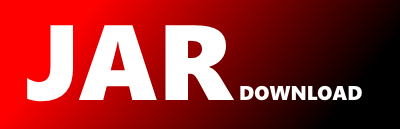
tech.carpentum.sdk.payment.internal.generated.model.UpiQRMethodResponseImpl Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of payment-client-v2 Show documentation
Show all versions of payment-client-v2 Show documentation
Carpentum Payment system Java SDK
The newest version!
//THE FILE IS GENERATED, DO NOT MODIFY IT MANUALLY!!!
package tech.carpentum.sdk.payment.internal.generated.model;
import com.squareup.moshi.JsonClass;
import java.util.Objects;
import java.util.Optional;
import org.jetbrains.annotations.NotNull;
import tech.carpentum.sdk.payment.internal.generated.model.*;
import tech.carpentum.sdk.payment.model.*;
/** UPIQR
*
*
*
*
*
* The model class is immutable.
* Use static {@link #builder} method to create a new {@link Builder} instance to build the model class instance.
*
*/
@JsonClass(generateAdapter = false)
public class UpiQRMethodResponseImpl implements UpiQRMethodResponse {
private final IdPayin idPayin;
@Override
public IdPayin getIdPayin() {
return idPayin;
}
private final IdPayment idPayment;
@Override
public IdPayment getIdPayment() {
return idPayment;
}
private final Optional accountCustomer;
@Override
public Optional getAccountCustomer() {
return accountCustomer;
}
private final MoneyPaymentResponse money;
@Override
public MoneyPaymentResponse getMoney() {
return money;
}
private final Optional vat;
@Override
public Optional getVat() {
return vat;
}
private final String merchantName;
@Override
public String getMerchantName() {
return merchantName;
}
/** Reference number of transaction. */
private final String reference;
@Override
public String getReference() {
return reference;
}
private final Optional externalReference;
@Override
public Optional getExternalReference() {
return externalReference;
}
/** The name of the QR code image to be scanned by a wallet or payment service compatible with this payment method. The QR code of the image can be labeled by qrName to increase the clarity of the payment instruction.
If this parameter contains any value, include it in the payment instructions for your customer. */
private final String qrName;
@Override
public String getQrName() {
return qrName;
}
/** The URL of the QR code image to be scanned by a wallet or payment service compatible with this payment method. The QR code encodes the instructions how make a payment.
If this parameter contains any value, include it in the payment instructions for your customer. */
private final String qrCode;
@Override
public String getQrCode() {
return qrCode;
}
/** It can be used as deep link button target (what is typically known as an intent trigger)
or to generate a QR code that can be scanned with any UPI enabled app. */
private final Optional upiQrDeepLink;
@Override
public Optional getUpiQrDeepLink() {
return upiQrDeepLink;
}
private final Optional paymentOperator;
@Override
public Optional getPaymentOperator() {
return paymentOperator;
}
/** Virtual payment address where we expect that your customer sends funds to make a payment. This parameter is to be shown to your customer. */
private final Optional upiId;
@Override
public Optional getUpiId() {
return upiId;
}
/** Virtual payment address of the customer on UPI (Unified Payment Interface) */
private final Optional upiIdCustomer;
@Override
public Optional getUpiIdCustomer() {
return upiIdCustomer;
}
/** Your customer mobile phone number in full international telephone number format, including country code. */
private final Optional phoneNumber;
@Override
public Optional getPhoneNumber() {
return phoneNumber;
}
/** This is the URL where the customers will be redirected after completing a payment.
The URL must be either IP or domain-based. */
private final String returnUrl;
@Override
public String getReturnUrl() {
return returnUrl;
}
/** Date and time when payment was accepted. */
private final java.time.OffsetDateTime acceptedAt;
@Override
public java.time.OffsetDateTime getAcceptedAt() {
return acceptedAt;
}
/** Date and time of payment expiration. If no money has been transferred to this time, payment is considered failed and callback with status change event will shortly follow. */
private final java.time.OffsetDateTime expireAt;
@Override
public java.time.OffsetDateTime getExpireAt() {
return expireAt;
}
@Override public PaymentMethodCode getPaymentMethodCode() { return PAYMENT_METHOD_CODE; }
private final int hashCode;
private final String toString;
private UpiQRMethodResponseImpl(BuilderImpl builder) {
this.idPayin = Objects.requireNonNull(builder.idPayin, "Property 'idPayin' is required.");
this.idPayment = Objects.requireNonNull(builder.idPayment, "Property 'idPayment' is required.");
this.accountCustomer = Optional.ofNullable(builder.accountCustomer);
this.money = Objects.requireNonNull(builder.money, "Property 'money' is required.");
this.vat = Optional.ofNullable(builder.vat);
this.merchantName = Objects.requireNonNull(builder.merchantName, "Property 'merchantName' is required.");
this.reference = Objects.requireNonNull(builder.reference, "Property 'reference' is required.");
this.externalReference = Optional.ofNullable(builder.externalReference);
this.qrName = Objects.requireNonNull(builder.qrName, "Property 'qrName' is required.");
this.qrCode = Objects.requireNonNull(builder.qrCode, "Property 'qrCode' is required.");
this.upiQrDeepLink = Optional.ofNullable(builder.upiQrDeepLink);
this.paymentOperator = Optional.ofNullable(builder.paymentOperator);
this.upiId = Optional.ofNullable(builder.upiId);
this.upiIdCustomer = Optional.ofNullable(builder.upiIdCustomer);
this.phoneNumber = Optional.ofNullable(builder.phoneNumber);
this.returnUrl = Objects.requireNonNull(builder.returnUrl, "Property 'returnUrl' is required.");
this.acceptedAt = Objects.requireNonNull(builder.acceptedAt, "Property 'acceptedAt' is required.");
this.expireAt = Objects.requireNonNull(builder.expireAt, "Property 'expireAt' is required.");
this.hashCode = Objects.hash(idPayin, idPayment, accountCustomer, money, vat, merchantName, reference, externalReference, qrName, qrCode, upiQrDeepLink, paymentOperator, upiId, upiIdCustomer, phoneNumber, returnUrl, acceptedAt, expireAt);
this.toString = builder.type + "(" +
"idPayin=" + idPayin +
", idPayment=" + idPayment +
", accountCustomer=" + accountCustomer +
", money=" + money +
", vat=" + vat +
", merchantName=" + merchantName +
", reference=" + reference +
", externalReference=" + externalReference +
", qrName=" + qrName +
", qrCode=" + qrCode +
", upiQrDeepLink=" + upiQrDeepLink +
", paymentOperator=" + paymentOperator +
", upiId=" + upiId +
", upiIdCustomer=" + upiIdCustomer +
", phoneNumber=" + phoneNumber +
", returnUrl=" + returnUrl +
", acceptedAt=" + acceptedAt +
", expireAt=" + expireAt +
')';
}
@Override
public int hashCode() {
return hashCode;
}
@Override
public boolean equals(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof UpiQRMethodResponseImpl)) {
return false;
}
UpiQRMethodResponseImpl that = (UpiQRMethodResponseImpl) obj;
if (!Objects.equals(this.idPayin, that.idPayin)) return false;
if (!Objects.equals(this.idPayment, that.idPayment)) return false;
if (!Objects.equals(this.accountCustomer, that.accountCustomer)) return false;
if (!Objects.equals(this.money, that.money)) return false;
if (!Objects.equals(this.vat, that.vat)) return false;
if (!Objects.equals(this.merchantName, that.merchantName)) return false;
if (!Objects.equals(this.reference, that.reference)) return false;
if (!Objects.equals(this.externalReference, that.externalReference)) return false;
if (!Objects.equals(this.qrName, that.qrName)) return false;
if (!Objects.equals(this.qrCode, that.qrCode)) return false;
if (!Objects.equals(this.upiQrDeepLink, that.upiQrDeepLink)) return false;
if (!Objects.equals(this.paymentOperator, that.paymentOperator)) return false;
if (!Objects.equals(this.upiId, that.upiId)) return false;
if (!Objects.equals(this.upiIdCustomer, that.upiIdCustomer)) return false;
if (!Objects.equals(this.phoneNumber, that.phoneNumber)) return false;
if (!Objects.equals(this.returnUrl, that.returnUrl)) return false;
if (!Objects.equals(this.acceptedAt, that.acceptedAt)) return false;
if (!Objects.equals(this.expireAt, that.expireAt)) return false;
return true;
}
@Override
public String toString() {
return toString;
}
/** Builder for {@link UpiQRMethodResponse} model class. */
public static class BuilderImpl implements UpiQRMethodResponse.Builder {
private IdPayin idPayin = null;
private IdPayment idPayment = null;
private AccountCustomerResponseUpiQR accountCustomer = null;
private MoneyPaymentResponse money = null;
private MoneyVat vat = null;
private String merchantName = null;
private String reference = null;
private ExternalReference externalReference = null;
private String qrName = null;
private String qrCode = null;
private String upiQrDeepLink = null;
private PaymentOperatorIncoming paymentOperator = null;
private String upiId = null;
private String upiIdCustomer = null;
private String phoneNumber = null;
private String returnUrl = null;
private java.time.OffsetDateTime acceptedAt = null;
private java.time.OffsetDateTime expireAt = null;
private final String type;
public BuilderImpl(String type) {
this.type = type;
}
public BuilderImpl() {
this("UpiQRMethodResponse");
}
/**
* Set {@link UpiQRMethodResponse#getIdPayin} property.
*
*
*/
@Override
public BuilderImpl idPayin(IdPayin idPayin) {
this.idPayin = idPayin;
return this;
}
/**
* Set {@link UpiQRMethodResponse#getIdPayment} property.
*
*
*/
@Override
public BuilderImpl idPayment(IdPayment idPayment) {
this.idPayment = idPayment;
return this;
}
/**
* Set {@link UpiQRMethodResponse#getAccountCustomer} property.
*
*
*/
@Override
public BuilderImpl accountCustomer(AccountCustomerResponseUpiQR accountCustomer) {
this.accountCustomer = accountCustomer;
return this;
}
/**
* Set {@link UpiQRMethodResponse#getMoney} property.
*
*
*/
@Override
public BuilderImpl money(MoneyPaymentResponse money) {
this.money = money;
return this;
}
/**
* Set {@link UpiQRMethodResponse#getVat} property.
*
*
*/
@Override
public BuilderImpl vat(MoneyVat vat) {
this.vat = vat;
return this;
}
/**
* Set {@link UpiQRMethodResponse#getMerchantName} property.
*
*
*/
@Override
public BuilderImpl merchantName(String merchantName) {
this.merchantName = merchantName;
return this;
}
/**
* Set {@link UpiQRMethodResponse#getReference} property.
*
* Reference number of transaction.
*/
@Override
public BuilderImpl reference(String reference) {
this.reference = reference;
return this;
}
/**
* Set {@link UpiQRMethodResponse#getExternalReference} property.
*
*
*/
@Override
public BuilderImpl externalReference(ExternalReference externalReference) {
this.externalReference = externalReference;
return this;
}
/**
* Set {@link UpiQRMethodResponse#getQrName} property.
*
* The name of the QR code image to be scanned by a wallet or payment service compatible with this payment method. The QR code of the image can be labeled by qrName to increase the clarity of the payment instruction.
If this parameter contains any value, include it in the payment instructions for your customer.
*/
@Override
public BuilderImpl qrName(String qrName) {
this.qrName = qrName;
return this;
}
/**
* Set {@link UpiQRMethodResponse#getQrCode} property.
*
* The URL of the QR code image to be scanned by a wallet or payment service compatible with this payment method. The QR code encodes the instructions how make a payment.
If this parameter contains any value, include it in the payment instructions for your customer.
*/
@Override
public BuilderImpl qrCode(String qrCode) {
this.qrCode = qrCode;
return this;
}
/**
* Set {@link UpiQRMethodResponse#getUpiQrDeepLink} property.
*
* It can be used as deep link button target (what is typically known as an intent trigger)
or to generate a QR code that can be scanned with any UPI enabled app.
*/
@Override
public BuilderImpl upiQrDeepLink(String upiQrDeepLink) {
this.upiQrDeepLink = upiQrDeepLink;
return this;
}
/**
* Set {@link UpiQRMethodResponse#getPaymentOperator} property.
*
*
*/
@Override
public BuilderImpl paymentOperator(PaymentOperatorIncoming paymentOperator) {
this.paymentOperator = paymentOperator;
return this;
}
/**
* Set {@link UpiQRMethodResponse#getUpiId} property.
*
* Virtual payment address where we expect that your customer sends funds to make a payment. This parameter is to be shown to your customer.
*/
@Override
public BuilderImpl upiId(String upiId) {
this.upiId = upiId;
return this;
}
/**
* Set {@link UpiQRMethodResponse#getUpiIdCustomer} property.
*
* Virtual payment address of the customer on UPI (Unified Payment Interface)
*/
@Override
public BuilderImpl upiIdCustomer(String upiIdCustomer) {
this.upiIdCustomer = upiIdCustomer;
return this;
}
/**
* Set {@link UpiQRMethodResponse#getPhoneNumber} property.
*
* Your customer mobile phone number in full international telephone number format, including country code.
*/
@Override
public BuilderImpl phoneNumber(String phoneNumber) {
this.phoneNumber = phoneNumber;
return this;
}
/**
* Set {@link UpiQRMethodResponse#getReturnUrl} property.
*
* This is the URL where the customers will be redirected after completing a payment.
The URL must be either IP or domain-based.
*/
@Override
public BuilderImpl returnUrl(String returnUrl) {
this.returnUrl = returnUrl;
return this;
}
/**
* Set {@link UpiQRMethodResponse#getAcceptedAt} property.
*
* Date and time when payment was accepted.
*/
@Override
public BuilderImpl acceptedAt(java.time.OffsetDateTime acceptedAt) {
this.acceptedAt = acceptedAt;
return this;
}
/**
* Set {@link UpiQRMethodResponse#getExpireAt} property.
*
* Date and time of payment expiration. If no money has been transferred to this time, payment is considered failed and callback with status change event will shortly follow.
*/
@Override
public BuilderImpl expireAt(java.time.OffsetDateTime expireAt) {
this.expireAt = expireAt;
return this;
}
/**
* Create new instance of {@link UpiQRMethodResponse} model class with the builder instance properties.
*
* @throws NullPointerException in case required properties are not specified.
*/
@Override
public UpiQRMethodResponseImpl build() {
return new UpiQRMethodResponseImpl(this);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy