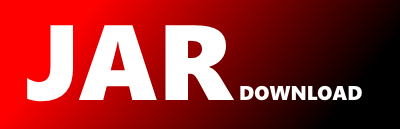
tech.picnic.errorprone.refasterrules.AssertJBigDecimalRulesRecipes Maven / Gradle / Ivy
Show all versions of error-prone-contrib Show documentation
package tech.picnic.errorprone.refasterrules;
import org.openrewrite.ExecutionContext;
import org.openrewrite.Preconditions;
import org.openrewrite.Recipe;
import org.openrewrite.TreeVisitor;
import org.openrewrite.internal.lang.NonNullApi;
import org.openrewrite.java.JavaParser;
import org.openrewrite.java.JavaTemplate;
import org.openrewrite.java.JavaVisitor;
import org.openrewrite.java.search.*;
import org.openrewrite.java.template.Primitive;
import org.openrewrite.java.template.function.*;
import org.openrewrite.java.template.internal.AbstractRefasterJavaVisitor;
import org.openrewrite.java.tree.*;
import javax.annotation.Generated;
import java.util.*;
import static org.openrewrite.java.template.internal.AbstractRefasterJavaVisitor.EmbeddingOption.*;
/**
* OpenRewrite recipes created for Refaster template {@code tech.picnic.errorprone.refasterrules.AssertJBigDecimalRules}.
*/
@SuppressWarnings("all")
@Generated("org.openrewrite.java.template.processor.RefasterTemplateProcessor")
public class AssertJBigDecimalRulesRecipes extends Recipe {
/**
* Instantiates a new instance.
*/
public AssertJBigDecimalRulesRecipes() {}
@Override
public String getDisplayName() {
return "Refaster rules related to AssertJ assertions over `BigDecimal`s";
}
@Override
public String getDescription() {
return "Note that, contrary to collections of Refaster rules for other `org.assertj.core.api.NumberAssert` subtypes, these rules do not rewrite to/from `BigDecimalAssert#isEqualTo(Object)` and `BigDecimalAssert#isNotEqualTo(Object)`. This is\n because `BigDecimal#equals(Object)` considers not only the numeric value of compared\n instances, but also their scale. As a result various seemingly straightforward transformations\n would actually subtly change the assertion's semantics. [Source](https://error-prone.picnic.tech/refasterrules/AssertJBigDecimalRules).";
}
@Override
public List getRecipeList() {
return Arrays.asList(
new AbstractBigDecimalAssertIsEqualByComparingToRecipe(),
new AbstractBigDecimalAssertIsNotEqualByComparingToRecipe()
);
}
/**
* OpenRewrite recipe created for Refaster template {@code AssertJBigDecimalRules.AbstractBigDecimalAssertIsEqualByComparingTo}.
*/
@SuppressWarnings("all")
@NonNullApi
@Generated("org.openrewrite.java.template.processor.RefasterTemplateProcessor")
public static class AbstractBigDecimalAssertIsEqualByComparingToRecipe extends Recipe {
/**
* Instantiates a new instance.
*/
public AbstractBigDecimalAssertIsEqualByComparingToRecipe() {}
@Override
public String getDisplayName() {
return "Refaster template `AssertJBigDecimalRules.AbstractBigDecimalAssertIsEqualByComparingTo`";
}
@Override
public String getDescription() {
return "Recipe created for the following Refaster template:\n```java\nstatic final class AbstractBigDecimalAssertIsEqualByComparingTo {\n \n @BeforeTemplate\n AbstractBigDecimalAssert> before(AbstractBigDecimalAssert> bigDecimalAssert, BigDecimal n) {\n return Refaster.anyOf(bigDecimalAssert.isCloseTo(n, offset(BigDecimal.ZERO)), bigDecimalAssert.isCloseTo(n, withPercentage(0)));\n }\n \n @AfterTemplate\n AbstractBigDecimalAssert> after(AbstractBigDecimalAssert> bigDecimalAssert, BigDecimal n) {\n return bigDecimalAssert.isEqualByComparingTo(n);\n }\n}\n```\n.";
}
@Override
public TreeVisitor, ExecutionContext> getVisitor() {
JavaVisitor javaVisitor = new AbstractRefasterJavaVisitor() {
final JavaTemplate before$0 = JavaTemplate
.builder("#{bigDecimalAssert:any(org.assertj.core.api.AbstractBigDecimalAssert>)}.isCloseTo(#{n:any(java.math.BigDecimal)}, org.assertj.core.data.Offset.offset(java.math.BigDecimal.ZERO))")
.javaParser(JavaParser.fromJavaVersion().classpath(JavaParser.runtimeClasspath()))
.build();
final JavaTemplate before$1 = JavaTemplate
.builder("#{bigDecimalAssert:any(org.assertj.core.api.AbstractBigDecimalAssert>)}.isCloseTo(#{n:any(java.math.BigDecimal)}, org.assertj.core.data.Percentage.withPercentage(0))")
.javaParser(JavaParser.fromJavaVersion().classpath(JavaParser.runtimeClasspath()))
.build();
final JavaTemplate after = JavaTemplate
.builder("#{bigDecimalAssert:any(org.assertj.core.api.AbstractBigDecimalAssert>)}.isEqualByComparingTo(#{n:any(java.math.BigDecimal)})")
.javaParser(JavaParser.fromJavaVersion().classpath(JavaParser.runtimeClasspath()))
.build();
@Override
public J visitMethodInvocation(J.MethodInvocation elem, ExecutionContext ctx) {
JavaTemplate.Matcher matcher;
if ((matcher = before$0.matcher(getCursor())).find()) {
maybeRemoveImport("org.assertj.core.data.Offset.offset");
return embed(
after.apply(getCursor(), elem.getCoordinates().replace(), matcher.parameter(0), matcher.parameter(1)),
getCursor(),
ctx,
SHORTEN_NAMES
);
}
if ((matcher = before$1.matcher(getCursor())).find()) {
maybeRemoveImport("org.assertj.core.data.Percentage.withPercentage");
return embed(
after.apply(getCursor(), elem.getCoordinates().replace(), matcher.parameter(0), matcher.parameter(1)),
getCursor(),
ctx,
SHORTEN_NAMES
);
}
return super.visitMethodInvocation(elem, ctx);
}
};
return Preconditions.check(
Preconditions.and(
new UsesType<>("java.math.BigDecimal", true),
new UsesType<>("org.assertj.core.api.AbstractBigDecimalAssert", true),
new UsesMethod<>("org.assertj.core.api.AbstractBigDecimalAssert isCloseTo(..)"),
Preconditions.or(
new UsesMethod<>("org.assertj.core.data.Offset offset(..)"),
new UsesMethod<>("org.assertj.core.data.Percentage withPercentage(..)")
)
),
javaVisitor
);
}
}
/**
* OpenRewrite recipe created for Refaster template {@code AssertJBigDecimalRules.AbstractBigDecimalAssertIsNotEqualByComparingTo}.
*/
@SuppressWarnings("all")
@NonNullApi
@Generated("org.openrewrite.java.template.processor.RefasterTemplateProcessor")
public static class AbstractBigDecimalAssertIsNotEqualByComparingToRecipe extends Recipe {
/**
* Instantiates a new instance.
*/
public AbstractBigDecimalAssertIsNotEqualByComparingToRecipe() {}
@Override
public String getDisplayName() {
return "Refaster template `AssertJBigDecimalRules.AbstractBigDecimalAssertIsNotEqualByComparingTo`";
}
@Override
public String getDescription() {
return "Recipe created for the following Refaster template:\n```java\nstatic final class AbstractBigDecimalAssertIsNotEqualByComparingTo {\n \n @BeforeTemplate\n AbstractBigDecimalAssert> before(AbstractBigDecimalAssert> bigDecimalAssert, BigDecimal n) {\n return Refaster.anyOf(bigDecimalAssert.isNotCloseTo(n, offset(BigDecimal.ZERO)), bigDecimalAssert.isNotCloseTo(n, withPercentage(0)));\n }\n \n @AfterTemplate\n AbstractBigDecimalAssert> after(AbstractBigDecimalAssert> bigDecimalAssert, BigDecimal n) {\n return bigDecimalAssert.isNotEqualByComparingTo(n);\n }\n}\n```\n.";
}
@Override
public TreeVisitor, ExecutionContext> getVisitor() {
JavaVisitor javaVisitor = new AbstractRefasterJavaVisitor() {
final JavaTemplate before$0 = JavaTemplate
.builder("#{bigDecimalAssert:any(org.assertj.core.api.AbstractBigDecimalAssert>)}.isNotCloseTo(#{n:any(java.math.BigDecimal)}, org.assertj.core.data.Offset.offset(java.math.BigDecimal.ZERO))")
.javaParser(JavaParser.fromJavaVersion().classpath(JavaParser.runtimeClasspath()))
.build();
final JavaTemplate before$1 = JavaTemplate
.builder("#{bigDecimalAssert:any(org.assertj.core.api.AbstractBigDecimalAssert>)}.isNotCloseTo(#{n:any(java.math.BigDecimal)}, org.assertj.core.data.Percentage.withPercentage(0))")
.javaParser(JavaParser.fromJavaVersion().classpath(JavaParser.runtimeClasspath()))
.build();
final JavaTemplate after = JavaTemplate
.builder("#{bigDecimalAssert:any(org.assertj.core.api.AbstractBigDecimalAssert>)}.isNotEqualByComparingTo(#{n:any(java.math.BigDecimal)})")
.javaParser(JavaParser.fromJavaVersion().classpath(JavaParser.runtimeClasspath()))
.build();
@Override
public J visitMethodInvocation(J.MethodInvocation elem, ExecutionContext ctx) {
JavaTemplate.Matcher matcher;
if ((matcher = before$0.matcher(getCursor())).find()) {
maybeRemoveImport("org.assertj.core.data.Offset.offset");
return embed(
after.apply(getCursor(), elem.getCoordinates().replace(), matcher.parameter(0), matcher.parameter(1)),
getCursor(),
ctx,
SHORTEN_NAMES
);
}
if ((matcher = before$1.matcher(getCursor())).find()) {
maybeRemoveImport("org.assertj.core.data.Percentage.withPercentage");
return embed(
after.apply(getCursor(), elem.getCoordinates().replace(), matcher.parameter(0), matcher.parameter(1)),
getCursor(),
ctx,
SHORTEN_NAMES
);
}
return super.visitMethodInvocation(elem, ctx);
}
};
return Preconditions.check(
Preconditions.and(
new UsesType<>("java.math.BigDecimal", true),
new UsesType<>("org.assertj.core.api.AbstractBigDecimalAssert", true),
new UsesMethod<>("org.assertj.core.api.AbstractBigDecimalAssert isNotCloseTo(..)"),
Preconditions.or(
new UsesMethod<>("org.assertj.core.data.Offset offset(..)"),
new UsesMethod<>("org.assertj.core.data.Percentage withPercentage(..)")
)
),
javaVisitor
);
}
}
}