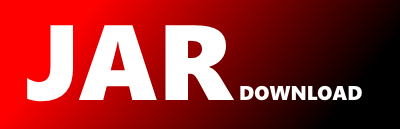
tech.picnic.errorprone.refasterrules.JUnitToAssertJRulesRecipes Maven / Gradle / Ivy
Show all versions of error-prone-contrib Show documentation
package tech.picnic.errorprone.refasterrules;
import org.openrewrite.ExecutionContext;
import org.openrewrite.Preconditions;
import org.openrewrite.Recipe;
import org.openrewrite.TreeVisitor;
import org.openrewrite.internal.lang.NonNullApi;
import org.openrewrite.java.JavaParser;
import org.openrewrite.java.JavaTemplate;
import org.openrewrite.java.JavaVisitor;
import org.openrewrite.java.search.*;
import org.openrewrite.java.template.Primitive;
import org.openrewrite.java.template.function.*;
import org.openrewrite.java.template.internal.AbstractRefasterJavaVisitor;
import org.openrewrite.java.tree.*;
import javax.annotation.Generated;
import java.util.*;
import static org.openrewrite.java.template.internal.AbstractRefasterJavaVisitor.EmbeddingOption.*;
/**
* OpenRewrite recipes created for Refaster template {@code tech.picnic.errorprone.refasterrules.JUnitToAssertJRules}.
*/
@SuppressWarnings("all")
@Generated("org.openrewrite.java.template.processor.RefasterTemplateProcessor")
public class JUnitToAssertJRulesRecipes extends Recipe {
/**
* Instantiates a new instance.
*/
public JUnitToAssertJRulesRecipes() {}
@Override
public String getDisplayName() {
return "Refaster rules to replace JUnit assertions with AssertJ equivalents";
}
@Override
public String getDescription() {
return "Note that, while both libraries throw an `AssertionError` in case of an assertion\n failure, the exact subtype used generally differs. [Source](https://error-prone.picnic.tech/refasterrules/JUnitToAssertJRules).";
}
@Override
public List getRecipeList() {
return Arrays.asList(
new AssertThatIsTrueRecipe(),
new AssertThatWithFailMessageStringIsTrueRecipe(),
new AssertThatWithFailMessageSupplierIsTrueRecipe(),
new AssertThatIsFalseRecipe(),
new AssertThatWithFailMessageStringIsFalseRecipe(),
new AssertThatWithFailMessageSupplierIsFalseRecipe(),
new AssertThatIsNullRecipe(),
new AssertThatWithFailMessageStringIsNullRecipe(),
new AssertThatWithFailMessageSupplierIsNullRecipe(),
new AssertThatIsNotNullRecipe(),
new AssertThatWithFailMessageStringIsNotNullRecipe(),
new AssertThatWithFailMessageSupplierIsNotNullRecipe(),
new AssertThatIsSameAsRecipe(),
new AssertThatWithFailMessageStringIsSameAsRecipe(),
new AssertThatWithFailMessageSupplierIsSameAsRecipe(),
new AssertThatIsNotSameAsRecipe(),
new AssertThatWithFailMessageStringIsNotSameAsRecipe(),
new AssertThatWithFailMessageSupplierIsNotSameAsRecipe(),
new AssertThatCodeDoesNotThrowAnyExceptionRecipe(),
new AssertThatCodeWithFailMessageStringDoesNotThrowAnyExceptionRecipe(),
new AssertThatCodeWithFailMessageSupplierDoesNotThrowAnyExceptionRecipe()
);
}
/**
* OpenRewrite recipe created for Refaster template {@code JUnitToAssertJRules.AssertThatIsTrue}.
*/
@SuppressWarnings("all")
@NonNullApi
@Generated("org.openrewrite.java.template.processor.RefasterTemplateProcessor")
public static class AssertThatIsTrueRecipe extends Recipe {
/**
* Instantiates a new instance.
*/
public AssertThatIsTrueRecipe() {}
@Override
public String getDisplayName() {
return "Refaster template `JUnitToAssertJRules.AssertThatIsTrue`";
}
@Override
public String getDescription() {
return "Recipe created for the following Refaster template:\n```java\nstatic final class AssertThatIsTrue {\n \n @BeforeTemplate\n void before(boolean actual) {\n assertTrue(actual);\n }\n \n @AfterTemplate\n @UseImportPolicy(value = STATIC_IMPORT_ALWAYS)\n void after(boolean actual) {\n assertThat(actual).isTrue();\n }\n}\n```\n.";
}
@Override
public TreeVisitor, ExecutionContext> getVisitor() {
JavaVisitor javaVisitor = new AbstractRefasterJavaVisitor() {
final JavaTemplate before = JavaTemplate
.builder("org.junit.jupiter.api.Assertions.assertTrue(#{actual:any(boolean)});")
.javaParser(JavaParser.fromJavaVersion().classpath(JavaParser.runtimeClasspath()))
.build();
final JavaTemplate after = JavaTemplate
.builder("org.assertj.core.api.Assertions.assertThat(#{actual:any(boolean)}).isTrue();")
.javaParser(JavaParser.fromJavaVersion().classpath(JavaParser.runtimeClasspath()))
.build();
@Override
public J visitMethodInvocation(J.MethodInvocation elem, ExecutionContext ctx) {
JavaTemplate.Matcher matcher;
if ((matcher = before.matcher(getCursor())).find()) {
maybeRemoveImport("org.junit.jupiter.api.Assertions.assertTrue");
return embed(
after.apply(getCursor(), elem.getCoordinates().replace(), matcher.parameter(0)),
getCursor(),
ctx,
SHORTEN_NAMES
);
}
return super.visitMethodInvocation(elem, ctx);
}
};
return Preconditions.check(
new UsesMethod<>("org.junit.jupiter.api.Assertions assertTrue(..)"),
javaVisitor
);
}
}
/**
* OpenRewrite recipe created for Refaster template {@code JUnitToAssertJRules.AssertThatWithFailMessageStringIsTrue}.
*/
@SuppressWarnings("all")
@NonNullApi
@Generated("org.openrewrite.java.template.processor.RefasterTemplateProcessor")
public static class AssertThatWithFailMessageStringIsTrueRecipe extends Recipe {
/**
* Instantiates a new instance.
*/
public AssertThatWithFailMessageStringIsTrueRecipe() {}
@Override
public String getDisplayName() {
return "Refaster template `JUnitToAssertJRules.AssertThatWithFailMessageStringIsTrue`";
}
@Override
public String getDescription() {
return "Recipe created for the following Refaster template:\n```java\nstatic final class AssertThatWithFailMessageStringIsTrue {\n \n @BeforeTemplate\n void before(boolean actual, String message) {\n assertTrue(actual, message);\n }\n \n @AfterTemplate\n @UseImportPolicy(value = STATIC_IMPORT_ALWAYS)\n void after(boolean actual, String message) {\n assertThat(actual).withFailMessage(message).isTrue();\n }\n}\n```\n.";
}
@Override
public TreeVisitor, ExecutionContext> getVisitor() {
JavaVisitor javaVisitor = new AbstractRefasterJavaVisitor() {
final JavaTemplate before = JavaTemplate
.builder("org.junit.jupiter.api.Assertions.assertTrue(#{actual:any(boolean)}, #{message:any(java.lang.String)});")
.javaParser(JavaParser.fromJavaVersion().classpath(JavaParser.runtimeClasspath()))
.build();
final JavaTemplate after = JavaTemplate
.builder("org.assertj.core.api.Assertions.assertThat(#{actual:any(boolean)}).withFailMessage(#{message:any(java.lang.String)}).isTrue();")
.javaParser(JavaParser.fromJavaVersion().classpath(JavaParser.runtimeClasspath()))
.build();
@Override
public J visitMethodInvocation(J.MethodInvocation elem, ExecutionContext ctx) {
JavaTemplate.Matcher matcher;
if ((matcher = before.matcher(getCursor())).find()) {
maybeRemoveImport("org.junit.jupiter.api.Assertions.assertTrue");
return embed(
after.apply(getCursor(), elem.getCoordinates().replace(), matcher.parameter(0), matcher.parameter(1)),
getCursor(),
ctx,
SHORTEN_NAMES
);
}
return super.visitMethodInvocation(elem, ctx);
}
};
return Preconditions.check(
new UsesMethod<>("org.junit.jupiter.api.Assertions assertTrue(..)"),
javaVisitor
);
}
}
/**
* OpenRewrite recipe created for Refaster template {@code JUnitToAssertJRules.AssertThatWithFailMessageSupplierIsTrue}.
*/
@SuppressWarnings("all")
@NonNullApi
@Generated("org.openrewrite.java.template.processor.RefasterTemplateProcessor")
public static class AssertThatWithFailMessageSupplierIsTrueRecipe extends Recipe {
/**
* Instantiates a new instance.
*/
public AssertThatWithFailMessageSupplierIsTrueRecipe() {}
@Override
public String getDisplayName() {
return "Refaster template `JUnitToAssertJRules.AssertThatWithFailMessageSupplierIsTrue`";
}
@Override
public String getDescription() {
return "Recipe created for the following Refaster template:\n```java\nstatic final class AssertThatWithFailMessageSupplierIsTrue {\n \n @BeforeTemplate\n void before(boolean actual, Supplier supplier) {\n assertTrue(actual, supplier);\n }\n \n @AfterTemplate\n @UseImportPolicy(value = STATIC_IMPORT_ALWAYS)\n void after(boolean actual, Supplier supplier) {\n assertThat(actual).withFailMessage(supplier).isTrue();\n }\n}\n```\n.";
}
@Override
public TreeVisitor, ExecutionContext> getVisitor() {
JavaVisitor javaVisitor = new AbstractRefasterJavaVisitor() {
final JavaTemplate before = JavaTemplate
.builder("org.junit.jupiter.api.Assertions.assertTrue(#{actual:any(boolean)}, #{supplier:any(java.util.function.Supplier)});")
.javaParser(JavaParser.fromJavaVersion().classpath(JavaParser.runtimeClasspath()))
.build();
final JavaTemplate after = JavaTemplate
.builder("org.assertj.core.api.Assertions.assertThat(#{actual:any(boolean)}).withFailMessage(#{supplier:any(java.util.function.Supplier)}).isTrue();")
.javaParser(JavaParser.fromJavaVersion().classpath(JavaParser.runtimeClasspath()))
.build();
@Override
public J visitMethodInvocation(J.MethodInvocation elem, ExecutionContext ctx) {
JavaTemplate.Matcher matcher;
if ((matcher = before.matcher(getCursor())).find()) {
maybeRemoveImport("org.junit.jupiter.api.Assertions.assertTrue");
return embed(
after.apply(getCursor(), elem.getCoordinates().replace(), matcher.parameter(0), matcher.parameter(1)),
getCursor(),
ctx,
SHORTEN_NAMES
);
}
return super.visitMethodInvocation(elem, ctx);
}
};
return Preconditions.check(
Preconditions.and(
new UsesType<>("java.util.function.Supplier", true),
new UsesMethod<>("org.junit.jupiter.api.Assertions assertTrue(..)")
),
javaVisitor
);
}
}
/**
* OpenRewrite recipe created for Refaster template {@code JUnitToAssertJRules.AssertThatIsFalse}.
*/
@SuppressWarnings("all")
@NonNullApi
@Generated("org.openrewrite.java.template.processor.RefasterTemplateProcessor")
public static class AssertThatIsFalseRecipe extends Recipe {
/**
* Instantiates a new instance.
*/
public AssertThatIsFalseRecipe() {}
@Override
public String getDisplayName() {
return "Refaster template `JUnitToAssertJRules.AssertThatIsFalse`";
}
@Override
public String getDescription() {
return "Recipe created for the following Refaster template:\n```java\nstatic final class AssertThatIsFalse {\n \n @BeforeTemplate\n void before(boolean actual) {\n assertFalse(actual);\n }\n \n @AfterTemplate\n @UseImportPolicy(value = STATIC_IMPORT_ALWAYS)\n void after(boolean actual) {\n assertThat(actual).isFalse();\n }\n}\n```\n.";
}
@Override
public TreeVisitor, ExecutionContext> getVisitor() {
JavaVisitor javaVisitor = new AbstractRefasterJavaVisitor() {
final JavaTemplate before = JavaTemplate
.builder("org.junit.jupiter.api.Assertions.assertFalse(#{actual:any(boolean)});")
.javaParser(JavaParser.fromJavaVersion().classpath(JavaParser.runtimeClasspath()))
.build();
final JavaTemplate after = JavaTemplate
.builder("org.assertj.core.api.Assertions.assertThat(#{actual:any(boolean)}).isFalse();")
.javaParser(JavaParser.fromJavaVersion().classpath(JavaParser.runtimeClasspath()))
.build();
@Override
public J visitMethodInvocation(J.MethodInvocation elem, ExecutionContext ctx) {
JavaTemplate.Matcher matcher;
if ((matcher = before.matcher(getCursor())).find()) {
maybeRemoveImport("org.junit.jupiter.api.Assertions.assertFalse");
return embed(
after.apply(getCursor(), elem.getCoordinates().replace(), matcher.parameter(0)),
getCursor(),
ctx,
SHORTEN_NAMES
);
}
return super.visitMethodInvocation(elem, ctx);
}
};
return Preconditions.check(
new UsesMethod<>("org.junit.jupiter.api.Assertions assertFalse(..)"),
javaVisitor
);
}
}
/**
* OpenRewrite recipe created for Refaster template {@code JUnitToAssertJRules.AssertThatWithFailMessageStringIsFalse}.
*/
@SuppressWarnings("all")
@NonNullApi
@Generated("org.openrewrite.java.template.processor.RefasterTemplateProcessor")
public static class AssertThatWithFailMessageStringIsFalseRecipe extends Recipe {
/**
* Instantiates a new instance.
*/
public AssertThatWithFailMessageStringIsFalseRecipe() {}
@Override
public String getDisplayName() {
return "Refaster template `JUnitToAssertJRules.AssertThatWithFailMessageStringIsFalse`";
}
@Override
public String getDescription() {
return "Recipe created for the following Refaster template:\n```java\nstatic final class AssertThatWithFailMessageStringIsFalse {\n \n @BeforeTemplate\n void before(boolean actual, String message) {\n assertFalse(actual, message);\n }\n \n @AfterTemplate\n @UseImportPolicy(value = STATIC_IMPORT_ALWAYS)\n void after(boolean actual, String message) {\n assertThat(actual).withFailMessage(message).isFalse();\n }\n}\n```\n.";
}
@Override
public TreeVisitor, ExecutionContext> getVisitor() {
JavaVisitor javaVisitor = new AbstractRefasterJavaVisitor() {
final JavaTemplate before = JavaTemplate
.builder("org.junit.jupiter.api.Assertions.assertFalse(#{actual:any(boolean)}, #{message:any(java.lang.String)});")
.javaParser(JavaParser.fromJavaVersion().classpath(JavaParser.runtimeClasspath()))
.build();
final JavaTemplate after = JavaTemplate
.builder("org.assertj.core.api.Assertions.assertThat(#{actual:any(boolean)}).withFailMessage(#{message:any(java.lang.String)}).isFalse();")
.javaParser(JavaParser.fromJavaVersion().classpath(JavaParser.runtimeClasspath()))
.build();
@Override
public J visitMethodInvocation(J.MethodInvocation elem, ExecutionContext ctx) {
JavaTemplate.Matcher matcher;
if ((matcher = before.matcher(getCursor())).find()) {
maybeRemoveImport("org.junit.jupiter.api.Assertions.assertFalse");
return embed(
after.apply(getCursor(), elem.getCoordinates().replace(), matcher.parameter(0), matcher.parameter(1)),
getCursor(),
ctx,
SHORTEN_NAMES
);
}
return super.visitMethodInvocation(elem, ctx);
}
};
return Preconditions.check(
new UsesMethod<>("org.junit.jupiter.api.Assertions assertFalse(..)"),
javaVisitor
);
}
}
/**
* OpenRewrite recipe created for Refaster template {@code JUnitToAssertJRules.AssertThatWithFailMessageSupplierIsFalse}.
*/
@SuppressWarnings("all")
@NonNullApi
@Generated("org.openrewrite.java.template.processor.RefasterTemplateProcessor")
public static class AssertThatWithFailMessageSupplierIsFalseRecipe extends Recipe {
/**
* Instantiates a new instance.
*/
public AssertThatWithFailMessageSupplierIsFalseRecipe() {}
@Override
public String getDisplayName() {
return "Refaster template `JUnitToAssertJRules.AssertThatWithFailMessageSupplierIsFalse`";
}
@Override
public String getDescription() {
return "Recipe created for the following Refaster template:\n```java\nstatic final class AssertThatWithFailMessageSupplierIsFalse {\n \n @BeforeTemplate\n void before(boolean actual, Supplier supplier) {\n assertFalse(actual, supplier);\n }\n \n @AfterTemplate\n @UseImportPolicy(value = STATIC_IMPORT_ALWAYS)\n void after(boolean actual, Supplier supplier) {\n assertThat(actual).withFailMessage(supplier).isFalse();\n }\n}\n```\n.";
}
@Override
public TreeVisitor, ExecutionContext> getVisitor() {
JavaVisitor javaVisitor = new AbstractRefasterJavaVisitor() {
final JavaTemplate before = JavaTemplate
.builder("org.junit.jupiter.api.Assertions.assertFalse(#{actual:any(boolean)}, #{supplier:any(java.util.function.Supplier)});")
.javaParser(JavaParser.fromJavaVersion().classpath(JavaParser.runtimeClasspath()))
.build();
final JavaTemplate after = JavaTemplate
.builder("org.assertj.core.api.Assertions.assertThat(#{actual:any(boolean)}).withFailMessage(#{supplier:any(java.util.function.Supplier)}).isFalse();")
.javaParser(JavaParser.fromJavaVersion().classpath(JavaParser.runtimeClasspath()))
.build();
@Override
public J visitMethodInvocation(J.MethodInvocation elem, ExecutionContext ctx) {
JavaTemplate.Matcher matcher;
if ((matcher = before.matcher(getCursor())).find()) {
maybeRemoveImport("org.junit.jupiter.api.Assertions.assertFalse");
return embed(
after.apply(getCursor(), elem.getCoordinates().replace(), matcher.parameter(0), matcher.parameter(1)),
getCursor(),
ctx,
SHORTEN_NAMES
);
}
return super.visitMethodInvocation(elem, ctx);
}
};
return Preconditions.check(
Preconditions.and(
new UsesType<>("java.util.function.Supplier", true),
new UsesMethod<>("org.junit.jupiter.api.Assertions assertFalse(..)")
),
javaVisitor
);
}
}
/**
* OpenRewrite recipe created for Refaster template {@code JUnitToAssertJRules.AssertThatIsNull}.
*/
@SuppressWarnings("all")
@NonNullApi
@Generated("org.openrewrite.java.template.processor.RefasterTemplateProcessor")
public static class AssertThatIsNullRecipe extends Recipe {
/**
* Instantiates a new instance.
*/
public AssertThatIsNullRecipe() {}
@Override
public String getDisplayName() {
return "Refaster template `JUnitToAssertJRules.AssertThatIsNull`";
}
@Override
public String getDescription() {
return "Recipe created for the following Refaster template:\n```java\nstatic final class AssertThatIsNull {\n \n @BeforeTemplate\n void before(Object actual) {\n assertNull(actual);\n }\n \n @AfterTemplate\n @UseImportPolicy(value = STATIC_IMPORT_ALWAYS)\n void after(Object actual) {\n assertThat(actual).isNull();\n }\n}\n```\n.";
}
@Override
public TreeVisitor, ExecutionContext> getVisitor() {
JavaVisitor javaVisitor = new AbstractRefasterJavaVisitor() {
final JavaTemplate before = JavaTemplate
.builder("org.junit.jupiter.api.Assertions.assertNull(#{actual:any(java.lang.Object)});")
.javaParser(JavaParser.fromJavaVersion().classpath(JavaParser.runtimeClasspath()))
.build();
final JavaTemplate after = JavaTemplate
.builder("org.assertj.core.api.Assertions.assertThat(#{actual:any(java.lang.Object)}).isNull();")
.javaParser(JavaParser.fromJavaVersion().classpath(JavaParser.runtimeClasspath()))
.build();
@Override
public J visitMethodInvocation(J.MethodInvocation elem, ExecutionContext ctx) {
JavaTemplate.Matcher matcher;
if ((matcher = before.matcher(getCursor())).find()) {
maybeRemoveImport("org.junit.jupiter.api.Assertions.assertNull");
return embed(
after.apply(getCursor(), elem.getCoordinates().replace(), matcher.parameter(0)),
getCursor(),
ctx,
SHORTEN_NAMES
);
}
return super.visitMethodInvocation(elem, ctx);
}
};
return Preconditions.check(
new UsesMethod<>("org.junit.jupiter.api.Assertions assertNull(..)"),
javaVisitor
);
}
}
/**
* OpenRewrite recipe created for Refaster template {@code JUnitToAssertJRules.AssertThatWithFailMessageStringIsNull}.
*/
@SuppressWarnings("all")
@NonNullApi
@Generated("org.openrewrite.java.template.processor.RefasterTemplateProcessor")
public static class AssertThatWithFailMessageStringIsNullRecipe extends Recipe {
/**
* Instantiates a new instance.
*/
public AssertThatWithFailMessageStringIsNullRecipe() {}
@Override
public String getDisplayName() {
return "Refaster template `JUnitToAssertJRules.AssertThatWithFailMessageStringIsNull`";
}
@Override
public String getDescription() {
return "Recipe created for the following Refaster template:\n```java\nstatic final class AssertThatWithFailMessageStringIsNull {\n \n @BeforeTemplate\n void before(Object actual, String message) {\n assertNull(actual, message);\n }\n \n @AfterTemplate\n @UseImportPolicy(value = STATIC_IMPORT_ALWAYS)\n void after(Object actual, String message) {\n assertThat(actual).withFailMessage(message).isNull();\n }\n}\n```\n.";
}
@Override
public TreeVisitor, ExecutionContext> getVisitor() {
JavaVisitor javaVisitor = new AbstractRefasterJavaVisitor() {
final JavaTemplate before = JavaTemplate
.builder("org.junit.jupiter.api.Assertions.assertNull(#{actual:any(java.lang.Object)}, #{message:any(java.lang.String)});")
.javaParser(JavaParser.fromJavaVersion().classpath(JavaParser.runtimeClasspath()))
.build();
final JavaTemplate after = JavaTemplate
.builder("org.assertj.core.api.Assertions.assertThat(#{actual:any(java.lang.Object)}).withFailMessage(#{message:any(java.lang.String)}).isNull();")
.javaParser(JavaParser.fromJavaVersion().classpath(JavaParser.runtimeClasspath()))
.build();
@Override
public J visitMethodInvocation(J.MethodInvocation elem, ExecutionContext ctx) {
JavaTemplate.Matcher matcher;
if ((matcher = before.matcher(getCursor())).find()) {
maybeRemoveImport("org.junit.jupiter.api.Assertions.assertNull");
return embed(
after.apply(getCursor(), elem.getCoordinates().replace(), matcher.parameter(0), matcher.parameter(1)),
getCursor(),
ctx,
SHORTEN_NAMES
);
}
return super.visitMethodInvocation(elem, ctx);
}
};
return Preconditions.check(
new UsesMethod<>("org.junit.jupiter.api.Assertions assertNull(..)"),
javaVisitor
);
}
}
/**
* OpenRewrite recipe created for Refaster template {@code JUnitToAssertJRules.AssertThatWithFailMessageSupplierIsNull}.
*/
@SuppressWarnings("all")
@NonNullApi
@Generated("org.openrewrite.java.template.processor.RefasterTemplateProcessor")
public static class AssertThatWithFailMessageSupplierIsNullRecipe extends Recipe {
/**
* Instantiates a new instance.
*/
public AssertThatWithFailMessageSupplierIsNullRecipe() {}
@Override
public String getDisplayName() {
return "Refaster template `JUnitToAssertJRules.AssertThatWithFailMessageSupplierIsNull`";
}
@Override
public String getDescription() {
return "Recipe created for the following Refaster template:\n```java\nstatic final class AssertThatWithFailMessageSupplierIsNull {\n \n @BeforeTemplate\n void before(Object actual, Supplier supplier) {\n assertNull(actual, supplier);\n }\n \n @AfterTemplate\n @UseImportPolicy(value = STATIC_IMPORT_ALWAYS)\n void after(Object actual, Supplier supplier) {\n assertThat(actual).withFailMessage(supplier).isNull();\n }\n}\n```\n.";
}
@Override
public TreeVisitor, ExecutionContext> getVisitor() {
JavaVisitor javaVisitor = new AbstractRefasterJavaVisitor() {
final JavaTemplate before = JavaTemplate
.builder("org.junit.jupiter.api.Assertions.assertNull(#{actual:any(java.lang.Object)}, #{supplier:any(java.util.function.Supplier)});")
.javaParser(JavaParser.fromJavaVersion().classpath(JavaParser.runtimeClasspath()))
.build();
final JavaTemplate after = JavaTemplate
.builder("org.assertj.core.api.Assertions.assertThat(#{actual:any(java.lang.Object)}).withFailMessage(#{supplier:any(java.util.function.Supplier)}).isNull();")
.javaParser(JavaParser.fromJavaVersion().classpath(JavaParser.runtimeClasspath()))
.build();
@Override
public J visitMethodInvocation(J.MethodInvocation elem, ExecutionContext ctx) {
JavaTemplate.Matcher matcher;
if ((matcher = before.matcher(getCursor())).find()) {
maybeRemoveImport("org.junit.jupiter.api.Assertions.assertNull");
return embed(
after.apply(getCursor(), elem.getCoordinates().replace(), matcher.parameter(0), matcher.parameter(1)),
getCursor(),
ctx,
SHORTEN_NAMES
);
}
return super.visitMethodInvocation(elem, ctx);
}
};
return Preconditions.check(
Preconditions.and(
new UsesType<>("java.util.function.Supplier", true),
new UsesMethod<>("org.junit.jupiter.api.Assertions assertNull(..)")
),
javaVisitor
);
}
}
/**
* OpenRewrite recipe created for Refaster template {@code JUnitToAssertJRules.AssertThatIsNotNull}.
*/
@SuppressWarnings("all")
@NonNullApi
@Generated("org.openrewrite.java.template.processor.RefasterTemplateProcessor")
public static class AssertThatIsNotNullRecipe extends Recipe {
/**
* Instantiates a new instance.
*/
public AssertThatIsNotNullRecipe() {}
@Override
public String getDisplayName() {
return "Refaster template `JUnitToAssertJRules.AssertThatIsNotNull`";
}
@Override
public String getDescription() {
return "Recipe created for the following Refaster template:\n```java\nstatic final class AssertThatIsNotNull {\n \n @BeforeTemplate\n void before(Object actual) {\n assertNotNull(actual);\n }\n \n @AfterTemplate\n @UseImportPolicy(value = STATIC_IMPORT_ALWAYS)\n void after(Object actual) {\n assertThat(actual).isNotNull();\n }\n}\n```\n.";
}
@Override
public TreeVisitor, ExecutionContext> getVisitor() {
JavaVisitor javaVisitor = new AbstractRefasterJavaVisitor() {
final JavaTemplate before = JavaTemplate
.builder("org.junit.jupiter.api.Assertions.assertNotNull(#{actual:any(java.lang.Object)});")
.javaParser(JavaParser.fromJavaVersion().classpath(JavaParser.runtimeClasspath()))
.build();
final JavaTemplate after = JavaTemplate
.builder("org.assertj.core.api.Assertions.assertThat(#{actual:any(java.lang.Object)}).isNotNull();")
.javaParser(JavaParser.fromJavaVersion().classpath(JavaParser.runtimeClasspath()))
.build();
@Override
public J visitMethodInvocation(J.MethodInvocation elem, ExecutionContext ctx) {
JavaTemplate.Matcher matcher;
if ((matcher = before.matcher(getCursor())).find()) {
maybeRemoveImport("org.junit.jupiter.api.Assertions.assertNotNull");
return embed(
after.apply(getCursor(), elem.getCoordinates().replace(), matcher.parameter(0)),
getCursor(),
ctx,
SHORTEN_NAMES
);
}
return super.visitMethodInvocation(elem, ctx);
}
};
return Preconditions.check(
new UsesMethod<>("org.junit.jupiter.api.Assertions assertNotNull(..)"),
javaVisitor
);
}
}
/**
* OpenRewrite recipe created for Refaster template {@code JUnitToAssertJRules.AssertThatWithFailMessageStringIsNotNull}.
*/
@SuppressWarnings("all")
@NonNullApi
@Generated("org.openrewrite.java.template.processor.RefasterTemplateProcessor")
public static class AssertThatWithFailMessageStringIsNotNullRecipe extends Recipe {
/**
* Instantiates a new instance.
*/
public AssertThatWithFailMessageStringIsNotNullRecipe() {}
@Override
public String getDisplayName() {
return "Refaster template `JUnitToAssertJRules.AssertThatWithFailMessageStringIsNotNull`";
}
@Override
public String getDescription() {
return "Recipe created for the following Refaster template:\n```java\nstatic final class AssertThatWithFailMessageStringIsNotNull {\n \n @BeforeTemplate\n void before(Object actual, String message) {\n assertNotNull(actual, message);\n }\n \n @AfterTemplate\n @UseImportPolicy(value = STATIC_IMPORT_ALWAYS)\n void after(Object actual, String message) {\n assertThat(actual).withFailMessage(message).isNotNull();\n }\n}\n```\n.";
}
@Override
public TreeVisitor, ExecutionContext> getVisitor() {
JavaVisitor javaVisitor = new AbstractRefasterJavaVisitor() {
final JavaTemplate before = JavaTemplate
.builder("org.junit.jupiter.api.Assertions.assertNotNull(#{actual:any(java.lang.Object)}, #{message:any(java.lang.String)});")
.javaParser(JavaParser.fromJavaVersion().classpath(JavaParser.runtimeClasspath()))
.build();
final JavaTemplate after = JavaTemplate
.builder("org.assertj.core.api.Assertions.assertThat(#{actual:any(java.lang.Object)}).withFailMessage(#{message:any(java.lang.String)}).isNotNull();")
.javaParser(JavaParser.fromJavaVersion().classpath(JavaParser.runtimeClasspath()))
.build();
@Override
public J visitMethodInvocation(J.MethodInvocation elem, ExecutionContext ctx) {
JavaTemplate.Matcher matcher;
if ((matcher = before.matcher(getCursor())).find()) {
maybeRemoveImport("org.junit.jupiter.api.Assertions.assertNotNull");
return embed(
after.apply(getCursor(), elem.getCoordinates().replace(), matcher.parameter(0), matcher.parameter(1)),
getCursor(),
ctx,
SHORTEN_NAMES
);
}
return super.visitMethodInvocation(elem, ctx);
}
};
return Preconditions.check(
new UsesMethod<>("org.junit.jupiter.api.Assertions assertNotNull(..)"),
javaVisitor
);
}
}
/**
* OpenRewrite recipe created for Refaster template {@code JUnitToAssertJRules.AssertThatWithFailMessageSupplierIsNotNull}.
*/
@SuppressWarnings("all")
@NonNullApi
@Generated("org.openrewrite.java.template.processor.RefasterTemplateProcessor")
public static class AssertThatWithFailMessageSupplierIsNotNullRecipe extends Recipe {
/**
* Instantiates a new instance.
*/
public AssertThatWithFailMessageSupplierIsNotNullRecipe() {}
@Override
public String getDisplayName() {
return "Refaster template `JUnitToAssertJRules.AssertThatWithFailMessageSupplierIsNotNull`";
}
@Override
public String getDescription() {
return "Recipe created for the following Refaster template:\n```java\nstatic final class AssertThatWithFailMessageSupplierIsNotNull {\n \n @BeforeTemplate\n void before(Object actual, Supplier supplier) {\n assertNotNull(actual, supplier);\n }\n \n @AfterTemplate\n @UseImportPolicy(value = STATIC_IMPORT_ALWAYS)\n void after(Object actual, Supplier supplier) {\n assertThat(actual).withFailMessage(supplier).isNotNull();\n }\n}\n```\n.";
}
@Override
public TreeVisitor, ExecutionContext> getVisitor() {
JavaVisitor javaVisitor = new AbstractRefasterJavaVisitor() {
final JavaTemplate before = JavaTemplate
.builder("org.junit.jupiter.api.Assertions.assertNotNull(#{actual:any(java.lang.Object)}, #{supplier:any(java.util.function.Supplier)});")
.javaParser(JavaParser.fromJavaVersion().classpath(JavaParser.runtimeClasspath()))
.build();
final JavaTemplate after = JavaTemplate
.builder("org.assertj.core.api.Assertions.assertThat(#{actual:any(java.lang.Object)}).withFailMessage(#{supplier:any(java.util.function.Supplier)}).isNotNull();")
.javaParser(JavaParser.fromJavaVersion().classpath(JavaParser.runtimeClasspath()))
.build();
@Override
public J visitMethodInvocation(J.MethodInvocation elem, ExecutionContext ctx) {
JavaTemplate.Matcher matcher;
if ((matcher = before.matcher(getCursor())).find()) {
maybeRemoveImport("org.junit.jupiter.api.Assertions.assertNotNull");
return embed(
after.apply(getCursor(), elem.getCoordinates().replace(), matcher.parameter(0), matcher.parameter(1)),
getCursor(),
ctx,
SHORTEN_NAMES
);
}
return super.visitMethodInvocation(elem, ctx);
}
};
return Preconditions.check(
Preconditions.and(
new UsesType<>("java.util.function.Supplier", true),
new UsesMethod<>("org.junit.jupiter.api.Assertions assertNotNull(..)")
),
javaVisitor
);
}
}
/**
* OpenRewrite recipe created for Refaster template {@code JUnitToAssertJRules.AssertThatIsSameAs}.
*/
@SuppressWarnings("all")
@NonNullApi
@Generated("org.openrewrite.java.template.processor.RefasterTemplateProcessor")
public static class AssertThatIsSameAsRecipe extends Recipe {
/**
* Instantiates a new instance.
*/
public AssertThatIsSameAsRecipe() {}
@Override
public String getDisplayName() {
return "Refaster template `JUnitToAssertJRules.AssertThatIsSameAs`";
}
@Override
public String getDescription() {
return "Recipe created for the following Refaster template:\n```java\nstatic final class AssertThatIsSameAs {\n \n @BeforeTemplate\n void before(Object actual, Object expected) {\n assertSame(expected, actual);\n }\n \n @AfterTemplate\n @UseImportPolicy(value = STATIC_IMPORT_ALWAYS)\n void after(Object actual, Object expected) {\n assertThat(actual).isSameAs(expected);\n }\n}\n```\n.";
}
@Override
public TreeVisitor, ExecutionContext> getVisitor() {
JavaVisitor javaVisitor = new AbstractRefasterJavaVisitor() {
final JavaTemplate before = JavaTemplate
.builder("org.junit.jupiter.api.Assertions.assertSame(#{expected:any(java.lang.Object)}, #{actual:any(java.lang.Object)});")
.javaParser(JavaParser.fromJavaVersion().classpath(JavaParser.runtimeClasspath()))
.build();
final JavaTemplate after = JavaTemplate
.builder("org.assertj.core.api.Assertions.assertThat(#{actual:any(java.lang.Object)}).isSameAs(#{expected:any(java.lang.Object)});")
.javaParser(JavaParser.fromJavaVersion().classpath(JavaParser.runtimeClasspath()))
.build();
@Override
public J visitMethodInvocation(J.MethodInvocation elem, ExecutionContext ctx) {
JavaTemplate.Matcher matcher;
if ((matcher = before.matcher(getCursor())).find()) {
maybeRemoveImport("org.junit.jupiter.api.Assertions.assertSame");
return embed(
after.apply(getCursor(), elem.getCoordinates().replace(), matcher.parameter(1), matcher.parameter(0)),
getCursor(),
ctx,
SHORTEN_NAMES
);
}
return super.visitMethodInvocation(elem, ctx);
}
};
return Preconditions.check(
new UsesMethod<>("org.junit.jupiter.api.Assertions assertSame(..)"),
javaVisitor
);
}
}
/**
* OpenRewrite recipe created for Refaster template {@code JUnitToAssertJRules.AssertThatWithFailMessageStringIsSameAs}.
*/
@SuppressWarnings("all")
@NonNullApi
@Generated("org.openrewrite.java.template.processor.RefasterTemplateProcessor")
public static class AssertThatWithFailMessageStringIsSameAsRecipe extends Recipe {
/**
* Instantiates a new instance.
*/
public AssertThatWithFailMessageStringIsSameAsRecipe() {}
@Override
public String getDisplayName() {
return "Refaster template `JUnitToAssertJRules.AssertThatWithFailMessageStringIsSameAs`";
}
@Override
public String getDescription() {
return "Recipe created for the following Refaster template:\n```java\nstatic final class AssertThatWithFailMessageStringIsSameAs {\n \n @BeforeTemplate\n void before(Object actual, String message, Object expected) {\n assertSame(expected, actual, message);\n }\n \n @AfterTemplate\n @UseImportPolicy(value = STATIC_IMPORT_ALWAYS)\n void after(Object actual, String message, Object expected) {\n assertThat(actual).withFailMessage(message).isSameAs(expected);\n }\n}\n```\n.";
}
@Override
public TreeVisitor, ExecutionContext> getVisitor() {
JavaVisitor javaVisitor = new AbstractRefasterJavaVisitor() {
final JavaTemplate before = JavaTemplate
.builder("org.junit.jupiter.api.Assertions.assertSame(#{expected:any(java.lang.Object)}, #{actual:any(java.lang.Object)}, #{message:any(java.lang.String)});")
.javaParser(JavaParser.fromJavaVersion().classpath(JavaParser.runtimeClasspath()))
.build();
final JavaTemplate after = JavaTemplate
.builder("org.assertj.core.api.Assertions.assertThat(#{actual:any(java.lang.Object)}).withFailMessage(#{message:any(java.lang.String)}).isSameAs(#{expected:any(java.lang.Object)});")
.javaParser(JavaParser.fromJavaVersion().classpath(JavaParser.runtimeClasspath()))
.build();
@Override
public J visitMethodInvocation(J.MethodInvocation elem, ExecutionContext ctx) {
JavaTemplate.Matcher matcher;
if ((matcher = before.matcher(getCursor())).find()) {
maybeRemoveImport("org.junit.jupiter.api.Assertions.assertSame");
return embed(
after.apply(getCursor(), elem.getCoordinates().replace(), matcher.parameter(1), matcher.parameter(2), matcher.parameter(0)),
getCursor(),
ctx,
SHORTEN_NAMES
);
}
return super.visitMethodInvocation(elem, ctx);
}
};
return Preconditions.check(
new UsesMethod<>("org.junit.jupiter.api.Assertions assertSame(..)"),
javaVisitor
);
}
}
/**
* OpenRewrite recipe created for Refaster template {@code JUnitToAssertJRules.AssertThatWithFailMessageSupplierIsSameAs}.
*/
@SuppressWarnings("all")
@NonNullApi
@Generated("org.openrewrite.java.template.processor.RefasterTemplateProcessor")
public static class AssertThatWithFailMessageSupplierIsSameAsRecipe extends Recipe {
/**
* Instantiates a new instance.
*/
public AssertThatWithFailMessageSupplierIsSameAsRecipe() {}
@Override
public String getDisplayName() {
return "Refaster template `JUnitToAssertJRules.AssertThatWithFailMessageSupplierIsSameAs`";
}
@Override
public String getDescription() {
return "Recipe created for the following Refaster template:\n```java\nstatic final class AssertThatWithFailMessageSupplierIsSameAs {\n \n @BeforeTemplate\n void before(Object actual, Supplier supplier, Object expected) {\n assertSame(expected, actual, supplier);\n }\n \n @AfterTemplate\n @UseImportPolicy(value = STATIC_IMPORT_ALWAYS)\n void after(Object actual, Supplier supplier, Object expected) {\n assertThat(actual).withFailMessage(supplier).isSameAs(expected);\n }\n}\n```\n.";
}
@Override
public TreeVisitor, ExecutionContext> getVisitor() {
JavaVisitor javaVisitor = new AbstractRefasterJavaVisitor() {
final JavaTemplate before = JavaTemplate
.builder("org.junit.jupiter.api.Assertions.assertSame(#{expected:any(java.lang.Object)}, #{actual:any(java.lang.Object)}, #{supplier:any(java.util.function.Supplier)});")
.javaParser(JavaParser.fromJavaVersion().classpath(JavaParser.runtimeClasspath()))
.build();
final JavaTemplate after = JavaTemplate
.builder("org.assertj.core.api.Assertions.assertThat(#{actual:any(java.lang.Object)}).withFailMessage(#{supplier:any(java.util.function.Supplier)}).isSameAs(#{expected:any(java.lang.Object)});")
.javaParser(JavaParser.fromJavaVersion().classpath(JavaParser.runtimeClasspath()))
.build();
@Override
public J visitMethodInvocation(J.MethodInvocation elem, ExecutionContext ctx) {
JavaTemplate.Matcher matcher;
if ((matcher = before.matcher(getCursor())).find()) {
maybeRemoveImport("org.junit.jupiter.api.Assertions.assertSame");
return embed(
after.apply(getCursor(), elem.getCoordinates().replace(), matcher.parameter(1), matcher.parameter(2), matcher.parameter(0)),
getCursor(),
ctx,
SHORTEN_NAMES
);
}
return super.visitMethodInvocation(elem, ctx);
}
};
return Preconditions.check(
Preconditions.and(
new UsesType<>("java.util.function.Supplier", true),
new UsesMethod<>("org.junit.jupiter.api.Assertions assertSame(..)")
),
javaVisitor
);
}
}
/**
* OpenRewrite recipe created for Refaster template {@code JUnitToAssertJRules.AssertThatIsNotSameAs}.
*/
@SuppressWarnings("all")
@NonNullApi
@Generated("org.openrewrite.java.template.processor.RefasterTemplateProcessor")
public static class AssertThatIsNotSameAsRecipe extends Recipe {
/**
* Instantiates a new instance.
*/
public AssertThatIsNotSameAsRecipe() {}
@Override
public String getDisplayName() {
return "Refaster template `JUnitToAssertJRules.AssertThatIsNotSameAs`";
}
@Override
public String getDescription() {
return "Recipe created for the following Refaster template:\n```java\nstatic final class AssertThatIsNotSameAs {\n \n @BeforeTemplate\n void before(Object actual, Object expected) {\n assertNotSame(expected, actual);\n }\n \n @AfterTemplate\n @UseImportPolicy(value = STATIC_IMPORT_ALWAYS)\n void after(Object actual, Object expected) {\n assertThat(actual).isNotSameAs(expected);\n }\n}\n```\n.";
}
@Override
public TreeVisitor, ExecutionContext> getVisitor() {
JavaVisitor javaVisitor = new AbstractRefasterJavaVisitor() {
final JavaTemplate before = JavaTemplate
.builder("org.junit.jupiter.api.Assertions.assertNotSame(#{expected:any(java.lang.Object)}, #{actual:any(java.lang.Object)});")
.javaParser(JavaParser.fromJavaVersion().classpath(JavaParser.runtimeClasspath()))
.build();
final JavaTemplate after = JavaTemplate
.builder("org.assertj.core.api.Assertions.assertThat(#{actual:any(java.lang.Object)}).isNotSameAs(#{expected:any(java.lang.Object)});")
.javaParser(JavaParser.fromJavaVersion().classpath(JavaParser.runtimeClasspath()))
.build();
@Override
public J visitMethodInvocation(J.MethodInvocation elem, ExecutionContext ctx) {
JavaTemplate.Matcher matcher;
if ((matcher = before.matcher(getCursor())).find()) {
maybeRemoveImport("org.junit.jupiter.api.Assertions.assertNotSame");
return embed(
after.apply(getCursor(), elem.getCoordinates().replace(), matcher.parameter(1), matcher.parameter(0)),
getCursor(),
ctx,
SHORTEN_NAMES
);
}
return super.visitMethodInvocation(elem, ctx);
}
};
return Preconditions.check(
new UsesMethod<>("org.junit.jupiter.api.Assertions assertNotSame(..)"),
javaVisitor
);
}
}
/**
* OpenRewrite recipe created for Refaster template {@code JUnitToAssertJRules.AssertThatWithFailMessageStringIsNotSameAs}.
*/
@SuppressWarnings("all")
@NonNullApi
@Generated("org.openrewrite.java.template.processor.RefasterTemplateProcessor")
public static class AssertThatWithFailMessageStringIsNotSameAsRecipe extends Recipe {
/**
* Instantiates a new instance.
*/
public AssertThatWithFailMessageStringIsNotSameAsRecipe() {}
@Override
public String getDisplayName() {
return "Refaster template `JUnitToAssertJRules.AssertThatWithFailMessageStringIsNotSameAs`";
}
@Override
public String getDescription() {
return "Recipe created for the following Refaster template:\n```java\nstatic final class AssertThatWithFailMessageStringIsNotSameAs {\n \n @BeforeTemplate\n void before(Object actual, String message, Object expected) {\n assertNotSame(expected, actual, message);\n }\n \n @AfterTemplate\n @UseImportPolicy(value = STATIC_IMPORT_ALWAYS)\n void after(Object actual, String message, Object expected) {\n assertThat(actual).withFailMessage(message).isNotSameAs(expected);\n }\n}\n```\n.";
}
@Override
public TreeVisitor, ExecutionContext> getVisitor() {
JavaVisitor javaVisitor = new AbstractRefasterJavaVisitor() {
final JavaTemplate before = JavaTemplate
.builder("org.junit.jupiter.api.Assertions.assertNotSame(#{expected:any(java.lang.Object)}, #{actual:any(java.lang.Object)}, #{message:any(java.lang.String)});")
.javaParser(JavaParser.fromJavaVersion().classpath(JavaParser.runtimeClasspath()))
.build();
final JavaTemplate after = JavaTemplate
.builder("org.assertj.core.api.Assertions.assertThat(#{actual:any(java.lang.Object)}).withFailMessage(#{message:any(java.lang.String)}).isNotSameAs(#{expected:any(java.lang.Object)});")
.javaParser(JavaParser.fromJavaVersion().classpath(JavaParser.runtimeClasspath()))
.build();
@Override
public J visitMethodInvocation(J.MethodInvocation elem, ExecutionContext ctx) {
JavaTemplate.Matcher matcher;
if ((matcher = before.matcher(getCursor())).find()) {
maybeRemoveImport("org.junit.jupiter.api.Assertions.assertNotSame");
return embed(
after.apply(getCursor(), elem.getCoordinates().replace(), matcher.parameter(1), matcher.parameter(2), matcher.parameter(0)),
getCursor(),
ctx,
SHORTEN_NAMES
);
}
return super.visitMethodInvocation(elem, ctx);
}
};
return Preconditions.check(
new UsesMethod<>("org.junit.jupiter.api.Assertions assertNotSame(..)"),
javaVisitor
);
}
}
/**
* OpenRewrite recipe created for Refaster template {@code JUnitToAssertJRules.AssertThatWithFailMessageSupplierIsNotSameAs}.
*/
@SuppressWarnings("all")
@NonNullApi
@Generated("org.openrewrite.java.template.processor.RefasterTemplateProcessor")
public static class AssertThatWithFailMessageSupplierIsNotSameAsRecipe extends Recipe {
/**
* Instantiates a new instance.
*/
public AssertThatWithFailMessageSupplierIsNotSameAsRecipe() {}
@Override
public String getDisplayName() {
return "Refaster template `JUnitToAssertJRules.AssertThatWithFailMessageSupplierIsNotSameAs`";
}
@Override
public String getDescription() {
return "Recipe created for the following Refaster template:\n```java\nstatic final class AssertThatWithFailMessageSupplierIsNotSameAs {\n \n @BeforeTemplate\n void before(Object actual, Supplier supplier, Object expected) {\n assertNotSame(expected, actual, supplier);\n }\n \n @AfterTemplate\n @UseImportPolicy(value = STATIC_IMPORT_ALWAYS)\n void after(Object actual, Supplier supplier, Object expected) {\n assertThat(actual).withFailMessage(supplier).isNotSameAs(expected);\n }\n}\n```\n.";
}
@Override
public TreeVisitor, ExecutionContext> getVisitor() {
JavaVisitor javaVisitor = new AbstractRefasterJavaVisitor() {
final JavaTemplate before = JavaTemplate
.builder("org.junit.jupiter.api.Assertions.assertNotSame(#{expected:any(java.lang.Object)}, #{actual:any(java.lang.Object)}, #{supplier:any(java.util.function.Supplier)});")
.javaParser(JavaParser.fromJavaVersion().classpath(JavaParser.runtimeClasspath()))
.build();
final JavaTemplate after = JavaTemplate
.builder("org.assertj.core.api.Assertions.assertThat(#{actual:any(java.lang.Object)}).withFailMessage(#{supplier:any(java.util.function.Supplier)}).isNotSameAs(#{expected:any(java.lang.Object)});")
.javaParser(JavaParser.fromJavaVersion().classpath(JavaParser.runtimeClasspath()))
.build();
@Override
public J visitMethodInvocation(J.MethodInvocation elem, ExecutionContext ctx) {
JavaTemplate.Matcher matcher;
if ((matcher = before.matcher(getCursor())).find()) {
maybeRemoveImport("org.junit.jupiter.api.Assertions.assertNotSame");
return embed(
after.apply(getCursor(), elem.getCoordinates().replace(), matcher.parameter(1), matcher.parameter(2), matcher.parameter(0)),
getCursor(),
ctx,
SHORTEN_NAMES
);
}
return super.visitMethodInvocation(elem, ctx);
}
};
return Preconditions.check(
Preconditions.and(
new UsesType<>("java.util.function.Supplier", true),
new UsesMethod<>("org.junit.jupiter.api.Assertions assertNotSame(..)")
),
javaVisitor
);
}
}
/**
* OpenRewrite recipe created for Refaster template {@code JUnitToAssertJRules.AssertThatCodeDoesNotThrowAnyException}.
*/
@SuppressWarnings("all")
@NonNullApi
@Generated("org.openrewrite.java.template.processor.RefasterTemplateProcessor")
public static class AssertThatCodeDoesNotThrowAnyExceptionRecipe extends Recipe {
/**
* Instantiates a new instance.
*/
public AssertThatCodeDoesNotThrowAnyExceptionRecipe() {}
@Override
public String getDisplayName() {
return "Refaster template `JUnitToAssertJRules.AssertThatCodeDoesNotThrowAnyException`";
}
@Override
public String getDescription() {
return "Recipe created for the following Refaster template:\n```java\nstatic final class AssertThatCodeDoesNotThrowAnyException {\n \n @BeforeTemplate\n void before(Executable throwingCallable) {\n assertDoesNotThrow(throwingCallable);\n }\n \n @BeforeTemplate\n void before(ThrowingSupplier> throwingCallable) {\n assertDoesNotThrow(throwingCallable);\n }\n \n @AfterTemplate\n @UseImportPolicy(value = STATIC_IMPORT_ALWAYS)\n void after(ThrowingCallable throwingCallable) {\n assertThatCode(throwingCallable).doesNotThrowAnyException();\n }\n}\n```\n.";
}
@Override
public TreeVisitor, ExecutionContext> getVisitor() {
JavaVisitor javaVisitor = new AbstractRefasterJavaVisitor() {
final JavaTemplate before = JavaTemplate
.builder("org.junit.jupiter.api.Assertions.assertDoesNotThrow(#{throwingCallable:any(org.junit.jupiter.api.function.Executable)});")
.javaParser(JavaParser.fromJavaVersion().classpath(JavaParser.runtimeClasspath()))
.build();
final JavaTemplate before0 = JavaTemplate
.builder("org.junit.jupiter.api.Assertions.assertDoesNotThrow(#{throwingCallable:any(org.junit.jupiter.api.function.ThrowingSupplier>)});")
.javaParser(JavaParser.fromJavaVersion().classpath(JavaParser.runtimeClasspath()))
.build();
final JavaTemplate after = JavaTemplate
.builder("org.assertj.core.api.Assertions.assertThatCode(#{throwingCallable:any(org.assertj.core.api.ThrowableAssert.ThrowingCallable)}).doesNotThrowAnyException();")
.javaParser(JavaParser.fromJavaVersion().classpath(JavaParser.runtimeClasspath()))
.build();
@Override
public J visitMethodInvocation(J.MethodInvocation elem, ExecutionContext ctx) {
JavaTemplate.Matcher matcher;
if ((matcher = before.matcher(getCursor())).find()) {
maybeRemoveImport("org.junit.jupiter.api.function.Executable");
maybeRemoveImport("org.junit.jupiter.api.Assertions.assertDoesNotThrow");
return embed(
after.apply(getCursor(), elem.getCoordinates().replace(), matcher.parameter(0)),
getCursor(),
ctx,
SHORTEN_NAMES
);
}
if ((matcher = before0.matcher(getCursor())).find()) {
maybeRemoveImport("org.junit.jupiter.api.function.ThrowingSupplier");
maybeRemoveImport("org.junit.jupiter.api.Assertions.assertDoesNotThrow");
return embed(
after.apply(getCursor(), elem.getCoordinates().replace(), matcher.parameter(0)),
getCursor(),
ctx,
SHORTEN_NAMES
);
}
return super.visitMethodInvocation(elem, ctx);
}
};
return Preconditions.check(
Preconditions.and(
new UsesType<>("org.junit.jupiter.api.function.ThrowingSupplier", true),
new UsesMethod<>("org.junit.jupiter.api.Assertions assertDoesNotThrow(..)")
),
javaVisitor
);
}
}
/**
* OpenRewrite recipe created for Refaster template {@code JUnitToAssertJRules.AssertThatCodeWithFailMessageStringDoesNotThrowAnyException}.
*/
@SuppressWarnings("all")
@NonNullApi
@Generated("org.openrewrite.java.template.processor.RefasterTemplateProcessor")
public static class AssertThatCodeWithFailMessageStringDoesNotThrowAnyExceptionRecipe extends Recipe {
/**
* Instantiates a new instance.
*/
public AssertThatCodeWithFailMessageStringDoesNotThrowAnyExceptionRecipe() {}
@Override
public String getDisplayName() {
return "Refaster template `JUnitToAssertJRules.AssertThatCodeWithFailMessageStringDoesNotThrowAnyException`";
}
@Override
public String getDescription() {
return "Recipe created for the following Refaster template:\n```java\nstatic final class AssertThatCodeWithFailMessageStringDoesNotThrowAnyException {\n \n @BeforeTemplate\n void before(Executable throwingCallable, String message) {\n assertDoesNotThrow(throwingCallable, message);\n }\n \n @BeforeTemplate\n void before(ThrowingSupplier> throwingCallable, String message) {\n assertDoesNotThrow(throwingCallable, message);\n }\n \n @AfterTemplate\n @UseImportPolicy(value = STATIC_IMPORT_ALWAYS)\n void after(ThrowingCallable throwingCallable, String message) {\n assertThatCode(throwingCallable).withFailMessage(message).doesNotThrowAnyException();\n }\n}\n```\n.";
}
@Override
public TreeVisitor, ExecutionContext> getVisitor() {
JavaVisitor javaVisitor = new AbstractRefasterJavaVisitor() {
final JavaTemplate before = JavaTemplate
.builder("org.junit.jupiter.api.Assertions.assertDoesNotThrow(#{throwingCallable:any(org.junit.jupiter.api.function.Executable)}, #{message:any(java.lang.String)});")
.javaParser(JavaParser.fromJavaVersion().classpath(JavaParser.runtimeClasspath()))
.build();
final JavaTemplate before0 = JavaTemplate
.builder("org.junit.jupiter.api.Assertions.assertDoesNotThrow(#{throwingCallable:any(org.junit.jupiter.api.function.ThrowingSupplier>)}, #{message:any(java.lang.String)});")
.javaParser(JavaParser.fromJavaVersion().classpath(JavaParser.runtimeClasspath()))
.build();
final JavaTemplate after = JavaTemplate
.builder("org.assertj.core.api.Assertions.assertThatCode(#{throwingCallable:any(org.assertj.core.api.ThrowableAssert.ThrowingCallable)}).withFailMessage(#{message:any(java.lang.String)}).doesNotThrowAnyException();")
.javaParser(JavaParser.fromJavaVersion().classpath(JavaParser.runtimeClasspath()))
.build();
@Override
public J visitMethodInvocation(J.MethodInvocation elem, ExecutionContext ctx) {
JavaTemplate.Matcher matcher;
if ((matcher = before.matcher(getCursor())).find()) {
maybeRemoveImport("org.junit.jupiter.api.function.Executable");
maybeRemoveImport("org.junit.jupiter.api.Assertions.assertDoesNotThrow");
return embed(
after.apply(getCursor(), elem.getCoordinates().replace(), matcher.parameter(0), matcher.parameter(1)),
getCursor(),
ctx,
SHORTEN_NAMES
);
}
if ((matcher = before0.matcher(getCursor())).find()) {
maybeRemoveImport("org.junit.jupiter.api.function.ThrowingSupplier");
maybeRemoveImport("org.junit.jupiter.api.Assertions.assertDoesNotThrow");
return embed(
after.apply(getCursor(), elem.getCoordinates().replace(), matcher.parameter(0), matcher.parameter(1)),
getCursor(),
ctx,
SHORTEN_NAMES
);
}
return super.visitMethodInvocation(elem, ctx);
}
};
return Preconditions.check(
Preconditions.and(
new UsesType<>("org.junit.jupiter.api.function.ThrowingSupplier", true),
new UsesMethod<>("org.junit.jupiter.api.Assertions assertDoesNotThrow(..)")
),
javaVisitor
);
}
}
/**
* OpenRewrite recipe created for Refaster template {@code JUnitToAssertJRules.AssertThatCodeWithFailMessageSupplierDoesNotThrowAnyException}.
*/
@SuppressWarnings("all")
@NonNullApi
@Generated("org.openrewrite.java.template.processor.RefasterTemplateProcessor")
public static class AssertThatCodeWithFailMessageSupplierDoesNotThrowAnyExceptionRecipe extends Recipe {
/**
* Instantiates a new instance.
*/
public AssertThatCodeWithFailMessageSupplierDoesNotThrowAnyExceptionRecipe() {}
@Override
public String getDisplayName() {
return "Refaster template `JUnitToAssertJRules.AssertThatCodeWithFailMessageSupplierDoesNotThrowAnyException`";
}
@Override
public String getDescription() {
return "Recipe created for the following Refaster template:\n```java\nstatic final class AssertThatCodeWithFailMessageSupplierDoesNotThrowAnyException {\n \n @BeforeTemplate\n void before(Executable throwingCallable, Supplier supplier) {\n assertDoesNotThrow(throwingCallable, supplier);\n }\n \n @BeforeTemplate\n void before(ThrowingSupplier> throwingCallable, Supplier supplier) {\n assertDoesNotThrow(throwingCallable, supplier);\n }\n \n @AfterTemplate\n @UseImportPolicy(value = STATIC_IMPORT_ALWAYS)\n void after(ThrowingCallable throwingCallable, Supplier supplier) {\n assertThatCode(throwingCallable).withFailMessage(supplier).doesNotThrowAnyException();\n }\n}\n```\n.";
}
@Override
public TreeVisitor, ExecutionContext> getVisitor() {
JavaVisitor javaVisitor = new AbstractRefasterJavaVisitor() {
final JavaTemplate before = JavaTemplate
.builder("org.junit.jupiter.api.Assertions.assertDoesNotThrow(#{throwingCallable:any(org.junit.jupiter.api.function.Executable)}, #{supplier:any(java.util.function.Supplier)});")
.javaParser(JavaParser.fromJavaVersion().classpath(JavaParser.runtimeClasspath()))
.build();
final JavaTemplate before0 = JavaTemplate
.builder("org.junit.jupiter.api.Assertions.assertDoesNotThrow(#{throwingCallable:any(org.junit.jupiter.api.function.ThrowingSupplier>)}, #{supplier:any(java.util.function.Supplier)});")
.javaParser(JavaParser.fromJavaVersion().classpath(JavaParser.runtimeClasspath()))
.build();
final JavaTemplate after = JavaTemplate
.builder("org.assertj.core.api.Assertions.assertThatCode(#{throwingCallable:any(org.assertj.core.api.ThrowableAssert.ThrowingCallable)}).withFailMessage(#{supplier:any(java.util.function.Supplier)}).doesNotThrowAnyException();")
.javaParser(JavaParser.fromJavaVersion().classpath(JavaParser.runtimeClasspath()))
.build();
@Override
public J visitMethodInvocation(J.MethodInvocation elem, ExecutionContext ctx) {
JavaTemplate.Matcher matcher;
if ((matcher = before.matcher(getCursor())).find()) {
maybeRemoveImport("org.junit.jupiter.api.function.Executable");
maybeRemoveImport("org.junit.jupiter.api.Assertions.assertDoesNotThrow");
return embed(
after.apply(getCursor(), elem.getCoordinates().replace(), matcher.parameter(0), matcher.parameter(1)),
getCursor(),
ctx,
SHORTEN_NAMES
);
}
if ((matcher = before0.matcher(getCursor())).find()) {
maybeRemoveImport("org.junit.jupiter.api.function.ThrowingSupplier");
maybeRemoveImport("org.junit.jupiter.api.Assertions.assertDoesNotThrow");
return embed(
after.apply(getCursor(), elem.getCoordinates().replace(), matcher.parameter(0), matcher.parameter(1)),
getCursor(),
ctx,
SHORTEN_NAMES
);
}
return super.visitMethodInvocation(elem, ctx);
}
};
return Preconditions.check(
Preconditions.and(
new UsesType<>("org.junit.jupiter.api.function.ThrowingSupplier", true),
new UsesType<>("java.util.function.Supplier", true),
new UsesMethod<>("org.junit.jupiter.api.Assertions assertDoesNotThrow(..)")
),
javaVisitor
);
}
}
}