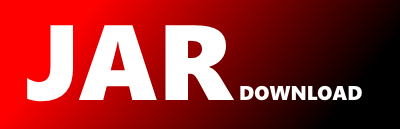
tech.carpentum.sdk.payment.internal.generated.api.OutgoingPaymentsApi.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of payment-client-v2 Show documentation
Show all versions of payment-client-v2 Show documentation
Carpentum Payment system Java SDK
The newest version!
/**
*
* Please note:
* This class is auto generated by OpenAPI Generator (https://openapi-generator.tech).
* Do not edit this file manually.
*
*/
@file:Suppress(
"ArrayInDataClass",
"EnumEntryName",
"RemoveRedundantQualifierName",
"UnusedImport"
)
package tech.carpentum.sdk.payment.internal.generated.api
import java.io.IOException
import okhttp3.OkHttpClient
import okhttp3.HttpUrl
import tech.carpentum.sdk.payment.model.AvailablePayoutOptionList
import tech.carpentum.sdk.payment.model.BasicError
import tech.carpentum.sdk.payment.model.GetPayoutError
import tech.carpentum.sdk.payment.model.PaymentRequested
import tech.carpentum.sdk.payment.model.Payout
import tech.carpentum.sdk.payment.model.PayoutAccepted
import tech.carpentum.sdk.payment.model.PayoutDetail
import tech.carpentum.sdk.payment.model.PostAvailablePayoutOptionsError
import tech.carpentum.sdk.payment.model.PostPayoutsError
import com.squareup.moshi.Json
import tech.carpentum.sdk.payment.internal.generated.infrastructure.ApiClient
import tech.carpentum.sdk.payment.internal.generated.infrastructure.ApiResponse
import tech.carpentum.sdk.payment.internal.generated.infrastructure.ClientException
import tech.carpentum.sdk.payment.internal.generated.infrastructure.ClientError
import tech.carpentum.sdk.payment.internal.generated.infrastructure.ServerException
import tech.carpentum.sdk.payment.internal.generated.infrastructure.ServerError
import tech.carpentum.sdk.payment.internal.generated.infrastructure.MultiValueMap
import tech.carpentum.sdk.payment.internal.generated.infrastructure.PartConfig
import tech.carpentum.sdk.payment.internal.generated.infrastructure.RequestConfig
import tech.carpentum.sdk.payment.internal.generated.infrastructure.RequestMethod
import tech.carpentum.sdk.payment.internal.generated.infrastructure.ResponseType
import tech.carpentum.sdk.payment.internal.generated.infrastructure.Success
import tech.carpentum.sdk.payment.internal.generated.infrastructure.toMultiValue
internal open class OutgoingPaymentsApi(basePath: kotlin.String = defaultBasePath, client: OkHttpClient = ApiClient.defaultClient) : ApiClient(basePath, client) {
companion object {
@JvmStatic
val defaultBasePath: String by lazy {
System.getProperties().getProperty(ApiClient.baseUrlKey, "https://api.sandbox.carpentum.tech")
}
}
/**
* Create outgoing payment.
* Create a new outgoing payment request (merchant send money to other account). A complete payment flow description can be found in [Outgoing Payment](payout.html) section.
* @param xAPIVersion Required API version. See [Versioning](general.html#versioning)
* @param idPayout Unique Merchant Order ID provided by merchant. See [Request IDs](general.html#request-ids).
* @param payout
* @return PayoutAccepted
* @throws IllegalStateException If the request is not correctly configured
* @throws IOException Rethrows the OkHttp execute method exception
* @throws UnsupportedOperationException If the API returns an informational or redirection response
* @throws ClientException If the API returns a client error response
* @throws ServerException If the API returns a server error response
*/
@Suppress("UNCHECKED_CAST")
@Throws(IllegalStateException::class, IOException::class, UnsupportedOperationException::class, ClientException::class, ServerException::class)
fun createPayout(xAPIVersion: kotlin.Int, idPayout: kotlin.String, payout: Payout) : PayoutAccepted {
val localVarResponse = createPayoutWithHttpInfo(xAPIVersion = xAPIVersion, idPayout = idPayout, payout = payout)
return when (localVarResponse.responseType) {
ResponseType.Success -> (localVarResponse as Success<*>).data as PayoutAccepted
ResponseType.Informational -> throw UnsupportedOperationException("Client does not support Informational responses.")
ResponseType.Redirection -> throw UnsupportedOperationException("Client does not support Redirection responses.")
ResponseType.ClientError -> {
val localVarError = localVarResponse as ClientError<*>
throw ClientException("Client error : ${localVarError.statusCode} ${localVarError.message.orEmpty()}", localVarError.statusCode, localVarResponse)
}
ResponseType.ServerError -> {
val localVarError = localVarResponse as ServerError<*>
throw ServerException("Server error : ${localVarError.statusCode} ${localVarError.message.orEmpty()} ${localVarError.body}", localVarError.statusCode, localVarResponse)
}
}
}
/**
* Create outgoing payment.
* Create a new outgoing payment request (merchant send money to other account). A complete payment flow description can be found in [Outgoing Payment](payout.html) section.
* @param xAPIVersion Required API version. See [Versioning](general.html#versioning)
* @param idPayout Unique Merchant Order ID provided by merchant. See [Request IDs](general.html#request-ids).
* @param payout
* @return ApiResponse
* @throws IllegalStateException If the request is not correctly configured
* @throws IOException Rethrows the OkHttp execute method exception
*/
@Suppress("UNCHECKED_CAST")
@Throws(IllegalStateException::class, IOException::class)
fun createPayoutWithHttpInfo(xAPIVersion: kotlin.Int, idPayout: kotlin.String, payout: Payout) : ApiResponse {
val localVariableConfig = createPayoutRequestConfig(xAPIVersion = xAPIVersion, idPayout = idPayout, payout = payout)
return request(
localVariableConfig
)
}
/**
* To obtain the request config of the operation createPayout
*
* @param xAPIVersion Required API version. See [Versioning](general.html#versioning)
* @param idPayout Unique Merchant Order ID provided by merchant. See [Request IDs](general.html#request-ids).
* @param payout
* @return RequestConfig
*/
fun createPayoutRequestConfig(xAPIVersion: kotlin.Int, idPayout: kotlin.String, payout: Payout) : RequestConfig {
val localVariableBody = payout
val localVariableQuery: MultiValueMap = mutableMapOf()
val localVariableHeaders: MutableMap = mutableMapOf()
xAPIVersion.apply { localVariableHeaders["X-API-Version"] = this.toString() }
localVariableHeaders["Content-Type"] = "application/json"
localVariableHeaders["Accept"] = "application/json"
return RequestConfig(
method = RequestMethod.POST,
path = "/payouts/{idPayout}".replace("{"+"idPayout"+"}", encodeURIComponent(idPayout.toString())),
query = localVariableQuery,
headers = localVariableHeaders,
requiresAuthentication = true,
body = localVariableBody
)
}
/**
* Outgoing payment detail.
* Payment details including payment status and all collected data.
* @param xAPIVersion Required API version. See [Versioning](general.html#versioning)
* @param idPayout Unique Merchant Order ID provided by merchant. See [Request IDs](general.html#request-ids).
* @return PayoutDetail
* @throws IllegalStateException If the request is not correctly configured
* @throws IOException Rethrows the OkHttp execute method exception
* @throws UnsupportedOperationException If the API returns an informational or redirection response
* @throws ClientException If the API returns a client error response
* @throws ServerException If the API returns a server error response
*/
@Suppress("UNCHECKED_CAST")
@Throws(IllegalStateException::class, IOException::class, UnsupportedOperationException::class, ClientException::class, ServerException::class)
fun getPayout(xAPIVersion: kotlin.Int, idPayout: kotlin.String) : PayoutDetail {
val localVarResponse = getPayoutWithHttpInfo(xAPIVersion = xAPIVersion, idPayout = idPayout)
return when (localVarResponse.responseType) {
ResponseType.Success -> (localVarResponse as Success<*>).data as PayoutDetail
ResponseType.Informational -> throw UnsupportedOperationException("Client does not support Informational responses.")
ResponseType.Redirection -> throw UnsupportedOperationException("Client does not support Redirection responses.")
ResponseType.ClientError -> {
val localVarError = localVarResponse as ClientError<*>
throw ClientException("Client error : ${localVarError.statusCode} ${localVarError.message.orEmpty()}", localVarError.statusCode, localVarResponse)
}
ResponseType.ServerError -> {
val localVarError = localVarResponse as ServerError<*>
throw ServerException("Server error : ${localVarError.statusCode} ${localVarError.message.orEmpty()} ${localVarError.body}", localVarError.statusCode, localVarResponse)
}
}
}
/**
* Outgoing payment detail.
* Payment details including payment status and all collected data.
* @param xAPIVersion Required API version. See [Versioning](general.html#versioning)
* @param idPayout Unique Merchant Order ID provided by merchant. See [Request IDs](general.html#request-ids).
* @return ApiResponse
* @throws IllegalStateException If the request is not correctly configured
* @throws IOException Rethrows the OkHttp execute method exception
*/
@Suppress("UNCHECKED_CAST")
@Throws(IllegalStateException::class, IOException::class)
fun getPayoutWithHttpInfo(xAPIVersion: kotlin.Int, idPayout: kotlin.String) : ApiResponse {
val localVariableConfig = getPayoutRequestConfig(xAPIVersion = xAPIVersion, idPayout = idPayout)
return request(
localVariableConfig
)
}
/**
* To obtain the request config of the operation getPayout
*
* @param xAPIVersion Required API version. See [Versioning](general.html#versioning)
* @param idPayout Unique Merchant Order ID provided by merchant. See [Request IDs](general.html#request-ids).
* @return RequestConfig
*/
fun getPayoutRequestConfig(xAPIVersion: kotlin.Int, idPayout: kotlin.String) : RequestConfig {
val localVariableBody = null
val localVariableQuery: MultiValueMap = mutableMapOf()
val localVariableHeaders: MutableMap = mutableMapOf()
xAPIVersion.apply { localVariableHeaders["X-API-Version"] = this.toString() }
localVariableHeaders["Accept"] = "application/json"
return RequestConfig(
method = RequestMethod.GET,
path = "/payouts/{idPayout}".replace("{"+"idPayout"+"}", encodeURIComponent(idPayout.toString())),
query = localVariableQuery,
headers = localVariableHeaders,
requiresAuthentication = true,
body = localVariableBody
)
}
/**
* Available payment options.
* Usually the first step when creating a new payment. Returns payment methods and payment operators available for the requested amount, currency and (optionally) the customer segment. The API documentation describes currently supported list of `paymentMethodCode`. Be aware that to return more items than described is classified as a backward-compatible change. The list of supported payment methods will grow in the future. New payment method is returned only when you have the method configured in your account. A complete payment flow description can be found in [Accept Outgoing Payment](payout.html) section of our documentation.
* @param xAPIVersion Required API version. See [Versioning](general.html#versioning)
* @param paymentRequested
* @return AvailablePayoutOptionList
* @throws IllegalStateException If the request is not correctly configured
* @throws IOException Rethrows the OkHttp execute method exception
* @throws UnsupportedOperationException If the API returns an informational or redirection response
* @throws ClientException If the API returns a client error response
* @throws ServerException If the API returns a server error response
*/
@Suppress("UNCHECKED_CAST")
@Throws(IllegalStateException::class, IOException::class, UnsupportedOperationException::class, ClientException::class, ServerException::class)
fun payoutAvailablePaymentOptions(xAPIVersion: kotlin.Int, paymentRequested: PaymentRequested) : AvailablePayoutOptionList {
val localVarResponse = payoutAvailablePaymentOptionsWithHttpInfo(xAPIVersion = xAPIVersion, paymentRequested = paymentRequested)
return when (localVarResponse.responseType) {
ResponseType.Success -> (localVarResponse as Success<*>).data as AvailablePayoutOptionList
ResponseType.Informational -> throw UnsupportedOperationException("Client does not support Informational responses.")
ResponseType.Redirection -> throw UnsupportedOperationException("Client does not support Redirection responses.")
ResponseType.ClientError -> {
val localVarError = localVarResponse as ClientError<*>
throw ClientException("Client error : ${localVarError.statusCode} ${localVarError.message.orEmpty()}", localVarError.statusCode, localVarResponse)
}
ResponseType.ServerError -> {
val localVarError = localVarResponse as ServerError<*>
throw ServerException("Server error : ${localVarError.statusCode} ${localVarError.message.orEmpty()} ${localVarError.body}", localVarError.statusCode, localVarResponse)
}
}
}
/**
* Available payment options.
* Usually the first step when creating a new payment. Returns payment methods and payment operators available for the requested amount, currency and (optionally) the customer segment. The API documentation describes currently supported list of `paymentMethodCode`. Be aware that to return more items than described is classified as a backward-compatible change. The list of supported payment methods will grow in the future. New payment method is returned only when you have the method configured in your account. A complete payment flow description can be found in [Accept Outgoing Payment](payout.html) section of our documentation.
* @param xAPIVersion Required API version. See [Versioning](general.html#versioning)
* @param paymentRequested
* @return ApiResponse
* @throws IllegalStateException If the request is not correctly configured
* @throws IOException Rethrows the OkHttp execute method exception
*/
@Suppress("UNCHECKED_CAST")
@Throws(IllegalStateException::class, IOException::class)
fun payoutAvailablePaymentOptionsWithHttpInfo(xAPIVersion: kotlin.Int, paymentRequested: PaymentRequested) : ApiResponse {
val localVariableConfig = payoutAvailablePaymentOptionsRequestConfig(xAPIVersion = xAPIVersion, paymentRequested = paymentRequested)
return request(
localVariableConfig
)
}
/**
* To obtain the request config of the operation payoutAvailablePaymentOptions
*
* @param xAPIVersion Required API version. See [Versioning](general.html#versioning)
* @param paymentRequested
* @return RequestConfig
*/
fun payoutAvailablePaymentOptionsRequestConfig(xAPIVersion: kotlin.Int, paymentRequested: PaymentRequested) : RequestConfig {
val localVariableBody = paymentRequested
val localVariableQuery: MultiValueMap = mutableMapOf()
val localVariableHeaders: MutableMap = mutableMapOf()
xAPIVersion.apply { localVariableHeaders["X-API-Version"] = this.toString() }
localVariableHeaders["Content-Type"] = "application/json"
localVariableHeaders["Accept"] = "application/json"
return RequestConfig(
method = RequestMethod.POST,
path = "/payouts/!availablePaymentOptions",
query = localVariableQuery,
headers = localVariableHeaders,
requiresAuthentication = true,
body = localVariableBody
)
}
private fun encodeURIComponent(uriComponent: kotlin.String): kotlin.String =
HttpUrl.Builder().scheme("http").host("localhost").addPathSegment(uriComponent).build().encodedPathSegments[0]
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy