.ProcessFunction {
public updateReactions() {
super("updateReactions");
}
public updateReactions_args getEmptyArgsInstance() {
return new updateReactions_args();
}
protected boolean isOneway() {
return false;
}
public updateReactions_result getResult(I iface, updateReactions_args args) throws org.apache.thrift.TException {
updateReactions_result result = new updateReactions_result();
try {
result.success = iface.updateReactions(args.request);
} catch (tech.aroma.thrift.exceptions.OperationFailedException ex1) {
result.ex1 = ex1;
} catch (tech.aroma.thrift.exceptions.InvalidArgumentException ex2) {
result.ex2 = ex2;
} catch (tech.aroma.thrift.exceptions.InvalidTokenException ex3) {
result.ex3 = ex3;
} catch (tech.aroma.thrift.exceptions.ApplicationDoesNotExistException ex4) {
result.ex4 = ex4;
} catch (tech.aroma.thrift.exceptions.UnauthorizedException ex5) {
result.ex5 = ex5;
}
return result;
}
}
public static class getActivity extends org.apache.thrift.ProcessFunction {
public getActivity() {
super("getActivity");
}
public getActivity_args getEmptyArgsInstance() {
return new getActivity_args();
}
protected boolean isOneway() {
return false;
}
public getActivity_result getResult(I iface, getActivity_args args) throws org.apache.thrift.TException {
getActivity_result result = new getActivity_result();
try {
result.success = iface.getActivity(args.request);
} catch (tech.aroma.thrift.exceptions.OperationFailedException ex1) {
result.ex1 = ex1;
} catch (tech.aroma.thrift.exceptions.InvalidArgumentException ex2) {
result.ex2 = ex2;
} catch (tech.aroma.thrift.exceptions.InvalidTokenException ex3) {
result.ex3 = ex3;
}
return result;
}
}
public static class getApplicationInfo extends org.apache.thrift.ProcessFunction {
public getApplicationInfo() {
super("getApplicationInfo");
}
public getApplicationInfo_args getEmptyArgsInstance() {
return new getApplicationInfo_args();
}
protected boolean isOneway() {
return false;
}
public getApplicationInfo_result getResult(I iface, getApplicationInfo_args args) throws org.apache.thrift.TException {
getApplicationInfo_result result = new getApplicationInfo_result();
try {
result.success = iface.getApplicationInfo(args.request);
} catch (tech.aroma.thrift.exceptions.OperationFailedException ex1) {
result.ex1 = ex1;
} catch (tech.aroma.thrift.exceptions.InvalidArgumentException ex2) {
result.ex2 = ex2;
} catch (tech.aroma.thrift.exceptions.InvalidTokenException ex3) {
result.ex3 = ex3;
} catch (tech.aroma.thrift.exceptions.ApplicationDoesNotExistException ex4) {
result.ex4 = ex4;
} catch (tech.aroma.thrift.exceptions.UnauthorizedException ex5) {
result.ex5 = ex5;
}
return result;
}
}
public static class getBuzz extends org.apache.thrift.ProcessFunction {
public getBuzz() {
super("getBuzz");
}
public getBuzz_args getEmptyArgsInstance() {
return new getBuzz_args();
}
protected boolean isOneway() {
return false;
}
public getBuzz_result getResult(I iface, getBuzz_args args) throws org.apache.thrift.TException {
getBuzz_result result = new getBuzz_result();
try {
result.success = iface.getBuzz(args.request);
} catch (tech.aroma.thrift.exceptions.OperationFailedException ex1) {
result.ex1 = ex1;
} catch (tech.aroma.thrift.exceptions.InvalidArgumentException ex2) {
result.ex2 = ex2;
} catch (tech.aroma.thrift.exceptions.InvalidTokenException ex3) {
result.ex3 = ex3;
} catch (tech.aroma.thrift.exceptions.ApplicationDoesNotExistException ex4) {
result.ex4 = ex4;
} catch (tech.aroma.thrift.exceptions.UnauthorizedException ex5) {
result.ex5 = ex5;
}
return result;
}
}
public static class getDashboard extends org.apache.thrift.ProcessFunction {
public getDashboard() {
super("getDashboard");
}
public getDashboard_args getEmptyArgsInstance() {
return new getDashboard_args();
}
protected boolean isOneway() {
return false;
}
public getDashboard_result getResult(I iface, getDashboard_args args) throws org.apache.thrift.TException {
getDashboard_result result = new getDashboard_result();
try {
result.success = iface.getDashboard(args.request);
} catch (tech.aroma.thrift.exceptions.OperationFailedException ex1) {
result.ex1 = ex1;
} catch (tech.aroma.thrift.exceptions.InvalidArgumentException ex2) {
result.ex2 = ex2;
} catch (tech.aroma.thrift.exceptions.InvalidTokenException ex3) {
result.ex3 = ex3;
}
return result;
}
}
public static class getApplicationMessages extends org.apache.thrift.ProcessFunction {
public getApplicationMessages() {
super("getApplicationMessages");
}
public getApplicationMessages_args getEmptyArgsInstance() {
return new getApplicationMessages_args();
}
protected boolean isOneway() {
return false;
}
public getApplicationMessages_result getResult(I iface, getApplicationMessages_args args) throws org.apache.thrift.TException {
getApplicationMessages_result result = new getApplicationMessages_result();
try {
result.success = iface.getApplicationMessages(args.request);
} catch (tech.aroma.thrift.exceptions.OperationFailedException ex1) {
result.ex1 = ex1;
} catch (tech.aroma.thrift.exceptions.InvalidArgumentException ex2) {
result.ex2 = ex2;
} catch (tech.aroma.thrift.exceptions.InvalidTokenException ex3) {
result.ex3 = ex3;
} catch (tech.aroma.thrift.exceptions.UnauthorizedException ex4) {
result.ex4 = ex4;
} catch (tech.aroma.thrift.exceptions.ApplicationDoesNotExistException ex5) {
result.ex5 = ex5;
}
return result;
}
}
public static class getInbox extends org.apache.thrift.ProcessFunction {
public getInbox() {
super("getInbox");
}
public getInbox_args getEmptyArgsInstance() {
return new getInbox_args();
}
protected boolean isOneway() {
return false;
}
public getInbox_result getResult(I iface, getInbox_args args) throws org.apache.thrift.TException {
getInbox_result result = new getInbox_result();
try {
result.success = iface.getInbox(args.request);
} catch (tech.aroma.thrift.exceptions.OperationFailedException ex1) {
result.ex1 = ex1;
} catch (tech.aroma.thrift.exceptions.InvalidArgumentException ex2) {
result.ex2 = ex2;
} catch (tech.aroma.thrift.exceptions.InvalidTokenException ex3) {
result.ex3 = ex3;
}
return result;
}
}
public static class getFullMessage extends org.apache.thrift.ProcessFunction {
public getFullMessage() {
super("getFullMessage");
}
public getFullMessage_args getEmptyArgsInstance() {
return new getFullMessage_args();
}
protected boolean isOneway() {
return false;
}
public getFullMessage_result getResult(I iface, getFullMessage_args args) throws org.apache.thrift.TException {
getFullMessage_result result = new getFullMessage_result();
try {
result.success = iface.getFullMessage(args.request);
} catch (tech.aroma.thrift.exceptions.OperationFailedException ex1) {
result.ex1 = ex1;
} catch (tech.aroma.thrift.exceptions.InvalidArgumentException ex2) {
result.ex2 = ex2;
} catch (tech.aroma.thrift.exceptions.InvalidTokenException ex3) {
result.ex3 = ex3;
}
return result;
}
}
public static class getMedia extends org.apache.thrift.ProcessFunction {
public getMedia() {
super("getMedia");
}
public getMedia_args getEmptyArgsInstance() {
return new getMedia_args();
}
protected boolean isOneway() {
return false;
}
public getMedia_result getResult(I iface, getMedia_args args) throws org.apache.thrift.TException {
getMedia_result result = new getMedia_result();
try {
result.success = iface.getMedia(args.request);
} catch (tech.aroma.thrift.exceptions.OperationFailedException ex1) {
result.ex1 = ex1;
} catch (tech.aroma.thrift.exceptions.InvalidArgumentException ex2) {
result.ex2 = ex2;
} catch (tech.aroma.thrift.exceptions.InvalidTokenException ex3) {
result.ex3 = ex3;
} catch (tech.aroma.thrift.exceptions.DoesNotExistException ex4) {
result.ex4 = ex4;
} catch (tech.aroma.thrift.exceptions.UnauthorizedException ex5) {
result.ex5 = ex5;
}
return result;
}
}
public static class getApplicationsOwnedBy extends org.apache.thrift.ProcessFunction {
public getApplicationsOwnedBy() {
super("getApplicationsOwnedBy");
}
public getApplicationsOwnedBy_args getEmptyArgsInstance() {
return new getApplicationsOwnedBy_args();
}
protected boolean isOneway() {
return false;
}
public getApplicationsOwnedBy_result getResult(I iface, getApplicationsOwnedBy_args args) throws org.apache.thrift.TException {
getApplicationsOwnedBy_result result = new getApplicationsOwnedBy_result();
try {
result.success = iface.getApplicationsOwnedBy(args.request);
} catch (tech.aroma.thrift.exceptions.OperationFailedException ex1) {
result.ex1 = ex1;
} catch (tech.aroma.thrift.exceptions.InvalidArgumentException ex2) {
result.ex2 = ex2;
} catch (tech.aroma.thrift.exceptions.InvalidTokenException ex3) {
result.ex3 = ex3;
}
return result;
}
}
public static class getApplicationsFollowedBy extends org.apache.thrift.ProcessFunction {
public getApplicationsFollowedBy() {
super("getApplicationsFollowedBy");
}
public getApplicationsFollowedBy_args getEmptyArgsInstance() {
return new getApplicationsFollowedBy_args();
}
protected boolean isOneway() {
return false;
}
public getApplicationsFollowedBy_result getResult(I iface, getApplicationsFollowedBy_args args) throws org.apache.thrift.TException {
getApplicationsFollowedBy_result result = new getApplicationsFollowedBy_result();
try {
result.success = iface.getApplicationsFollowedBy(args.request);
} catch (tech.aroma.thrift.exceptions.OperationFailedException ex1) {
result.ex1 = ex1;
} catch (tech.aroma.thrift.exceptions.InvalidArgumentException ex2) {
result.ex2 = ex2;
} catch (tech.aroma.thrift.exceptions.InvalidTokenException ex3) {
result.ex3 = ex3;
}
return result;
}
}
public static class getMySavedChannels extends org.apache.thrift.ProcessFunction {
public getMySavedChannels() {
super("getMySavedChannels");
}
public getMySavedChannels_args getEmptyArgsInstance() {
return new getMySavedChannels_args();
}
protected boolean isOneway() {
return false;
}
public getMySavedChannels_result getResult(I iface, getMySavedChannels_args args) throws org.apache.thrift.TException {
getMySavedChannels_result result = new getMySavedChannels_result();
try {
result.success = iface.getMySavedChannels(args.request);
} catch (tech.aroma.thrift.exceptions.OperationFailedException ex1) {
result.ex1 = ex1;
} catch (tech.aroma.thrift.exceptions.InvalidArgumentException ex2) {
result.ex2 = ex2;
} catch (tech.aroma.thrift.exceptions.InvalidTokenException ex3) {
result.ex3 = ex3;
}
return result;
}
}
public static class getReactions extends org.apache.thrift.ProcessFunction {
public getReactions() {
super("getReactions");
}
public getReactions_args getEmptyArgsInstance() {
return new getReactions_args();
}
protected boolean isOneway() {
return false;
}
public getReactions_result getResult(I iface, getReactions_args args) throws org.apache.thrift.TException {
getReactions_result result = new getReactions_result();
try {
result.success = iface.getReactions(args.request);
} catch (tech.aroma.thrift.exceptions.OperationFailedException ex1) {
result.ex1 = ex1;
} catch (tech.aroma.thrift.exceptions.InvalidArgumentException ex2) {
result.ex2 = ex2;
} catch (tech.aroma.thrift.exceptions.InvalidTokenException ex3) {
result.ex3 = ex3;
} catch (tech.aroma.thrift.exceptions.ApplicationDoesNotExistException ex4) {
result.ex4 = ex4;
} catch (tech.aroma.thrift.exceptions.UnauthorizedException ex5) {
result.ex5 = ex5;
}
return result;
}
}
public static class getUserInfo extends org.apache.thrift.ProcessFunction {
public getUserInfo() {
super("getUserInfo");
}
public getUserInfo_args getEmptyArgsInstance() {
return new getUserInfo_args();
}
protected boolean isOneway() {
return false;
}
public getUserInfo_result getResult(I iface, getUserInfo_args args) throws org.apache.thrift.TException {
getUserInfo_result result = new getUserInfo_result();
try {
result.success = iface.getUserInfo(args.request);
} catch (tech.aroma.thrift.exceptions.OperationFailedException ex1) {
result.ex1 = ex1;
} catch (tech.aroma.thrift.exceptions.InvalidArgumentException ex2) {
result.ex2 = ex2;
} catch (tech.aroma.thrift.exceptions.InvalidTokenException ex3) {
result.ex3 = ex3;
} catch (tech.aroma.thrift.exceptions.UnauthorizedException ex4) {
result.ex4 = ex4;
} catch (tech.aroma.thrift.exceptions.UserDoesNotExistException ex5) {
result.ex5 = ex5;
}
return result;
}
}
public static class searchForApplications extends org.apache.thrift.ProcessFunction {
public searchForApplications() {
super("searchForApplications");
}
public searchForApplications_args getEmptyArgsInstance() {
return new searchForApplications_args();
}
protected boolean isOneway() {
return false;
}
public searchForApplications_result getResult(I iface, searchForApplications_args args) throws org.apache.thrift.TException {
searchForApplications_result result = new searchForApplications_result();
try {
result.success = iface.searchForApplications(args.request);
} catch (tech.aroma.thrift.exceptions.OperationFailedException ex1) {
result.ex1 = ex1;
} catch (tech.aroma.thrift.exceptions.InvalidArgumentException ex2) {
result.ex2 = ex2;
} catch (tech.aroma.thrift.exceptions.InvalidTokenException ex3) {
result.ex3 = ex3;
} catch (tech.aroma.thrift.exceptions.UnauthorizedException ex4) {
result.ex4 = ex4;
}
return result;
}
}
}
public static class AsyncProcessor extends org.apache.thrift.TBaseAsyncProcessor {
private static final Logger LOGGER = LoggerFactory.getLogger(AsyncProcessor.class.getName());
public AsyncProcessor(I iface) {
super(iface, getProcessMap(new HashMap>()));
}
protected AsyncProcessor(I iface, Map> processMap) {
super(iface, getProcessMap(processMap));
}
private static Map> getProcessMap(Map> processMap) {
processMap.put("getApiVersion", new getApiVersion());
processMap.put("deleteApplication", new deleteApplication());
processMap.put("deleteMessage", new deleteMessage());
processMap.put("dismissMessage", new dismissMessage());
processMap.put("provisionApplication", new provisionApplication());
processMap.put("regenerateToken", new regenerateToken());
processMap.put("registerHealthCheck", new registerHealthCheck());
processMap.put("removeSavedChannel", new removeSavedChannel());
processMap.put("renewApplicationToken", new renewApplicationToken());
processMap.put("saveChannel", new saveChannel());
processMap.put("signIn", new signIn());
processMap.put("signUp", new signUp());
processMap.put("snoozeChannel", new snoozeChannel());
processMap.put("followApplication", new followApplication());
processMap.put("unfollowApplication", new unfollowApplication());
processMap.put("updateApplication", new updateApplication());
processMap.put("updateReactions", new updateReactions());
processMap.put("getActivity", new getActivity());
processMap.put("getApplicationInfo", new getApplicationInfo());
processMap.put("getBuzz", new getBuzz());
processMap.put("getDashboard", new getDashboard());
processMap.put("getApplicationMessages", new getApplicationMessages());
processMap.put("getInbox", new getInbox());
processMap.put("getFullMessage", new getFullMessage());
processMap.put("getMedia", new getMedia());
processMap.put("getApplicationsOwnedBy", new getApplicationsOwnedBy());
processMap.put("getApplicationsFollowedBy", new getApplicationsFollowedBy());
processMap.put("getMySavedChannels", new getMySavedChannels());
processMap.put("getReactions", new getReactions());
processMap.put("getUserInfo", new getUserInfo());
processMap.put("searchForApplications", new searchForApplications());
return processMap;
}
public static class getApiVersion extends org.apache.thrift.AsyncProcessFunction {
public getApiVersion() {
super("getApiVersion");
}
public getApiVersion_args getEmptyArgsInstance() {
return new getApiVersion_args();
}
public AsyncMethodCallback getResultHandler(final AsyncFrameBuffer fb, final int seqid) {
final org.apache.thrift.AsyncProcessFunction fcall = this;
return new AsyncMethodCallback() {
public void onComplete(Double o) {
getApiVersion_result result = new getApiVersion_result();
result.success = o;
result.setSuccessIsSet(true);
try {
fcall.sendResponse(fb,result, org.apache.thrift.protocol.TMessageType.REPLY,seqid);
return;
} catch (Exception e) {
LOGGER.error("Exception writing to internal frame buffer", e);
}
fb.close();
}
public void onError(Exception e) {
byte msgType = org.apache.thrift.protocol.TMessageType.REPLY;
org.apache.thrift.TBase msg;
getApiVersion_result result = new getApiVersion_result();
{
msgType = org.apache.thrift.protocol.TMessageType.EXCEPTION;
msg = (org.apache.thrift.TBase)new org.apache.thrift.TApplicationException(org.apache.thrift.TApplicationException.INTERNAL_ERROR, e.getMessage());
}
try {
fcall.sendResponse(fb,msg,msgType,seqid);
return;
} catch (Exception ex) {
LOGGER.error("Exception writing to internal frame buffer", ex);
}
fb.close();
}
};
}
protected boolean isOneway() {
return false;
}
public void start(I iface, getApiVersion_args args, org.apache.thrift.async.AsyncMethodCallback resultHandler) throws TException {
iface.getApiVersion(resultHandler);
}
}
public static class deleteApplication extends org.apache.thrift.AsyncProcessFunction {
public deleteApplication() {
super("deleteApplication");
}
public deleteApplication_args getEmptyArgsInstance() {
return new deleteApplication_args();
}
public AsyncMethodCallback getResultHandler(final AsyncFrameBuffer fb, final int seqid) {
final org.apache.thrift.AsyncProcessFunction fcall = this;
return new AsyncMethodCallback() {
public void onComplete(DeleteApplicationResponse o) {
deleteApplication_result result = new deleteApplication_result();
result.success = o;
try {
fcall.sendResponse(fb,result, org.apache.thrift.protocol.TMessageType.REPLY,seqid);
return;
} catch (Exception e) {
LOGGER.error("Exception writing to internal frame buffer", e);
}
fb.close();
}
public void onError(Exception e) {
byte msgType = org.apache.thrift.protocol.TMessageType.REPLY;
org.apache.thrift.TBase msg;
deleteApplication_result result = new deleteApplication_result();
if (e instanceof tech.aroma.thrift.exceptions.OperationFailedException) {
result.ex1 = (tech.aroma.thrift.exceptions.OperationFailedException) e;
result.setEx1IsSet(true);
msg = result;
}
else if (e instanceof tech.aroma.thrift.exceptions.InvalidArgumentException) {
result.ex2 = (tech.aroma.thrift.exceptions.InvalidArgumentException) e;
result.setEx2IsSet(true);
msg = result;
}
else if (e instanceof tech.aroma.thrift.exceptions.InvalidTokenException) {
result.ex3 = (tech.aroma.thrift.exceptions.InvalidTokenException) e;
result.setEx3IsSet(true);
msg = result;
}
else if (e instanceof tech.aroma.thrift.exceptions.ApplicationDoesNotExistException) {
result.ex4 = (tech.aroma.thrift.exceptions.ApplicationDoesNotExistException) e;
result.setEx4IsSet(true);
msg = result;
}
else if (e instanceof tech.aroma.thrift.exceptions.UnauthorizedException) {
result.ex5 = (tech.aroma.thrift.exceptions.UnauthorizedException) e;
result.setEx5IsSet(true);
msg = result;
}
else
{
msgType = org.apache.thrift.protocol.TMessageType.EXCEPTION;
msg = (org.apache.thrift.TBase)new org.apache.thrift.TApplicationException(org.apache.thrift.TApplicationException.INTERNAL_ERROR, e.getMessage());
}
try {
fcall.sendResponse(fb,msg,msgType,seqid);
return;
} catch (Exception ex) {
LOGGER.error("Exception writing to internal frame buffer", ex);
}
fb.close();
}
};
}
protected boolean isOneway() {
return false;
}
public void start(I iface, deleteApplication_args args, org.apache.thrift.async.AsyncMethodCallback resultHandler) throws TException {
iface.deleteApplication(args.request,resultHandler);
}
}
public static class deleteMessage extends org.apache.thrift.AsyncProcessFunction {
public deleteMessage() {
super("deleteMessage");
}
public deleteMessage_args getEmptyArgsInstance() {
return new deleteMessage_args();
}
public AsyncMethodCallback getResultHandler(final AsyncFrameBuffer fb, final int seqid) {
final org.apache.thrift.AsyncProcessFunction fcall = this;
return new AsyncMethodCallback() {
public void onComplete(DeleteMessageResponse o) {
deleteMessage_result result = new deleteMessage_result();
result.success = o;
try {
fcall.sendResponse(fb,result, org.apache.thrift.protocol.TMessageType.REPLY,seqid);
return;
} catch (Exception e) {
LOGGER.error("Exception writing to internal frame buffer", e);
}
fb.close();
}
public void onError(Exception e) {
byte msgType = org.apache.thrift.protocol.TMessageType.REPLY;
org.apache.thrift.TBase msg;
deleteMessage_result result = new deleteMessage_result();
if (e instanceof tech.aroma.thrift.exceptions.OperationFailedException) {
result.ex1 = (tech.aroma.thrift.exceptions.OperationFailedException) e;
result.setEx1IsSet(true);
msg = result;
}
else if (e instanceof tech.aroma.thrift.exceptions.InvalidArgumentException) {
result.ex2 = (tech.aroma.thrift.exceptions.InvalidArgumentException) e;
result.setEx2IsSet(true);
msg = result;
}
else if (e instanceof tech.aroma.thrift.exceptions.InvalidTokenException) {
result.ex3 = (tech.aroma.thrift.exceptions.InvalidTokenException) e;
result.setEx3IsSet(true);
msg = result;
}
else if (e instanceof tech.aroma.thrift.exceptions.MessageDoesNotExistException) {
result.ex4 = (tech.aroma.thrift.exceptions.MessageDoesNotExistException) e;
result.setEx4IsSet(true);
msg = result;
}
else if (e instanceof tech.aroma.thrift.exceptions.UnauthorizedException) {
result.ex5 = (tech.aroma.thrift.exceptions.UnauthorizedException) e;
result.setEx5IsSet(true);
msg = result;
}
else
{
msgType = org.apache.thrift.protocol.TMessageType.EXCEPTION;
msg = (org.apache.thrift.TBase)new org.apache.thrift.TApplicationException(org.apache.thrift.TApplicationException.INTERNAL_ERROR, e.getMessage());
}
try {
fcall.sendResponse(fb,msg,msgType,seqid);
return;
} catch (Exception ex) {
LOGGER.error("Exception writing to internal frame buffer", ex);
}
fb.close();
}
};
}
protected boolean isOneway() {
return false;
}
public void start(I iface, deleteMessage_args args, org.apache.thrift.async.AsyncMethodCallback resultHandler) throws TException {
iface.deleteMessage(args.request,resultHandler);
}
}
public static class dismissMessage extends org.apache.thrift.AsyncProcessFunction {
public dismissMessage() {
super("dismissMessage");
}
public dismissMessage_args getEmptyArgsInstance() {
return new dismissMessage_args();
}
public AsyncMethodCallback getResultHandler(final AsyncFrameBuffer fb, final int seqid) {
final org.apache.thrift.AsyncProcessFunction fcall = this;
return new AsyncMethodCallback() {
public void onComplete(DismissMessageResponse o) {
dismissMessage_result result = new dismissMessage_result();
result.success = o;
try {
fcall.sendResponse(fb,result, org.apache.thrift.protocol.TMessageType.REPLY,seqid);
return;
} catch (Exception e) {
LOGGER.error("Exception writing to internal frame buffer", e);
}
fb.close();
}
public void onError(Exception e) {
byte msgType = org.apache.thrift.protocol.TMessageType.REPLY;
org.apache.thrift.TBase msg;
dismissMessage_result result = new dismissMessage_result();
if (e instanceof tech.aroma.thrift.exceptions.OperationFailedException) {
result.ex1 = (tech.aroma.thrift.exceptions.OperationFailedException) e;
result.setEx1IsSet(true);
msg = result;
}
else if (e instanceof tech.aroma.thrift.exceptions.InvalidArgumentException) {
result.ex2 = (tech.aroma.thrift.exceptions.InvalidArgumentException) e;
result.setEx2IsSet(true);
msg = result;
}
else if (e instanceof tech.aroma.thrift.exceptions.InvalidTokenException) {
result.ex3 = (tech.aroma.thrift.exceptions.InvalidTokenException) e;
result.setEx3IsSet(true);
msg = result;
}
else if (e instanceof tech.aroma.thrift.exceptions.MessageDoesNotExistException) {
result.ex4 = (tech.aroma.thrift.exceptions.MessageDoesNotExistException) e;
result.setEx4IsSet(true);
msg = result;
}
else if (e instanceof tech.aroma.thrift.exceptions.UnauthorizedException) {
result.ex5 = (tech.aroma.thrift.exceptions.UnauthorizedException) e;
result.setEx5IsSet(true);
msg = result;
}
else
{
msgType = org.apache.thrift.protocol.TMessageType.EXCEPTION;
msg = (org.apache.thrift.TBase)new org.apache.thrift.TApplicationException(org.apache.thrift.TApplicationException.INTERNAL_ERROR, e.getMessage());
}
try {
fcall.sendResponse(fb,msg,msgType,seqid);
return;
} catch (Exception ex) {
LOGGER.error("Exception writing to internal frame buffer", ex);
}
fb.close();
}
};
}
protected boolean isOneway() {
return false;
}
public void start(I iface, dismissMessage_args args, org.apache.thrift.async.AsyncMethodCallback resultHandler) throws TException {
iface.dismissMessage(args.request,resultHandler);
}
}
public static class provisionApplication extends org.apache.thrift.AsyncProcessFunction {
public provisionApplication() {
super("provisionApplication");
}
public provisionApplication_args getEmptyArgsInstance() {
return new provisionApplication_args();
}
public AsyncMethodCallback getResultHandler(final AsyncFrameBuffer fb, final int seqid) {
final org.apache.thrift.AsyncProcessFunction fcall = this;
return new AsyncMethodCallback() {
public void onComplete(ProvisionApplicationResponse o) {
provisionApplication_result result = new provisionApplication_result();
result.success = o;
try {
fcall.sendResponse(fb,result, org.apache.thrift.protocol.TMessageType.REPLY,seqid);
return;
} catch (Exception e) {
LOGGER.error("Exception writing to internal frame buffer", e);
}
fb.close();
}
public void onError(Exception e) {
byte msgType = org.apache.thrift.protocol.TMessageType.REPLY;
org.apache.thrift.TBase msg;
provisionApplication_result result = new provisionApplication_result();
if (e instanceof tech.aroma.thrift.exceptions.OperationFailedException) {
result.ex1 = (tech.aroma.thrift.exceptions.OperationFailedException) e;
result.setEx1IsSet(true);
msg = result;
}
else if (e instanceof tech.aroma.thrift.exceptions.InvalidArgumentException) {
result.ex2 = (tech.aroma.thrift.exceptions.InvalidArgumentException) e;
result.setEx2IsSet(true);
msg = result;
}
else if (e instanceof tech.aroma.thrift.exceptions.InvalidTokenException) {
result.ex3 = (tech.aroma.thrift.exceptions.InvalidTokenException) e;
result.setEx3IsSet(true);
msg = result;
}
else if (e instanceof tech.aroma.thrift.exceptions.ApplicationDoesNotExistException) {
result.ex4 = (tech.aroma.thrift.exceptions.ApplicationDoesNotExistException) e;
result.setEx4IsSet(true);
msg = result;
}
else if (e instanceof tech.aroma.thrift.exceptions.UnauthorizedException) {
result.ex5 = (tech.aroma.thrift.exceptions.UnauthorizedException) e;
result.setEx5IsSet(true);
msg = result;
}
else
{
msgType = org.apache.thrift.protocol.TMessageType.EXCEPTION;
msg = (org.apache.thrift.TBase)new org.apache.thrift.TApplicationException(org.apache.thrift.TApplicationException.INTERNAL_ERROR, e.getMessage());
}
try {
fcall.sendResponse(fb,msg,msgType,seqid);
return;
} catch (Exception ex) {
LOGGER.error("Exception writing to internal frame buffer", ex);
}
fb.close();
}
};
}
protected boolean isOneway() {
return false;
}
public void start(I iface, provisionApplication_args args, org.apache.thrift.async.AsyncMethodCallback resultHandler) throws TException {
iface.provisionApplication(args.request,resultHandler);
}
}
public static class regenerateToken extends org.apache.thrift.AsyncProcessFunction {
public regenerateToken() {
super("regenerateToken");
}
public regenerateToken_args getEmptyArgsInstance() {
return new regenerateToken_args();
}
public AsyncMethodCallback getResultHandler(final AsyncFrameBuffer fb, final int seqid) {
final org.apache.thrift.AsyncProcessFunction fcall = this;
return new AsyncMethodCallback() {
public void onComplete(RegenerateApplicationTokenResponse o) {
regenerateToken_result result = new regenerateToken_result();
result.success = o;
try {
fcall.sendResponse(fb,result, org.apache.thrift.protocol.TMessageType.REPLY,seqid);
return;
} catch (Exception e) {
LOGGER.error("Exception writing to internal frame buffer", e);
}
fb.close();
}
public void onError(Exception e) {
byte msgType = org.apache.thrift.protocol.TMessageType.REPLY;
org.apache.thrift.TBase msg;
regenerateToken_result result = new regenerateToken_result();
if (e instanceof tech.aroma.thrift.exceptions.OperationFailedException) {
result.ex1 = (tech.aroma.thrift.exceptions.OperationFailedException) e;
result.setEx1IsSet(true);
msg = result;
}
else if (e instanceof tech.aroma.thrift.exceptions.InvalidArgumentException) {
result.ex2 = (tech.aroma.thrift.exceptions.InvalidArgumentException) e;
result.setEx2IsSet(true);
msg = result;
}
else if (e instanceof tech.aroma.thrift.exceptions.InvalidTokenException) {
result.ex3 = (tech.aroma.thrift.exceptions.InvalidTokenException) e;
result.setEx3IsSet(true);
msg = result;
}
else if (e instanceof tech.aroma.thrift.exceptions.ApplicationDoesNotExistException) {
result.ex4 = (tech.aroma.thrift.exceptions.ApplicationDoesNotExistException) e;
result.setEx4IsSet(true);
msg = result;
}
else if (e instanceof tech.aroma.thrift.exceptions.UnauthorizedException) {
result.ex5 = (tech.aroma.thrift.exceptions.UnauthorizedException) e;
result.setEx5IsSet(true);
msg = result;
}
else
{
msgType = org.apache.thrift.protocol.TMessageType.EXCEPTION;
msg = (org.apache.thrift.TBase)new org.apache.thrift.TApplicationException(org.apache.thrift.TApplicationException.INTERNAL_ERROR, e.getMessage());
}
try {
fcall.sendResponse(fb,msg,msgType,seqid);
return;
} catch (Exception ex) {
LOGGER.error("Exception writing to internal frame buffer", ex);
}
fb.close();
}
};
}
protected boolean isOneway() {
return false;
}
public void start(I iface, regenerateToken_args args, org.apache.thrift.async.AsyncMethodCallback resultHandler) throws TException {
iface.regenerateToken(args.request,resultHandler);
}
}
public static class registerHealthCheck extends org.apache.thrift.AsyncProcessFunction {
public registerHealthCheck() {
super("registerHealthCheck");
}
public registerHealthCheck_args getEmptyArgsInstance() {
return new registerHealthCheck_args();
}
public AsyncMethodCallback getResultHandler(final AsyncFrameBuffer fb, final int seqid) {
final org.apache.thrift.AsyncProcessFunction fcall = this;
return new AsyncMethodCallback() {
public void onComplete(RegisterHealthCheckResponse o) {
registerHealthCheck_result result = new registerHealthCheck_result();
result.success = o;
try {
fcall.sendResponse(fb,result, org.apache.thrift.protocol.TMessageType.REPLY,seqid);
return;
} catch (Exception e) {
LOGGER.error("Exception writing to internal frame buffer", e);
}
fb.close();
}
public void onError(Exception e) {
byte msgType = org.apache.thrift.protocol.TMessageType.REPLY;
org.apache.thrift.TBase msg;
registerHealthCheck_result result = new registerHealthCheck_result();
if (e instanceof tech.aroma.thrift.exceptions.OperationFailedException) {
result.ex1 = (tech.aroma.thrift.exceptions.OperationFailedException) e;
result.setEx1IsSet(true);
msg = result;
}
else if (e instanceof tech.aroma.thrift.exceptions.InvalidArgumentException) {
result.ex2 = (tech.aroma.thrift.exceptions.InvalidArgumentException) e;
result.setEx2IsSet(true);
msg = result;
}
else if (e instanceof tech.aroma.thrift.exceptions.InvalidTokenException) {
result.ex3 = (tech.aroma.thrift.exceptions.InvalidTokenException) e;
result.setEx3IsSet(true);
msg = result;
}
else if (e instanceof tech.aroma.thrift.exceptions.ApplicationDoesNotExistException) {
result.ex4 = (tech.aroma.thrift.exceptions.ApplicationDoesNotExistException) e;
result.setEx4IsSet(true);
msg = result;
}
else if (e instanceof tech.aroma.thrift.exceptions.UnauthorizedException) {
result.ex5 = (tech.aroma.thrift.exceptions.UnauthorizedException) e;
result.setEx5IsSet(true);
msg = result;
}
else
{
msgType = org.apache.thrift.protocol.TMessageType.EXCEPTION;
msg = (org.apache.thrift.TBase)new org.apache.thrift.TApplicationException(org.apache.thrift.TApplicationException.INTERNAL_ERROR, e.getMessage());
}
try {
fcall.sendResponse(fb,msg,msgType,seqid);
return;
} catch (Exception ex) {
LOGGER.error("Exception writing to internal frame buffer", ex);
}
fb.close();
}
};
}
protected boolean isOneway() {
return false;
}
public void start(I iface, registerHealthCheck_args args, org.apache.thrift.async.AsyncMethodCallback resultHandler) throws TException {
iface.registerHealthCheck(args.request,resultHandler);
}
}
public static class removeSavedChannel extends org.apache.thrift.AsyncProcessFunction {
public removeSavedChannel() {
super("removeSavedChannel");
}
public removeSavedChannel_args getEmptyArgsInstance() {
return new removeSavedChannel_args();
}
public AsyncMethodCallback getResultHandler(final AsyncFrameBuffer fb, final int seqid) {
final org.apache.thrift.AsyncProcessFunction fcall = this;
return new AsyncMethodCallback() {
public void onComplete(RemoveSavedChannelResponse o) {
removeSavedChannel_result result = new removeSavedChannel_result();
result.success = o;
try {
fcall.sendResponse(fb,result, org.apache.thrift.protocol.TMessageType.REPLY,seqid);
return;
} catch (Exception e) {
LOGGER.error("Exception writing to internal frame buffer", e);
}
fb.close();
}
public void onError(Exception e) {
byte msgType = org.apache.thrift.protocol.TMessageType.REPLY;
org.apache.thrift.TBase msg;
removeSavedChannel_result result = new removeSavedChannel_result();
if (e instanceof tech.aroma.thrift.exceptions.OperationFailedException) {
result.ex1 = (tech.aroma.thrift.exceptions.OperationFailedException) e;
result.setEx1IsSet(true);
msg = result;
}
else if (e instanceof tech.aroma.thrift.exceptions.InvalidArgumentException) {
result.ex2 = (tech.aroma.thrift.exceptions.InvalidArgumentException) e;
result.setEx2IsSet(true);
msg = result;
}
else if (e instanceof tech.aroma.thrift.exceptions.InvalidTokenException) {
result.ex3 = (tech.aroma.thrift.exceptions.InvalidTokenException) e;
result.setEx3IsSet(true);
msg = result;
}
else if (e instanceof tech.aroma.thrift.exceptions.UnauthorizedException) {
result.ex4 = (tech.aroma.thrift.exceptions.UnauthorizedException) e;
result.setEx4IsSet(true);
msg = result;
}
else if (e instanceof tech.aroma.thrift.exceptions.ChannelDoesNotExistException) {
result.ex5 = (tech.aroma.thrift.exceptions.ChannelDoesNotExistException) e;
result.setEx5IsSet(true);
msg = result;
}
else
{
msgType = org.apache.thrift.protocol.TMessageType.EXCEPTION;
msg = (org.apache.thrift.TBase)new org.apache.thrift.TApplicationException(org.apache.thrift.TApplicationException.INTERNAL_ERROR, e.getMessage());
}
try {
fcall.sendResponse(fb,msg,msgType,seqid);
return;
} catch (Exception ex) {
LOGGER.error("Exception writing to internal frame buffer", ex);
}
fb.close();
}
};
}
protected boolean isOneway() {
return false;
}
public void start(I iface, removeSavedChannel_args args, org.apache.thrift.async.AsyncMethodCallback resultHandler) throws TException {
iface.removeSavedChannel(args.request,resultHandler);
}
}
public static class renewApplicationToken extends org.apache.thrift.AsyncProcessFunction {
public renewApplicationToken() {
super("renewApplicationToken");
}
public renewApplicationToken_args getEmptyArgsInstance() {
return new renewApplicationToken_args();
}
public AsyncMethodCallback getResultHandler(final AsyncFrameBuffer fb, final int seqid) {
final org.apache.thrift.AsyncProcessFunction fcall = this;
return new AsyncMethodCallback() {
public void onComplete(RenewApplicationTokenResponse o) {
renewApplicationToken_result result = new renewApplicationToken_result();
result.success = o;
try {
fcall.sendResponse(fb,result, org.apache.thrift.protocol.TMessageType.REPLY,seqid);
return;
} catch (Exception e) {
LOGGER.error("Exception writing to internal frame buffer", e);
}
fb.close();
}
public void onError(Exception e) {
byte msgType = org.apache.thrift.protocol.TMessageType.REPLY;
org.apache.thrift.TBase msg;
renewApplicationToken_result result = new renewApplicationToken_result();
if (e instanceof tech.aroma.thrift.exceptions.OperationFailedException) {
result.ex1 = (tech.aroma.thrift.exceptions.OperationFailedException) e;
result.setEx1IsSet(true);
msg = result;
}
else if (e instanceof tech.aroma.thrift.exceptions.InvalidArgumentException) {
result.ex2 = (tech.aroma.thrift.exceptions.InvalidArgumentException) e;
result.setEx2IsSet(true);
msg = result;
}
else if (e instanceof tech.aroma.thrift.exceptions.InvalidTokenException) {
result.ex3 = (tech.aroma.thrift.exceptions.InvalidTokenException) e;
result.setEx3IsSet(true);
msg = result;
}
else if (e instanceof tech.aroma.thrift.exceptions.ApplicationDoesNotExistException) {
result.ex4 = (tech.aroma.thrift.exceptions.ApplicationDoesNotExistException) e;
result.setEx4IsSet(true);
msg = result;
}
else if (e instanceof tech.aroma.thrift.exceptions.UnauthorizedException) {
result.ex5 = (tech.aroma.thrift.exceptions.UnauthorizedException) e;
result.setEx5IsSet(true);
msg = result;
}
else
{
msgType = org.apache.thrift.protocol.TMessageType.EXCEPTION;
msg = (org.apache.thrift.TBase)new org.apache.thrift.TApplicationException(org.apache.thrift.TApplicationException.INTERNAL_ERROR, e.getMessage());
}
try {
fcall.sendResponse(fb,msg,msgType,seqid);
return;
} catch (Exception ex) {
LOGGER.error("Exception writing to internal frame buffer", ex);
}
fb.close();
}
};
}
protected boolean isOneway() {
return false;
}
public void start(I iface, renewApplicationToken_args args, org.apache.thrift.async.AsyncMethodCallback resultHandler) throws TException {
iface.renewApplicationToken(args.request,resultHandler);
}
}
public static class saveChannel extends org.apache.thrift.AsyncProcessFunction {
public saveChannel() {
super("saveChannel");
}
public saveChannel_args getEmptyArgsInstance() {
return new saveChannel_args();
}
public AsyncMethodCallback getResultHandler(final AsyncFrameBuffer fb, final int seqid) {
final org.apache.thrift.AsyncProcessFunction fcall = this;
return new AsyncMethodCallback() {
public void onComplete(SaveChannelResponse o) {
saveChannel_result result = new saveChannel_result();
result.success = o;
try {
fcall.sendResponse(fb,result, org.apache.thrift.protocol.TMessageType.REPLY,seqid);
return;
} catch (Exception e) {
LOGGER.error("Exception writing to internal frame buffer", e);
}
fb.close();
}
public void onError(Exception e) {
byte msgType = org.apache.thrift.protocol.TMessageType.REPLY;
org.apache.thrift.TBase msg;
saveChannel_result result = new saveChannel_result();
if (e instanceof tech.aroma.thrift.exceptions.OperationFailedException) {
result.ex1 = (tech.aroma.thrift.exceptions.OperationFailedException) e;
result.setEx1IsSet(true);
msg = result;
}
else if (e instanceof tech.aroma.thrift.exceptions.InvalidArgumentException) {
result.ex2 = (tech.aroma.thrift.exceptions.InvalidArgumentException) e;
result.setEx2IsSet(true);
msg = result;
}
else if (e instanceof tech.aroma.thrift.exceptions.InvalidTokenException) {
result.ex3 = (tech.aroma.thrift.exceptions.InvalidTokenException) e;
result.setEx3IsSet(true);
msg = result;
}
else if (e instanceof tech.aroma.thrift.exceptions.UnauthorizedException) {
result.ex4 = (tech.aroma.thrift.exceptions.UnauthorizedException) e;
result.setEx4IsSet(true);
msg = result;
}
else
{
msgType = org.apache.thrift.protocol.TMessageType.EXCEPTION;
msg = (org.apache.thrift.TBase)new org.apache.thrift.TApplicationException(org.apache.thrift.TApplicationException.INTERNAL_ERROR, e.getMessage());
}
try {
fcall.sendResponse(fb,msg,msgType,seqid);
return;
} catch (Exception ex) {
LOGGER.error("Exception writing to internal frame buffer", ex);
}
fb.close();
}
};
}
protected boolean isOneway() {
return false;
}
public void start(I iface, saveChannel_args args, org.apache.thrift.async.AsyncMethodCallback resultHandler) throws TException {
iface.saveChannel(args.request,resultHandler);
}
}
public static class signIn extends org.apache.thrift.AsyncProcessFunction {
public signIn() {
super("signIn");
}
public signIn_args getEmptyArgsInstance() {
return new signIn_args();
}
public AsyncMethodCallback getResultHandler(final AsyncFrameBuffer fb, final int seqid) {
final org.apache.thrift.AsyncProcessFunction fcall = this;
return new AsyncMethodCallback() {
public void onComplete(SignInResponse o) {
signIn_result result = new signIn_result();
result.success = o;
try {
fcall.sendResponse(fb,result, org.apache.thrift.protocol.TMessageType.REPLY,seqid);
return;
} catch (Exception e) {
LOGGER.error("Exception writing to internal frame buffer", e);
}
fb.close();
}
public void onError(Exception e) {
byte msgType = org.apache.thrift.protocol.TMessageType.REPLY;
org.apache.thrift.TBase msg;
signIn_result result = new signIn_result();
if (e instanceof tech.aroma.thrift.exceptions.OperationFailedException) {
result.ex1 = (tech.aroma.thrift.exceptions.OperationFailedException) e;
result.setEx1IsSet(true);
msg = result;
}
else if (e instanceof tech.aroma.thrift.exceptions.InvalidArgumentException) {
result.ex2 = (tech.aroma.thrift.exceptions.InvalidArgumentException) e;
result.setEx2IsSet(true);
msg = result;
}
else if (e instanceof tech.aroma.thrift.exceptions.InvalidCredentialsException) {
result.ex3 = (tech.aroma.thrift.exceptions.InvalidCredentialsException) e;
result.setEx3IsSet(true);
msg = result;
}
else if (e instanceof tech.aroma.thrift.exceptions.UserDoesNotExistException) {
result.ex4 = (tech.aroma.thrift.exceptions.UserDoesNotExistException) e;
result.setEx4IsSet(true);
msg = result;
}
else
{
msgType = org.apache.thrift.protocol.TMessageType.EXCEPTION;
msg = (org.apache.thrift.TBase)new org.apache.thrift.TApplicationException(org.apache.thrift.TApplicationException.INTERNAL_ERROR, e.getMessage());
}
try {
fcall.sendResponse(fb,msg,msgType,seqid);
return;
} catch (Exception ex) {
LOGGER.error("Exception writing to internal frame buffer", ex);
}
fb.close();
}
};
}
protected boolean isOneway() {
return false;
}
public void start(I iface, signIn_args args, org.apache.thrift.async.AsyncMethodCallback resultHandler) throws TException {
iface.signIn(args.request,resultHandler);
}
}
public static class signUp extends org.apache.thrift.AsyncProcessFunction {
public signUp() {
super("signUp");
}
public signUp_args getEmptyArgsInstance() {
return new signUp_args();
}
public AsyncMethodCallback getResultHandler(final AsyncFrameBuffer fb, final int seqid) {
final org.apache.thrift.AsyncProcessFunction fcall = this;
return new AsyncMethodCallback() {
public void onComplete(SignUpResponse o) {
signUp_result result = new signUp_result();
result.success = o;
try {
fcall.sendResponse(fb,result, org.apache.thrift.protocol.TMessageType.REPLY,seqid);
return;
} catch (Exception e) {
LOGGER.error("Exception writing to internal frame buffer", e);
}
fb.close();
}
public void onError(Exception e) {
byte msgType = org.apache.thrift.protocol.TMessageType.REPLY;
org.apache.thrift.TBase msg;
signUp_result result = new signUp_result();
if (e instanceof tech.aroma.thrift.exceptions.OperationFailedException) {
result.ex1 = (tech.aroma.thrift.exceptions.OperationFailedException) e;
result.setEx1IsSet(true);
msg = result;
}
else if (e instanceof tech.aroma.thrift.exceptions.InvalidArgumentException) {
result.ex2 = (tech.aroma.thrift.exceptions.InvalidArgumentException) e;
result.setEx2IsSet(true);
msg = result;
}
else if (e instanceof tech.aroma.thrift.exceptions.InvalidCredentialsException) {
result.ex3 = (tech.aroma.thrift.exceptions.InvalidCredentialsException) e;
result.setEx3IsSet(true);
msg = result;
}
else if (e instanceof tech.aroma.thrift.exceptions.AccountAlreadyExistsException) {
result.ex4 = (tech.aroma.thrift.exceptions.AccountAlreadyExistsException) e;
result.setEx4IsSet(true);
msg = result;
}
else
{
msgType = org.apache.thrift.protocol.TMessageType.EXCEPTION;
msg = (org.apache.thrift.TBase)new org.apache.thrift.TApplicationException(org.apache.thrift.TApplicationException.INTERNAL_ERROR, e.getMessage());
}
try {
fcall.sendResponse(fb,msg,msgType,seqid);
return;
} catch (Exception ex) {
LOGGER.error("Exception writing to internal frame buffer", ex);
}
fb.close();
}
};
}
protected boolean isOneway() {
return false;
}
public void start(I iface, signUp_args args, org.apache.thrift.async.AsyncMethodCallback resultHandler) throws TException {
iface.signUp(args.request,resultHandler);
}
}
public static class snoozeChannel extends org.apache.thrift.AsyncProcessFunction {
public snoozeChannel() {
super("snoozeChannel");
}
public snoozeChannel_args getEmptyArgsInstance() {
return new snoozeChannel_args();
}
public AsyncMethodCallback getResultHandler(final AsyncFrameBuffer fb, final int seqid) {
final org.apache.thrift.AsyncProcessFunction fcall = this;
return new AsyncMethodCallback() {
public void onComplete(SnoozeChannelResponse o) {
snoozeChannel_result result = new snoozeChannel_result();
result.success = o;
try {
fcall.sendResponse(fb,result, org.apache.thrift.protocol.TMessageType.REPLY,seqid);
return;
} catch (Exception e) {
LOGGER.error("Exception writing to internal frame buffer", e);
}
fb.close();
}
public void onError(Exception e) {
byte msgType = org.apache.thrift.protocol.TMessageType.REPLY;
org.apache.thrift.TBase msg;
snoozeChannel_result result = new snoozeChannel_result();
if (e instanceof tech.aroma.thrift.exceptions.OperationFailedException) {
result.ex1 = (tech.aroma.thrift.exceptions.OperationFailedException) e;
result.setEx1IsSet(true);
msg = result;
}
else if (e instanceof tech.aroma.thrift.exceptions.InvalidArgumentException) {
result.ex2 = (tech.aroma.thrift.exceptions.InvalidArgumentException) e;
result.setEx2IsSet(true);
msg = result;
}
else if (e instanceof tech.aroma.thrift.exceptions.InvalidTokenException) {
result.ex3 = (tech.aroma.thrift.exceptions.InvalidTokenException) e;
result.setEx3IsSet(true);
msg = result;
}
else if (e instanceof tech.aroma.thrift.exceptions.UnauthorizedException) {
result.ex4 = (tech.aroma.thrift.exceptions.UnauthorizedException) e;
result.setEx4IsSet(true);
msg = result;
}
else if (e instanceof tech.aroma.thrift.exceptions.ChannelDoesNotExistException) {
result.ex5 = (tech.aroma.thrift.exceptions.ChannelDoesNotExistException) e;
result.setEx5IsSet(true);
msg = result;
}
else
{
msgType = org.apache.thrift.protocol.TMessageType.EXCEPTION;
msg = (org.apache.thrift.TBase)new org.apache.thrift.TApplicationException(org.apache.thrift.TApplicationException.INTERNAL_ERROR, e.getMessage());
}
try {
fcall.sendResponse(fb,msg,msgType,seqid);
return;
} catch (Exception ex) {
LOGGER.error("Exception writing to internal frame buffer", ex);
}
fb.close();
}
};
}
protected boolean isOneway() {
return false;
}
public void start(I iface, snoozeChannel_args args, org.apache.thrift.async.AsyncMethodCallback resultHandler) throws TException {
iface.snoozeChannel(args.request,resultHandler);
}
}
public static class followApplication extends org.apache.thrift.AsyncProcessFunction {
public followApplication() {
super("followApplication");
}
public followApplication_args getEmptyArgsInstance() {
return new followApplication_args();
}
public AsyncMethodCallback getResultHandler(final AsyncFrameBuffer fb, final int seqid) {
final org.apache.thrift.AsyncProcessFunction fcall = this;
return new AsyncMethodCallback() {
public void onComplete(FollowApplicationResponse o) {
followApplication_result result = new followApplication_result();
result.success = o;
try {
fcall.sendResponse(fb,result, org.apache.thrift.protocol.TMessageType.REPLY,seqid);
return;
} catch (Exception e) {
LOGGER.error("Exception writing to internal frame buffer", e);
}
fb.close();
}
public void onError(Exception e) {
byte msgType = org.apache.thrift.protocol.TMessageType.REPLY;
org.apache.thrift.TBase msg;
followApplication_result result = new followApplication_result();
if (e instanceof tech.aroma.thrift.exceptions.OperationFailedException) {
result.ex1 = (tech.aroma.thrift.exceptions.OperationFailedException) e;
result.setEx1IsSet(true);
msg = result;
}
else if (e instanceof tech.aroma.thrift.exceptions.InvalidArgumentException) {
result.ex2 = (tech.aroma.thrift.exceptions.InvalidArgumentException) e;
result.setEx2IsSet(true);
msg = result;
}
else if (e instanceof tech.aroma.thrift.exceptions.InvalidTokenException) {
result.ex3 = (tech.aroma.thrift.exceptions.InvalidTokenException) e;
result.setEx3IsSet(true);
msg = result;
}
else if (e instanceof tech.aroma.thrift.exceptions.ApplicationDoesNotExistException) {
result.ex4 = (tech.aroma.thrift.exceptions.ApplicationDoesNotExistException) e;
result.setEx4IsSet(true);
msg = result;
}
else if (e instanceof tech.aroma.thrift.exceptions.UnauthorizedException) {
result.ex5 = (tech.aroma.thrift.exceptions.UnauthorizedException) e;
result.setEx5IsSet(true);
msg = result;
}
else
{
msgType = org.apache.thrift.protocol.TMessageType.EXCEPTION;
msg = (org.apache.thrift.TBase)new org.apache.thrift.TApplicationException(org.apache.thrift.TApplicationException.INTERNAL_ERROR, e.getMessage());
}
try {
fcall.sendResponse(fb,msg,msgType,seqid);
return;
} catch (Exception ex) {
LOGGER.error("Exception writing to internal frame buffer", ex);
}
fb.close();
}
};
}
protected boolean isOneway() {
return false;
}
public void start(I iface, followApplication_args args, org.apache.thrift.async.AsyncMethodCallback resultHandler) throws TException {
iface.followApplication(args.request,resultHandler);
}
}
public static class unfollowApplication extends org.apache.thrift.AsyncProcessFunction {
public unfollowApplication() {
super("unfollowApplication");
}
public unfollowApplication_args getEmptyArgsInstance() {
return new unfollowApplication_args();
}
public AsyncMethodCallback getResultHandler(final AsyncFrameBuffer fb, final int seqid) {
final org.apache.thrift.AsyncProcessFunction fcall = this;
return new AsyncMethodCallback() {
public void onComplete(UnfollowApplicationResponse o) {
unfollowApplication_result result = new unfollowApplication_result();
result.success = o;
try {
fcall.sendResponse(fb,result, org.apache.thrift.protocol.TMessageType.REPLY,seqid);
return;
} catch (Exception e) {
LOGGER.error("Exception writing to internal frame buffer", e);
}
fb.close();
}
public void onError(Exception e) {
byte msgType = org.apache.thrift.protocol.TMessageType.REPLY;
org.apache.thrift.TBase msg;
unfollowApplication_result result = new unfollowApplication_result();
if (e instanceof tech.aroma.thrift.exceptions.OperationFailedException) {
result.ex1 = (tech.aroma.thrift.exceptions.OperationFailedException) e;
result.setEx1IsSet(true);
msg = result;
}
else if (e instanceof tech.aroma.thrift.exceptions.InvalidArgumentException) {
result.ex2 = (tech.aroma.thrift.exceptions.InvalidArgumentException) e;
result.setEx2IsSet(true);
msg = result;
}
else if (e instanceof tech.aroma.thrift.exceptions.InvalidTokenException) {
result.ex3 = (tech.aroma.thrift.exceptions.InvalidTokenException) e;
result.setEx3IsSet(true);
msg = result;
}
else if (e instanceof tech.aroma.thrift.exceptions.ApplicationDoesNotExistException) {
result.ex4 = (tech.aroma.thrift.exceptions.ApplicationDoesNotExistException) e;
result.setEx4IsSet(true);
msg = result;
}
else if (e instanceof tech.aroma.thrift.exceptions.UnauthorizedException) {
result.ex5 = (tech.aroma.thrift.exceptions.UnauthorizedException) e;
result.setEx5IsSet(true);
msg = result;
}
else
{
msgType = org.apache.thrift.protocol.TMessageType.EXCEPTION;
msg = (org.apache.thrift.TBase)new org.apache.thrift.TApplicationException(org.apache.thrift.TApplicationException.INTERNAL_ERROR, e.getMessage());
}
try {
fcall.sendResponse(fb,msg,msgType,seqid);
return;
} catch (Exception ex) {
LOGGER.error("Exception writing to internal frame buffer", ex);
}
fb.close();
}
};
}
protected boolean isOneway() {
return false;
}
public void start(I iface, unfollowApplication_args args, org.apache.thrift.async.AsyncMethodCallback resultHandler) throws TException {
iface.unfollowApplication(args.request,resultHandler);
}
}
public static class updateApplication extends org.apache.thrift.AsyncProcessFunction {
public updateApplication() {
super("updateApplication");
}
public updateApplication_args getEmptyArgsInstance() {
return new updateApplication_args();
}
public AsyncMethodCallback getResultHandler(final AsyncFrameBuffer fb, final int seqid) {
final org.apache.thrift.AsyncProcessFunction fcall = this;
return new AsyncMethodCallback() {
public void onComplete(UpdateApplicationResponse o) {
updateApplication_result result = new updateApplication_result();
result.success = o;
try {
fcall.sendResponse(fb,result, org.apache.thrift.protocol.TMessageType.REPLY,seqid);
return;
} catch (Exception e) {
LOGGER.error("Exception writing to internal frame buffer", e);
}
fb.close();
}
public void onError(Exception e) {
byte msgType = org.apache.thrift.protocol.TMessageType.REPLY;
org.apache.thrift.TBase msg;
updateApplication_result result = new updateApplication_result();
if (e instanceof tech.aroma.thrift.exceptions.OperationFailedException) {
result.ex1 = (tech.aroma.thrift.exceptions.OperationFailedException) e;
result.setEx1IsSet(true);
msg = result;
}
else if (e instanceof tech.aroma.thrift.exceptions.InvalidArgumentException) {
result.ex2 = (tech.aroma.thrift.exceptions.InvalidArgumentException) e;
result.setEx2IsSet(true);
msg = result;
}
else if (e instanceof tech.aroma.thrift.exceptions.InvalidTokenException) {
result.ex3 = (tech.aroma.thrift.exceptions.InvalidTokenException) e;
result.setEx3IsSet(true);
msg = result;
}
else if (e instanceof tech.aroma.thrift.exceptions.ApplicationDoesNotExistException) {
result.ex4 = (tech.aroma.thrift.exceptions.ApplicationDoesNotExistException) e;
result.setEx4IsSet(true);
msg = result;
}
else if (e instanceof tech.aroma.thrift.exceptions.UnauthorizedException) {
result.ex5 = (tech.aroma.thrift.exceptions.UnauthorizedException) e;
result.setEx5IsSet(true);
msg = result;
}
else
{
msgType = org.apache.thrift.protocol.TMessageType.EXCEPTION;
msg = (org.apache.thrift.TBase)new org.apache.thrift.TApplicationException(org.apache.thrift.TApplicationException.INTERNAL_ERROR, e.getMessage());
}
try {
fcall.sendResponse(fb,msg,msgType,seqid);
return;
} catch (Exception ex) {
LOGGER.error("Exception writing to internal frame buffer", ex);
}
fb.close();
}
};
}
protected boolean isOneway() {
return false;
}
public void start(I iface, updateApplication_args args, org.apache.thrift.async.AsyncMethodCallback resultHandler) throws TException {
iface.updateApplication(args.request,resultHandler);
}
}
public static class updateReactions extends org.apache.thrift.AsyncProcessFunction {
public updateReactions() {
super("updateReactions");
}
public updateReactions_args getEmptyArgsInstance() {
return new updateReactions_args();
}
public AsyncMethodCallback getResultHandler(final AsyncFrameBuffer fb, final int seqid) {
final org.apache.thrift.AsyncProcessFunction fcall = this;
return new AsyncMethodCallback() {
public void onComplete(UpdateReactionsResponse o) {
updateReactions_result result = new updateReactions_result();
result.success = o;
try {
fcall.sendResponse(fb,result, org.apache.thrift.protocol.TMessageType.REPLY,seqid);
return;
} catch (Exception e) {
LOGGER.error("Exception writing to internal frame buffer", e);
}
fb.close();
}
public void onError(Exception e) {
byte msgType = org.apache.thrift.protocol.TMessageType.REPLY;
org.apache.thrift.TBase msg;
updateReactions_result result = new updateReactions_result();
if (e instanceof tech.aroma.thrift.exceptions.OperationFailedException) {
result.ex1 = (tech.aroma.thrift.exceptions.OperationFailedException) e;
result.setEx1IsSet(true);
msg = result;
}
else if (e instanceof tech.aroma.thrift.exceptions.InvalidArgumentException) {
result.ex2 = (tech.aroma.thrift.exceptions.InvalidArgumentException) e;
result.setEx2IsSet(true);
msg = result;
}
else if (e instanceof tech.aroma.thrift.exceptions.InvalidTokenException) {
result.ex3 = (tech.aroma.thrift.exceptions.InvalidTokenException) e;
result.setEx3IsSet(true);
msg = result;
}
else if (e instanceof tech.aroma.thrift.exceptions.ApplicationDoesNotExistException) {
result.ex4 = (tech.aroma.thrift.exceptions.ApplicationDoesNotExistException) e;
result.setEx4IsSet(true);
msg = result;
}
else if (e instanceof tech.aroma.thrift.exceptions.UnauthorizedException) {
result.ex5 = (tech.aroma.thrift.exceptions.UnauthorizedException) e;
result.setEx5IsSet(true);
msg = result;
}
else
{
msgType = org.apache.thrift.protocol.TMessageType.EXCEPTION;
msg = (org.apache.thrift.TBase)new org.apache.thrift.TApplicationException(org.apache.thrift.TApplicationException.INTERNAL_ERROR, e.getMessage());
}
try {
fcall.sendResponse(fb,msg,msgType,seqid);
return;
} catch (Exception ex) {
LOGGER.error("Exception writing to internal frame buffer", ex);
}
fb.close();
}
};
}
protected boolean isOneway() {
return false;
}
public void start(I iface, updateReactions_args args, org.apache.thrift.async.AsyncMethodCallback resultHandler) throws TException {
iface.updateReactions(args.request,resultHandler);
}
}
public static class getActivity extends org.apache.thrift.AsyncProcessFunction {
public getActivity() {
super("getActivity");
}
public getActivity_args getEmptyArgsInstance() {
return new getActivity_args();
}
public AsyncMethodCallback getResultHandler(final AsyncFrameBuffer fb, final int seqid) {
final org.apache.thrift.AsyncProcessFunction fcall = this;
return new AsyncMethodCallback() {
public void onComplete(GetActivityResponse o) {
getActivity_result result = new getActivity_result();
result.success = o;
try {
fcall.sendResponse(fb,result, org.apache.thrift.protocol.TMessageType.REPLY,seqid);
return;
} catch (Exception e) {
LOGGER.error("Exception writing to internal frame buffer", e);
}
fb.close();
}
public void onError(Exception e) {
byte msgType = org.apache.thrift.protocol.TMessageType.REPLY;
org.apache.thrift.TBase msg;
getActivity_result result = new getActivity_result();
if (e instanceof tech.aroma.thrift.exceptions.OperationFailedException) {
result.ex1 = (tech.aroma.thrift.exceptions.OperationFailedException) e;
result.setEx1IsSet(true);
msg = result;
}
else if (e instanceof tech.aroma.thrift.exceptions.InvalidArgumentException) {
result.ex2 = (tech.aroma.thrift.exceptions.InvalidArgumentException) e;
result.setEx2IsSet(true);
msg = result;
}
else if (e instanceof tech.aroma.thrift.exceptions.InvalidTokenException) {
result.ex3 = (tech.aroma.thrift.exceptions.InvalidTokenException) e;
result.setEx3IsSet(true);
msg = result;
}
else
{
msgType = org.apache.thrift.protocol.TMessageType.EXCEPTION;
msg = (org.apache.thrift.TBase)new org.apache.thrift.TApplicationException(org.apache.thrift.TApplicationException.INTERNAL_ERROR, e.getMessage());
}
try {
fcall.sendResponse(fb,msg,msgType,seqid);
return;
} catch (Exception ex) {
LOGGER.error("Exception writing to internal frame buffer", ex);
}
fb.close();
}
};
}
protected boolean isOneway() {
return false;
}
public void start(I iface, getActivity_args args, org.apache.thrift.async.AsyncMethodCallback resultHandler) throws TException {
iface.getActivity(args.request,resultHandler);
}
}
public static class getApplicationInfo extends org.apache.thrift.AsyncProcessFunction {
public getApplicationInfo() {
super("getApplicationInfo");
}
public getApplicationInfo_args getEmptyArgsInstance() {
return new getApplicationInfo_args();
}
public AsyncMethodCallback getResultHandler(final AsyncFrameBuffer fb, final int seqid) {
final org.apache.thrift.AsyncProcessFunction fcall = this;
return new AsyncMethodCallback() {
public void onComplete(GetApplicationInfoResponse o) {
getApplicationInfo_result result = new getApplicationInfo_result();
result.success = o;
try {
fcall.sendResponse(fb,result, org.apache.thrift.protocol.TMessageType.REPLY,seqid);
return;
} catch (Exception e) {
LOGGER.error("Exception writing to internal frame buffer", e);
}
fb.close();
}
public void onError(Exception e) {
byte msgType = org.apache.thrift.protocol.TMessageType.REPLY;
org.apache.thrift.TBase msg;
getApplicationInfo_result result = new getApplicationInfo_result();
if (e instanceof tech.aroma.thrift.exceptions.OperationFailedException) {
result.ex1 = (tech.aroma.thrift.exceptions.OperationFailedException) e;
result.setEx1IsSet(true);
msg = result;
}
else if (e instanceof tech.aroma.thrift.exceptions.InvalidArgumentException) {
result.ex2 = (tech.aroma.thrift.exceptions.InvalidArgumentException) e;
result.setEx2IsSet(true);
msg = result;
}
else if (e instanceof tech.aroma.thrift.exceptions.InvalidTokenException) {
result.ex3 = (tech.aroma.thrift.exceptions.InvalidTokenException) e;
result.setEx3IsSet(true);
msg = result;
}
else if (e instanceof tech.aroma.thrift.exceptions.ApplicationDoesNotExistException) {
result.ex4 = (tech.aroma.thrift.exceptions.ApplicationDoesNotExistException) e;
result.setEx4IsSet(true);
msg = result;
}
else if (e instanceof tech.aroma.thrift.exceptions.UnauthorizedException) {
result.ex5 = (tech.aroma.thrift.exceptions.UnauthorizedException) e;
result.setEx5IsSet(true);
msg = result;
}
else
{
msgType = org.apache.thrift.protocol.TMessageType.EXCEPTION;
msg = (org.apache.thrift.TBase)new org.apache.thrift.TApplicationException(org.apache.thrift.TApplicationException.INTERNAL_ERROR, e.getMessage());
}
try {
fcall.sendResponse(fb,msg,msgType,seqid);
return;
} catch (Exception ex) {
LOGGER.error("Exception writing to internal frame buffer", ex);
}
fb.close();
}
};
}
protected boolean isOneway() {
return false;
}
public void start(I iface, getApplicationInfo_args args, org.apache.thrift.async.AsyncMethodCallback resultHandler) throws TException {
iface.getApplicationInfo(args.request,resultHandler);
}
}
public static class getBuzz extends org.apache.thrift.AsyncProcessFunction {
public getBuzz() {
super("getBuzz");
}
public getBuzz_args getEmptyArgsInstance() {
return new getBuzz_args();
}
public AsyncMethodCallback getResultHandler(final AsyncFrameBuffer fb, final int seqid) {
final org.apache.thrift.AsyncProcessFunction fcall = this;
return new AsyncMethodCallback() {
public void onComplete(GetBuzzResponse o) {
getBuzz_result result = new getBuzz_result();
result.success = o;
try {
fcall.sendResponse(fb,result, org.apache.thrift.protocol.TMessageType.REPLY,seqid);
return;
} catch (Exception e) {
LOGGER.error("Exception writing to internal frame buffer", e);
}
fb.close();
}
public void onError(Exception e) {
byte msgType = org.apache.thrift.protocol.TMessageType.REPLY;
org.apache.thrift.TBase msg;
getBuzz_result result = new getBuzz_result();
if (e instanceof tech.aroma.thrift.exceptions.OperationFailedException) {
result.ex1 = (tech.aroma.thrift.exceptions.OperationFailedException) e;
result.setEx1IsSet(true);
msg = result;
}
else if (e instanceof tech.aroma.thrift.exceptions.InvalidArgumentException) {
result.ex2 = (tech.aroma.thrift.exceptions.InvalidArgumentException) e;
result.setEx2IsSet(true);
msg = result;
}
else if (e instanceof tech.aroma.thrift.exceptions.InvalidTokenException) {
result.ex3 = (tech.aroma.thrift.exceptions.InvalidTokenException) e;
result.setEx3IsSet(true);
msg = result;
}
else if (e instanceof tech.aroma.thrift.exceptions.ApplicationDoesNotExistException) {
result.ex4 = (tech.aroma.thrift.exceptions.ApplicationDoesNotExistException) e;
result.setEx4IsSet(true);
msg = result;
}
else if (e instanceof tech.aroma.thrift.exceptions.UnauthorizedException) {
result.ex5 = (tech.aroma.thrift.exceptions.UnauthorizedException) e;
result.setEx5IsSet(true);
msg = result;
}
else
{
msgType = org.apache.thrift.protocol.TMessageType.EXCEPTION;
msg = (org.apache.thrift.TBase)new org.apache.thrift.TApplicationException(org.apache.thrift.TApplicationException.INTERNAL_ERROR, e.getMessage());
}
try {
fcall.sendResponse(fb,msg,msgType,seqid);
return;
} catch (Exception ex) {
LOGGER.error("Exception writing to internal frame buffer", ex);
}
fb.close();
}
};
}
protected boolean isOneway() {
return false;
}
public void start(I iface, getBuzz_args args, org.apache.thrift.async.AsyncMethodCallback resultHandler) throws TException {
iface.getBuzz(args.request,resultHandler);
}
}
public static class getDashboard extends org.apache.thrift.AsyncProcessFunction {
public getDashboard() {
super("getDashboard");
}
public getDashboard_args getEmptyArgsInstance() {
return new getDashboard_args();
}
public AsyncMethodCallback getResultHandler(final AsyncFrameBuffer fb, final int seqid) {
final org.apache.thrift.AsyncProcessFunction fcall = this;
return new AsyncMethodCallback() {
public void onComplete(GetDashboardResponse o) {
getDashboard_result result = new getDashboard_result();
result.success = o;
try {
fcall.sendResponse(fb,result, org.apache.thrift.protocol.TMessageType.REPLY,seqid);
return;
} catch (Exception e) {
LOGGER.error("Exception writing to internal frame buffer", e);
}
fb.close();
}
public void onError(Exception e) {
byte msgType = org.apache.thrift.protocol.TMessageType.REPLY;
org.apache.thrift.TBase msg;
getDashboard_result result = new getDashboard_result();
if (e instanceof tech.aroma.thrift.exceptions.OperationFailedException) {
result.ex1 = (tech.aroma.thrift.exceptions.OperationFailedException) e;
result.setEx1IsSet(true);
msg = result;
}
else if (e instanceof tech.aroma.thrift.exceptions.InvalidArgumentException) {
result.ex2 = (tech.aroma.thrift.exceptions.InvalidArgumentException) e;
result.setEx2IsSet(true);
msg = result;
}
else if (e instanceof tech.aroma.thrift.exceptions.InvalidTokenException) {
result.ex3 = (tech.aroma.thrift.exceptions.InvalidTokenException) e;
result.setEx3IsSet(true);
msg = result;
}
else
{
msgType = org.apache.thrift.protocol.TMessageType.EXCEPTION;
msg = (org.apache.thrift.TBase)new org.apache.thrift.TApplicationException(org.apache.thrift.TApplicationException.INTERNAL_ERROR, e.getMessage());
}
try {
fcall.sendResponse(fb,msg,msgType,seqid);
return;
} catch (Exception ex) {
LOGGER.error("Exception writing to internal frame buffer", ex);
}
fb.close();
}
};
}
protected boolean isOneway() {
return false;
}
public void start(I iface, getDashboard_args args, org.apache.thrift.async.AsyncMethodCallback resultHandler) throws TException {
iface.getDashboard(args.request,resultHandler);
}
}
public static class getApplicationMessages extends org.apache.thrift.AsyncProcessFunction {
public getApplicationMessages() {
super("getApplicationMessages");
}
public getApplicationMessages_args getEmptyArgsInstance() {
return new getApplicationMessages_args();
}
public AsyncMethodCallback getResultHandler(final AsyncFrameBuffer fb, final int seqid) {
final org.apache.thrift.AsyncProcessFunction fcall = this;
return new AsyncMethodCallback() {
public void onComplete(GetApplicationMessagesResponse o) {
getApplicationMessages_result result = new getApplicationMessages_result();
result.success = o;
try {
fcall.sendResponse(fb,result, org.apache.thrift.protocol.TMessageType.REPLY,seqid);
return;
} catch (Exception e) {
LOGGER.error("Exception writing to internal frame buffer", e);
}
fb.close();
}
public void onError(Exception e) {
byte msgType = org.apache.thrift.protocol.TMessageType.REPLY;
org.apache.thrift.TBase msg;
getApplicationMessages_result result = new getApplicationMessages_result();
if (e instanceof tech.aroma.thrift.exceptions.OperationFailedException) {
result.ex1 = (tech.aroma.thrift.exceptions.OperationFailedException) e;
result.setEx1IsSet(true);
msg = result;
}
else if (e instanceof tech.aroma.thrift.exceptions.InvalidArgumentException) {
result.ex2 = (tech.aroma.thrift.exceptions.InvalidArgumentException) e;
result.setEx2IsSet(true);
msg = result;
}
else if (e instanceof tech.aroma.thrift.exceptions.InvalidTokenException) {
result.ex3 = (tech.aroma.thrift.exceptions.InvalidTokenException) e;
result.setEx3IsSet(true);
msg = result;
}
else if (e instanceof tech.aroma.thrift.exceptions.UnauthorizedException) {
result.ex4 = (tech.aroma.thrift.exceptions.UnauthorizedException) e;
result.setEx4IsSet(true);
msg = result;
}
else if (e instanceof tech.aroma.thrift.exceptions.ApplicationDoesNotExistException) {
result.ex5 = (tech.aroma.thrift.exceptions.ApplicationDoesNotExistException) e;
result.setEx5IsSet(true);
msg = result;
}
else
{
msgType = org.apache.thrift.protocol.TMessageType.EXCEPTION;
msg = (org.apache.thrift.TBase)new org.apache.thrift.TApplicationException(org.apache.thrift.TApplicationException.INTERNAL_ERROR, e.getMessage());
}
try {
fcall.sendResponse(fb,msg,msgType,seqid);
return;
} catch (Exception ex) {
LOGGER.error("Exception writing to internal frame buffer", ex);
}
fb.close();
}
};
}
protected boolean isOneway() {
return false;
}
public void start(I iface, getApplicationMessages_args args, org.apache.thrift.async.AsyncMethodCallback resultHandler) throws TException {
iface.getApplicationMessages(args.request,resultHandler);
}
}
public static class getInbox extends org.apache.thrift.AsyncProcessFunction {
public getInbox() {
super("getInbox");
}
public getInbox_args getEmptyArgsInstance() {
return new getInbox_args();
}
public AsyncMethodCallback getResultHandler(final AsyncFrameBuffer fb, final int seqid) {
final org.apache.thrift.AsyncProcessFunction fcall = this;
return new AsyncMethodCallback() {
public void onComplete(GetInboxResponse o) {
getInbox_result result = new getInbox_result();
result.success = o;
try {
fcall.sendResponse(fb,result, org.apache.thrift.protocol.TMessageType.REPLY,seqid);
return;
} catch (Exception e) {
LOGGER.error("Exception writing to internal frame buffer", e);
}
fb.close();
}
public void onError(Exception e) {
byte msgType = org.apache.thrift.protocol.TMessageType.REPLY;
org.apache.thrift.TBase msg;
getInbox_result result = new getInbox_result();
if (e instanceof tech.aroma.thrift.exceptions.OperationFailedException) {
result.ex1 = (tech.aroma.thrift.exceptions.OperationFailedException) e;
result.setEx1IsSet(true);
msg = result;
}
else if (e instanceof tech.aroma.thrift.exceptions.InvalidArgumentException) {
result.ex2 = (tech.aroma.thrift.exceptions.InvalidArgumentException) e;
result.setEx2IsSet(true);
msg = result;
}
else if (e instanceof tech.aroma.thrift.exceptions.InvalidTokenException) {
result.ex3 = (tech.aroma.thrift.exceptions.InvalidTokenException) e;
result.setEx3IsSet(true);
msg = result;
}
else
{
msgType = org.apache.thrift.protocol.TMessageType.EXCEPTION;
msg = (org.apache.thrift.TBase)new org.apache.thrift.TApplicationException(org.apache.thrift.TApplicationException.INTERNAL_ERROR, e.getMessage());
}
try {
fcall.sendResponse(fb,msg,msgType,seqid);
return;
} catch (Exception ex) {
LOGGER.error("Exception writing to internal frame buffer", ex);
}
fb.close();
}
};
}
protected boolean isOneway() {
return false;
}
public void start(I iface, getInbox_args args, org.apache.thrift.async.AsyncMethodCallback resultHandler) throws TException {
iface.getInbox(args.request,resultHandler);
}
}
public static class getFullMessage extends org.apache.thrift.AsyncProcessFunction {
public getFullMessage() {
super("getFullMessage");
}
public getFullMessage_args getEmptyArgsInstance() {
return new getFullMessage_args();
}
public AsyncMethodCallback getResultHandler(final AsyncFrameBuffer fb, final int seqid) {
final org.apache.thrift.AsyncProcessFunction fcall = this;
return new AsyncMethodCallback() {
public void onComplete(GetFullMessageResponse o) {
getFullMessage_result result = new getFullMessage_result();
result.success = o;
try {
fcall.sendResponse(fb,result, org.apache.thrift.protocol.TMessageType.REPLY,seqid);
return;
} catch (Exception e) {
LOGGER.error("Exception writing to internal frame buffer", e);
}
fb.close();
}
public void onError(Exception e) {
byte msgType = org.apache.thrift.protocol.TMessageType.REPLY;
org.apache.thrift.TBase msg;
getFullMessage_result result = new getFullMessage_result();
if (e instanceof tech.aroma.thrift.exceptions.OperationFailedException) {
result.ex1 = (tech.aroma.thrift.exceptions.OperationFailedException) e;
result.setEx1IsSet(true);
msg = result;
}
else if (e instanceof tech.aroma.thrift.exceptions.InvalidArgumentException) {
result.ex2 = (tech.aroma.thrift.exceptions.InvalidArgumentException) e;
result.setEx2IsSet(true);
msg = result;
}
else if (e instanceof tech.aroma.thrift.exceptions.InvalidTokenException) {
result.ex3 = (tech.aroma.thrift.exceptions.InvalidTokenException) e;
result.setEx3IsSet(true);
msg = result;
}
else
{
msgType = org.apache.thrift.protocol.TMessageType.EXCEPTION;
msg = (org.apache.thrift.TBase)new org.apache.thrift.TApplicationException(org.apache.thrift.TApplicationException.INTERNAL_ERROR, e.getMessage());
}
try {
fcall.sendResponse(fb,msg,msgType,seqid);
return;
} catch (Exception ex) {
LOGGER.error("Exception writing to internal frame buffer", ex);
}
fb.close();
}
};
}
protected boolean isOneway() {
return false;
}
public void start(I iface, getFullMessage_args args, org.apache.thrift.async.AsyncMethodCallback resultHandler) throws TException {
iface.getFullMessage(args.request,resultHandler);
}
}
public static class getMedia extends org.apache.thrift.AsyncProcessFunction {
public getMedia() {
super("getMedia");
}
public getMedia_args getEmptyArgsInstance() {
return new getMedia_args();
}
public AsyncMethodCallback getResultHandler(final AsyncFrameBuffer fb, final int seqid) {
final org.apache.thrift.AsyncProcessFunction fcall = this;
return new AsyncMethodCallback() {
public void onComplete(GetMediaResponse o) {
getMedia_result result = new getMedia_result();
result.success = o;
try {
fcall.sendResponse(fb,result, org.apache.thrift.protocol.TMessageType.REPLY,seqid);
return;
} catch (Exception e) {
LOGGER.error("Exception writing to internal frame buffer", e);
}
fb.close();
}
public void onError(Exception e) {
byte msgType = org.apache.thrift.protocol.TMessageType.REPLY;
org.apache.thrift.TBase msg;
getMedia_result result = new getMedia_result();
if (e instanceof tech.aroma.thrift.exceptions.OperationFailedException) {
result.ex1 = (tech.aroma.thrift.exceptions.OperationFailedException) e;
result.setEx1IsSet(true);
msg = result;
}
else if (e instanceof tech.aroma.thrift.exceptions.InvalidArgumentException) {
result.ex2 = (tech.aroma.thrift.exceptions.InvalidArgumentException) e;
result.setEx2IsSet(true);
msg = result;
}
else if (e instanceof tech.aroma.thrift.exceptions.InvalidTokenException) {
result.ex3 = (tech.aroma.thrift.exceptions.InvalidTokenException) e;
result.setEx3IsSet(true);
msg = result;
}
else if (e instanceof tech.aroma.thrift.exceptions.DoesNotExistException) {
result.ex4 = (tech.aroma.thrift.exceptions.DoesNotExistException) e;
result.setEx4IsSet(true);
msg = result;
}
else if (e instanceof tech.aroma.thrift.exceptions.UnauthorizedException) {
result.ex5 = (tech.aroma.thrift.exceptions.UnauthorizedException) e;
result.setEx5IsSet(true);
msg = result;
}
else
{
msgType = org.apache.thrift.protocol.TMessageType.EXCEPTION;
msg = (org.apache.thrift.TBase)new org.apache.thrift.TApplicationException(org.apache.thrift.TApplicationException.INTERNAL_ERROR, e.getMessage());
}
try {
fcall.sendResponse(fb,msg,msgType,seqid);
return;
} catch (Exception ex) {
LOGGER.error("Exception writing to internal frame buffer", ex);
}
fb.close();
}
};
}
protected boolean isOneway() {
return false;
}
public void start(I iface, getMedia_args args, org.apache.thrift.async.AsyncMethodCallback resultHandler) throws TException {
iface.getMedia(args.request,resultHandler);
}
}
public static class getApplicationsOwnedBy extends org.apache.thrift.AsyncProcessFunction {
public getApplicationsOwnedBy() {
super("getApplicationsOwnedBy");
}
public getApplicationsOwnedBy_args getEmptyArgsInstance() {
return new getApplicationsOwnedBy_args();
}
public AsyncMethodCallback getResultHandler(final AsyncFrameBuffer fb, final int seqid) {
final org.apache.thrift.AsyncProcessFunction fcall = this;
return new AsyncMethodCallback() {
public void onComplete(GetApplicationsOwnedByResponse o) {
getApplicationsOwnedBy_result result = new getApplicationsOwnedBy_result();
result.success = o;
try {
fcall.sendResponse(fb,result, org.apache.thrift.protocol.TMessageType.REPLY,seqid);
return;
} catch (Exception e) {
LOGGER.error("Exception writing to internal frame buffer", e);
}
fb.close();
}
public void onError(Exception e) {
byte msgType = org.apache.thrift.protocol.TMessageType.REPLY;
org.apache.thrift.TBase msg;
getApplicationsOwnedBy_result result = new getApplicationsOwnedBy_result();
if (e instanceof tech.aroma.thrift.exceptions.OperationFailedException) {
result.ex1 = (tech.aroma.thrift.exceptions.OperationFailedException) e;
result.setEx1IsSet(true);
msg = result;
}
else if (e instanceof tech.aroma.thrift.exceptions.InvalidArgumentException) {
result.ex2 = (tech.aroma.thrift.exceptions.InvalidArgumentException) e;
result.setEx2IsSet(true);
msg = result;
}
else if (e instanceof tech.aroma.thrift.exceptions.InvalidTokenException) {
result.ex3 = (tech.aroma.thrift.exceptions.InvalidTokenException) e;
result.setEx3IsSet(true);
msg = result;
}
else
{
msgType = org.apache.thrift.protocol.TMessageType.EXCEPTION;
msg = (org.apache.thrift.TBase)new org.apache.thrift.TApplicationException(org.apache.thrift.TApplicationException.INTERNAL_ERROR, e.getMessage());
}
try {
fcall.sendResponse(fb,msg,msgType,seqid);
return;
} catch (Exception ex) {
LOGGER.error("Exception writing to internal frame buffer", ex);
}
fb.close();
}
};
}
protected boolean isOneway() {
return false;
}
public void start(I iface, getApplicationsOwnedBy_args args, org.apache.thrift.async.AsyncMethodCallback resultHandler) throws TException {
iface.getApplicationsOwnedBy(args.request,resultHandler);
}
}
public static class getApplicationsFollowedBy extends org.apache.thrift.AsyncProcessFunction {
public getApplicationsFollowedBy() {
super("getApplicationsFollowedBy");
}
public getApplicationsFollowedBy_args getEmptyArgsInstance() {
return new getApplicationsFollowedBy_args();
}
public AsyncMethodCallback getResultHandler(final AsyncFrameBuffer fb, final int seqid) {
final org.apache.thrift.AsyncProcessFunction fcall = this;
return new AsyncMethodCallback() {
public void onComplete(GetApplicationsFollowedByResponse o) {
getApplicationsFollowedBy_result result = new getApplicationsFollowedBy_result();
result.success = o;
try {
fcall.sendResponse(fb,result, org.apache.thrift.protocol.TMessageType.REPLY,seqid);
return;
} catch (Exception e) {
LOGGER.error("Exception writing to internal frame buffer", e);
}
fb.close();
}
public void onError(Exception e) {
byte msgType = org.apache.thrift.protocol.TMessageType.REPLY;
org.apache.thrift.TBase msg;
getApplicationsFollowedBy_result result = new getApplicationsFollowedBy_result();
if (e instanceof tech.aroma.thrift.exceptions.OperationFailedException) {
result.ex1 = (tech.aroma.thrift.exceptions.OperationFailedException) e;
result.setEx1IsSet(true);
msg = result;
}
else if (e instanceof tech.aroma.thrift.exceptions.InvalidArgumentException) {
result.ex2 = (tech.aroma.thrift.exceptions.InvalidArgumentException) e;
result.setEx2IsSet(true);
msg = result;
}
else if (e instanceof tech.aroma.thrift.exceptions.InvalidTokenException) {
result.ex3 = (tech.aroma.thrift.exceptions.InvalidTokenException) e;
result.setEx3IsSet(true);
msg = result;
}
else
{
msgType = org.apache.thrift.protocol.TMessageType.EXCEPTION;
msg = (org.apache.thrift.TBase)new org.apache.thrift.TApplicationException(org.apache.thrift.TApplicationException.INTERNAL_ERROR, e.getMessage());
}
try {
fcall.sendResponse(fb,msg,msgType,seqid);
return;
} catch (Exception ex) {
LOGGER.error("Exception writing to internal frame buffer", ex);
}
fb.close();
}
};
}
protected boolean isOneway() {
return false;
}
public void start(I iface, getApplicationsFollowedBy_args args, org.apache.thrift.async.AsyncMethodCallback resultHandler) throws TException {
iface.getApplicationsFollowedBy(args.request,resultHandler);
}
}
public static class getMySavedChannels extends org.apache.thrift.AsyncProcessFunction {
public getMySavedChannels() {
super("getMySavedChannels");
}
public getMySavedChannels_args getEmptyArgsInstance() {
return new getMySavedChannels_args();
}
public AsyncMethodCallback getResultHandler(final AsyncFrameBuffer fb, final int seqid) {
final org.apache.thrift.AsyncProcessFunction fcall = this;
return new AsyncMethodCallback() {
public void onComplete(GetMySavedChannelsResponse o) {
getMySavedChannels_result result = new getMySavedChannels_result();
result.success = o;
try {
fcall.sendResponse(fb,result, org.apache.thrift.protocol.TMessageType.REPLY,seqid);
return;
} catch (Exception e) {
LOGGER.error("Exception writing to internal frame buffer", e);
}
fb.close();
}
public void onError(Exception e) {
byte msgType = org.apache.thrift.protocol.TMessageType.REPLY;
org.apache.thrift.TBase msg;
getMySavedChannels_result result = new getMySavedChannels_result();
if (e instanceof tech.aroma.thrift.exceptions.OperationFailedException) {
result.ex1 = (tech.aroma.thrift.exceptions.OperationFailedException) e;
result.setEx1IsSet(true);
msg = result;
}
else if (e instanceof tech.aroma.thrift.exceptions.InvalidArgumentException) {
result.ex2 = (tech.aroma.thrift.exceptions.InvalidArgumentException) e;
result.setEx2IsSet(true);
msg = result;
}
else if (e instanceof tech.aroma.thrift.exceptions.InvalidTokenException) {
result.ex3 = (tech.aroma.thrift.exceptions.InvalidTokenException) e;
result.setEx3IsSet(true);
msg = result;
}
else
{
msgType = org.apache.thrift.protocol.TMessageType.EXCEPTION;
msg = (org.apache.thrift.TBase)new org.apache.thrift.TApplicationException(org.apache.thrift.TApplicationException.INTERNAL_ERROR, e.getMessage());
}
try {
fcall.sendResponse(fb,msg,msgType,seqid);
return;
} catch (Exception ex) {
LOGGER.error("Exception writing to internal frame buffer", ex);
}
fb.close();
}
};
}
protected boolean isOneway() {
return false;
}
public void start(I iface, getMySavedChannels_args args, org.apache.thrift.async.AsyncMethodCallback resultHandler) throws TException {
iface.getMySavedChannels(args.request,resultHandler);
}
}
public static class getReactions extends org.apache.thrift.AsyncProcessFunction {
public getReactions() {
super("getReactions");
}
public getReactions_args getEmptyArgsInstance() {
return new getReactions_args();
}
public AsyncMethodCallback getResultHandler(final AsyncFrameBuffer fb, final int seqid) {
final org.apache.thrift.AsyncProcessFunction fcall = this;
return new AsyncMethodCallback() {
public void onComplete(GetReactionsResponse o) {
getReactions_result result = new getReactions_result();
result.success = o;
try {
fcall.sendResponse(fb,result, org.apache.thrift.protocol.TMessageType.REPLY,seqid);
return;
} catch (Exception e) {
LOGGER.error("Exception writing to internal frame buffer", e);
}
fb.close();
}
public void onError(Exception e) {
byte msgType = org.apache.thrift.protocol.TMessageType.REPLY;
org.apache.thrift.TBase msg;
getReactions_result result = new getReactions_result();
if (e instanceof tech.aroma.thrift.exceptions.OperationFailedException) {
result.ex1 = (tech.aroma.thrift.exceptions.OperationFailedException) e;
result.setEx1IsSet(true);
msg = result;
}
else if (e instanceof tech.aroma.thrift.exceptions.InvalidArgumentException) {
result.ex2 = (tech.aroma.thrift.exceptions.InvalidArgumentException) e;
result.setEx2IsSet(true);
msg = result;
}
else if (e instanceof tech.aroma.thrift.exceptions.InvalidTokenException) {
result.ex3 = (tech.aroma.thrift.exceptions.InvalidTokenException) e;
result.setEx3IsSet(true);
msg = result;
}
else if (e instanceof tech.aroma.thrift.exceptions.ApplicationDoesNotExistException) {
result.ex4 = (tech.aroma.thrift.exceptions.ApplicationDoesNotExistException) e;
result.setEx4IsSet(true);
msg = result;
}
else if (e instanceof tech.aroma.thrift.exceptions.UnauthorizedException) {
result.ex5 = (tech.aroma.thrift.exceptions.UnauthorizedException) e;
result.setEx5IsSet(true);
msg = result;
}
else
{
msgType = org.apache.thrift.protocol.TMessageType.EXCEPTION;
msg = (org.apache.thrift.TBase)new org.apache.thrift.TApplicationException(org.apache.thrift.TApplicationException.INTERNAL_ERROR, e.getMessage());
}
try {
fcall.sendResponse(fb,msg,msgType,seqid);
return;
} catch (Exception ex) {
LOGGER.error("Exception writing to internal frame buffer", ex);
}
fb.close();
}
};
}
protected boolean isOneway() {
return false;
}
public void start(I iface, getReactions_args args, org.apache.thrift.async.AsyncMethodCallback resultHandler) throws TException {
iface.getReactions(args.request,resultHandler);
}
}
public static class getUserInfo extends org.apache.thrift.AsyncProcessFunction {
public getUserInfo() {
super("getUserInfo");
}
public getUserInfo_args getEmptyArgsInstance() {
return new getUserInfo_args();
}
public AsyncMethodCallback getResultHandler(final AsyncFrameBuffer fb, final int seqid) {
final org.apache.thrift.AsyncProcessFunction fcall = this;
return new AsyncMethodCallback() {
public void onComplete(GetUserInfoResponse o) {
getUserInfo_result result = new getUserInfo_result();
result.success = o;
try {
fcall.sendResponse(fb,result, org.apache.thrift.protocol.TMessageType.REPLY,seqid);
return;
} catch (Exception e) {
LOGGER.error("Exception writing to internal frame buffer", e);
}
fb.close();
}
public void onError(Exception e) {
byte msgType = org.apache.thrift.protocol.TMessageType.REPLY;
org.apache.thrift.TBase msg;
getUserInfo_result result = new getUserInfo_result();
if (e instanceof tech.aroma.thrift.exceptions.OperationFailedException) {
result.ex1 = (tech.aroma.thrift.exceptions.OperationFailedException) e;
result.setEx1IsSet(true);
msg = result;
}
else if (e instanceof tech.aroma.thrift.exceptions.InvalidArgumentException) {
result.ex2 = (tech.aroma.thrift.exceptions.InvalidArgumentException) e;
result.setEx2IsSet(true);
msg = result;
}
else if (e instanceof tech.aroma.thrift.exceptions.InvalidTokenException) {
result.ex3 = (tech.aroma.thrift.exceptions.InvalidTokenException) e;
result.setEx3IsSet(true);
msg = result;
}
else if (e instanceof tech.aroma.thrift.exceptions.UnauthorizedException) {
result.ex4 = (tech.aroma.thrift.exceptions.UnauthorizedException) e;
result.setEx4IsSet(true);
msg = result;
}
else if (e instanceof tech.aroma.thrift.exceptions.UserDoesNotExistException) {
result.ex5 = (tech.aroma.thrift.exceptions.UserDoesNotExistException) e;
result.setEx5IsSet(true);
msg = result;
}
else
{
msgType = org.apache.thrift.protocol.TMessageType.EXCEPTION;
msg = (org.apache.thrift.TBase)new org.apache.thrift.TApplicationException(org.apache.thrift.TApplicationException.INTERNAL_ERROR, e.getMessage());
}
try {
fcall.sendResponse(fb,msg,msgType,seqid);
return;
} catch (Exception ex) {
LOGGER.error("Exception writing to internal frame buffer", ex);
}
fb.close();
}
};
}
protected boolean isOneway() {
return false;
}
public void start(I iface, getUserInfo_args args, org.apache.thrift.async.AsyncMethodCallback resultHandler) throws TException {
iface.getUserInfo(args.request,resultHandler);
}
}
public static class searchForApplications extends org.apache.thrift.AsyncProcessFunction {
public searchForApplications() {
super("searchForApplications");
}
public searchForApplications_args getEmptyArgsInstance() {
return new searchForApplications_args();
}
public AsyncMethodCallback getResultHandler(final AsyncFrameBuffer fb, final int seqid) {
final org.apache.thrift.AsyncProcessFunction fcall = this;
return new AsyncMethodCallback() {
public void onComplete(SearchForApplicationsResponse o) {
searchForApplications_result result = new searchForApplications_result();
result.success = o;
try {
fcall.sendResponse(fb,result, org.apache.thrift.protocol.TMessageType.REPLY,seqid);
return;
} catch (Exception e) {
LOGGER.error("Exception writing to internal frame buffer", e);
}
fb.close();
}
public void onError(Exception e) {
byte msgType = org.apache.thrift.protocol.TMessageType.REPLY;
org.apache.thrift.TBase msg;
searchForApplications_result result = new searchForApplications_result();
if (e instanceof tech.aroma.thrift.exceptions.OperationFailedException) {
result.ex1 = (tech.aroma.thrift.exceptions.OperationFailedException) e;
result.setEx1IsSet(true);
msg = result;
}
else if (e instanceof tech.aroma.thrift.exceptions.InvalidArgumentException) {
result.ex2 = (tech.aroma.thrift.exceptions.InvalidArgumentException) e;
result.setEx2IsSet(true);
msg = result;
}
else if (e instanceof tech.aroma.thrift.exceptions.InvalidTokenException) {
result.ex3 = (tech.aroma.thrift.exceptions.InvalidTokenException) e;
result.setEx3IsSet(true);
msg = result;
}
else if (e instanceof tech.aroma.thrift.exceptions.UnauthorizedException) {
result.ex4 = (tech.aroma.thrift.exceptions.UnauthorizedException) e;
result.setEx4IsSet(true);
msg = result;
}
else
{
msgType = org.apache.thrift.protocol.TMessageType.EXCEPTION;
msg = (org.apache.thrift.TBase)new org.apache.thrift.TApplicationException(org.apache.thrift.TApplicationException.INTERNAL_ERROR, e.getMessage());
}
try {
fcall.sendResponse(fb,msg,msgType,seqid);
return;
} catch (Exception ex) {
LOGGER.error("Exception writing to internal frame buffer", ex);
}
fb.close();
}
};
}
protected boolean isOneway() {
return false;
}
public void start(I iface, searchForApplications_args args, org.apache.thrift.async.AsyncMethodCallback resultHandler) throws TException {
iface.searchForApplications(args.request,resultHandler);
}
}
}
public static class getApiVersion_args implements org.apache.thrift.TBase, java.io.Serializable, Cloneable, Comparable {
private static final org.apache.thrift.protocol.TStruct STRUCT_DESC = new org.apache.thrift.protocol.TStruct("getApiVersion_args");
private static final Map, SchemeFactory> schemes = new HashMap, SchemeFactory>();
static {
schemes.put(StandardScheme.class, new getApiVersion_argsStandardSchemeFactory());
schemes.put(TupleScheme.class, new getApiVersion_argsTupleSchemeFactory());
}
/** The set of fields this struct contains, along with convenience methods for finding and manipulating them. */
public enum _Fields implements org.apache.thrift.TFieldIdEnum {
;
private static final Map byName = new HashMap();
static {
for (_Fields field : EnumSet.allOf(_Fields.class)) {
byName.put(field.getFieldName(), field);
}
}
/**
* Find the _Fields constant that matches fieldId, or null if its not found.
*/
public static _Fields findByThriftId(int fieldId) {
switch(fieldId) {
default:
return null;
}
}
/**
* Find the _Fields constant that matches fieldId, throwing an exception
* if it is not found.
*/
public static _Fields findByThriftIdOrThrow(int fieldId) {
_Fields fields = findByThriftId(fieldId);
if (fields == null) throw new IllegalArgumentException("Field " + fieldId + " doesn't exist!");
return fields;
}
/**
* Find the _Fields constant that matches name, or null if its not found.
*/
public static _Fields findByName(String name) {
return byName.get(name);
}
private final short _thriftId;
private final String _fieldName;
_Fields(short thriftId, String fieldName) {
_thriftId = thriftId;
_fieldName = fieldName;
}
public short getThriftFieldId() {
return _thriftId;
}
public String getFieldName() {
return _fieldName;
}
}
public static final Map<_Fields, org.apache.thrift.meta_data.FieldMetaData> metaDataMap;
static {
Map<_Fields, org.apache.thrift.meta_data.FieldMetaData> tmpMap = new EnumMap<_Fields, org.apache.thrift.meta_data.FieldMetaData>(_Fields.class);
metaDataMap = Collections.unmodifiableMap(tmpMap);
org.apache.thrift.meta_data.FieldMetaData.addStructMetaDataMap(getApiVersion_args.class, metaDataMap);
}
public getApiVersion_args() {
}
/**
* Performs a deep copy on other.
*/
public getApiVersion_args(getApiVersion_args other) {
}
public getApiVersion_args deepCopy() {
return new getApiVersion_args(this);
}
@Override
public void clear() {
}
public void setFieldValue(_Fields field, Object value) {
switch (field) {
}
}
public Object getFieldValue(_Fields field) {
switch (field) {
}
throw new IllegalStateException();
}
/** Returns true if field corresponding to fieldID is set (has been assigned a value) and false otherwise */
public boolean isSet(_Fields field) {
if (field == null) {
throw new IllegalArgumentException();
}
switch (field) {
}
throw new IllegalStateException();
}
@Override
public boolean equals(Object that) {
if (that == null)
return false;
if (that instanceof getApiVersion_args)
return this.equals((getApiVersion_args)that);
return false;
}
public boolean equals(getApiVersion_args that) {
if (that == null)
return false;
return true;
}
@Override
public int hashCode() {
List