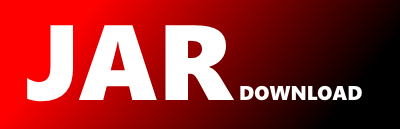
tech.carpentum.sdk.payment.internal.api.ResponseExceptionUtils.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of payment-client-v2 Show documentation
Show all versions of payment-client-v2 Show documentation
Carpentum Payment system Java SDK
package tech.carpentum.sdk.payment.internal.api
import org.slf4j.LoggerFactory
import tech.carpentum.sdk.payment.ClientBasicErrorException
import tech.carpentum.sdk.payment.ClientErrorException
import tech.carpentum.sdk.payment.ResponseException
import tech.carpentum.sdk.payment.ServerBasicErrorException
import tech.carpentum.sdk.payment.ServerErrorException
import tech.carpentum.sdk.payment.internal.generated.infrastructure.ClientError
import tech.carpentum.sdk.payment.internal.generated.infrastructure.ClientException
import tech.carpentum.sdk.payment.internal.generated.infrastructure.Serializer
import tech.carpentum.sdk.payment.internal.generated.infrastructure.ServerError
import tech.carpentum.sdk.payment.internal.generated.infrastructure.ServerException
import tech.carpentum.sdk.payment.model.BasicError
import java.io.InterruptedIOException
import java.net.HttpURLConnection
/**
* See [ResponseException].
*/
object ResponseExceptionUtils {
private val logger = LoggerFactory.getLogger(ResponseExceptionUtils::class.java)
private val jsonAdapterBasicError = Serializer.moshi.adapter(BasicError::class.java)
private fun clientException(cause: ClientException, businessValidationErrorExceptionFactory: BusinessValidationErrorExceptionFactory<*>): ClientErrorException {
val statusCode: Int = cause.statusCode
val bodyContent = (cause.response as ClientError<*>).body.toString()
return try {
if (statusCode == HttpURLConnection.HTTP_NOT_ACCEPTABLE) {
businessValidationErrorExceptionFactory.create(cause, statusCode, bodyContent)
} else {
ClientBasicErrorException(cause, statusCode, jsonAdapterBasicError.fromJson(bodyContent)!!)
}
} catch (ex: Exception) {
logger.debug("Error parsing response: {}", cause.response, ex)
ClientErrorException(cause, statusCode, bodyContent)
}
}
private fun serverException(cause: ServerException): ServerErrorException {
val statusCode: Int = cause.statusCode
val bodyContent = (cause.response as ServerError<*>).body.toString()
return try {
ServerBasicErrorException(cause, statusCode, jsonAdapterBasicError.fromJson(bodyContent)!!)
} catch (ex: Exception) {
logger.debug("Error parsing response: {}", cause.response, ex)
ServerErrorException(cause, statusCode)
}
}
@Throws(ResponseException::class, InterruptedIOException::class)
fun wrap(businessValidationErrorExceptionFactory: BusinessValidationErrorExceptionFactory<*>, apiCall: () -> T): T {
return try {
apiCall()
} catch (ex: ClientException) {
throw clientException(ex, businessValidationErrorExceptionFactory)
} catch (ex: ServerException) {
throw serverException(ex)
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy