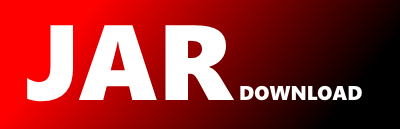
tech.carpentum.sdk.payment.internal.generated.model.PaymentProcessImpl Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of payment-client-v2 Show documentation
Show all versions of payment-client-v2 Show documentation
Carpentum Payment system Java SDK
//THE FILE IS GENERATED, DO NOT MODIFY IT MANUALLY!!!
package tech.carpentum.sdk.payment.internal.generated.model;
import com.squareup.moshi.JsonClass;
import java.util.Objects;
import java.util.Optional;
import org.jetbrains.annotations.NotNull;
import tech.carpentum.sdk.payment.internal.generated.model.*;
import tech.carpentum.sdk.payment.model.*;
/** PaymentProcess
*
*
*
*
*
* The model class is immutable.
* Use static {@link #builder} method to create a new {@link Builder} instance to build the model class instance.
*
*/
@JsonClass(generateAdapter = false)
public class PaymentProcessImpl implements PaymentProcess {
private final PaymentStatus status;
@Override
public PaymentStatus getStatus() {
return status;
}
private final Optional failureReasons;
@Override
public Optional getFailureReasons() {
return failureReasons;
}
/** Date and time when payment was accepted by platform. */
private final java.time.OffsetDateTime createdAt;
@Override
public java.time.OffsetDateTime getCreatedAt() {
return createdAt;
}
/** Date and time when payment was processed by platform. */
private final Optional processedAt;
@Override
public Optional getProcessedAt() {
return processedAt;
}
/** This flag is set to `true` when payment was done with testing merchant or testing channel. */
private final Boolean isTest;
@Override
public Boolean getIsTest() {
return isTest;
}
private final Optional processorStatus;
@Override
public Optional getProcessorStatus() {
return processorStatus;
}
private final int hashCode;
private final String toString;
private PaymentProcessImpl(BuilderImpl builder) {
this.status = Objects.requireNonNull(builder.status, "Property 'status' is required.");
this.failureReasons = Optional.ofNullable(builder.failureReasons);
this.createdAt = Objects.requireNonNull(builder.createdAt, "Property 'createdAt' is required.");
this.processedAt = Optional.ofNullable(builder.processedAt);
this.isTest = Objects.requireNonNull(builder.isTest, "Property 'isTest' is required.");
this.processorStatus = Optional.ofNullable(builder.processorStatus);
this.hashCode = Objects.hash(status, failureReasons, createdAt, processedAt, isTest, processorStatus);
this.toString = builder.type + "(" +
"status=" + status +
", failureReasons=" + failureReasons +
", createdAt=" + createdAt +
", processedAt=" + processedAt +
", isTest=" + isTest +
", processorStatus=" + processorStatus +
')';
}
@Override
public int hashCode() {
return hashCode;
}
@Override
public boolean equals(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof PaymentProcessImpl)) {
return false;
}
PaymentProcessImpl that = (PaymentProcessImpl) obj;
if (!Objects.equals(this.status, that.status)) return false;
if (!Objects.equals(this.failureReasons, that.failureReasons)) return false;
if (!Objects.equals(this.createdAt, that.createdAt)) return false;
if (!Objects.equals(this.processedAt, that.processedAt)) return false;
if (!Objects.equals(this.isTest, that.isTest)) return false;
if (!Objects.equals(this.processorStatus, that.processorStatus)) return false;
return true;
}
@Override
public String toString() {
return toString;
}
/** Builder for {@link PaymentProcess} model class. */
public static class BuilderImpl implements PaymentProcess.Builder {
private PaymentStatus status = null;
private FailureReasons failureReasons = null;
private java.time.OffsetDateTime createdAt = null;
private java.time.OffsetDateTime processedAt = null;
private Boolean isTest = null;
private ProcessorStatus processorStatus = null;
private final String type;
public BuilderImpl(String type) {
this.type = type;
}
public BuilderImpl() {
this("PaymentProcess");
}
/**
* Set {@link PaymentProcess#getStatus} property.
*
*
*/
@Override
public BuilderImpl status(PaymentStatus status) {
this.status = status;
return this;
}
/**
* Set {@link PaymentProcess#getFailureReasons} property.
*
*
*/
@Override
public BuilderImpl failureReasons(FailureReasons failureReasons) {
this.failureReasons = failureReasons;
return this;
}
/**
* Set {@link PaymentProcess#getCreatedAt} property.
*
* Date and time when payment was accepted by platform.
*/
@Override
public BuilderImpl createdAt(java.time.OffsetDateTime createdAt) {
this.createdAt = createdAt;
return this;
}
/**
* Set {@link PaymentProcess#getProcessedAt} property.
*
* Date and time when payment was processed by platform.
*/
@Override
public BuilderImpl processedAt(java.time.OffsetDateTime processedAt) {
this.processedAt = processedAt;
return this;
}
/**
* Set {@link PaymentProcess#getIsTest} property.
*
* This flag is set to `true` when payment was done with testing merchant or testing channel.
*/
@Override
public BuilderImpl isTest(Boolean isTest) {
this.isTest = isTest;
return this;
}
/**
* Set {@link PaymentProcess#getProcessorStatus} property.
*
*
*/
@Override
public BuilderImpl processorStatus(ProcessorStatus processorStatus) {
this.processorStatus = processorStatus;
return this;
}
/**
* Create new instance of {@link PaymentProcess} model class with the builder instance properties.
*
* @throws NullPointerException in case required properties are not specified.
*/
@Override
public PaymentProcessImpl build() {
return new PaymentProcessImpl(this);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy