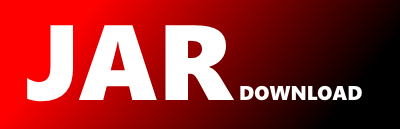
tech.carpentum.sdk.payment.model.AccountPayoutRequestBankTransfer Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of payment-client-v2 Show documentation
Show all versions of payment-client-v2 Show documentation
Carpentum Payment system Java SDK
The newest version!
//THE FILE IS GENERATED, DO NOT MODIFY IT MANUALLY!!!
package tech.carpentum.sdk.payment.model;
import com.squareup.moshi.JsonClass;
import java.util.Objects;
import java.util.Optional;
import org.jetbrains.annotations.NotNull;
import tech.carpentum.sdk.payment.internal.generated.model.*;
import tech.carpentum.sdk.payment.model.*;
/** AccountPayoutRequestBankTransfer
*
* Parameters of a customer's bank account where your customer would like his funds to be transferred.
The returned parameters are depended on the payout request currency.
*
*
*
* The model class is immutable.
* Use static {@link #builder} method to create a new {@link Builder} instance to build the model class instance.
*
*/
@JsonClass(generateAdapter = false)
public interface AccountPayoutRequestBankTransfer {
/** Name of a customer's bank account where your customer would like his funds to be transferred. */
@NotNull String getAccountName();
/** Number of a customer's bank account where your customer would like his funds to be transferred. */
@NotNull String getAccountNumber();
/** Account type of the receiving bank account.
* If payout currency is JPY, then accountType field is required and requested value has to be "savings", "checking", "private", "corporate", "general" or "current".
* If payout currency is ZAR, then accountType field is required and requested value has to be "savings", "current", "cheque", "transmission".
Otherwise, accountType is optional. */
@NotNull Optional getAccountType();
/** Bank code of the bank where your customer would like his funds to be transferred.
If currency is INR, then bankCode field is required and requested value has to be an Indian Financial System Code (IFSC bank code). Otherwise, it is optional. */
@NotNull Optional getBankCode();
/** Name of the bank where your customer would like his funds to be transferred. */
@NotNull Optional getBankName();
/** Branch name of the bank where your customer would like his funds to be transferred.
If payouts currency is JPY or TWD, then bankBranch field is required. Otherwise, it is optional. */
@NotNull Optional getBankBranch();
/** City of the bank where your customer would like his funds to be transferred. */
@NotNull Optional getBankCity();
/** Province of the bank where your customer would like his funds to be transferred. */
@NotNull Optional getBankProvince();
@NotNull static Builder builder(AccountPayoutRequestBankTransfer copyOf) {
Builder builder = builder();
builder.accountName(copyOf.getAccountName());
builder.accountNumber(copyOf.getAccountNumber());
builder.accountType(copyOf.getAccountType().orElse(null));
builder.bankCode(copyOf.getBankCode().orElse(null));
builder.bankName(copyOf.getBankName().orElse(null));
builder.bankBranch(copyOf.getBankBranch().orElse(null));
builder.bankCity(copyOf.getBankCity().orElse(null));
builder.bankProvince(copyOf.getBankProvince().orElse(null));
return builder;
}
@NotNull static Builder builder() {
return new AccountPayoutRequestBankTransferImpl.BuilderImpl();
}
/** Builder for {@link AccountPayoutRequestBankTransfer} model class. */
interface Builder {
/**
* Set {@link AccountPayoutRequestBankTransfer#getAccountName} property.
*
* Name of a customer's bank account where your customer would like his funds to be transferred.
*/
@NotNull Builder accountName(String accountName);
/**
* Set {@link AccountPayoutRequestBankTransfer#getAccountNumber} property.
*
* Number of a customer's bank account where your customer would like his funds to be transferred.
*/
@NotNull Builder accountNumber(String accountNumber);
/**
* Set {@link AccountPayoutRequestBankTransfer#getAccountType} property.
*
* Account type of the receiving bank account.
* If payout currency is JPY, then accountType field is required and requested value has to be "savings", "checking", "private", "corporate", "general" or "current".
* If payout currency is ZAR, then accountType field is required and requested value has to be "savings", "current", "cheque", "transmission".
Otherwise, accountType is optional.
*/
@NotNull Builder accountType(String accountType);
/**
* Set {@link AccountPayoutRequestBankTransfer#getBankCode} property.
*
* Bank code of the bank where your customer would like his funds to be transferred.
If currency is INR, then bankCode field is required and requested value has to be an Indian Financial System Code (IFSC bank code). Otherwise, it is optional.
*/
@NotNull Builder bankCode(String bankCode);
/**
* Set {@link AccountPayoutRequestBankTransfer#getBankName} property.
*
* Name of the bank where your customer would like his funds to be transferred.
*/
@NotNull Builder bankName(String bankName);
/**
* Set {@link AccountPayoutRequestBankTransfer#getBankBranch} property.
*
* Branch name of the bank where your customer would like his funds to be transferred.
If payouts currency is JPY or TWD, then bankBranch field is required. Otherwise, it is optional.
*/
@NotNull Builder bankBranch(String bankBranch);
/**
* Set {@link AccountPayoutRequestBankTransfer#getBankCity} property.
*
* City of the bank where your customer would like his funds to be transferred.
*/
@NotNull Builder bankCity(String bankCity);
/**
* Set {@link AccountPayoutRequestBankTransfer#getBankProvince} property.
*
* Province of the bank where your customer would like his funds to be transferred.
*/
@NotNull Builder bankProvince(String bankProvince);
/**
* Create new instance of {@link AccountPayoutRequestBankTransfer} model class with the builder instance properties.
*
* @throws NullPointerException in case required properties are not specified.
*/
@NotNull AccountPayoutRequestBankTransfer build();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy