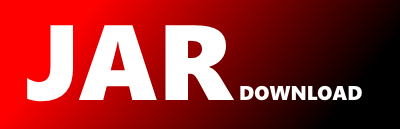
tech.picnic.errorprone.refasterrules.BigDecimalRulesRecipes Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of error-prone-contrib Show documentation
Show all versions of error-prone-contrib Show documentation
Extra Error Prone plugins by Picnic.
package tech.picnic.errorprone.refasterrules;
import org.openrewrite.ExecutionContext;
import org.openrewrite.Preconditions;
import org.openrewrite.Recipe;
import org.openrewrite.TreeVisitor;
import org.openrewrite.internal.lang.NonNullApi;
import org.openrewrite.java.JavaParser;
import org.openrewrite.java.JavaTemplate;
import org.openrewrite.java.JavaVisitor;
import org.openrewrite.java.search.*;
import org.openrewrite.java.template.Primitive;
import org.openrewrite.java.template.function.*;
import org.openrewrite.java.template.internal.AbstractRefasterJavaVisitor;
import org.openrewrite.java.tree.*;
import javax.annotation.Generated;
import java.util.*;
import static org.openrewrite.java.template.internal.AbstractRefasterJavaVisitor.EmbeddingOption.*;
/**
* OpenRewrite recipes created for Refaster template {@code tech.picnic.errorprone.refasterrules.BigDecimalRules}.
*/
@SuppressWarnings("all")
@Generated("org.openrewrite.java.template.processor.RefasterTemplateProcessor")
public class BigDecimalRulesRecipes extends Recipe {
/**
* Instantiates a new instance.
*/
public BigDecimalRulesRecipes() {}
@Override
public String getDisplayName() {
return "Refaster rules related to expressions dealing with `BigDecimal`s";
}
@Override
public String getDescription() {
return "Refaster template recipes for `tech.picnic.errorprone.refasterrules.BigDecimalRules`. [Source](https://error-prone.picnic.tech/refasterrules/BigDecimalRules).";
}
@Override
public List getRecipeList() {
return Arrays.asList(
new BigDecimalZeroRecipe(),
new BigDecimalOneRecipe(),
new BigDecimalTenRecipe(),
new BigDecimalValueOfRecipe(),
new BigDecimalSignumIsZeroRecipe(),
new BigDecimalSignumIsPositiveRecipe(),
new BigDecimalSignumIsNegativeRecipe()
);
}
/**
* OpenRewrite recipe created for Refaster template {@code BigDecimalRules.BigDecimalZero}.
*/
@SuppressWarnings("all")
@NonNullApi
@Generated("org.openrewrite.java.template.processor.RefasterTemplateProcessor")
public static class BigDecimalZeroRecipe extends Recipe {
/**
* Instantiates a new instance.
*/
public BigDecimalZeroRecipe() {}
@Override
public String getDisplayName() {
return "Prefer using the constant `BigDecimal#ZERO` when possible";
}
@Override
public String getDescription() {
return "Recipe created for the following Refaster template:\n```java\nstatic final class BigDecimalZero {\n \n @BeforeTemplate\n BigDecimal before() {\n return Refaster.anyOf(BigDecimal.valueOf(0), new BigDecimal(\"0\"));\n }\n \n @AfterTemplate\n BigDecimal after() {\n return BigDecimal.ZERO;\n }\n}\n```\n.";
}
@Override
public TreeVisitor, ExecutionContext> getVisitor() {
JavaVisitor javaVisitor = new AbstractRefasterJavaVisitor() {
final JavaTemplate before$0 = JavaTemplate
.builder("java.math.BigDecimal.valueOf(0)")
.build();
final JavaTemplate before$1 = JavaTemplate
.builder("new java.math.BigDecimal(\"0\")")
.build();
final JavaTemplate after = JavaTemplate
.builder("java.math.BigDecimal.ZERO")
.build();
@Override
public J visitMethodInvocation(J.MethodInvocation elem, ExecutionContext ctx) {
JavaTemplate.Matcher matcher;
if ((matcher = before$0.matcher(getCursor())).find()) {
return embed(
after.apply(getCursor(), elem.getCoordinates().replace()),
getCursor(),
ctx,
SHORTEN_NAMES
);
}
if ((matcher = before$1.matcher(getCursor())).find()) {
return embed(
after.apply(getCursor(), elem.getCoordinates().replace()),
getCursor(),
ctx,
SHORTEN_NAMES
);
}
return super.visitMethodInvocation(elem, ctx);
}
};
return Preconditions.check(
Preconditions.and(
new UsesType<>("java.math.BigDecimal", true),
Preconditions.or(
new UsesMethod<>("java.math.BigDecimal valueOf(..)"),
new UsesMethod<>("java.math.BigDecimal (..)")
)
),
javaVisitor
);
}
}
/**
* OpenRewrite recipe created for Refaster template {@code BigDecimalRules.BigDecimalOne}.
*/
@SuppressWarnings("all")
@NonNullApi
@Generated("org.openrewrite.java.template.processor.RefasterTemplateProcessor")
public static class BigDecimalOneRecipe extends Recipe {
/**
* Instantiates a new instance.
*/
public BigDecimalOneRecipe() {}
@Override
public String getDisplayName() {
return "Prefer using the constant `BigDecimal#ONE` when possible";
}
@Override
public String getDescription() {
return "Recipe created for the following Refaster template:\n```java\nstatic final class BigDecimalOne {\n \n @BeforeTemplate\n BigDecimal before() {\n return Refaster.anyOf(BigDecimal.valueOf(1), new BigDecimal(\"1\"));\n }\n \n @AfterTemplate\n BigDecimal after() {\n return BigDecimal.ONE;\n }\n}\n```\n.";
}
@Override
public TreeVisitor, ExecutionContext> getVisitor() {
JavaVisitor javaVisitor = new AbstractRefasterJavaVisitor() {
final JavaTemplate before$0 = JavaTemplate
.builder("java.math.BigDecimal.valueOf(1)")
.build();
final JavaTemplate before$1 = JavaTemplate
.builder("new java.math.BigDecimal(\"1\")")
.build();
final JavaTemplate after = JavaTemplate
.builder("java.math.BigDecimal.ONE")
.build();
@Override
public J visitMethodInvocation(J.MethodInvocation elem, ExecutionContext ctx) {
JavaTemplate.Matcher matcher;
if ((matcher = before$0.matcher(getCursor())).find()) {
return embed(
after.apply(getCursor(), elem.getCoordinates().replace()),
getCursor(),
ctx,
SHORTEN_NAMES
);
}
if ((matcher = before$1.matcher(getCursor())).find()) {
return embed(
after.apply(getCursor(), elem.getCoordinates().replace()),
getCursor(),
ctx,
SHORTEN_NAMES
);
}
return super.visitMethodInvocation(elem, ctx);
}
};
return Preconditions.check(
Preconditions.and(
new UsesType<>("java.math.BigDecimal", true),
Preconditions.or(
new UsesMethod<>("java.math.BigDecimal valueOf(..)"),
new UsesMethod<>("java.math.BigDecimal (..)")
)
),
javaVisitor
);
}
}
/**
* OpenRewrite recipe created for Refaster template {@code BigDecimalRules.BigDecimalTen}.
*/
@SuppressWarnings("all")
@NonNullApi
@Generated("org.openrewrite.java.template.processor.RefasterTemplateProcessor")
public static class BigDecimalTenRecipe extends Recipe {
/**
* Instantiates a new instance.
*/
public BigDecimalTenRecipe() {}
@Override
public String getDisplayName() {
return "Prefer using the constant `BigDecimal#TEN` when possible";
}
@Override
public String getDescription() {
return "Recipe created for the following Refaster template:\n```java\nstatic final class BigDecimalTen {\n \n @BeforeTemplate\n BigDecimal before() {\n return Refaster.anyOf(BigDecimal.valueOf(10), new BigDecimal(\"10\"));\n }\n \n @AfterTemplate\n BigDecimal after() {\n return BigDecimal.TEN;\n }\n}\n```\n.";
}
@Override
public TreeVisitor, ExecutionContext> getVisitor() {
JavaVisitor javaVisitor = new AbstractRefasterJavaVisitor() {
final JavaTemplate before$0 = JavaTemplate
.builder("java.math.BigDecimal.valueOf(10)")
.build();
final JavaTemplate before$1 = JavaTemplate
.builder("new java.math.BigDecimal(\"10\")")
.build();
final JavaTemplate after = JavaTemplate
.builder("java.math.BigDecimal.TEN")
.build();
@Override
public J visitMethodInvocation(J.MethodInvocation elem, ExecutionContext ctx) {
JavaTemplate.Matcher matcher;
if ((matcher = before$0.matcher(getCursor())).find()) {
return embed(
after.apply(getCursor(), elem.getCoordinates().replace()),
getCursor(),
ctx,
SHORTEN_NAMES
);
}
if ((matcher = before$1.matcher(getCursor())).find()) {
return embed(
after.apply(getCursor(), elem.getCoordinates().replace()),
getCursor(),
ctx,
SHORTEN_NAMES
);
}
return super.visitMethodInvocation(elem, ctx);
}
};
return Preconditions.check(
Preconditions.and(
new UsesType<>("java.math.BigDecimal", true),
Preconditions.or(
new UsesMethod<>("java.math.BigDecimal valueOf(..)"),
new UsesMethod<>("java.math.BigDecimal (..)")
)
),
javaVisitor
);
}
}
/**
* OpenRewrite recipe created for Refaster template {@code BigDecimalRules.BigDecimalValueOf}.
*/
@SuppressWarnings("all")
@NonNullApi
@Generated("org.openrewrite.java.template.processor.RefasterTemplateProcessor")
public static class BigDecimalValueOfRecipe extends Recipe {
/**
* Instantiates a new instance.
*/
public BigDecimalValueOfRecipe() {}
@Override
public String getDisplayName() {
return "Prefer `BigDecimal#valueOf(double)` over the associated constructor";
}
@Override
public String getDescription() {
return "Recipe created for the following Refaster template:\n```java\nstatic final class BigDecimalValueOf {\n \n @BeforeTemplate\n @SuppressWarnings(value = \"java:S2111\")\n BigDecimal before(double value) {\n return new BigDecimal(value);\n }\n \n @AfterTemplate\n BigDecimal after(double value) {\n return BigDecimal.valueOf(value);\n }\n}\n```\n.";
}
@Override
public TreeVisitor, ExecutionContext> getVisitor() {
JavaVisitor javaVisitor = new AbstractRefasterJavaVisitor() {
final JavaTemplate before = JavaTemplate
.builder("new java.math.BigDecimal(#{value:any(double)})")
.build();
final JavaTemplate after = JavaTemplate
.builder("java.math.BigDecimal.valueOf(#{value:any(double)})")
.build();
@Override
public J visitNewClass(J.NewClass elem, ExecutionContext ctx) {
JavaTemplate.Matcher matcher;
if ((matcher = before.matcher(getCursor())).find()) {
return embed(
after.apply(getCursor(), elem.getCoordinates().replace(), matcher.parameter(0)),
getCursor(),
ctx,
SHORTEN_NAMES
);
}
return super.visitNewClass(elem, ctx);
}
};
return Preconditions.check(
Preconditions.and(
new UsesType<>("java.math.BigDecimal", true),
new UsesMethod<>("java.math.BigDecimal (..)")
),
javaVisitor
);
}
}
/**
* OpenRewrite recipe created for Refaster template {@code BigDecimalRules.BigDecimalSignumIsZero}.
*/
@SuppressWarnings("all")
@NonNullApi
@Generated("org.openrewrite.java.template.processor.RefasterTemplateProcessor")
public static class BigDecimalSignumIsZeroRecipe extends Recipe {
/**
* Instantiates a new instance.
*/
public BigDecimalSignumIsZeroRecipe() {}
@Override
public String getDisplayName() {
return "Prefer using `BigDecimal#signum()` over more contrived alternatives";
}
@Override
public String getDescription() {
return "Recipe created for the following Refaster template:\n```java\nstatic final class BigDecimalSignumIsZero {\n \n @BeforeTemplate\n boolean before(BigDecimal value) {\n return Refaster.anyOf(value.compareTo(BigDecimal.ZERO) == 0, BigDecimal.ZERO.compareTo(value) == 0);\n }\n \n @AfterTemplate\n @AlsoNegation\n boolean after(BigDecimal value) {\n return value.signum() == 0;\n }\n}\n```\n.";
}
@Override
public TreeVisitor, ExecutionContext> getVisitor() {
JavaVisitor javaVisitor = new AbstractRefasterJavaVisitor() {
final JavaTemplate before$0 = JavaTemplate
.builder("#{value:any(java.math.BigDecimal)}.compareTo(java.math.BigDecimal.ZERO) == 0")
.build();
final JavaTemplate before$1 = JavaTemplate
.builder("java.math.BigDecimal.ZERO.compareTo(#{value:any(java.math.BigDecimal)}) == 0")
.build();
final JavaTemplate after = JavaTemplate
.builder("#{value:any(java.math.BigDecimal)}.signum() == 0")
.build();
@Override
public J visitMethodInvocation(J.MethodInvocation elem, ExecutionContext ctx) {
JavaTemplate.Matcher matcher;
if ((matcher = before$0.matcher(getCursor())).find()) {
return embed(
after.apply(getCursor(), elem.getCoordinates().replace(), matcher.parameter(0)),
getCursor(),
ctx,
SHORTEN_NAMES, SIMPLIFY_BOOLEANS
);
}
if ((matcher = before$1.matcher(getCursor())).find()) {
return embed(
after.apply(getCursor(), elem.getCoordinates().replace(), matcher.parameter(0)),
getCursor(),
ctx,
SHORTEN_NAMES, SIMPLIFY_BOOLEANS
);
}
return super.visitMethodInvocation(elem, ctx);
}
};
return Preconditions.check(
Preconditions.and(
new UsesType<>("java.math.BigDecimal", true),
new UsesMethod<>("java.math.BigDecimal compareTo(..)")
),
javaVisitor
);
}
}
/**
* OpenRewrite recipe created for Refaster template {@code BigDecimalRules.BigDecimalSignumIsPositive}.
*/
@SuppressWarnings("all")
@NonNullApi
@Generated("org.openrewrite.java.template.processor.RefasterTemplateProcessor")
public static class BigDecimalSignumIsPositiveRecipe extends Recipe {
/**
* Instantiates a new instance.
*/
public BigDecimalSignumIsPositiveRecipe() {}
@Override
public String getDisplayName() {
return "Prefer a `BigDecimal#signum()` comparison to 1 over more contrived or less clear alternatives";
}
@Override
public String getDescription() {
return "Recipe created for the following Refaster template:\n```java\nstatic final class BigDecimalSignumIsPositive {\n \n @BeforeTemplate\n boolean before(BigDecimal value) {\n return Refaster.anyOf(value.compareTo(BigDecimal.ZERO) > 0, BigDecimal.ZERO.compareTo(value) < 0, value.signum() > 0, value.signum() >= 1);\n }\n \n @AfterTemplate\n @AlsoNegation\n boolean after(BigDecimal value) {\n return value.signum() == 1;\n }\n}\n```\n.";
}
@Override
public TreeVisitor, ExecutionContext> getVisitor() {
JavaVisitor javaVisitor = new AbstractRefasterJavaVisitor() {
final JavaTemplate before$0 = JavaTemplate
.builder("#{value:any(java.math.BigDecimal)}.compareTo(java.math.BigDecimal.ZERO) > 0")
.build();
final JavaTemplate before$1 = JavaTemplate
.builder("java.math.BigDecimal.ZERO.compareTo(#{value:any(java.math.BigDecimal)}) < 0")
.build();
final JavaTemplate before$2 = JavaTemplate
.builder("#{value:any(java.math.BigDecimal)}.signum() > 0")
.build();
final JavaTemplate before$3 = JavaTemplate
.builder("#{value:any(java.math.BigDecimal)}.signum() >= 1")
.build();
final JavaTemplate after = JavaTemplate
.builder("#{value:any(java.math.BigDecimal)}.signum() == 1")
.build();
@Override
public J visitMethodInvocation(J.MethodInvocation elem, ExecutionContext ctx) {
JavaTemplate.Matcher matcher;
if ((matcher = before$0.matcher(getCursor())).find()) {
return embed(
after.apply(getCursor(), elem.getCoordinates().replace(), matcher.parameter(0)),
getCursor(),
ctx,
SHORTEN_NAMES, SIMPLIFY_BOOLEANS
);
}
if ((matcher = before$1.matcher(getCursor())).find()) {
return embed(
after.apply(getCursor(), elem.getCoordinates().replace(), matcher.parameter(0)),
getCursor(),
ctx,
SHORTEN_NAMES, SIMPLIFY_BOOLEANS
);
}
if ((matcher = before$2.matcher(getCursor())).find()) {
return embed(
after.apply(getCursor(), elem.getCoordinates().replace(), matcher.parameter(0)),
getCursor(),
ctx,
SHORTEN_NAMES, SIMPLIFY_BOOLEANS
);
}
if ((matcher = before$3.matcher(getCursor())).find()) {
return embed(
after.apply(getCursor(), elem.getCoordinates().replace(), matcher.parameter(0)),
getCursor(),
ctx,
SHORTEN_NAMES, SIMPLIFY_BOOLEANS
);
}
return super.visitMethodInvocation(elem, ctx);
}
};
return Preconditions.check(
Preconditions.and(
new UsesType<>("java.math.BigDecimal", true),
Preconditions.or(
new UsesMethod<>("java.math.BigDecimal compareTo(..)"),
new UsesMethod<>("java.math.BigDecimal compareTo(..)"),
new UsesMethod<>("java.math.BigDecimal signum(..)"),
new UsesMethod<>("java.math.BigDecimal signum(..)")
)
),
javaVisitor
);
}
}
/**
* OpenRewrite recipe created for Refaster template {@code BigDecimalRules.BigDecimalSignumIsNegative}.
*/
@SuppressWarnings("all")
@NonNullApi
@Generated("org.openrewrite.java.template.processor.RefasterTemplateProcessor")
public static class BigDecimalSignumIsNegativeRecipe extends Recipe {
/**
* Instantiates a new instance.
*/
public BigDecimalSignumIsNegativeRecipe() {}
@Override
public String getDisplayName() {
return "Prefer a `BigDecimal#signum()` comparison to -1 over more contrived or less clear alternatives";
}
@Override
public String getDescription() {
return "Recipe created for the following Refaster template:\n```java\nstatic final class BigDecimalSignumIsNegative {\n \n @BeforeTemplate\n boolean before(BigDecimal value) {\n return Refaster.anyOf(value.compareTo(BigDecimal.ZERO) < 0, BigDecimal.ZERO.compareTo(value) > 0, value.signum() < 0, value.signum() <= -1);\n }\n \n @AfterTemplate\n @AlsoNegation\n boolean after(BigDecimal value) {\n return value.signum() == -1;\n }\n}\n```\n.";
}
@Override
public TreeVisitor, ExecutionContext> getVisitor() {
JavaVisitor javaVisitor = new AbstractRefasterJavaVisitor() {
final JavaTemplate before$0 = JavaTemplate
.builder("#{value:any(java.math.BigDecimal)}.compareTo(java.math.BigDecimal.ZERO) < 0")
.build();
final JavaTemplate before$1 = JavaTemplate
.builder("java.math.BigDecimal.ZERO.compareTo(#{value:any(java.math.BigDecimal)}) > 0")
.build();
final JavaTemplate before$2 = JavaTemplate
.builder("#{value:any(java.math.BigDecimal)}.signum() < 0")
.build();
final JavaTemplate before$3 = JavaTemplate
.builder("#{value:any(java.math.BigDecimal)}.signum() <= -1")
.build();
final JavaTemplate after = JavaTemplate
.builder("#{value:any(java.math.BigDecimal)}.signum() == -1")
.build();
@Override
public J visitMethodInvocation(J.MethodInvocation elem, ExecutionContext ctx) {
JavaTemplate.Matcher matcher;
if ((matcher = before$0.matcher(getCursor())).find()) {
return embed(
after.apply(getCursor(), elem.getCoordinates().replace(), matcher.parameter(0)),
getCursor(),
ctx,
SHORTEN_NAMES, SIMPLIFY_BOOLEANS
);
}
if ((matcher = before$1.matcher(getCursor())).find()) {
return embed(
after.apply(getCursor(), elem.getCoordinates().replace(), matcher.parameter(0)),
getCursor(),
ctx,
SHORTEN_NAMES, SIMPLIFY_BOOLEANS
);
}
if ((matcher = before$2.matcher(getCursor())).find()) {
return embed(
after.apply(getCursor(), elem.getCoordinates().replace(), matcher.parameter(0)),
getCursor(),
ctx,
SHORTEN_NAMES, SIMPLIFY_BOOLEANS
);
}
if ((matcher = before$3.matcher(getCursor())).find()) {
return embed(
after.apply(getCursor(), elem.getCoordinates().replace(), matcher.parameter(0)),
getCursor(),
ctx,
SHORTEN_NAMES, SIMPLIFY_BOOLEANS
);
}
return super.visitMethodInvocation(elem, ctx);
}
};
return Preconditions.check(
Preconditions.and(
new UsesType<>("java.math.BigDecimal", true),
Preconditions.or(
new UsesMethod<>("java.math.BigDecimal compareTo(..)"),
new UsesMethod<>("java.math.BigDecimal compareTo(..)"),
new UsesMethod<>("java.math.BigDecimal signum(..)"),
new UsesMethod<>("java.math.BigDecimal signum(..)")
)
),
javaVisitor
);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy