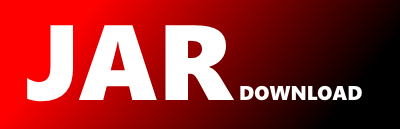
tech.carpentum.sdk.payment.model.AccountResponseOffline Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of payment-client-v2 Show documentation
Show all versions of payment-client-v2 Show documentation
Carpentum Payment system Java SDK
The newest version!
//THE FILE IS GENERATED, DO NOT MODIFY IT MANUALLY!!!
package tech.carpentum.sdk.payment.model;
import com.squareup.moshi.JsonClass;
import java.util.Objects;
import java.util.Optional;
import org.jetbrains.annotations.NotNull;
import tech.carpentum.sdk.payment.internal.generated.model.*;
import tech.carpentum.sdk.payment.model.*;
/** AccountResponseOffline
*
* Parameters of a bank account where we expect that your customer send funds to make a payment. These account parameters has to be provided to your customer in form of an payment instructions.
The returned parameters are depended on the payment method and currency your customer choose to pay.
*
*
*
* The model class is immutable.
* Use static {@link #builder} method to create a new {@link Builder} instance to build the model class instance.
*
*/
@JsonClass(generateAdapter = false)
public interface AccountResponseOffline extends AccountResponseWithBank {
/** Name of the bank account where we expect that your customer sends funds to make a payment.
This parameter is to be shown to your customer in the payment instructions. */
@NotNull String getAccountName();
/** Number of the bank account where we expect that your customer sends funds to make a payment.
This parameter is to be shown to your customer in the payment instructions. */
@NotNull String getAccountNumber();
/** Account type of the receiving bank account e.g. Normal / Current. It is used for payments in JPY currency. */
@NotNull Optional getAccountType();
/** Bank code of the bank where we expect that your customer sends funds to make a payment.
If this parameter contains any value then show it to your customer in the payment instructions. */
@NotNull Optional getBankCode();
/** Name of the bank where we expect that your customer sends funds to make a payment.
If this parameter contains any value then show it to your customer in the payment instructions. */
@NotNull Optional getBankName();
/** Branch name of the bank where we expect that your customer sends funds to make a payment.
If this parameter contains any value then show it to your customer in the payment instructions. */
@NotNull Optional getBankBranch();
/** City of the bank where we expect that your customer sends funds to make a payment.
If this parameter contains any value then show it to your customer in the payment instructions. */
@NotNull Optional getBankCity();
/** Province of the bank where we expect that your customer sends funds to make a payment.
If this parameter contains any value then show it to your customer in the payment instructions. */
@NotNull Optional getBankProvince();
@NotNull static Builder builder(AccountResponseOffline copyOf) {
Builder builder = builder();
builder.accountName(copyOf.getAccountName());
builder.accountNumber(copyOf.getAccountNumber());
builder.accountType(copyOf.getAccountType().orElse(null));
builder.bankCode(copyOf.getBankCode().orElse(null));
builder.bankName(copyOf.getBankName().orElse(null));
builder.bankBranch(copyOf.getBankBranch().orElse(null));
builder.bankCity(copyOf.getBankCity().orElse(null));
builder.bankProvince(copyOf.getBankProvince().orElse(null));
return builder;
}
@NotNull static Builder builder() {
return new AccountResponseOfflineImpl.BuilderImpl();
}
/** Builder for {@link AccountResponseOffline} model class. */
interface Builder {
/**
* Set {@link AccountResponseOffline#getAccountName} property.
*
* Name of the bank account where we expect that your customer sends funds to make a payment.
This parameter is to be shown to your customer in the payment instructions.
*/
@NotNull Builder accountName(String accountName);
/**
* Set {@link AccountResponseOffline#getAccountNumber} property.
*
* Number of the bank account where we expect that your customer sends funds to make a payment.
This parameter is to be shown to your customer in the payment instructions.
*/
@NotNull Builder accountNumber(String accountNumber);
/**
* Set {@link AccountResponseOffline#getAccountType} property.
*
* Account type of the receiving bank account e.g. Normal / Current. It is used for payments in JPY currency.
*/
@NotNull Builder accountType(String accountType);
/**
* Set {@link AccountResponseOffline#getBankCode} property.
*
* Bank code of the bank where we expect that your customer sends funds to make a payment.
If this parameter contains any value then show it to your customer in the payment instructions.
*/
@NotNull Builder bankCode(String bankCode);
/**
* Set {@link AccountResponseOffline#getBankName} property.
*
* Name of the bank where we expect that your customer sends funds to make a payment.
If this parameter contains any value then show it to your customer in the payment instructions.
*/
@NotNull Builder bankName(String bankName);
/**
* Set {@link AccountResponseOffline#getBankBranch} property.
*
* Branch name of the bank where we expect that your customer sends funds to make a payment.
If this parameter contains any value then show it to your customer in the payment instructions.
*/
@NotNull Builder bankBranch(String bankBranch);
/**
* Set {@link AccountResponseOffline#getBankCity} property.
*
* City of the bank where we expect that your customer sends funds to make a payment.
If this parameter contains any value then show it to your customer in the payment instructions.
*/
@NotNull Builder bankCity(String bankCity);
/**
* Set {@link AccountResponseOffline#getBankProvince} property.
*
* Province of the bank where we expect that your customer sends funds to make a payment.
If this parameter contains any value then show it to your customer in the payment instructions.
*/
@NotNull Builder bankProvince(String bankProvince);
/**
* Create new instance of {@link AccountResponseOffline} model class with the builder instance properties.
*
* @throws NullPointerException in case required properties are not specified.
*/
@NotNull AccountResponseOffline build();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy