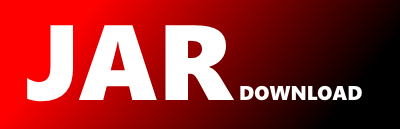
tech.carpentum.sdk.payment.model.FailureReasonCode Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of payment-client-v2 Show documentation
Show all versions of payment-client-v2 Show documentation
Carpentum Payment system Java SDK
The newest version!
//THE FILE IS GENERATED, DO NOT MODIFY IT MANUALLY!!!
package tech.carpentum.sdk.payment.model;
import com.squareup.moshi.JsonClass;
import java.util.Objects;
import java.util.Optional;
import org.jetbrains.annotations.NotNull;
import tech.carpentum.sdk.payment.internal.generated.model.*;
import tech.carpentum.sdk.payment.model.*;
/** FailureReasonCode
*
* * `SYSTEM` - Internal error. Contact support for more info.
* `PAYMENT_PROCESS_ERROR` - There has been error in payment process. Contact support for more info.
* `ENTITY_EXPIRED` - When entity (incoming payment, outgoing payment) has expired.
* `DIFFERENT_AMOUNT_CONFIRMED` - Customer sent different amount then requested.
* `CURRENCY_NOT_SUPPORTED` - The selected currency is not supported by merchant.
* `CURRENCY_PRECISION_EXCEEDED` - The provided payment amount exceeds the smallest fractional unit allowed for the specified currency.
* `PAYMENT_METHOD_NOT_FOUND` - No payment method has been found for seleceted payment criteria. Verify your product configuration and contact support for further details.
* `PAYMENT_METHOD_ERROR` - A processing error has been encountered for payment. An unexpected scenario with required operator intervention, contact support for further details.
* `PAYMENT_OPERATOR_NOT_FOUND` - The payment operator has not been found.
* `PAYMENT_OPERATOR_INVALID` - The selected payment operator is not supported for a used payment method.
* `PAYMENT_OPERATOR_UNAVAILABLE` - The selected payment operator is not available for a used payment method.
* `PAYMENT_CHANNEL_NO_ACTIVE_FOUND`- No active payment channel that can accept the payment has been found.
* `PAYMENT_CHANNEL_NO_OPENED_FOUND` - No opened payment channel that can accept the payment has been found.
* `PAYMENT_CHANNEL_NO_SEGMENT_FOUND` - No payment channel that can accept the payment with the specified segment has been found.
* `PAYMENT_CHANNEL_AMOUNT_LIMITS` - No payment channel with amount limits suitable for the payment amount has been found. Limits can be found with [`GET /payment-options`](#operations-Payments-getPaymentOptions) API.
* `PAYMENT_CHANNEL_DAILY_LIMITS` - All the suitable channels reached their daily limits on payment amount. Select different payment method or contact support for limits adjustments.
* `IP_DENIED` - The provided IP address is denied. It is either found in the blacklist or is not found in the whitelist.
* `BALANCE_INSUFFICIENT` - The balance is not sufficient for payment.
* `INVALID_ACCOUNT_NUMBER` - Account number is invalid or does not exist.
* `INVALID_ACCOUNT_NAME` - Account name provided in the payment does not match the name assigned to the account.
* `INVALID_PHONE_NUMBER` - Provided phone number is invalid or does not exist.
* `INVALID_ID_NUMBER` - Provided ID number is invalid or does not exist.
* `INCORRECT_CURRENCY` - Paid in different currency than payorder currency.
* `CUSTOMER_VERIFICATION_REJECTED` - Customer rejected payment verification.
* `CUSTOMER_VERIFICATION_FAILED` - Customer failed payment verification.
* `ACCOUNT_TYPE_INELIGIBLE` - Account type of provided account cannot be used for this payment.
*
*
*
* The model class is immutable.
*
* Use static {@link #of} method to create a new model class instance.
*/
public interface FailureReasonCode {
FailureReasonCode PAYMENT_METHOD_NOT_FOUND = FailureReasonCode.of("PAYMENT_METHOD_NOT_FOUND");
FailureReasonCode INVALID_ID_NUMBER = FailureReasonCode.of("INVALID_ID_NUMBER");
FailureReasonCode IP_DENIED = FailureReasonCode.of("IP_DENIED");
FailureReasonCode DIFFERENT_AMOUNT_CONFIRMED = FailureReasonCode.of("DIFFERENT_AMOUNT_CONFIRMED");
FailureReasonCode PAYMENT_OPERATOR_UNAVAILABLE = FailureReasonCode.of("PAYMENT_OPERATOR_UNAVAILABLE");
FailureReasonCode INVALID_ACCOUNT_NUMBER = FailureReasonCode.of("INVALID_ACCOUNT_NUMBER");
FailureReasonCode CUSTOMER_VERIFICATION_FAILED = FailureReasonCode.of("CUSTOMER_VERIFICATION_FAILED");
FailureReasonCode INVALID_ACCOUNT_NAME = FailureReasonCode.of("INVALID_ACCOUNT_NAME");
FailureReasonCode PAYMENT_CHANNEL_NO_ACTIVE_FOUND = FailureReasonCode.of("PAYMENT_CHANNEL_NO_ACTIVE_FOUND");
FailureReasonCode PAYMENT_CHANNEL_NO_OPENED_FOUND = FailureReasonCode.of("PAYMENT_CHANNEL_NO_OPENED_FOUND");
FailureReasonCode SYSTEM = FailureReasonCode.of("SYSTEM");
FailureReasonCode CURRENCY_PRECISION_EXCEEDED = FailureReasonCode.of("CURRENCY_PRECISION_EXCEEDED");
FailureReasonCode CURRENCY_NOT_SUPPORTED = FailureReasonCode.of("CURRENCY_NOT_SUPPORTED");
FailureReasonCode BALANCE_INSUFFICIENT = FailureReasonCode.of("BALANCE_INSUFFICIENT");
FailureReasonCode INCORRECT_CURRENCY = FailureReasonCode.of("INCORRECT_CURRENCY");
FailureReasonCode PAYMENT_METHOD_ERROR = FailureReasonCode.of("PAYMENT_METHOD_ERROR");
FailureReasonCode PAYMENT_OPERATOR_INVALID = FailureReasonCode.of("PAYMENT_OPERATOR_INVALID");
FailureReasonCode PAYMENT_PROCESS_ERROR = FailureReasonCode.of("PAYMENT_PROCESS_ERROR");
FailureReasonCode CUSTOMER_VERIFICATION_REJECTED = FailureReasonCode.of("CUSTOMER_VERIFICATION_REJECTED");
FailureReasonCode PAYMENT_OPERATOR_NOT_FOUND = FailureReasonCode.of("PAYMENT_OPERATOR_NOT_FOUND");
FailureReasonCode PAYMENT_CHANNEL_DAILY_LIMITS = FailureReasonCode.of("PAYMENT_CHANNEL_DAILY_LIMITS");
FailureReasonCode ACCOUNT_TYPE_INELIGIBLE = FailureReasonCode.of("ACCOUNT_TYPE_INELIGIBLE");
FailureReasonCode PAYMENT_CHANNEL_AMOUNT_LIMITS = FailureReasonCode.of("PAYMENT_CHANNEL_AMOUNT_LIMITS");
FailureReasonCode ENTITY_EXPIRED = FailureReasonCode.of("ENTITY_EXPIRED");
FailureReasonCode PAYMENT_CHANNEL_NO_SEGMENT_FOUND = FailureReasonCode.of("PAYMENT_CHANNEL_NO_SEGMENT_FOUND");
FailureReasonCode INVALID_PHONE_NUMBER = FailureReasonCode.of("INVALID_PHONE_NUMBER");
@NotNull String getValue();
static FailureReasonCode of(@NotNull String value) {
return new FailureReasonCodeImpl(value);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy