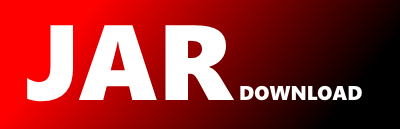
tech.msop.core.http.CssQueryMethodInterceptor Maven / Gradle / Ivy
package tech.msop.core.http;
import tech.msop.core.tool.constant.StringConstant;
import tech.msop.core.tool.utils.ConvertUtil;
import tech.msop.core.tool.utils.StringUtil;
import lombok.RequiredArgsConstructor;
import org.jsoup.nodes.Element;
import org.jsoup.nodes.TextNode;
import org.jsoup.select.Elements;
import org.jsoup.select.Selector;
import org.springframework.cglib.proxy.MethodInterceptor;
import org.springframework.cglib.proxy.MethodProxy;
import org.springframework.core.ResolvableType;
import org.springframework.core.convert.TypeDescriptor;
import javax.annotation.Nullable;
import java.lang.reflect.Field;
import java.lang.reflect.Method;
import java.util.ArrayList;
import java.util.Collection;
import java.util.HashSet;
import java.util.Set;
import java.util.regex.Matcher;
import java.util.regex.Pattern;
import java.util.stream.Collectors;
/**
* 代理模型
*
* @author ruozhuliufeng
*/
@RequiredArgsConstructor
public class CssQueryMethodInterceptor implements MethodInterceptor {
private final Class> clazz;
private final Element element;
@Nullable
@Override
public Object intercept(Object object, Method method, Object[] args, MethodProxy methodProxy) throws Throwable {
// 只处理 get 方法 is
String name = method.getName();
if (!StringUtil.startsWithIgnoreCase(name, StringConstant.GET)) {
return methodProxy.invokeSuper(object, args);
}
Field field = clazz.getDeclaredField(StringUtil.firstCharToLower(name.substring(3)));
CssQuery cssQuery = field.getAnnotation(CssQuery.class);
// 没有注解,不代理
if (cssQuery == null) {
return methodProxy.invokeSuper(object, args);
}
Class> returnType = method.getReturnType();
boolean isColl = Collection.class.isAssignableFrom(returnType);
String cssQueryValue = cssQuery.value();
// 是否为 bean 中 bean
boolean isInner = cssQuery.inner();
if (isInner) {
return proxyInner(cssQueryValue, method, returnType, isColl);
}
Object proxyValue = proxyValue(cssQueryValue, cssQuery, returnType, isColl);
if (String.class.isAssignableFrom(returnType)) {
return proxyValue;
}
// 用于读取 field 上的注解
TypeDescriptor typeDescriptor = new TypeDescriptor(field);
return ConvertUtil.convert(proxyValue, typeDescriptor);
}
@Nullable
private Object proxyValue(String cssQueryValue, CssQuery cssQuery, Class> returnType, boolean isColl) {
if (isColl) {
Elements elements = Selector.select(cssQueryValue, element);
Collection
© 2015 - 2025 Weber Informatics LLC | Privacy Policy