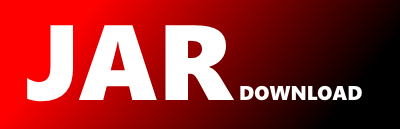
tech.carpentum.sdk.payment.PaymentsApi.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of payment-client-v2 Show documentation
Show all versions of payment-client-v2 Show documentation
Carpentum Payment system Java SDK
@file:JvmName("PaymentsApiUtils")
package tech.carpentum.sdk.payment
import tech.carpentum.sdk.payment.EndpointDefinition.Method.GET
import tech.carpentum.sdk.payment.PaymentsApi.Factory
import tech.carpentum.sdk.payment.internal.api.ClientErrorExceptionFactory
import tech.carpentum.sdk.payment.internal.api.CommaSeparatedCodes
import tech.carpentum.sdk.payment.internal.api.EnhancedPaymentsApi
import tech.carpentum.sdk.payment.internal.api.GetPaymentOptionsErrorExceptionFactory
import tech.carpentum.sdk.payment.internal.api.ResponseExceptionUtils
import tech.carpentum.sdk.payment.internal.generated.api.PaymentsApi.PaymentTypeCodeGetPaymentOptions
import tech.carpentum.sdk.payment.model.CurrencyList
import tech.carpentum.sdk.payment.model.GetPaymentOptionsError
import tech.carpentum.sdk.payment.model.PaymentMethodsList
import tech.carpentum.sdk.payment.model.PaymentOperatorList
import tech.carpentum.sdk.payment.model.PaymentOptionsList
import tech.carpentum.sdk.payment.model.SegmentList
import java.io.InterruptedIOException
import java.time.Duration
import java.util.function.Supplier
/**
* The Common methods that can be used for payin and payouts RESTful API.
*
* Use [Factory] to create new instance of the class.
*/
class PaymentsApi private constructor(
private val apiVersion: Int,
private val api: EnhancedPaymentsApi
) {
/**
* Throws [InterruptedIOException] in case of timeout.
*/
@Throws(ResponseException::class, InterruptedIOException::class)
// tag::userGuidePublicApi[]
fun listCurrencies(): CurrencyList
// end::userGuidePublicApi[]
{
return ResponseExceptionUtils.wrap(ClientErrorExceptionFactory.instance) {
api.getCurrencies(xAPIVersion = apiVersion)
}
}
/**
* Throws [InterruptedIOException] in case of timeout.
*/
@Throws(ResponseException::class, InterruptedIOException::class)
// tag::userGuidePublicApi[]
fun listSegments(): SegmentList
// end::userGuidePublicApi[]
{
return ResponseExceptionUtils.wrap(ClientErrorExceptionFactory.instance) {
api.getSegments(xAPIVersion = apiVersion)
}
}
/**
* Throws [InterruptedIOException] in case of timeout.
*/
@Throws(ResponseException::class, InterruptedIOException::class)
// tag::userGuidePublicApi[]
fun listPaymentOperators(): PaymentOperatorList
// end::userGuidePublicApi[]
{
return ResponseExceptionUtils.wrap(ClientErrorExceptionFactory.instance) {
api.getPaymentOperators(xAPIVersion = apiVersion)
}
}
/**
* Throws [InterruptedIOException] in case of timeout.
*/
@Throws(ResponseException::class, InterruptedIOException::class)
// tag::userGuidePublicApi[]
fun listPaymentMethods(): PaymentMethodsList
// end::userGuidePublicApi[]
{
return ResponseExceptionUtils.wrap(ClientErrorExceptionFactory.instance) {
api.getPaymentMethods(xAPIVersion = apiVersion)
}
}
/**
* Throws [GetPaymentOptionsErrorException] ("406" response) with one of defined
* [GetPaymentOptionsError] business validation error code.
* Throws [InterruptedIOException] in case of timeout.
*/
@Throws(ResponseException::class, InterruptedIOException::class)
@JvmOverloads
// tag::userGuidePublicApi[]
fun listPaymentOptions(query: ListPaymentOptionsQuery = ListPaymentOptionsQuery.any()): PaymentOptionsList
// end::userGuidePublicApi[]
{
return ResponseExceptionUtils.wrap(GetPaymentOptionsErrorExceptionFactory.instance) {
api.getPaymentOptions(
xAPIVersion = apiVersion,
paymentTypeCode = query.paymentTypeCode?.toModel(),
paymentMethodCodes = CommaSeparatedCodes.format(query.paymentMethodCodes),
currencyCodes = CommaSeparatedCodes.format(query.currencyCodes),
segmentCodes = CommaSeparatedCodes.format(query.segmentCodes),
paymentOperatorCodes = CommaSeparatedCodes.format(query.paymentOperatorCodes)
)
}
}
/**
* Throws [GetPaymentOptionsErrorException] ("406" response) with one of defined
* [GetPaymentOptionsError] business validation error code.
* Throws [InterruptedIOException] in case of timeout.
*/
@Throws(ResponseException::class, InterruptedIOException::class)
// tag::userGuidePublicApi[]
fun listPaymentOptions(query: Supplier): PaymentOptionsList
// end::userGuidePublicApi[]
{
return listPaymentOptions(query.get())
}
/**
* Parameter class for [PaymentsApi]'s `listPaymentOptions` methods.
*/
class ListPaymentOptionsQuery private constructor(
val paymentTypeCode: PaymentTypeCode? = null,
val paymentMethodCodes: Set? = null,
val currencyCodes: Set? = null,
val segmentCodes: Set? = null,
val paymentOperatorCodes: Set? = null
) {
private constructor(builder: BuilderImpl) : this(
builder.paymentTypeCode,
builder.paymentMethodCodes,
builder.currencyCodes,
builder.segmentCodes,
builder.paymentOperatorCodes
)
/**
* Used to specify [ListPaymentOptionsQuery.Builder.paymentTypeCode].
*/
enum class PaymentTypeCode {
PAYIN,
PAYOUT,
TOPUP_WALLET;
internal fun toModel(): PaymentTypeCodeGetPaymentOptions {
return PaymentTypeCodeGetPaymentOptions.valueOf(name)
}
}
/**
* Factory to create a new instance of [PaymentsApi]'s [ListPaymentOptionsQuery].
*/
companion object Factory : Builder {
private val empty: ListPaymentOptionsQuery = builder().get()
@JvmStatic
fun builder(): Builder = BuilderImpl()
@JvmStatic
override fun paymentTypeCode(paymentTypeCode: PaymentTypeCode): Builder {
return builder().paymentTypeCode(paymentTypeCode)
}
@JvmStatic
override fun paymentMethodCodes(vararg codes: String): Builder {
return builder().paymentMethodCodes(*codes)
}
@JvmStatic
override fun paymentMethodCodes(vararg codes: Enum<*>): Builder {
return builder().paymentMethodCodes(*(codes.map { it.name }.toTypedArray()))
}
@JvmStatic
override fun currencyCodes(vararg codes: String): Builder {
return builder().currencyCodes(*codes)
}
@JvmStatic
override fun segmentCodes(vararg codes: String): Builder {
return builder().segmentCodes(*codes)
}
@JvmStatic
override fun paymentOperatorCodes(vararg codes: String): Builder {
return builder().paymentOperatorCodes(*codes)
}
override fun get(): ListPaymentOptionsQuery {
return builder().get()
}
@JvmStatic
fun any(): ListPaymentOptionsQuery = empty
}
/**
* Builder to create a new instance of [PaymentsApi]'s [ListPaymentOptionsQuery].
*/
interface Builder : Supplier {
fun paymentTypeCode(paymentTypeCode: PaymentTypeCode): Builder
/**
* See [tech.carpentum.sdk.payment.model.PayinMethod.PaymentMethodCode].
* See [tech.carpentum.sdk.payment.model.PayoutMethod.PaymentMethodCode].
*/
fun paymentMethodCodes(vararg codes: String): Builder
/**
* See [tech.carpentum.sdk.payment.model.PayinMethod.PaymentMethodCode].
* See [tech.carpentum.sdk.payment.model.PayoutMethod.PaymentMethodCode].
*/
fun paymentMethodCodes(vararg codes: Enum<*>): Builder
fun currencyCodes(vararg codes: String): Builder
fun segmentCodes(vararg codes: String): Builder
fun paymentOperatorCodes(vararg codes: String): Builder
}
private class BuilderImpl : Builder {
var paymentTypeCode: PaymentTypeCode? = null
private set
var paymentMethodCodes: Set? = null
private set
var currencyCodes: Set? = null
private set
var segmentCodes: Set? = null
private set
var paymentOperatorCodes: Set? = null
private set
override fun paymentTypeCode(paymentTypeCode: PaymentTypeCode) = apply {
this.paymentTypeCode = paymentTypeCode
}
override fun paymentMethodCodes(vararg codes: String) = apply {
this.paymentMethodCodes = CommaSeparatedCodes.of(*codes)
}
override fun paymentMethodCodes(vararg codes: Enum<*>) = apply {
this.paymentMethodCodes = CommaSeparatedCodes.of(*(codes.map { it.name }.toTypedArray()))
}
override fun currencyCodes(vararg codes: String) = apply {
this.currencyCodes = CommaSeparatedCodes.of(*codes)
}
override fun segmentCodes(vararg codes: String) = apply {
this.segmentCodes = CommaSeparatedCodes.of(*codes)
}
override fun paymentOperatorCodes(vararg codes: String) = apply {
this.paymentOperatorCodes = CommaSeparatedCodes.of(*codes)
}
override fun get(): ListPaymentOptionsQuery {
return ListPaymentOptionsQuery(this)
}
}
}
/**
* Factory to create a new instance of [PaymentsApi].
*/
companion object Factory {
/**
* Endpoint definition for [PaymentsApi.listCurrencies] method.
*/
@JvmStatic
fun defineListCurrenciesEndpoint(): EndpointDefinition = EndpointDefinition(GET, "/currencies")
/**
* Endpoint definition for [PaymentsApi.listPaymentMethods] method.
*/
@JvmStatic
fun defineListPaymentMethodsEndpoint(): EndpointDefinition = EndpointDefinition(GET, "/payment-methods")
/**
* Endpoint definition for [PaymentsApi.listPaymentOperators] method.
*/
@JvmStatic
fun defineListPaymentOperatorsEndpoint(): EndpointDefinition = EndpointDefinition(GET, "/payment-operators")
/**
* Endpoint definition for [PaymentsApi.listPaymentOptions] method.
*/
@JvmStatic
fun defineListPaymentOptionsEndpoint(): EndpointDefinition = EndpointDefinition(GET, "/payment-options")
/**
* Endpoint definition for [PaymentsApi.listSegments] method.
*/
@JvmStatic
fun defineListSegmentsEndpoint(): EndpointDefinition = EndpointDefinition(GET, "/segments")
@JvmStatic
@JvmOverloads
fun create(context: PaymentContext, accessToken: String, callTimeout: Duration? = null): PaymentsApi {
return PaymentsApi(
context.apiVersion,
EnhancedPaymentsApi(basePath = context.apiBaseUrl, accessToken = accessToken, brand = context.brand, callTimeout = callTimeout ?: context.defaultCallTimeout)
)
}
}
}
/**
* Grants [PaymentsApi.listCurrencies] endpoint, see [PaymentsApi.defineListCurrenciesEndpoint] definition.
*/
@JvmName("grantListCurrenciesEndpoint")
fun AuthTokenOperations.grantPaymentsApiListCurrenciesEndpoint(): AuthTokenOperations =
this.grant(PaymentsApi.defineListCurrenciesEndpoint())
/**
* Grants [PaymentsApi.listSegments] endpoint, see [PaymentsApi.defineListSegmentsEndpoint] definition.
*/
@JvmName("grantListSegmentsEndpoint")
fun AuthTokenOperations.grantPaymentsApiListSegmentsEndpoint(): AuthTokenOperations =
this.grant(PaymentsApi.defineListSegmentsEndpoint())
/**
* Grants [PaymentsApi.listPaymentOperators] endpoint, see [PaymentsApi.defineListPaymentOperatorsEndpoint] definition.
*/
@JvmName("grantListPaymentOperatorsEndpoint")
fun AuthTokenOperations.grantPaymentsApiListPaymentOperatorsEndpoint(): AuthTokenOperations =
this.grant(PaymentsApi.defineListPaymentOperatorsEndpoint())
/**
* Grants [PaymentsApi.listPaymentMethods] endpoint, see [PaymentsApi.defineListPaymentMethodsEndpoint] definition.
*/
@JvmName("grantListPaymentMethodsEndpoint")
fun AuthTokenOperations.grantPaymentsApiListPaymentMethodsEndpoint(): AuthTokenOperations =
this.grant(PaymentsApi.defineListPaymentMethodsEndpoint())
/**
* Grants [PaymentsApi.listPaymentOptions] endpoint, see [PaymentsApi.defineListPaymentOptionsEndpoint] definition.
*/
@JvmName("grantListPaymentOptionsEndpoint")
fun AuthTokenOperations.grantPaymentsApiListPaymentOptionsEndpoint(): AuthTokenOperations =
this.grant(PaymentsApi.defineListPaymentOptionsEndpoint())
© 2015 - 2025 Weber Informatics LLC | Privacy Policy