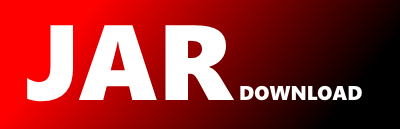
tech.carpentum.sdk.payment.internal.generated.api.AccountsApi.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of payment-client-v2 Show documentation
Show all versions of payment-client-v2 Show documentation
Carpentum Payment system Java SDK
The newest version!
/**
*
* Please note:
* This class is auto generated by OpenAPI Generator (https://openapi-generator.tech).
* Do not edit this file manually.
*
*/
@file:Suppress(
"ArrayInDataClass",
"EnumEntryName",
"RemoveRedundantQualifierName",
"UnusedImport"
)
package tech.carpentum.sdk.payment.internal.generated.api
import java.io.IOException
import okhttp3.OkHttpClient
import okhttp3.HttpUrl
import tech.carpentum.sdk.payment.model.BalanceList
import tech.carpentum.sdk.payment.model.BasicError
import tech.carpentum.sdk.payment.model.GetPayoutError
import com.squareup.moshi.Json
import tech.carpentum.sdk.payment.internal.generated.infrastructure.ApiClient
import tech.carpentum.sdk.payment.internal.generated.infrastructure.ApiResponse
import tech.carpentum.sdk.payment.internal.generated.infrastructure.ClientException
import tech.carpentum.sdk.payment.internal.generated.infrastructure.ClientError
import tech.carpentum.sdk.payment.internal.generated.infrastructure.ServerException
import tech.carpentum.sdk.payment.internal.generated.infrastructure.ServerError
import tech.carpentum.sdk.payment.internal.generated.infrastructure.MultiValueMap
import tech.carpentum.sdk.payment.internal.generated.infrastructure.PartConfig
import tech.carpentum.sdk.payment.internal.generated.infrastructure.RequestConfig
import tech.carpentum.sdk.payment.internal.generated.infrastructure.RequestMethod
import tech.carpentum.sdk.payment.internal.generated.infrastructure.ResponseType
import tech.carpentum.sdk.payment.internal.generated.infrastructure.Success
import tech.carpentum.sdk.payment.internal.generated.infrastructure.toMultiValue
internal open class AccountsApi(basePath: kotlin.String = defaultBasePath, client: OkHttpClient = ApiClient.defaultClient) : ApiClient(basePath, client) {
companion object {
@JvmStatic
val defaultBasePath: String by lazy {
System.getProperties().getProperty(ApiClient.baseUrlKey, "https://api.sandbox.carpentum.tech")
}
}
/**
* Account balance.
* Account balances for all selected currencies. If no currency is selected, all available are returned. If account in selected currency is not available, currency is ignored and no balance is returned.
* @param xAPIVersion Required API version. See [Versioning](general.html#versioning)
* @param currencyCodes (optional)
* @return BalanceList
* @throws IllegalStateException If the request is not correctly configured
* @throws IOException Rethrows the OkHttp execute method exception
* @throws UnsupportedOperationException If the API returns an informational or redirection response
* @throws ClientException If the API returns a client error response
* @throws ServerException If the API returns a server error response
*/
@Suppress("UNCHECKED_CAST")
@Throws(IllegalStateException::class, IOException::class, UnsupportedOperationException::class, ClientException::class, ServerException::class)
fun getBalances(xAPIVersion: kotlin.Int, currencyCodes: kotlin.String? = null) : BalanceList {
val localVarResponse = getBalancesWithHttpInfo(xAPIVersion = xAPIVersion, currencyCodes = currencyCodes)
return when (localVarResponse.responseType) {
ResponseType.Success -> (localVarResponse as Success<*>).data as BalanceList
ResponseType.Informational -> throw UnsupportedOperationException("Client does not support Informational responses.")
ResponseType.Redirection -> throw UnsupportedOperationException("Client does not support Redirection responses.")
ResponseType.ClientError -> {
val localVarError = localVarResponse as ClientError<*>
throw ClientException("Client error : ${localVarError.statusCode} ${localVarError.message.orEmpty()}", localVarError.statusCode, localVarResponse)
}
ResponseType.ServerError -> {
val localVarError = localVarResponse as ServerError<*>
throw ServerException("Server error : ${localVarError.statusCode} ${localVarError.message.orEmpty()} ${localVarError.body}", localVarError.statusCode, localVarResponse)
}
}
}
/**
* Account balance.
* Account balances for all selected currencies. If no currency is selected, all available are returned. If account in selected currency is not available, currency is ignored and no balance is returned.
* @param xAPIVersion Required API version. See [Versioning](general.html#versioning)
* @param currencyCodes (optional)
* @return ApiResponse
* @throws IllegalStateException If the request is not correctly configured
* @throws IOException Rethrows the OkHttp execute method exception
*/
@Suppress("UNCHECKED_CAST")
@Throws(IllegalStateException::class, IOException::class)
fun getBalancesWithHttpInfo(xAPIVersion: kotlin.Int, currencyCodes: kotlin.String?) : ApiResponse {
val localVariableConfig = getBalancesRequestConfig(xAPIVersion = xAPIVersion, currencyCodes = currencyCodes)
return request(
localVariableConfig
)
}
/**
* To obtain the request config of the operation getBalances
*
* @param xAPIVersion Required API version. See [Versioning](general.html#versioning)
* @param currencyCodes (optional)
* @return RequestConfig
*/
fun getBalancesRequestConfig(xAPIVersion: kotlin.Int, currencyCodes: kotlin.String?) : RequestConfig {
val localVariableBody = null
val localVariableQuery: MultiValueMap = mutableMapOf>()
.apply {
if (currencyCodes != null) {
put("currencyCodes", listOf(currencyCodes.toString()))
}
}
val localVariableHeaders: MutableMap = mutableMapOf()
xAPIVersion.apply { localVariableHeaders["X-API-Version"] = this.toString() }
localVariableHeaders["Accept"] = "application/json"
return RequestConfig(
method = RequestMethod.GET,
path = "/balances",
query = localVariableQuery,
headers = localVariableHeaders,
requiresAuthentication = true,
body = localVariableBody
)
}
private fun encodeURIComponent(uriComponent: kotlin.String): kotlin.String =
HttpUrl.Builder().scheme("http").host("localhost").addPathSegment(uriComponent).build().encodedPathSegments[0]
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy