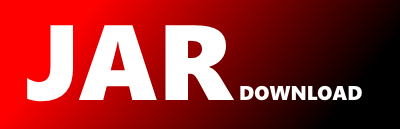
tech.aroma.thrift.service.DeleteMessageRequest Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aroma-thrift Show documentation
Show all versions of aroma-thrift Show documentation
Part of the Aroma Project.
This project contains the Service and Model Definitions.
From this the Server and Client interfaces are generated for the Aroma Service.
/**
* Autogenerated by Thrift Compiler (0.9.3)
*
* DO NOT EDIT UNLESS YOU ARE SURE THAT YOU KNOW WHAT YOU ARE DOING
* @generated
*/
package tech.aroma.thrift.service;
import org.apache.thrift.scheme.IScheme;
import org.apache.thrift.scheme.SchemeFactory;
import org.apache.thrift.scheme.StandardScheme;
import org.apache.thrift.scheme.TupleScheme;
import org.apache.thrift.protocol.TTupleProtocol;
import org.apache.thrift.protocol.TProtocolException;
import org.apache.thrift.EncodingUtils;
import org.apache.thrift.TException;
import org.apache.thrift.async.AsyncMethodCallback;
import org.apache.thrift.server.AbstractNonblockingServer.*;
import java.util.List;
import java.util.ArrayList;
import java.util.Map;
import java.util.HashMap;
import java.util.EnumMap;
import java.util.Set;
import java.util.HashSet;
import java.util.EnumSet;
import java.util.Collections;
import java.util.BitSet;
import java.nio.ByteBuffer;
import java.util.Arrays;
import javax.annotation.Generated;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
@SuppressWarnings({"cast", "rawtypes", "serial", "unchecked"})
/**
* Deletes a Message.
*
* #owner
*/
@Generated(value = "Autogenerated by Thrift Compiler (0.9.3)", date = "2016-05-10")
public class DeleteMessageRequest implements org.apache.thrift.TBase, java.io.Serializable, Cloneable, Comparable {
private static final org.apache.thrift.protocol.TStruct STRUCT_DESC = new org.apache.thrift.protocol.TStruct("DeleteMessageRequest");
private static final org.apache.thrift.protocol.TField TOKEN_FIELD_DESC = new org.apache.thrift.protocol.TField("token", org.apache.thrift.protocol.TType.STRUCT, (short)1);
private static final org.apache.thrift.protocol.TField MESSAGE_ID_FIELD_DESC = new org.apache.thrift.protocol.TField("messageId", org.apache.thrift.protocol.TType.STRING, (short)2);
private static final org.apache.thrift.protocol.TField APPLICATION_ID_FIELD_DESC = new org.apache.thrift.protocol.TField("applicationId", org.apache.thrift.protocol.TType.STRING, (short)3);
private static final org.apache.thrift.protocol.TField MESSAGE_IDS_FIELD_DESC = new org.apache.thrift.protocol.TField("messageIds", org.apache.thrift.protocol.TType.LIST, (short)4);
private static final org.apache.thrift.protocol.TField DELETE_ALL_FIELD_DESC = new org.apache.thrift.protocol.TField("deleteAll", org.apache.thrift.protocol.TType.BOOL, (short)5);
private static final Map, SchemeFactory> schemes = new HashMap, SchemeFactory>();
static {
schemes.put(StandardScheme.class, new DeleteMessageRequestStandardSchemeFactory());
schemes.put(TupleScheme.class, new DeleteMessageRequestTupleSchemeFactory());
}
public tech.aroma.thrift.authentication.UserToken token; // required
public String messageId; // required
public String applicationId; // required
/**
* Use for Batch Deletes.
*/
public List messageIds; // optional
/**
* Use for deleting all the Messages stored for
* an Application. Note that this overrides other options.
* Use with care.
*/
public boolean deleteAll; // optional
/** The set of fields this struct contains, along with convenience methods for finding and manipulating them. */
public enum _Fields implements org.apache.thrift.TFieldIdEnum {
TOKEN((short)1, "token"),
MESSAGE_ID((short)2, "messageId"),
APPLICATION_ID((short)3, "applicationId"),
/**
* Use for Batch Deletes.
*/
MESSAGE_IDS((short)4, "messageIds"),
/**
* Use for deleting all the Messages stored for
* an Application. Note that this overrides other options.
* Use with care.
*/
DELETE_ALL((short)5, "deleteAll");
private static final Map byName = new HashMap();
static {
for (_Fields field : EnumSet.allOf(_Fields.class)) {
byName.put(field.getFieldName(), field);
}
}
/**
* Find the _Fields constant that matches fieldId, or null if its not found.
*/
public static _Fields findByThriftId(int fieldId) {
switch(fieldId) {
case 1: // TOKEN
return TOKEN;
case 2: // MESSAGE_ID
return MESSAGE_ID;
case 3: // APPLICATION_ID
return APPLICATION_ID;
case 4: // MESSAGE_IDS
return MESSAGE_IDS;
case 5: // DELETE_ALL
return DELETE_ALL;
default:
return null;
}
}
/**
* Find the _Fields constant that matches fieldId, throwing an exception
* if it is not found.
*/
public static _Fields findByThriftIdOrThrow(int fieldId) {
_Fields fields = findByThriftId(fieldId);
if (fields == null) throw new IllegalArgumentException("Field " + fieldId + " doesn't exist!");
return fields;
}
/**
* Find the _Fields constant that matches name, or null if its not found.
*/
public static _Fields findByName(String name) {
return byName.get(name);
}
private final short _thriftId;
private final String _fieldName;
_Fields(short thriftId, String fieldName) {
_thriftId = thriftId;
_fieldName = fieldName;
}
public short getThriftFieldId() {
return _thriftId;
}
public String getFieldName() {
return _fieldName;
}
}
// isset id assignments
private static final int __DELETEALL_ISSET_ID = 0;
private byte __isset_bitfield = 0;
private static final _Fields optionals[] = {_Fields.MESSAGE_IDS,_Fields.DELETE_ALL};
public static final Map<_Fields, org.apache.thrift.meta_data.FieldMetaData> metaDataMap;
static {
Map<_Fields, org.apache.thrift.meta_data.FieldMetaData> tmpMap = new EnumMap<_Fields, org.apache.thrift.meta_data.FieldMetaData>(_Fields.class);
tmpMap.put(_Fields.TOKEN, new org.apache.thrift.meta_data.FieldMetaData("token", org.apache.thrift.TFieldRequirementType.DEFAULT,
new org.apache.thrift.meta_data.FieldValueMetaData(org.apache.thrift.protocol.TType.STRUCT , "UserToken")));
tmpMap.put(_Fields.MESSAGE_ID, new org.apache.thrift.meta_data.FieldMetaData("messageId", org.apache.thrift.TFieldRequirementType.DEFAULT,
new org.apache.thrift.meta_data.FieldValueMetaData(org.apache.thrift.protocol.TType.STRING , "uuid")));
tmpMap.put(_Fields.APPLICATION_ID, new org.apache.thrift.meta_data.FieldMetaData("applicationId", org.apache.thrift.TFieldRequirementType.DEFAULT,
new org.apache.thrift.meta_data.FieldValueMetaData(org.apache.thrift.protocol.TType.STRING , "uuid")));
tmpMap.put(_Fields.MESSAGE_IDS, new org.apache.thrift.meta_data.FieldMetaData("messageIds", org.apache.thrift.TFieldRequirementType.OPTIONAL,
new org.apache.thrift.meta_data.ListMetaData(org.apache.thrift.protocol.TType.LIST,
new org.apache.thrift.meta_data.FieldValueMetaData(org.apache.thrift.protocol.TType.STRING , "uuid"))));
tmpMap.put(_Fields.DELETE_ALL, new org.apache.thrift.meta_data.FieldMetaData("deleteAll", org.apache.thrift.TFieldRequirementType.OPTIONAL,
new org.apache.thrift.meta_data.FieldValueMetaData(org.apache.thrift.protocol.TType.BOOL)));
metaDataMap = Collections.unmodifiableMap(tmpMap);
org.apache.thrift.meta_data.FieldMetaData.addStructMetaDataMap(DeleteMessageRequest.class, metaDataMap);
}
public DeleteMessageRequest() {
this.messageIds = new ArrayList();
this.deleteAll = false;
}
public DeleteMessageRequest(
tech.aroma.thrift.authentication.UserToken token,
String messageId,
String applicationId)
{
this();
this.token = token;
this.messageId = messageId;
this.applicationId = applicationId;
}
/**
* Performs a deep copy on other.
*/
public DeleteMessageRequest(DeleteMessageRequest other) {
__isset_bitfield = other.__isset_bitfield;
if (other.isSetToken()) {
this.token = other.token;
}
if (other.isSetMessageId()) {
this.messageId = other.messageId;
}
if (other.isSetApplicationId()) {
this.applicationId = other.applicationId;
}
if (other.isSetMessageIds()) {
List __this__messageIds = new ArrayList(other.messageIds.size());
for (String other_element : other.messageIds) {
__this__messageIds.add(other_element);
}
this.messageIds = __this__messageIds;
}
this.deleteAll = other.deleteAll;
}
public DeleteMessageRequest deepCopy() {
return new DeleteMessageRequest(this);
}
@Override
public void clear() {
this.token = null;
this.messageId = null;
this.applicationId = null;
this.messageIds = new ArrayList();
this.deleteAll = false;
}
public tech.aroma.thrift.authentication.UserToken getToken() {
return this.token;
}
public DeleteMessageRequest setToken(tech.aroma.thrift.authentication.UserToken token) {
this.token = token;
return this;
}
public void unsetToken() {
this.token = null;
}
/** Returns true if field token is set (has been assigned a value) and false otherwise */
public boolean isSetToken() {
return this.token != null;
}
public void setTokenIsSet(boolean value) {
if (!value) {
this.token = null;
}
}
public String getMessageId() {
return this.messageId;
}
public DeleteMessageRequest setMessageId(String messageId) {
this.messageId = messageId;
return this;
}
public void unsetMessageId() {
this.messageId = null;
}
/** Returns true if field messageId is set (has been assigned a value) and false otherwise */
public boolean isSetMessageId() {
return this.messageId != null;
}
public void setMessageIdIsSet(boolean value) {
if (!value) {
this.messageId = null;
}
}
public String getApplicationId() {
return this.applicationId;
}
public DeleteMessageRequest setApplicationId(String applicationId) {
this.applicationId = applicationId;
return this;
}
public void unsetApplicationId() {
this.applicationId = null;
}
/** Returns true if field applicationId is set (has been assigned a value) and false otherwise */
public boolean isSetApplicationId() {
return this.applicationId != null;
}
public void setApplicationIdIsSet(boolean value) {
if (!value) {
this.applicationId = null;
}
}
public int getMessageIdsSize() {
return (this.messageIds == null) ? 0 : this.messageIds.size();
}
public java.util.Iterator getMessageIdsIterator() {
return (this.messageIds == null) ? null : this.messageIds.iterator();
}
public void addToMessageIds(String elem) {
if (this.messageIds == null) {
this.messageIds = new ArrayList();
}
this.messageIds.add(elem);
}
/**
* Use for Batch Deletes.
*/
public List getMessageIds() {
return this.messageIds;
}
/**
* Use for Batch Deletes.
*/
public DeleteMessageRequest setMessageIds(List messageIds) {
this.messageIds = messageIds;
return this;
}
public void unsetMessageIds() {
this.messageIds = null;
}
/** Returns true if field messageIds is set (has been assigned a value) and false otherwise */
public boolean isSetMessageIds() {
return this.messageIds != null;
}
public void setMessageIdsIsSet(boolean value) {
if (!value) {
this.messageIds = null;
}
}
/**
* Use for deleting all the Messages stored for
* an Application. Note that this overrides other options.
* Use with care.
*/
public boolean isDeleteAll() {
return this.deleteAll;
}
/**
* Use for deleting all the Messages stored for
* an Application. Note that this overrides other options.
* Use with care.
*/
public DeleteMessageRequest setDeleteAll(boolean deleteAll) {
this.deleteAll = deleteAll;
setDeleteAllIsSet(true);
return this;
}
public void unsetDeleteAll() {
__isset_bitfield = EncodingUtils.clearBit(__isset_bitfield, __DELETEALL_ISSET_ID);
}
/** Returns true if field deleteAll is set (has been assigned a value) and false otherwise */
public boolean isSetDeleteAll() {
return EncodingUtils.testBit(__isset_bitfield, __DELETEALL_ISSET_ID);
}
public void setDeleteAllIsSet(boolean value) {
__isset_bitfield = EncodingUtils.setBit(__isset_bitfield, __DELETEALL_ISSET_ID, value);
}
public void setFieldValue(_Fields field, Object value) {
switch (field) {
case TOKEN:
if (value == null) {
unsetToken();
} else {
setToken((tech.aroma.thrift.authentication.UserToken)value);
}
break;
case MESSAGE_ID:
if (value == null) {
unsetMessageId();
} else {
setMessageId((String)value);
}
break;
case APPLICATION_ID:
if (value == null) {
unsetApplicationId();
} else {
setApplicationId((String)value);
}
break;
case MESSAGE_IDS:
if (value == null) {
unsetMessageIds();
} else {
setMessageIds((List)value);
}
break;
case DELETE_ALL:
if (value == null) {
unsetDeleteAll();
} else {
setDeleteAll((Boolean)value);
}
break;
}
}
public Object getFieldValue(_Fields field) {
switch (field) {
case TOKEN:
return getToken();
case MESSAGE_ID:
return getMessageId();
case APPLICATION_ID:
return getApplicationId();
case MESSAGE_IDS:
return getMessageIds();
case DELETE_ALL:
return isDeleteAll();
}
throw new IllegalStateException();
}
/** Returns true if field corresponding to fieldID is set (has been assigned a value) and false otherwise */
public boolean isSet(_Fields field) {
if (field == null) {
throw new IllegalArgumentException();
}
switch (field) {
case TOKEN:
return isSetToken();
case MESSAGE_ID:
return isSetMessageId();
case APPLICATION_ID:
return isSetApplicationId();
case MESSAGE_IDS:
return isSetMessageIds();
case DELETE_ALL:
return isSetDeleteAll();
}
throw new IllegalStateException();
}
@Override
public boolean equals(Object that) {
if (that == null)
return false;
if (that instanceof DeleteMessageRequest)
return this.equals((DeleteMessageRequest)that);
return false;
}
public boolean equals(DeleteMessageRequest that) {
if (that == null)
return false;
boolean this_present_token = true && this.isSetToken();
boolean that_present_token = true && that.isSetToken();
if (this_present_token || that_present_token) {
if (!(this_present_token && that_present_token))
return false;
if (!this.token.equals(that.token))
return false;
}
boolean this_present_messageId = true && this.isSetMessageId();
boolean that_present_messageId = true && that.isSetMessageId();
if (this_present_messageId || that_present_messageId) {
if (!(this_present_messageId && that_present_messageId))
return false;
if (!this.messageId.equals(that.messageId))
return false;
}
boolean this_present_applicationId = true && this.isSetApplicationId();
boolean that_present_applicationId = true && that.isSetApplicationId();
if (this_present_applicationId || that_present_applicationId) {
if (!(this_present_applicationId && that_present_applicationId))
return false;
if (!this.applicationId.equals(that.applicationId))
return false;
}
boolean this_present_messageIds = true && this.isSetMessageIds();
boolean that_present_messageIds = true && that.isSetMessageIds();
if (this_present_messageIds || that_present_messageIds) {
if (!(this_present_messageIds && that_present_messageIds))
return false;
if (!this.messageIds.equals(that.messageIds))
return false;
}
boolean this_present_deleteAll = true && this.isSetDeleteAll();
boolean that_present_deleteAll = true && that.isSetDeleteAll();
if (this_present_deleteAll || that_present_deleteAll) {
if (!(this_present_deleteAll && that_present_deleteAll))
return false;
if (this.deleteAll != that.deleteAll)
return false;
}
return true;
}
@Override
public int hashCode() {
List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy