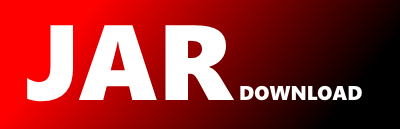
tech.carpentum.sdk.payment.internal.generated.model.VaPayMethodImpl Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of payment-client-v2 Show documentation
Show all versions of payment-client-v2 Show documentation
Carpentum Payment system Java SDK
The newest version!
//THE FILE IS GENERATED, DO NOT MODIFY IT MANUALLY!!!
package tech.carpentum.sdk.payment.internal.generated.model;
import com.squareup.moshi.JsonClass;
import java.util.Objects;
import java.util.Optional;
import org.jetbrains.annotations.NotNull;
import tech.carpentum.sdk.payment.internal.generated.model.*;
import tech.carpentum.sdk.payment.model.*;
/** VAPAY
*
* Virtual Accounts. Payment method which requires customer to copy Payment instruction with generated Virtual Account from the Payment application right after the payment is submitted and create the Payment transfer using the instructions within customer's own payment service such as Internet or mobile banking, wallet or ATM.
One Virtual account cannot be used for sending funds repeatedly.
*
*
*
* The model class is immutable.
* Use static {@link #builder} method to create a new {@link Builder} instance to build the model class instance.
*
*/
@JsonClass(generateAdapter = false)
public class VaPayMethodImpl implements VaPayMethod {
private final Optional account;
@Override
public Optional getAccount() {
return account;
}
/** One of following can serve as Payment Operator:
* Financial or other institution (such as bank, card payment processor, ...) that manages transactions for your customers
* Mobile wallet
* Blockchain protocol for crypto currency payments
Customer is informed with the payment instructions where funds have to be transferred efficiently based on the selected Payment operator.
For getting list of the available payment options for payins use [POST /payins/!availablePaymentOptions](#operations-Incoming_payments-availablePaymentOptions) API, for payouts use [POST /payouts/!availablePaymentOptions](#operations-Outgoing_payments-availablePaymentOptions) API.
If currency is KRW or TWD, then paymentOperatorCode field is optional. Otherwise, it is required.
*/
private final Optional paymentOperatorCode;
@Override
public Optional getPaymentOperatorCode() {
return paymentOperatorCode;
}
/** Your customer e-mail address in RFC 5322 format that is used for identification of the customer's payins. */
private final Optional emailAddress;
@Override
public Optional getEmailAddress() {
return emailAddress;
}
@Override public PaymentMethodCode getPaymentMethodCode() { return PAYMENT_METHOD_CODE; }
private final int hashCode;
private final String toString;
private VaPayMethodImpl(BuilderImpl builder) {
this.account = Optional.ofNullable(builder.account);
this.paymentOperatorCode = Optional.ofNullable(builder.paymentOperatorCode);
this.emailAddress = Optional.ofNullable(builder.emailAddress);
this.hashCode = Objects.hash(account, paymentOperatorCode, emailAddress);
this.toString = builder.type + "(" +
"account=" + account +
", paymentOperatorCode=" + paymentOperatorCode +
", emailAddress=" + emailAddress +
')';
}
@Override
public int hashCode() {
return hashCode;
}
@Override
public boolean equals(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof VaPayMethodImpl)) {
return false;
}
VaPayMethodImpl that = (VaPayMethodImpl) obj;
if (!Objects.equals(this.account, that.account)) return false;
if (!Objects.equals(this.paymentOperatorCode, that.paymentOperatorCode)) return false;
if (!Objects.equals(this.emailAddress, that.emailAddress)) return false;
return true;
}
@Override
public String toString() {
return toString;
}
/** Builder for {@link VaPayMethod} model class. */
public static class BuilderImpl implements VaPayMethod.Builder {
private AccountPayinRequestVaPay account = null;
private String paymentOperatorCode = null;
private String emailAddress = null;
private final String type;
public BuilderImpl(String type) {
this.type = type;
}
public BuilderImpl() {
this("VaPayMethod");
}
/**
* Set {@link VaPayMethod#getAccount} property.
*
*
*/
@Override
public BuilderImpl account(AccountPayinRequestVaPay account) {
this.account = account;
return this;
}
/**
* Set {@link VaPayMethod#getPaymentOperatorCode} property.
*
* One of following can serve as Payment Operator:
* Financial or other institution (such as bank, card payment processor, ...) that manages transactions for your customers
* Mobile wallet
* Blockchain protocol for crypto currency payments
Customer is informed with the payment instructions where funds have to be transferred efficiently based on the selected Payment operator.
For getting list of the available payment options for payins use [POST /payins/!availablePaymentOptions](#operations-Incoming_payments-availablePaymentOptions) API, for payouts use [POST /payouts/!availablePaymentOptions](#operations-Outgoing_payments-availablePaymentOptions) API.
If currency is KRW or TWD, then paymentOperatorCode field is optional. Otherwise, it is required.
*/
@Override
public BuilderImpl paymentOperatorCode(String paymentOperatorCode) {
this.paymentOperatorCode = paymentOperatorCode;
return this;
}
/**
* Set {@link VaPayMethod#getEmailAddress} property.
*
* Your customer e-mail address in RFC 5322 format that is used for identification of the customer's payins.
*/
@Override
public BuilderImpl emailAddress(String emailAddress) {
this.emailAddress = emailAddress;
return this;
}
/**
* Create new instance of {@link VaPayMethod} model class with the builder instance properties.
*
* @throws NullPointerException in case required properties are not specified.
*/
@Override
public VaPayMethodImpl build() {
return new VaPayMethodImpl(this);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy