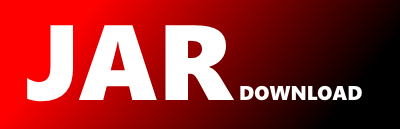
tech.carpentum.sdk.payment.internal.generated.api.IncomingPaymentsApi.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of payment-client-v2 Show documentation
Show all versions of payment-client-v2 Show documentation
Carpentum Payment system Java SDK
The newest version!
/**
*
* Please note:
* This class is auto generated by OpenAPI Generator (https://openapi-generator.tech).
* Do not edit this file manually.
*
*/
@file:Suppress(
"ArrayInDataClass",
"EnumEntryName",
"RemoveRedundantQualifierName",
"UnusedImport"
)
package tech.carpentum.sdk.payment.internal.generated.api
import java.io.IOException
import okhttp3.OkHttpClient
import okhttp3.HttpUrl
import tech.carpentum.sdk.payment.model.AvailablePayinOptionList
import tech.carpentum.sdk.payment.model.AvailableTopUpOptions
import tech.carpentum.sdk.payment.model.BasicError
import tech.carpentum.sdk.payment.model.ExternalReference
import tech.carpentum.sdk.payment.model.GetPayinError
import tech.carpentum.sdk.payment.model.GetTopupError
import tech.carpentum.sdk.payment.model.Payin
import tech.carpentum.sdk.payment.model.PayinAcceptedResponse
import tech.carpentum.sdk.payment.model.PayinDetail
import tech.carpentum.sdk.payment.model.PaymentAccountDetail
import tech.carpentum.sdk.payment.model.PaymentRequested
import tech.carpentum.sdk.payment.model.PostAvailablePayinOptionsError
import tech.carpentum.sdk.payment.model.PostAvailableTopUpOptionsError
import tech.carpentum.sdk.payment.model.PostPayinsError
import tech.carpentum.sdk.payment.model.TopUp
import tech.carpentum.sdk.payment.model.TopUpAcceptedResponse
import tech.carpentum.sdk.payment.model.TopUpDetail
import tech.carpentum.sdk.payment.model.TopUpRequested
import com.squareup.moshi.Json
import tech.carpentum.sdk.payment.internal.generated.infrastructure.ApiClient
import tech.carpentum.sdk.payment.internal.generated.infrastructure.ApiResponse
import tech.carpentum.sdk.payment.internal.generated.infrastructure.ClientException
import tech.carpentum.sdk.payment.internal.generated.infrastructure.ClientError
import tech.carpentum.sdk.payment.internal.generated.infrastructure.ServerException
import tech.carpentum.sdk.payment.internal.generated.infrastructure.ServerError
import tech.carpentum.sdk.payment.internal.generated.infrastructure.MultiValueMap
import tech.carpentum.sdk.payment.internal.generated.infrastructure.PartConfig
import tech.carpentum.sdk.payment.internal.generated.infrastructure.RequestConfig
import tech.carpentum.sdk.payment.internal.generated.infrastructure.RequestMethod
import tech.carpentum.sdk.payment.internal.generated.infrastructure.ResponseType
import tech.carpentum.sdk.payment.internal.generated.infrastructure.Success
import tech.carpentum.sdk.payment.internal.generated.infrastructure.toMultiValue
internal open class IncomingPaymentsApi(basePath: kotlin.String = defaultBasePath, client: OkHttpClient = ApiClient.defaultClient) : ApiClient(basePath, client) {
companion object {
@JvmStatic
val defaultBasePath: String by lazy {
System.getProperties().getProperty(ApiClient.baseUrlKey, "https://api.sandbox.carpentum.tech")
}
}
/**
* Create payment.
* Create a new payment request (incoming payment - merchant will get money from other account). For getting list of the available payment options use [`POST /payins/!availablePaymentOptions`](#operations-Incoming_payments-availablePaymentOptions) API. A complete payment flow description can be found in [Accept Payment](payorder.html) section.
* @param xAPIVersion Required API version. See [Versioning](general.html#versioning)
* @param idPayin Unique Merchant Order ID provided by merchant. See [Request IDs](general.html#request-ids).
* @param payin
* @return PayinAcceptedResponse
* @throws IllegalStateException If the request is not correctly configured
* @throws IOException Rethrows the OkHttp execute method exception
* @throws UnsupportedOperationException If the API returns an informational or redirection response
* @throws ClientException If the API returns a client error response
* @throws ServerException If the API returns a server error response
*/
@Suppress("UNCHECKED_CAST")
@Throws(IllegalStateException::class, IOException::class, UnsupportedOperationException::class, ClientException::class, ServerException::class)
fun createPayin(xAPIVersion: kotlin.Int, idPayin: kotlin.String, payin: Payin) : PayinAcceptedResponse {
val localVarResponse = createPayinWithHttpInfo(xAPIVersion = xAPIVersion, idPayin = idPayin, payin = payin)
return when (localVarResponse.responseType) {
ResponseType.Success -> (localVarResponse as Success<*>).data as PayinAcceptedResponse
ResponseType.Informational -> throw UnsupportedOperationException("Client does not support Informational responses.")
ResponseType.Redirection -> throw UnsupportedOperationException("Client does not support Redirection responses.")
ResponseType.ClientError -> {
val localVarError = localVarResponse as ClientError<*>
throw ClientException("Client error : ${localVarError.statusCode} ${localVarError.message.orEmpty()}", localVarError.statusCode, localVarResponse)
}
ResponseType.ServerError -> {
val localVarError = localVarResponse as ServerError<*>
throw ServerException("Server error : ${localVarError.statusCode} ${localVarError.message.orEmpty()} ${localVarError.body}", localVarError.statusCode, localVarResponse)
}
}
}
/**
* Create payment.
* Create a new payment request (incoming payment - merchant will get money from other account). For getting list of the available payment options use [`POST /payins/!availablePaymentOptions`](#operations-Incoming_payments-availablePaymentOptions) API. A complete payment flow description can be found in [Accept Payment](payorder.html) section.
* @param xAPIVersion Required API version. See [Versioning](general.html#versioning)
* @param idPayin Unique Merchant Order ID provided by merchant. See [Request IDs](general.html#request-ids).
* @param payin
* @return ApiResponse
* @throws IllegalStateException If the request is not correctly configured
* @throws IOException Rethrows the OkHttp execute method exception
*/
@Suppress("UNCHECKED_CAST")
@Throws(IllegalStateException::class, IOException::class)
fun createPayinWithHttpInfo(xAPIVersion: kotlin.Int, idPayin: kotlin.String, payin: Payin) : ApiResponse {
val localVariableConfig = createPayinRequestConfig(xAPIVersion = xAPIVersion, idPayin = idPayin, payin = payin)
return request(
localVariableConfig
)
}
/**
* To obtain the request config of the operation createPayin
*
* @param xAPIVersion Required API version. See [Versioning](general.html#versioning)
* @param idPayin Unique Merchant Order ID provided by merchant. See [Request IDs](general.html#request-ids).
* @param payin
* @return RequestConfig
*/
fun createPayinRequestConfig(xAPIVersion: kotlin.Int, idPayin: kotlin.String, payin: Payin) : RequestConfig {
val localVariableBody = payin
val localVariableQuery: MultiValueMap = mutableMapOf()
val localVariableHeaders: MutableMap = mutableMapOf()
xAPIVersion.apply { localVariableHeaders["X-API-Version"] = this.toString() }
localVariableHeaders["Content-Type"] = "application/json"
localVariableHeaders["Accept"] = "application/json"
return RequestConfig(
method = RequestMethod.POST,
path = "/payins/{idPayin}".replace("{"+"idPayin"+"}", encodeURIComponent(idPayin.toString())),
query = localVariableQuery,
headers = localVariableHeaders,
requiresAuthentication = true,
body = localVariableBody
)
}
/**
* Create a top-up payment.
* Create a new top-up payment request (incoming payment - wallet will get money from other account). For getting list of the available payment options use [`POST /payins/!availablePaymentOptions`](#operations-Incoming_payments-availablePaymentOptions) API. A complete payment flow description can be found in [Accept Payment](payorder.html) section.
* @param xAPIVersion Required API version. See [Versioning](general.html#versioning)
* @param idTopUp Unique Merchant Order ID provided by merchant. See [Request IDs](general.html#request-ids).
* @param topUp
* @return TopUpAcceptedResponse
* @throws IllegalStateException If the request is not correctly configured
* @throws IOException Rethrows the OkHttp execute method exception
* @throws UnsupportedOperationException If the API returns an informational or redirection response
* @throws ClientException If the API returns a client error response
* @throws ServerException If the API returns a server error response
*/
@Suppress("UNCHECKED_CAST")
@Throws(IllegalStateException::class, IOException::class, UnsupportedOperationException::class, ClientException::class, ServerException::class)
fun createTopup(xAPIVersion: kotlin.Int, idTopUp: kotlin.String, topUp: TopUp) : TopUpAcceptedResponse {
val localVarResponse = createTopupWithHttpInfo(xAPIVersion = xAPIVersion, idTopUp = idTopUp, topUp = topUp)
return when (localVarResponse.responseType) {
ResponseType.Success -> (localVarResponse as Success<*>).data as TopUpAcceptedResponse
ResponseType.Informational -> throw UnsupportedOperationException("Client does not support Informational responses.")
ResponseType.Redirection -> throw UnsupportedOperationException("Client does not support Redirection responses.")
ResponseType.ClientError -> {
val localVarError = localVarResponse as ClientError<*>
throw ClientException("Client error : ${localVarError.statusCode} ${localVarError.message.orEmpty()}", localVarError.statusCode, localVarResponse)
}
ResponseType.ServerError -> {
val localVarError = localVarResponse as ServerError<*>
throw ServerException("Server error : ${localVarError.statusCode} ${localVarError.message.orEmpty()} ${localVarError.body}", localVarError.statusCode, localVarResponse)
}
}
}
/**
* Create a top-up payment.
* Create a new top-up payment request (incoming payment - wallet will get money from other account). For getting list of the available payment options use [`POST /payins/!availablePaymentOptions`](#operations-Incoming_payments-availablePaymentOptions) API. A complete payment flow description can be found in [Accept Payment](payorder.html) section.
* @param xAPIVersion Required API version. See [Versioning](general.html#versioning)
* @param idTopUp Unique Merchant Order ID provided by merchant. See [Request IDs](general.html#request-ids).
* @param topUp
* @return ApiResponse
* @throws IllegalStateException If the request is not correctly configured
* @throws IOException Rethrows the OkHttp execute method exception
*/
@Suppress("UNCHECKED_CAST")
@Throws(IllegalStateException::class, IOException::class)
fun createTopupWithHttpInfo(xAPIVersion: kotlin.Int, idTopUp: kotlin.String, topUp: TopUp) : ApiResponse {
val localVariableConfig = createTopupRequestConfig(xAPIVersion = xAPIVersion, idTopUp = idTopUp, topUp = topUp)
return request(
localVariableConfig
)
}
/**
* To obtain the request config of the operation createTopup
*
* @param xAPIVersion Required API version. See [Versioning](general.html#versioning)
* @param idTopUp Unique Merchant Order ID provided by merchant. See [Request IDs](general.html#request-ids).
* @param topUp
* @return RequestConfig
*/
fun createTopupRequestConfig(xAPIVersion: kotlin.Int, idTopUp: kotlin.String, topUp: TopUp) : RequestConfig {
val localVariableBody = topUp
val localVariableQuery: MultiValueMap = mutableMapOf()
val localVariableHeaders: MutableMap = mutableMapOf()
xAPIVersion.apply { localVariableHeaders["X-API-Version"] = this.toString() }
localVariableHeaders["Content-Type"] = "application/json"
localVariableHeaders["Accept"] = "application/json"
return RequestConfig(
method = RequestMethod.POST,
path = "/topups/{idTopUp}".replace("{"+"idTopUp"+"}", encodeURIComponent(idTopUp.toString())),
query = localVariableQuery,
headers = localVariableHeaders,
requiresAuthentication = true,
body = localVariableBody
)
}
/**
* Incoming payment detail.
* Payment details including payment status and all collected data.
* @param xAPIVersion Required API version. See [Versioning](general.html#versioning)
* @param idPayin Unique Merchant Order ID provided by merchant. See [Request IDs](general.html#request-ids).
* @return PayinDetail
* @throws IllegalStateException If the request is not correctly configured
* @throws IOException Rethrows the OkHttp execute method exception
* @throws UnsupportedOperationException If the API returns an informational or redirection response
* @throws ClientException If the API returns a client error response
* @throws ServerException If the API returns a server error response
*/
@Suppress("UNCHECKED_CAST")
@Throws(IllegalStateException::class, IOException::class, UnsupportedOperationException::class, ClientException::class, ServerException::class)
fun getPayin(xAPIVersion: kotlin.Int, idPayin: kotlin.String) : PayinDetail {
val localVarResponse = getPayinWithHttpInfo(xAPIVersion = xAPIVersion, idPayin = idPayin)
return when (localVarResponse.responseType) {
ResponseType.Success -> (localVarResponse as Success<*>).data as PayinDetail
ResponseType.Informational -> throw UnsupportedOperationException("Client does not support Informational responses.")
ResponseType.Redirection -> throw UnsupportedOperationException("Client does not support Redirection responses.")
ResponseType.ClientError -> {
val localVarError = localVarResponse as ClientError<*>
throw ClientException("Client error : ${localVarError.statusCode} ${localVarError.message.orEmpty()}", localVarError.statusCode, localVarResponse)
}
ResponseType.ServerError -> {
val localVarError = localVarResponse as ServerError<*>
throw ServerException("Server error : ${localVarError.statusCode} ${localVarError.message.orEmpty()} ${localVarError.body}", localVarError.statusCode, localVarResponse)
}
}
}
/**
* Incoming payment detail.
* Payment details including payment status and all collected data.
* @param xAPIVersion Required API version. See [Versioning](general.html#versioning)
* @param idPayin Unique Merchant Order ID provided by merchant. See [Request IDs](general.html#request-ids).
* @return ApiResponse
* @throws IllegalStateException If the request is not correctly configured
* @throws IOException Rethrows the OkHttp execute method exception
*/
@Suppress("UNCHECKED_CAST")
@Throws(IllegalStateException::class, IOException::class)
fun getPayinWithHttpInfo(xAPIVersion: kotlin.Int, idPayin: kotlin.String) : ApiResponse {
val localVariableConfig = getPayinRequestConfig(xAPIVersion = xAPIVersion, idPayin = idPayin)
return request(
localVariableConfig
)
}
/**
* To obtain the request config of the operation getPayin
*
* @param xAPIVersion Required API version. See [Versioning](general.html#versioning)
* @param idPayin Unique Merchant Order ID provided by merchant. See [Request IDs](general.html#request-ids).
* @return RequestConfig
*/
fun getPayinRequestConfig(xAPIVersion: kotlin.Int, idPayin: kotlin.String) : RequestConfig {
val localVariableBody = null
val localVariableQuery: MultiValueMap = mutableMapOf()
val localVariableHeaders: MutableMap = mutableMapOf()
xAPIVersion.apply { localVariableHeaders["X-API-Version"] = this.toString() }
localVariableHeaders["Accept"] = "application/json"
return RequestConfig(
method = RequestMethod.GET,
path = "/payins/{idPayin}".replace("{"+"idPayin"+"}", encodeURIComponent(idPayin.toString())),
query = localVariableQuery,
headers = localVariableHeaders,
requiresAuthentication = true,
body = localVariableBody
)
}
/**
* Top up payment detail.
* Payment details including payment status and all collected data.
* @param xAPIVersion Required API version. See [Versioning](general.html#versioning)
* @param idTopUp Unique Merchant Order ID provided by merchant. See [Request IDs](general.html#request-ids).
* @return TopUpDetail
* @throws IllegalStateException If the request is not correctly configured
* @throws IOException Rethrows the OkHttp execute method exception
* @throws UnsupportedOperationException If the API returns an informational or redirection response
* @throws ClientException If the API returns a client error response
* @throws ServerException If the API returns a server error response
*/
@Suppress("UNCHECKED_CAST")
@Throws(IllegalStateException::class, IOException::class, UnsupportedOperationException::class, ClientException::class, ServerException::class)
fun getTopup(xAPIVersion: kotlin.Int, idTopUp: kotlin.String) : TopUpDetail {
val localVarResponse = getTopupWithHttpInfo(xAPIVersion = xAPIVersion, idTopUp = idTopUp)
return when (localVarResponse.responseType) {
ResponseType.Success -> (localVarResponse as Success<*>).data as TopUpDetail
ResponseType.Informational -> throw UnsupportedOperationException("Client does not support Informational responses.")
ResponseType.Redirection -> throw UnsupportedOperationException("Client does not support Redirection responses.")
ResponseType.ClientError -> {
val localVarError = localVarResponse as ClientError<*>
throw ClientException("Client error : ${localVarError.statusCode} ${localVarError.message.orEmpty()}", localVarError.statusCode, localVarResponse)
}
ResponseType.ServerError -> {
val localVarError = localVarResponse as ServerError<*>
throw ServerException("Server error : ${localVarError.statusCode} ${localVarError.message.orEmpty()} ${localVarError.body}", localVarError.statusCode, localVarResponse)
}
}
}
/**
* Top up payment detail.
* Payment details including payment status and all collected data.
* @param xAPIVersion Required API version. See [Versioning](general.html#versioning)
* @param idTopUp Unique Merchant Order ID provided by merchant. See [Request IDs](general.html#request-ids).
* @return ApiResponse
* @throws IllegalStateException If the request is not correctly configured
* @throws IOException Rethrows the OkHttp execute method exception
*/
@Suppress("UNCHECKED_CAST")
@Throws(IllegalStateException::class, IOException::class)
fun getTopupWithHttpInfo(xAPIVersion: kotlin.Int, idTopUp: kotlin.String) : ApiResponse {
val localVariableConfig = getTopupRequestConfig(xAPIVersion = xAPIVersion, idTopUp = idTopUp)
return request(
localVariableConfig
)
}
/**
* To obtain the request config of the operation getTopup
*
* @param xAPIVersion Required API version. See [Versioning](general.html#versioning)
* @param idTopUp Unique Merchant Order ID provided by merchant. See [Request IDs](general.html#request-ids).
* @return RequestConfig
*/
fun getTopupRequestConfig(xAPIVersion: kotlin.Int, idTopUp: kotlin.String) : RequestConfig {
val localVariableBody = null
val localVariableQuery: MultiValueMap = mutableMapOf()
val localVariableHeaders: MutableMap = mutableMapOf()
xAPIVersion.apply { localVariableHeaders["X-API-Version"] = this.toString() }
localVariableHeaders["Accept"] = "application/json"
return RequestConfig(
method = RequestMethod.GET,
path = "/topups/{idTopUp}".replace("{"+"idTopUp"+"}", encodeURIComponent(idTopUp.toString())),
query = localVariableQuery,
headers = localVariableHeaders,
requiresAuthentication = true,
body = localVariableBody
)
}
/**
* Incoming payment accounts details.
* Payment account details including data collected during the payment process.
* @param xAPIVersion Required API version. See [Versioning](general.html#versioning)
* @param idPayin Unique Merchant Order ID provided by merchant. See [Request IDs](general.html#request-ids).
* @return PaymentAccountDetail
* @throws IllegalStateException If the request is not correctly configured
* @throws IOException Rethrows the OkHttp execute method exception
* @throws UnsupportedOperationException If the API returns an informational or redirection response
* @throws ClientException If the API returns a client error response
* @throws ServerException If the API returns a server error response
*/
@Suppress("UNCHECKED_CAST")
@Throws(IllegalStateException::class, IOException::class, UnsupportedOperationException::class, ClientException::class, ServerException::class)
fun payinAccounts(xAPIVersion: kotlin.Int, idPayin: kotlin.String) : PaymentAccountDetail {
val localVarResponse = payinAccountsWithHttpInfo(xAPIVersion = xAPIVersion, idPayin = idPayin)
return when (localVarResponse.responseType) {
ResponseType.Success -> (localVarResponse as Success<*>).data as PaymentAccountDetail
ResponseType.Informational -> throw UnsupportedOperationException("Client does not support Informational responses.")
ResponseType.Redirection -> throw UnsupportedOperationException("Client does not support Redirection responses.")
ResponseType.ClientError -> {
val localVarError = localVarResponse as ClientError<*>
throw ClientException("Client error : ${localVarError.statusCode} ${localVarError.message.orEmpty()}", localVarError.statusCode, localVarResponse)
}
ResponseType.ServerError -> {
val localVarError = localVarResponse as ServerError<*>
throw ServerException("Server error : ${localVarError.statusCode} ${localVarError.message.orEmpty()} ${localVarError.body}", localVarError.statusCode, localVarResponse)
}
}
}
/**
* Incoming payment accounts details.
* Payment account details including data collected during the payment process.
* @param xAPIVersion Required API version. See [Versioning](general.html#versioning)
* @param idPayin Unique Merchant Order ID provided by merchant. See [Request IDs](general.html#request-ids).
* @return ApiResponse
* @throws IllegalStateException If the request is not correctly configured
* @throws IOException Rethrows the OkHttp execute method exception
*/
@Suppress("UNCHECKED_CAST")
@Throws(IllegalStateException::class, IOException::class)
fun payinAccountsWithHttpInfo(xAPIVersion: kotlin.Int, idPayin: kotlin.String) : ApiResponse {
val localVariableConfig = payinAccountsRequestConfig(xAPIVersion = xAPIVersion, idPayin = idPayin)
return request(
localVariableConfig
)
}
/**
* To obtain the request config of the operation payinAccounts
*
* @param xAPIVersion Required API version. See [Versioning](general.html#versioning)
* @param idPayin Unique Merchant Order ID provided by merchant. See [Request IDs](general.html#request-ids).
* @return RequestConfig
*/
fun payinAccountsRequestConfig(xAPIVersion: kotlin.Int, idPayin: kotlin.String) : RequestConfig {
val localVariableBody = null
val localVariableQuery: MultiValueMap = mutableMapOf()
val localVariableHeaders: MutableMap = mutableMapOf()
xAPIVersion.apply { localVariableHeaders["X-API-Version"] = this.toString() }
localVariableHeaders["Accept"] = "application/json"
return RequestConfig(
method = RequestMethod.GET,
path = "/payins/{idPayin}/accounts".replace("{"+"idPayin"+"}", encodeURIComponent(idPayin.toString())),
query = localVariableQuery,
headers = localVariableHeaders,
requiresAuthentication = true,
body = localVariableBody
)
}
/**
* Available payment options.
* Usually the first step when creating a new payment. Returns payment methods and payment operators available for the requested amount, currency and (optionally) the customer segment. The API documentation describes currently supported list of `paymentMethodCode`. Be aware that to return more items than described is classified as a backward-compatible change. The list of supported payment methods will grow in the future. New payment method is returned only when you have the method configured in your account. A complete payment flow description can be found in [Accept Incoming Payment](payin.html) section of our documentation.
* @param xAPIVersion Required API version. See [Versioning](general.html#versioning)
* @param paymentRequested
* @return AvailablePayinOptionList
* @throws IllegalStateException If the request is not correctly configured
* @throws IOException Rethrows the OkHttp execute method exception
* @throws UnsupportedOperationException If the API returns an informational or redirection response
* @throws ClientException If the API returns a client error response
* @throws ServerException If the API returns a server error response
*/
@Suppress("UNCHECKED_CAST")
@Throws(IllegalStateException::class, IOException::class, UnsupportedOperationException::class, ClientException::class, ServerException::class)
fun payinAvailablePaymentOptions(xAPIVersion: kotlin.Int, paymentRequested: PaymentRequested) : AvailablePayinOptionList {
val localVarResponse = payinAvailablePaymentOptionsWithHttpInfo(xAPIVersion = xAPIVersion, paymentRequested = paymentRequested)
return when (localVarResponse.responseType) {
ResponseType.Success -> (localVarResponse as Success<*>).data as AvailablePayinOptionList
ResponseType.Informational -> throw UnsupportedOperationException("Client does not support Informational responses.")
ResponseType.Redirection -> throw UnsupportedOperationException("Client does not support Redirection responses.")
ResponseType.ClientError -> {
val localVarError = localVarResponse as ClientError<*>
throw ClientException("Client error : ${localVarError.statusCode} ${localVarError.message.orEmpty()}", localVarError.statusCode, localVarResponse)
}
ResponseType.ServerError -> {
val localVarError = localVarResponse as ServerError<*>
throw ServerException("Server error : ${localVarError.statusCode} ${localVarError.message.orEmpty()} ${localVarError.body}", localVarError.statusCode, localVarResponse)
}
}
}
/**
* Available payment options.
* Usually the first step when creating a new payment. Returns payment methods and payment operators available for the requested amount, currency and (optionally) the customer segment. The API documentation describes currently supported list of `paymentMethodCode`. Be aware that to return more items than described is classified as a backward-compatible change. The list of supported payment methods will grow in the future. New payment method is returned only when you have the method configured in your account. A complete payment flow description can be found in [Accept Incoming Payment](payin.html) section of our documentation.
* @param xAPIVersion Required API version. See [Versioning](general.html#versioning)
* @param paymentRequested
* @return ApiResponse
* @throws IllegalStateException If the request is not correctly configured
* @throws IOException Rethrows the OkHttp execute method exception
*/
@Suppress("UNCHECKED_CAST")
@Throws(IllegalStateException::class, IOException::class)
fun payinAvailablePaymentOptionsWithHttpInfo(xAPIVersion: kotlin.Int, paymentRequested: PaymentRequested) : ApiResponse {
val localVariableConfig = payinAvailablePaymentOptionsRequestConfig(xAPIVersion = xAPIVersion, paymentRequested = paymentRequested)
return request(
localVariableConfig
)
}
/**
* To obtain the request config of the operation payinAvailablePaymentOptions
*
* @param xAPIVersion Required API version. See [Versioning](general.html#versioning)
* @param paymentRequested
* @return RequestConfig
*/
fun payinAvailablePaymentOptionsRequestConfig(xAPIVersion: kotlin.Int, paymentRequested: PaymentRequested) : RequestConfig {
val localVariableBody = paymentRequested
val localVariableQuery: MultiValueMap = mutableMapOf()
val localVariableHeaders: MutableMap = mutableMapOf()
xAPIVersion.apply { localVariableHeaders["X-API-Version"] = this.toString() }
localVariableHeaders["Content-Type"] = "application/json"
localVariableHeaders["Accept"] = "application/json"
return RequestConfig(
method = RequestMethod.POST,
path = "/payins/!availablePaymentOptions",
query = localVariableQuery,
headers = localVariableHeaders,
requiresAuthentication = true,
body = localVariableBody
)
}
/**
* Set external reference for the payment.
* Set external reference for the payment. Usually offline payments methods require additional payment reference as last step of payment process, these references are provided manully by the customer when payment is completed. See list of our [supported payment methods](payin-methods.html) to check which of them require to set the external payment reference.
* @param xAPIVersion Required API version. See [Versioning](general.html#versioning)
* @param idPayin Unique Merchant Order ID provided by merchant. See [Request IDs](general.html#request-ids).
* @param externalReference
* @return void
* @throws IllegalStateException If the request is not correctly configured
* @throws IOException Rethrows the OkHttp execute method exception
* @throws UnsupportedOperationException If the API returns an informational or redirection response
* @throws ClientException If the API returns a client error response
* @throws ServerException If the API returns a server error response
*/
@Throws(IllegalStateException::class, IOException::class, UnsupportedOperationException::class, ClientException::class, ServerException::class)
fun payinSetExternalReference(xAPIVersion: kotlin.Int, idPayin: kotlin.String, externalReference: ExternalReference) : Unit {
val localVarResponse = payinSetExternalReferenceWithHttpInfo(xAPIVersion = xAPIVersion, idPayin = idPayin, externalReference = externalReference)
return when (localVarResponse.responseType) {
ResponseType.Success -> Unit
ResponseType.Informational -> throw UnsupportedOperationException("Client does not support Informational responses.")
ResponseType.Redirection -> throw UnsupportedOperationException("Client does not support Redirection responses.")
ResponseType.ClientError -> {
val localVarError = localVarResponse as ClientError<*>
throw ClientException("Client error : ${localVarError.statusCode} ${localVarError.message.orEmpty()}", localVarError.statusCode, localVarResponse)
}
ResponseType.ServerError -> {
val localVarError = localVarResponse as ServerError<*>
throw ServerException("Server error : ${localVarError.statusCode} ${localVarError.message.orEmpty()} ${localVarError.body}", localVarError.statusCode, localVarResponse)
}
}
}
/**
* Set external reference for the payment.
* Set external reference for the payment. Usually offline payments methods require additional payment reference as last step of payment process, these references are provided manully by the customer when payment is completed. See list of our [supported payment methods](payin-methods.html) to check which of them require to set the external payment reference.
* @param xAPIVersion Required API version. See [Versioning](general.html#versioning)
* @param idPayin Unique Merchant Order ID provided by merchant. See [Request IDs](general.html#request-ids).
* @param externalReference
* @return ApiResponse
* @throws IllegalStateException If the request is not correctly configured
* @throws IOException Rethrows the OkHttp execute method exception
*/
@Throws(IllegalStateException::class, IOException::class)
fun payinSetExternalReferenceWithHttpInfo(xAPIVersion: kotlin.Int, idPayin: kotlin.String, externalReference: ExternalReference) : ApiResponse {
val localVariableConfig = payinSetExternalReferenceRequestConfig(xAPIVersion = xAPIVersion, idPayin = idPayin, externalReference = externalReference)
return request(
localVariableConfig
)
}
/**
* To obtain the request config of the operation payinSetExternalReference
*
* @param xAPIVersion Required API version. See [Versioning](general.html#versioning)
* @param idPayin Unique Merchant Order ID provided by merchant. See [Request IDs](general.html#request-ids).
* @param externalReference
* @return RequestConfig
*/
fun payinSetExternalReferenceRequestConfig(xAPIVersion: kotlin.Int, idPayin: kotlin.String, externalReference: ExternalReference) : RequestConfig {
val localVariableBody = externalReference
val localVariableQuery: MultiValueMap = mutableMapOf()
val localVariableHeaders: MutableMap = mutableMapOf()
xAPIVersion.apply { localVariableHeaders["X-API-Version"] = this.toString() }
localVariableHeaders["Content-Type"] = "application/json"
localVariableHeaders["Accept"] = "application/json"
return RequestConfig(
method = RequestMethod.POST,
path = "/payins/{idPayin}/!setExternalReference".replace("{"+"idPayin"+"}", encodeURIComponent(idPayin.toString())),
query = localVariableQuery,
headers = localVariableHeaders,
requiresAuthentication = true,
body = localVariableBody
)
}
/**
* Available top up payment options.
* Usually the first step when creating a new payment. Returns payment methods and payment operators available for the requested amount, currency and (optionally) the customer segment. The API documentation describes currently supported list of `paymentMethodCode`. Be aware that to return more items than described is classified as a backward-compatible change. The list of supported payment methods will grow in the future. New payment method is returned only when you have the method configured in your account. A complete payment flow description can be found in [Accept Incoming Payment](payin.html) section of our documentation.
* @param xAPIVersion Required API version. See [Versioning](general.html#versioning)
* @param topUpRequested
* @return AvailableTopUpOptions
* @throws IllegalStateException If the request is not correctly configured
* @throws IOException Rethrows the OkHttp execute method exception
* @throws UnsupportedOperationException If the API returns an informational or redirection response
* @throws ClientException If the API returns a client error response
* @throws ServerException If the API returns a server error response
*/
@Suppress("UNCHECKED_CAST")
@Throws(IllegalStateException::class, IOException::class, UnsupportedOperationException::class, ClientException::class, ServerException::class)
fun topupAvailablePaymentOptions(xAPIVersion: kotlin.Int, topUpRequested: TopUpRequested) : AvailableTopUpOptions {
val localVarResponse = topupAvailablePaymentOptionsWithHttpInfo(xAPIVersion = xAPIVersion, topUpRequested = topUpRequested)
return when (localVarResponse.responseType) {
ResponseType.Success -> (localVarResponse as Success<*>).data as AvailableTopUpOptions
ResponseType.Informational -> throw UnsupportedOperationException("Client does not support Informational responses.")
ResponseType.Redirection -> throw UnsupportedOperationException("Client does not support Redirection responses.")
ResponseType.ClientError -> {
val localVarError = localVarResponse as ClientError<*>
throw ClientException("Client error : ${localVarError.statusCode} ${localVarError.message.orEmpty()}", localVarError.statusCode, localVarResponse)
}
ResponseType.ServerError -> {
val localVarError = localVarResponse as ServerError<*>
throw ServerException("Server error : ${localVarError.statusCode} ${localVarError.message.orEmpty()} ${localVarError.body}", localVarError.statusCode, localVarResponse)
}
}
}
/**
* Available top up payment options.
* Usually the first step when creating a new payment. Returns payment methods and payment operators available for the requested amount, currency and (optionally) the customer segment. The API documentation describes currently supported list of `paymentMethodCode`. Be aware that to return more items than described is classified as a backward-compatible change. The list of supported payment methods will grow in the future. New payment method is returned only when you have the method configured in your account. A complete payment flow description can be found in [Accept Incoming Payment](payin.html) section of our documentation.
* @param xAPIVersion Required API version. See [Versioning](general.html#versioning)
* @param topUpRequested
* @return ApiResponse
* @throws IllegalStateException If the request is not correctly configured
* @throws IOException Rethrows the OkHttp execute method exception
*/
@Suppress("UNCHECKED_CAST")
@Throws(IllegalStateException::class, IOException::class)
fun topupAvailablePaymentOptionsWithHttpInfo(xAPIVersion: kotlin.Int, topUpRequested: TopUpRequested) : ApiResponse {
val localVariableConfig = topupAvailablePaymentOptionsRequestConfig(xAPIVersion = xAPIVersion, topUpRequested = topUpRequested)
return request(
localVariableConfig
)
}
/**
* To obtain the request config of the operation topupAvailablePaymentOptions
*
* @param xAPIVersion Required API version. See [Versioning](general.html#versioning)
* @param topUpRequested
* @return RequestConfig
*/
fun topupAvailablePaymentOptionsRequestConfig(xAPIVersion: kotlin.Int, topUpRequested: TopUpRequested) : RequestConfig {
val localVariableBody = topUpRequested
val localVariableQuery: MultiValueMap = mutableMapOf()
val localVariableHeaders: MutableMap = mutableMapOf()
xAPIVersion.apply { localVariableHeaders["X-API-Version"] = this.toString() }
localVariableHeaders["Content-Type"] = "application/json"
localVariableHeaders["Accept"] = "application/json"
return RequestConfig(
method = RequestMethod.POST,
path = "/topups/!availablePaymentOptions",
query = localVariableQuery,
headers = localVariableHeaders,
requiresAuthentication = true,
body = localVariableBody
)
}
private fun encodeURIComponent(uriComponent: kotlin.String): kotlin.String =
HttpUrl.Builder().scheme("http").host("localhost").addPathSegment(uriComponent).build().encodedPathSegments[0]
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy