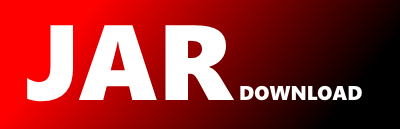
tech.picnic.errorprone.refasterrules.JUnitToAssertJRules Maven / Gradle / Ivy
Show all versions of error-prone-contrib Show documentation
package tech.picnic.errorprone.refasterrules;
import static com.google.errorprone.refaster.ImportPolicy.STATIC_IMPORT_ALWAYS;
import static org.assertj.core.api.Assertions.assertThat;
import static org.assertj.core.api.Assertions.assertThatCode;
import static org.assertj.core.api.Assertions.assertThatThrownBy;
import static org.assertj.core.api.Assertions.fail;
import static org.junit.jupiter.api.Assertions.assertDoesNotThrow;
import static org.junit.jupiter.api.Assertions.assertFalse;
import static org.junit.jupiter.api.Assertions.assertInstanceOf;
import static org.junit.jupiter.api.Assertions.assertNotNull;
import static org.junit.jupiter.api.Assertions.assertNotSame;
import static org.junit.jupiter.api.Assertions.assertNull;
import static org.junit.jupiter.api.Assertions.assertSame;
import static org.junit.jupiter.api.Assertions.assertThrows;
import static org.junit.jupiter.api.Assertions.assertThrowsExactly;
import static org.junit.jupiter.api.Assertions.assertTrue;
import com.google.errorprone.annotations.DoNotCall;
import com.google.errorprone.refaster.annotation.AfterTemplate;
import com.google.errorprone.refaster.annotation.BeforeTemplate;
import com.google.errorprone.refaster.annotation.UseImportPolicy;
import java.util.function.Supplier;
import org.assertj.core.api.ThrowableAssert.ThrowingCallable;
import org.junit.jupiter.api.Assertions;
import org.junit.jupiter.api.function.Executable;
import org.junit.jupiter.api.function.ThrowingSupplier;
import tech.picnic.errorprone.refaster.annotation.OnlineDocumentation;
import tech.picnic.errorprone.refaster.annotation.TypeMigration;
/**
* Refaster rules to replace JUnit assertions with AssertJ equivalents.
*
* Note that, while both libraries throw an {@link AssertionError} in case of an assertion
* failure, the exact subtype used generally differs.
*/
// XXX: Not all JUnit `Assertions` methods have an associated Refaster rule yet; expand this class.
// XXX: Introduce a `@Matcher` on `Executable` and `ThrowingSupplier` expressions, such that they
// are only matched if they are also compatible with the `ThrowingCallable` functional interface.
// When implementing such a matcher, note that expressions with a non-void return type such as
// `() -> toString()` match both `ThrowingSupplier` and `ThrowingCallable`, but `() -> "constant"`
// is only compatible with the former.
@OnlineDocumentation
@TypeMigration(
of = Assertions.class,
unmigratedMethods = {
"assertAll(Collection)",
"assertAll(Executable[])",
"assertAll(Stream)",
"assertAll(String, Collection)",
"assertAll(String, Executable[])",
"assertAll(String, Stream)",
"assertArrayEquals(boolean[], boolean[])",
"assertArrayEquals(boolean[], boolean[], String)",
"assertArrayEquals(boolean[], boolean[], Supplier)",
"assertArrayEquals(byte[], byte[])",
"assertArrayEquals(byte[], byte[], String)",
"assertArrayEquals(byte[], byte[], Supplier)",
"assertArrayEquals(char[], char[])",
"assertArrayEquals(char[], char[], String)",
"assertArrayEquals(char[], char[], Supplier)",
"assertArrayEquals(double[], double[])",
"assertArrayEquals(double[], double[], double)",
"assertArrayEquals(double[], double[], double, String)",
"assertArrayEquals(double[], double[], double, Supplier)",
"assertArrayEquals(double[], double[], String)",
"assertArrayEquals(double[], double[], Supplier)",
"assertArrayEquals(float[], float[])",
"assertArrayEquals(float[], float[], float)",
"assertArrayEquals(float[], float[], float, String)",
"assertArrayEquals(float[], float[], float, Supplier)",
"assertArrayEquals(float[], float[], String)",
"assertArrayEquals(float[], float[], Supplier)",
"assertArrayEquals(int[], int[])",
"assertArrayEquals(int[], int[], String)",
"assertArrayEquals(int[], int[], Supplier)",
"assertArrayEquals(long[], long[])",
"assertArrayEquals(long[], long[], String)",
"assertArrayEquals(long[], long[], Supplier)",
"assertArrayEquals(Object[], Object[])",
"assertArrayEquals(Object[], Object[], String)",
"assertArrayEquals(Object[], Object[], Supplier)",
"assertArrayEquals(short[], short[])",
"assertArrayEquals(short[], short[], String)",
"assertArrayEquals(short[], short[], Supplier)",
"assertEquals(Byte, Byte)",
"assertEquals(Byte, byte)",
"assertEquals(byte, Byte)",
"assertEquals(byte, byte)",
"assertEquals(Byte, Byte, String)",
"assertEquals(Byte, byte, String)",
"assertEquals(byte, Byte, String)",
"assertEquals(byte, byte, String)",
"assertEquals(Byte, Byte, Supplier)",
"assertEquals(Byte, byte, Supplier)",
"assertEquals(byte, Byte, Supplier)",
"assertEquals(byte, byte, Supplier)",
"assertEquals(char, char)",
"assertEquals(char, char, String)",
"assertEquals(char, char, Supplier)",
"assertEquals(char, Character)",
"assertEquals(char, Character, String)",
"assertEquals(char, Character, Supplier)",
"assertEquals(Character, char)",
"assertEquals(Character, char, String)",
"assertEquals(Character, char, Supplier)",
"assertEquals(Character, Character)",
"assertEquals(Character, Character, String)",
"assertEquals(Character, Character, Supplier)",
"assertEquals(Double, Double)",
"assertEquals(Double, double)",
"assertEquals(double, Double)",
"assertEquals(double, double)",
"assertEquals(double, double, double)",
"assertEquals(double, double, double, String)",
"assertEquals(double, double, double, Supplier)",
"assertEquals(Double, Double, String)",
"assertEquals(Double, double, String)",
"assertEquals(double, Double, String)",
"assertEquals(double, double, String)",
"assertEquals(Double, Double, Supplier)",
"assertEquals(Double, double, Supplier)",
"assertEquals(double, Double, Supplier)",
"assertEquals(double, double, Supplier)",
"assertEquals(Float, Float)",
"assertEquals(Float, float)",
"assertEquals(float, Float)",
"assertEquals(float, float)",
"assertEquals(float, float, float)",
"assertEquals(float, float, float, String)",
"assertEquals(float, float, float, Supplier)",
"assertEquals(Float, Float, String)",
"assertEquals(Float, float, String)",
"assertEquals(float, Float, String)",
"assertEquals(float, float, String)",
"assertEquals(Float, Float, Supplier)",
"assertEquals(Float, float, Supplier)",
"assertEquals(float, Float, Supplier)",
"assertEquals(float, float, Supplier)",
"assertEquals(int, int)",
"assertEquals(int, int, String)",
"assertEquals(int, int, Supplier)",
"assertEquals(int, Integer)",
"assertEquals(int, Integer, String)",
"assertEquals(int, Integer, Supplier)",
"assertEquals(Integer, int)",
"assertEquals(Integer, int, String)",
"assertEquals(Integer, int, Supplier)",
"assertEquals(Integer, Integer)",
"assertEquals(Integer, Integer, String)",
"assertEquals(Integer, Integer, Supplier)",
"assertEquals(Long, Long)",
"assertEquals(Long, long)",
"assertEquals(long, Long)",
"assertEquals(long, long)",
"assertEquals(Long, Long, String)",
"assertEquals(Long, long, String)",
"assertEquals(long, Long, String)",
"assertEquals(long, long, String)",
"assertEquals(Long, Long, Supplier)",
"assertEquals(Long, long, Supplier)",
"assertEquals(long, Long, Supplier)",
"assertEquals(long, long, Supplier)",
"assertEquals(Object, Object)",
"assertEquals(Object, Object, String)",
"assertEquals(Object, Object, Supplier)",
"assertEquals(Short, Short)",
"assertEquals(Short, short)",
"assertEquals(short, Short)",
"assertEquals(short, short)",
"assertEquals(Short, Short, String)",
"assertEquals(Short, short, String)",
"assertEquals(short, Short, String)",
"assertEquals(short, short, String)",
"assertEquals(Short, Short, Supplier)",
"assertEquals(Short, short, Supplier)",
"assertEquals(short, Short, Supplier)",
"assertEquals(short, short, Supplier)",
"assertFalse(BooleanSupplier)",
"assertFalse(BooleanSupplier, String)",
"assertFalse(BooleanSupplier, Supplier)",
"assertIterableEquals(Iterable>, Iterable>)",
"assertIterableEquals(Iterable>, Iterable>, String)",
"assertIterableEquals(Iterable>, Iterable>, Supplier)",
"assertLinesMatch(List, List)",
"assertLinesMatch(List, List, String)",
"assertLinesMatch(List, List, Supplier)",
"assertLinesMatch(Stream, Stream)",
"assertLinesMatch(Stream, Stream, String)",
"assertLinesMatch(Stream, Stream, Supplier)",
"assertNotEquals(Byte, Byte)",
"assertNotEquals(Byte, byte)",
"assertNotEquals(byte, Byte)",
"assertNotEquals(byte, byte)",
"assertNotEquals(Byte, Byte, String)",
"assertNotEquals(Byte, byte, String)",
"assertNotEquals(byte, Byte, String)",
"assertNotEquals(byte, byte, String)",
"assertNotEquals(Byte, Byte, Supplier)",
"assertNotEquals(Byte, byte, Supplier)",
"assertNotEquals(byte, Byte, Supplier)",
"assertNotEquals(byte, byte, Supplier)",
"assertNotEquals(char, char)",
"assertNotEquals(char, char, String)",
"assertNotEquals(char, char, Supplier)",
"assertNotEquals(char, Character)",
"assertNotEquals(char, Character, String)",
"assertNotEquals(char, Character, Supplier)",
"assertNotEquals(Character, char)",
"assertNotEquals(Character, char, String)",
"assertNotEquals(Character, char, Supplier)",
"assertNotEquals(Character, Character)",
"assertNotEquals(Character, Character, String)",
"assertNotEquals(Character, Character, Supplier)",
"assertNotEquals(Double, Double)",
"assertNotEquals(Double, double)",
"assertNotEquals(double, Double)",
"assertNotEquals(double, double)",
"assertNotEquals(double, double, double)",
"assertNotEquals(double, double, double, String)",
"assertNotEquals(double, double, double, Supplier)",
"assertNotEquals(Double, Double, String)",
"assertNotEquals(Double, double, String)",
"assertNotEquals(double, Double, String)",
"assertNotEquals(double, double, String)",
"assertNotEquals(Double, Double, Supplier)",
"assertNotEquals(Double, double, Supplier)",
"assertNotEquals(double, Double, Supplier)",
"assertNotEquals(double, double, Supplier)",
"assertNotEquals(Float, Float)",
"assertNotEquals(Float, float)",
"assertNotEquals(float, Float)",
"assertNotEquals(float, float)",
"assertNotEquals(float, float, float)",
"assertNotEquals(float, float, float, String)",
"assertNotEquals(float, float, float, Supplier)",
"assertNotEquals(Float, Float, String)",
"assertNotEquals(Float, float, String)",
"assertNotEquals(float, Float, String)",
"assertNotEquals(float, float, String)",
"assertNotEquals(Float, Float, Supplier)",
"assertNotEquals(Float, float, Supplier)",
"assertNotEquals(float, Float, Supplier)",
"assertNotEquals(float, float, Supplier)",
"assertNotEquals(int, int)",
"assertNotEquals(int, int, String)",
"assertNotEquals(int, int, Supplier)",
"assertNotEquals(int, Integer)",
"assertNotEquals(int, Integer, String)",
"assertNotEquals(int, Integer, Supplier)",
"assertNotEquals(Integer, int)",
"assertNotEquals(Integer, int, String)",
"assertNotEquals(Integer, int, Supplier)",
"assertNotEquals(Integer, Integer)",
"assertNotEquals(Integer, Integer, String)",
"assertNotEquals(Integer, Integer, Supplier)",
"assertNotEquals(Long, Long)",
"assertNotEquals(Long, long)",
"assertNotEquals(long, Long)",
"assertNotEquals(long, long)",
"assertNotEquals(Long, Long, String)",
"assertNotEquals(Long, long, String)",
"assertNotEquals(long, Long, String)",
"assertNotEquals(long, long, String)",
"assertNotEquals(Long, Long, Supplier)",
"assertNotEquals(Long, long, Supplier)",
"assertNotEquals(long, Long, Supplier)",
"assertNotEquals(long, long, Supplier)",
"assertNotEquals(Object, Object)",
"assertNotEquals(Object, Object, String)",
"assertNotEquals(Object, Object, Supplier)",
"assertNotEquals(Short, Short)",
"assertNotEquals(Short, short)",
"assertNotEquals(short, Short)",
"assertNotEquals(short, short)",
"assertNotEquals(Short, Short, String)",
"assertNotEquals(Short, short, String)",
"assertNotEquals(short, Short, String)",
"assertNotEquals(short, short, String)",
"assertNotEquals(Short, Short, Supplier)",
"assertNotEquals(Short, short, Supplier)",
"assertNotEquals(short, Short, Supplier)",
"assertNotEquals(short, short, Supplier)",
"assertTimeout(Duration, Executable)",
"assertTimeout(Duration, Executable, String)",
"assertTimeout(Duration, Executable, Supplier)",
"assertTimeout(Duration, ThrowingSupplier)",
"assertTimeout(Duration, ThrowingSupplier, String)",
"assertTimeout(Duration, ThrowingSupplier, Supplier)",
"assertTimeoutPreemptively(Duration, Executable)",
"assertTimeoutPreemptively(Duration, Executable, String)",
"assertTimeoutPreemptively(Duration, Executable, Supplier)",
"assertTimeoutPreemptively(Duration, ThrowingSupplier)",
"assertTimeoutPreemptively(Duration, ThrowingSupplier, String)",
"assertTimeoutPreemptively(Duration, ThrowingSupplier, Supplier)",
"assertTimeoutPreemptively(Duration, ThrowingSupplier, Supplier, TimeoutFailureFactory)",
"assertTrue(BooleanSupplier)",
"assertTrue(BooleanSupplier, String)",
"assertTrue(BooleanSupplier, Supplier)",
"fail(Supplier)"
})
final class JUnitToAssertJRules {
private JUnitToAssertJRules() {}
static final class Fail {
@BeforeTemplate
T before() {
return Assertions.fail();
}
// XXX: Add `@UseImportPolicy(STATIC_IMPORT_ALWAYS)` once
// https://github.com/google/error-prone/pull/3584 is resolved. Until that time, statically
// importing AssertJ's `fail` is likely to clash with an existing static import of JUnit's
// `fail`. Note that combining Error Prone's `RemoveUnusedImports` and
// `UnnecessarilyFullyQualified` checks and our `StaticImport` check will anyway cause the
// method to be imported statically if possible; just in a less efficient manner.
@AfterTemplate
@DoNotCall
T after() {
return fail();
}
}
static final class FailWithMessage {
@BeforeTemplate
T before(String message) {
return Assertions.fail(message);
}
// XXX: Add `@UseImportPolicy(STATIC_IMPORT_ALWAYS)`. See `Fail` comment.
@AfterTemplate
T after(String message) {
return fail(message);
}
}
static final class FailWithMessageAndThrowable {
@BeforeTemplate
T before(String message, Throwable throwable) {
return Assertions.fail(message, throwable);
}
// XXX: Add `@UseImportPolicy(STATIC_IMPORT_ALWAYS)`. See `Fail` comment.
@AfterTemplate
T after(String message, Throwable throwable) {
return fail(message, throwable);
}
}
static final class FailWithThrowable {
@BeforeTemplate
T before(Throwable throwable) {
return Assertions.fail(throwable);
}
// XXX: Add `@UseImportPolicy(STATIC_IMPORT_ALWAYS)`. See `Fail` comment.
@AfterTemplate
@DoNotCall
T after(Throwable throwable) {
return fail(throwable);
}
}
static final class AssertThatIsTrue {
@BeforeTemplate
void before(boolean actual) {
assertTrue(actual);
}
@AfterTemplate
@UseImportPolicy(STATIC_IMPORT_ALWAYS)
void after(boolean actual) {
assertThat(actual).isTrue();
}
}
static final class AssertThatWithFailMessageStringIsTrue {
@BeforeTemplate
void before(boolean actual, String message) {
assertTrue(actual, message);
}
@AfterTemplate
@UseImportPolicy(STATIC_IMPORT_ALWAYS)
void after(boolean actual, String message) {
assertThat(actual).withFailMessage(message).isTrue();
}
}
static final class AssertThatWithFailMessageSupplierIsTrue {
@BeforeTemplate
void before(boolean actual, Supplier supplier) {
assertTrue(actual, supplier);
}
@AfterTemplate
@UseImportPolicy(STATIC_IMPORT_ALWAYS)
void after(boolean actual, Supplier supplier) {
assertThat(actual).withFailMessage(supplier).isTrue();
}
}
static final class AssertThatIsFalse {
@BeforeTemplate
void before(boolean actual) {
assertFalse(actual);
}
@AfterTemplate
@UseImportPolicy(STATIC_IMPORT_ALWAYS)
void after(boolean actual) {
assertThat(actual).isFalse();
}
}
static final class AssertThatWithFailMessageStringIsFalse {
@BeforeTemplate
void before(boolean actual, String message) {
assertFalse(actual, message);
}
@AfterTemplate
@UseImportPolicy(STATIC_IMPORT_ALWAYS)
void after(boolean actual, String message) {
assertThat(actual).withFailMessage(message).isFalse();
}
}
static final class AssertThatWithFailMessageSupplierIsFalse {
@BeforeTemplate
void before(boolean actual, Supplier supplier) {
assertFalse(actual, supplier);
}
@AfterTemplate
@UseImportPolicy(STATIC_IMPORT_ALWAYS)
void after(boolean actual, Supplier supplier) {
assertThat(actual).withFailMessage(supplier).isFalse();
}
}
static final class AssertThatIsNull {
@BeforeTemplate
void before(Object actual) {
assertNull(actual);
}
@AfterTemplate
@UseImportPolicy(STATIC_IMPORT_ALWAYS)
void after(Object actual) {
assertThat(actual).isNull();
}
}
static final class AssertThatWithFailMessageStringIsNull {
@BeforeTemplate
void before(Object actual, String message) {
assertNull(actual, message);
}
@AfterTemplate
@UseImportPolicy(STATIC_IMPORT_ALWAYS)
void after(Object actual, String message) {
assertThat(actual).withFailMessage(message).isNull();
}
}
static final class AssertThatWithFailMessageSupplierIsNull {
@BeforeTemplate
void before(Object actual, Supplier supplier) {
assertNull(actual, supplier);
}
@AfterTemplate
@UseImportPolicy(STATIC_IMPORT_ALWAYS)
void after(Object actual, Supplier supplier) {
assertThat(actual).withFailMessage(supplier).isNull();
}
}
static final class AssertThatIsNotNull {
@BeforeTemplate
void before(Object actual) {
assertNotNull(actual);
}
@AfterTemplate
@UseImportPolicy(STATIC_IMPORT_ALWAYS)
void after(Object actual) {
assertThat(actual).isNotNull();
}
}
static final class AssertThatWithFailMessageStringIsNotNull {
@BeforeTemplate
void before(Object actual, String message) {
assertNotNull(actual, message);
}
@AfterTemplate
@UseImportPolicy(STATIC_IMPORT_ALWAYS)
void after(Object actual, String message) {
assertThat(actual).withFailMessage(message).isNotNull();
}
}
static final class AssertThatWithFailMessageSupplierIsNotNull {
@BeforeTemplate
void before(Object actual, Supplier supplier) {
assertNotNull(actual, supplier);
}
@AfterTemplate
@UseImportPolicy(STATIC_IMPORT_ALWAYS)
void after(Object actual, Supplier supplier) {
assertThat(actual).withFailMessage(supplier).isNotNull();
}
}
static final class AssertThatIsSameAs {
@BeforeTemplate
void before(Object actual, Object expected) {
assertSame(expected, actual);
}
@AfterTemplate
@UseImportPolicy(STATIC_IMPORT_ALWAYS)
void after(Object actual, Object expected) {
assertThat(actual).isSameAs(expected);
}
}
static final class AssertThatWithFailMessageStringIsSameAs {
@BeforeTemplate
void before(Object actual, String message, Object expected) {
assertSame(expected, actual, message);
}
@AfterTemplate
@UseImportPolicy(STATIC_IMPORT_ALWAYS)
void after(Object actual, String message, Object expected) {
assertThat(actual).withFailMessage(message).isSameAs(expected);
}
}
static final class AssertThatWithFailMessageSupplierIsSameAs {
@BeforeTemplate
void before(Object actual, Supplier supplier, Object expected) {
assertSame(expected, actual, supplier);
}
@AfterTemplate
@UseImportPolicy(STATIC_IMPORT_ALWAYS)
void after(Object actual, Supplier supplier, Object expected) {
assertThat(actual).withFailMessage(supplier).isSameAs(expected);
}
}
static final class AssertThatIsNotSameAs {
@BeforeTemplate
void before(Object actual, Object expected) {
assertNotSame(expected, actual);
}
@AfterTemplate
@UseImportPolicy(STATIC_IMPORT_ALWAYS)
void after(Object actual, Object expected) {
assertThat(actual).isNotSameAs(expected);
}
}
static final class AssertThatWithFailMessageStringIsNotSameAs {
@BeforeTemplate
void before(Object actual, String message, Object expected) {
assertNotSame(expected, actual, message);
}
@AfterTemplate
@UseImportPolicy(STATIC_IMPORT_ALWAYS)
void after(Object actual, String message, Object expected) {
assertThat(actual).withFailMessage(message).isNotSameAs(expected);
}
}
static final class AssertThatWithFailMessageSupplierIsNotSameAs {
@BeforeTemplate
void before(Object actual, Supplier supplier, Object expected) {
assertNotSame(expected, actual, supplier);
}
@AfterTemplate
@UseImportPolicy(STATIC_IMPORT_ALWAYS)
void after(Object actual, Supplier supplier, Object expected) {
assertThat(actual).withFailMessage(supplier).isNotSameAs(expected);
}
}
static final class AssertThatThrownByIsExactlyInstanceOf {
@BeforeTemplate
void before(Executable throwingCallable, Class clazz) {
assertThrowsExactly(clazz, throwingCallable);
}
@AfterTemplate
@UseImportPolicy(STATIC_IMPORT_ALWAYS)
void after(ThrowingCallable throwingCallable, Class clazz) {
assertThatThrownBy(throwingCallable).isExactlyInstanceOf(clazz);
}
}
static final class AssertThatThrownByWithFailMessageStringIsExactlyInstanceOf<
T extends Throwable> {
@BeforeTemplate
void before(Executable throwingCallable, String message, Class clazz) {
assertThrowsExactly(clazz, throwingCallable, message);
}
@AfterTemplate
@UseImportPolicy(STATIC_IMPORT_ALWAYS)
void after(ThrowingCallable throwingCallable, String message, Class clazz) {
assertThatThrownBy(throwingCallable).withFailMessage(message).isExactlyInstanceOf(clazz);
}
}
static final class AssertThatThrownByWithFailMessageSupplierIsExactlyInstanceOf<
T extends Throwable> {
@BeforeTemplate
void before(Executable throwingCallable, Supplier supplier, Class clazz) {
assertThrowsExactly(clazz, throwingCallable, supplier);
}
@AfterTemplate
@UseImportPolicy(STATIC_IMPORT_ALWAYS)
void after(ThrowingCallable throwingCallable, Supplier supplier, Class clazz) {
assertThatThrownBy(throwingCallable).withFailMessage(supplier).isExactlyInstanceOf(clazz);
}
}
static final class AssertThatThrownByIsInstanceOf {
@BeforeTemplate
void before(Executable throwingCallable, Class clazz) {
assertThrows(clazz, throwingCallable);
}
@AfterTemplate
@UseImportPolicy(STATIC_IMPORT_ALWAYS)
void after(ThrowingCallable throwingCallable, Class clazz) {
assertThatThrownBy(throwingCallable).isInstanceOf(clazz);
}
}
static final class AssertThatThrownByWithFailMessageStringIsInstanceOf {
@BeforeTemplate
void before(Executable throwingCallable, String message, Class clazz) {
assertThrows(clazz, throwingCallable, message);
}
@AfterTemplate
@UseImportPolicy(STATIC_IMPORT_ALWAYS)
void after(ThrowingCallable throwingCallable, String message, Class clazz) {
assertThatThrownBy(throwingCallable).withFailMessage(message).isInstanceOf(clazz);
}
}
static final class AssertThatThrownByWithFailMessageSupplierIsInstanceOf {
@BeforeTemplate
void before(Executable throwingCallable, Supplier supplier, Class clazz) {
assertThrows(clazz, throwingCallable, supplier);
}
@AfterTemplate
@UseImportPolicy(STATIC_IMPORT_ALWAYS)
void after(ThrowingCallable throwingCallable, Supplier supplier, Class clazz) {
assertThatThrownBy(throwingCallable).withFailMessage(supplier).isInstanceOf(clazz);
}
}
static final class AssertThatCodeDoesNotThrowAnyException {
@BeforeTemplate
void before(Executable throwingCallable) {
assertDoesNotThrow(throwingCallable);
}
@BeforeTemplate
void before(ThrowingSupplier> throwingCallable) {
assertDoesNotThrow(throwingCallable);
}
@AfterTemplate
@UseImportPolicy(STATIC_IMPORT_ALWAYS)
void after(ThrowingCallable throwingCallable) {
assertThatCode(throwingCallable).doesNotThrowAnyException();
}
}
static final class AssertThatCodeWithFailMessageStringDoesNotThrowAnyException {
@BeforeTemplate
void before(Executable throwingCallable, String message) {
assertDoesNotThrow(throwingCallable, message);
}
@BeforeTemplate
void before(ThrowingSupplier> throwingCallable, String message) {
assertDoesNotThrow(throwingCallable, message);
}
@AfterTemplate
@UseImportPolicy(STATIC_IMPORT_ALWAYS)
void after(ThrowingCallable throwingCallable, String message) {
assertThatCode(throwingCallable).withFailMessage(message).doesNotThrowAnyException();
}
}
static final class AssertThatCodeWithFailMessageSupplierDoesNotThrowAnyException {
@BeforeTemplate
void before(Executable throwingCallable, Supplier supplier) {
assertDoesNotThrow(throwingCallable, supplier);
}
@BeforeTemplate
void before(ThrowingSupplier> throwingCallable, Supplier supplier) {
assertDoesNotThrow(throwingCallable, supplier);
}
@AfterTemplate
@UseImportPolicy(STATIC_IMPORT_ALWAYS)
void after(ThrowingCallable throwingCallable, Supplier supplier) {
assertThatCode(throwingCallable).withFailMessage(supplier).doesNotThrowAnyException();
}
}
static final class AssertThatIsInstanceOf {
@BeforeTemplate
void before(Object actual, Class clazz) {
assertInstanceOf(clazz, actual);
}
@AfterTemplate
@UseImportPolicy(STATIC_IMPORT_ALWAYS)
void after(Object actual, Class clazz) {
assertThat(actual).isInstanceOf(clazz);
}
}
static final class AssertThatWithFailMessageStringIsInstanceOf {
@BeforeTemplate
void before(Object actual, String message, Class clazz) {
assertInstanceOf(clazz, actual, message);
}
@AfterTemplate
@UseImportPolicy(STATIC_IMPORT_ALWAYS)
void after(Object actual, String message, Class clazz) {
assertThat(actual).withFailMessage(message).isInstanceOf(clazz);
}
}
static final class AssertThatWithFailMessageSupplierIsInstanceOf {
@BeforeTemplate
void before(Object actual, Supplier supplier, Class clazz) {
assertInstanceOf(clazz, actual, supplier);
}
@AfterTemplate
@UseImportPolicy(STATIC_IMPORT_ALWAYS)
void after(Object actual, Supplier supplier, Class clazz) {
assertThat(actual).withFailMessage(supplier).isInstanceOf(clazz);
}
}
}