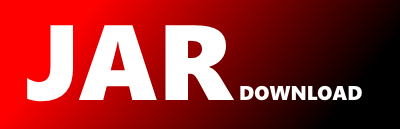
tech.carpentum.sdk.payment.model.PaymentProcess Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of payment-client-v2 Show documentation
Show all versions of payment-client-v2 Show documentation
Carpentum Payment system Java SDK
//THE FILE IS GENERATED, DO NOT MODIFY IT MANUALLY!!!
package tech.carpentum.sdk.payment.model;
import com.squareup.moshi.JsonClass;
import java.util.Objects;
import java.util.Optional;
import org.jetbrains.annotations.NotNull;
import tech.carpentum.sdk.payment.internal.generated.model.*;
import tech.carpentum.sdk.payment.model.*;
/** PaymentProcess
*
*
*
*
*
* The model class is immutable.
* Use static {@link #builder} method to create a new {@link Builder} instance to build the model class instance.
*
*/
@JsonClass(generateAdapter = false)
public interface PaymentProcess {
@NotNull PaymentStatus getStatus();
@NotNull Optional getFailureReasons();
/** Date and time when payment was accepted by platform. */
@NotNull java.time.OffsetDateTime getCreatedAt();
/** Date and time when payment was processed by platform. */
@NotNull Optional getProcessedAt();
/** This flag is set to `true` when payment was done with testing merchant or testing channel. */
@NotNull Boolean getIsTest();
@NotNull Optional getProcessorStatus();
@NotNull static Builder builder(PaymentProcess copyOf) {
Builder builder = builder();
builder.status(copyOf.getStatus());
builder.failureReasons(copyOf.getFailureReasons().orElse(null));
builder.createdAt(copyOf.getCreatedAt());
builder.processedAt(copyOf.getProcessedAt().orElse(null));
builder.isTest(copyOf.getIsTest());
builder.processorStatus(copyOf.getProcessorStatus().orElse(null));
return builder;
}
@NotNull static Builder builder() {
return new PaymentProcessImpl.BuilderImpl();
}
/** Builder for {@link PaymentProcess} model class. */
interface Builder {
/**
* Set {@link PaymentProcess#getStatus} property.
*
*
*/
@NotNull Builder status(PaymentStatus status);
/**
* Set {@link PaymentProcess#getFailureReasons} property.
*
*
*/
@NotNull Builder failureReasons(FailureReasons failureReasons);
/**
* Set {@link PaymentProcess#getCreatedAt} property.
*
* Date and time when payment was accepted by platform.
*/
@NotNull Builder createdAt(java.time.OffsetDateTime createdAt);
/**
* Set {@link PaymentProcess#getProcessedAt} property.
*
* Date and time when payment was processed by platform.
*/
@NotNull Builder processedAt(java.time.OffsetDateTime processedAt);
/**
* Set {@link PaymentProcess#getIsTest} property.
*
* This flag is set to `true` when payment was done with testing merchant or testing channel.
*/
@NotNull Builder isTest(Boolean isTest);
/**
* Set {@link PaymentProcess#getProcessorStatus} property.
*
*
*/
@NotNull Builder processorStatus(ProcessorStatus processorStatus);
/**
* Create new instance of {@link PaymentProcess} model class with the builder instance properties.
*
* @throws NullPointerException in case required properties are not specified.
*/
@NotNull PaymentProcess build();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy