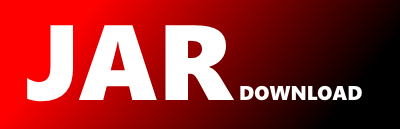
tech.carpentum.sdk.payment.internal.generated.model.AccountPayoutRequestBankTransferImpl Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of payment-client-v2 Show documentation
Show all versions of payment-client-v2 Show documentation
Carpentum Payment system Java SDK
The newest version!
//THE FILE IS GENERATED, DO NOT MODIFY IT MANUALLY!!!
package tech.carpentum.sdk.payment.internal.generated.model;
import com.squareup.moshi.JsonClass;
import java.util.Objects;
import java.util.Optional;
import org.jetbrains.annotations.NotNull;
import tech.carpentum.sdk.payment.internal.generated.model.*;
import tech.carpentum.sdk.payment.model.*;
/** AccountPayoutRequestBankTransfer
*
* Parameters of a customer's bank account where your customer would like his funds to be transferred.
The returned parameters are depended on the payout request currency.
*
*
*
* The model class is immutable.
* Use static {@link #builder} method to create a new {@link Builder} instance to build the model class instance.
*
*/
@JsonClass(generateAdapter = false)
public class AccountPayoutRequestBankTransferImpl implements AccountPayoutRequestBankTransfer {
/** Name of a customer's bank account where your customer would like his funds to be transferred. */
private final String accountName;
@Override
public String getAccountName() {
return accountName;
}
/** Number of a customer's bank account where your customer would like his funds to be transferred. */
private final String accountNumber;
@Override
public String getAccountNumber() {
return accountNumber;
}
/** Account type of the receiving bank account.
* If payout currency is JPY, then accountType field is required and requested value has to be "savings", "checking", "private", "corporate", "general" or "current".
* If payout currency is ZAR, then accountType field is required and requested value has to be "savings", "current", "cheque", "transmission".
Otherwise, accountType is optional. */
private final Optional accountType;
@Override
public Optional getAccountType() {
return accountType;
}
/** Bank code of the bank where your customer would like his funds to be transferred.
If currency is INR, then bankCode field is required and requested value has to be an Indian Financial System Code (IFSC bank code). Otherwise, it is optional. */
private final Optional bankCode;
@Override
public Optional getBankCode() {
return bankCode;
}
/** Name of the bank where your customer would like his funds to be transferred. */
private final Optional bankName;
@Override
public Optional getBankName() {
return bankName;
}
/** Branch name of the bank where your customer would like his funds to be transferred.
If payouts currency is JPY or TWD, then bankBranch field is required. Otherwise, it is optional. */
private final Optional bankBranch;
@Override
public Optional getBankBranch() {
return bankBranch;
}
/** City of the bank where your customer would like his funds to be transferred. */
private final Optional bankCity;
@Override
public Optional getBankCity() {
return bankCity;
}
/** Province of the bank where your customer would like his funds to be transferred. */
private final Optional bankProvince;
@Override
public Optional getBankProvince() {
return bankProvince;
}
private final int hashCode;
private final String toString;
private AccountPayoutRequestBankTransferImpl(BuilderImpl builder) {
this.accountName = Objects.requireNonNull(builder.accountName, "Property 'accountName' is required.");
this.accountNumber = Objects.requireNonNull(builder.accountNumber, "Property 'accountNumber' is required.");
this.accountType = Optional.ofNullable(builder.accountType);
this.bankCode = Optional.ofNullable(builder.bankCode);
this.bankName = Optional.ofNullable(builder.bankName);
this.bankBranch = Optional.ofNullable(builder.bankBranch);
this.bankCity = Optional.ofNullable(builder.bankCity);
this.bankProvince = Optional.ofNullable(builder.bankProvince);
this.hashCode = Objects.hash(accountName, accountNumber, accountType, bankCode, bankName, bankBranch, bankCity, bankProvince);
this.toString = builder.type + "(" +
"accountName=" + accountName +
", accountNumber=" + accountNumber +
", accountType=" + accountType +
", bankCode=" + bankCode +
", bankName=" + bankName +
", bankBranch=" + bankBranch +
", bankCity=" + bankCity +
", bankProvince=" + bankProvince +
')';
}
@Override
public int hashCode() {
return hashCode;
}
@Override
public boolean equals(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof AccountPayoutRequestBankTransferImpl)) {
return false;
}
AccountPayoutRequestBankTransferImpl that = (AccountPayoutRequestBankTransferImpl) obj;
if (!Objects.equals(this.accountName, that.accountName)) return false;
if (!Objects.equals(this.accountNumber, that.accountNumber)) return false;
if (!Objects.equals(this.accountType, that.accountType)) return false;
if (!Objects.equals(this.bankCode, that.bankCode)) return false;
if (!Objects.equals(this.bankName, that.bankName)) return false;
if (!Objects.equals(this.bankBranch, that.bankBranch)) return false;
if (!Objects.equals(this.bankCity, that.bankCity)) return false;
if (!Objects.equals(this.bankProvince, that.bankProvince)) return false;
return true;
}
@Override
public String toString() {
return toString;
}
/** Builder for {@link AccountPayoutRequestBankTransfer} model class. */
public static class BuilderImpl implements AccountPayoutRequestBankTransfer.Builder {
private String accountName = null;
private String accountNumber = null;
private String accountType = null;
private String bankCode = null;
private String bankName = null;
private String bankBranch = null;
private String bankCity = null;
private String bankProvince = null;
private final String type;
public BuilderImpl(String type) {
this.type = type;
}
public BuilderImpl() {
this("AccountPayoutRequestBankTransfer");
}
/**
* Set {@link AccountPayoutRequestBankTransfer#getAccountName} property.
*
* Name of a customer's bank account where your customer would like his funds to be transferred.
*/
@Override
public BuilderImpl accountName(String accountName) {
this.accountName = accountName;
return this;
}
/**
* Set {@link AccountPayoutRequestBankTransfer#getAccountNumber} property.
*
* Number of a customer's bank account where your customer would like his funds to be transferred.
*/
@Override
public BuilderImpl accountNumber(String accountNumber) {
this.accountNumber = accountNumber;
return this;
}
/**
* Set {@link AccountPayoutRequestBankTransfer#getAccountType} property.
*
* Account type of the receiving bank account.
* If payout currency is JPY, then accountType field is required and requested value has to be "savings", "checking", "private", "corporate", "general" or "current".
* If payout currency is ZAR, then accountType field is required and requested value has to be "savings", "current", "cheque", "transmission".
Otherwise, accountType is optional.
*/
@Override
public BuilderImpl accountType(String accountType) {
this.accountType = accountType;
return this;
}
/**
* Set {@link AccountPayoutRequestBankTransfer#getBankCode} property.
*
* Bank code of the bank where your customer would like his funds to be transferred.
If currency is INR, then bankCode field is required and requested value has to be an Indian Financial System Code (IFSC bank code). Otherwise, it is optional.
*/
@Override
public BuilderImpl bankCode(String bankCode) {
this.bankCode = bankCode;
return this;
}
/**
* Set {@link AccountPayoutRequestBankTransfer#getBankName} property.
*
* Name of the bank where your customer would like his funds to be transferred.
*/
@Override
public BuilderImpl bankName(String bankName) {
this.bankName = bankName;
return this;
}
/**
* Set {@link AccountPayoutRequestBankTransfer#getBankBranch} property.
*
* Branch name of the bank where your customer would like his funds to be transferred.
If payouts currency is JPY or TWD, then bankBranch field is required. Otherwise, it is optional.
*/
@Override
public BuilderImpl bankBranch(String bankBranch) {
this.bankBranch = bankBranch;
return this;
}
/**
* Set {@link AccountPayoutRequestBankTransfer#getBankCity} property.
*
* City of the bank where your customer would like his funds to be transferred.
*/
@Override
public BuilderImpl bankCity(String bankCity) {
this.bankCity = bankCity;
return this;
}
/**
* Set {@link AccountPayoutRequestBankTransfer#getBankProvince} property.
*
* Province of the bank where your customer would like his funds to be transferred.
*/
@Override
public BuilderImpl bankProvince(String bankProvince) {
this.bankProvince = bankProvince;
return this;
}
/**
* Create new instance of {@link AccountPayoutRequestBankTransfer} model class with the builder instance properties.
*
* @throws NullPointerException in case required properties are not specified.
*/
@Override
public AccountPayoutRequestBankTransferImpl build() {
return new AccountPayoutRequestBankTransferImpl(this);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy