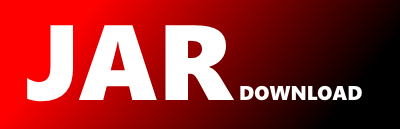
tech.picnic.errorprone.refasterrules.AssertJThrowingCallableRulesRecipes Maven / Gradle / Ivy
Show all versions of error-prone-contrib Show documentation
package tech.picnic.errorprone.refasterrules;
import org.openrewrite.ExecutionContext;
import org.openrewrite.Preconditions;
import org.openrewrite.Recipe;
import org.openrewrite.TreeVisitor;
import org.openrewrite.internal.lang.NonNullApi;
import org.openrewrite.java.JavaParser;
import org.openrewrite.java.JavaTemplate;
import org.openrewrite.java.JavaVisitor;
import org.openrewrite.java.search.*;
import org.openrewrite.java.template.Primitive;
import org.openrewrite.java.template.function.*;
import org.openrewrite.java.template.internal.AbstractRefasterJavaVisitor;
import org.openrewrite.java.tree.*;
import javax.annotation.Generated;
import java.util.*;
import static org.openrewrite.java.template.internal.AbstractRefasterJavaVisitor.EmbeddingOption.*;
/**
* OpenRewrite recipes created for Refaster template {@code tech.picnic.errorprone.refasterrules.AssertJThrowingCallableRules}.
*/
@SuppressWarnings("all")
@Generated("org.openrewrite.java.template.processor.RefasterTemplateProcessor")
public class AssertJThrowingCallableRulesRecipes extends Recipe {
/**
* Instantiates a new instance.
*/
public AssertJThrowingCallableRulesRecipes() {}
@Override
public String getDisplayName() {
return "Refaster rules related to AssertJ assertions over expressions that may throw a `Throwable` subtype";
}
@Override
public String getDescription() {
return "For reasons of consistency we prefer `org.assertj.core.api.Assertions#assertThatThrownBy` over static methods for specific exception\n types. Note that only the most common assertion expressions are rewritten here; covering all\n cases would require the implementation of an Error Prone check instead. [Source](https://error-prone.picnic.tech/refasterrules/AssertJThrowingCallableRules).";
}
@Override
public List getRecipeList() {
return Arrays.asList(
new AssertThatThrownByIllegalArgumentExceptionRecipe(),
new AssertThatThrownByIllegalArgumentExceptionHasMessageRecipe(),
new AssertThatThrownByIllegalArgumentExceptionHasMessageStartingWithRecipe(),
new AssertThatThrownByIllegalArgumentExceptionHasMessageContainingRecipe(),
new AssertThatThrownByIllegalStateExceptionRecipe(),
new AssertThatThrownByIllegalStateExceptionHasMessageRecipe(),
new AssertThatThrownByIllegalStateExceptionHasMessageStartingWithRecipe(),
new AssertThatThrownByIllegalStateExceptionHasMessageContainingRecipe(),
new AssertThatThrownByIllegalStateExceptionHasMessageNotContainingRecipe(),
new AssertThatThrownByNullPointerExceptionRecipe(),
new AssertThatThrownByNullPointerExceptionHasMessageRecipe(),
new AssertThatThrownByNullPointerExceptionHasMessageStartingWithRecipe(),
new AssertThatThrownByNullPointerExceptionHasMessageContainingRecipe(),
new AssertThatThrownByNullPointerExceptionHasMessageNotContainingRecipe(),
new AssertThatThrownByIOExceptionRecipe(),
new AssertThatThrownByIOExceptionHasMessageRecipe(),
new AssertThatThrownByIOExceptionHasMessageStartingWithRecipe(),
new AssertThatThrownByIOExceptionHasMessageContainingRecipe(),
new AssertThatThrownByIOExceptionHasMessageNotContainingRecipe(),
new AssertThatThrownByRecipe(),
new AssertThatThrownByHasMessageRecipe(),
new AssertThatThrownByHasMessageStartingWithRecipe(),
new AssertThatThrownByHasMessageContainingRecipe(),
new AssertThatThrownByHasMessageNotContainingRecipe()
);
}
/**
* OpenRewrite recipe created for Refaster template {@code AssertJThrowingCallableRules.AssertThatThrownByIllegalArgumentException}.
*/
@SuppressWarnings("all")
@NonNullApi
@Generated("org.openrewrite.java.template.processor.RefasterTemplateProcessor")
public static class AssertThatThrownByIllegalArgumentExceptionRecipe extends Recipe {
/**
* Instantiates a new instance.
*/
public AssertThatThrownByIllegalArgumentExceptionRecipe() {}
@Override
public String getDisplayName() {
return "Refaster template `AssertJThrowingCallableRules.AssertThatThrownByIllegalArgumentException`";
}
@Override
public String getDescription() {
return "Recipe created for the following Refaster template:\n```java\nstatic final class AssertThatThrownByIllegalArgumentException {\n \n @BeforeTemplate\n AbstractObjectAssert, ?> before(ThrowingCallable throwingCallable) {\n return assertThatIllegalArgumentException().isThrownBy(throwingCallable);\n }\n \n @AfterTemplate\n @UseImportPolicy(value = STATIC_IMPORT_ALWAYS)\n AbstractObjectAssert, ?> after(ThrowingCallable throwingCallable) {\n return assertThatThrownBy(throwingCallable).isInstanceOf(IllegalArgumentException.class);\n }\n}\n```\n.";
}
@Override
public TreeVisitor, ExecutionContext> getVisitor() {
JavaVisitor javaVisitor = new AbstractRefasterJavaVisitor() {
final JavaTemplate before = JavaTemplate
.builder("org.assertj.core.api.Assertions.assertThatIllegalArgumentException().isThrownBy(#{throwingCallable:any(org.assertj.core.api.ThrowableAssert.ThrowingCallable)})")
.javaParser(JavaParser.fromJavaVersion().classpath(JavaParser.runtimeClasspath()))
.build();
final JavaTemplate after = JavaTemplate
.builder("org.assertj.core.api.Assertions.assertThatThrownBy(#{throwingCallable:any(org.assertj.core.api.ThrowableAssert.ThrowingCallable)}).isInstanceOf(IllegalArgumentException.class)")
.javaParser(JavaParser.fromJavaVersion().classpath(JavaParser.runtimeClasspath()))
.build();
@Override
public J visitMethodInvocation(J.MethodInvocation elem, ExecutionContext ctx) {
JavaTemplate.Matcher matcher;
if ((matcher = before.matcher(getCursor())).find()) {
maybeRemoveImport("org.assertj.core.api.Assertions.assertThatIllegalArgumentException");
return embed(
after.apply(getCursor(), elem.getCoordinates().replace(), matcher.parameter(0)),
getCursor(),
ctx,
SHORTEN_NAMES
);
}
return super.visitMethodInvocation(elem, ctx);
}
};
return Preconditions.check(
Preconditions.and(
new UsesType<>("org.assertj.core.api.AbstractObjectAssert", true),
new UsesType<>("org.assertj.core.api.ThrowableAssert.ThrowingCallable", true),
new UsesMethod<>("org.assertj.core.api.ThrowableTypeAssert isThrownBy(..)"),
new UsesMethod<>("org.assertj.core.api.Assertions assertThatIllegalArgumentException(..)")
),
javaVisitor
);
}
}
/**
* OpenRewrite recipe created for Refaster template {@code AssertJThrowingCallableRules.AssertThatThrownByIllegalArgumentExceptionHasMessage}.
*/
@SuppressWarnings("all")
@NonNullApi
@Generated("org.openrewrite.java.template.processor.RefasterTemplateProcessor")
public static class AssertThatThrownByIllegalArgumentExceptionHasMessageRecipe extends Recipe {
/**
* Instantiates a new instance.
*/
public AssertThatThrownByIllegalArgumentExceptionHasMessageRecipe() {}
@Override
public String getDisplayName() {
return "Refaster template `AssertJThrowingCallableRules.AssertThatThrownByIllegalArgumentExceptionHasMessage`";
}
@Override
public String getDescription() {
return "Recipe created for the following Refaster template:\n```java\nstatic final class AssertThatThrownByIllegalArgumentExceptionHasMessage {\n \n @BeforeTemplate\n @SuppressWarnings(value = \"AssertThatThrownByIllegalArgumentException\")\n AbstractObjectAssert, ?> before(ThrowingCallable throwingCallable, String message) {\n return assertThatIllegalArgumentException().isThrownBy(throwingCallable).withMessage(message);\n }\n \n @AfterTemplate\n @UseImportPolicy(value = STATIC_IMPORT_ALWAYS)\n AbstractObjectAssert, ?> after(ThrowingCallable throwingCallable, String message) {\n return assertThatThrownBy(throwingCallable).isInstanceOf(IllegalArgumentException.class).hasMessage(message);\n }\n}\n```\n.";
}
@Override
public TreeVisitor, ExecutionContext> getVisitor() {
JavaVisitor javaVisitor = new AbstractRefasterJavaVisitor() {
final JavaTemplate before = JavaTemplate
.builder("org.assertj.core.api.Assertions.assertThatIllegalArgumentException().isThrownBy(#{throwingCallable:any(org.assertj.core.api.ThrowableAssert.ThrowingCallable)}).withMessage(#{message:any(java.lang.String)})")
.javaParser(JavaParser.fromJavaVersion().classpath(JavaParser.runtimeClasspath()))
.build();
final JavaTemplate after = JavaTemplate
.builder("org.assertj.core.api.Assertions.assertThatThrownBy(#{throwingCallable:any(org.assertj.core.api.ThrowableAssert.ThrowingCallable)}).isInstanceOf(IllegalArgumentException.class).hasMessage(#{message:any(java.lang.String)})")
.javaParser(JavaParser.fromJavaVersion().classpath(JavaParser.runtimeClasspath()))
.build();
@Override
public J visitMethodInvocation(J.MethodInvocation elem, ExecutionContext ctx) {
JavaTemplate.Matcher matcher;
if ((matcher = before.matcher(getCursor())).find()) {
maybeRemoveImport("org.assertj.core.api.Assertions.assertThatIllegalArgumentException");
return embed(
after.apply(getCursor(), elem.getCoordinates().replace(), matcher.parameter(0), matcher.parameter(1)),
getCursor(),
ctx,
SHORTEN_NAMES
);
}
return super.visitMethodInvocation(elem, ctx);
}
};
return Preconditions.check(
Preconditions.and(
new UsesType<>("org.assertj.core.api.AbstractObjectAssert", true),
new UsesType<>("org.assertj.core.api.ThrowableAssert.ThrowingCallable", true),
new UsesMethod<>("org.assertj.core.api.ThrowableAssertAlternative withMessage(..)"),
new UsesMethod<>("org.assertj.core.api.ThrowableTypeAssert isThrownBy(..)"),
new UsesMethod<>("org.assertj.core.api.Assertions assertThatIllegalArgumentException(..)")
),
javaVisitor
);
}
}
/**
* OpenRewrite recipe created for Refaster template {@code AssertJThrowingCallableRules.AssertThatThrownByIllegalArgumentExceptionHasMessageStartingWith}.
*/
@SuppressWarnings("all")
@NonNullApi
@Generated("org.openrewrite.java.template.processor.RefasterTemplateProcessor")
public static class AssertThatThrownByIllegalArgumentExceptionHasMessageStartingWithRecipe extends Recipe {
/**
* Instantiates a new instance.
*/
public AssertThatThrownByIllegalArgumentExceptionHasMessageStartingWithRecipe() {}
@Override
public String getDisplayName() {
return "Refaster template `AssertJThrowingCallableRules.AssertThatThrownByIllegalArgumentExceptionHasMessageStartingWith`";
}
@Override
public String getDescription() {
return "Recipe created for the following Refaster template:\n```java\nstatic final class AssertThatThrownByIllegalArgumentExceptionHasMessageStartingWith {\n \n @BeforeTemplate\n @SuppressWarnings(value = \"AssertThatThrownByIllegalArgumentException\")\n AbstractObjectAssert, ?> before(ThrowingCallable throwingCallable, String message) {\n return assertThatIllegalArgumentException().isThrownBy(throwingCallable).withMessageStartingWith(message);\n }\n \n @AfterTemplate\n @UseImportPolicy(value = STATIC_IMPORT_ALWAYS)\n AbstractObjectAssert, ?> after(ThrowingCallable throwingCallable, String message) {\n return assertThatThrownBy(throwingCallable).isInstanceOf(IllegalArgumentException.class).hasMessageStartingWith(message);\n }\n}\n```\n.";
}
@Override
public TreeVisitor, ExecutionContext> getVisitor() {
JavaVisitor javaVisitor = new AbstractRefasterJavaVisitor() {
final JavaTemplate before = JavaTemplate
.builder("org.assertj.core.api.Assertions.assertThatIllegalArgumentException().isThrownBy(#{throwingCallable:any(org.assertj.core.api.ThrowableAssert.ThrowingCallable)}).withMessageStartingWith(#{message:any(java.lang.String)})")
.javaParser(JavaParser.fromJavaVersion().classpath(JavaParser.runtimeClasspath()))
.build();
final JavaTemplate after = JavaTemplate
.builder("org.assertj.core.api.Assertions.assertThatThrownBy(#{throwingCallable:any(org.assertj.core.api.ThrowableAssert.ThrowingCallable)}).isInstanceOf(IllegalArgumentException.class).hasMessageStartingWith(#{message:any(java.lang.String)})")
.javaParser(JavaParser.fromJavaVersion().classpath(JavaParser.runtimeClasspath()))
.build();
@Override
public J visitMethodInvocation(J.MethodInvocation elem, ExecutionContext ctx) {
JavaTemplate.Matcher matcher;
if ((matcher = before.matcher(getCursor())).find()) {
maybeRemoveImport("org.assertj.core.api.Assertions.assertThatIllegalArgumentException");
return embed(
after.apply(getCursor(), elem.getCoordinates().replace(), matcher.parameter(0), matcher.parameter(1)),
getCursor(),
ctx,
SHORTEN_NAMES
);
}
return super.visitMethodInvocation(elem, ctx);
}
};
return Preconditions.check(
Preconditions.and(
new UsesType<>("org.assertj.core.api.AbstractObjectAssert", true),
new UsesType<>("org.assertj.core.api.ThrowableAssert.ThrowingCallable", true),
new UsesMethod<>("org.assertj.core.api.ThrowableAssertAlternative withMessageStartingWith(..)"),
new UsesMethod<>("org.assertj.core.api.ThrowableTypeAssert isThrownBy(..)"),
new UsesMethod<>("org.assertj.core.api.Assertions assertThatIllegalArgumentException(..)")
),
javaVisitor
);
}
}
/**
* OpenRewrite recipe created for Refaster template {@code AssertJThrowingCallableRules.AssertThatThrownByIllegalArgumentExceptionHasMessageContaining}.
*/
@SuppressWarnings("all")
@NonNullApi
@Generated("org.openrewrite.java.template.processor.RefasterTemplateProcessor")
public static class AssertThatThrownByIllegalArgumentExceptionHasMessageContainingRecipe extends Recipe {
/**
* Instantiates a new instance.
*/
public AssertThatThrownByIllegalArgumentExceptionHasMessageContainingRecipe() {}
@Override
public String getDisplayName() {
return "Refaster template `AssertJThrowingCallableRules.AssertThatThrownByIllegalArgumentExceptionHasMessageContaining`";
}
@Override
public String getDescription() {
return "Recipe created for the following Refaster template:\n```java\nstatic final class AssertThatThrownByIllegalArgumentExceptionHasMessageContaining {\n \n @BeforeTemplate\n @SuppressWarnings(value = \"AssertThatThrownByIllegalArgumentException\")\n AbstractObjectAssert, ?> before(ThrowingCallable throwingCallable, String message) {\n return assertThatIllegalArgumentException().isThrownBy(throwingCallable).withMessageContaining(message);\n }\n \n @AfterTemplate\n @UseImportPolicy(value = STATIC_IMPORT_ALWAYS)\n AbstractObjectAssert, ?> after(ThrowingCallable throwingCallable, String message) {\n return assertThatThrownBy(throwingCallable).isInstanceOf(IllegalArgumentException.class).hasMessageContaining(message);\n }\n}\n```\n.";
}
@Override
public TreeVisitor, ExecutionContext> getVisitor() {
JavaVisitor javaVisitor = new AbstractRefasterJavaVisitor() {
final JavaTemplate before = JavaTemplate
.builder("org.assertj.core.api.Assertions.assertThatIllegalArgumentException().isThrownBy(#{throwingCallable:any(org.assertj.core.api.ThrowableAssert.ThrowingCallable)}).withMessageContaining(#{message:any(java.lang.String)})")
.javaParser(JavaParser.fromJavaVersion().classpath(JavaParser.runtimeClasspath()))
.build();
final JavaTemplate after = JavaTemplate
.builder("org.assertj.core.api.Assertions.assertThatThrownBy(#{throwingCallable:any(org.assertj.core.api.ThrowableAssert.ThrowingCallable)}).isInstanceOf(IllegalArgumentException.class).hasMessageContaining(#{message:any(java.lang.String)})")
.javaParser(JavaParser.fromJavaVersion().classpath(JavaParser.runtimeClasspath()))
.build();
@Override
public J visitMethodInvocation(J.MethodInvocation elem, ExecutionContext ctx) {
JavaTemplate.Matcher matcher;
if ((matcher = before.matcher(getCursor())).find()) {
maybeRemoveImport("org.assertj.core.api.Assertions.assertThatIllegalArgumentException");
return embed(
after.apply(getCursor(), elem.getCoordinates().replace(), matcher.parameter(0), matcher.parameter(1)),
getCursor(),
ctx,
SHORTEN_NAMES
);
}
return super.visitMethodInvocation(elem, ctx);
}
};
return Preconditions.check(
Preconditions.and(
new UsesType<>("org.assertj.core.api.AbstractObjectAssert", true),
new UsesType<>("org.assertj.core.api.ThrowableAssert.ThrowingCallable", true),
new UsesMethod<>("org.assertj.core.api.ThrowableAssertAlternative withMessageContaining(..)"),
new UsesMethod<>("org.assertj.core.api.ThrowableTypeAssert isThrownBy(..)"),
new UsesMethod<>("org.assertj.core.api.Assertions assertThatIllegalArgumentException(..)")
),
javaVisitor
);
}
}
/**
* OpenRewrite recipe created for Refaster template {@code AssertJThrowingCallableRules.AssertThatThrownByIllegalStateException}.
*/
@SuppressWarnings("all")
@NonNullApi
@Generated("org.openrewrite.java.template.processor.RefasterTemplateProcessor")
public static class AssertThatThrownByIllegalStateExceptionRecipe extends Recipe {
/**
* Instantiates a new instance.
*/
public AssertThatThrownByIllegalStateExceptionRecipe() {}
@Override
public String getDisplayName() {
return "Refaster template `AssertJThrowingCallableRules.AssertThatThrownByIllegalStateException`";
}
@Override
public String getDescription() {
return "Recipe created for the following Refaster template:\n```java\nstatic final class AssertThatThrownByIllegalStateException {\n \n @BeforeTemplate\n AbstractObjectAssert, ?> before(ThrowingCallable throwingCallable) {\n return assertThatIllegalStateException().isThrownBy(throwingCallable);\n }\n \n @AfterTemplate\n @UseImportPolicy(value = STATIC_IMPORT_ALWAYS)\n AbstractObjectAssert, ?> after(ThrowingCallable throwingCallable) {\n return assertThatThrownBy(throwingCallable).isInstanceOf(IllegalStateException.class);\n }\n}\n```\n.";
}
@Override
public TreeVisitor, ExecutionContext> getVisitor() {
JavaVisitor javaVisitor = new AbstractRefasterJavaVisitor() {
final JavaTemplate before = JavaTemplate
.builder("org.assertj.core.api.Assertions.assertThatIllegalStateException().isThrownBy(#{throwingCallable:any(org.assertj.core.api.ThrowableAssert.ThrowingCallable)})")
.javaParser(JavaParser.fromJavaVersion().classpath(JavaParser.runtimeClasspath()))
.build();
final JavaTemplate after = JavaTemplate
.builder("org.assertj.core.api.Assertions.assertThatThrownBy(#{throwingCallable:any(org.assertj.core.api.ThrowableAssert.ThrowingCallable)}).isInstanceOf(IllegalStateException.class)")
.javaParser(JavaParser.fromJavaVersion().classpath(JavaParser.runtimeClasspath()))
.build();
@Override
public J visitMethodInvocation(J.MethodInvocation elem, ExecutionContext ctx) {
JavaTemplate.Matcher matcher;
if ((matcher = before.matcher(getCursor())).find()) {
maybeRemoveImport("org.assertj.core.api.Assertions.assertThatIllegalStateException");
return embed(
after.apply(getCursor(), elem.getCoordinates().replace(), matcher.parameter(0)),
getCursor(),
ctx,
SHORTEN_NAMES
);
}
return super.visitMethodInvocation(elem, ctx);
}
};
return Preconditions.check(
Preconditions.and(
new UsesType<>("org.assertj.core.api.AbstractObjectAssert", true),
new UsesType<>("org.assertj.core.api.ThrowableAssert.ThrowingCallable", true),
new UsesMethod<>("org.assertj.core.api.ThrowableTypeAssert isThrownBy(..)"),
new UsesMethod<>("org.assertj.core.api.Assertions assertThatIllegalStateException(..)")
),
javaVisitor
);
}
}
/**
* OpenRewrite recipe created for Refaster template {@code AssertJThrowingCallableRules.AssertThatThrownByIllegalStateExceptionHasMessage}.
*/
@SuppressWarnings("all")
@NonNullApi
@Generated("org.openrewrite.java.template.processor.RefasterTemplateProcessor")
public static class AssertThatThrownByIllegalStateExceptionHasMessageRecipe extends Recipe {
/**
* Instantiates a new instance.
*/
public AssertThatThrownByIllegalStateExceptionHasMessageRecipe() {}
@Override
public String getDisplayName() {
return "Refaster template `AssertJThrowingCallableRules.AssertThatThrownByIllegalStateExceptionHasMessage`";
}
@Override
public String getDescription() {
return "Recipe created for the following Refaster template:\n```java\nstatic final class AssertThatThrownByIllegalStateExceptionHasMessage {\n \n @BeforeTemplate\n @SuppressWarnings(value = \"AssertThatThrownByIllegalStateException\")\n AbstractObjectAssert, ?> before(ThrowingCallable throwingCallable, String message) {\n return assertThatIllegalStateException().isThrownBy(throwingCallable).withMessage(message);\n }\n \n @AfterTemplate\n @UseImportPolicy(value = STATIC_IMPORT_ALWAYS)\n AbstractObjectAssert, ?> after(ThrowingCallable throwingCallable, String message) {\n return assertThatThrownBy(throwingCallable).isInstanceOf(IllegalStateException.class).hasMessage(message);\n }\n}\n```\n.";
}
@Override
public TreeVisitor, ExecutionContext> getVisitor() {
JavaVisitor javaVisitor = new AbstractRefasterJavaVisitor() {
final JavaTemplate before = JavaTemplate
.builder("org.assertj.core.api.Assertions.assertThatIllegalStateException().isThrownBy(#{throwingCallable:any(org.assertj.core.api.ThrowableAssert.ThrowingCallable)}).withMessage(#{message:any(java.lang.String)})")
.javaParser(JavaParser.fromJavaVersion().classpath(JavaParser.runtimeClasspath()))
.build();
final JavaTemplate after = JavaTemplate
.builder("org.assertj.core.api.Assertions.assertThatThrownBy(#{throwingCallable:any(org.assertj.core.api.ThrowableAssert.ThrowingCallable)}).isInstanceOf(IllegalStateException.class).hasMessage(#{message:any(java.lang.String)})")
.javaParser(JavaParser.fromJavaVersion().classpath(JavaParser.runtimeClasspath()))
.build();
@Override
public J visitMethodInvocation(J.MethodInvocation elem, ExecutionContext ctx) {
JavaTemplate.Matcher matcher;
if ((matcher = before.matcher(getCursor())).find()) {
maybeRemoveImport("org.assertj.core.api.Assertions.assertThatIllegalStateException");
return embed(
after.apply(getCursor(), elem.getCoordinates().replace(), matcher.parameter(0), matcher.parameter(1)),
getCursor(),
ctx,
SHORTEN_NAMES
);
}
return super.visitMethodInvocation(elem, ctx);
}
};
return Preconditions.check(
Preconditions.and(
new UsesType<>("org.assertj.core.api.AbstractObjectAssert", true),
new UsesType<>("org.assertj.core.api.ThrowableAssert.ThrowingCallable", true),
new UsesMethod<>("org.assertj.core.api.ThrowableAssertAlternative withMessage(..)"),
new UsesMethod<>("org.assertj.core.api.ThrowableTypeAssert isThrownBy(..)"),
new UsesMethod<>("org.assertj.core.api.Assertions assertThatIllegalStateException(..)")
),
javaVisitor
);
}
}
/**
* OpenRewrite recipe created for Refaster template {@code AssertJThrowingCallableRules.AssertThatThrownByIllegalStateExceptionHasMessageStartingWith}.
*/
@SuppressWarnings("all")
@NonNullApi
@Generated("org.openrewrite.java.template.processor.RefasterTemplateProcessor")
public static class AssertThatThrownByIllegalStateExceptionHasMessageStartingWithRecipe extends Recipe {
/**
* Instantiates a new instance.
*/
public AssertThatThrownByIllegalStateExceptionHasMessageStartingWithRecipe() {}
@Override
public String getDisplayName() {
return "Refaster template `AssertJThrowingCallableRules.AssertThatThrownByIllegalStateExceptionHasMessageStartingWith`";
}
@Override
public String getDescription() {
return "Recipe created for the following Refaster template:\n```java\nstatic final class AssertThatThrownByIllegalStateExceptionHasMessageStartingWith {\n \n @BeforeTemplate\n @SuppressWarnings(value = \"AssertThatThrownByIllegalStateException\")\n AbstractObjectAssert, ?> before(ThrowingCallable throwingCallable, String message) {\n return assertThatIllegalStateException().isThrownBy(throwingCallable).withMessageStartingWith(message);\n }\n \n @AfterTemplate\n @UseImportPolicy(value = STATIC_IMPORT_ALWAYS)\n AbstractObjectAssert, ?> after(ThrowingCallable throwingCallable, String message) {\n return assertThatThrownBy(throwingCallable).isInstanceOf(IllegalStateException.class).hasMessageStartingWith(message);\n }\n}\n```\n.";
}
@Override
public TreeVisitor, ExecutionContext> getVisitor() {
JavaVisitor javaVisitor = new AbstractRefasterJavaVisitor() {
final JavaTemplate before = JavaTemplate
.builder("org.assertj.core.api.Assertions.assertThatIllegalStateException().isThrownBy(#{throwingCallable:any(org.assertj.core.api.ThrowableAssert.ThrowingCallable)}).withMessageStartingWith(#{message:any(java.lang.String)})")
.javaParser(JavaParser.fromJavaVersion().classpath(JavaParser.runtimeClasspath()))
.build();
final JavaTemplate after = JavaTemplate
.builder("org.assertj.core.api.Assertions.assertThatThrownBy(#{throwingCallable:any(org.assertj.core.api.ThrowableAssert.ThrowingCallable)}).isInstanceOf(IllegalStateException.class).hasMessageStartingWith(#{message:any(java.lang.String)})")
.javaParser(JavaParser.fromJavaVersion().classpath(JavaParser.runtimeClasspath()))
.build();
@Override
public J visitMethodInvocation(J.MethodInvocation elem, ExecutionContext ctx) {
JavaTemplate.Matcher matcher;
if ((matcher = before.matcher(getCursor())).find()) {
maybeRemoveImport("org.assertj.core.api.Assertions.assertThatIllegalStateException");
return embed(
after.apply(getCursor(), elem.getCoordinates().replace(), matcher.parameter(0), matcher.parameter(1)),
getCursor(),
ctx,
SHORTEN_NAMES
);
}
return super.visitMethodInvocation(elem, ctx);
}
};
return Preconditions.check(
Preconditions.and(
new UsesType<>("org.assertj.core.api.AbstractObjectAssert", true),
new UsesType<>("org.assertj.core.api.ThrowableAssert.ThrowingCallable", true),
new UsesMethod<>("org.assertj.core.api.ThrowableAssertAlternative withMessageStartingWith(..)"),
new UsesMethod<>("org.assertj.core.api.ThrowableTypeAssert isThrownBy(..)"),
new UsesMethod<>("org.assertj.core.api.Assertions assertThatIllegalStateException(..)")
),
javaVisitor
);
}
}
/**
* OpenRewrite recipe created for Refaster template {@code AssertJThrowingCallableRules.AssertThatThrownByIllegalStateExceptionHasMessageContaining}.
*/
@SuppressWarnings("all")
@NonNullApi
@Generated("org.openrewrite.java.template.processor.RefasterTemplateProcessor")
public static class AssertThatThrownByIllegalStateExceptionHasMessageContainingRecipe extends Recipe {
/**
* Instantiates a new instance.
*/
public AssertThatThrownByIllegalStateExceptionHasMessageContainingRecipe() {}
@Override
public String getDisplayName() {
return "Refaster template `AssertJThrowingCallableRules.AssertThatThrownByIllegalStateExceptionHasMessageContaining`";
}
@Override
public String getDescription() {
return "Recipe created for the following Refaster template:\n```java\nstatic final class AssertThatThrownByIllegalStateExceptionHasMessageContaining {\n \n @BeforeTemplate\n @SuppressWarnings(value = \"AssertThatThrownByIllegalStateException\")\n AbstractObjectAssert, ?> before(ThrowingCallable throwingCallable, String message) {\n return assertThatIllegalStateException().isThrownBy(throwingCallable).withMessageContaining(message);\n }\n \n @AfterTemplate\n @UseImportPolicy(value = STATIC_IMPORT_ALWAYS)\n AbstractObjectAssert, ?> after(ThrowingCallable throwingCallable, String message) {\n return assertThatThrownBy(throwingCallable).isInstanceOf(IllegalStateException.class).hasMessageContaining(message);\n }\n}\n```\n.";
}
@Override
public TreeVisitor, ExecutionContext> getVisitor() {
JavaVisitor javaVisitor = new AbstractRefasterJavaVisitor() {
final JavaTemplate before = JavaTemplate
.builder("org.assertj.core.api.Assertions.assertThatIllegalStateException().isThrownBy(#{throwingCallable:any(org.assertj.core.api.ThrowableAssert.ThrowingCallable)}).withMessageContaining(#{message:any(java.lang.String)})")
.javaParser(JavaParser.fromJavaVersion().classpath(JavaParser.runtimeClasspath()))
.build();
final JavaTemplate after = JavaTemplate
.builder("org.assertj.core.api.Assertions.assertThatThrownBy(#{throwingCallable:any(org.assertj.core.api.ThrowableAssert.ThrowingCallable)}).isInstanceOf(IllegalStateException.class).hasMessageContaining(#{message:any(java.lang.String)})")
.javaParser(JavaParser.fromJavaVersion().classpath(JavaParser.runtimeClasspath()))
.build();
@Override
public J visitMethodInvocation(J.MethodInvocation elem, ExecutionContext ctx) {
JavaTemplate.Matcher matcher;
if ((matcher = before.matcher(getCursor())).find()) {
maybeRemoveImport("org.assertj.core.api.Assertions.assertThatIllegalStateException");
return embed(
after.apply(getCursor(), elem.getCoordinates().replace(), matcher.parameter(0), matcher.parameter(1)),
getCursor(),
ctx,
SHORTEN_NAMES
);
}
return super.visitMethodInvocation(elem, ctx);
}
};
return Preconditions.check(
Preconditions.and(
new UsesType<>("org.assertj.core.api.AbstractObjectAssert", true),
new UsesType<>("org.assertj.core.api.ThrowableAssert.ThrowingCallable", true),
new UsesMethod<>("org.assertj.core.api.ThrowableAssertAlternative withMessageContaining(..)"),
new UsesMethod<>("org.assertj.core.api.ThrowableTypeAssert isThrownBy(..)"),
new UsesMethod<>("org.assertj.core.api.Assertions assertThatIllegalStateException(..)")
),
javaVisitor
);
}
}
/**
* OpenRewrite recipe created for Refaster template {@code AssertJThrowingCallableRules.AssertThatThrownByIllegalStateExceptionHasMessageNotContaining}.
*/
@SuppressWarnings("all")
@NonNullApi
@Generated("org.openrewrite.java.template.processor.RefasterTemplateProcessor")
public static class AssertThatThrownByIllegalStateExceptionHasMessageNotContainingRecipe extends Recipe {
/**
* Instantiates a new instance.
*/
public AssertThatThrownByIllegalStateExceptionHasMessageNotContainingRecipe() {}
@Override
public String getDisplayName() {
return "Refaster template `AssertJThrowingCallableRules.AssertThatThrownByIllegalStateExceptionHasMessageNotContaining`";
}
@Override
public String getDescription() {
return "Recipe created for the following Refaster template:\n```java\nstatic final class AssertThatThrownByIllegalStateExceptionHasMessageNotContaining {\n \n @BeforeTemplate\n @SuppressWarnings(value = \"AssertThatThrownByIllegalStateException\")\n AbstractObjectAssert, ?> before(ThrowingCallable throwingCallable, String message) {\n return assertThatIllegalStateException().isThrownBy(throwingCallable).withMessageNotContaining(message);\n }\n \n @AfterTemplate\n @UseImportPolicy(value = STATIC_IMPORT_ALWAYS)\n AbstractObjectAssert, ?> after(ThrowingCallable throwingCallable, String message) {\n return assertThatThrownBy(throwingCallable).isInstanceOf(IllegalStateException.class).hasMessageNotContaining(message);\n }\n}\n```\n.";
}
@Override
public TreeVisitor, ExecutionContext> getVisitor() {
JavaVisitor javaVisitor = new AbstractRefasterJavaVisitor() {
final JavaTemplate before = JavaTemplate
.builder("org.assertj.core.api.Assertions.assertThatIllegalStateException().isThrownBy(#{throwingCallable:any(org.assertj.core.api.ThrowableAssert.ThrowingCallable)}).withMessageNotContaining(#{message:any(java.lang.String)})")
.javaParser(JavaParser.fromJavaVersion().classpath(JavaParser.runtimeClasspath()))
.build();
final JavaTemplate after = JavaTemplate
.builder("org.assertj.core.api.Assertions.assertThatThrownBy(#{throwingCallable:any(org.assertj.core.api.ThrowableAssert.ThrowingCallable)}).isInstanceOf(IllegalStateException.class).hasMessageNotContaining(#{message:any(java.lang.String)})")
.javaParser(JavaParser.fromJavaVersion().classpath(JavaParser.runtimeClasspath()))
.build();
@Override
public J visitMethodInvocation(J.MethodInvocation elem, ExecutionContext ctx) {
JavaTemplate.Matcher matcher;
if ((matcher = before.matcher(getCursor())).find()) {
maybeRemoveImport("org.assertj.core.api.Assertions.assertThatIllegalStateException");
return embed(
after.apply(getCursor(), elem.getCoordinates().replace(), matcher.parameter(0), matcher.parameter(1)),
getCursor(),
ctx,
SHORTEN_NAMES
);
}
return super.visitMethodInvocation(elem, ctx);
}
};
return Preconditions.check(
Preconditions.and(
new UsesType<>("org.assertj.core.api.AbstractObjectAssert", true),
new UsesType<>("org.assertj.core.api.ThrowableAssert.ThrowingCallable", true),
new UsesMethod<>("org.assertj.core.api.ThrowableAssertAlternative withMessageNotContaining(..)"),
new UsesMethod<>("org.assertj.core.api.ThrowableTypeAssert isThrownBy(..)"),
new UsesMethod<>("org.assertj.core.api.Assertions assertThatIllegalStateException(..)")
),
javaVisitor
);
}
}
/**
* OpenRewrite recipe created for Refaster template {@code AssertJThrowingCallableRules.AssertThatThrownByNullPointerException}.
*/
@SuppressWarnings("all")
@NonNullApi
@Generated("org.openrewrite.java.template.processor.RefasterTemplateProcessor")
public static class AssertThatThrownByNullPointerExceptionRecipe extends Recipe {
/**
* Instantiates a new instance.
*/
public AssertThatThrownByNullPointerExceptionRecipe() {}
@Override
public String getDisplayName() {
return "Refaster template `AssertJThrowingCallableRules.AssertThatThrownByNullPointerException`";
}
@Override
public String getDescription() {
return "Recipe created for the following Refaster template:\n```java\nstatic final class AssertThatThrownByNullPointerException {\n \n @BeforeTemplate\n AbstractObjectAssert, ?> before(ThrowingCallable throwingCallable) {\n return assertThatNullPointerException().isThrownBy(throwingCallable);\n }\n \n @AfterTemplate\n @UseImportPolicy(value = STATIC_IMPORT_ALWAYS)\n AbstractObjectAssert, ?> after(ThrowingCallable throwingCallable) {\n return assertThatThrownBy(throwingCallable).isInstanceOf(NullPointerException.class);\n }\n}\n```\n.";
}
@Override
public TreeVisitor, ExecutionContext> getVisitor() {
JavaVisitor javaVisitor = new AbstractRefasterJavaVisitor() {
final JavaTemplate before = JavaTemplate
.builder("org.assertj.core.api.Assertions.assertThatNullPointerException().isThrownBy(#{throwingCallable:any(org.assertj.core.api.ThrowableAssert.ThrowingCallable)})")
.javaParser(JavaParser.fromJavaVersion().classpath(JavaParser.runtimeClasspath()))
.build();
final JavaTemplate after = JavaTemplate
.builder("org.assertj.core.api.Assertions.assertThatThrownBy(#{throwingCallable:any(org.assertj.core.api.ThrowableAssert.ThrowingCallable)}).isInstanceOf(NullPointerException.class)")
.javaParser(JavaParser.fromJavaVersion().classpath(JavaParser.runtimeClasspath()))
.build();
@Override
public J visitMethodInvocation(J.MethodInvocation elem, ExecutionContext ctx) {
JavaTemplate.Matcher matcher;
if ((matcher = before.matcher(getCursor())).find()) {
maybeRemoveImport("org.assertj.core.api.Assertions.assertThatNullPointerException");
return embed(
after.apply(getCursor(), elem.getCoordinates().replace(), matcher.parameter(0)),
getCursor(),
ctx,
SHORTEN_NAMES
);
}
return super.visitMethodInvocation(elem, ctx);
}
};
return Preconditions.check(
Preconditions.and(
new UsesType<>("org.assertj.core.api.AbstractObjectAssert", true),
new UsesType<>("org.assertj.core.api.ThrowableAssert.ThrowingCallable", true),
new UsesMethod<>("org.assertj.core.api.ThrowableTypeAssert isThrownBy(..)"),
new UsesMethod<>("org.assertj.core.api.Assertions assertThatNullPointerException(..)")
),
javaVisitor
);
}
}
/**
* OpenRewrite recipe created for Refaster template {@code AssertJThrowingCallableRules.AssertThatThrownByNullPointerExceptionHasMessage}.
*/
@SuppressWarnings("all")
@NonNullApi
@Generated("org.openrewrite.java.template.processor.RefasterTemplateProcessor")
public static class AssertThatThrownByNullPointerExceptionHasMessageRecipe extends Recipe {
/**
* Instantiates a new instance.
*/
public AssertThatThrownByNullPointerExceptionHasMessageRecipe() {}
@Override
public String getDisplayName() {
return "Refaster template `AssertJThrowingCallableRules.AssertThatThrownByNullPointerExceptionHasMessage`";
}
@Override
public String getDescription() {
return "Recipe created for the following Refaster template:\n```java\nstatic final class AssertThatThrownByNullPointerExceptionHasMessage {\n \n @BeforeTemplate\n @SuppressWarnings(value = \"AssertThatThrownByNullPointerException\")\n AbstractObjectAssert, ?> before(ThrowingCallable throwingCallable, String message) {\n return assertThatNullPointerException().isThrownBy(throwingCallable).withMessage(message);\n }\n \n @AfterTemplate\n @UseImportPolicy(value = STATIC_IMPORT_ALWAYS)\n AbstractObjectAssert, ?> after(ThrowingCallable throwingCallable, String message) {\n return assertThatThrownBy(throwingCallable).isInstanceOf(NullPointerException.class).hasMessage(message);\n }\n}\n```\n.";
}
@Override
public TreeVisitor, ExecutionContext> getVisitor() {
JavaVisitor javaVisitor = new AbstractRefasterJavaVisitor() {
final JavaTemplate before = JavaTemplate
.builder("org.assertj.core.api.Assertions.assertThatNullPointerException().isThrownBy(#{throwingCallable:any(org.assertj.core.api.ThrowableAssert.ThrowingCallable)}).withMessage(#{message:any(java.lang.String)})")
.javaParser(JavaParser.fromJavaVersion().classpath(JavaParser.runtimeClasspath()))
.build();
final JavaTemplate after = JavaTemplate
.builder("org.assertj.core.api.Assertions.assertThatThrownBy(#{throwingCallable:any(org.assertj.core.api.ThrowableAssert.ThrowingCallable)}).isInstanceOf(NullPointerException.class).hasMessage(#{message:any(java.lang.String)})")
.javaParser(JavaParser.fromJavaVersion().classpath(JavaParser.runtimeClasspath()))
.build();
@Override
public J visitMethodInvocation(J.MethodInvocation elem, ExecutionContext ctx) {
JavaTemplate.Matcher matcher;
if ((matcher = before.matcher(getCursor())).find()) {
maybeRemoveImport("org.assertj.core.api.Assertions.assertThatNullPointerException");
return embed(
after.apply(getCursor(), elem.getCoordinates().replace(), matcher.parameter(0), matcher.parameter(1)),
getCursor(),
ctx,
SHORTEN_NAMES
);
}
return super.visitMethodInvocation(elem, ctx);
}
};
return Preconditions.check(
Preconditions.and(
new UsesType<>("org.assertj.core.api.AbstractObjectAssert", true),
new UsesType<>("org.assertj.core.api.ThrowableAssert.ThrowingCallable", true),
new UsesMethod<>("org.assertj.core.api.ThrowableAssertAlternative withMessage(..)"),
new UsesMethod<>("org.assertj.core.api.ThrowableTypeAssert isThrownBy(..)"),
new UsesMethod<>("org.assertj.core.api.Assertions assertThatNullPointerException(..)")
),
javaVisitor
);
}
}
/**
* OpenRewrite recipe created for Refaster template {@code AssertJThrowingCallableRules.AssertThatThrownByNullPointerExceptionHasMessageStartingWith}.
*/
@SuppressWarnings("all")
@NonNullApi
@Generated("org.openrewrite.java.template.processor.RefasterTemplateProcessor")
public static class AssertThatThrownByNullPointerExceptionHasMessageStartingWithRecipe extends Recipe {
/**
* Instantiates a new instance.
*/
public AssertThatThrownByNullPointerExceptionHasMessageStartingWithRecipe() {}
@Override
public String getDisplayName() {
return "Refaster template `AssertJThrowingCallableRules.AssertThatThrownByNullPointerExceptionHasMessageStartingWith`";
}
@Override
public String getDescription() {
return "Recipe created for the following Refaster template:\n```java\nstatic final class AssertThatThrownByNullPointerExceptionHasMessageStartingWith {\n \n @BeforeTemplate\n @SuppressWarnings(value = \"AssertThatThrownByNullPointerException\")\n AbstractObjectAssert, ?> before(ThrowingCallable throwingCallable, String message) {\n return assertThatNullPointerException().isThrownBy(throwingCallable).withMessageStartingWith(message);\n }\n \n @AfterTemplate\n @UseImportPolicy(value = STATIC_IMPORT_ALWAYS)\n AbstractObjectAssert, ?> after(ThrowingCallable throwingCallable, String message) {\n return assertThatThrownBy(throwingCallable).isInstanceOf(NullPointerException.class).hasMessageStartingWith(message);\n }\n}\n```\n.";
}
@Override
public TreeVisitor, ExecutionContext> getVisitor() {
JavaVisitor javaVisitor = new AbstractRefasterJavaVisitor() {
final JavaTemplate before = JavaTemplate
.builder("org.assertj.core.api.Assertions.assertThatNullPointerException().isThrownBy(#{throwingCallable:any(org.assertj.core.api.ThrowableAssert.ThrowingCallable)}).withMessageStartingWith(#{message:any(java.lang.String)})")
.javaParser(JavaParser.fromJavaVersion().classpath(JavaParser.runtimeClasspath()))
.build();
final JavaTemplate after = JavaTemplate
.builder("org.assertj.core.api.Assertions.assertThatThrownBy(#{throwingCallable:any(org.assertj.core.api.ThrowableAssert.ThrowingCallable)}).isInstanceOf(NullPointerException.class).hasMessageStartingWith(#{message:any(java.lang.String)})")
.javaParser(JavaParser.fromJavaVersion().classpath(JavaParser.runtimeClasspath()))
.build();
@Override
public J visitMethodInvocation(J.MethodInvocation elem, ExecutionContext ctx) {
JavaTemplate.Matcher matcher;
if ((matcher = before.matcher(getCursor())).find()) {
maybeRemoveImport("org.assertj.core.api.Assertions.assertThatNullPointerException");
return embed(
after.apply(getCursor(), elem.getCoordinates().replace(), matcher.parameter(0), matcher.parameter(1)),
getCursor(),
ctx,
SHORTEN_NAMES
);
}
return super.visitMethodInvocation(elem, ctx);
}
};
return Preconditions.check(
Preconditions.and(
new UsesType<>("org.assertj.core.api.AbstractObjectAssert", true),
new UsesType<>("org.assertj.core.api.ThrowableAssert.ThrowingCallable", true),
new UsesMethod<>("org.assertj.core.api.ThrowableAssertAlternative withMessageStartingWith(..)"),
new UsesMethod<>("org.assertj.core.api.ThrowableTypeAssert isThrownBy(..)"),
new UsesMethod<>("org.assertj.core.api.Assertions assertThatNullPointerException(..)")
),
javaVisitor
);
}
}
/**
* OpenRewrite recipe created for Refaster template {@code AssertJThrowingCallableRules.AssertThatThrownByNullPointerExceptionHasMessageContaining}.
*/
@SuppressWarnings("all")
@NonNullApi
@Generated("org.openrewrite.java.template.processor.RefasterTemplateProcessor")
public static class AssertThatThrownByNullPointerExceptionHasMessageContainingRecipe extends Recipe {
/**
* Instantiates a new instance.
*/
public AssertThatThrownByNullPointerExceptionHasMessageContainingRecipe() {}
@Override
public String getDisplayName() {
return "Refaster template `AssertJThrowingCallableRules.AssertThatThrownByNullPointerExceptionHasMessageContaining`";
}
@Override
public String getDescription() {
return "Recipe created for the following Refaster template:\n```java\nstatic final class AssertThatThrownByNullPointerExceptionHasMessageContaining {\n \n @BeforeTemplate\n @SuppressWarnings(value = \"AssertThatThrownByNullPointerException\")\n AbstractObjectAssert, ?> before(ThrowingCallable throwingCallable, String message) {\n return assertThatNullPointerException().isThrownBy(throwingCallable).withMessageContaining(message);\n }\n \n @AfterTemplate\n @UseImportPolicy(value = STATIC_IMPORT_ALWAYS)\n AbstractObjectAssert, ?> after(ThrowingCallable throwingCallable, String message) {\n return assertThatThrownBy(throwingCallable).isInstanceOf(NullPointerException.class).hasMessageContaining(message);\n }\n}\n```\n.";
}
@Override
public TreeVisitor, ExecutionContext> getVisitor() {
JavaVisitor javaVisitor = new AbstractRefasterJavaVisitor() {
final JavaTemplate before = JavaTemplate
.builder("org.assertj.core.api.Assertions.assertThatNullPointerException().isThrownBy(#{throwingCallable:any(org.assertj.core.api.ThrowableAssert.ThrowingCallable)}).withMessageContaining(#{message:any(java.lang.String)})")
.javaParser(JavaParser.fromJavaVersion().classpath(JavaParser.runtimeClasspath()))
.build();
final JavaTemplate after = JavaTemplate
.builder("org.assertj.core.api.Assertions.assertThatThrownBy(#{throwingCallable:any(org.assertj.core.api.ThrowableAssert.ThrowingCallable)}).isInstanceOf(NullPointerException.class).hasMessageContaining(#{message:any(java.lang.String)})")
.javaParser(JavaParser.fromJavaVersion().classpath(JavaParser.runtimeClasspath()))
.build();
@Override
public J visitMethodInvocation(J.MethodInvocation elem, ExecutionContext ctx) {
JavaTemplate.Matcher matcher;
if ((matcher = before.matcher(getCursor())).find()) {
maybeRemoveImport("org.assertj.core.api.Assertions.assertThatNullPointerException");
return embed(
after.apply(getCursor(), elem.getCoordinates().replace(), matcher.parameter(0), matcher.parameter(1)),
getCursor(),
ctx,
SHORTEN_NAMES
);
}
return super.visitMethodInvocation(elem, ctx);
}
};
return Preconditions.check(
Preconditions.and(
new UsesType<>("org.assertj.core.api.AbstractObjectAssert", true),
new UsesType<>("org.assertj.core.api.ThrowableAssert.ThrowingCallable", true),
new UsesMethod<>("org.assertj.core.api.ThrowableAssertAlternative withMessageContaining(..)"),
new UsesMethod<>("org.assertj.core.api.ThrowableTypeAssert isThrownBy(..)"),
new UsesMethod<>("org.assertj.core.api.Assertions assertThatNullPointerException(..)")
),
javaVisitor
);
}
}
/**
* OpenRewrite recipe created for Refaster template {@code AssertJThrowingCallableRules.AssertThatThrownByNullPointerExceptionHasMessageNotContaining}.
*/
@SuppressWarnings("all")
@NonNullApi
@Generated("org.openrewrite.java.template.processor.RefasterTemplateProcessor")
public static class AssertThatThrownByNullPointerExceptionHasMessageNotContainingRecipe extends Recipe {
/**
* Instantiates a new instance.
*/
public AssertThatThrownByNullPointerExceptionHasMessageNotContainingRecipe() {}
@Override
public String getDisplayName() {
return "Refaster template `AssertJThrowingCallableRules.AssertThatThrownByNullPointerExceptionHasMessageNotContaining`";
}
@Override
public String getDescription() {
return "Recipe created for the following Refaster template:\n```java\nstatic final class AssertThatThrownByNullPointerExceptionHasMessageNotContaining {\n \n @BeforeTemplate\n @SuppressWarnings(value = \"AssertThatThrownByNullPointerException\")\n AbstractObjectAssert, ?> before(ThrowingCallable throwingCallable, String message) {\n return assertThatNullPointerException().isThrownBy(throwingCallable).withMessageNotContaining(message);\n }\n \n @AfterTemplate\n @UseImportPolicy(value = STATIC_IMPORT_ALWAYS)\n AbstractObjectAssert, ?> after(ThrowingCallable throwingCallable, String message) {\n return assertThatThrownBy(throwingCallable).isInstanceOf(NullPointerException.class).hasMessageNotContaining(message);\n }\n}\n```\n.";
}
@Override
public TreeVisitor, ExecutionContext> getVisitor() {
JavaVisitor javaVisitor = new AbstractRefasterJavaVisitor() {
final JavaTemplate before = JavaTemplate
.builder("org.assertj.core.api.Assertions.assertThatNullPointerException().isThrownBy(#{throwingCallable:any(org.assertj.core.api.ThrowableAssert.ThrowingCallable)}).withMessageNotContaining(#{message:any(java.lang.String)})")
.javaParser(JavaParser.fromJavaVersion().classpath(JavaParser.runtimeClasspath()))
.build();
final JavaTemplate after = JavaTemplate
.builder("org.assertj.core.api.Assertions.assertThatThrownBy(#{throwingCallable:any(org.assertj.core.api.ThrowableAssert.ThrowingCallable)}).isInstanceOf(NullPointerException.class).hasMessageNotContaining(#{message:any(java.lang.String)})")
.javaParser(JavaParser.fromJavaVersion().classpath(JavaParser.runtimeClasspath()))
.build();
@Override
public J visitMethodInvocation(J.MethodInvocation elem, ExecutionContext ctx) {
JavaTemplate.Matcher matcher;
if ((matcher = before.matcher(getCursor())).find()) {
maybeRemoveImport("org.assertj.core.api.Assertions.assertThatNullPointerException");
return embed(
after.apply(getCursor(), elem.getCoordinates().replace(), matcher.parameter(0), matcher.parameter(1)),
getCursor(),
ctx,
SHORTEN_NAMES
);
}
return super.visitMethodInvocation(elem, ctx);
}
};
return Preconditions.check(
Preconditions.and(
new UsesType<>("org.assertj.core.api.AbstractObjectAssert", true),
new UsesType<>("org.assertj.core.api.ThrowableAssert.ThrowingCallable", true),
new UsesMethod<>("org.assertj.core.api.ThrowableAssertAlternative withMessageNotContaining(..)"),
new UsesMethod<>("org.assertj.core.api.ThrowableTypeAssert isThrownBy(..)"),
new UsesMethod<>("org.assertj.core.api.Assertions assertThatNullPointerException(..)")
),
javaVisitor
);
}
}
/**
* OpenRewrite recipe created for Refaster template {@code AssertJThrowingCallableRules.AssertThatThrownByIOException}.
*/
@SuppressWarnings("all")
@NonNullApi
@Generated("org.openrewrite.java.template.processor.RefasterTemplateProcessor")
public static class AssertThatThrownByIOExceptionRecipe extends Recipe {
/**
* Instantiates a new instance.
*/
public AssertThatThrownByIOExceptionRecipe() {}
@Override
public String getDisplayName() {
return "Refaster template `AssertJThrowingCallableRules.AssertThatThrownByIOException`";
}
@Override
public String getDescription() {
return "Recipe created for the following Refaster template:\n```java\nstatic final class AssertThatThrownByIOException {\n \n @BeforeTemplate\n AbstractObjectAssert, ?> before(ThrowingCallable throwingCallable) {\n return assertThatIOException().isThrownBy(throwingCallable);\n }\n \n @AfterTemplate\n @UseImportPolicy(value = STATIC_IMPORT_ALWAYS)\n AbstractObjectAssert, ?> after(ThrowingCallable throwingCallable) {\n return assertThatThrownBy(throwingCallable).isInstanceOf(IOException.class);\n }\n}\n```\n.";
}
@Override
public TreeVisitor, ExecutionContext> getVisitor() {
JavaVisitor javaVisitor = new AbstractRefasterJavaVisitor() {
final JavaTemplate before = JavaTemplate
.builder("org.assertj.core.api.Assertions.assertThatIOException().isThrownBy(#{throwingCallable:any(org.assertj.core.api.ThrowableAssert.ThrowingCallable)})")
.javaParser(JavaParser.fromJavaVersion().classpath(JavaParser.runtimeClasspath()))
.build();
final JavaTemplate after = JavaTemplate
.builder("org.assertj.core.api.Assertions.assertThatThrownBy(#{throwingCallable:any(org.assertj.core.api.ThrowableAssert.ThrowingCallable)}).isInstanceOf(java.io.IOException.class)")
.javaParser(JavaParser.fromJavaVersion().classpath(JavaParser.runtimeClasspath()))
.build();
@Override
public J visitMethodInvocation(J.MethodInvocation elem, ExecutionContext ctx) {
JavaTemplate.Matcher matcher;
if ((matcher = before.matcher(getCursor())).find()) {
maybeRemoveImport("org.assertj.core.api.Assertions.assertThatIOException");
return embed(
after.apply(getCursor(), elem.getCoordinates().replace(), matcher.parameter(0)),
getCursor(),
ctx,
SHORTEN_NAMES
);
}
return super.visitMethodInvocation(elem, ctx);
}
};
return Preconditions.check(
Preconditions.and(
new UsesType<>("org.assertj.core.api.AbstractObjectAssert", true),
new UsesType<>("org.assertj.core.api.ThrowableAssert.ThrowingCallable", true),
new UsesMethod<>("org.assertj.core.api.ThrowableTypeAssert isThrownBy(..)"),
new UsesMethod<>("org.assertj.core.api.Assertions assertThatIOException(..)")
),
javaVisitor
);
}
}
/**
* OpenRewrite recipe created for Refaster template {@code AssertJThrowingCallableRules.AssertThatThrownByIOExceptionHasMessage}.
*/
@SuppressWarnings("all")
@NonNullApi
@Generated("org.openrewrite.java.template.processor.RefasterTemplateProcessor")
public static class AssertThatThrownByIOExceptionHasMessageRecipe extends Recipe {
/**
* Instantiates a new instance.
*/
public AssertThatThrownByIOExceptionHasMessageRecipe() {}
@Override
public String getDisplayName() {
return "Refaster template `AssertJThrowingCallableRules.AssertThatThrownByIOExceptionHasMessage`";
}
@Override
public String getDescription() {
return "Recipe created for the following Refaster template:\n```java\nstatic final class AssertThatThrownByIOExceptionHasMessage {\n \n @BeforeTemplate\n @SuppressWarnings(value = \"AssertThatThrownByIOException\")\n AbstractObjectAssert, ?> before(ThrowingCallable throwingCallable, String message) {\n return assertThatIOException().isThrownBy(throwingCallable).withMessage(message);\n }\n \n @AfterTemplate\n @UseImportPolicy(value = STATIC_IMPORT_ALWAYS)\n AbstractObjectAssert, ?> after(ThrowingCallable throwingCallable, String message) {\n return assertThatThrownBy(throwingCallable).isInstanceOf(IOException.class).hasMessage(message);\n }\n}\n```\n.";
}
@Override
public TreeVisitor, ExecutionContext> getVisitor() {
JavaVisitor javaVisitor = new AbstractRefasterJavaVisitor() {
final JavaTemplate before = JavaTemplate
.builder("org.assertj.core.api.Assertions.assertThatIOException().isThrownBy(#{throwingCallable:any(org.assertj.core.api.ThrowableAssert.ThrowingCallable)}).withMessage(#{message:any(java.lang.String)})")
.javaParser(JavaParser.fromJavaVersion().classpath(JavaParser.runtimeClasspath()))
.build();
final JavaTemplate after = JavaTemplate
.builder("org.assertj.core.api.Assertions.assertThatThrownBy(#{throwingCallable:any(org.assertj.core.api.ThrowableAssert.ThrowingCallable)}).isInstanceOf(java.io.IOException.class).hasMessage(#{message:any(java.lang.String)})")
.javaParser(JavaParser.fromJavaVersion().classpath(JavaParser.runtimeClasspath()))
.build();
@Override
public J visitMethodInvocation(J.MethodInvocation elem, ExecutionContext ctx) {
JavaTemplate.Matcher matcher;
if ((matcher = before.matcher(getCursor())).find()) {
maybeRemoveImport("org.assertj.core.api.Assertions.assertThatIOException");
return embed(
after.apply(getCursor(), elem.getCoordinates().replace(), matcher.parameter(0), matcher.parameter(1)),
getCursor(),
ctx,
SHORTEN_NAMES
);
}
return super.visitMethodInvocation(elem, ctx);
}
};
return Preconditions.check(
Preconditions.and(
new UsesType<>("org.assertj.core.api.AbstractObjectAssert", true),
new UsesType<>("org.assertj.core.api.ThrowableAssert.ThrowingCallable", true),
new UsesMethod<>("org.assertj.core.api.ThrowableAssertAlternative withMessage(..)"),
new UsesMethod<>("org.assertj.core.api.ThrowableTypeAssert isThrownBy(..)"),
new UsesMethod<>("org.assertj.core.api.Assertions assertThatIOException(..)")
),
javaVisitor
);
}
}
/**
* OpenRewrite recipe created for Refaster template {@code AssertJThrowingCallableRules.AssertThatThrownByIOExceptionHasMessageStartingWith}.
*/
@SuppressWarnings("all")
@NonNullApi
@Generated("org.openrewrite.java.template.processor.RefasterTemplateProcessor")
public static class AssertThatThrownByIOExceptionHasMessageStartingWithRecipe extends Recipe {
/**
* Instantiates a new instance.
*/
public AssertThatThrownByIOExceptionHasMessageStartingWithRecipe() {}
@Override
public String getDisplayName() {
return "Refaster template `AssertJThrowingCallableRules.AssertThatThrownByIOExceptionHasMessageStartingWith`";
}
@Override
public String getDescription() {
return "Recipe created for the following Refaster template:\n```java\nstatic final class AssertThatThrownByIOExceptionHasMessageStartingWith {\n \n @BeforeTemplate\n @SuppressWarnings(value = \"AssertThatThrownByIOException\")\n AbstractObjectAssert, ?> before(ThrowingCallable throwingCallable, String message) {\n return assertThatIOException().isThrownBy(throwingCallable).withMessageStartingWith(message);\n }\n \n @AfterTemplate\n @UseImportPolicy(value = STATIC_IMPORT_ALWAYS)\n AbstractObjectAssert, ?> after(ThrowingCallable throwingCallable, String message) {\n return assertThatThrownBy(throwingCallable).isInstanceOf(IOException.class).hasMessageStartingWith(message);\n }\n}\n```\n.";
}
@Override
public TreeVisitor, ExecutionContext> getVisitor() {
JavaVisitor javaVisitor = new AbstractRefasterJavaVisitor() {
final JavaTemplate before = JavaTemplate
.builder("org.assertj.core.api.Assertions.assertThatIOException().isThrownBy(#{throwingCallable:any(org.assertj.core.api.ThrowableAssert.ThrowingCallable)}).withMessageStartingWith(#{message:any(java.lang.String)})")
.javaParser(JavaParser.fromJavaVersion().classpath(JavaParser.runtimeClasspath()))
.build();
final JavaTemplate after = JavaTemplate
.builder("org.assertj.core.api.Assertions.assertThatThrownBy(#{throwingCallable:any(org.assertj.core.api.ThrowableAssert.ThrowingCallable)}).isInstanceOf(java.io.IOException.class).hasMessageStartingWith(#{message:any(java.lang.String)})")
.javaParser(JavaParser.fromJavaVersion().classpath(JavaParser.runtimeClasspath()))
.build();
@Override
public J visitMethodInvocation(J.MethodInvocation elem, ExecutionContext ctx) {
JavaTemplate.Matcher matcher;
if ((matcher = before.matcher(getCursor())).find()) {
maybeRemoveImport("org.assertj.core.api.Assertions.assertThatIOException");
return embed(
after.apply(getCursor(), elem.getCoordinates().replace(), matcher.parameter(0), matcher.parameter(1)),
getCursor(),
ctx,
SHORTEN_NAMES
);
}
return super.visitMethodInvocation(elem, ctx);
}
};
return Preconditions.check(
Preconditions.and(
new UsesType<>("org.assertj.core.api.AbstractObjectAssert", true),
new UsesType<>("org.assertj.core.api.ThrowableAssert.ThrowingCallable", true),
new UsesMethod<>("org.assertj.core.api.ThrowableAssertAlternative withMessageStartingWith(..)"),
new UsesMethod<>("org.assertj.core.api.ThrowableTypeAssert isThrownBy(..)"),
new UsesMethod<>("org.assertj.core.api.Assertions assertThatIOException(..)")
),
javaVisitor
);
}
}
/**
* OpenRewrite recipe created for Refaster template {@code AssertJThrowingCallableRules.AssertThatThrownByIOExceptionHasMessageContaining}.
*/
@SuppressWarnings("all")
@NonNullApi
@Generated("org.openrewrite.java.template.processor.RefasterTemplateProcessor")
public static class AssertThatThrownByIOExceptionHasMessageContainingRecipe extends Recipe {
/**
* Instantiates a new instance.
*/
public AssertThatThrownByIOExceptionHasMessageContainingRecipe() {}
@Override
public String getDisplayName() {
return "Refaster template `AssertJThrowingCallableRules.AssertThatThrownByIOExceptionHasMessageContaining`";
}
@Override
public String getDescription() {
return "Recipe created for the following Refaster template:\n```java\nstatic final class AssertThatThrownByIOExceptionHasMessageContaining {\n \n @BeforeTemplate\n @SuppressWarnings(value = \"AssertThatThrownByIOException\")\n AbstractObjectAssert, ?> before(ThrowingCallable throwingCallable, String message) {\n return assertThatIOException().isThrownBy(throwingCallable).withMessageContaining(message);\n }\n \n @AfterTemplate\n @UseImportPolicy(value = STATIC_IMPORT_ALWAYS)\n AbstractObjectAssert, ?> after(ThrowingCallable throwingCallable, String message) {\n return assertThatThrownBy(throwingCallable).isInstanceOf(IOException.class).hasMessageContaining(message);\n }\n}\n```\n.";
}
@Override
public TreeVisitor, ExecutionContext> getVisitor() {
JavaVisitor javaVisitor = new AbstractRefasterJavaVisitor() {
final JavaTemplate before = JavaTemplate
.builder("org.assertj.core.api.Assertions.assertThatIOException().isThrownBy(#{throwingCallable:any(org.assertj.core.api.ThrowableAssert.ThrowingCallable)}).withMessageContaining(#{message:any(java.lang.String)})")
.javaParser(JavaParser.fromJavaVersion().classpath(JavaParser.runtimeClasspath()))
.build();
final JavaTemplate after = JavaTemplate
.builder("org.assertj.core.api.Assertions.assertThatThrownBy(#{throwingCallable:any(org.assertj.core.api.ThrowableAssert.ThrowingCallable)}).isInstanceOf(java.io.IOException.class).hasMessageContaining(#{message:any(java.lang.String)})")
.javaParser(JavaParser.fromJavaVersion().classpath(JavaParser.runtimeClasspath()))
.build();
@Override
public J visitMethodInvocation(J.MethodInvocation elem, ExecutionContext ctx) {
JavaTemplate.Matcher matcher;
if ((matcher = before.matcher(getCursor())).find()) {
maybeRemoveImport("org.assertj.core.api.Assertions.assertThatIOException");
return embed(
after.apply(getCursor(), elem.getCoordinates().replace(), matcher.parameter(0), matcher.parameter(1)),
getCursor(),
ctx,
SHORTEN_NAMES
);
}
return super.visitMethodInvocation(elem, ctx);
}
};
return Preconditions.check(
Preconditions.and(
new UsesType<>("org.assertj.core.api.AbstractObjectAssert", true),
new UsesType<>("org.assertj.core.api.ThrowableAssert.ThrowingCallable", true),
new UsesMethod<>("org.assertj.core.api.ThrowableAssertAlternative withMessageContaining(..)"),
new UsesMethod<>("org.assertj.core.api.ThrowableTypeAssert isThrownBy(..)"),
new UsesMethod<>("org.assertj.core.api.Assertions assertThatIOException(..)")
),
javaVisitor
);
}
}
/**
* OpenRewrite recipe created for Refaster template {@code AssertJThrowingCallableRules.AssertThatThrownByIOExceptionHasMessageNotContaining}.
*/
@SuppressWarnings("all")
@NonNullApi
@Generated("org.openrewrite.java.template.processor.RefasterTemplateProcessor")
public static class AssertThatThrownByIOExceptionHasMessageNotContainingRecipe extends Recipe {
/**
* Instantiates a new instance.
*/
public AssertThatThrownByIOExceptionHasMessageNotContainingRecipe() {}
@Override
public String getDisplayName() {
return "Refaster template `AssertJThrowingCallableRules.AssertThatThrownByIOExceptionHasMessageNotContaining`";
}
@Override
public String getDescription() {
return "Recipe created for the following Refaster template:\n```java\nstatic final class AssertThatThrownByIOExceptionHasMessageNotContaining {\n \n @BeforeTemplate\n @SuppressWarnings(value = \"AssertThatThrownByIOException\")\n AbstractObjectAssert, ?> before(ThrowingCallable throwingCallable, String message) {\n return assertThatIOException().isThrownBy(throwingCallable).withMessageNotContaining(message);\n }\n \n @AfterTemplate\n @UseImportPolicy(value = STATIC_IMPORT_ALWAYS)\n AbstractObjectAssert, ?> after(ThrowingCallable throwingCallable, String message) {\n return assertThatThrownBy(throwingCallable).isInstanceOf(IOException.class).hasMessageNotContaining(message);\n }\n}\n```\n.";
}
@Override
public TreeVisitor, ExecutionContext> getVisitor() {
JavaVisitor javaVisitor = new AbstractRefasterJavaVisitor() {
final JavaTemplate before = JavaTemplate
.builder("org.assertj.core.api.Assertions.assertThatIOException().isThrownBy(#{throwingCallable:any(org.assertj.core.api.ThrowableAssert.ThrowingCallable)}).withMessageNotContaining(#{message:any(java.lang.String)})")
.javaParser(JavaParser.fromJavaVersion().classpath(JavaParser.runtimeClasspath()))
.build();
final JavaTemplate after = JavaTemplate
.builder("org.assertj.core.api.Assertions.assertThatThrownBy(#{throwingCallable:any(org.assertj.core.api.ThrowableAssert.ThrowingCallable)}).isInstanceOf(java.io.IOException.class).hasMessageNotContaining(#{message:any(java.lang.String)})")
.javaParser(JavaParser.fromJavaVersion().classpath(JavaParser.runtimeClasspath()))
.build();
@Override
public J visitMethodInvocation(J.MethodInvocation elem, ExecutionContext ctx) {
JavaTemplate.Matcher matcher;
if ((matcher = before.matcher(getCursor())).find()) {
maybeRemoveImport("org.assertj.core.api.Assertions.assertThatIOException");
return embed(
after.apply(getCursor(), elem.getCoordinates().replace(), matcher.parameter(0), matcher.parameter(1)),
getCursor(),
ctx,
SHORTEN_NAMES
);
}
return super.visitMethodInvocation(elem, ctx);
}
};
return Preconditions.check(
Preconditions.and(
new UsesType<>("org.assertj.core.api.AbstractObjectAssert", true),
new UsesType<>("org.assertj.core.api.ThrowableAssert.ThrowingCallable", true),
new UsesMethod<>("org.assertj.core.api.ThrowableAssertAlternative withMessageNotContaining(..)"),
new UsesMethod<>("org.assertj.core.api.ThrowableTypeAssert isThrownBy(..)"),
new UsesMethod<>("org.assertj.core.api.Assertions assertThatIOException(..)")
),
javaVisitor
);
}
}
/**
* OpenRewrite recipe created for Refaster template {@code AssertJThrowingCallableRules.AssertThatThrownBy}.
*/
@SuppressWarnings("all")
@NonNullApi
@Generated("org.openrewrite.java.template.processor.RefasterTemplateProcessor")
public static class AssertThatThrownByRecipe extends Recipe {
/**
* Instantiates a new instance.
*/
public AssertThatThrownByRecipe() {}
@Override
public String getDisplayName() {
return "Refaster template `AssertJThrowingCallableRules.AssertThatThrownBy`";
}
@Override
public String getDescription() {
return "Recipe created for the following Refaster template:\n```java\nstatic final class AssertThatThrownBy {\n \n @BeforeTemplate\n AbstractObjectAssert, ?> before(ThrowingCallable throwingCallable, Class extends Throwable> exceptionType) {\n return assertThatExceptionOfType(exceptionType).isThrownBy(throwingCallable);\n }\n \n @AfterTemplate\n @UseImportPolicy(value = STATIC_IMPORT_ALWAYS)\n AbstractObjectAssert, ?> after(ThrowingCallable throwingCallable, Class extends Throwable> exceptionType) {\n return assertThatThrownBy(throwingCallable).isInstanceOf(exceptionType);\n }\n}\n```\n.";
}
@Override
public TreeVisitor, ExecutionContext> getVisitor() {
JavaVisitor javaVisitor = new AbstractRefasterJavaVisitor() {
final JavaTemplate before = JavaTemplate
.builder("org.assertj.core.api.Assertions.assertThatExceptionOfType(#{exceptionType:any(java.lang.Class extends java.lang.Throwable>)}).isThrownBy(#{throwingCallable:any(org.assertj.core.api.ThrowableAssert.ThrowingCallable)})")
.javaParser(JavaParser.fromJavaVersion().classpath(JavaParser.runtimeClasspath()))
.build();
final JavaTemplate after = JavaTemplate
.builder("org.assertj.core.api.Assertions.assertThatThrownBy(#{throwingCallable:any(org.assertj.core.api.ThrowableAssert.ThrowingCallable)}).isInstanceOf(#{exceptionType:any(java.lang.Class extends java.lang.Throwable>)})")
.javaParser(JavaParser.fromJavaVersion().classpath(JavaParser.runtimeClasspath()))
.build();
@Override
public J visitMethodInvocation(J.MethodInvocation elem, ExecutionContext ctx) {
JavaTemplate.Matcher matcher;
if ((matcher = before.matcher(getCursor())).find()) {
maybeRemoveImport("org.assertj.core.api.Assertions.assertThatExceptionOfType");
return embed(
after.apply(getCursor(), elem.getCoordinates().replace(), matcher.parameter(1), matcher.parameter(0)),
getCursor(),
ctx,
SHORTEN_NAMES
);
}
return super.visitMethodInvocation(elem, ctx);
}
};
return Preconditions.check(
Preconditions.and(
new UsesType<>("org.assertj.core.api.AbstractObjectAssert", true),
new UsesType<>("org.assertj.core.api.ThrowableAssert.ThrowingCallable", true),
new UsesMethod<>("org.assertj.core.api.ThrowableTypeAssert isThrownBy(..)"),
new UsesMethod<>("org.assertj.core.api.Assertions assertThatExceptionOfType(..)")
),
javaVisitor
);
}
}
/**
* OpenRewrite recipe created for Refaster template {@code AssertJThrowingCallableRules.AssertThatThrownByHasMessage}.
*/
@SuppressWarnings("all")
@NonNullApi
@Generated("org.openrewrite.java.template.processor.RefasterTemplateProcessor")
public static class AssertThatThrownByHasMessageRecipe extends Recipe {
/**
* Instantiates a new instance.
*/
public AssertThatThrownByHasMessageRecipe() {}
@Override
public String getDisplayName() {
return "Refaster template `AssertJThrowingCallableRules.AssertThatThrownByHasMessage`";
}
@Override
public String getDescription() {
return "Recipe created for the following Refaster template:\n```java\nstatic final class AssertThatThrownByHasMessage {\n \n @BeforeTemplate\n @SuppressWarnings(value = \"AssertThatThrownBy\")\n AbstractObjectAssert, ?> before(ThrowingCallable throwingCallable, Class extends Throwable> exceptionType, String message) {\n return assertThatExceptionOfType(exceptionType).isThrownBy(throwingCallable).withMessage(message);\n }\n \n @AfterTemplate\n @UseImportPolicy(value = STATIC_IMPORT_ALWAYS)\n AbstractObjectAssert, ?> after(ThrowingCallable throwingCallable, Class extends Throwable> exceptionType, String message) {\n return assertThatThrownBy(throwingCallable).isInstanceOf(exceptionType).hasMessage(message);\n }\n}\n```\n.";
}
@Override
public TreeVisitor, ExecutionContext> getVisitor() {
JavaVisitor javaVisitor = new AbstractRefasterJavaVisitor() {
final JavaTemplate before = JavaTemplate
.builder("org.assertj.core.api.Assertions.assertThatExceptionOfType(#{exceptionType:any(java.lang.Class extends java.lang.Throwable>)}).isThrownBy(#{throwingCallable:any(org.assertj.core.api.ThrowableAssert.ThrowingCallable)}).withMessage(#{message:any(java.lang.String)})")
.javaParser(JavaParser.fromJavaVersion().classpath(JavaParser.runtimeClasspath()))
.build();
final JavaTemplate after = JavaTemplate
.builder("org.assertj.core.api.Assertions.assertThatThrownBy(#{throwingCallable:any(org.assertj.core.api.ThrowableAssert.ThrowingCallable)}).isInstanceOf(#{exceptionType:any(java.lang.Class extends java.lang.Throwable>)}).hasMessage(#{message:any(java.lang.String)})")
.javaParser(JavaParser.fromJavaVersion().classpath(JavaParser.runtimeClasspath()))
.build();
@Override
public J visitMethodInvocation(J.MethodInvocation elem, ExecutionContext ctx) {
JavaTemplate.Matcher matcher;
if ((matcher = before.matcher(getCursor())).find()) {
maybeRemoveImport("org.assertj.core.api.Assertions.assertThatExceptionOfType");
return embed(
after.apply(getCursor(), elem.getCoordinates().replace(), matcher.parameter(1), matcher.parameter(0), matcher.parameter(2)),
getCursor(),
ctx,
SHORTEN_NAMES
);
}
return super.visitMethodInvocation(elem, ctx);
}
};
return Preconditions.check(
Preconditions.and(
new UsesType<>("org.assertj.core.api.AbstractObjectAssert", true),
new UsesType<>("org.assertj.core.api.ThrowableAssert.ThrowingCallable", true),
new UsesMethod<>("org.assertj.core.api.ThrowableAssertAlternative withMessage(..)"),
new UsesMethod<>("org.assertj.core.api.ThrowableTypeAssert isThrownBy(..)"),
new UsesMethod<>("org.assertj.core.api.Assertions assertThatExceptionOfType(..)")
),
javaVisitor
);
}
}
/**
* OpenRewrite recipe created for Refaster template {@code AssertJThrowingCallableRules.AssertThatThrownByHasMessageStartingWith}.
*/
@SuppressWarnings("all")
@NonNullApi
@Generated("org.openrewrite.java.template.processor.RefasterTemplateProcessor")
public static class AssertThatThrownByHasMessageStartingWithRecipe extends Recipe {
/**
* Instantiates a new instance.
*/
public AssertThatThrownByHasMessageStartingWithRecipe() {}
@Override
public String getDisplayName() {
return "Refaster template `AssertJThrowingCallableRules.AssertThatThrownByHasMessageStartingWith`";
}
@Override
public String getDescription() {
return "Recipe created for the following Refaster template:\n```java\nstatic final class AssertThatThrownByHasMessageStartingWith {\n \n @BeforeTemplate\n @SuppressWarnings(value = \"AssertThatThrownBy\")\n AbstractObjectAssert, ?> before(ThrowingCallable throwingCallable, Class extends Throwable> exceptionType, String message) {\n return assertThatExceptionOfType(exceptionType).isThrownBy(throwingCallable).withMessageStartingWith(message);\n }\n \n @AfterTemplate\n @UseImportPolicy(value = STATIC_IMPORT_ALWAYS)\n AbstractObjectAssert, ?> after(ThrowingCallable throwingCallable, Class extends Throwable> exceptionType, String message) {\n return assertThatThrownBy(throwingCallable).isInstanceOf(exceptionType).hasMessageStartingWith(message);\n }\n}\n```\n.";
}
@Override
public TreeVisitor, ExecutionContext> getVisitor() {
JavaVisitor javaVisitor = new AbstractRefasterJavaVisitor() {
final JavaTemplate before = JavaTemplate
.builder("org.assertj.core.api.Assertions.assertThatExceptionOfType(#{exceptionType:any(java.lang.Class extends java.lang.Throwable>)}).isThrownBy(#{throwingCallable:any(org.assertj.core.api.ThrowableAssert.ThrowingCallable)}).withMessageStartingWith(#{message:any(java.lang.String)})")
.javaParser(JavaParser.fromJavaVersion().classpath(JavaParser.runtimeClasspath()))
.build();
final JavaTemplate after = JavaTemplate
.builder("org.assertj.core.api.Assertions.assertThatThrownBy(#{throwingCallable:any(org.assertj.core.api.ThrowableAssert.ThrowingCallable)}).isInstanceOf(#{exceptionType:any(java.lang.Class extends java.lang.Throwable>)}).hasMessageStartingWith(#{message:any(java.lang.String)})")
.javaParser(JavaParser.fromJavaVersion().classpath(JavaParser.runtimeClasspath()))
.build();
@Override
public J visitMethodInvocation(J.MethodInvocation elem, ExecutionContext ctx) {
JavaTemplate.Matcher matcher;
if ((matcher = before.matcher(getCursor())).find()) {
maybeRemoveImport("org.assertj.core.api.Assertions.assertThatExceptionOfType");
return embed(
after.apply(getCursor(), elem.getCoordinates().replace(), matcher.parameter(1), matcher.parameter(0), matcher.parameter(2)),
getCursor(),
ctx,
SHORTEN_NAMES
);
}
return super.visitMethodInvocation(elem, ctx);
}
};
return Preconditions.check(
Preconditions.and(
new UsesType<>("org.assertj.core.api.AbstractObjectAssert", true),
new UsesType<>("org.assertj.core.api.ThrowableAssert.ThrowingCallable", true),
new UsesMethod<>("org.assertj.core.api.ThrowableAssertAlternative withMessageStartingWith(..)"),
new UsesMethod<>("org.assertj.core.api.ThrowableTypeAssert isThrownBy(..)"),
new UsesMethod<>("org.assertj.core.api.Assertions assertThatExceptionOfType(..)")
),
javaVisitor
);
}
}
/**
* OpenRewrite recipe created for Refaster template {@code AssertJThrowingCallableRules.AssertThatThrownByHasMessageContaining}.
*/
@SuppressWarnings("all")
@NonNullApi
@Generated("org.openrewrite.java.template.processor.RefasterTemplateProcessor")
public static class AssertThatThrownByHasMessageContainingRecipe extends Recipe {
/**
* Instantiates a new instance.
*/
public AssertThatThrownByHasMessageContainingRecipe() {}
@Override
public String getDisplayName() {
return "Refaster template `AssertJThrowingCallableRules.AssertThatThrownByHasMessageContaining`";
}
@Override
public String getDescription() {
return "Recipe created for the following Refaster template:\n```java\nstatic final class AssertThatThrownByHasMessageContaining {\n \n @BeforeTemplate\n @SuppressWarnings(value = \"AssertThatThrownBy\")\n AbstractObjectAssert, ?> before(ThrowingCallable throwingCallable, Class extends Throwable> exceptionType, String message) {\n return assertThatExceptionOfType(exceptionType).isThrownBy(throwingCallable).withMessageContaining(message);\n }\n \n @AfterTemplate\n @UseImportPolicy(value = STATIC_IMPORT_ALWAYS)\n AbstractObjectAssert, ?> after(ThrowingCallable throwingCallable, Class extends Throwable> exceptionType, String message) {\n return assertThatThrownBy(throwingCallable).isInstanceOf(exceptionType).hasMessageContaining(message);\n }\n}\n```\n.";
}
@Override
public TreeVisitor, ExecutionContext> getVisitor() {
JavaVisitor javaVisitor = new AbstractRefasterJavaVisitor() {
final JavaTemplate before = JavaTemplate
.builder("org.assertj.core.api.Assertions.assertThatExceptionOfType(#{exceptionType:any(java.lang.Class extends java.lang.Throwable>)}).isThrownBy(#{throwingCallable:any(org.assertj.core.api.ThrowableAssert.ThrowingCallable)}).withMessageContaining(#{message:any(java.lang.String)})")
.javaParser(JavaParser.fromJavaVersion().classpath(JavaParser.runtimeClasspath()))
.build();
final JavaTemplate after = JavaTemplate
.builder("org.assertj.core.api.Assertions.assertThatThrownBy(#{throwingCallable:any(org.assertj.core.api.ThrowableAssert.ThrowingCallable)}).isInstanceOf(#{exceptionType:any(java.lang.Class extends java.lang.Throwable>)}).hasMessageContaining(#{message:any(java.lang.String)})")
.javaParser(JavaParser.fromJavaVersion().classpath(JavaParser.runtimeClasspath()))
.build();
@Override
public J visitMethodInvocation(J.MethodInvocation elem, ExecutionContext ctx) {
JavaTemplate.Matcher matcher;
if ((matcher = before.matcher(getCursor())).find()) {
maybeRemoveImport("org.assertj.core.api.Assertions.assertThatExceptionOfType");
return embed(
after.apply(getCursor(), elem.getCoordinates().replace(), matcher.parameter(1), matcher.parameter(0), matcher.parameter(2)),
getCursor(),
ctx,
SHORTEN_NAMES
);
}
return super.visitMethodInvocation(elem, ctx);
}
};
return Preconditions.check(
Preconditions.and(
new UsesType<>("org.assertj.core.api.AbstractObjectAssert", true),
new UsesType<>("org.assertj.core.api.ThrowableAssert.ThrowingCallable", true),
new UsesMethod<>("org.assertj.core.api.ThrowableAssertAlternative withMessageContaining(..)"),
new UsesMethod<>("org.assertj.core.api.ThrowableTypeAssert isThrownBy(..)"),
new UsesMethod<>("org.assertj.core.api.Assertions assertThatExceptionOfType(..)")
),
javaVisitor
);
}
}
/**
* OpenRewrite recipe created for Refaster template {@code AssertJThrowingCallableRules.AssertThatThrownByHasMessageNotContaining}.
*/
@SuppressWarnings("all")
@NonNullApi
@Generated("org.openrewrite.java.template.processor.RefasterTemplateProcessor")
public static class AssertThatThrownByHasMessageNotContainingRecipe extends Recipe {
/**
* Instantiates a new instance.
*/
public AssertThatThrownByHasMessageNotContainingRecipe() {}
@Override
public String getDisplayName() {
return "Refaster template `AssertJThrowingCallableRules.AssertThatThrownByHasMessageNotContaining`";
}
@Override
public String getDescription() {
return "Recipe created for the following Refaster template:\n```java\nstatic final class AssertThatThrownByHasMessageNotContaining {\n \n @BeforeTemplate\n @SuppressWarnings(value = \"AssertThatThrownBy\")\n AbstractObjectAssert, ?> before(ThrowingCallable throwingCallable, Class extends Throwable> exceptionType, String message) {\n return assertThatExceptionOfType(exceptionType).isThrownBy(throwingCallable).withMessageNotContaining(message);\n }\n \n @AfterTemplate\n @UseImportPolicy(value = STATIC_IMPORT_ALWAYS)\n AbstractObjectAssert, ?> after(ThrowingCallable throwingCallable, Class extends Throwable> exceptionType, String message) {\n return assertThatThrownBy(throwingCallable).isInstanceOf(exceptionType).hasMessageNotContaining(message);\n }\n}\n```\n.";
}
@Override
public TreeVisitor, ExecutionContext> getVisitor() {
JavaVisitor javaVisitor = new AbstractRefasterJavaVisitor() {
final JavaTemplate before = JavaTemplate
.builder("org.assertj.core.api.Assertions.assertThatExceptionOfType(#{exceptionType:any(java.lang.Class extends java.lang.Throwable>)}).isThrownBy(#{throwingCallable:any(org.assertj.core.api.ThrowableAssert.ThrowingCallable)}).withMessageNotContaining(#{message:any(java.lang.String)})")
.javaParser(JavaParser.fromJavaVersion().classpath(JavaParser.runtimeClasspath()))
.build();
final JavaTemplate after = JavaTemplate
.builder("org.assertj.core.api.Assertions.assertThatThrownBy(#{throwingCallable:any(org.assertj.core.api.ThrowableAssert.ThrowingCallable)}).isInstanceOf(#{exceptionType:any(java.lang.Class extends java.lang.Throwable>)}).hasMessageNotContaining(#{message:any(java.lang.String)})")
.javaParser(JavaParser.fromJavaVersion().classpath(JavaParser.runtimeClasspath()))
.build();
@Override
public J visitMethodInvocation(J.MethodInvocation elem, ExecutionContext ctx) {
JavaTemplate.Matcher matcher;
if ((matcher = before.matcher(getCursor())).find()) {
maybeRemoveImport("org.assertj.core.api.Assertions.assertThatExceptionOfType");
return embed(
after.apply(getCursor(), elem.getCoordinates().replace(), matcher.parameter(1), matcher.parameter(0), matcher.parameter(2)),
getCursor(),
ctx,
SHORTEN_NAMES
);
}
return super.visitMethodInvocation(elem, ctx);
}
};
return Preconditions.check(
Preconditions.and(
new UsesType<>("org.assertj.core.api.AbstractObjectAssert", true),
new UsesType<>("org.assertj.core.api.ThrowableAssert.ThrowingCallable", true),
new UsesMethod<>("org.assertj.core.api.ThrowableAssertAlternative withMessageNotContaining(..)"),
new UsesMethod<>("org.assertj.core.api.ThrowableTypeAssert isThrownBy(..)"),
new UsesMethod<>("org.assertj.core.api.Assertions assertThatExceptionOfType(..)")
),
javaVisitor
);
}
}
}