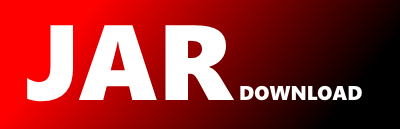
tech.carpentum.sdk.payment.internal.generated.model.AccountResponseOnlyWithBankImpl Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of payment-client-v2 Show documentation
Show all versions of payment-client-v2 Show documentation
Carpentum Payment system Java SDK
The newest version!
//THE FILE IS GENERATED, DO NOT MODIFY IT MANUALLY!!!
package tech.carpentum.sdk.payment.internal.generated.model;
import com.squareup.moshi.JsonClass;
import java.util.Objects;
import java.util.Optional;
import org.jetbrains.annotations.NotNull;
import tech.carpentum.sdk.payment.internal.generated.model.*;
import tech.carpentum.sdk.payment.model.*;
/** AccountResponseOnlyWithBank
*
* Parameters of a bank account where we expect that your customer send funds to make a payment. These account parameters has to be provided to your customer in form of an payment instructions.
The returned parameters are depended on the payment method and currency your customer choose to pay.
*
*
*
* The model class is immutable.
* Use static {@link #builder} method to create a new {@link Builder} instance to build the model class instance.
*
*/
@Deprecated
@JsonClass(generateAdapter = false)
public class AccountResponseOnlyWithBankImpl implements AccountResponseOnlyWithBank {
private final Optional accountName;
@Override
public Optional getAccountName() {
return accountName;
}
private final Optional accountNumber;
@Override
public Optional getAccountNumber() {
return accountNumber;
}
/** Bank code of the bank where we expect that your customer sends funds to make a payment.
If this parameter contains any value then show it to your customer in the payment instructions. */
private final Optional bankCode;
@Override
public Optional getBankCode() {
return bankCode;
}
/** Name of the bank where we expect that your customer sends funds to make a payment.
If this parameter contains any value then show it to your customer in the payment instructions. */
private final Optional bankName;
@Override
public Optional getBankName() {
return bankName;
}
/** Branch name of the bank where we expect that your customer sends funds to make a payment.
If this parameter contains any value then show it to your customer in the payment instructions. */
private final Optional bankBranch;
@Override
public Optional getBankBranch() {
return bankBranch;
}
/** City of the bank where we expect that your customer sends funds to make a payment.
If this parameter contains any value then show it to your customer in the payment instructions. */
private final Optional bankCity;
@Override
public Optional getBankCity() {
return bankCity;
}
/** Province of the bank where we expect that your customer sends funds to make a payment.
If this parameter contains any value then show it to your customer in the payment instructions. */
private final Optional bankProvince;
@Override
public Optional getBankProvince() {
return bankProvince;
}
private final int hashCode;
private final String toString;
private AccountResponseOnlyWithBankImpl(BuilderImpl builder) {
this.accountName = Optional.ofNullable(builder.accountName);
this.accountNumber = Optional.ofNullable(builder.accountNumber);
this.bankCode = Optional.ofNullable(builder.bankCode);
this.bankName = Optional.ofNullable(builder.bankName);
this.bankBranch = Optional.ofNullable(builder.bankBranch);
this.bankCity = Optional.ofNullable(builder.bankCity);
this.bankProvince = Optional.ofNullable(builder.bankProvince);
this.hashCode = Objects.hash(accountName, accountNumber, bankCode, bankName, bankBranch, bankCity, bankProvince);
this.toString = builder.type + "(" +
"accountName=" + accountName +
", accountNumber=" + accountNumber +
", bankCode=" + bankCode +
", bankName=" + bankName +
", bankBranch=" + bankBranch +
", bankCity=" + bankCity +
", bankProvince=" + bankProvince +
')';
}
@Override
public int hashCode() {
return hashCode;
}
@Override
public boolean equals(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof AccountResponseOnlyWithBankImpl)) {
return false;
}
AccountResponseOnlyWithBankImpl that = (AccountResponseOnlyWithBankImpl) obj;
if (!Objects.equals(this.accountName, that.accountName)) return false;
if (!Objects.equals(this.accountNumber, that.accountNumber)) return false;
if (!Objects.equals(this.bankCode, that.bankCode)) return false;
if (!Objects.equals(this.bankName, that.bankName)) return false;
if (!Objects.equals(this.bankBranch, that.bankBranch)) return false;
if (!Objects.equals(this.bankCity, that.bankCity)) return false;
if (!Objects.equals(this.bankProvince, that.bankProvince)) return false;
return true;
}
@Override
public String toString() {
return toString;
}
/** Builder for {@link AccountResponseOnlyWithBank} model class. */
public static class BuilderImpl implements AccountResponseOnlyWithBank.Builder {
private String accountName = null;
private String accountNumber = null;
private String bankCode = null;
private String bankName = null;
private String bankBranch = null;
private String bankCity = null;
private String bankProvince = null;
private final String type;
public BuilderImpl(String type) {
this.type = type;
}
public BuilderImpl() {
this("AccountResponseOnlyWithBank");
}
/**
* Set {@link AccountResponseOnlyWithBank#getAccountName} property.
*
*
*/
@Override
public BuilderImpl accountName(String accountName) {
this.accountName = accountName;
return this;
}
/**
* Set {@link AccountResponseOnlyWithBank#getAccountNumber} property.
*
*
*/
@Override
public BuilderImpl accountNumber(String accountNumber) {
this.accountNumber = accountNumber;
return this;
}
/**
* Set {@link AccountResponseOnlyWithBank#getBankCode} property.
*
* Bank code of the bank where we expect that your customer sends funds to make a payment.
If this parameter contains any value then show it to your customer in the payment instructions.
*/
@Override
public BuilderImpl bankCode(String bankCode) {
this.bankCode = bankCode;
return this;
}
/**
* Set {@link AccountResponseOnlyWithBank#getBankName} property.
*
* Name of the bank where we expect that your customer sends funds to make a payment.
If this parameter contains any value then show it to your customer in the payment instructions.
*/
@Override
public BuilderImpl bankName(String bankName) {
this.bankName = bankName;
return this;
}
/**
* Set {@link AccountResponseOnlyWithBank#getBankBranch} property.
*
* Branch name of the bank where we expect that your customer sends funds to make a payment.
If this parameter contains any value then show it to your customer in the payment instructions.
*/
@Override
public BuilderImpl bankBranch(String bankBranch) {
this.bankBranch = bankBranch;
return this;
}
/**
* Set {@link AccountResponseOnlyWithBank#getBankCity} property.
*
* City of the bank where we expect that your customer sends funds to make a payment.
If this parameter contains any value then show it to your customer in the payment instructions.
*/
@Override
public BuilderImpl bankCity(String bankCity) {
this.bankCity = bankCity;
return this;
}
/**
* Set {@link AccountResponseOnlyWithBank#getBankProvince} property.
*
* Province of the bank where we expect that your customer sends funds to make a payment.
If this parameter contains any value then show it to your customer in the payment instructions.
*/
@Override
public BuilderImpl bankProvince(String bankProvince) {
this.bankProvince = bankProvince;
return this;
}
/**
* Create new instance of {@link AccountResponseOnlyWithBank} model class with the builder instance properties.
*
* @throws NullPointerException in case required properties are not specified.
*/
@Override
public AccountResponseOnlyWithBankImpl build() {
return new AccountResponseOnlyWithBankImpl(this);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy