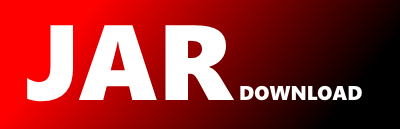
tech.carpentum.sdk.payment.model.QrisPayMethodResponse Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of payment-client-v2 Show documentation
Show all versions of payment-client-v2 Show documentation
Carpentum Payment system Java SDK
The newest version!
//THE FILE IS GENERATED, DO NOT MODIFY IT MANUALLY!!!
package tech.carpentum.sdk.payment.model;
import com.squareup.moshi.JsonClass;
import java.util.Objects;
import java.util.Optional;
import org.jetbrains.annotations.NotNull;
import tech.carpentum.sdk.payment.internal.generated.model.*;
import tech.carpentum.sdk.payment.model.*;
/** QRISPAY
*
*
*
*
*
* The model class is immutable.
* Use static {@link #builder} method to create a new {@link Builder} instance to build the model class instance.
*
*/
@JsonClass(generateAdapter = false)
public interface QrisPayMethodResponse extends PayinMethodResponse {
/** A discriminator value of property {@link #getPaymentMethodCode}. The model class extends {@link PayinMethodResponse}. */
PayinMethodResponse.PaymentMethodCode PAYMENT_METHOD_CODE = PayinMethodResponse.PaymentMethodCode.QRISPAY;
@NotNull IdPayin getIdPayin();
@NotNull IdPayment getIdPayment();
@NotNull MoneyPaymentResponse getMoney();
@NotNull Optional getVat();
@NotNull String getMerchantName();
/** Reference number of transaction. */
@NotNull String getReference();
/** The name of the QR code image to be scanned by a wallet or payment service compatible with this payment method. The QR code of the image can be labeled by qrName to increase the clarity of the payment instruction.
If this parameter contains any value, include it in the payment instructions for your customer. */
@NotNull String getQrName();
/** The URL of the QR code image to be scanned by a wallet or payment service compatible with this payment method. The QR code encodes the instructions how make a payment.
If this parameter contains any value, include it in the payment instructions for your customer. */
@NotNull String getQrCode();
/** This is the URL where the customers will be redirected after completing a payment.
The URL must be either IP or domain-based. */
@NotNull String getReturnUrl();
/** Date and time when payment was accepted. */
@NotNull java.time.OffsetDateTime getAcceptedAt();
/** Date and time of payment expiration. If no money has been transferred to this time, payment is considered failed and callback with status change event will shortly follow. */
@NotNull java.time.OffsetDateTime getExpireAt();
@NotNull static Builder builder(QrisPayMethodResponse copyOf) {
Builder builder = builder();
builder.idPayin(copyOf.getIdPayin());
builder.idPayment(copyOf.getIdPayment());
builder.money(copyOf.getMoney());
builder.vat(copyOf.getVat().orElse(null));
builder.merchantName(copyOf.getMerchantName());
builder.reference(copyOf.getReference());
builder.qrName(copyOf.getQrName());
builder.qrCode(copyOf.getQrCode());
builder.returnUrl(copyOf.getReturnUrl());
builder.acceptedAt(copyOf.getAcceptedAt());
builder.expireAt(copyOf.getExpireAt());
return builder;
}
@NotNull static Builder builder() {
return new QrisPayMethodResponseImpl.BuilderImpl();
}
/** Builder for {@link QrisPayMethodResponse} model class. */
interface Builder {
/**
* Set {@link QrisPayMethodResponse#getIdPayin} property.
*
*
*/
@NotNull Builder idPayin(IdPayin idPayin);
/**
* Set {@link QrisPayMethodResponse#getIdPayment} property.
*
*
*/
@NotNull Builder idPayment(IdPayment idPayment);
/**
* Set {@link QrisPayMethodResponse#getMoney} property.
*
*
*/
@NotNull Builder money(MoneyPaymentResponse money);
/**
* Set {@link QrisPayMethodResponse#getVat} property.
*
*
*/
@NotNull Builder vat(MoneyVat vat);
/**
* Set {@link QrisPayMethodResponse#getMerchantName} property.
*
*
*/
@NotNull Builder merchantName(String merchantName);
/**
* Set {@link QrisPayMethodResponse#getReference} property.
*
* Reference number of transaction.
*/
@NotNull Builder reference(String reference);
/**
* Set {@link QrisPayMethodResponse#getQrName} property.
*
* The name of the QR code image to be scanned by a wallet or payment service compatible with this payment method. The QR code of the image can be labeled by qrName to increase the clarity of the payment instruction.
If this parameter contains any value, include it in the payment instructions for your customer.
*/
@NotNull Builder qrName(String qrName);
/**
* Set {@link QrisPayMethodResponse#getQrCode} property.
*
* The URL of the QR code image to be scanned by a wallet or payment service compatible with this payment method. The QR code encodes the instructions how make a payment.
If this parameter contains any value, include it in the payment instructions for your customer.
*/
@NotNull Builder qrCode(String qrCode);
/**
* Set {@link QrisPayMethodResponse#getReturnUrl} property.
*
* This is the URL where the customers will be redirected after completing a payment.
The URL must be either IP or domain-based.
*/
@NotNull Builder returnUrl(String returnUrl);
/**
* Set {@link QrisPayMethodResponse#getAcceptedAt} property.
*
* Date and time when payment was accepted.
*/
@NotNull Builder acceptedAt(java.time.OffsetDateTime acceptedAt);
/**
* Set {@link QrisPayMethodResponse#getExpireAt} property.
*
* Date and time of payment expiration. If no money has been transferred to this time, payment is considered failed and callback with status change event will shortly follow.
*/
@NotNull Builder expireAt(java.time.OffsetDateTime expireAt);
/**
* Create new instance of {@link QrisPayMethodResponse} model class with the builder instance properties.
*
* @throws NullPointerException in case required properties are not specified.
*/
@NotNull QrisPayMethodResponse build();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy