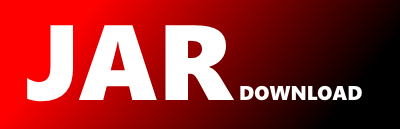
tech.carpentum.sdk.payment.internal.generated.model.CodePaymentMethodImpl Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of payment-client-v2 Show documentation
Show all versions of payment-client-v2 Show documentation
Carpentum Payment system Java SDK
The newest version!
//THE FILE IS GENERATED, DO NOT MODIFY IT MANUALLY!!!
package tech.carpentum.sdk.payment.internal.generated.model;
import com.squareup.moshi.JsonClass;
import java.util.Objects;
import java.util.Optional;
import org.jetbrains.annotations.NotNull;
import tech.carpentum.sdk.payment.internal.generated.model.*;
import tech.carpentum.sdk.payment.model.*;
/** CODE_PAYMENT
*
* Convenience Store Payments. Payment method which requires customer to copy Payment Code from the Payment application right after the payment is submitted into kiosk in convenience store during the payment on the cashier.
Each Payment Code is assigned to one and only pne payment and should be used only once.
*
*
*
* The model class is immutable.
* Use static {@link #builder} method to create a new {@link Builder} instance to build the model class instance.
*
*/
@JsonClass(generateAdapter = false)
public class CodePaymentMethodImpl implements CodePaymentMethod {
private final Optional account;
@Override
public Optional getAccount() {
return account;
}
/** One of following can serve as Payment Operator:
* Financial or other institution (such as bank, card payment processor, ...) that manages transactions for your customers
* Mobile wallet
* Blockchain protocol for crypto currency payments
Customer is informed with the payment instructions where funds have to be transferred efficiently based on the selected Payment operator.
For getting list of the available payment options for payins use [POST /payins/!availablePaymentOptions](#operations-Incoming_payments-availablePaymentOptions) API, for payouts use [POST /payouts/!availablePaymentOptions](#operations-Outgoing_payments-availablePaymentOptions) API. */
private final String paymentOperatorCode;
@Override
public String getPaymentOperatorCode() {
return paymentOperatorCode;
}
/** Your customer e-mail address in RFC 5322 format that is used for identification of the customer's payins. */
private final Optional emailAddress;
@Override
public Optional getEmailAddress() {
return emailAddress;
}
@Override public PaymentMethodCode getPaymentMethodCode() { return PAYMENT_METHOD_CODE; }
private final int hashCode;
private final String toString;
private CodePaymentMethodImpl(BuilderImpl builder) {
this.account = Optional.ofNullable(builder.account);
this.paymentOperatorCode = Objects.requireNonNull(builder.paymentOperatorCode, "Property 'paymentOperatorCode' is required.");
this.emailAddress = Optional.ofNullable(builder.emailAddress);
this.hashCode = Objects.hash(account, paymentOperatorCode, emailAddress);
this.toString = builder.type + "(" +
"account=" + account +
", paymentOperatorCode=" + paymentOperatorCode +
", emailAddress=" + emailAddress +
')';
}
@Override
public int hashCode() {
return hashCode;
}
@Override
public boolean equals(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof CodePaymentMethodImpl)) {
return false;
}
CodePaymentMethodImpl that = (CodePaymentMethodImpl) obj;
if (!Objects.equals(this.account, that.account)) return false;
if (!Objects.equals(this.paymentOperatorCode, that.paymentOperatorCode)) return false;
if (!Objects.equals(this.emailAddress, that.emailAddress)) return false;
return true;
}
@Override
public String toString() {
return toString;
}
/** Builder for {@link CodePaymentMethod} model class. */
public static class BuilderImpl implements CodePaymentMethod.Builder {
private AccountPayinRequestCodePayment account = null;
private String paymentOperatorCode = null;
private String emailAddress = null;
private final String type;
public BuilderImpl(String type) {
this.type = type;
}
public BuilderImpl() {
this("CodePaymentMethod");
}
/**
* Set {@link CodePaymentMethod#getAccount} property.
*
*
*/
@Override
public BuilderImpl account(AccountPayinRequestCodePayment account) {
this.account = account;
return this;
}
/**
* Set {@link CodePaymentMethod#getPaymentOperatorCode} property.
*
* One of following can serve as Payment Operator:
* Financial or other institution (such as bank, card payment processor, ...) that manages transactions for your customers
* Mobile wallet
* Blockchain protocol for crypto currency payments
Customer is informed with the payment instructions where funds have to be transferred efficiently based on the selected Payment operator.
For getting list of the available payment options for payins use [POST /payins/!availablePaymentOptions](#operations-Incoming_payments-availablePaymentOptions) API, for payouts use [POST /payouts/!availablePaymentOptions](#operations-Outgoing_payments-availablePaymentOptions) API.
*/
@Override
public BuilderImpl paymentOperatorCode(String paymentOperatorCode) {
this.paymentOperatorCode = paymentOperatorCode;
return this;
}
/**
* Set {@link CodePaymentMethod#getEmailAddress} property.
*
* Your customer e-mail address in RFC 5322 format that is used for identification of the customer's payins.
*/
@Override
public BuilderImpl emailAddress(String emailAddress) {
this.emailAddress = emailAddress;
return this;
}
/**
* Create new instance of {@link CodePaymentMethod} model class with the builder instance properties.
*
* @throws NullPointerException in case required properties are not specified.
*/
@Override
public CodePaymentMethodImpl build() {
return new CodePaymentMethodImpl(this);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy