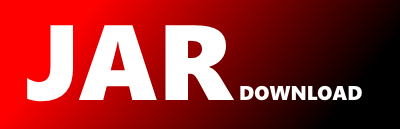
tech.carpentum.sdk.payment.internal.generated.api.SettlementsApi.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of payment-client-v2 Show documentation
Show all versions of payment-client-v2 Show documentation
Carpentum Payment system Java SDK
The newest version!
/**
*
* Please note:
* This class is auto generated by OpenAPI Generator (https://openapi-generator.tech).
* Do not edit this file manually.
*
*/
@file:Suppress(
"ArrayInDataClass",
"EnumEntryName",
"RemoveRedundantQualifierName",
"UnusedImport"
)
package tech.carpentum.sdk.payment.internal.generated.api
import java.io.IOException
import okhttp3.OkHttpClient
import okhttp3.HttpUrl
import tech.carpentum.sdk.payment.model.BasicError
import tech.carpentum.sdk.payment.model.GetPaymentOptionsError
import tech.carpentum.sdk.payment.model.SettlementPaymentOptionsList
import com.squareup.moshi.Json
import tech.carpentum.sdk.payment.internal.generated.infrastructure.ApiClient
import tech.carpentum.sdk.payment.internal.generated.infrastructure.ApiResponse
import tech.carpentum.sdk.payment.internal.generated.infrastructure.ClientException
import tech.carpentum.sdk.payment.internal.generated.infrastructure.ClientError
import tech.carpentum.sdk.payment.internal.generated.infrastructure.ServerException
import tech.carpentum.sdk.payment.internal.generated.infrastructure.ServerError
import tech.carpentum.sdk.payment.internal.generated.infrastructure.MultiValueMap
import tech.carpentum.sdk.payment.internal.generated.infrastructure.PartConfig
import tech.carpentum.sdk.payment.internal.generated.infrastructure.RequestConfig
import tech.carpentum.sdk.payment.internal.generated.infrastructure.RequestMethod
import tech.carpentum.sdk.payment.internal.generated.infrastructure.ResponseType
import tech.carpentum.sdk.payment.internal.generated.infrastructure.Success
import tech.carpentum.sdk.payment.internal.generated.infrastructure.toMultiValue
internal open class SettlementsApi(basePath: kotlin.String = defaultBasePath, client: OkHttpClient = ApiClient.defaultClient) : ApiClient(basePath, client) {
companion object {
@JvmStatic
val defaultBasePath: String by lazy {
System.getProperties().getProperty(ApiClient.baseUrlKey, "https://api.sandbox.carpentum.tech")
}
}
/**
* Settlement payment options.
* Return [payment options](terminology.html#term-Payment-option) (combination of payment methods and corresponding payment operators) (possibly) available for settlements for the merchant specified in the authorization token, optionally filtered by the query parameters. The API documentation describes currently supported list of `paymentMethodCode`. Be aware that to return more items than described is classified as a backward-compatible change. The list of supported payment methods will grow in the future. New payment method is returned only when you have the method configured in your account.
* @param xAPIVersion Required API version. See [Versioning](general.html#versioning)
* @param currencyCodes (optional)
* @param paymentOperatorCodes (optional)
* @return SettlementPaymentOptionsList
* @throws IllegalStateException If the request is not correctly configured
* @throws IOException Rethrows the OkHttp execute method exception
* @throws UnsupportedOperationException If the API returns an informational or redirection response
* @throws ClientException If the API returns a client error response
* @throws ServerException If the API returns a server error response
*/
@Suppress("UNCHECKED_CAST")
@Throws(IllegalStateException::class, IOException::class, UnsupportedOperationException::class, ClientException::class, ServerException::class)
fun getSettlementPaymentOptions(xAPIVersion: kotlin.Int, currencyCodes: kotlin.String? = null, paymentOperatorCodes: kotlin.String? = null) : SettlementPaymentOptionsList {
val localVarResponse = getSettlementPaymentOptionsWithHttpInfo(xAPIVersion = xAPIVersion, currencyCodes = currencyCodes, paymentOperatorCodes = paymentOperatorCodes)
return when (localVarResponse.responseType) {
ResponseType.Success -> (localVarResponse as Success<*>).data as SettlementPaymentOptionsList
ResponseType.Informational -> throw UnsupportedOperationException("Client does not support Informational responses.")
ResponseType.Redirection -> throw UnsupportedOperationException("Client does not support Redirection responses.")
ResponseType.ClientError -> {
val localVarError = localVarResponse as ClientError<*>
throw ClientException("Client error : ${localVarError.statusCode} ${localVarError.message.orEmpty()}", localVarError.statusCode, localVarResponse)
}
ResponseType.ServerError -> {
val localVarError = localVarResponse as ServerError<*>
throw ServerException("Server error : ${localVarError.statusCode} ${localVarError.message.orEmpty()} ${localVarError.body}", localVarError.statusCode, localVarResponse)
}
}
}
/**
* Settlement payment options.
* Return [payment options](terminology.html#term-Payment-option) (combination of payment methods and corresponding payment operators) (possibly) available for settlements for the merchant specified in the authorization token, optionally filtered by the query parameters. The API documentation describes currently supported list of `paymentMethodCode`. Be aware that to return more items than described is classified as a backward-compatible change. The list of supported payment methods will grow in the future. New payment method is returned only when you have the method configured in your account.
* @param xAPIVersion Required API version. See [Versioning](general.html#versioning)
* @param currencyCodes (optional)
* @param paymentOperatorCodes (optional)
* @return ApiResponse
* @throws IllegalStateException If the request is not correctly configured
* @throws IOException Rethrows the OkHttp execute method exception
*/
@Suppress("UNCHECKED_CAST")
@Throws(IllegalStateException::class, IOException::class)
fun getSettlementPaymentOptionsWithHttpInfo(xAPIVersion: kotlin.Int, currencyCodes: kotlin.String?, paymentOperatorCodes: kotlin.String?) : ApiResponse {
val localVariableConfig = getSettlementPaymentOptionsRequestConfig(xAPIVersion = xAPIVersion, currencyCodes = currencyCodes, paymentOperatorCodes = paymentOperatorCodes)
return request(
localVariableConfig
)
}
/**
* To obtain the request config of the operation getSettlementPaymentOptions
*
* @param xAPIVersion Required API version. See [Versioning](general.html#versioning)
* @param currencyCodes (optional)
* @param paymentOperatorCodes (optional)
* @return RequestConfig
*/
fun getSettlementPaymentOptionsRequestConfig(xAPIVersion: kotlin.Int, currencyCodes: kotlin.String?, paymentOperatorCodes: kotlin.String?) : RequestConfig {
val localVariableBody = null
val localVariableQuery: MultiValueMap = mutableMapOf>()
.apply {
if (currencyCodes != null) {
put("currencyCodes", listOf(currencyCodes.toString()))
}
if (paymentOperatorCodes != null) {
put("paymentOperatorCodes", listOf(paymentOperatorCodes.toString()))
}
}
val localVariableHeaders: MutableMap = mutableMapOf()
xAPIVersion.apply { localVariableHeaders["X-API-Version"] = this.toString() }
localVariableHeaders["Accept"] = "application/json"
return RequestConfig(
method = RequestMethod.GET,
path = "/settlement-payment-options",
query = localVariableQuery,
headers = localVariableHeaders,
requiresAuthentication = true,
body = localVariableBody
)
}
private fun encodeURIComponent(uriComponent: kotlin.String): kotlin.String =
HttpUrl.Builder().scheme("http").host("localhost").addPathSegment(uriComponent).build().encodedPathSegments[0]
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy