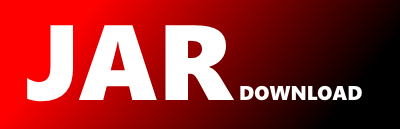
tech.carpentum.sdk.payment.model.BusinessValidationError Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of payment-client-v2 Show documentation
Show all versions of payment-client-v2 Show documentation
Carpentum Payment system Java SDK
The newest version!
//THE FILE IS GENERATED, DO NOT MODIFY IT MANUALLY!!!
package tech.carpentum.sdk.payment.model;
import org.jetbrains.annotations.NotNull;
import java.util.Objects;
import java.util.Optional;
/**
* Mark interface for business validation error model classes:
*
* - {@link PostAvailablePayoutOptionsError}
* - {@link GetFxRatesError}
* - {@link PostPayinsError}
* - {@link GetPayoutError}
* - {@link PostPayoutsError}
* - {@link GetPaymentOptionsError}
* - {@link PostAuthTokensError}
* - {@link PostAvailablePayinOptionsError}
* - {@link GetTopupError}
* - {@link PostAvailableTopUpOptionsError}
* - {@link GetPayinError}
*
*/
public abstract class BusinessValidationError {
public final @NotNull String code;
/** Optional error description. */
public final @NotNull Optional description;
/** Optional identification of the request attribute name that caused the error. */
public final @NotNull Optional attrCode;
/** Optional request attribute value that caused the error. */
public final @NotNull Optional attrValue;
private final int hashCode;
private final String toString;
protected > BusinessValidationError(Builder builder) {
this.code = Objects.requireNonNull(builder.code, "Property 'code' is required.");
this.description = Optional.ofNullable(builder.description);
this.attrCode = Optional.ofNullable(builder.attrCode);
this.attrValue = Optional.ofNullable(builder.attrValue);
this.hashCode = Objects.hash(code, description, attrCode, attrValue);
this.toString = getClass().getSimpleName() + "(" +
"code=" + code +
", description=" + description +
", attrCode=" + attrCode +
", attrValue=" + attrValue +
')';
}
@Override
public int hashCode() {
return hashCode;
}
@Override
public boolean equals(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!this.getClass().isAssignableFrom(obj.getClass())) {
return false;
}
BusinessValidationError that = (BusinessValidationError) obj;
if (!this.code.equals(that.code)) return false;
if (!this.description.equals(that.description)) return false;
if (!this.attrCode.equals(that.attrCode)) return false;
if (!this.attrValue.equals(that.attrValue)) return false;
return true;
}
@Override
public String toString() {
return toString;
}
public static abstract class Builder> {
private String code = null;
private String description = null;
private String attrCode = null;
private String attrValue = null;
protected Builder() {}
/**
* Set {@link BusinessValidationError#code} property.
*/
public Builder code(String code) {
this.code = code;
return this;
}
/**
* Set {@link BusinessValidationError#description} property.
*
* Optional error description.
*/
public Builder description(String description) {
this.description = description;
return this;
}
/**
* Set {@link BusinessValidationError#attrCode} property.
*
* Optional identification of the request attribute name that caused the error.
*/
public Builder attrCode(String attrCode) {
this.attrCode = attrCode;
return this;
}
/**
* Set {@link BusinessValidationError#attrValue} property.
*
* Optional identification of the request attribute name that caused the error.
*/
public Builder attrValue(String attrValue) {
this.attrValue = attrValue;
return this;
}
/**
* Create new instance of model class with the builder instance properties.
*
* @throws NullPointerException in case required properties are not specified.
*/
public abstract M build();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy