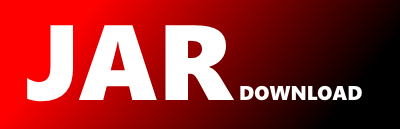
tech.picnic.errorprone.refasterrules.MockitoRulesRecipes Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of error-prone-contrib Show documentation
Show all versions of error-prone-contrib Show documentation
Extra Error Prone plugins by Picnic.
package tech.picnic.errorprone.refasterrules;
import org.openrewrite.ExecutionContext;
import org.openrewrite.Preconditions;
import org.openrewrite.Recipe;
import org.openrewrite.TreeVisitor;
import org.openrewrite.internal.lang.NonNullApi;
import org.openrewrite.java.JavaParser;
import org.openrewrite.java.JavaTemplate;
import org.openrewrite.java.JavaVisitor;
import org.openrewrite.java.search.*;
import org.openrewrite.java.template.Primitive;
import org.openrewrite.java.template.function.*;
import org.openrewrite.java.template.internal.AbstractRefasterJavaVisitor;
import org.openrewrite.java.tree.*;
import javax.annotation.Generated;
import java.util.*;
import static org.openrewrite.java.template.internal.AbstractRefasterJavaVisitor.EmbeddingOption.*;
/**
* OpenRewrite recipes created for Refaster template {@code tech.picnic.errorprone.refasterrules.MockitoRules}.
*/
@SuppressWarnings("all")
@Generated("org.openrewrite.java.template.processor.RefasterTemplateProcessor")
public class MockitoRulesRecipes extends Recipe {
/**
* Instantiates a new instance.
*/
public MockitoRulesRecipes() {}
@Override
public String getDisplayName() {
return "Refaster rules related to Mockito expressions and statements";
}
@Override
public String getDescription() {
return "Refaster template recipes for `tech.picnic.errorprone.refasterrules.MockitoRules`. [Source](https://error-prone.picnic.tech/refasterrules/MockitoRules).";
}
@Override
public List getRecipeList() {
return Arrays.asList(
new NeverRecipe(),
new InvocationOnMockGetArgumentsRecipe()
);
}
/**
* OpenRewrite recipe created for Refaster template {@code MockitoRules.Never}.
*/
@SuppressWarnings("all")
@NonNullApi
@Generated("org.openrewrite.java.template.processor.RefasterTemplateProcessor")
public static class NeverRecipe extends Recipe {
/**
* Instantiates a new instance.
*/
public NeverRecipe() {}
@Override
public String getDisplayName() {
return "Prefer `Mockito#never()`} over explicitly specifying that the associated invocation must happen precisely zero times";
}
@Override
public String getDescription() {
return "Recipe created for the following Refaster template:\n```java\nstatic final class Never {\n \n @BeforeTemplate\n VerificationMode before() {\n return times(0);\n }\n \n @AfterTemplate\n @UseImportPolicy(value = STATIC_IMPORT_ALWAYS)\n VerificationMode after() {\n return never();\n }\n}\n```\n.";
}
@Override
public TreeVisitor, ExecutionContext> getVisitor() {
JavaVisitor javaVisitor = new AbstractRefasterJavaVisitor() {
final JavaTemplate before = JavaTemplate
.builder("org.mockito.Mockito.times(0)")
.javaParser(JavaParser.fromJavaVersion().classpath(JavaParser.runtimeClasspath()))
.build();
final JavaTemplate after = JavaTemplate
.builder("org.mockito.Mockito.never()")
.javaParser(JavaParser.fromJavaVersion().classpath(JavaParser.runtimeClasspath()))
.build();
@Override
public J visitMethodInvocation(J.MethodInvocation elem, ExecutionContext ctx) {
JavaTemplate.Matcher matcher;
if ((matcher = before.matcher(getCursor())).find()) {
maybeRemoveImport("org.mockito.Mockito.times");
return embed(
after.apply(getCursor(), elem.getCoordinates().replace()),
getCursor(),
ctx,
SHORTEN_NAMES
);
}
return super.visitMethodInvocation(elem, ctx);
}
};
return Preconditions.check(
Preconditions.and(
new UsesType<>("org.mockito.verification.VerificationMode", true),
new UsesMethod<>("org.mockito.Mockito times(..)")
),
javaVisitor
);
}
}
/**
* OpenRewrite recipe created for Refaster template {@code MockitoRules.InvocationOnMockGetArguments}.
*/
@SuppressWarnings("all")
@NonNullApi
@Generated("org.openrewrite.java.template.processor.RefasterTemplateProcessor")
public static class InvocationOnMockGetArgumentsRecipe extends Recipe {
/**
* Instantiates a new instance.
*/
public InvocationOnMockGetArgumentsRecipe() {}
@Override
public String getDisplayName() {
return "Refaster template `MockitoRules.InvocationOnMockGetArguments`";
}
@Override
public String getDescription() {
return "Recipe created for the following Refaster template:\n```java\nstatic final class InvocationOnMockGetArguments {\n \n @BeforeTemplate\n Object before(InvocationOnMock invocation, int i) {\n return invocation.getArguments()[i];\n }\n \n @AfterTemplate\n Object after(InvocationOnMock invocation, int i) {\n return invocation.getArgument(i);\n }\n}\n```\n.";
}
@Override
public TreeVisitor, ExecutionContext> getVisitor() {
JavaVisitor javaVisitor = new AbstractRefasterJavaVisitor() {
final JavaTemplate before = JavaTemplate
.builder("#{invocation:any(org.mockito.invocation.InvocationOnMock)}.getArguments()[#{i:any(int)}]")
.javaParser(JavaParser.fromJavaVersion().classpath(JavaParser.runtimeClasspath()))
.build();
final JavaTemplate after = JavaTemplate
.builder("#{invocation:any(org.mockito.invocation.InvocationOnMock)}.getArgument(#{i:any(int)})")
.javaParser(JavaParser.fromJavaVersion().classpath(JavaParser.runtimeClasspath()))
.build();
@Override
public J visitExpression(Expression elem, ExecutionContext ctx) {
JavaTemplate.Matcher matcher;
if ((matcher = before.matcher(getCursor())).find()) {
return embed(
after.apply(getCursor(), elem.getCoordinates().replace(), matcher.parameter(0), matcher.parameter(1)),
getCursor(),
ctx,
SHORTEN_NAMES
);
}
return super.visitExpression(elem, ctx);
}
};
return Preconditions.check(
Preconditions.and(
new UsesType<>("org.mockito.invocation.InvocationOnMock", true),
new UsesMethod<>("org.mockito.invocation.InvocationOnMock getArguments(..)")
),
javaVisitor
);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy