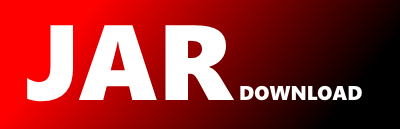
tech.carpentum.sdk.payment.internal.generated.model.MoneyFeeImpl Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of payment-client-v2 Show documentation
Show all versions of payment-client-v2 Show documentation
Carpentum Payment system Java SDK
The newest version!
//THE FILE IS GENERATED, DO NOT MODIFY IT MANUALLY!!!
package tech.carpentum.sdk.payment.internal.generated.model;
import com.squareup.moshi.JsonClass;
import java.util.Objects;
import java.util.Optional;
import org.jetbrains.annotations.NotNull;
import tech.carpentum.sdk.payment.internal.generated.model.*;
import tech.carpentum.sdk.payment.model.*;
/** MoneyFee
*
* Fees charged for this payment.
*
*
*
* The model class is immutable.
* Use static {@link #builder} method to create a new {@link Builder} instance to build the model class instance.
*
*/
@JsonClass(generateAdapter = false)
public class MoneyFeeImpl implements MoneyFee {
/** Amount is accepted in the smallest currency unit. For fiat currencies the smallest currency unit are based on ISO 4217 (e.g. for USD two decimal places are available so amount can be accepted as 12.34).
The exceptions are IDR and INR currencies: zero amount in decimal places are allowed for them (e.g. 42.05 is not allowed, while 42 or 42.00 or 43 or 43.00 are allowed).
If more decimal places than supported is provided request will fail on HTTP 406 error (e.g. for USD the amount 12.345 will not be accepted). */
private final java.math.BigDecimal amount;
@Override
public java.math.BigDecimal getAmount() {
return amount;
}
private final CurrencyCode currencyCode;
@Override
public CurrencyCode getCurrencyCode() {
return currencyCode;
}
private final int hashCode;
private final String toString;
private MoneyFeeImpl(BuilderImpl builder) {
this.amount = Objects.requireNonNull(builder.amount, "Property 'amount' is required.");
this.currencyCode = Objects.requireNonNull(builder.currencyCode, "Property 'currencyCode' is required.");
this.hashCode = Objects.hash(amount, currencyCode);
this.toString = builder.type + "(" +
"amount=" + amount +
", currencyCode=" + currencyCode +
')';
}
@Override
public int hashCode() {
return hashCode;
}
@Override
public boolean equals(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof MoneyFeeImpl)) {
return false;
}
MoneyFeeImpl that = (MoneyFeeImpl) obj;
if (!Objects.equals(this.amount, that.amount)) return false;
if (!Objects.equals(this.currencyCode, that.currencyCode)) return false;
return true;
}
@Override
public String toString() {
return toString;
}
/** Builder for {@link MoneyFee} model class. */
public static class BuilderImpl implements MoneyFee.Builder {
private java.math.BigDecimal amount = null;
private CurrencyCode currencyCode = null;
private final String type;
public BuilderImpl(String type) {
this.type = type;
}
public BuilderImpl() {
this("MoneyFee");
}
/**
* Set {@link MoneyFee#getAmount} property.
*
* Amount is accepted in the smallest currency unit. For fiat currencies the smallest currency unit are based on ISO 4217 (e.g. for USD two decimal places are available so amount can be accepted as 12.34).
The exceptions are IDR and INR currencies: zero amount in decimal places are allowed for them (e.g. 42.05 is not allowed, while 42 or 42.00 or 43 or 43.00 are allowed).
If more decimal places than supported is provided request will fail on HTTP 406 error (e.g. for USD the amount 12.345 will not be accepted).
*/
@Override
public BuilderImpl amount(java.math.BigDecimal amount) {
this.amount = amount;
return this;
}
/**
* Set {@link MoneyFee#getCurrencyCode} property.
*
*
*/
@Override
public BuilderImpl currencyCode(CurrencyCode currencyCode) {
this.currencyCode = currencyCode;
return this;
}
/**
* Create new instance of {@link MoneyFee} model class with the builder instance properties.
*
* @throws NullPointerException in case required properties are not specified.
*/
@Override
public MoneyFeeImpl build() {
return new MoneyFeeImpl(this);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy