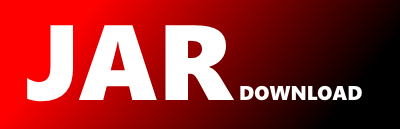
tech.codingzen.kata.konfig.StringParsers.kt Maven / Gradle / Ivy
package tech.codingzen.kata.konfig
import tech.codingzen.kata.konfig.annotations.InternalKataKonf
import tech.codingzen.kata.result.Res
import tech.codingzen.kata.result.ResDsl
import tech.codingzen.kata.result.res
import java.math.BigDecimal
import java.math.BigInteger
object StringParsers {
@PublishedApi
@InternalKataKonf
internal inline fun sourceParser(crossinline block: ResDsl.(SRC) -> T): (SRC) -> Res = { src -> res { block(src) } }
val string = sourceParser { it }
val byte =
sourceParser { s ->
s.toByteOrNull() ?: err("cannot parse String into Byte")
}
val uByte =
sourceParser { s ->
s.toUByteOrNull() ?: err("cannot parse String into UByte")
}
val short =
sourceParser { s ->
s.toShortOrNull() ?: err("cannot parse String into Short")
}
val uShort =
sourceParser { s ->
s.toUShortOrNull() ?: err("cannot parse String into UShort")
}
val int =
sourceParser { s ->
s.toIntOrNull() ?: err("cannot parse String into Int")
}
val uInt =
sourceParser { s ->
s.toUIntOrNull() ?: err("cannot parse String into UInt")
}
val long =
sourceParser { s ->
s.toLongOrNull() ?: err("cannot parse String into Long")
}
val uLong =
sourceParser { s ->
s.toULongOrNull() ?: err("cannot parse String into ULong")
}
val float =
sourceParser { s ->
s.toFloatOrNull() ?: err("cannot parse String into Float")
}
val double =
sourceParser { s ->
s.toDoubleOrNull() ?: err("cannot parse String into Double")
}
val boolean = sourceParser { s ->
s.lowercase().toBooleanStrictOrNull() ?: err("cannot parse String into Boolean")
}
val char = sourceParser { s ->
if (s.isEmpty()) err("cannot parse String into Char")
else s[0]
}
val bigInteger = sourceParser { s ->
s.toBigIntegerOrNull() ?: err("cannot parse String into BigInteger")
}
val bigDecimal = sourceParser { s ->
s.toBigDecimalOrNull() ?: err("cannot parse String into BigDecimal")
}
inline fun parser(): ResDsl.(String) -> V = { src ->
val x = when (V::class) {
String::class -> string(src).value()
Byte::class -> byte(src).value()
UByte::class -> uByte(src).value()
Short::class -> short(src).value()
UShort::class -> uShort(src).value()
Int::class -> int(src).value()
UInt::class -> uInt(src).value()
Long::class -> long(src).value()
ULong::class -> uLong(src).value()
Float::class -> float(src).value()
Double::class -> double(src).value()
Boolean::class -> boolean(src).value()
Char::class -> char(src).value()
BigInteger::class -> bigInteger(src).value()
BigDecimal::class -> bigDecimal(src).value()
else -> err("cannot decode String into type: ${V::class.qualifiedName}. Consider using a custom KonfigDecoder")
}
x as V
}
inline fun , reified T : V?> enum(): (String) -> Res = sourceParser { s ->
catch { enumValueOf(s) as T } context { "cannot parse String into enum ${V::class.qualifiedName}" }
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy