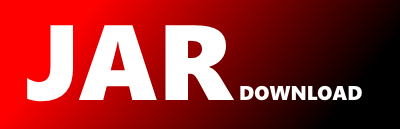
tech.aroma.thrift.service.ProvisionApplicationRequest Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aroma-thrift Show documentation
Show all versions of aroma-thrift Show documentation
Part of the Aroma Project.
This project contains the Service and Model Definitions.
From this the Server and Client interfaces are generated for the Aroma Service.
/**
* Autogenerated by Thrift Compiler (0.9.3)
*
* DO NOT EDIT UNLESS YOU ARE SURE THAT YOU KNOW WHAT YOU ARE DOING
* @generated
*/
package tech.aroma.thrift.service;
import org.apache.thrift.scheme.IScheme;
import org.apache.thrift.scheme.SchemeFactory;
import org.apache.thrift.scheme.StandardScheme;
import org.apache.thrift.scheme.TupleScheme;
import org.apache.thrift.protocol.TTupleProtocol;
import org.apache.thrift.protocol.TProtocolException;
import org.apache.thrift.EncodingUtils;
import org.apache.thrift.TException;
import org.apache.thrift.async.AsyncMethodCallback;
import org.apache.thrift.server.AbstractNonblockingServer.*;
import java.util.List;
import java.util.ArrayList;
import java.util.Map;
import java.util.HashMap;
import java.util.EnumMap;
import java.util.Set;
import java.util.HashSet;
import java.util.EnumSet;
import java.util.Collections;
import java.util.BitSet;
import java.nio.ByteBuffer;
import java.util.Arrays;
import javax.annotation.Generated;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
@SuppressWarnings({"cast", "rawtypes", "serial", "unchecked"})
/**
* Defines the required information to provision
* an Application with Aroma.
*/
@Generated(value = "Autogenerated by Thrift Compiler (0.9.3)", date = "2016-05-10")
public class ProvisionApplicationRequest implements org.apache.thrift.TBase, java.io.Serializable, Cloneable, Comparable {
private static final org.apache.thrift.protocol.TStruct STRUCT_DESC = new org.apache.thrift.protocol.TStruct("ProvisionApplicationRequest");
private static final org.apache.thrift.protocol.TField TOKEN_FIELD_DESC = new org.apache.thrift.protocol.TField("token", org.apache.thrift.protocol.TType.STRUCT, (short)1);
private static final org.apache.thrift.protocol.TField APPLICATION_NAME_FIELD_DESC = new org.apache.thrift.protocol.TField("applicationName", org.apache.thrift.protocol.TType.STRING, (short)2);
private static final org.apache.thrift.protocol.TField PROGRAMMING_LANGUAGE_FIELD_DESC = new org.apache.thrift.protocol.TField("programmingLanguage", org.apache.thrift.protocol.TType.I32, (short)3);
private static final org.apache.thrift.protocol.TField ORGANIZATION_ID_FIELD_DESC = new org.apache.thrift.protocol.TField("organizationId", org.apache.thrift.protocol.TType.STRING, (short)4);
private static final org.apache.thrift.protocol.TField ICON_FIELD_DESC = new org.apache.thrift.protocol.TField("icon", org.apache.thrift.protocol.TType.STRUCT, (short)5);
private static final org.apache.thrift.protocol.TField OWNERS_FIELD_DESC = new org.apache.thrift.protocol.TField("owners", org.apache.thrift.protocol.TType.SET, (short)6);
private static final org.apache.thrift.protocol.TField APPLICATION_DESCRIPTION_FIELD_DESC = new org.apache.thrift.protocol.TField("applicationDescription", org.apache.thrift.protocol.TType.STRING, (short)7);
private static final org.apache.thrift.protocol.TField TIER_FIELD_DESC = new org.apache.thrift.protocol.TField("tier", org.apache.thrift.protocol.TType.I32, (short)8);
private static final Map, SchemeFactory> schemes = new HashMap, SchemeFactory>();
static {
schemes.put(StandardScheme.class, new ProvisionApplicationRequestStandardSchemeFactory());
schemes.put(TupleScheme.class, new ProvisionApplicationRequestTupleSchemeFactory());
}
public tech.aroma.thrift.authentication.UserToken token; // required
public String applicationName; // required
/**
*
* @see tech.aroma.thrift.ProgrammingLanguage
*/
public tech.aroma.thrift.ProgrammingLanguage programmingLanguage; // optional
public String organizationId; // required
public tech.aroma.thrift.Image icon; // optional
public Set owners; // optional
public String applicationDescription; // optional
/**
*
* @see tech.aroma.thrift.Tier
*/
public tech.aroma.thrift.Tier tier; // optional
/** The set of fields this struct contains, along with convenience methods for finding and manipulating them. */
public enum _Fields implements org.apache.thrift.TFieldIdEnum {
TOKEN((short)1, "token"),
APPLICATION_NAME((short)2, "applicationName"),
/**
*
* @see tech.aroma.thrift.ProgrammingLanguage
*/
PROGRAMMING_LANGUAGE((short)3, "programmingLanguage"),
ORGANIZATION_ID((short)4, "organizationId"),
ICON((short)5, "icon"),
OWNERS((short)6, "owners"),
APPLICATION_DESCRIPTION((short)7, "applicationDescription"),
/**
*
* @see tech.aroma.thrift.Tier
*/
TIER((short)8, "tier");
private static final Map byName = new HashMap();
static {
for (_Fields field : EnumSet.allOf(_Fields.class)) {
byName.put(field.getFieldName(), field);
}
}
/**
* Find the _Fields constant that matches fieldId, or null if its not found.
*/
public static _Fields findByThriftId(int fieldId) {
switch(fieldId) {
case 1: // TOKEN
return TOKEN;
case 2: // APPLICATION_NAME
return APPLICATION_NAME;
case 3: // PROGRAMMING_LANGUAGE
return PROGRAMMING_LANGUAGE;
case 4: // ORGANIZATION_ID
return ORGANIZATION_ID;
case 5: // ICON
return ICON;
case 6: // OWNERS
return OWNERS;
case 7: // APPLICATION_DESCRIPTION
return APPLICATION_DESCRIPTION;
case 8: // TIER
return TIER;
default:
return null;
}
}
/**
* Find the _Fields constant that matches fieldId, throwing an exception
* if it is not found.
*/
public static _Fields findByThriftIdOrThrow(int fieldId) {
_Fields fields = findByThriftId(fieldId);
if (fields == null) throw new IllegalArgumentException("Field " + fieldId + " doesn't exist!");
return fields;
}
/**
* Find the _Fields constant that matches name, or null if its not found.
*/
public static _Fields findByName(String name) {
return byName.get(name);
}
private final short _thriftId;
private final String _fieldName;
_Fields(short thriftId, String fieldName) {
_thriftId = thriftId;
_fieldName = fieldName;
}
public short getThriftFieldId() {
return _thriftId;
}
public String getFieldName() {
return _fieldName;
}
}
// isset id assignments
private static final _Fields optionals[] = {_Fields.PROGRAMMING_LANGUAGE,_Fields.ICON,_Fields.OWNERS,_Fields.APPLICATION_DESCRIPTION,_Fields.TIER};
public static final Map<_Fields, org.apache.thrift.meta_data.FieldMetaData> metaDataMap;
static {
Map<_Fields, org.apache.thrift.meta_data.FieldMetaData> tmpMap = new EnumMap<_Fields, org.apache.thrift.meta_data.FieldMetaData>(_Fields.class);
tmpMap.put(_Fields.TOKEN, new org.apache.thrift.meta_data.FieldMetaData("token", org.apache.thrift.TFieldRequirementType.DEFAULT,
new org.apache.thrift.meta_data.FieldValueMetaData(org.apache.thrift.protocol.TType.STRUCT , "UserToken")));
tmpMap.put(_Fields.APPLICATION_NAME, new org.apache.thrift.meta_data.FieldMetaData("applicationName", org.apache.thrift.TFieldRequirementType.DEFAULT,
new org.apache.thrift.meta_data.FieldValueMetaData(org.apache.thrift.protocol.TType.STRING)));
tmpMap.put(_Fields.PROGRAMMING_LANGUAGE, new org.apache.thrift.meta_data.FieldMetaData("programmingLanguage", org.apache.thrift.TFieldRequirementType.OPTIONAL,
new org.apache.thrift.meta_data.EnumMetaData(org.apache.thrift.protocol.TType.ENUM, tech.aroma.thrift.ProgrammingLanguage.class)));
tmpMap.put(_Fields.ORGANIZATION_ID, new org.apache.thrift.meta_data.FieldMetaData("organizationId", org.apache.thrift.TFieldRequirementType.DEFAULT,
new org.apache.thrift.meta_data.FieldValueMetaData(org.apache.thrift.protocol.TType.STRING , "uuid")));
tmpMap.put(_Fields.ICON, new org.apache.thrift.meta_data.FieldMetaData("icon", org.apache.thrift.TFieldRequirementType.OPTIONAL,
new org.apache.thrift.meta_data.FieldValueMetaData(org.apache.thrift.protocol.TType.STRUCT , "Image")));
tmpMap.put(_Fields.OWNERS, new org.apache.thrift.meta_data.FieldMetaData("owners", org.apache.thrift.TFieldRequirementType.OPTIONAL,
new org.apache.thrift.meta_data.SetMetaData(org.apache.thrift.protocol.TType.SET,
new org.apache.thrift.meta_data.FieldValueMetaData(org.apache.thrift.protocol.TType.STRING , "uuid"))));
tmpMap.put(_Fields.APPLICATION_DESCRIPTION, new org.apache.thrift.meta_data.FieldMetaData("applicationDescription", org.apache.thrift.TFieldRequirementType.OPTIONAL,
new org.apache.thrift.meta_data.FieldValueMetaData(org.apache.thrift.protocol.TType.STRING)));
tmpMap.put(_Fields.TIER, new org.apache.thrift.meta_data.FieldMetaData("tier", org.apache.thrift.TFieldRequirementType.OPTIONAL,
new org.apache.thrift.meta_data.EnumMetaData(org.apache.thrift.protocol.TType.ENUM, tech.aroma.thrift.Tier.class)));
metaDataMap = Collections.unmodifiableMap(tmpMap);
org.apache.thrift.meta_data.FieldMetaData.addStructMetaDataMap(ProvisionApplicationRequest.class, metaDataMap);
}
public ProvisionApplicationRequest() {
this.applicationDescription = "";
this.tier = tech.aroma.thrift.Tier.FREE;
}
public ProvisionApplicationRequest(
tech.aroma.thrift.authentication.UserToken token,
String applicationName,
String organizationId)
{
this();
this.token = token;
this.applicationName = applicationName;
this.organizationId = organizationId;
}
/**
* Performs a deep copy on other.
*/
public ProvisionApplicationRequest(ProvisionApplicationRequest other) {
if (other.isSetToken()) {
this.token = other.token;
}
if (other.isSetApplicationName()) {
this.applicationName = other.applicationName;
}
if (other.isSetProgrammingLanguage()) {
this.programmingLanguage = other.programmingLanguage;
}
if (other.isSetOrganizationId()) {
this.organizationId = other.organizationId;
}
if (other.isSetIcon()) {
this.icon = other.icon;
}
if (other.isSetOwners()) {
Set __this__owners = new HashSet(other.owners.size());
for (String other_element : other.owners) {
__this__owners.add(other_element);
}
this.owners = __this__owners;
}
if (other.isSetApplicationDescription()) {
this.applicationDescription = other.applicationDescription;
}
if (other.isSetTier()) {
this.tier = other.tier;
}
}
public ProvisionApplicationRequest deepCopy() {
return new ProvisionApplicationRequest(this);
}
@Override
public void clear() {
this.token = null;
this.applicationName = null;
this.programmingLanguage = null;
this.organizationId = null;
this.icon = null;
this.owners = null;
this.applicationDescription = "";
this.tier = tech.aroma.thrift.Tier.FREE;
}
public tech.aroma.thrift.authentication.UserToken getToken() {
return this.token;
}
public ProvisionApplicationRequest setToken(tech.aroma.thrift.authentication.UserToken token) {
this.token = token;
return this;
}
public void unsetToken() {
this.token = null;
}
/** Returns true if field token is set (has been assigned a value) and false otherwise */
public boolean isSetToken() {
return this.token != null;
}
public void setTokenIsSet(boolean value) {
if (!value) {
this.token = null;
}
}
public String getApplicationName() {
return this.applicationName;
}
public ProvisionApplicationRequest setApplicationName(String applicationName) {
this.applicationName = applicationName;
return this;
}
public void unsetApplicationName() {
this.applicationName = null;
}
/** Returns true if field applicationName is set (has been assigned a value) and false otherwise */
public boolean isSetApplicationName() {
return this.applicationName != null;
}
public void setApplicationNameIsSet(boolean value) {
if (!value) {
this.applicationName = null;
}
}
/**
*
* @see tech.aroma.thrift.ProgrammingLanguage
*/
public tech.aroma.thrift.ProgrammingLanguage getProgrammingLanguage() {
return this.programmingLanguage;
}
/**
*
* @see tech.aroma.thrift.ProgrammingLanguage
*/
public ProvisionApplicationRequest setProgrammingLanguage(tech.aroma.thrift.ProgrammingLanguage programmingLanguage) {
this.programmingLanguage = programmingLanguage;
return this;
}
public void unsetProgrammingLanguage() {
this.programmingLanguage = null;
}
/** Returns true if field programmingLanguage is set (has been assigned a value) and false otherwise */
public boolean isSetProgrammingLanguage() {
return this.programmingLanguage != null;
}
public void setProgrammingLanguageIsSet(boolean value) {
if (!value) {
this.programmingLanguage = null;
}
}
public String getOrganizationId() {
return this.organizationId;
}
public ProvisionApplicationRequest setOrganizationId(String organizationId) {
this.organizationId = organizationId;
return this;
}
public void unsetOrganizationId() {
this.organizationId = null;
}
/** Returns true if field organizationId is set (has been assigned a value) and false otherwise */
public boolean isSetOrganizationId() {
return this.organizationId != null;
}
public void setOrganizationIdIsSet(boolean value) {
if (!value) {
this.organizationId = null;
}
}
public tech.aroma.thrift.Image getIcon() {
return this.icon;
}
public ProvisionApplicationRequest setIcon(tech.aroma.thrift.Image icon) {
this.icon = icon;
return this;
}
public void unsetIcon() {
this.icon = null;
}
/** Returns true if field icon is set (has been assigned a value) and false otherwise */
public boolean isSetIcon() {
return this.icon != null;
}
public void setIconIsSet(boolean value) {
if (!value) {
this.icon = null;
}
}
public int getOwnersSize() {
return (this.owners == null) ? 0 : this.owners.size();
}
public java.util.Iterator getOwnersIterator() {
return (this.owners == null) ? null : this.owners.iterator();
}
public void addToOwners(String elem) {
if (this.owners == null) {
this.owners = new HashSet();
}
this.owners.add(elem);
}
public Set getOwners() {
return this.owners;
}
public ProvisionApplicationRequest setOwners(Set owners) {
this.owners = owners;
return this;
}
public void unsetOwners() {
this.owners = null;
}
/** Returns true if field owners is set (has been assigned a value) and false otherwise */
public boolean isSetOwners() {
return this.owners != null;
}
public void setOwnersIsSet(boolean value) {
if (!value) {
this.owners = null;
}
}
public String getApplicationDescription() {
return this.applicationDescription;
}
public ProvisionApplicationRequest setApplicationDescription(String applicationDescription) {
this.applicationDescription = applicationDescription;
return this;
}
public void unsetApplicationDescription() {
this.applicationDescription = null;
}
/** Returns true if field applicationDescription is set (has been assigned a value) and false otherwise */
public boolean isSetApplicationDescription() {
return this.applicationDescription != null;
}
public void setApplicationDescriptionIsSet(boolean value) {
if (!value) {
this.applicationDescription = null;
}
}
/**
*
* @see tech.aroma.thrift.Tier
*/
public tech.aroma.thrift.Tier getTier() {
return this.tier;
}
/**
*
* @see tech.aroma.thrift.Tier
*/
public ProvisionApplicationRequest setTier(tech.aroma.thrift.Tier tier) {
this.tier = tier;
return this;
}
public void unsetTier() {
this.tier = null;
}
/** Returns true if field tier is set (has been assigned a value) and false otherwise */
public boolean isSetTier() {
return this.tier != null;
}
public void setTierIsSet(boolean value) {
if (!value) {
this.tier = null;
}
}
public void setFieldValue(_Fields field, Object value) {
switch (field) {
case TOKEN:
if (value == null) {
unsetToken();
} else {
setToken((tech.aroma.thrift.authentication.UserToken)value);
}
break;
case APPLICATION_NAME:
if (value == null) {
unsetApplicationName();
} else {
setApplicationName((String)value);
}
break;
case PROGRAMMING_LANGUAGE:
if (value == null) {
unsetProgrammingLanguage();
} else {
setProgrammingLanguage((tech.aroma.thrift.ProgrammingLanguage)value);
}
break;
case ORGANIZATION_ID:
if (value == null) {
unsetOrganizationId();
} else {
setOrganizationId((String)value);
}
break;
case ICON:
if (value == null) {
unsetIcon();
} else {
setIcon((tech.aroma.thrift.Image)value);
}
break;
case OWNERS:
if (value == null) {
unsetOwners();
} else {
setOwners((Set)value);
}
break;
case APPLICATION_DESCRIPTION:
if (value == null) {
unsetApplicationDescription();
} else {
setApplicationDescription((String)value);
}
break;
case TIER:
if (value == null) {
unsetTier();
} else {
setTier((tech.aroma.thrift.Tier)value);
}
break;
}
}
public Object getFieldValue(_Fields field) {
switch (field) {
case TOKEN:
return getToken();
case APPLICATION_NAME:
return getApplicationName();
case PROGRAMMING_LANGUAGE:
return getProgrammingLanguage();
case ORGANIZATION_ID:
return getOrganizationId();
case ICON:
return getIcon();
case OWNERS:
return getOwners();
case APPLICATION_DESCRIPTION:
return getApplicationDescription();
case TIER:
return getTier();
}
throw new IllegalStateException();
}
/** Returns true if field corresponding to fieldID is set (has been assigned a value) and false otherwise */
public boolean isSet(_Fields field) {
if (field == null) {
throw new IllegalArgumentException();
}
switch (field) {
case TOKEN:
return isSetToken();
case APPLICATION_NAME:
return isSetApplicationName();
case PROGRAMMING_LANGUAGE:
return isSetProgrammingLanguage();
case ORGANIZATION_ID:
return isSetOrganizationId();
case ICON:
return isSetIcon();
case OWNERS:
return isSetOwners();
case APPLICATION_DESCRIPTION:
return isSetApplicationDescription();
case TIER:
return isSetTier();
}
throw new IllegalStateException();
}
@Override
public boolean equals(Object that) {
if (that == null)
return false;
if (that instanceof ProvisionApplicationRequest)
return this.equals((ProvisionApplicationRequest)that);
return false;
}
public boolean equals(ProvisionApplicationRequest that) {
if (that == null)
return false;
boolean this_present_token = true && this.isSetToken();
boolean that_present_token = true && that.isSetToken();
if (this_present_token || that_present_token) {
if (!(this_present_token && that_present_token))
return false;
if (!this.token.equals(that.token))
return false;
}
boolean this_present_applicationName = true && this.isSetApplicationName();
boolean that_present_applicationName = true && that.isSetApplicationName();
if (this_present_applicationName || that_present_applicationName) {
if (!(this_present_applicationName && that_present_applicationName))
return false;
if (!this.applicationName.equals(that.applicationName))
return false;
}
boolean this_present_programmingLanguage = true && this.isSetProgrammingLanguage();
boolean that_present_programmingLanguage = true && that.isSetProgrammingLanguage();
if (this_present_programmingLanguage || that_present_programmingLanguage) {
if (!(this_present_programmingLanguage && that_present_programmingLanguage))
return false;
if (!this.programmingLanguage.equals(that.programmingLanguage))
return false;
}
boolean this_present_organizationId = true && this.isSetOrganizationId();
boolean that_present_organizationId = true && that.isSetOrganizationId();
if (this_present_organizationId || that_present_organizationId) {
if (!(this_present_organizationId && that_present_organizationId))
return false;
if (!this.organizationId.equals(that.organizationId))
return false;
}
boolean this_present_icon = true && this.isSetIcon();
boolean that_present_icon = true && that.isSetIcon();
if (this_present_icon || that_present_icon) {
if (!(this_present_icon && that_present_icon))
return false;
if (!this.icon.equals(that.icon))
return false;
}
boolean this_present_owners = true && this.isSetOwners();
boolean that_present_owners = true && that.isSetOwners();
if (this_present_owners || that_present_owners) {
if (!(this_present_owners && that_present_owners))
return false;
if (!this.owners.equals(that.owners))
return false;
}
boolean this_present_applicationDescription = true && this.isSetApplicationDescription();
boolean that_present_applicationDescription = true && that.isSetApplicationDescription();
if (this_present_applicationDescription || that_present_applicationDescription) {
if (!(this_present_applicationDescription && that_present_applicationDescription))
return false;
if (!this.applicationDescription.equals(that.applicationDescription))
return false;
}
boolean this_present_tier = true && this.isSetTier();
boolean that_present_tier = true && that.isSetTier();
if (this_present_tier || that_present_tier) {
if (!(this_present_tier && that_present_tier))
return false;
if (!this.tier.equals(that.tier))
return false;
}
return true;
}
@Override
public int hashCode() {
List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy