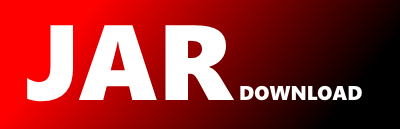
tech.carpentum.sdk.payment.OutgoingPaymentsApi.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of payment-client-v2 Show documentation
Show all versions of payment-client-v2 Show documentation
Carpentum Payment system Java SDK
@file:JvmName("OutgoingPaymentsApiUtils")
package tech.carpentum.sdk.payment
import tech.carpentum.sdk.payment.EndpointDefinition.Method.GET
import tech.carpentum.sdk.payment.EndpointDefinition.Method.POST
import tech.carpentum.sdk.payment.OutgoingPaymentsApi.Factory
import tech.carpentum.sdk.payment.internal.api.EnhancedOutgoingPaymentsApi
import tech.carpentum.sdk.payment.internal.api.GetPayoutErrorExceptionFactory
import tech.carpentum.sdk.payment.internal.api.PostAvailablePayoutOptionsErrorExceptionFactory
import tech.carpentum.sdk.payment.internal.api.PostPayoutsErrorExceptionFactory
import tech.carpentum.sdk.payment.internal.api.ResponseExceptionUtils
import tech.carpentum.sdk.payment.model.AvailablePayoutOptionList
import tech.carpentum.sdk.payment.model.GetPayoutError
import tech.carpentum.sdk.payment.model.PaymentRequested
import tech.carpentum.sdk.payment.model.Payout
import tech.carpentum.sdk.payment.model.PayoutAccepted
import tech.carpentum.sdk.payment.model.PayoutDetail
import tech.carpentum.sdk.payment.model.PostAvailablePayoutOptionsError
import tech.carpentum.sdk.payment.model.PostPayoutsError
import java.io.InterruptedIOException
import java.time.Duration
/**
* The Outgoing payment (payout) is an operation where money is sent from the merchant account to another account RESTful API.
*
* Use [Factory] to create new instance of the class.
*/
class OutgoingPaymentsApi private constructor(
private val apiVersion: Int,
private val api: EnhancedOutgoingPaymentsApi
) {
/**
* Throws [PostAvailablePayoutOptionsErrorException] ("406" response) with one of defined
* [PostAvailablePayoutOptionsError] business validation error code.
* Throws [InterruptedIOException] in case of timeout.
*/
@Throws(ResponseException::class, InterruptedIOException::class)
// tag::userGuidePublicApi[]
fun availablePaymentOptions(paymentRequested: PaymentRequested): AvailablePayoutOptionList
// end::userGuidePublicApi[]
{
return ResponseExceptionUtils.wrap(PostAvailablePayoutOptionsErrorExceptionFactory.instance) {
api.payoutAvailablePaymentOptions(xAPIVersion = apiVersion, paymentRequested = paymentRequested)
}
}
/**
* Throws [PostPayoutsErrorException] ("406" response) with one of defined
* [PostPayoutsError] business validation error code.
* Throws [InterruptedIOException] in case of timeout.
*/
@Throws(ResponseException::class, InterruptedIOException::class)
// tag::userGuidePublicApi[]
fun createPayout(idPayout: String, payout: Payout): PayoutAccepted
// end::userGuidePublicApi[]
{
return ResponseExceptionUtils.wrap(PostPayoutsErrorExceptionFactory.instance) {
api.createPayout(xAPIVersion = apiVersion, idPayout = idPayout, payout = payout)
}
}
/**
* Throws [GetPayoutErrorException] ("406" response) with one of defined
* [GetPayoutError] business validation error code.
* Throws [InterruptedIOException] in case of timeout.
*/
@Throws(ResponseException::class, InterruptedIOException::class)
// tag::userGuidePublicApi[]
fun getPayout(idPayout: String): PayoutDetail
// end::userGuidePublicApi[]
{
return ResponseExceptionUtils.wrap(GetPayoutErrorExceptionFactory.instance) {
api.getPayout(xAPIVersion = apiVersion, idPayout = idPayout)
}
}
/**
* Factory to create a new instance of [OutgoingPaymentsApi].
*/
companion object Factory {
/**
* Endpoint definition for [OutgoingPaymentsApi.availablePaymentOptions] method.
*/
@JvmStatic
fun defineAvailablePaymentOptionsEndpoint(): EndpointDefinition =
EndpointDefinition(POST, "/payouts/!availablePaymentOptions")
/**
* Endpoint definition for [OutgoingPaymentsApi.createPayout] method.
*/
@JvmStatic
fun defineCreatePayoutEndpoint(): EndpointWithIdDefinition = EndpointWithIdDefinition(POST, "/payouts/{id}")
/**
* Endpoint definition for [OutgoingPaymentsApi.getPayout] method.
*/
@JvmStatic
fun defineGetPayoutEndpoint(): EndpointWithIdDefinition = EndpointWithIdDefinition(GET, "/payouts/{id}")
@JvmStatic
@JvmOverloads
fun create(context: PaymentContext, accessToken: String, callTimeout: Duration? = null): OutgoingPaymentsApi {
return OutgoingPaymentsApi(
apiVersion = context.apiVersion,
api = EnhancedOutgoingPaymentsApi(basePath = context.apiBaseUrl, accessToken = accessToken, brand = context.brand, callTimeout = callTimeout ?: context.defaultCallTimeout)
)
}
}
}
/**
* Grants [OutgoingPaymentsApi.availablePaymentOptions] endpoint, see [OutgoingPaymentsApi.defineAvailablePaymentOptionsEndpoint] definition.
*/
@JvmName("grantAvailablePaymentOptionsEndpoint")
fun AuthTokenOperations.grantOutgoingPaymentsApiAvailablePaymentOptionsEndpoint(): AuthTokenOperations =
this.grant(IncomingPaymentsApi.defineAvailablePaymentOptionsEndpoint())
/**
* Grants [OutgoingPaymentsApi.createPayout] endpoint, see [OutgoingPaymentsApi.defineCreatePayoutEndpoint] definition.
*/
@JvmName("grantCreatePayoutEndpointForId")
fun AuthTokenOperations.grantOutgoingPaymentsApiCreatePayoutEndpointForId(id: String): AuthTokenOperations =
this.grant(OutgoingPaymentsApi.defineCreatePayoutEndpoint().forId(id))
/**
* Grants [OutgoingPaymentsApi.createPayout] endpoint, see [OutgoingPaymentsApi.defineCreatePayoutEndpoint] definition.
*/
@JvmName("grantCreatePayoutEndpointAnyId")
fun AuthTokenOperations.grantOutgoingPaymentsApiCreatePayoutEndpointAnyId(): AuthTokenOperations =
this.grant(OutgoingPaymentsApi.defineCreatePayoutEndpoint().anyId())
/**
* Grants [OutgoingPaymentsApi.getPayout] endpoint, see [OutgoingPaymentsApi.defineGetPayoutEndpoint] definition.
*/
@JvmName("grantGetPayoutEndpointForId")
fun AuthTokenOperations.grantOutgoingPaymentsApiGetPayoutEndpointForId(id: String): AuthTokenOperations =
this.grant(OutgoingPaymentsApi.defineGetPayoutEndpoint().forId(id))
/**
* Grants [OutgoingPaymentsApi.getPayout] endpoint, see [OutgoingPaymentsApi.defineGetPayoutEndpoint] definition.
*/
@JvmName("grantGetPayoutEndpointAnyId")
fun AuthTokenOperations.grantOutgoingPaymentsApiGetPayoutEndpointAnyId(): AuthTokenOperations =
this.grant(OutgoingPaymentsApi.defineGetPayoutEndpoint().anyId())
© 2015 - 2025 Weber Informatics LLC | Privacy Policy