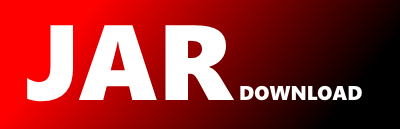
tech.carpentum.sdk.payment.internal.generated.model.NetBankingMethodResponseImpl Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of payment-client-v2 Show documentation
Show all versions of payment-client-v2 Show documentation
Carpentum Payment system Java SDK
The newest version!
//THE FILE IS GENERATED, DO NOT MODIFY IT MANUALLY!!!
package tech.carpentum.sdk.payment.internal.generated.model;
import com.squareup.moshi.JsonClass;
import java.util.Objects;
import java.util.Optional;
import org.jetbrains.annotations.NotNull;
import tech.carpentum.sdk.payment.internal.generated.model.*;
import tech.carpentum.sdk.payment.model.*;
/** NETBANKING
*
*
*
*
*
* The model class is immutable.
* Use static {@link #builder} method to create a new {@link Builder} instance to build the model class instance.
*
*/
@JsonClass(generateAdapter = false)
public class NetBankingMethodResponseImpl implements NetBankingMethodResponse {
private final IdPayin idPayin;
@Override
public IdPayin getIdPayin() {
return idPayin;
}
private final IdPayment idPayment;
@Override
public IdPayment getIdPayment() {
return idPayment;
}
private final MoneyPaymentResponse money;
@Override
public MoneyPaymentResponse getMoney() {
return money;
}
private final Optional vat;
@Override
public Optional getVat() {
return vat;
}
private final String merchantName;
@Override
public String getMerchantName() {
return merchantName;
}
/** Reference number of transaction. */
private final String reference;
@Override
public String getReference() {
return reference;
}
/** This is the URL where the customers will be redirected after completing a payment.
The URL must be either IP or domain-based. */
private final String returnUrl;
@Override
public String getReturnUrl() {
return returnUrl;
}
/** Virtual payment address where we expect that your customer sends funds to make a payment. This parameter is to be shown to your customer. */
private final Optional upiId;
@Override
public Optional getUpiId() {
return upiId;
}
/** It can be used as deep link button target (what is typically known as an intent trigger)
or to generate a QR code that can be scanned with any UPI enabled app. */
private final Optional deepLink;
@Override
public Optional getDeepLink() {
return deepLink;
}
private final Optional paymentOperator;
@Override
public Optional getPaymentOperator() {
return paymentOperator;
}
/** Date and time when payment was accepted. */
private final java.time.OffsetDateTime acceptedAt;
@Override
public java.time.OffsetDateTime getAcceptedAt() {
return acceptedAt;
}
/** Date and time of payment expiration. If no money has been transferred to this time, payment is considered failed and callback with status change event will shortly follow. */
private final java.time.OffsetDateTime expireAt;
@Override
public java.time.OffsetDateTime getExpireAt() {
return expireAt;
}
@Override public PaymentMethodCode getPaymentMethodCode() { return PAYMENT_METHOD_CODE; }
private final int hashCode;
private final String toString;
private NetBankingMethodResponseImpl(BuilderImpl builder) {
this.idPayin = Objects.requireNonNull(builder.idPayin, "Property 'idPayin' is required.");
this.idPayment = Objects.requireNonNull(builder.idPayment, "Property 'idPayment' is required.");
this.money = Objects.requireNonNull(builder.money, "Property 'money' is required.");
this.vat = Optional.ofNullable(builder.vat);
this.merchantName = Objects.requireNonNull(builder.merchantName, "Property 'merchantName' is required.");
this.reference = Objects.requireNonNull(builder.reference, "Property 'reference' is required.");
this.returnUrl = Objects.requireNonNull(builder.returnUrl, "Property 'returnUrl' is required.");
this.upiId = Optional.ofNullable(builder.upiId);
this.deepLink = Optional.ofNullable(builder.deepLink);
this.paymentOperator = Optional.ofNullable(builder.paymentOperator);
this.acceptedAt = Objects.requireNonNull(builder.acceptedAt, "Property 'acceptedAt' is required.");
this.expireAt = Objects.requireNonNull(builder.expireAt, "Property 'expireAt' is required.");
this.hashCode = Objects.hash(idPayin, idPayment, money, vat, merchantName, reference, returnUrl, upiId, deepLink, paymentOperator, acceptedAt, expireAt);
this.toString = builder.type + "(" +
"idPayin=" + idPayin +
", idPayment=" + idPayment +
", money=" + money +
", vat=" + vat +
", merchantName=" + merchantName +
", reference=" + reference +
", returnUrl=" + returnUrl +
", upiId=" + upiId +
", deepLink=" + deepLink +
", paymentOperator=" + paymentOperator +
", acceptedAt=" + acceptedAt +
", expireAt=" + expireAt +
')';
}
@Override
public int hashCode() {
return hashCode;
}
@Override
public boolean equals(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof NetBankingMethodResponseImpl)) {
return false;
}
NetBankingMethodResponseImpl that = (NetBankingMethodResponseImpl) obj;
if (!Objects.equals(this.idPayin, that.idPayin)) return false;
if (!Objects.equals(this.idPayment, that.idPayment)) return false;
if (!Objects.equals(this.money, that.money)) return false;
if (!Objects.equals(this.vat, that.vat)) return false;
if (!Objects.equals(this.merchantName, that.merchantName)) return false;
if (!Objects.equals(this.reference, that.reference)) return false;
if (!Objects.equals(this.returnUrl, that.returnUrl)) return false;
if (!Objects.equals(this.upiId, that.upiId)) return false;
if (!Objects.equals(this.deepLink, that.deepLink)) return false;
if (!Objects.equals(this.paymentOperator, that.paymentOperator)) return false;
if (!Objects.equals(this.acceptedAt, that.acceptedAt)) return false;
if (!Objects.equals(this.expireAt, that.expireAt)) return false;
return true;
}
@Override
public String toString() {
return toString;
}
/** Builder for {@link NetBankingMethodResponse} model class. */
public static class BuilderImpl implements NetBankingMethodResponse.Builder {
private IdPayin idPayin = null;
private IdPayment idPayment = null;
private MoneyPaymentResponse money = null;
private MoneyVat vat = null;
private String merchantName = null;
private String reference = null;
private String returnUrl = null;
private String upiId = null;
private String deepLink = null;
private PaymentOperatorIncoming paymentOperator = null;
private java.time.OffsetDateTime acceptedAt = null;
private java.time.OffsetDateTime expireAt = null;
private final String type;
public BuilderImpl(String type) {
this.type = type;
}
public BuilderImpl() {
this("NetBankingMethodResponse");
}
/**
* Set {@link NetBankingMethodResponse#getIdPayin} property.
*
*
*/
@Override
public BuilderImpl idPayin(IdPayin idPayin) {
this.idPayin = idPayin;
return this;
}
/**
* Set {@link NetBankingMethodResponse#getIdPayment} property.
*
*
*/
@Override
public BuilderImpl idPayment(IdPayment idPayment) {
this.idPayment = idPayment;
return this;
}
/**
* Set {@link NetBankingMethodResponse#getMoney} property.
*
*
*/
@Override
public BuilderImpl money(MoneyPaymentResponse money) {
this.money = money;
return this;
}
/**
* Set {@link NetBankingMethodResponse#getVat} property.
*
*
*/
@Override
public BuilderImpl vat(MoneyVat vat) {
this.vat = vat;
return this;
}
/**
* Set {@link NetBankingMethodResponse#getMerchantName} property.
*
*
*/
@Override
public BuilderImpl merchantName(String merchantName) {
this.merchantName = merchantName;
return this;
}
/**
* Set {@link NetBankingMethodResponse#getReference} property.
*
* Reference number of transaction.
*/
@Override
public BuilderImpl reference(String reference) {
this.reference = reference;
return this;
}
/**
* Set {@link NetBankingMethodResponse#getReturnUrl} property.
*
* This is the URL where the customers will be redirected after completing a payment.
The URL must be either IP or domain-based.
*/
@Override
public BuilderImpl returnUrl(String returnUrl) {
this.returnUrl = returnUrl;
return this;
}
/**
* Set {@link NetBankingMethodResponse#getUpiId} property.
*
* Virtual payment address where we expect that your customer sends funds to make a payment. This parameter is to be shown to your customer.
*/
@Override
public BuilderImpl upiId(String upiId) {
this.upiId = upiId;
return this;
}
/**
* Set {@link NetBankingMethodResponse#getDeepLink} property.
*
* It can be used as deep link button target (what is typically known as an intent trigger)
or to generate a QR code that can be scanned with any UPI enabled app.
*/
@Override
public BuilderImpl deepLink(String deepLink) {
this.deepLink = deepLink;
return this;
}
/**
* Set {@link NetBankingMethodResponse#getPaymentOperator} property.
*
*
*/
@Override
public BuilderImpl paymentOperator(PaymentOperatorIncoming paymentOperator) {
this.paymentOperator = paymentOperator;
return this;
}
/**
* Set {@link NetBankingMethodResponse#getAcceptedAt} property.
*
* Date and time when payment was accepted.
*/
@Override
public BuilderImpl acceptedAt(java.time.OffsetDateTime acceptedAt) {
this.acceptedAt = acceptedAt;
return this;
}
/**
* Set {@link NetBankingMethodResponse#getExpireAt} property.
*
* Date and time of payment expiration. If no money has been transferred to this time, payment is considered failed and callback with status change event will shortly follow.
*/
@Override
public BuilderImpl expireAt(java.time.OffsetDateTime expireAt) {
this.expireAt = expireAt;
return this;
}
/**
* Create new instance of {@link NetBankingMethodResponse} model class with the builder instance properties.
*
* @throws NullPointerException in case required properties are not specified.
*/
@Override
public NetBankingMethodResponseImpl build() {
return new NetBankingMethodResponseImpl(this);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy