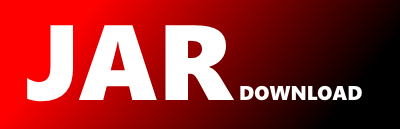
tech.carcadex.kotlinbukkitkit.messages.container.Messages Maven / Gradle / Ivy
package tech.carcadex.kotlinbukkitkit.messages.container;
import tech.carcadex.kotlinbukkitkit.messages.factory.impl.MessageFactoryImpl;
import tech.carcadex.kotlinbukkitkit.messages.model.Message;
import tech.carcadex.kotlinbukkitkit.messages.container.impl.MessagesImpl;
import tech.carcadex.kotlinbukkitkit.messages.factory.MessageFactory;
import tech.carcadex.kotlinbukkitkit.messages.factory.impl.LegacyFactoryImpl;
import tech.carcadex.kotlinbukkitkit.messages.model.impl.NullMessage;
import tech.carcadex.kotlinbukkitkit.messages.verifier.MessageVerifier;
import tech.carcadex.kotlinbukkitkit.messages.verifier.impl.FileMessageVerifier;
import org.bukkit.configuration.ConfigurationSection;
import org.bukkit.configuration.file.YamlConfiguration;
import org.bukkit.plugin.Plugin;
import org.jetbrains.annotations.NotNull;
import java.io.File;
import java.nio.file.Path;
/**
* Container of messages
* Create container by plugin with file messages.yml
* Step 1. Create file messages.yml in resources folder (in jar of plugin)
* Write in file your messages in YAML format. Use MiniMessages format for color messages.
* See https://docs.advntr.dev/minimessage/format.html
* For example I will be use this content:
*
* {@code
* error: "Error!"
* someRoot:
* message1: "Message"
* message2:
* - "Message start!"
* - "Message end!"
*
* }
*
* Step 2. Load Messages container in your onEnable method
*
* {@code
* Messages messages = Messages.of(this);
* }
*
* Step 3. Done! Now you can use the messages variable to manage messages.
* Create container by plugin with custom file
* Everything is the same, the same, only in jar create a file with the name you need (or several such files)
* and change OnEnable like this:
*
* {@code
* Messages messages = Messages.of(this, "YOURFILENAME.yml");
* }
*
* Create container by ConfigurationSection
* Here are a couple of examples of how this can be done:
*
* {@code
* Messages messages = Messages.of(getConfig().getConfigurationSection("messages"));
*
* //or so
*
* ConfigurationSection section = ...;
* Messages messages = Messages.of(section);
* }
*
* Reloadable Container:
* section - ConfigurationSection with messages
* file - File with messages
* SectionProvider - provider of section with messages
* @since 1.0.0
* @author itzRedTea
*/
public interface Messages {
/**
* Get message from container by key/yaml path
*
* For example
* Yaml structure:
*
* {@code
* error: "Error!"
* someRoot:
* message1: "Message"
* message2:
* - "Message start!"
* - "Message end!"
*
* }
*
*
* Getting messages in code:
*
* {@code
* Messages messages = ...; //Init Messages
* CommandSender sender = ...; //Getting CommandSender
* messages.get("error").send(sender); //Getting error message
* message.get("someRoot.message1").send(sender); //Getting someRoot.message1 message
* message.get("someRoot.message2").send(sender); //Getting someRoot.message2 message
* }
*
*
* @param key key of message
* @return Message
* @see Message
*/
@NotNull Message get(@NotNull String key);
/**
* Put message to container
* @param key key/yaml path to message
* @param message message to mut
* @return putted message
* @see Message
*/
@NotNull Message put(@NotNull String key, @NotNull Message message);
/**
* @param key key/yaml path to message
* @return is there a message with such a key in the container?
*/
boolean has(String key);
/**
* Reload container with messages in section from args.
* @param section - ConfigurationSection with messages
*/
void reload(@NotNull ConfigurationSection section);
/**
* Sets custom message parse factory.
*/
void factory(@NotNull MessageFactory messageFactory);
/**
* Parses all messages in stored section
*/
void parse();
void verifier(MessageVerifier verifier);
/**
* Create container using configuration section
* Create container using messages from configuration section.
*
* {@code
* ConfigurationSection section = ...;
* Messages messages = Messages.of(section);
* }
*
* @param section - configuration section with messages
* @return container with messages
*/
static Messages of(ConfigurationSection section, MessageFactory factory) {
return new MessagesImpl(section, factory);
}
/**
* Create container using current plugin and custom filename
* Create container using file with your name from jar entry by default.
* A new file in plugin folder will be created with content from your file.
*
* {@code
* Messages messages = Messages.of(this, "YOURFILENAME.yml");
* }
*
* @param plugin - plugin that creates a container
* @return container with messages
*/
static Messages of(String fileName, Plugin plugin) {
File file = new File(plugin.getDataFolder(), fileName);
if(!file.exists()) plugin.saveResource(fileName, false);
Messages messages = of(YamlConfiguration.loadConfiguration(file), new MessageFactoryImpl(plugin));
messages.verifier(new FileMessageVerifier(plugin.getResource(fileName), file));
return messages;
}
/**
* Create container using current plugin
* Create container using messages.yml file by default from jar entry.
* A new file in plugin folder will be created with content from messages.yml
*
* {@code
* Messages messages = Messages.of(this);
* }
*
* @param plugin - plugin that creates a container
* @return container with messages
*/
static Messages of(Plugin plugin) {
return of("messages.yml", plugin);
}
/**
* Create container using file
*
* {@code
* File file = new File("msg_file.yml");
* Messages messages = Messages.of(file);
* }
*
* @param file - file with messages
* @return container with messages
*/
static Messages of(File file, Plugin plugin) {
return of(YamlConfiguration.loadConfiguration(file), new MessageFactoryImpl(plugin));
}
/**
* Create container using path
*
* @param path - file with messages
* @return container with messages
* @see Messages#of(File, Plugin)
*/
static Messages of(Path path, Plugin plugin) {
return of(path.toFile(), plugin);
}
/**
* Only legacy color codes. For versions lower than 1.18
* @return container with messages
*/
static Messages legacy(ConfigurationSection section, Plugin plugin) {
return new MessagesImpl(section, new LegacyFactoryImpl(plugin));
}
/** @see Messages#legacy(ConfigurationSection, Plugin) */
static Messages legacy(String fileName, Plugin plugin) {
File file = new File(plugin.getDataFolder(), fileName);
if(!file.exists()) plugin.saveResource(fileName, false);
Messages messages = new MessagesImpl(file, new LegacyFactoryImpl(plugin));
messages.verifier(new FileMessageVerifier(plugin.getResource(fileName), file));
return messages;
}
/** @see Messages#legacy(ConfigurationSection, Plugin) */
static Messages legacy(Plugin plugin) {
return legacy("messages.yml", plugin);
}
/** @see Messages#legacy(ConfigurationSection, Plugin) */
static Messages legacy(File file, Plugin plugin) {
return new MessagesImpl(file, new LegacyFactoryImpl(plugin));
}
/**
* @return empty messages container
* Use this with Reloader
*/
static @NotNull Messages empty() {
return new MessagesImpl();
}
/**
* Singleton NULL_MESSAGE
* @see NullMessage
*/
Message NULL_MESSAGE = new NullMessage();
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy