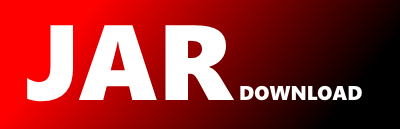
tech.carpentum.sdk.payment.IncomingPaymentsApi.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of payment-client-v2 Show documentation
Show all versions of payment-client-v2 Show documentation
Carpentum Payment system Java SDK
@file:JvmName("IncomingPaymentsApiUtils")
package tech.carpentum.sdk.payment
import tech.carpentum.sdk.payment.EndpointDefinition.Method.GET
import tech.carpentum.sdk.payment.EndpointDefinition.Method.POST
import tech.carpentum.sdk.payment.IncomingPaymentsApi.Factory
import tech.carpentum.sdk.payment.internal.api.ClientErrorExceptionFactory
import tech.carpentum.sdk.payment.internal.api.EnhancedIncomingPaymentsApi
import tech.carpentum.sdk.payment.internal.api.GetPayinErrorExceptionFactory
import tech.carpentum.sdk.payment.internal.api.PostAvailablePayinOptionsErrorExceptionFactory
import tech.carpentum.sdk.payment.internal.api.PostPayinsErrorExceptionFactory
import tech.carpentum.sdk.payment.internal.api.ResponseExceptionUtils
import tech.carpentum.sdk.payment.model.AvailablePayinOptionList
import tech.carpentum.sdk.payment.model.ExternalReference
import tech.carpentum.sdk.payment.model.GetPayinError
import tech.carpentum.sdk.payment.model.Payin
import tech.carpentum.sdk.payment.model.PayinAcceptedResponse
import tech.carpentum.sdk.payment.model.PayinDetail
import tech.carpentum.sdk.payment.model.PaymentRequested
import tech.carpentum.sdk.payment.model.PostAvailablePayinOptionsError
import tech.carpentum.sdk.payment.model.PostPayinsError
import java.io.InterruptedIOException
import java.time.Duration
/**
* The Incoming payment (payin) is an operation where money is sent from the customer account to the merchant account RESTful API.
*
* Use [Factory] to create new instance of the class.
*/
class IncomingPaymentsApi private constructor(
private val apiVersion: Int,
private val api: EnhancedIncomingPaymentsApi
) {
/**
* Throws [PostAvailablePayinOptionsErrorException] ("406" response) with one of defined
* [PostAvailablePayinOptionsError] business validation error code.
* Throws [InterruptedIOException] in case of timeout.
*/
@Throws(ResponseException::class, InterruptedIOException::class)
// tag::userGuidePublicApi[]
fun availablePaymentOptions(paymentRequested: PaymentRequested): AvailablePayinOptionList
// end::userGuidePublicApi[]
{
return ResponseExceptionUtils.wrap(PostAvailablePayinOptionsErrorExceptionFactory.instance) {
api.payinAvailablePaymentOptions(xAPIVersion = apiVersion, paymentRequested = paymentRequested)
}
}
/**
* Throws [PostPayinsErrorException] ("406" response) with one of defined
* [PostPayinsError] business validation error code.
* Throws [InterruptedIOException] in case of timeout.
*/
@Throws(ResponseException::class, InterruptedIOException::class)
// tag::userGuidePublicApi[]
fun createPayin(idPayin: String, payin: Payin): PayinAcceptedResponse
// end::userGuidePublicApi[]
{
return ResponseExceptionUtils.wrap(PostPayinsErrorExceptionFactory.instance) {
api.createPayin(xAPIVersion = apiVersion, idPayin = idPayin, payin = payin)
}
}
/**
* Throws [InterruptedIOException] in case of timeout.
*/
@Throws(ResponseException::class, InterruptedIOException::class)
// tag::userGuidePublicApi[]
fun setExternalReference(idPayin: String, reference: String)
// end::userGuidePublicApi[]
{
return ResponseExceptionUtils.wrap(ClientErrorExceptionFactory.instance) {
api.payinSetExternalReference(
xAPIVersion = apiVersion,
idPayin = idPayin,
externalReference = ExternalReference.ofReference(reference)
)
}
}
/**
* Throws [GetPayinErrorException] ("406" response) with one of defined
* [GetPayinError] business validation error code.
* Throws [InterruptedIOException] in case of timeout.
*/
@Throws(ResponseException::class, InterruptedIOException::class)
// tag::userGuidePublicApi[]
fun getPayin(idPayin: String): PayinDetail
// end::userGuidePublicApi[]
{
return ResponseExceptionUtils.wrap(GetPayinErrorExceptionFactory.instance) {
api.getPayin(xAPIVersion = apiVersion, idPayin = idPayin)
}
}
/**
* Factory to create a new instance of [IncomingPaymentsApi].
*/
companion object Factory {
/**
* Endpoint definition for [IncomingPaymentsApi.availablePaymentOptions] method.
*/
@JvmStatic
fun defineAvailablePaymentOptionsEndpoint(): EndpointDefinition =
EndpointDefinition(POST, "/payins/!availablePaymentOptions")
/**
* Endpoint definition for [IncomingPaymentsApi.createPayin] method.
*/
@JvmStatic
fun defineCreatePayinEndpoint(): EndpointWithIdDefinition = EndpointWithIdDefinition(POST, "/payins/{id}")
/**
* Endpoint definition for [IncomingPaymentsApi.getPayin] method.
*/
@JvmStatic
fun defineGetPayinEndpoint(): EndpointWithIdDefinition = EndpointWithIdDefinition(GET, "/payins/{id}")
/**
* Endpoint definition for [IncomingPaymentsApi.setExternalReference] method.
*/
@JvmStatic
fun defineSetExternalReferenceEndpoint(): EndpointWithIdDefinition =
EndpointWithIdDefinition(POST, "/payins/{id}/!setExternalReference")
@JvmStatic
@JvmOverloads
fun create(context: PaymentContext, accessToken: String, callTimeout: Duration? = null): IncomingPaymentsApi {
return IncomingPaymentsApi(
apiVersion = context.apiVersion,
api = EnhancedIncomingPaymentsApi(basePath = context.apiBaseUrl, accessToken = accessToken, brand = context.brand, callTimeout = callTimeout ?: context.defaultCallTimeout)
)
}
}
}
/**
* Grants [IncomingPaymentsApi.availablePaymentOptions] endpoint, see [IncomingPaymentsApi.defineAvailablePaymentOptionsEndpoint] definition.
*/
@JvmName("grantAvailablePaymentOptionsEndpoint")
fun AuthTokenOperations.grantIncomingPaymentsApiAvailablePaymentOptionsEndpoint(): AuthTokenOperations =
this.grant(IncomingPaymentsApi.defineAvailablePaymentOptionsEndpoint())
/**
* Grants [IncomingPaymentsApi.createPayin] endpoint, see [IncomingPaymentsApi.defineCreatePayinEndpoint] definition.
*/
@JvmName("grantCreatePayinEndpointForId")
fun AuthTokenOperations.grantIncomingPaymentsApiCreatePayinEndpointForId(id: String): AuthTokenOperations =
this.grant(IncomingPaymentsApi.defineCreatePayinEndpoint().forId(id))
/**
* Grants [IncomingPaymentsApi.createPayin] endpoint, see [IncomingPaymentsApi.defineCreatePayinEndpoint] definition.
*/
@JvmName("grantCreatePayinEndpointAnyId")
fun AuthTokenOperations.grantIncomingPaymentsApiCreatePayinEndpointAnyId(): AuthTokenOperations =
this.grant(IncomingPaymentsApi.defineCreatePayinEndpoint().anyId())
/**
* Grants [IncomingPaymentsApi.setExternalReference] endpoint, see [IncomingPaymentsApi.defineSetExternalReferenceEndpoint] definition.
*/
@JvmName("grantSetExternalReferenceEndpointForId")
fun AuthTokenOperations.grantIncomingPaymentsApiSetExternalReferenceEndpointForId(id: String): AuthTokenOperations =
this.grant(IncomingPaymentsApi.defineSetExternalReferenceEndpoint().forId(id))
/**
* Grants [IncomingPaymentsApi.setExternalReference] endpoint, see [IncomingPaymentsApi.defineSetExternalReferenceEndpoint] definition.
*/
@JvmName("grantSetExternalReferenceEndpointAnyId")
fun AuthTokenOperations.grantIncomingPaymentsApiSetExternalReferenceEndpointAnyId(): AuthTokenOperations =
this.grant(IncomingPaymentsApi.defineSetExternalReferenceEndpoint().anyId())
/**
* Grants [IncomingPaymentsApi.getPayin] endpoint, see [IncomingPaymentsApi.defineGetPayinEndpoint] definition.
*/
@JvmName("grantGetPayinEndpointForId")
fun AuthTokenOperations.grantIncomingPaymentsApiGetPayinEndpointForId(id: String): AuthTokenOperations =
this.grant(IncomingPaymentsApi.defineGetPayinEndpoint().forId(id))
/**
* Grants [IncomingPaymentsApi.getPayin] endpoint, see [IncomingPaymentsApi.defineGetPayinEndpoint] definition.
*/
@JvmName("grantGetPayinEndpointAnyId")
fun AuthTokenOperations.grantIncomingPaymentsApiGetPayinEndpointAnyId(): AuthTokenOperations =
this.grant(IncomingPaymentsApi.defineGetPayinEndpoint().anyId())
© 2015 - 2025 Weber Informatics LLC | Privacy Policy